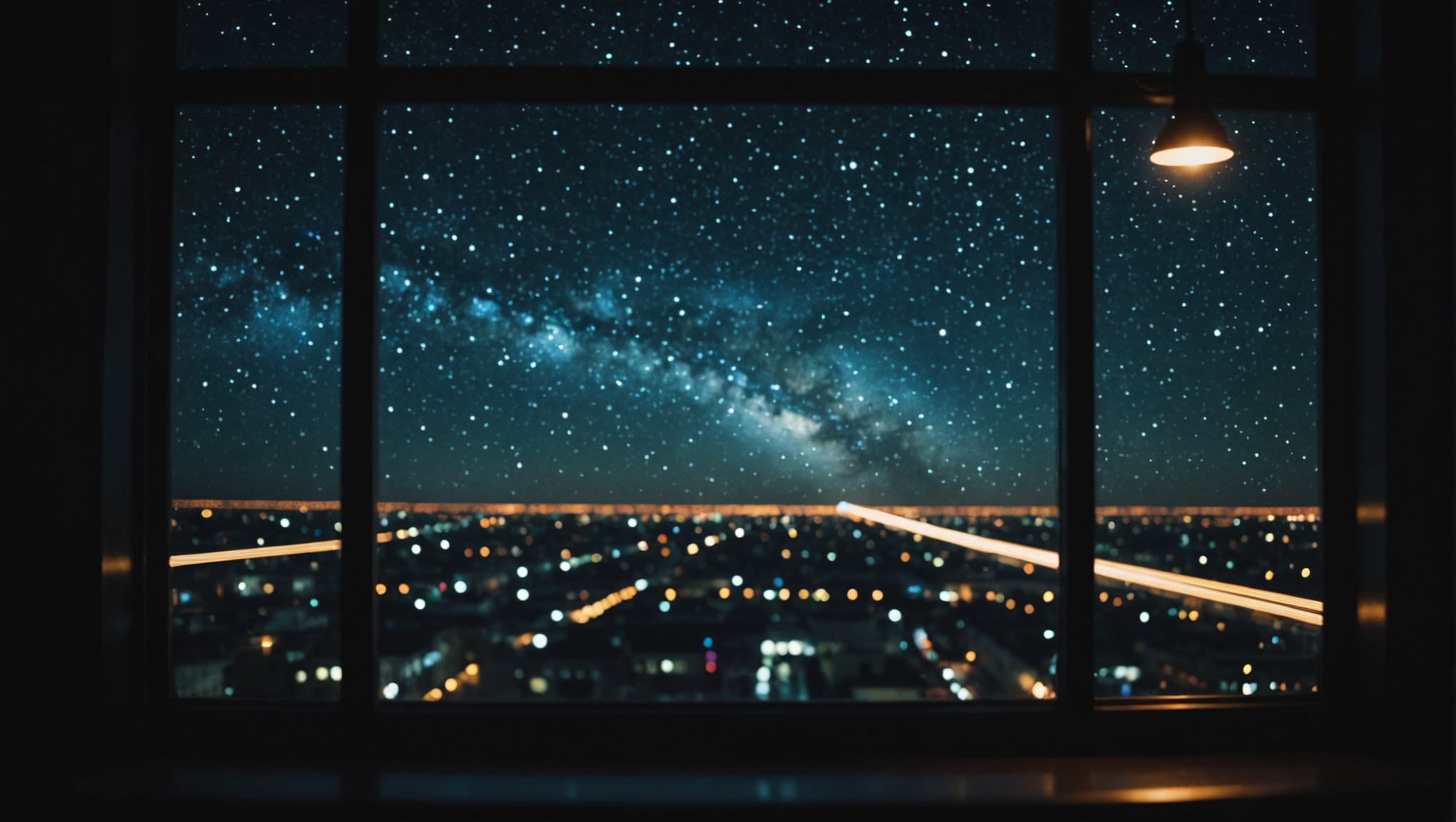
File Permissions and Bash
File permissions in Linux are fundamental to the operating system’s security model. They govern who can read, write, or execute files and directories, acting as a gatekeeper to your system’s resources. Each file and directory is associated with a specific owner, a group, and a set of permissions that dictate what actions can be performed by users.
In Linux, every file and directory has three types of permissions:
- This permission allows a user to view the contents of a file or list the contents of a directory.
- This permission enables a user to modify the contents of a file or add, delete, or rename files within a directory.
- For files, this permission allows a user to run a program or script. For directories, it grants the ability to enter and search the directory.
Permissions are assigned at three different levels:
- The owner of the file or directory.
- The group associated with the file or directory.
- All other users on the system who are not the owner or part of the group.
Permissions are displayed using a 10-character string, such as -rwxr-xr--
, where:
- The first character indicates the type of file (e.g.,
-
for regular files,d
for directories). - The next three characters represent the owner’s permissions.
- The following three characters represent the group’s permissions.
- The last three characters indicate the permissions for others.
This permission structure allows for finely tuned control over who can access and manipulate files, reflecting a balance between usability and security. Understanding these permissions very important for maintaining a secure and functional Linux environment.
To view the current permissions of files and directories, you can use the ls
command with the -l
option:
ls -l
In this output, the first column displays the permissions in the format described above, providing a clear snapshot of who can do what with each file or directory.
Types of File Permissions
File permissions in Linux are categorized into three distinct types: read, write, and execute. Each of these permissions serves a specific purpose and can be applied to files and directories in different ways. Understanding the nuances of these permissions is essential for effective file management and security.
Read (r): The read permission allows a user to access the contents of a file. For directories, having read permission means the user can list the names of the files and subdirectories contained within. Without read permission, users cannot see what files exist in a directory, nor can they open any files to view their contents. This permission is denoted by the character ‘r’ in the permission string.
# Example: Check permissions on a file ls -l myfile.txt # Output: -r--r--r-- 1 user group 0 Oct 10 12:00 myfile.txt
Write (w): Write permission allows a user to modify a file’s contents or, in the case of directories, to create, delete, or rename files within that directory. If a user does not have write permission on a file, they cannot make any changes to it, while lacking write permission on a directory prevents any alterations to its contents. This permission is represented by the character ‘w’ in the permission string.
# Example: Attempt to modify a file without write permission echo "New content" > myfile.txt # Output: bash: myfile.txt: Permission denied
Execute (x): The execute permission is somewhat unique in that it has different implications depending on whether it is applied to a file or a directory. For files, execute permission permits a user to run the file as a program or script. For directories, execute permission allows a user to enter the directory and access its contents, assuming they also have read permission. This permission is indicated by the character ‘x’ in the permission string.
# Example: Running a script with execute permission chmod +x myscript.sh ./myscript.sh
These three basic permissions—read, write, and execute—combine to form the foundation of file access control in Linux. By assigning different combinations of these permissions to the owner, group, and others, you can create complex access mechanisms tailored to your system’s needs. For example, you might want to grant full control to the owner, limit the group to read and execute only, and deny all access to others, represented as:
# Permissions example: Owner full, group read/execute, others none chmod 750 mydirectory
Understanding these types of permissions is the first step toward mastering file management in Linux, allowing users to navigate the balance between accessibility and security effectively.
Changing File Permissions with chmod
Changing file permissions in Linux is primarily accomplished through the chmod command, which stands for "change mode." This powerful tool allows users to modify the permissions of files and directories according to their needs. The flexibility of chmod is evident in its various modes of operation—both symbolic and numeric. Symbolic Mode: In symbolic mode, you specify permissions using letters and operators. The letters represent the permissions (r, w, x), while operators indicate how to adjust those permissions: - + adds a permission. - - removes a permission. - = sets the permission exactly, overwriting any existing permissions. For example, to grant the execute permission to the owner of a file named myfile.txt, you would use the following command:chmod u+x myfile.txtHere, u represents the user (owner), and +x adds the execute permission. If you wanted to remove write permission from the group, you would do:
chmod g-w myfile.txtThe symbolic approach provides an intuitive way to specify changes, especially when dealing with specific users or groups.
Numeric Mode: Alternatively, the numeric mode allows for a more compact representation of permissions using three-digit octal numbers. Each digit represents a different level of permissions for the owner, group, and others, respectively. The digits correspond to the following access levels:
- 4 for read (r)
- 2 for write (w)
- 1 for execute (x)To combine permissions, simply sum the values. For instance, if you want to grant the owner full permissions (read, write, and execute), the group read and execute permissions, and no permissions to others, you would express this as:
chmod 750 myfile.txtHere, 7 (4+2+1) represents the owner's permissions, 5 (4+1) for the group's permissions, and 0 indicates no permissions for others.
It's also important to note that you can change permissions for directories in the same manner. For example, to grant the entire group read and execute access to a directory while denying access to others, you would execute:
chmod 750 mydirectoryThe chmod command is invaluable for ensuring that files and directories have the appropriate permissions set, enhancing security and functionality within your Linux environment. Understanding how to effectively utilize both symbolic and numeric modes will empower you to manage your file permissions with precision and confidence.
Viewing File Permissions using ls
When you want to examine the permissions of files and directories in your Linux environment, the
ls
command is your first line of inquiry. By appending the-l
flag tols
, you gain access to a wealth of information, including permissions, ownership, size, and modification date. This long listing format reveals the intricate details of each file and directory in the current directory.Executing
ls -l
produces output formatted as follows:ls -l total 12 -rwxr-xr-- 1 user group 4096 Oct 10 12:00 myfile.txt drwxr-xr-x 2 user group 4096 Oct 10 12:01 mydirectoryThe first column of this output is particularly significant—it presents the permission string. Let’s break down the example:
- This permission string indicates that myfile.txt is a regular file (the leading
-
indicates a file, whiled
would indicate a directory). The subsequent characters indicate permissions in groups of three: the owner has read, write, and execute permissions (rwx
), the group has read and execute permissions (r-x
), and others have only read permission (r--
). - This permission string for mydirectory reveals that it is a directory (indicated by the leading
d
). The permissions indicate that the owner can read, write, and execute, the group can read and execute, and others can also read and execute, allowing access to the directory’s contents.
Understanding this output is critical for managing access to your files and directories. If, for instance, you notice that an important file has permissions set to rw-r—–, it means only the owner has read and write access, while the group and others are effectively locked out. This could be detrimental if the file needs to be accessed by a script running under a different user or a service account.
To quickly assess permissions across multiple files, you can simply run:
ls -l file1.txt file2.txt mydirectory
This command provides a concise view of the permissions for each file and directory listed, enabling a fast assessment of access levels.
Moreover, the ls
command supports additional flags that can enhance your visibility into file permissions. For example, adding the -h
option allows you to view file sizes in a human-readable format, making it easier to determine which files might need your attention:
ls -lh
This can be especially useful when managing directories containing a high number of files, as larger files may require different handling based on their permissions.
By mastering the output of ls -l
and its variations, you place yourself in a strong position to maintain your Linux system’s security and functionality. This knowledge allows you to quickly identify potential permission issues and respond accordingly, whether by adjusting permissions with chmod
or by reviewing ownership with the chown
command.
Understanding Special Permissions and Their Use Cases
Special permissions in Linux provide additional control over file access, beyond the standard read, write, and execute permissions. These permissions include setuid, setgid, and the sticky bit. Understanding how and when to use these special permissions can help you better manage security and functionality within your system.
Setuid (Set User ID): The setuid permission allows a user to execute a file with the permissions of the file owner rather than the permissions of the user executing the file. That’s particularly useful for programs that require elevated privileges to perform specific tasks. For example, the passwd command needs to modify the system’s password database, which is only writable by the root user. By setting the setuid bit on this command, regular users can execute it to change their passwords without needing direct root access.
chmod u+s /usr/bin/passwd
When you list the permissions of a file with the setuid bit set, you’ll see an ‘s’ in place of the owner’s execute permission:
ls -l /usr/bin/passwd -rwsr-xr-x 1 root root 54288 Oct 10 12:00 /usr/bin/passwd
In this example, the ‘s’ indicates that the setuid bit is set, allowing users to run the passwd command with root privileges.
Setgid (Set Group ID): Similar to setuid, the setgid permission grants the ability to execute a file with the permissions of the group associated with the file. When applied to a directory, setgid ensures that new files created within that directory inherit the group ownership of the directory rather than the primary group of the user creating the file. This feature is especially useful for collaborative environments where files should belong to a shared group.
chmod g+s /path/to/directory
When you list the permissions of a directory with the setgid bit set, you will see an ‘s’ in place of the group’s execute permission:
ls -ld /path/to/directory drwxr-sr-x 2 user group 4096 Oct 10 12:01 /path/to/directory
In this case, any new files created in /path/to/directory will automatically have the group set to ‘group’, fostering collaboration among users.
The Sticky Bit: The sticky bit is a special permission that can be set on directories. It restricts file deletion within the directory so that only the file’s owner, the directory’s owner, or the root user can delete or rename files. That is particularly relevant for directories like /tmp, where many users have write access. By applying the sticky bit, you prevent users from accidentally or maliciously deleting each other’s files.
chmod +t /tmp
When you check the permissions for a directory with the sticky bit set, you will see a ‘t’ in place of the others’ execute permission:
ls -ld /tmp drwxrwxrwt 10 root root 4096 Oct 10 12:01 /tmp
Understanding and appropriately using special permissions such as setuid, setgid, and the sticky bit allows you to fine-tune the access controls on your Linux system. They provide powerful mechanisms for managing user permissions while maintaining security, especially in multi-user environments. Careful application of these permissions can aid in preventing unauthorized access while allowing necessary functionality to users.