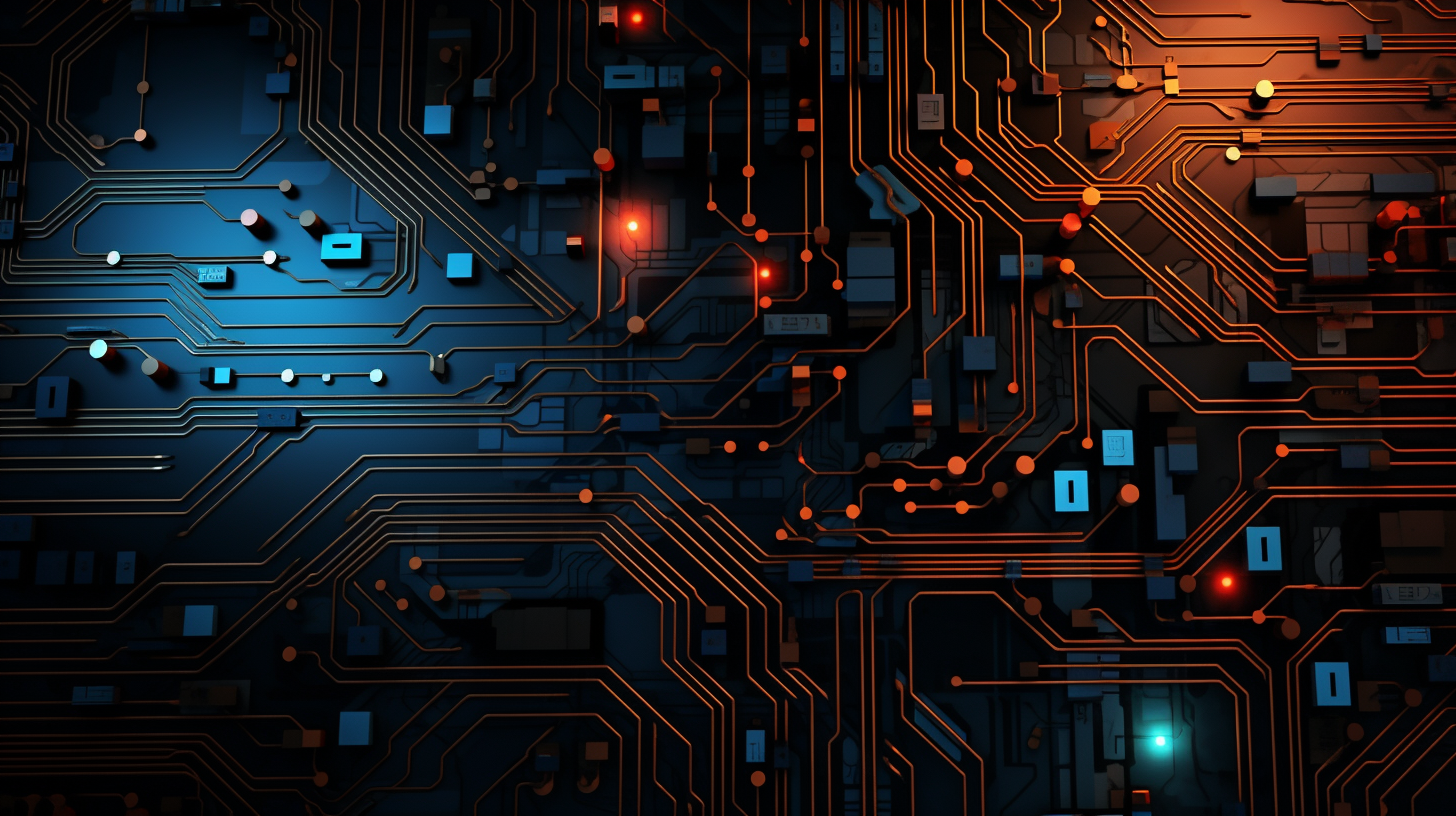
Gesture Recognition in Swift
In UIKit, gesture recognizers play a pivotal role in enhancing user interactivity by detecting and responding to touch gestures. They act as intermediaries between the user’s touch interactions and the underlying views, allowing developers to focus on the logic of their applications rather than the minutiae of touch event handling. At a high level, gesture recognizers are subclasses of UIGestureRecognizer
, and they encapsulate various types of gestures, such as taps, swipes, pinches, and rotations.
When a user interacts with the screen—whether by tapping, dragging, or performing more complex gestures—the gesture recognizers translate these actions into recognizable events. That is achieved through a combination of touch event tracking and state management. Each gesture recognizer keeps track of its own state, which can be one of several defined states: possible, began, changed, ended, cancelled, or failed. This state management very important for ensuring that gestures are recognized accurately and are contextually relevant to the current user interaction.
To utilize gesture recognizers in an iOS application, developers generally follow a simpler process:
let tapGestureRecognizer = UITapGestureRecognizer(target: self, action: #selector(handleTap)) view.addGestureRecognizer(tapGestureRecognizer) @objc func handleTap(gesture: UITapGestureRecognizer) { if gesture.state == .ended { // Handle the tap event } }
In the code above, we create an instance of UITapGestureRecognizer
and associate it with a target action, in this case, handleTap
. We then add the gesture recognizer to the view to begin listening for tap events. Inside the handler, we check the state of the gesture recognizer to ensure we respond only when the gesture has ended, preventing unnecessary logic from executing during the gesture’s lifecycle.
Gesture recognizers can also be combined. For instance, a view can recognize both tap and long-press gestures at the same time. That is accomplished by adding multiple gesture recognizers to the same view and configuring their properties to work in harmony.
Understanding how gesture recognizers work within UIKit allows developers to create more interactive and responsive applications. By using the built-in capabilities of gesture recognizers, you can enhance the user experience while maintaining clean and maintainable code.
Types of Gesture Recognizers
In UIKit, there are several types of gesture recognizers, each designed to detect specific interactions. Understanding these types is essential for creating a rich user experience, as each gesture recognizer serves a distinct purpose and has its own properties and methods to customize its behavior. Here’s a breakdown of the most commonly used gesture recognizers:
1. UITapGestureRecognizer: This recognizes one or more taps on a view. It’s often used for actions like selecting items or triggering animations. You can configure it to recognize double-taps or multiple taps.
let tapGestureRecognizer = UITapGestureRecognizer(target: self, action: #selector(handleTap)) tapGestureRecognizer.numberOfTapsRequired = 2 // Recognizes double taps view.addGestureRecognizer(tapGestureRecognizer)
2. UIPinchGestureRecognizer: That’s used to recognize pinch gestures, typically for zooming in and out on images or maps. You can access the scale factor to determine how much the user has zoomed in or out.
let pinchGestureRecognizer = UIPinchGestureRecognizer(target: self, action: #selector(handlePinch)) view.addGestureRecognizer(pinchGestureRecognizer) @objc func handlePinch(gesture: UIPinchGestureRecognizer) { if gesture.state == .changed { let scale = gesture.scale // Apply zoom based on scale } }
3. UIRotationGestureRecognizer: This recognizes rotation gestures, which are useful for rotating objects on the screen. It provides the angle of rotation, allowing for intuitive manipulation of graphical elements.
let rotationGestureRecognizer = UIRotationGestureRecognizer(target: self, action: #selector(handleRotation)) view.addGestureRecognizer(rotationGestureRecognizer) @objc func handleRotation(gesture: UIRotationGestureRecognizer) { if gesture.state == .changed { let rotation = gesture.rotation // Apply rotation based on angle } }
4. UIPanGestureRecognizer: This recognizes dragging gestures, allowing users to move objects across the screen. It tracks the movement of the touch and provides the translation of the gesture.
let panGestureRecognizer = UIPanGestureRecognizer(target: self, action: #selector(handlePan)) view.addGestureRecognizer(panGestureRecognizer) @objc func handlePan(gesture: UIPanGestureRecognizer) { if gesture.state == .changed { let translation = gesture.translation(in: view) // Move the object based on translation } }
5. UILongPressGestureRecognizer: This recognizes long press gestures, which can be useful for triggering context menus or additional actions. You can specify the minimum duration required for the gesture to be recognized.
let longPressGestureRecognizer = UILongPressGestureRecognizer(target: self, action: #selector(handleLongPress)) longPressGestureRecognizer.minimumPressDuration = 0.5 // 0.5 seconds view.addGestureRecognizer(longPressGestureRecognizer) @objc func handleLongPress(gesture: UILongPressGestureRecognizer) { if gesture.state == .began { // Trigger long press action } }
Each of these gesture recognizers can be customized further with properties such as cancelsTouchesInView and numberOfTouchesRequired, enabling you to refine their behavior. By combining multiple gesture recognizers on a single view, as mentioned before, you can create complex interactions while maintaining clarity in your code.
By understanding and effectively using these different types of gesture recognizers, you can significantly enhance the interactivity of your iOS applications, making them more engaging and responsive to user input.
Implementing Gesture Recognizers in Your App
Implementing gesture recognizers in your app is a simpler and rewarding process that empowers developers to craft intuitive user interfaces. The key to success lies in understanding both the lifecycle of gesture recognizers and how to manage their interactions within your views. Below, we’ll delve into the step-by-step implementation of gesture recognizers, illustrated with practical Swift code examples.
To begin with, you need to choose the appropriate gesture recognizer(s) based on the interactions you want to support in your app. For instance, if you want users to tap on an image to trigger an action, you would use a UITapGestureRecognizer
. Once you’ve decided on the gesture, you can instantiate it and add it to the relevant view.
let tapGestureRecognizer = UITapGestureRecognizer(target: self, action: #selector(handleTap)) view.addGestureRecognizer(tapGestureRecognizer) @objc func handleTap(gesture: UITapGestureRecognizer) { if gesture.state == .ended { // Handle the tap event } }
This code snippet creates a tap gesture recognizer and attaches it to a view. The handleTap
method is called when the user taps on the view. Notice how we check the gesture’s state to ensure that we only respond when the gesture has fully completed.
In cases where your app requires more complex gestures, such as pinch-to-zoom or rotation, you would similarly instantiate the corresponding gesture recognizers and define their handling methods. Here’s how you’d implement a pinch gesture recognizer:
let pinchGestureRecognizer = UIPinchGestureRecognizer(target: self, action: #selector(handlePinch)) view.addGestureRecognizer(pinchGestureRecognizer) @objc func handlePinch(gesture: UIPinchGestureRecognizer) { if gesture.state == .changed { let scale = gesture.scale // Adjust the view's transform based on the pinch scale view.transform = view.transform.scaledBy(x: scale, y: scale) gesture.scale = 1.0 // Reset scale to 1.0 for cumulative scaling } }
In this example, the pinch gesture recognizer captures the scale factor as the user pinches in or out. The transform of the view is adjusted accordingly. Importantly, the gesture.scale
is reset to 1.0 after applying the transformation. This prevents the scaling effect from compounding on itself with each subsequent change.
It’s crucial to note that gesture recognizers can interfere with one another if not managed properly. For instance, if a tap gesture recognizer and a pan gesture recognizer are both added to the same view, they may compete for touch interactions. To resolve such conflicts, you can implement the delegate method gestureRecognizer(_:shouldRecognizeSimultaneouslyWith:)
of the UIGestureRecognizerDelegate
protocol:
class YourViewController: UIViewController, UIGestureRecognizerDelegate { override func viewDidLoad() { super.viewDidLoad() let tapGestureRecognizer = UITapGestureRecognizer(target: self, action: #selector(handleTap)) let panGestureRecognizer = UIPanGestureRecognizer(target: self, action: #selector(handlePan)) tapGestureRecognizer.delegate = self panGestureRecognizer.delegate = self view.addGestureRecognizer(tapGestureRecognizer) view.addGestureRecognizer(panGestureRecognizer) } func gestureRecognizer(_ gestureRecognizer: UIGestureRecognizer, shouldRecognizeSimultaneouslyWith otherGestureRecognizer: UIGestureRecognizer) -> Bool { return true // Allow simultaneous recognition } }
By implementing the delegate method, you enable both gesture recognizers to detect their respective gestures simultaneously. This provides a smoother user experience, especially in interfaces that require multi-touch capabilities.
Implementing gesture recognizers in Swift is about using these tools to make your application’s interface feel natural and responsive. The proper setup and management of gestures can significantly enhance user engagement, turning simple interactions into meaningful experiences. As you incorporate these elements into your iOS applications, remember to continuously test and iterate on their behavior to achieve the best user experience possible.
Handling Gesture Recognition States
When handling gesture recognition states, it’s essential to understand the lifecycle of a gesture recognizer. Each gesture recognizer undergoes several states as the user interacts with the screen. The states are: possible, began, changed, ended, cancelled, and failed. This state management allows developers to respond appropriately to user interactions at each phase of the gesture.
Here’s a detailed look at each state:
- That’s the initial state, indicating that the recognizer is waiting for a gesture to begin. If a gesture is recognized, the state transitions to began.
- This state indicates that the gesture has started. Actions associated with the gesture should be initiated here.
- As the gesture continues, this state represents ongoing changes. That’s where you typically update the UI based on user interactions.
- This state shows that the gesture has completed successfully, allowing you to finalize any actions and potentially trigger animations or transitions.
- This state occurs when the gesture is interrupted (e.g., a phone call or the user’s finger leaves the screen). You should handle clean-up actions here.
- This state indicates that the gesture could not be recognized. You can use this state to reset any UI elements or state variables.
To demonstrate how to handle these states effectively, consider the following Swift code example, which demonstrates the implementation of a UIPanGestureRecognizer:
let panGestureRecognizer = UIPanGestureRecognizer(target: self, action: #selector(handlePan)) view.addGestureRecognizer(panGestureRecognizer) @objc func handlePan(gesture: UIPanGestureRecognizer) { switch gesture.state { case .began: // Perform actions at the start of the gesture print("Gesture began") case .changed: let translation = gesture.translation(in: view) // Update the position of the view based on the translation view.center = CGPoint(x: view.center.x + translation.x, y: view.center.y + translation.y) gesture.setTranslation(.zero, in: view) // Reset translation case .ended: // Finalize any actions after the gesture has ended print("Gesture ended") case .cancelled: // Handle cleanup print("Gesture cancelled") case .failed: // Reset any UI elements or state variables print("Gesture failed") default: break } }
In this code, we set up a pan gesture recognizer and implement a switch statement to handle various states. When the gesture begins, you might want to prepare the UI; during the “changed” phase, the view’s position is updated based on the user’s finger movement. When the gesture ends, you can finalize actions, while “cancelled” and “failed” states allow for clean-up or resetting any temporary state.
It’s important to remember that each gesture recognizer’s state transitions are triggered by user interactions, so it especially important to handle these states efficiently. By managing gesture states accurately, you can create responsive and intuitive user interfaces that enhance the overall experience of your iOS app.
Best Practices for Gesture Recognition in Swift
When working with gesture recognizers in Swift, following best practices can significantly enhance user experience and maintain the efficiency of your application. Here are several key considerations to keep in mind when implementing gesture recognition:
1. Use Specific Gesture Recognizers: Choose the most appropriate gesture recognizer for the interaction you intend to capture. For instance, use UITapGestureRecognizer
for taps, UIPinchGestureRecognizer
for pinch actions, and UIPanGestureRecognizer
for dragging. Avoid using general-purpose recognizers when specific ones can provide clearer code and better user interactions.
2. Manage Gesture Recognizers Wisely: When adding multiple gesture recognizers to a single view, ensure they can coexist without conflicts. Implement the gestureRecognizer(_:shouldRecognizeSimultaneouslyWith:)
method of the UIGestureRecognizerDelegate
protocol to allow gestures to be recognized at once when appropriate. For example:
class YourViewController: UIViewController, UIGestureRecognizerDelegate { override func viewDidLoad() { super.viewDidLoad() let tapGesture = UITapGestureRecognizer(target: self, action: #selector(handleTap)) let panGesture = UIPanGestureRecognizer(target: self, action: #selector(handlePan)) tapGesture.delegate = self panGesture.delegate = self view.addGestureRecognizer(tapGesture) view.addGestureRecognizer(panGesture) } func gestureRecognizer(_ gestureRecognizer: UIGestureRecognizer, shouldRecognizeSimultaneouslyWith otherGestureRecognizer: UIGestureRecognizer) -> Bool { return true } }
3. Monitor Gesture States: Always pay attention to the different states of a gesture recognizer. Manage your UI updates based on the state transitions (began, changed, ended, cancelled, failed) to create a smooth interaction experience. This helps to ensure that actions are only taken when appropriate, which can avoid user confusion or unintended behaviors.
@objc func handlePan(gesture: UIPanGestureRecognizer) { switch gesture.state { case .began: // Initialize actions at the start of the pan case .changed: // Update the UI as the pan changes case .ended: // Finalize the action case .cancelled, .failed: // Clean up if necessary default: break } }
4. Set Proper Gesture Requirements: Utilize properties like numberOfTouchesRequired
or minimumPressDuration
to fine-tune how gestures are recognized. For example, if you only want a tap to register with two fingers, you can set this property on your tap gesture recognizer:
let tapGesture = UITapGestureRecognizer(target: self, action: #selector(handleTap)) tapGesture.numberOfTouchesRequired = 2 // Requires two fingers for the tap view.addGestureRecognizer(tapGesture)
5. Prevent Gesture Conflicts: Be cautious of gesture recognizers that might conflict with each other, especially on the same view. If two gestures might interfere, think using the require(toFail:)
method to specify that one gesture should only recognize after another has failed. This can help you manage complex interactions effectively.
tapGesture.require(toFail: panGesture)
6. Optimize Performance: For views with heavy content or complex layouts, keep gesture recognizer overhead in mind. Avoid adding unnecessary gesture recognizers to views that do not need them. Instead, abstract gesture handling into dedicated manager classes when working with multiple views or complex interactions. This can help you keep your code organized and responsive.
7. Test Extensively: Testing your gesture interactions on different devices especially important. User expectations can vary across platforms and screen sizes, so ensure that gestures feel natural across all scenarios. Consider how different gestures may behave in the context of various user interactions.
By adhering to these best practices, you can create a fluid and intuitive user experience that capitalizes on the capabilities of gesture recognizers in UIKit. The goal is to ensure that gestures feel seamless and enhance the overall functionality of your applications.