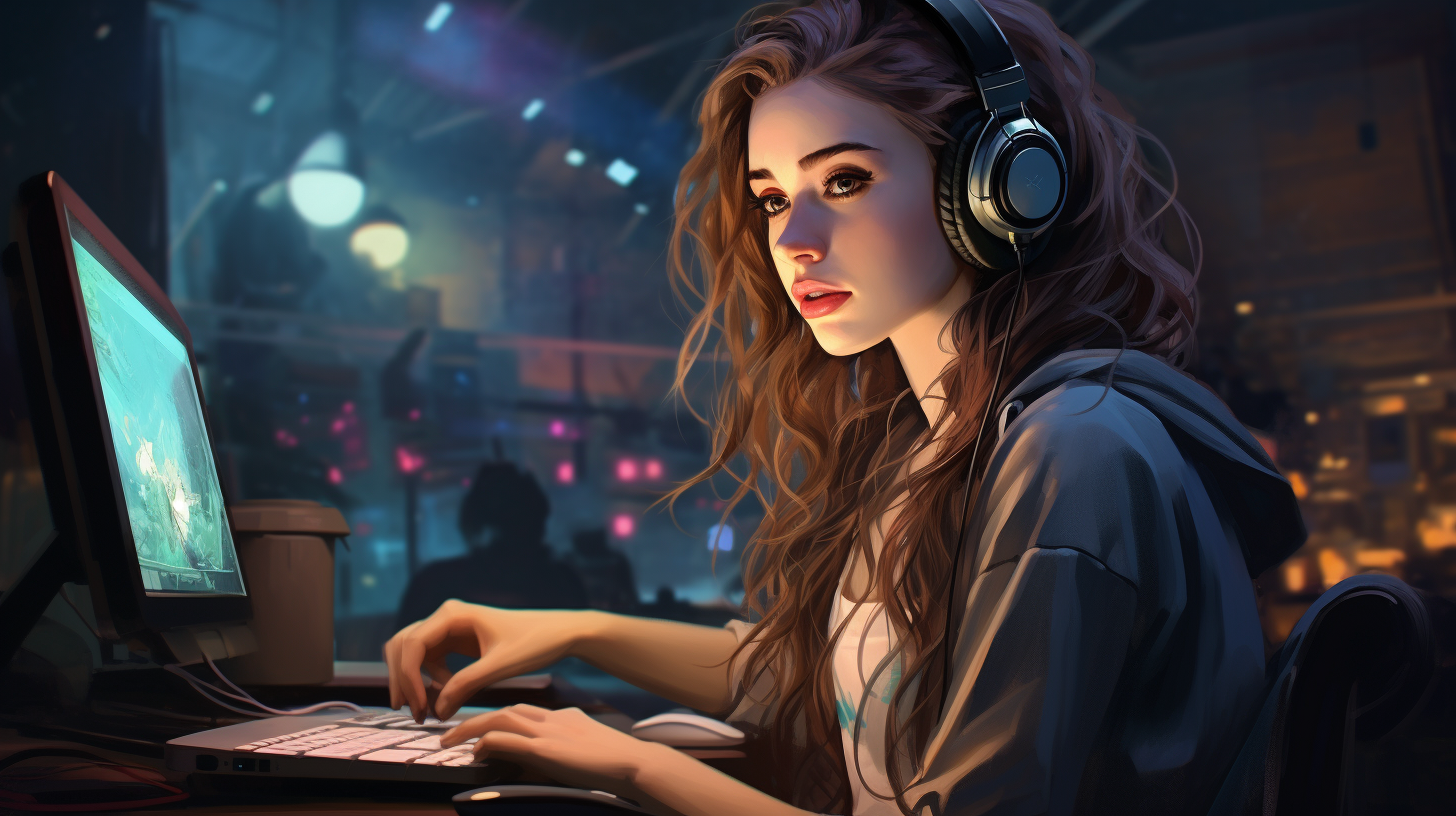
Higher Order Functions in Swift
Higher order functions are a cornerstone of functional programming in Swift, allowing developers to write more concise and expressive code. At their core, these functions can take other functions as parameters, return them as results, or do both. This capability enables a level of abstraction that simplifies complex operations and enhances code reusability.
In Swift, higher order functions can transform data structures, apply operations over collections, and create dynamic behavior based on passed-in functions. Consider the intrinsic nature of functions themselves—they are first-class citizens in Swift, meaning they can be used like any other data type. This allows for an elegant approach to defining behavior and manipulating data.
For example, if you have a function that processes an array of numbers, you can pass in a function that defines how to process each number. This leads to powerful patterns where you can decouple the logic of data processing from the data itself, resulting in cleaner and more maintainable code.
func processNumbers(numbers: [Int], operation: (Int) -> Int) -> [Int] { var results: [Int] = [] for number in numbers { results.append(operation(number)) } return results } let squareOperation: (Int) -> Int = { $0 * $0 } let numbers = [1, 2, 3, 4, 5] let squaredNumbers = processNumbers(numbers: numbers, operation: squareOperation) print(squaredNumbers) // Output: [1, 4, 9, 16, 25]
In this example, the processNumbers
function takes an array of integers and a closure that defines the operation to perform on each integer. The beauty lies in the fact that you can swap out squareOperation
for any other operation without changing the processNumbers
function. This results in flexible and reusable code components.
The use of higher order functions promotes a declarative style of programming, where you specify what you want to achieve rather than how to achieve it. This abstraction not only improves readability but also aligns with Swift’s emphasis on safety and clarity.
Types of Higher Order Functions in Swift
In Swift, higher order functions can be broadly categorized into two types: those that modify existing collections and those that produce new ones. Each type serves different purposes and can significantly streamline the way you handle data within your applications. Understanding these categories is essential for using the full power of higher order functions.
The first type involves functions that operate on collections and often return a modified version of the same collection. That is common with functions like map
and filter
. The map
function transforms each element of a collection based on a provided function, while filter
selects elements that meet a specified criterion. Both of these functions allow you to succinctly express transformations and filtering of data without the need for explicit loops.
let numbers = [1, 2, 3, 4, 5] let doubledNumbers = numbers.map { $0 * 2 } let evenNumbers = numbers.filter { $0 % 2 == 0 } print(doubledNumbers) // Output: [2, 4, 6, 8, 10] print(evenNumbers) // Output: [2, 4]
In these examples, doubledNumbers
and evenNumbers
are derived from the original numbers
array without the need for verbose iteration structures. The intent of the code is clear and direct.
The second type encompasses functions that produce entirely new collections or outcomes based on the transformations applied to the input. A notable example is the reduce
function, which condenses a collection into a single value by iteratively applying a provided function. That is particularly useful for aggregating values or producing results that are derived from the entirety of a collection.
let sum = numbers.reduce(0, { $0 + $1 }) print(sum) // Output: 15
In this instance, reduce
starts with an initial value of 0
and accumulates the total of all elements in the numbers
array. By using higher order functions like reduce
, you can express complex aggregation logic in a compact and readable manner.
Both types of higher order functions are foundational to functional programming in Swift, promoting code reuse and reducing boilerplate. They encourage a style of coding that emphasizes immutability and side-effect-free operations, which is aligned with Swift’s safety and performance goals. By using these functions, developers can create clean, efficient, and highly maintainable codebases.
Using Closures with Higher Order Functions
Closures are a fundamental part of using higher order functions in Swift, so that you can encapsulate functionality and behavior in a succinct manner. A closure in Swift is essentially a self-contained block of functionality that you can pass around in your code. When working with higher order functions, closures enable you to define the exact behavior that will be applied to elements of a collection without defining a separate function each time.
To demonstrate how closures integrate with higher order functions, let’s revisit the operation method we defined earlier. Instead of passing in a predefined function, we can define the closure inline when calling the higher order function. This can simplify your code and improve readability.
let numbers = [1, 2, 3, 4, 5] let cubedNumbers = processNumbers(numbers: numbers, operation: { $0 * $0 * $0 }) print(cubedNumbers) // Output: [1, 8, 27, 64, 125]
In this example, we directly pass a closure that cubes the numbers inline. This demonstrates the flexibility of closures, as it allows you to keep related functionality closely tied together, enhancing the clarity of your intent.
Moreover, closures can capture and store references to variables and constants from the context in which they are defined. This characteristic enables powerful patterns, such as creating functions with “remembered” state. Ponder this example:
func makeIncrementer(incrementAmount: Int) -> (Int) -> Int { var total = 0 return { number in total += incrementAmount return number + total } } let incrementByTwo = makeIncrementer(incrementAmount: 2) print(incrementByTwo(3)) // Output: 5 print(incrementByTwo(3)) // Output: 8
The function `makeIncrementer` returns a closure that, when called, increments a total sum based on a specified `incrementAmount`. Each time you call `incrementByTwo`, it retains its state, demonstrating how closures can provide encapsulated behavior while also maintaining context.
When integrating closures with higher order functions, you can also leverage trailing closure syntax for improved readability. That’s particularly useful when the closure is the last argument of a function, which will allow you to write cleaner and more expressive code. For example:
let filteredNumbers = numbers.filter { $0 > 2 } print(filteredNumbers) // Output: [3, 4, 5]
In this case, the filter function takes a closure that checks if each number is greater than 2, clearly conveying the intent without additional syntactic clutter.
Closures also support capturing values, allowing them to carry over information between invocations. This capability is particularly advantageous in asynchronous programming, where you might want to retain context across different scopes or time frames. In practical applications, this means you can create callbacks and completion handlers that execute once an operation is completed, keeping your code organized and coherent.
By mastering the use of closures with higher order functions, you unlock a powerful toolkit that allows you to express complex logic in a highly maintainable format. The synergy between closures and higher order functions in Swift not only enhances the expressiveness of your code but also aligns seamlessly with the principles of state-of-the-art software development, facilitating a functional programming paradigm that is both efficient and elegant.
Common Higher Order Functions: map, filter, and reduce
Within the scope of Swift programming, three common higher order functions—map, filter, and reduce—serve as essential tools for transforming and manipulating collections. Each of these functions embodies a distinct purpose and operational paradigm, but they share a fundamental trait: they take a function as a parameter and apply it to the elements of a collection. Let’s delve into them one by one, examining their workings and providing practical examples.
The map function is designed to transform each element of a collection based on a closure that you provide. It applies the closure to each element in the original collection and returns a new array containing the results of applying that transformation. This makes it a powerful tool for deriving new collections from existing ones without the verbosity of loops.
let numbers = [1, 2, 3, 4, 5] let squaredNumbers = numbers.map { $0 * $0 } print(squaredNumbers) // Output: [1, 4, 9, 16, 25]
In this example, the map function takes each number from the original array and returns its square, resulting in a new array of squared values.
Next, we have filter, which serves a different purpose: it selects elements from a collection based on a specified condition. The closure passed to filter returns a Boolean value, determining whether each element should be included in the resulting collection. This function is particularly useful for narrowing down data based on specific criteria.
let originalNumbers = [1, 2, 3, 4, 5] let evenNumbers = originalNumbers.filter { $0 % 2 == 0 } print(evenNumbers) // Output: [2, 4]
In this case, filter evaluates each element to see if it’s even, creating a new array that consists only of the even numbers from the original collection.
Finally, we encounter reduce, which is arguably the most versatile of these functions. Reduce takes an initial value and a closure that combines elements of the collection into a single output. This function is extremely useful for aggregating values or performing computations that summarize or transform the entire collection into a single result.
let totalSum = originalNumbers.reduce(0, { $0 + $1 }) print(totalSum) // Output: 15
Here, reduce starts with an initial value of 0 and iteratively adds each element of the original array to it, ultimately yielding the total sum of all elements.
These three higher order functions—map, filter, and reduce—are not just syntactic sugar; they embody a style of programming that emphasizes immutability and declarative logic. They allow developers to express complex data manipulations succinctly and clearly, promoting a more functional programming approach. Using them effectively can lead to cleaner, more maintainable code that’s easier to reason about, ultimately aligning with Swift’s goals of safety and expressiveness.
Practical Examples and Use Cases
When it comes to practical applications of higher order functions in Swift, their utility spans a wide range of scenarios, from simple data transformations to complex business logic implementations. Let’s explore some concrete use cases that showcase the power and flexibility of map, filter, and reduce in real-world situations.
Ponder a scenario where you are working with a collection of user data, specifically names and ages. You may want to perform several operations, such as transforming names to uppercase, filtering out users under a specific age, and calculating the total age of the remaining users. Here’s how you could achieve this using higher order functions:
struct User { let name: String let age: Int } let users = [ User(name: "Alice", age: 30), User(name: "Bob", age: 22), User(name: "Charlie", age: 25) ] // Transform names to uppercase let uppercasedNames = users.map { $0.name.uppercased() } print(uppercasedNames) // Output: ["ALICE", "BOB", "CHARLIE"] // Filter users over the age of 24 let filteredUsers = users.filter { $0.age > 24 } print(filteredUsers.map { $0.name }) // Output: ["Alice", "Charlie"] // Calculate total age of remaining users let totalAge = filteredUsers.reduce(0) { $0 + $1.age } print(totalAge) // Output: 55
In this example, the map function is used to convert all user names to uppercase, showcasing how you can apply a transformation to each element of a collection succinctly. The filter function then narrows down the users to only those who are older than 24, which will allow you to easily handle conditional logic. Finally, the reduce function aggregates the ages of the filtered users, demonstrating its power in summarizing data.
Another compelling use case for higher order functions arises in data visualization, where you may need to prepare data for graphs or charts. Suppose you have an array of sales data represented as tuples containing the month and sales figures. You might want to calculate the total sales for each month, filter out months with sales below a certain threshold, and then prepare the data for presentation.
let salesData: [(String, Double)] = [ ("January", 1500), ("February", 3000), ("March", 2000), ("April", 1800) ] // Calculate total sales let totalSales = salesData.reduce(0) { $0 + $1.1 } print(totalSales) // Output: 8300 // Filter months with sales above 2000 let highSalesMonths = salesData.filter { $1 > 2000 } print(highSalesMonths.map { $0.0 }) // Output: ["February"]
In this scenario, reduce computes the total sales across all months while the filter function isolates months with sales that exceed a specified threshold. This highlights how higher order functions can be instrumental in data processing tasks that involve summarization and filtering.
Moreover, when dealing with asynchronous data loading, higher order functions can enhance clarity and maintainability. Ponder an application where you fetch user data from a remote source, and upon receiving the data, you need to clean it up before displaying it in the user interface. Using map and filter in this context can make the transformation pipeline clearer:
func fetchUserData(completion: @escaping ([User]) -> Void) { // Simulate network fetch let fetchedUsers = [ User(name: "Alice", age: 30), User(name: "Bob", age: 22), User(name: "Charlie", age: 25) ] completion(fetchedUsers) } fetchUserData { users in let validUsers = users.filter { $0.age >= 18 } let names = validUsers.map { $0.name } print(names) // Output: ["Alice", "Charlie"] }
Here, the fetchUserData function simulates an asynchronous network operation. Once the data is received, we filter out users who are not adults and map the remaining users to their names. This approach not only maintains readability but also highlights how higher order functions can seamlessly integrate into asynchronous workflows.
Through these examples, it becomes evident that higher order functions like map, filter, and reduce provide developers with powerful tools to transform and manipulate data efficiently. By embracing these functional paradigms, Swift developers can create more expressive, maintainable, and elegant code that naturally aligns with the demands of modern software development.