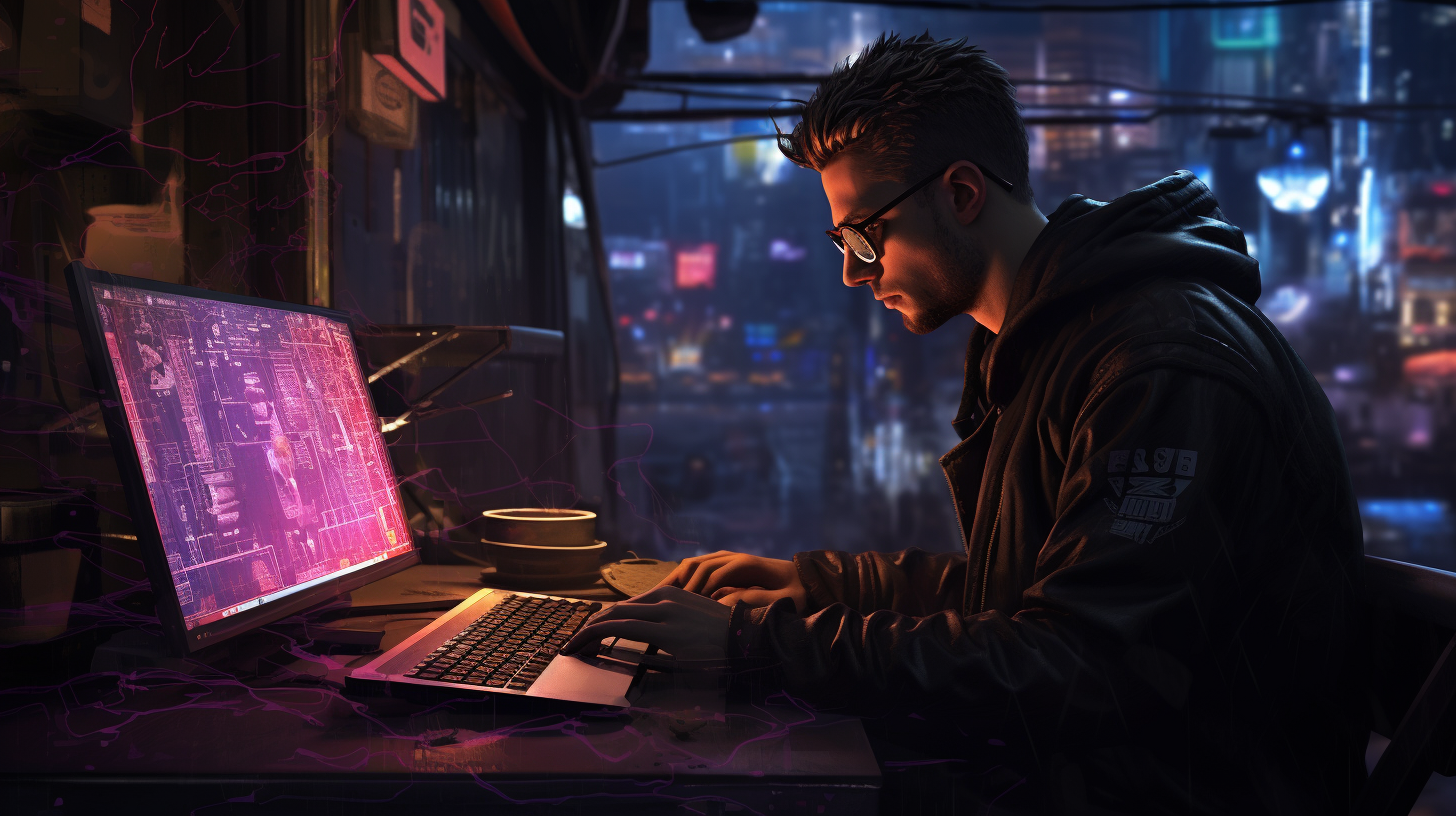
Functions in JavaScript: Basics and Beyond
In JavaScript, functions are one of the fundamental building blocks that allow developers to encapsulate code for reuse and modularity. Understanding the syntax and structure of functions is important for writing effective JavaScript. A function is declared using the function keyword, followed by a name, parentheses for parameters, and curly braces containing the code to be executed.
Here’s a basic example of a function declaration:
function greet(name) { return "Hello, " + name + "!"; }
In this example, the function greet takes a single parameter name. When invoked, it returns a greeting string. To call this function, you would do the following:
console.log(greet("Alice")); // Output: Hello, Alice!
Functions can also be defined using function expressions. Here’s how you can create a function expression and assign it to a variable:
const add = function(a, b) { return a + b; };
In this case, the function add is anonymous and is stored in the variable add. You can call it like this:
console.log(add(5, 3)); // Output: 8
Additionally, since JavaScript supports first-class functions, you can also define functions as part of an object or as arguments to other functions. Ponder this example of a method within an object:
const calculator = { multiply: function(x, y) { return x * y; } }; console.log(calculator.multiply(4, 5)); // Output: 20
JavaScript also provides a concise syntax for defining functions using arrow functions, which are especially useful for maintaining the lexical scope of this:
const square = (x) => x * x; console.log(square(6)); // Output: 36
Understanding these various ways to define functions in JavaScript enhances flexibility in your coding approach and allows for more elegant solutions to common programming tasks. This richness in function syntax and structure is what makes JavaScript a versatile language, capable of handling both simple and complex operations efficiently.
Function Scope and Closure Concepts
Function scope in JavaScript is a vital concept that dictates how and where variables can be accessed within your code. Every function in JavaScript creates a new scope, meaning that variables defined within a function are not accessible from the outside unless explicitly returned or manipulated through closures. This encapsulation helps prevent variable name collisions and keeps your code modular.
Think the following example where we define a variable inside a function:
function outerFunction() { let outerVar = 'I am outside!'; function innerFunction() { let innerVar = 'I am inside!'; console.log(outerVar); // Accessing variable from outer function } innerFunction(); console.log(innerVar); // This will cause a ReferenceError } outerFunction();
In this example, the variable outerVar
is accessible within innerFunction
because of the way scope works in JavaScript. However, innerVar
cannot be accessed from outerFunction
, demonstrating the principle of function scope.
Closures play a critical role in JavaScript by allowing functions to have access to their outer scope even after the outer function has finished executing. A closure is formed whenever a function references variables from outside its scope. This capability can be used to create private variables, which are not accessible from the global scope.
Here’s an example of a closure in action:
function createCounter() { let count = 0; // This variable is private to the counter return { increment: function() { count++; return count; }, decrement: function() { count--; return count; }, getCount: function() { return count; } }; } const counter = createCounter(); console.log(counter.increment()); // Output: 1 console.log(counter.increment()); // Output: 2 console.log(counter.getCount()); // Output: 2 console.log(counter.decrement()); // Output: 1
In this example, count
is encapsulated within createCounter
. The returned object contains methods that can modify and retrieve the private count
variable, demonstrating the power of closures in managing state.
Understanding function scope and closures not only allows you to write cleaner and more maintainable code but also empowers you to leverage JavaScript’s unique capabilities to handle complex programming tasks efficiently. By mastering these concepts, you can create functions that are robust, encapsulated, and capable of maintaining their own state, which is an essential skill in any JavaScript developer’s toolkit.
Advanced Function Techniques: Callbacks and Promises
In JavaScript, advanced function techniques such as callbacks and promises are essential for managing asynchronous operations. When executing tasks that take time, like fetching data from an API, JavaScript allows us to handle these tasks without blocking the main thread, leading to a more responsive application. Callbacks are one of the primary methods for handling asynchronous behavior.
A callback is simply a function this is passed as an argument to another function and is executed after some operation completes. That’s particularly useful in scenarios like event handling or making network requests. Let’s look at a basic example of a callback function:
function fetchData(callback) { setTimeout(() => { const data = { user: 'Alice', age: 25 }; callback(data); }, 2000); // Simulating a delay of 2 seconds } fetchData((data) => { console.log('Data received:', data); });
In this example, the fetchData
function simulates an asynchronous operation using setTimeout
. After a 2-second delay, it calls the provided callback function with the data. The callback function then logs the received data to the console. This pattern of passing functions as arguments allows for flexible and powerful handling of asynchronous events.
However, the callback approach can lead to “callback hell,” where multiple nested callbacks make the code difficult to read and maintain. That’s where promises come into play. A promise represents a value that may be available now, or in the future, or never. Promises provide a more manageable way to handle asynchronous operations.
Here’s how you can refactor the previous example to use promises:
function fetchData() { return new Promise((resolve) => { setTimeout(() => { const data = { user: 'Alice', age: 25 }; resolve(data); }, 2000); // Simulating a delay of 2 seconds }); } fetchData() .then((data) => { console.log('Data received:', data); }) .catch((error) => { console.error('Error fetching data:', error); });
In this promise-based example, fetchData
now returns a promise. When the asynchronous operation completes, the promise is resolved with the data. The then
method is used to handle the resolved value, while catch
captures any errors that may occur. This structure makes the code cleaner and easier to read.
For even more sophisticated asynchronous programming, JavaScript introduces async/await, which allows you to write asynchronous code that looks synchronous. That is particularly useful when working with multiple asynchronous operations in sequence. Here’s an example:
async function getUserData() { try { const data = await fetchData(); console.log('Data received:', data); } catch (error) { console.error('Error fetching data:', error); } } getUserData();
In this example, the getUserData
function is declared with the async
keyword, allowing the use of await
within its body. When await
is used, it pauses the execution of the function until the promise resolves, making the code flow much easier to understand. Error handling is neatly managed with try/catch
, enhancing the reliability of your asynchronous code.
Mastering these advanced function techniques—callbacks, promises, and async/await—will greatly enhance your ability to write clean, efficient, and maintainable JavaScript code. By using these concepts, you can effectively manage asynchronous operations, leading to a better user experience in your applications.
Best Practices for Writing Efficient Functions
When writing functions in JavaScript, following best practices can significantly enhance performance, readability, and maintainability of your code. Efficient functions are not just about doing things quickly; they’re about writing code that’s easy to understand and debug. Below are some key practices to consider when developing functions.
1. Keep Functions Small and Focused: Each function should have a single responsibility. This makes functions easier to read, test, and reuse. If a function is doing more than one thing, ponder breaking it down into smaller, more manageable pieces. For example:
function calculateArea(radius) { return Math.PI * radius * radius; } function calculateCircumference(radius) { return 2 * Math.PI * radius; }
Here, the functions calculateArea and calculateCircumference each perform one clear task, making them easier to understand and maintain.
2. Use Descriptive Names: Function names should be descriptive enough to convey their purpose. Avoid generic names; instead, choose names that describe what the function does:
function fetchUserData(userId) { // Fetch user data logic here }
In this case, fetchUserData clearly indicates that the function is responsible for retrieving user data based on the userId.
3. Parameter Defaults: When functions can have optional parameters, ponder using default parameter values. This improves the function’s flexibility and reduces the need for additional checks inside the function:
function greet(name = 'Guest') { return `Hello, ${name}!`; } console.log(greet()); // Output: Hello, Guest!
This allows greet to be called without any arguments while still providing a sensible default.
4. Avoid Side Effects: Functions should not produce side effects, such as modifying global variables or changing the state of objects that are passed to them. This separation of concerns makes your functions predictable and easier to debug:
function add(a, b) { return a + b; // Pure function, does not modify any external state }
By adhering to this principle, your functions become more reliable and reusable in different contexts.
5. Leverage Functional Programming: JavaScript’s support for first-class functions makes it a good candidate for functional programming paradigms. Embrace immutability, use array methods like map and reduce, and avoid changing objects directly:
const numbers = [1, 2, 3, 4, 5]; const squares = numbers.map(num => num * num); console.log(squares); // Output: [1, 4, 9, 16, 25]
This approach minimizes the chances of bugs and makes your code cleaner and easier to follow.
6. Optimize Performance: While premature optimization is discouraged, being aware of performance can help in specific scenarios. For instance, if a function is called frequently, consider caching results or using techniques like debouncing or throttling for optimizing performance:
function debounce(func, delay) { let timeout; return function(...args) { clearTimeout(timeout); timeout = setTimeout(() => func.apply(this, args), delay); }; } const saveInput = debounce(() => console.log('Input saved!'), 1000);
This debounce function ensures that the saveInput function is not called excessively, allowing it to execute only after a specified delay, improving performance in scenarios like handling user input events.
By adhering to these best practices, developers can write efficient JavaScript functions that are not only performant but also maintainable and easy to understand. As you continue to refine your coding style and practices, you will find that these techniques will serve as a strong foundation for building robust applications.