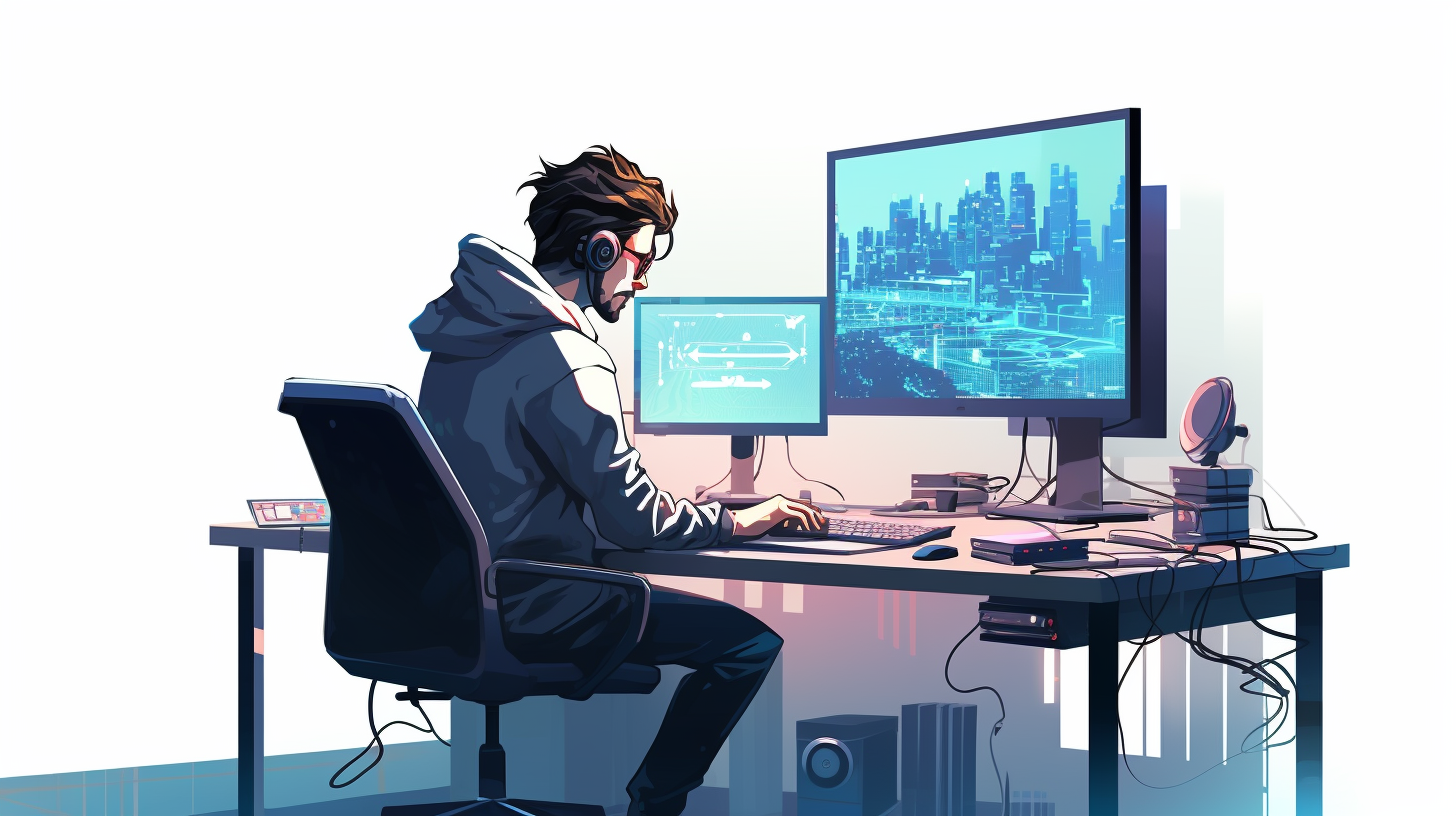
Garbage Collection in Java: How It Works
In Java, memory management is largely handled by the garbage collector, an automatic process designed to reclaim memory taken up by objects that are no longer needed by a program. This process operates in the background, ensuring that developers can focus on writing code without having to manually manage memory allocation and deallocation. Understanding how garbage collection works especially important for building efficient Java applications.
At its core, garbage collection involves identifying and disposing of objects that are unreachable, meaning they can no longer be accessed by the application. The Java Virtual Machine (JVM) uses various algorithms to determine which objects are eligible for garbage collection, and it does this in a way that minimizes the impact on application performance.
Java employs a generational garbage collection strategy, which is based on the observation that most objects are short-lived. This strategy divides the heap memory into different generations:
Young Generation: - Newly created objects are allocated here. - Comprises Eden Space and Survivor Spaces. Old Generation: - Holds long-lived objects that have survived multiple garbage collection cycles. Permanent Generation (or Metaspace in newer versions): - Stores metadata about classes and methods.
The Young Generation is where the majority of objects are created. When this space fills up, a minor garbage collection occurs, which typically runs quickly and collects only the garbage from this area. Objects that survive this process are moved to the Old Generation, where they’re less frequently collected.
The Old Generation is managed differently, as it usually contains objects that have longer lifetimes. Major garbage collection events are called when this area fills up, and these are generally more time-consuming than minor collections.
One of the key components of garbage collection is the idea of reachability. An object is considered reachable if it can be accessed through a chain of references from a set of root objects, known as the root set. The root set typically includes:
- Active threads - Static fields - Local variables in stack frames
When a garbage collection cycle starts, the garbage collector will traverse this root set to identify all reachable objects. Any object that cannot be reached from the root set is marked for collection. This approach ensures that only unused objects are cleaned up, thus optimizing memory usage.
Garbage collection in Java is a sophisticated mechanism that helps developers manage memory efficiently. By understanding its operations, including the nuances of object reachability and the generational memory model, developers can write better-performing applications while alleviating the burden of manual memory management.
Types of Garbage Collectors
Java provides several types of garbage collectors, each designed to address different performance needs and application requirements. The choice of garbage collector can have a significant impact on application throughput, latency, and overall responsiveness. Here, we delve into the main types of garbage collectors available in Java, along with their characteristics.
Serial Garbage Collector
The Serial Garbage Collector is the simplest form of garbage collection available in Java. It employs a single thread for both application execution and garbage collection, making it suitable for small applications with manageable memory footprints. The Serial GC is often used in environments with limited resources or in applications where simplicity is more critical than performance.
java -XX:+UseSerialGC -jar YourApplication.jar
Parallel Garbage Collector
Also known as the throughput collector, the Parallel Garbage Collector improves upon the Serial GC by using multiple threads for managing heap memory. This collector is designed for high throughput, making it perfect for batch processing or applications that require significant processing power. It minimizes pause times during garbage collection by executing multiple garbage collection tasks at the same time.
java -XX:+UseParallelGC -jar YourApplication.jar
Concurrent Mark-Sweep (CMS) Collector
The Concurrent Mark-Sweep (CMS) Collector is designed to minimize pause times during garbage collection, making it suitable for applications with low-latency requirements. CMS performs most of its work concurrently with the application threads, which allows for shorter pause times. However, it can fall prey to fragmentation issues over time, necessitating occasional Full GCs to compact the heap.
java -XX:+UseConcMarkSweepGC -jar YourApplication.jar
G1 Garbage Collector
The G1 Garbage Collector is a more recent addition to the Java garbage collection arsenal. G1 divides the heap into regions and collects them in a way that aims to achieve both high throughput and low pause times. It is particularly well-suited for applications with large heap sizes, as it can perform garbage collection in a predictable manner, controlling pause times effectively.
java -XX:+UseG1GC -jar YourApplication.jar
Z Garbage Collector (ZGC)
Introduced in JDK 11, the Z Garbage Collector is designed for applications requiring extremely low pause times. ZGC achieves this goal by using a technique called “load barriers” to perform garbage collection simultaneously with application threads. It can handle very large heaps (multi-terabyte) and is aimed at providing a scalable solution for modern applications.
java -XX:+UseZGC -jar YourApplication.jar
Shenandoah Garbage Collector
Similar to ZGC, the Shenandoah Garbage Collector also focuses on reducing pause times. It works by performing most of its garbage collection work at the same time, allowing application threads to run with minimal interruptions. Shenandoah is designed to provide consistent low-latency performance even as the heap size increases.
java -XX:+UseShenandoahGC -jar YourApplication.jar
Choosing the right garbage collector involves understanding the specific needs of your application, including memory usage patterns and performance requirements. By using the appropriate garbage collector, developers can optimize their Java applications effectively, ensuring that they run smoothly and efficiently without undue memory management overhead.
How Garbage Collection Works
When a garbage collection cycle is initiated in Java, the process involves several distinct phases to ensure that memory is reclaimed efficiently. Understanding how these phases work especially important for optimizing application performance and minimizing pause times. The typical garbage collection process can be broken down into the following stages:
1. Mark Phase: The first step in the garbage collection process is marking all reachable objects. This involves traversing the object graph starting from the root set and marking each object that can be accessed. The garbage collector uses various data structures, such as a stack or a queue, to keep track of the objects that need to be marked. The marking process can be done using a depth-first or breadth-first traversal, depending on the collector’s algorithm.
public class GarbageCollectionExample { public static class Node { Node reference; } public static void mark(Node node) { if (node == null) return; // Simulate marking the node System.out.println("Marking node: " + node); mark(node.reference); // Recursively mark reachable objects } }
2. Sweep Phase: After marking is complete, the garbage collector enters the sweep phase. In this phase, the collector traverses the heap and deallocates memory for objects that were not marked. This step is critical for reclaiming memory and ensuring that it can be reused for new objects. Depending on the specific garbage collector algorithm, the sweeping can be done in a single pass or involve multiple passes for different regions of the heap.
public static void sweep(Object[] heap) { for (Object obj : heap) { if (!isMarked(obj)) { // Simulate deallocation System.out.println("Deallocating: " + obj); } } }
3. Compact Phase (Optional): Some garbage collectors include an optional compact phase to reduce fragmentation in the heap. This phase involves moving the remaining marked objects together to create larger contiguous blocks of free memory. Compaction can lead to improved performance as it reduces the time required to allocate new objects. However, this phase can introduce additional latency, so it isn’t always employed.
public static void compact(Object[] heap) { int freeIndex = 0; for (int i = 0; i < heap.length; i++) { if (isMarked(heap[i])) { heap[freeIndex++] = heap[i]; // Move marked object to the front } } // Fill the rest with null, simulating deallocation for (int i = freeIndex; i < heap.length; i++) { heap[i] = null; } }
As the garbage collector performs these phases, it also must be aware of concurrent applications. In the case of concurrent garbage collectors like CMS or G1, certain phases such as marking can occur while the application threads continue to execute. This concurrent execution aims to minimize pause times, allowing for more responsive applications. However, care must be taken to ensure that the marking and sweeping processes remain consistent, avoiding scenarios where the application modifies objects while they are being collected.
Garbage collection in Java is more than just reclaiming memory; it involves optimizing the memory lifecycle to ensure that applications perform effectively under varying loads. By grasping the intricacies of how garbage collection operates, developers can proactively manage memory usage patterns and anticipate potential challenges that arise during application execution.
Tuning Garbage Collection Performance
Tuning garbage collection performance in Java is an essential practice for optimizing application efficiency, particularly in environments where performance is critical. The JVM offers a variety of parameters that developers can tweak to influence how garbage collection is performed. Understanding these tuning options allows developers to tailor the garbage collection process to better suit their application’s needs.
One of the first steps in tuning garbage collection performance is to monitor existing behavior. JVM provides several options for logging garbage collection activity, which can be invaluable in identifying bottlenecks or inefficiencies. You can enable garbage collection logging by adding the following flags when starting the JVM:
java -Xlog:gc*:file=gc.log -jar YourApplication.jar
This command will create a `gc.log` file containing detailed information about garbage collection events, including frequency, duration, and the amount of memory reclaimed. Analyzing this log can help you make informed decisions about tuning.
Once you have a grasp of how garbage collection is performing, you can start tuning the parameters related to the different garbage collectors. Each collector may have specific flags that can be adjusted to enhance performance:
- Adjusting the heap size can have a substantial impact on garbage collection performance. You can specify the minimum and maximum heap sizes using:
-Xms512m -Xmx4g
-Xmn2g
-XX:MaxGCPauseMillis=200
Moreover, understanding the application’s memory allocation patterns can provide key insights into tuning. If your application allocates many short-lived objects, ensuring that the Young Generation is adequately sized and that you’re using a collector optimized for such allocations can lead to significant performance improvements. Conversely, for applications with long-lived objects, tuning parameters for the Old Generation can help manage the frequency of major garbage collections.
It’s important to test each change in a controlled environment to measure its impact on performance. The right balance of parameters will vary significantly based on the specifics of the application and the workload it handles. Additionally, ensure that you are using the latest version of the JDK, as performance improvements and new features are continually added to the garbage collectors.
Tuning garbage collection performance in Java is not a one-size-fits-all approach; it requires a detailed understanding of both the application’s behavior and the underlying garbage collection mechanisms. By carefully monitoring, adjusting parameters, and testing configurations, developers can significantly enhance application performance and responsiveness in memory management.
Common Garbage Collection Issues and Best Practices
Common Garbage Collection issues can significantly impact the performance of Java applications if not addressed properly. Developers must be aware of potential pitfalls associated with garbage collection and adopt best practices to mitigate these issues. Here are some of the most prevalent garbage collection challenges along with strategies to tackle them.
1. Long Garbage Collection Pause Times: One of the most significant impacts of garbage collection is the pause time experienced by applications during collection cycles. Long pauses can lead to noticeable latency, particularly in latency-sensitive applications. To address this, think the following:
java -XX:MaxGCPauseMillis=200 -XX:+UseG1GC -jar YourApplication.jar
This command sets a target for maximum garbage collection pause time while employing the G1 collector, which is designed for low-latency applications.
2. Frequent Full GCs: Full garbage collections can be particularly detrimental due to their longer duration compared to minor collections. Frequent full GCs may indicate that the heap size is too small or that the application is creating too many long-lived objects. To address this issue:
java -Xms2g -Xmx8g -jar YourApplication.jar
Here, you’re setting a minimum and maximum heap size to ensure the application has sufficient memory to operate without frequent full GCs.
3. Fragmentation: Memory fragmentation can occur over time, especially with collectors like CMS, which do not compact memory by default. Fragmentation can lead to inefficient memory use and increased allocation times. To deal with fragmentation:
Think using the G1 collector, which handles memory in a more adaptive manner, or employ the following command to trigger a full compaction:
java -XX:+UseConcMarkSweepGC -XX:+UseCMSCompactAtFullCollection -jar YourApplication.jar
4. Object Lifetimes and Allocation Patterns: Understanding your application’s object allocation patterns is vital. If your application generates many short-lived objects, the young generation should be adequately sized. Conversely, if objects tend to have longer lifetimes, ensure that the old generation is appropriately configured. Monitor and adjust the size dynamically based on profiling results:
java -Xmn512m -jar YourApplication.jar
5. Monitoring and Analysis: Regularly monitoring garbage collection logs can reveal underlying issues in memory management. Utilize tools such as JVisualVM or Java Mission Control to gain insights into your application’s memory usage trends and garbage collection behavior. Log garbage collection statistics for analysis:
java -Xlog:gc*:file=gc.log -jar YourApplication.jar
6. Tuning JVM Parameters: The JVM provides numerous flags that can be adjusted to improve garbage collection performance. It is essential to experiment with these parameters and profile your application under various loads. Some common flags are:
- NewRatio: Controls the size of the young generation to old generation ratio.
- SurvivorRatio: Adjusts the ratio of Eden Space to Survivor Space.
- +PrintGCDetails: Provides detailed information about each garbage collection event.
By understanding these common garbage collection issues and implementing best practices, Java developers can improve application performance, reduce latency, and enhance overall responsiveness. Continuous monitoring and tuning based on application behavior are key to achieving efficient memory management in Java applications.