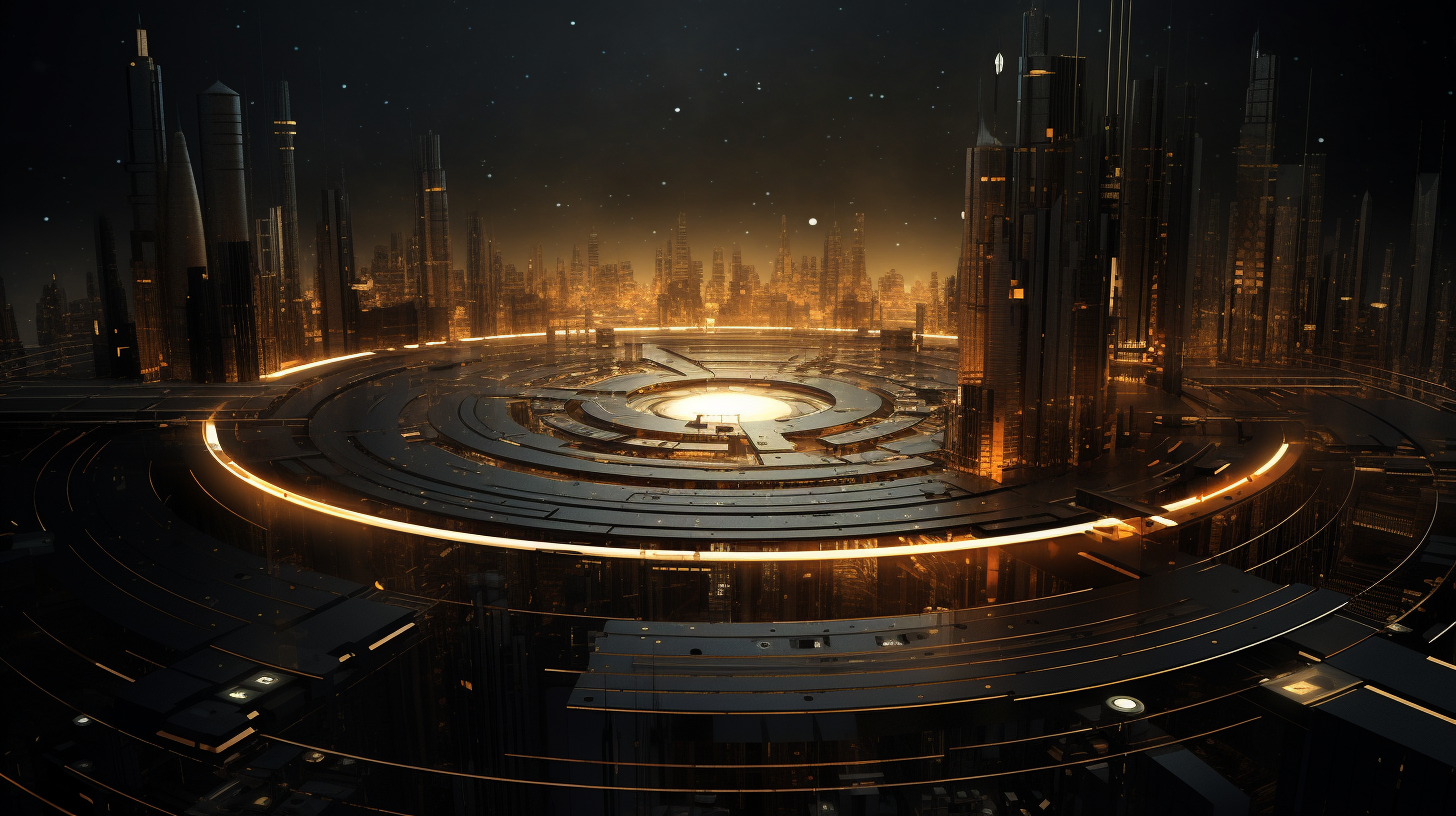
Index Selection Strategies in SQL
When it comes to indexing in SQL, understanding the various types of indexes and their specific use cases is paramount for optimizing database performance. Each index type serves distinct purposes and is suited for particular scenarios, making the choice of the right index critical.
Here’s a rundown of the most common index types:
-
B-Tree Indexes:
B-Tree (Balanced Tree) indexes are the default indexing structure for many SQL databases. They’re efficiently used for equality and range queries. The tree structure allows for quick traversal, which means both search and insertion can be performed in logarithmic time.
CREATE INDEX idx_name ON table_name (column_name);
-
Hash Indexes:
Hash indexes are designed for equality comparisons, using a hash table for fast lookups. They’re optimal for situations where you need to find a specific value. However, they don’t support range queries, which limits their use cases.
CREATE INDEX idx_name ON table_name USING HASH (column_name);
-
Bitmap Indexes:
Bitmap indexes use a bitmap to represent the existence of a value in a column. They are particularly effective for low-cardinality columns (columns with a small number of distinct values), such as gender or status fields. Their compact representation allows for fast query performance in analytical applications.
CREATE BITMAP INDEX idx_name ON table_name (column_name);
-
Full-Text Indexes:
Full-text indexes are used for searching text-based data. They allow for more complex queries such as searching for words or phrases within large text fields. This makes them perfect for applications like content management systems or any scenario where text search functionality is required.
CREATE FULLTEXT INDEX idx_name ON table_name (text_column);
-
Spatial Indexes:
Spatial indexes are specialized for geographic data types and are used to optimize queries involving spatial relationships, such as distance calculations or containment queries. They’re essential for GIS (Geographic Information Systems) applications.
CREATE SPATIAL INDEX idx_name ON table_name (geometry_column);
Choosing the right index type depends on the specific needs of the application, the nature of the data, and the types of queries that will be executed. Each index type has its strengths and weaknesses, and understanding these will guide you towards making informed decisions for optimal indexing strategies.
Factors Influencing Index Selection
Several factors come into play when determining the best indexing strategy for your SQL database. Understanding these factors can significantly enhance your ability to select the most effective indexes for your application, ultimately improving query performance and resource management.
1. Query Patterns: The nature and frequency of your queries are critical in index selection. Analyzing the types of queries—whether they are primarily read-heavy or involve frequent writes—helps in choosing the appropriate index. If your application predominantly performs read operations, consider using indexes that facilitate faster lookups. Conversely, if there are a high number of write operations, be mindful of the overhead that indexes can introduce during data modification.
EXPLAIN SELECT * FROM table_name WHERE column_name = 'value';
This command will show you how SQL plans to execute your query, revealing whether an index is utilized.
2. Data Characteristics: The nature of the data within your tables also influences index selection. Factors such as data distribution, column cardinality (the uniqueness of data within a column), and data type play a significant role. For example, if a column has low cardinality, a bitmap index may be appropriate, while high-cardinality columns might benefit from B-Tree indexes for efficient searching.
CREATE INDEX idx_high_card ON table_name (high_card_column);
3. Update Frequency: The frequency of updates to the indexed columns must also be considered. High-frequency updates can lead to performance degradation because the database must maintain the index structure, creating overhead during insert, update, and delete operations. In such cases, it might be prudent to limit the number of indexes or utilize indexed views if applicable.
CREATE INDEX idx_updates ON table_name (update_column);
4. Size of the Dataset: The volume of data stored within a table can heavily influence the choice of indexing strategy. Larger datasets might benefit from partitioned indexes or specialized indexing techniques tailored for big data to optimize both read and write operations. Conversely, smaller datasets may not require complex indexing strategies, as table scans could be efficient enough.
CREATE INDEX idx_partitioned ON large_table (partition_column);
5. Use of Composite Indexes: When queries involve multiple columns, composite indexes can be beneficial. A composite index allows the database to maintain a single index covering multiple columns, which can dramatically improve the performance of queries that use those columns in their filtering conditions. However, it’s essential to ponder the order of columns in the index, as it impacts its effectiveness.
CREATE INDEX idx_composite ON table_name (column1, column2);
6. Database Engine and Version: The capabilities and limitations of the database engine and its version should also inform your index selection. Different SQL engines may have unique features or optimizations related to indexing. Staying updated on the latest developments can lead to better indexing practices and strategies.
By carefully evaluating these factors, you can make informed decisions that align with the specific requirements of your application, leading to improved performance and efficiency in your SQL database operations.
Cost Analysis of Indexing Strategies
Cost analysis is essential when assessing indexing strategies, as it allows database administrators to understand the trade-offs associated with creating and maintaining indexes. Every index incurs certain costs, including storage, maintenance overhead, and potential performance implications during data modifications. Evaluating these costs against the benefits gained from improved query performance is key to making informed decisions.
1. Storage Costs: Every index consumes disk space, and this can become significant depending on the number and types of indexes created. For instance, B-Tree indexes typically consume more space than bitmap indexes due to their structural complexity. When planning your indexing strategy, ponder the total storage cost of the indexes compared to the performance improvements they provide.
SELECT pg_size_pretty(pg_total_relation_size('table_name')) AS total_size;
This query provides insight into the total size of a table, including its indexes, helping to evaluate the storage overhead introduced by indexing.
2. Maintenance Costs: Indexes require maintenance, particularly during INSERT, UPDATE, and DELETE operations. Each modification to the underlying data may necessitate adjustments to the index structures, resulting in additional I/O operations. Understanding the frequency of these modifications is vital; excessive updates can lead to degraded performance. For high-update tables, it may be beneficial to minimize the number of indexes or employ strategies like indexed views to balance performance.
CREATE INDEX idx_updates ON table_name (update_column);
Here, the maintenance overhead of the `idx_updates` index should be evaluated against the frequency of updates to the indexed column.
3. Performance Gains: Ultimately, the core motivation for implementing indexes is to boost query performance. The benefits gained from faster retrieval times must be weighed against the costs incurred. Analyzing query performance before and after indexing can provide a clearer picture of the effectiveness of your indexing strategy. The EXPLAIN command can be employed to visualize how queries utilize indexes.
EXPLAIN ANALYZE SELECT * FROM table_name WHERE column_name = 'value';
This command will output the execution plan, showing how the query optimizer uses the indexes and providing metrics on query performance, which can guide further indexing decisions.
4. Break-Even Analysis: A break-even analysis can help determine the point at which the costs of maintaining an index outweigh the performance benefits it provides. This involves calculating the cost of the index maintenance over time and comparing it to the time saved from improved query performance. If the cost of maintenance exceeds the time saved through faster queries, it may be time to reconsider the index.
5. Index Tuning: The process of index tuning involves adjusting existing indexes based on their usage patterns and performance metrics. Regularly monitoring index performance may uncover underutilized or redundant indexes. In cases where an index does not significantly contribute to query performance or maintenance costs are high, it may be prudent to drop the index.
DROP INDEX idx_unnecessary;
By performing this analysis regularly, database administrators can maintain an optimal balance between performance and cost over time.
Best Practices for Index Maintenance
Index maintenance is a critical aspect of database management that can significantly influence overall performance. As data changes, the integrity and efficiency of indexes must be preserved to ensure that they continue to serve their intended purpose. A well-maintained index can lead to faster query performance, while neglected indexes may cause slowdowns and increased resource consumption. Here are some best practices to consider for effective index maintenance:
1. Regular Index Rebuilding and Reorganization: Over time, indexes can become fragmented due to ongoing data modifications. Fragmentation occurs when the logical order of index entries becomes disorganized, leading to inefficient data retrieval. Regularly rebuilding or reorganizing indexes can mitigate this issue, restoring optimal performance.
ALTER INDEX idx_name REBUILD;
Alternatively, for less severe fragmentation, you might ponder reorganizing the index instead:
ALTER INDEX idx_name REORGANIZE;
2. Analyze Index Usage: Use monitoring tools or queries to analyze how often each index is utilized. Identifying unused indexes can help reduce maintenance costs and improve write performance. If an index is seldom or never used, it may be a candidate for removal.
SELECT * FROM sys.dm_db_index_usage_stats WHERE object_id = OBJECT_ID('table_name');
3. Update Statistics: Statistics play an important role in the SQL query optimizer’s ability to make informed decisions about using indexes. Outdated statistics can lead to suboptimal query plans, causing the optimizer to ignore indexes that would otherwise enhance performance. Regularly updating statistics ensures that the optimizer has the latest information about the distribution of data within indexed columns.
UPDATE STATISTICS table_name;
4. Think the Impact of Data Growth: When the size of your dataset increases, the effectiveness of existing indexes can change. Periodically reassess your indexing strategy in light of data growth. You may need to create new indexes or adjust existing ones to accommodate changes in query patterns and data distribution.
5. Monitor for Redundant Indexes: Redundant indexes—those that cover the same data as other indexes—can detract from performance and increase maintenance overhead. Conduct regular reviews to identify and eliminate redundant indexes, thereby simplifying index management.
SELECT i.name AS IndexName, i.type_desc AS IndexType, COUNT(*) AS DuplicateCount FROM sys.indexes i JOIN sys.index_columns ic ON i.object_id = ic.object_id AND i.index_id = ic.index_id GROUP BY i.name, i.type_desc HAVING COUNT(*) > 1;
6. Evaluate Indexes in Relation to Query Performance: Periodically assess how indexes are impacting the performance of your queries. Utilize the EXPLAIN command to view query execution plans and understand how indexes are being utilized. This evaluation can inform adjustments and optimizations to your indexing strategy.
EXPLAIN SELECT * FROM table_name WHERE column_name = 'value';
7. Automate Maintenance Tasks: Many database systems provide options to automate index maintenance tasks, such as rebuilding or reorganizing indexes and updating statistics. Using these automated processes ensures that your indexes are consistently maintained without manual intervention, reducing the risk of performance degradation.
By adhering to these best practices for index maintenance, database administrators can optimize the performance of their SQL databases, ensuring that indexes contribute positively to query efficiency and resource use. As the database environment evolves, continuous assessment and adjustment of your indexing strategy will keep your database responsive and performant.
Evaluating Index Performance
Evaluating index performance is a critical step in ensuring that your SQL database remains efficient and responsive to queries. Performance evaluation involves measuring the impact of indexes on query execution times, resource consumption, and overall database performance. By understanding how indexes are utilized within your queries, you can make informed decisions about index creation, modification, or removal. Here are some methodologies and techniques to effectively evaluate index performance:
1. **Use of Execution Plans:** The first and foremost tool at your disposal is the execution plan. By employing the EXPLAIN
command, you can retrieve valuable insights into how the SQL engine processes a query, including whether an index is being utilized. This information can help pinpoint which indexes are beneficial and which may be underperforming.
EXPLAIN SELECT * FROM table_name WHERE column_name = 'value';
This command will reveal the execution plan, detailing how the database engine retrieves data, thus so that you can assess the effectiveness of the current indexing strategy.
2. **Measure Query Performance:** Comparing the execution time of queries with and without indexes can provide direct evidence of their impact. To do this, you can utilize timing functions to capture the execution duration. If removing or altering an index leads to significantly slower query performance, this can indicate the necessity of that index.
SET STATISTICS TIME ON; SELECT * FROM table_name WHERE column_name = 'value'; SET STATISTICS TIME OFF;
3. **Analyze I/O Statistics:** Understanding how many disk reads and writes a particular query incurs can shed light on the efficiency of your indexes. The SET STATISTICS IO ON
command can provide this information, helping you identify potential bottlenecks caused by inefficient indexing.
SET STATISTICS IO ON; SELECT * FROM table_name WHERE column_name = 'value'; SET STATISTICS IO OFF;
4. **Monitor Wait Statistics:** Database performance issues often arise from waits caused by inefficient indexing. Monitoring wait statistics can help identify if queries are waiting on index-related locks or if they are experiencing contention. Analyzing this data can guide you in deciding whether to optimize existing indexes or create new ones.
SELECT * FROM sys.dm_os_wait_stats ORDER BY wait_time_ms DESC;
5. **Utilize Performance Monitoring Tools:** Many modern SQL database systems come equipped with performance monitoring tools that can provide real-time insights into index usage. These tools often include dashboards that visualize index use, I/O statistics, and query performance metrics, making it easier to identify areas for improvement.
6. **Evaluate Index Hit Ratios:** The index hit ratio indicates how often a query can retrieve data using an index versus having to scan the table. A high hit ratio suggests that the index is being utilized effectively, while a low ratio may indicate that the index is not beneficial or that queries need to be rewritten to take better advantage of existing indexes.
SELECT OBJECT_NAME(object_id) AS TableName, name AS IndexName, user_seeks, user_scans, user_lookups FROM sys.dm_db_index_usage_stats WHERE database_id = DB_ID('YourDatabaseName');
7. **Regular Index Review:** Conducting regular reviews of index performance is essential. Over time, as data and query patterns evolve, certain indexes may become less relevant or underutilized. Periodic assessments can help identify these indexes, enabling you to optimize the index set to align with current usage patterns.
8. **Consider Composite Indexes:** If you notice that queries often filter or sort by multiple columns, think implementing composite indexes. Evaluating the performance impact of these indexes can lead to significant improvements in query execution times.
CREATE INDEX idx_composite ON table_name (column1, column2);
By employing these techniques to evaluate index performance, you can ensure that your indexing strategies remain aligned with the evolving needs of your SQL database. Optimizing index performance leads to enhanced query efficiency, reduced latency, and better overall database management.
Case Studies of Successful Index Implementations
When discussing successful index implementations, it is essential to delve into real-world examples that illustrate the complexities and advantages of effective indexing strategies. These case studies provide valuable insights into how businesses have leveraged indexing techniques to improve performance and optimize resource use in their SQL databases.
One notable case is that of an e-commerce platform that faced significant performance challenges as its product catalog expanded. The increasing volume of data led to slow query responses during peak usage times, particularly for product searches. After a thorough analysis of their query patterns and data structure, the database administrators decided to implement composite indexes on the product listings table. Specifically, they created an index that combined the columns for category, price, and rating.
CREATE INDEX idx_product_search ON products (category_id, price, rating);
This composite index drastically reduced the search time for users looking for products within specific categories and price ranges. The execution plan revealed that the database now effectively utilized the index, resulting in reduced table scans and improved overall query performance. Post-implementation analytics showed a 40% decrease in average query response times, leading to enhanced user satisfaction and increased sales during peak periods.
Another compelling case comes from a financial institution that needed to optimize their reporting queries, which often involved aggregating vast amounts of transactional data. The original setup included multiple individual indexes, which were not sufficiently addressing the performance bottlenecks observed during monthly reporting cycles. A decision was made to implement bitmap indexes on lower-cardinality columns, such as transaction types and status flags.
CREATE BITMAP INDEX idx_transaction_status ON transactions (transaction_status);
By using bitmap indexes, the financial institution achieved significant performance improvements, particularly in their analytical queries that required aggregating data across various dimensions. The reduction in query execution time was marked—some reports that once took several minutes were completed in under a second. This not only optimized query performance but also reduced server load during critical reporting periods.
Similarly, a health care provider faced challenges in managing patient records due to the rapid growth of their database. With frequent updates and a need for quick retrievals, they decided to implement full-text indexing on their patient notes. This allowed for efficient searches through extensive text fields, enabling clinicians to find relevant information quickly.
CREATE FULLTEXT INDEX idx_patient_notes ON patient_records (notes);
As a result, the time taken to search through patient notes for specific keywords was reduced significantly, enabling healthcare professionals to provide timely care based on accurate and fast information retrieval. The implementation of full-text indexing proved to be a game changer, demonstrating how tailored indexing strategies can dramatically improve operational efficiency in high-stakes environments.
These examples highlight the diverse approaches organizations can take to implement effective indexing strategies. From e-commerce to healthcare and finance, the careful consideration of data characteristics and query patterns has led to substantial performance gains. The success stories illustrate not only the importance of proper index selection but also the necessity of ongoing evaluation and adjustment to indexing strategies to adapt to evolving data and usage requirements.