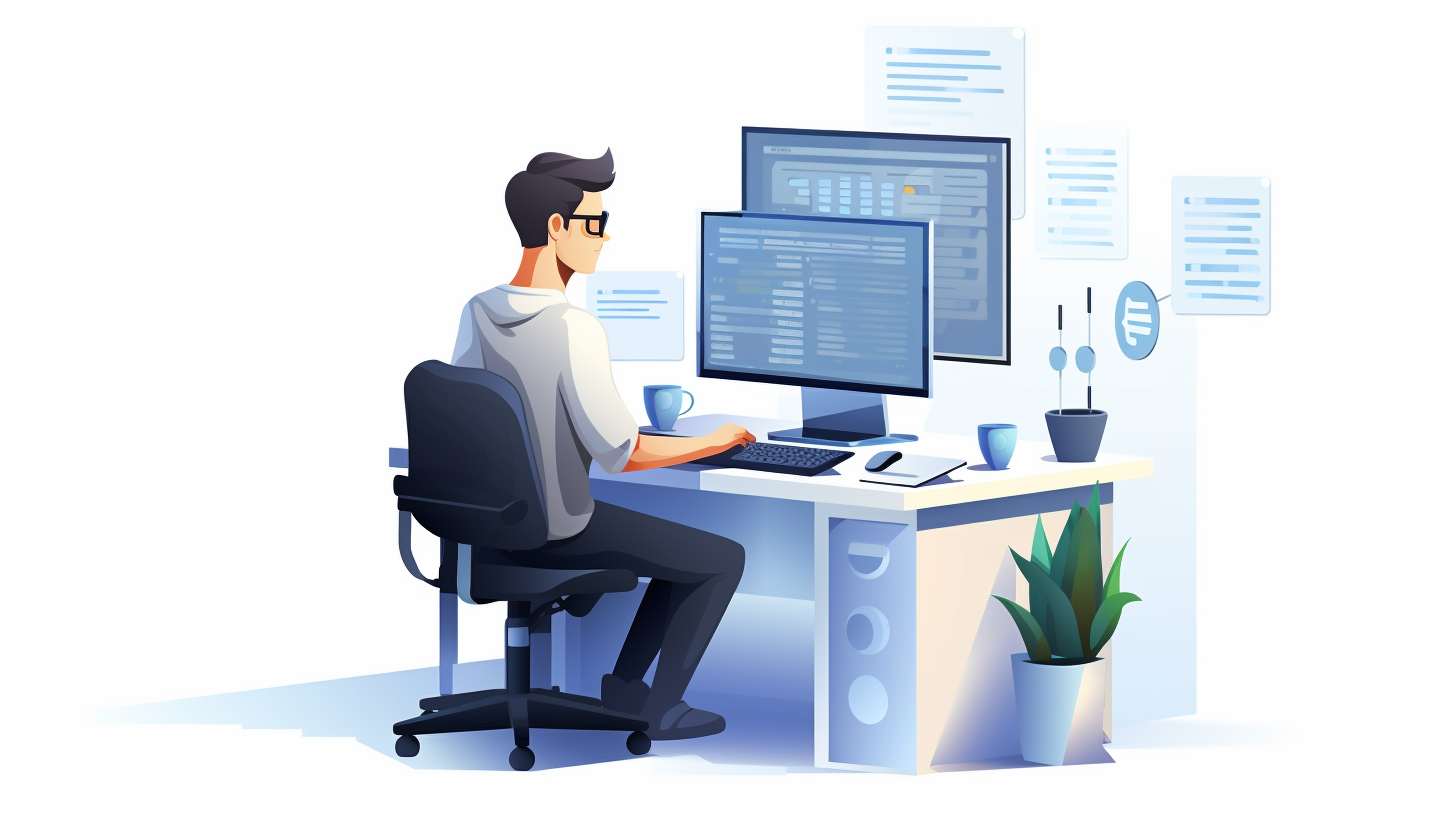
Interactive Database Access with Bash
When delving into the realm of database connectivity using Bash, it is vital to grasp the underlying principles that allow Bash to interact seamlessly with databases. While Bash is primarily a shell scripting language, its utility extends far beyond simple file manipulation or shell command execution. It can be effectively employed to create scripts that communicate with databases, making it a powerful tool for automation and data management.
At its core, Bash interfaces with databases through command-line utilities and libraries tailored for various database systems. For instance, if you’re working with MySQL, you would typically employ the mysql
command-line client, while PostgreSQL users would rely on psql
. Understanding how these tools operate especially important, as they serve as the bridge between your Bash scripts and the database.
Each database system has its unique connection string format and authentication requirements. In general, you’ll need to provide details including the database host, port, username, password, and the specific database you wish to access. Here’s a basic structure of how you might connect to a MySQL database:
mysql -h hostname -u username -p database_name
In this command, replace hostname
with your database server’s address, username
with your database username, and database_name
with the name of your target database. Upon execution, you will be prompted for your password.
Similarly, connecting to a PostgreSQL database can be accomplished with the following command:
psql -h hostname -U username -d database_name
When it comes to databases, understanding and managing connections efficiently is paramount. You might want to explore storing credentials securely, using environment variables or configuration files, to prevent exposure in your scripts. Here’s a simple example of using environment variables:
export DB_HOST="hostname" export DB_USER="username" export DB_PASS="password" export DB_NAME="database_name" mysql -h $DB_HOST -u $DB_USER -p$DB_PASS $DB_NAME
This approach enhances security and simplifies your scripts, allowing for a cleaner, more maintainable codebase.
Once a connection is established, you can begin executing queries. But before diving into that, it’s worth noting the differences in the interaction styles across various databases. For example, while MySQL utilizes a fairly simpler syntax, PostgreSQL offers advanced features like window functions and common table expressions that can significantly enhance your querying capabilities.
Understanding the fundamentals of Bash database connectivity equips you with the knowledge to harness the full potential of your shell scripts for complex database operations. By mastering connections, authentication, and query execution, you pave the way for more efficient data manipulation and automation.
Setting Up a Database Connection in Bash
When setting up a database connection in Bash, it’s not only about using the appropriate command-line utility but also about establishing a reliable and efficient method to manage that connection. Typically, the first step is to ensure that you have the necessary client tools installed on your system. For MySQL, you would need the ‘mysql-client’ package, while for PostgreSQL, the ‘postgresql-client’ is essential.
Once the client is installed, the next step involves crafting your connection command. It’s prudent to encapsulate this within a function to streamline your connection process. Here’s how you might structure a reusable connection function for MySQL:
function connect_mysql() { local host="$1" local user="$2" local password="$3" local dbname="$4" mysql -h "$host" -u "$user" -p"$password" "$dbname" }
This function takes four parameters: host, user, password, and database name. By encapsulating the connection logic within a function, you can easily reuse it throughout your script, promoting DRY (Don’t Repeat Yourself) principles.
For PostgreSQL, you can create a similar function:
function connect_postgresql() { local host="$1" local user="$2" local dbname="$3" PGPASSWORD="$4" psql -h "$host" -U "$user" -d "$dbname" }
In this case, the password is set using the environment variable PGPASSWORD, which is a handy trick to avoid having the password prompt during execution. This convenience is particularly beneficial in automated scripts.
To further enhance the security of your database connections, think implementing error handling to manage any connection failures. This can be achieved by checking the exit status of the command immediately after trying to connect. Here’s an example of how to do this for the MySQL connection:
if ! connect_mysql "$DB_HOST" "$DB_USER" "$DB_PASS" "$DB_NAME"; then echo "Failed to connect to MySQL database." exit 1 fi
This snippet attempts to connect to the MySQL database using the previously defined function. If it fails, it produces an error message and exits the script with a non-zero status, which is a standard way in Unix-like systems to indicate an error.
For PostgreSQL connections, you can apply a similar error handling approach:
if ! connect_postgresql "$DB_HOST" "$DB_USER" "$DB_NAME" "$DB_PASS"; then echo "Failed to connect to PostgreSQL database." exit 1 fi
By incorporating these connection functions and error handling mechanisms, you can significantly streamline your database interaction processes within Bash scripts. This setup not only enhances code readability but also provides a solid foundation for building more complex database operations as you move forward.
Executing Queries and Fetching Results
Once you have established a connection to your database, the next step is executing queries and fetching results. This process can be as simple as running a basic SELECT statement or as complex as executing stored procedures and managing transactions. Understanding how to effectively execute queries in Bash is fundamental for any script that deals with database operations.
In Bash, executing a query can typically be done by passing the SQL command as an argument to the database command-line utility. For example, to execute a simple SELECT query in MySQL, you would use the following command:
mysql -h "$DB_HOST" -u "$DB_USER" -p"$DB_PASS" -D "$DB_NAME" -e "SELECT * FROM your_table;"
This command connects to the MySQL database and executes the specified SQL query. The `-e` option allows you to run the command directly from the command line. Note that `your_table` should be replaced with the actual table name from which you wish to retrieve data.
Similarly, for PostgreSQL, executing a query can be accomplished as follows:
psql -h "$DB_HOST" -U "$DB_USER" -d "$DB_NAME" -c "SELECT * FROM your_table;"
Here, the `-c` option serves a similar purpose to MySQL’s `-e`, which will allow you to run the SQL command directly in the terminal.
Fetching results and processing them is where Bash scripting shines. To capture the output of your query for further manipulation, you can use command substitution. For example:
results=$(mysql -h "$DB_HOST" -u "$DB_USER" -p"$DB_PASS" -D "$DB_NAME" -e "SELECT * FROM your_table;")
This command stores the results of the query into the `results` variable. You can then process this variable as needed, such as parsing the output line by line or filtering specific fields.
For PostgreSQL, you can similarly capture the output:
results=$(psql -h "$DB_HOST" -U "$DB_USER" -d "$DB_NAME" -c "SELECT * FROM your_table;" --no-align --tuples-only)
The `–no-align` and `–tuples-only` options in this command provide cleaner output by removing additional formatting, making it easier for further manipulation.
To loop through the results and process each row, you can utilize a while-read loop. Here’s an example for MySQL:
echo "$results" | while IFS=$'t' read -r column1 column2 column3; do echo "Column 1: $column1, Column 2: $column2, Column 3: $column3" done
In this snippet, we use `IFS=$’t’` to specify the tab character as the delimiter, making it suitable for parsing the output. The loop reads each row of data into variables that can be easily used within the loop body.
For PostgreSQL, you can apply the same concept:
echo "$results" | while IFS='|' read -r column1 column2 column3; do echo "Column 1: $column1, Column 2: $column2, Column 3: $column3" done
As you can see, the principle remains the same; you just need to adjust the delimiter to match the format of the output. Additionally, you can further enhance your scripts by implementing conditionals and error checks to ensure robust handling of the data.
Executing queries and fetching results in Bash requires a solid understanding of both SQL syntax and how to properly interface with command-line database tools. With the ability to capture and process results, you can build powerful scripts that automate complex workflows, paving the way for efficient data handling and manipulation directly from your shell.
Error Handling and Debugging Techniques in Bash Database Scripts
When diving into database scripting with Bash, error handling and debugging are critical components that can make or break the reliability of your scripts. Without appropriate error handling, your scripts may fail silently or produce erroneous results, leading to data corruption or loss. This section will explore various techniques for error handling and debugging in Bash as they pertain to database interactions.
In Bash, you can check the exit status of the last executed command using the special variable $?
. A value of 0
indicates success, while any non-zero value signifies an error. Employing this strategy is essential after executing database commands to determine whether they succeeded or failed. For example, if you execute a query, you can immediately check the exit status:
mysql -h "$DB_HOST" -u "$DB_USER" -p"$DB_PASS" -D "$DB_NAME" -e "SELECT * FROM your_table;" if [ $? -ne 0 ]; then echo "Query execution failed." exit 1 fi
This snippet will echo a failure message and exit if the query execution fails, which will allow you to prevent further actions based on an invalid state.
Another effective method for error handling is to utilize functions that wrap your database calls. By doing so, you can centralize the error checking and handle specific actions for different error types. Here’s how you might structure such a function for MySQL:
function execute_query() { local query="$1" mysql -h "$DB_HOST" -u "$DB_USER" -p"$DB_PASS" -D "$DB_NAME" -e "$query" if [ $? -ne 0 ]; then echo "Error executing query: $query" exit 1 fi }
Using this function, you can execute queries while ensuring that any failures are captured and reported. Each time you need to run a query, simply call:
execute_query "SELECT * FROM your_table;"
When it comes to debugging, incorporating verbose output can significantly aid in tracking the flow of your script. The set -x
command can be used at the start of your script or function to enable debugging mode, which prints each command before execution. This can help you identify where things might be going wrong:
set -x execute_query "SELECT * FROM your_table;" set +x # Disable debugging
Additionally, you can log errors to a file for later inspection. Redirecting error output to a log file can help you keep track of issues without cluttering your terminal output. Here’s an example of how to do this:
execute_query "SELECT * FROM your_table;" 2>>error.log
This command appends any error messages to error.log
, which will allow you to review them later. Combining this with timestamped logs can make it easier to pinpoint when issues occurred.
Moreover, you can implement retry logic for transient errors, such as temporary network issues. A simple retry mechanism could look like this:
function execute_with_retry() { local query="$1" local retries=3 local count=0 until mysql -h "$DB_HOST" -u "$DB_USER" -p"$DB_PASS" -D "$DB_NAME" -e "$query"; do count=$((count + 1)) if [ $count -eq $retries ]; then echo "Query failed after $retries attempts." exit 1 fi echo "Retrying... ($count)" sleep 1 # Wait before retrying done }
This function attempts to execute the query and will retry up to three times if it encounters a failure. This can be particularly useful for improving the robustness of your scripts when dealing with intermittent connectivity issues.
Effective error handling and debugging practices are essential for successful Bash database scripts. By checking exit statuses, centralizing error handling in functions, enabling verbose debugging, logging errors, and implementing retry logic, you can create robust scripts that gracefully handle failures and provide clear feedback for troubleshooting.