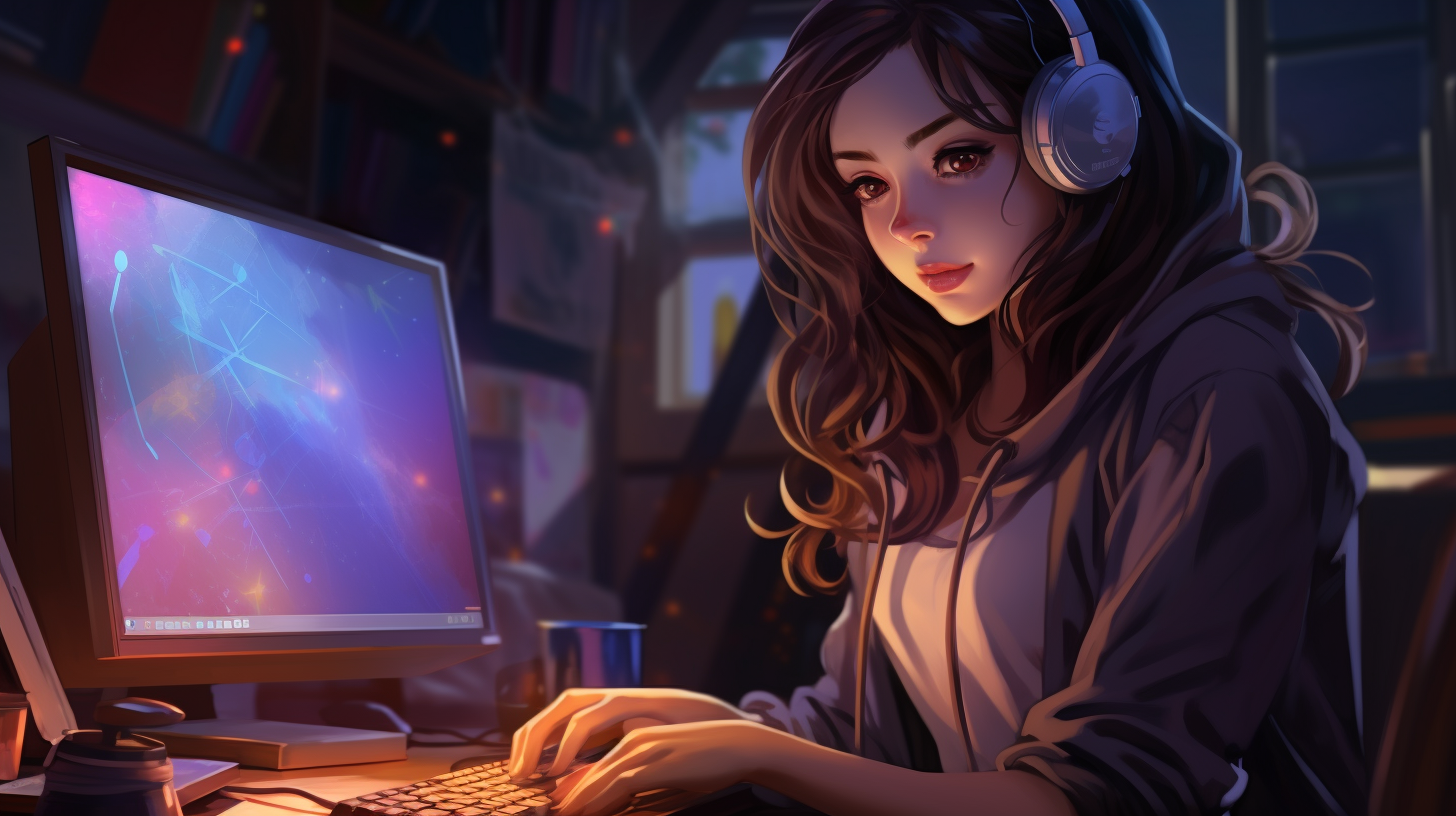
Implementing Efficient SQL Joins
Within the scope of SQL, joins are the fundamental constructs that allow us to combine data from different tables based on related columns. Understanding the various types of joins and their use cases is important for any developer aiming to harness the full power of relational databases.
There are several types of joins, each serving a distinct purpose:
- This join retrieves records that have matching values in both tables. It is the most commonly used join and is ideal when you need to find overlaps between two datasets.
SELECT a.column1, b.column2 FROM tableA a INNER JOIN tableB b ON a.common_column = b.common_column;
SELECT a.column1, b.column2 FROM tableA a LEFT JOIN tableB b ON a.common_column = b.common_column;
SELECT a.column1, b.column2 FROM tableA a RIGHT JOIN tableB b ON a.common_column = b.common_column;
SELECT a.column1, b.column2 FROM tableA a FULL JOIN tableB b ON a.common_column = b.common_column;
SELECT a.column1, b.column2 FROM tableA a CROSS JOIN tableB b;
Choosing the appropriate type of join is essential. Inner joins are your go-to for finding commonalities, while outer joins help maintain comprehensive datasets where relationships might be sparse. Understanding when to use each join type can significantly affect the efficiency and clarity of your SQL queries.
In many scenarios, especially in data analytics, you may find yourself needing to join tables not only for simple relationships but to analyze data across complex structures. For instance, when working with a database containing sales and customer information, an inner join could reveal which customers purchased certain products, while a left join could help identify customers who did not make any purchases.
Best Practices for Writing Efficient Joins
When it comes to writing efficient SQL joins, there are several best practices to keep in mind. These guidelines ensure not only that your queries perform well but also that they remain readable and maintainable. Here are key strategies to consider:
1. Choose the Right Type of Join
As we have discussed, selecting the appropriate join type is fundamental. Carefully assess the relationships between your tables and choose either inner, left, right, or full joins based on your specific needs. This choice impacts both performance and the integrity of your results.
2. Use Aliases for Readability
Using table aliases can significantly enhance the readability of your SQL queries, especially when joining multiple tables. By providing a shorthand reference to each table, you can simplify both your syntax and the mental overhead required to parse your joins.
SELECT c.customer_name, o.order_date FROM customers c INNER JOIN orders o ON c.customer_id = o.customer_id;
3. Filter Early with WHERE Clauses
Applying filters as early as possible in your joins can reduce the amount of data processed. Ensure to use WHERE clauses effectively to limit the number of records being pulled from each table. This not only speeds up execution but also lowers memory consumption.
SELECT p.product_name, o.order_date FROM products p INNER JOIN orders o ON p.product_id = o.product_id WHERE p.category = 'Electronics';
4. Be Mindful of Join Order
The order in which you join tables can impact performance significantly. SQL engines may optimize query execution plans differently based on the order, so try to join smaller tables first or those with fewer matching records to reduce the overall workload.
5. Avoid SELECT *
While it may be tempting to use SELECT * to retrieve all columns from the joined tables, this practice can lead to inefficiencies. Instead, explicitly specify the columns you need. This not only enhances performance but also makes your SQL statements clearer and more maintainable.
SELECT c.customer_name, o.order_total FROM customers c INNER JOIN orders o ON c.customer_id = o.customer_id;
6. Utilize Indexes
Indexes play an important role in join performance. Ensure that the columns used in JOIN conditions and WHERE clauses are indexed appropriately. This minimizes the time required for lookup operations, drastically improving query speed.
7. Analyze Query Performance
Regularly review the execution plans for your SQL queries to understand how they perform. Tools such as the SQL Server Management Studio’s execution plan feature can provide insights into potential bottlenecks and areas for optimization.
8. Test with Sample Data
Before finalizing your joins in production settings, test them with a representative subset of your data. This practice can reveal unexpected performance issues and help you optimize your queries before they impact a larger dataset.
Optimizing Join Performance with Indexes
When it comes to the performance of SQL joins, one of the most effective strategies for optimization lies in the use of indexes. Indexes serve as pointers to data within a table, significantly speeding up data retrieval operations. They’re especially vital when joining tables, as they allow the database engine to quickly locate the rows that meet the join condition. However, implementing indexes requires a nuanced understanding to balance performance gains against the overhead of maintaining those indexes.
To optimize join performance with indexes, the following guidelines should be observed:
1. Index Join Columns
To improve the speed of join operations, it’s critical to index the columns used in your JOIN conditions. For example, if you frequently join the `orders` and `customers` tables on their `customer_id`, think creating indexes on the `customer_id` columns in both tables.
CREATE INDEX idx_customer_id ON customers(customer_id); CREATE INDEX idx_customer_id ON orders(customer_id);
2. Use Composite Indexes for Multiple Columns
In cases where your join involves multiple columns, composite indexes can further improve performance. For instance, if you are joining `orders` and `products` on both `product_id` and `order_date`, a composite index on these columns in the `orders` table can be beneficial.
CREATE INDEX idx_product_order ON orders(product_id, order_date);
3. Consider Index Selectivity
The selectivity of an index (the ratio of unique values to the total number of values) greatly influences its effectiveness. High selectivity indexes tend to provide better performance. For example, indexing a boolean column may not yield significant performance improvements due to low selectivity. Always prioritize columns with higher variability for indexing.
4. Regularly Maintain Indexes
Indexes require maintenance to remain efficient. Over time, as data is added, updated, or deleted, indexes can become fragmented, leading to degraded performance. Regularly rebuilding or reorganizing indexes very important to ensure they remain optimized.
ALTER INDEX idx_customer_id ON customers REBUILD;
5. Analyze Query Plans
Utilize the database’s query analysis tools to inspect the execution plans of your join queries. This will help you determine if your indexes are being utilized effectively. If you find that SQL is performing a full table scan instead of using an index, it may be a signal to adjust your indexing strategy.
6. Avoid Over-Indexing
While indexes speed up read operations, they introduce overhead for write operations. Every time a record is inserted, updated, or deleted, the corresponding indexes must also be updated. Over-indexing can lead to performance bottlenecks on write-heavy operations, so it’s vital to find a balance.
Avoiding Common Pitfalls in SQL Joins
When working with SQL joins, developers often encounter common pitfalls that can lead to inefficient queries, incorrect results, or unexpected behavior. Recognizing and avoiding these issues is important for writing effective SQL code. Here are some of the most frequent pitfalls to be aware of when implementing joins:
1. Ignoring NULL Values
One of the most significant pitfalls arises from not accounting for NULL values, especially when using outer joins. In a LEFT JOIN, if a record in the left table has no matching record in the right table, all columns from the right table will return NULL. Failing to handle these NULLs in subsequent processing can lead to misleading results or errors in calculations.
SELECT c.customer_id, c.customer_name, o.order_total FROM customers c LEFT JOIN orders o ON c.customer_id = o.customer_id WHERE o.order_total IS NOT NULL; -- This can lead to missing customers with no orders
2. Using Non-Indexed Columns for Joins
Joining on columns that are not indexed can severely degrade performance, especially with large datasets. The database has to perform full table scans to find matching rows, which is inefficient. Always ensure that the columns used in JOIN conditions are indexed to speed up the query execution.
SELECT c.customer_name, o.order_date FROM customers c INNER JOIN orders o ON c.customer_id = o.customer_id; -- Ensure customer_id is indexed
3. Cartesian Products
Accidentally creating a Cartesian product due to missing join conditions is a common mistake. This occurs when two tables are joined without an ON clause, resulting in a combination of every row from the first table with every row from the second, which can lead to massive data sets and performance issues.
SELECT a.column1, b.column2 FROM tableA a, tableB b; -- Missing ON clause leading to a Cartesian product
4. Overusing SELECT *
While it may seem convenient to use SELECT * to fetch all columns from joined tables, this practice can lead to performance degradation and unnecessary data transfer. Instead, explicitly specify only the columns you need. This not only improves performance but also enhances clarity and maintainability in your SQL code.
SELECT c.customer_name, o.order_date FROM customers c INNER JOIN orders o ON c.customer_id = o.customer_id; -- Specify only needed columns
5. Failing to Understand Join Logic
Misunderstanding the logic of different joins can lead to incorrect results. For instance, using an INNER JOIN when you need a LEFT JOIN can exclude necessary records. Always clarify the business requirements and ensure that the join type aligns with the intended data extraction needs.
SELECT c.customer_name, o.order_total FROM customers c LEFT JOIN orders o ON c.customer_id = o.customer_id; -- Necessary for retaining all customers
6. Not Testing with Various Data Sets
Failing to test SQL joins across diverse datasets can lead to unforeseen issues in production. Be sure to test with edge cases, such as tables with no data, tables with NULL values, and different distributions of data to ensure that your joins behave as expected under all conditions.
-- Test with empty tables SELECT c.customer_name, o.order_date FROM customers c LEFT JOIN orders o ON c.customer_id = o.customer_id WHERE o.order_date IS NULL;
Advanced Join Techniques for Complex Queries
When dealing with complex queries, advanced join techniques can be invaluable. They allow for more sophisticated data retrieval patterns that go beyond simple relationships. Understanding these techniques can significantly enhance the capability of your SQL queries and the insights you can derive from your data.
One such technique involves using Subqueries in conjunction with joins. A subquery, or a query nested within another SQL query, can be employed to filter or aggregate data before performing the join. This approach often simplifies the logic of the main query and can enhance performance by reducing the dataset size prior to the join operation.
SELECT c.customer_name, (SELECT COUNT(*) FROM orders o WHERE o.customer_id = c.customer_id) AS order_count FROM customers c WHERE c.customer_id IN (SELECT DISTINCT customer_id FROM orders);
This query retrieves customer names alongside the count of their orders, using a subquery to first filter relevant customers. By incorporating the subquery, you ensure that only customers with orders are considered, thus streamlining the data retrieval process.
Another powerful technique is employing Self Joins. A self join is a regular join that joins a table to itself. That is particularly useful for hierarchical or recursive data structures, such as organizational charts or product categories, where each record may have a relationship with another record in the same table.
SELECT a.employee_name AS Employee, b.employee_name AS Manager FROM employees a LEFT JOIN employees b ON a.manager_id = b.employee_id;
In this query, the employees table is joined to itself to find each employee’s manager. By using a self join, you can easily represent hierarchies within your data, allowing for insightful queries that reveal relationships within the same dataset.
Cross Joins can also serve advanced purposes, particularly when combined with filtering clauses. While cross joins generate the Cartesian product of two tables, they can be effective in scenarios where you want every combination of two sets of data, followed by a specific filtering operation.
SELECT a.product_name, b.supplier_name FROM products a CROSS JOIN suppliers b WHERE a.category = 'Electronics' AND b.region = 'North America';
This example retrieves combinations of products and suppliers filtered by specific categories, showcasing how cross joins can yield valuable insights when used judiciously.
Additionally, Common Table Expressions (CTEs) can enhance the readability and structure of complex joins. CTEs allow you to define temporary result sets that can be referenced within the main query. This is especially useful for breaking down complex joins into manageable components, improving both readability and maintainability.
WITH OrderSummary AS ( SELECT customer_id, COUNT(*) AS order_count FROM orders GROUP BY customer_id ) SELECT c.customer_name, os.order_count FROM customers c JOIN OrderSummary os ON c.customer_id = os.customer_id;
In this case, the CTE `OrderSummary` simplifies the main query by pre-aggregating order counts, thereby enhancing clarity and performance.
Lastly, don’t overlook the utility of UNION and UNION ALL in scenarios where you need to combine results from multiple queries. While they don’t directly relate to joins, they can often be used alongside joins when different datasets need to be merged, providing a holistic view of your data.
SELECT customer_id, 'existing' AS status FROM customers UNION ALL SELECT customer_id, 'new' AS status FROM new_customers;
This query combines existing customer IDs with those from a new customers table, indicating the source of each record. This technique can be particularly effective in reporting scenarios.
Case Studies: Real-World Applications of SQL Joins
In the context of data management, the application of SQL joins goes beyond theoretical understanding; real-world case studies illuminate their practical significance. Examining how organizations leverage SQL joins can provide invaluable insights into best practices and innovative strategies for data retrieval.
For instance, ponder a retail company that utilizes a relational database to manage its customer and sales data. To analyze customer purchasing behavior, the marketing team may need to determine which products are most popular among different customer demographics. By implementing an INNER JOIN between the customers
and sales
tables, they can retrieve pertinent data that correlates customer profiles with their purchasing history. The query might look like this:
SELECT c.customer_id, c.age_group, s.product_id, COUNT(s.product_id) AS purchase_count FROM customers c INNER JOIN sales s ON c.customer_id = s.customer_id GROUP BY c.customer_id, c.age_group, s.product_id ORDER BY purchase_count DESC;
This query allows the marketing team to understand which age groups are buying specific products, enabling targeted promotions and marketing strategies.
Another compelling case study involves a financial institution managing accounts and transactions. To comply with regulatory requirements and enhance customer service, the bank might need to identify customers with low balances who have not made recent transactions. A LEFT JOIN can help retain all customer records while retrieving relevant transaction data:
SELECT c.customer_id, c.account_balance, t.transaction_date FROM customers c LEFT JOIN transactions t ON c.customer_id = t.customer_id WHERE c.account_balance < 100 AND t.transaction_date IS NULL;
In this scenario, the bank can identify potentially at-risk customers, leading to proactive outreach and improved customer retention strategies.
In the healthcare sector, hospitals often need to consolidate patient records and treatment histories to enhance care quality. By employing a FULL JOIN between patient data and treatment history, healthcare administrators can gain a comprehensive view of patient interactions:
SELECT p.patient_id, p.patient_name, t.treatment_date, t.treatment_type FROM patients p FULL JOIN treatments t ON p.patient_id = t.patient_id;
This query ensures that all patients, regardless of whether they have received treatment, are included in the analysis, providing insights into overall healthcare delivery and treatment gaps.
Moreover, ponder a logistics company that manages shipments and delivery routes. They might leverage a SELF JOIN to optimize route planning by analyzing how different shipments relate to each other based on their origins and destinations:
SELECT s1.shipment_id AS shipment1, s2.shipment_id AS shipment2 FROM shipments s1 JOIN shipments s2 ON s1.destination = s2.origin WHERE s1.shipment_id s2.shipment_id;
This query can help identify opportunities for consolidating shipments, thus increasing efficiency and reducing costs.
Lastly, in the context of e-commerce, businesses often need to analyze user behavior across different platforms. By using UNION ALL to combine data from web and mobile user interactions, companies can gain a holistic understanding of customer engagement:
SELECT user_id, 'web' AS source, page_viewed FROM web_user_activity UNION ALL SELECT user_id, 'mobile' AS source, screen_viewed AS page_viewed FROM mobile_user_activity;
This approach allows for a unified view of user activity across platforms, enabling businesses to refine their user experience strategies.