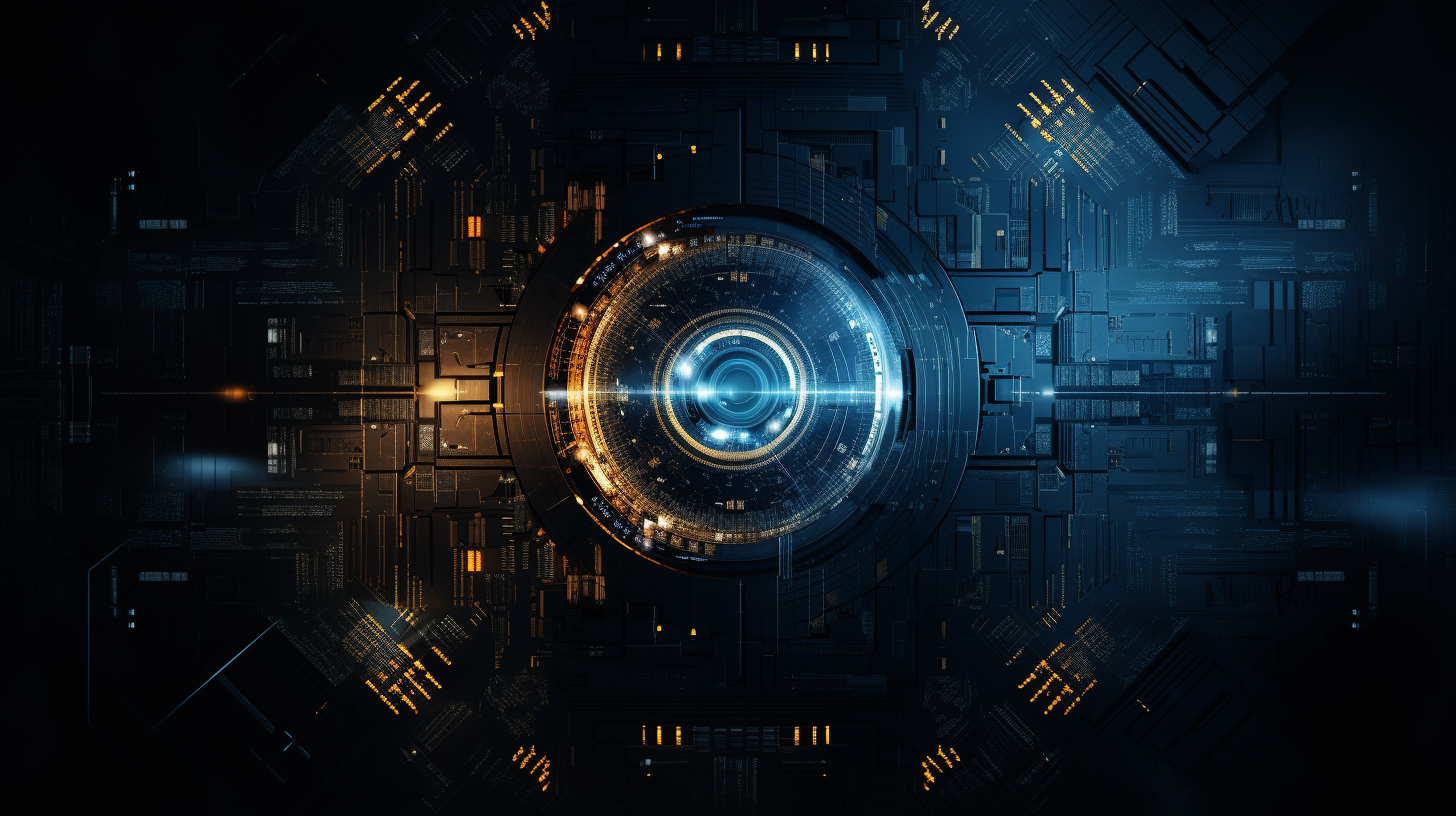
Getting Started with Python Programming
Python is a high-level, interpreted programming language known for its readability and simplicity. Developed by Guido van Rossum and released in 1991, Python emphasizes code clarity and enables developers to express concepts in fewer lines of code than languages like C++ or Java. This feature makes Python particularly appealing for both beginners and skilled software developers.
One of the key strengths of Python is its extensive standard library, which provides a wide range of functionalities that can be used without the need to install additional packages. This library includes modules for handling everything from basic file operations to complex data analysis and web development.
Python’s syntax is designed to be intuitive and simpler. For example, defining a function in Python is done using the def keyword, followed by the function name and its parameters:
def greet(name): print(f"Hello, {name}!")
In the example above, the function greet
takes a single parameter, name
, and prints a greeting message. The use of formatted strings (f-strings) simplifies the process of building strings with dynamic content.
Python supports multiple programming paradigms, including procedural, object-oriented, and functional programming. This flexibility allows developers to choose the best approach for their specific tasks. For example, you can create classes and objects to encapsulate data and behavior, making your code more modular and reusable:
class Dog: def __init__(self, name): self.name = name def bark(self): print(f"{self.name} says woof!")
In this snippet, we define a Dog
class with an initializer method and a method to make the dog bark. Such object-oriented features enable the encapsulation of functionality, which can lead to better organized and maintained code.
The Python community is another significant aspect of its ecosystem. With a wealth of resources, libraries, and frameworks, you can find solutions to many challenges you may encounter while programming. Popular libraries such as NumPy for numerical computation, Pandas for data manipulation, and Flask for web development illustrate Python’s versatility and wide-ranging applicability.
Setting Up Your Python Environment
Setting up your Python environment is an important first step towards productive programming. Whether you are on Windows, macOS, or Linux, the process involves several key components: installing Python, choosing an Integrated Development Environment (IDE), and managing packages effectively. Let’s walk through these steps to get your Python environment up and running.
Installing Python
The first thing you need is the Python interpreter. The official Python website offers the latest version of Python for all major operating systems. Visit python.org/downloads to download the installer suitable for your OS. During installation, make sure to check the box that says “Add Python to PATH”—this allows you to run Python from the command line.
After installation, you can verify that Python is installed correctly by opening a terminal or command prompt and typing:
python --version
This command should return the version of Python that you have installed.
Choosing an IDE
While you can write Python code in any text editor, an IDE can significantly enhance your development experience. Popular choices include:
- A powerful IDE that offers intelligent code assistance and a rich set of features tailored for Python development.
- A lightweight, extensible code editor with great support for Python through extensions.
- Ideal for data science and interactive computing, which will allow you to run Python code in a web-based environment.
After you have selected an IDE, follow its setup instructions to configure it for Python development. For instance, if you choose Visual Studio Code, you should install the Python extension for enhanced functionality, including syntax highlighting and debugging support.
Managing Packages
Python’s power is augmented by its vast ecosystem of third-party packages. The most common tool for managing these packages is pip, which comes pre-installed with Python. You can use pip to install new packages from the command line. For example, to install the popular requests library, simply run:
pip install requests
To keep your project’s dependencies organized, ponder using a virtual environment. A virtual environment allows you to create isolated spaces for your projects, ensuring that packages do not conflict with each other. You can create a virtual environment using the following commands:
# Create a new virtual environment python -m venv myenv # Activate the virtual environment # On Windows myenvScriptsactivate # On macOS/Linux source myenv/bin/activate
Once activated, you can use pip to install packages within this isolated environment, which keeps your global site-packages clean and reduces dependency conflicts.
Basic Syntax and Data Types
The basic syntax of Python is designed to be clean and easy to understand, which allows developers to focus on solving problems rather than getting bogged down by complex syntax rules. One of the first things you will notice is that Python uses indentation to define blocks of code rather than braces or keywords, which contributes to its readability.
To illustrate this concept, ponder a simple example of a conditional statement. In Python, an if statement might look like this:
age = 18 if age >= 18: print("You are an adult.") else: print("You are a minor.")
In this example, the indentation indicates which code belongs to the if and else blocks. This structure not only makes the code more readable but also enforces a consistent style across Python codebases.
Python supports a variety of data types, which can be broadly categorized into built-in types like integers, floats, strings, and booleans, as well as more complex types like lists, tuples, sets, and dictionaries. Understanding how to work with these data types is essential for effective programming.
For instance, integers and floats represent numeric values, where integers are whole numbers and floats can contain decimal points:
integer_value = 42 float_value = 3.14
Strings are sequences of characters, enclosed in either single or double quotes:
greeting = "Hello, World!"
Booleans are a special type that represents one of two values: True or False. They are often used in conditional expressions:
is_active = True
One of Python’s most powerful features is its collection types. Lists are ordered, mutable sequences that can hold a variety of data types. You can create a list like this:
fruits = ["apple", "banana", "cherry"]
You can access elements in a list by their index, starting at 0:
first_fruit = fruits[0] # 'apple'
Tuples, on the other hand, are similar to lists but are immutable, meaning they cannot be changed after creation:
coordinates = (10, 20)
Dictionaries are a collection of key-value pairs, enabling you to store and retrieve data using unique keys:
person = { "name": "Alice", "age": 30 }
You can access a value in a dictionary by referencing its key:
name = person["name"] # 'Alice'
Finally, sets are unordered collections of unique elements, which can be useful for membership testing and removing duplicates from a list:
unique_fruits = set(fruits)
Control Structures and Loops
Control structures and loops are fundamental components in any programming language, including Python. They provide the necessary mechanisms to dictate the flow of execution in your programs. By mastering these constructs, you can create logic that enables your programs to make decisions, repeat actions, and handle various conditions effectively.
At the heart of control structures is the conditional statement. In Python, the most common conditional statement is the if statement. This allows you to execute code based on whether a condition is true or false. The syntax is straightforward:
age = 20 if age >= 18: print("You are an adult.") else: print("You are a minor.")
In this example, Python evaluates the condition age >= 18. If the condition holds true, the program prints “You are an adult.” If not, it defaults to the else block. You can also chain multiple conditions using elif:
grade = 85 if grade >= 90: print("Grade: A") elif grade >= 80: print("Grade: B") elif grade >= 70: print("Grade: C") else: print("Grade: F")
In this case, the program checks each condition in sequence until it finds one this is true.
Next, we have loops, which allow you to execute a block of code multiple times. The two primary loop constructs in Python are for loops and while loops. A for loop iterates over a sequence (like a list, tuple, or string):
for fruit in ["apple", "banana", "cherry"]: print(fruit)
This loop will print each fruit in the list, one at a time. It’s particularly useful when you know the number of iterations beforehand.
On the other hand, a while loop continues executing as long as a specified condition remains true:
count = 0 while count < 5: print(count) count += 1
This loop will print numbers from 0 to 4. It is essential to ensure that the condition will eventually become false; otherwise, you may end up with an infinite loop.
Python also provides control statements like break and continue to manage loop execution. The break statement immediately exits the loop, while continue skips the current iteration and moves to the next one:
for num in range(10): if num == 5: break # Exit the loop when num is 5 print(num) print("Loop exited.")
In this example, the loop will print numbers 0 through 4 and then exit when it encounters 5.
for num in range(5): if num == 2: continue # Skip the number 2 print(num)
This time, the loop will print 0, 1, 3, and 4, effectively skipping the iteration when num is 2.
Functions and Modules
In Python, functions and modules are essential building blocks that facilitate code organization, reusability, and maintainability. Functions allow you to encapsulate logic into reusable units, while modules enable you to group related functions and variables together. This structure not only makes your code cleaner but also promotes separation of concerns, which is a hallmark of good programming practice.
Creating a function in Python is simpler. The def keyword precedes the function name, followed by parentheses containing any parameters. The body of the function is indented, which indicates the scope of the function. Here’s a simple example of a function that calculates the square of a number:
def square(num): return num * num result = square(5) print(result) # Output: 25
In this example, the square function takes one argument, num, and returns its square. To invoke the function, you simply call it with an argument, and it returns the computed value.
Functions can also have default parameters, which allow you to provide default values for arguments. If an argument is not supplied when the function is called, the default value is used. Here’s an example:
def greet(name="World"): print(f"Hello, {name}!") greet() # Output: Hello, World! greet("Alice") # Output: Hello, Alice!
In this case, if the name argument is omitted, the function will greet “World” by default.
Python allows for flexible function definitions, including keyword arguments, which let you specify arguments by name, regardless of their position. This can enhance clarity in function calls:
def introduce(name, age): print(f"My name is {name} and I am {age} years old.") introduce(age=30, name="Bob") # Output: My name is Bob and I am 30 years old.
Modules in Python are files containing Python code, which can include functions, classes, and variables. By organizing code into modules, you can create a logical structure for your application, making it easier to manage and maintain. To create a module, simply save your Python functions in a file with a .py extension. For instance, let’s create a module called math_utils.py:
# math_utils.py def add(a, b): return a + b def subtract(a, b): return a - b
Once you have your module, you can import it into another Python script using the import statement:
import math_utils result_add = math_utils.add(10, 5) result_subtract = math_utils.subtract(10, 5) print(result_add) # Output: 15 print(result_subtract) # Output: 5
Python also allows selective importing of specific functions from a module, which can reduce namespace clutter:
from math_utils import add result = add(3, 7) print(result) # Output: 10
Another powerful aspect of modules is the ability to create packages, which are directories that contain multiple modules. A package can be imported as a single entity, which will allow you to organize larger applications into manageable components. To create a package, simply create a directory and place an __init__.py file inside it. This file can be empty or can contain initialization code for the package. Here’s a directory structure example:
my_package/ __init__.py math_utils.py string_utils.py
Debugging and Best Practices
Debugging is an essential skill for any programmer, particularly for those working with Python. The process of identifying and fixing issues in your code can sometimes feel like a daunting task, but with the right techniques and tools, you can streamline your debugging process significantly. In Python, there are several built-in features and external tools that can aid in debugging, helping you to efficiently track down errors and improve your code quality.
One of the simplest and most effective methods for debugging in Python is the use of print statements. By strategically placing print statements in your code, you can monitor the flow of execution and the state of variables at different points. This technique can be particularly useful for understanding where your code may not be behaving as expected. For example:
def calculate_average(numbers): total = sum(numbers) count = len(numbers) print(f"Total: {total}, Count: {count}") # Debug print return total / count average = calculate_average([10, 20, 30]) print(f"Average: {average}")
In the above code, the print statement provides insights into the values of total and count before the average is calculated. This can help you verify that your calculations are correct.
Another powerful debugging tool is Python’s built-in pdb module, which stands for Python Debugger. The pdb module allows you to set breakpoints, step through your code line by line, and inspect the current state of your program. To use pdb, you simply need to import it and set a breakpoint with the following command:
import pdb def faulty_function(): pdb.set_trace() # Set a breakpoint x = 10 y = 0 return x / y # This will raise a ZeroDivisionError faulty_function()
When the program reaches pdb.set_trace(), it will pause execution, enabling you to inspect variables and execute Python commands in the context of your program. You can use commands like n (next) to go to the next line, c (continue) to resume execution, and q (quit) to exit the debugger.
Beyond these techniques, adopting best practices can greatly enhance your debugging experience. Writing clear and simple code, using meaningful variable names, and following a consistent formatting style can help prevent many common errors. Additionally, implementing unit tests can catch bugs early in the development process. The unittest module in Python makes it easy to create and run tests for your code:
import unittest def multiply(a, b): return a * b class TestMathFunctions(unittest.TestCase): def test_multiply(self): self.assertEqual(multiply(2, 3), 6) self.assertEqual(multiply(-1, 5), -5) self.assertEqual(multiply(0, 100), 0) if __name__ == "__main__": unittest.main()
In this example, the unittest framework is used to create a test case for the multiply function. By running this test, you can ensure that the function behaves as expected across various scenarios, catching potential errors before your code goes into production.
Lastly, it’s essential to learn from the debugging process. Take note of the types of errors you encounter frequently and think implementing coding standards or using linters like pylint or flake8 to catch stylistic issues before they become bugs. Regularly reviewing and refactoring your code can also lead to a better understanding of your codebase, making future debugging sessions less painful.