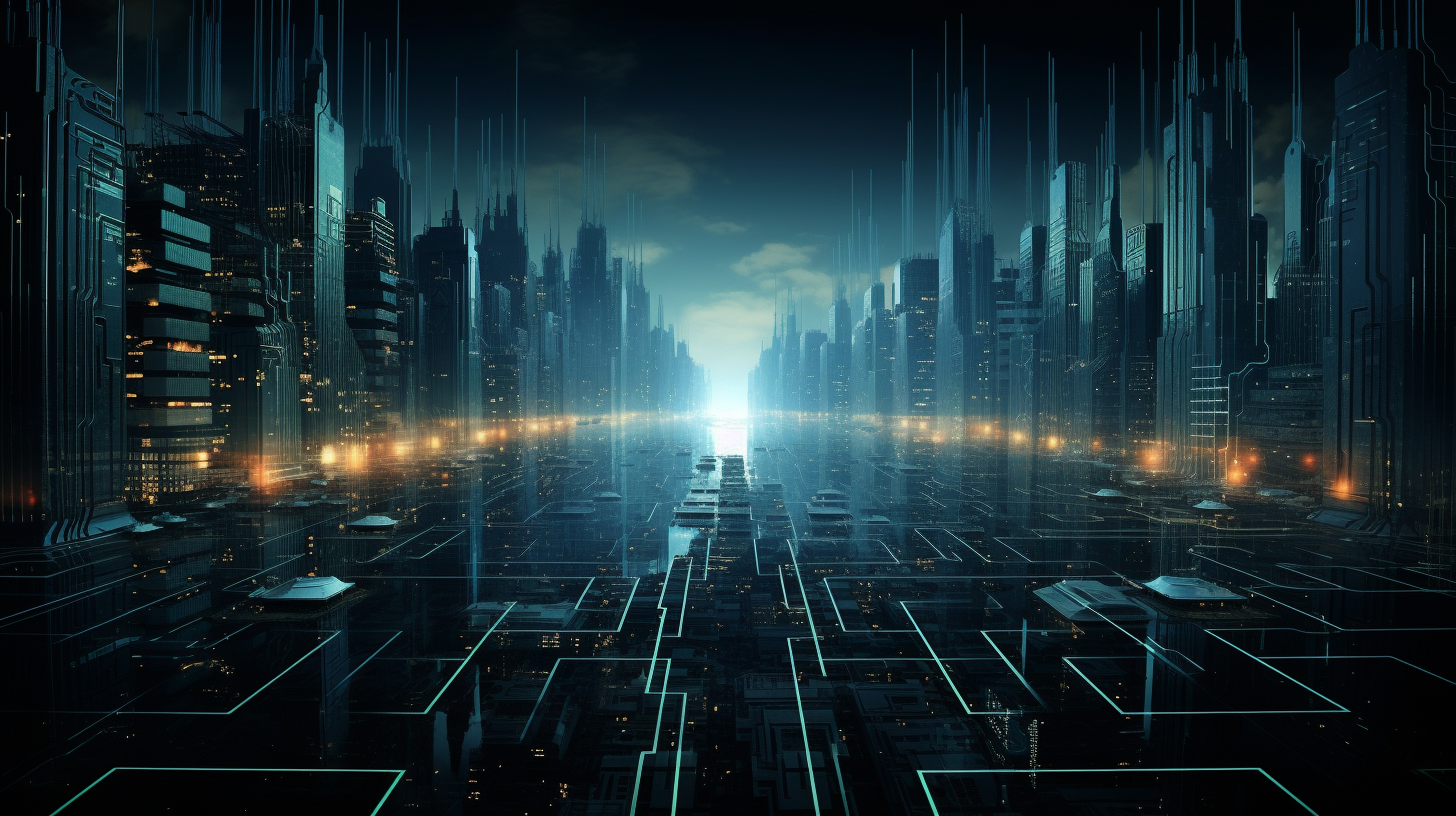
Introduction to Swift Programming
Swift, a language designed for safety and performance, boasts a clear and expressive syntax that makes it approachable for beginners while offering powerful features for seasoned developers. Understanding the basic syntax and structure is important for writing effective Swift code.
At its core, Swift code is comprised of statements and expressions, and it uses a combination of keywords, operators, and punctuation to determine the flow of execution. One key aspect of Swift syntax is its reliance on type inference, which allows the compiler to deduce the type of a variable based on its initial value. This leads to cleaner and more readable code.
For instance, think the following example, where we declare a variable without explicitly specifying its type:
let greeting = "Hello, Swift!"
In the above snippet, the variable greeting
is inferred to be of type String
because it is initialized with a string literal. This feature reduces the need for verbose type declarations, allowing developers to focus on the logic of their programs.
Swift also employs a range of punctuation and symbols to create a clear structure. Curly braces {}
define code blocks, while parentheses ()
are used in function declarations and calls. For example:
func sayHello() { print(greeting) } sayHello()
In this case, the function sayHello
encapsulates a block of code that prints a message. The structure is simpler and visually distinct, rendering it effortless to follow.
Another significant element of Swift’s syntax is the use of optional types, which serve to indicate the absence of a value. This is a powerful feature for handling nullability, allowing developers to write safer code. Here’s how you can declare an optional:
var optionalGreeting: String? = nil
In this example, optionalGreeting
is declared as an optional string, which means it can either hold a string value or be nil
. This helps prevent runtime crashes due to unexpected null values.
Swift syntax also promotes readability by enabling developers to use descriptive names for functions and variables. This practice aids in understanding the purpose of various components in the code. For example, using calculateAreaOfRectangle
as a function name immediately conveys its functionality:
func calculateAreaOfRectangle(length: Double, width: Double) -> Double { return length * width }
Swift’s syntax and structure emphasize clarity, safety, and expressiveness. By using type inference, optional types, and clear naming conventions, Swift enables developers to write robust code this is easy to read and maintain.
Data Types and Variables in Swift
Understanding data types and variables in Swift is fundamental to creating effective applications. Swift is a type-safe language, meaning that it enforces strict rules on how different types of data are handled. This design choice minimizes the risks associated with type errors, making Swift a preferred choice for developers who prioritize safety and reliability.
Swift provides a variety of built-in data types that cater to different kinds of data. The most commonly used data types include:
- Represents integer values.
- Represents floating-point numbers with double precision.
- Represents a sequence of characters.
- Represents Boolean values (true or false).
- Represents a collection of values of the same type.
- Represents a collection of key-value pairs.
When declaring variables in Swift, you have the option to use either let or var. The let keyword is used for constants, which cannot be changed once assigned, while var is used for variables, which can be modified. This distinction is useful for avoiding accidental changes to values that should remain constant.
let pi = 3.14159 // A constant value var radius = 5.0 // A variable value
In the example above, pi is defined as a constant, meaning its value cannot be altered later in the program. On the other hand, radius is a variable, and its value can be changed as needed.
Swift also supports type annotations, which allow you to explicitly specify the type of a variable or constant when it’s declared. This can enhance code readability and provide additional context for other developers. For instance:
var temperature: Double = 36.6 // Explicitly declared as Double let name: String = "Swift Programming"
Moreover, Swift provides a robust way to work with collections. Arrays and dictionaries are two primary collection types that allow you to group and manage multiple values. For example, to create an array of integers:
var numbers: [Int] = [1, 2, 3, 4, 5]
In this snippet, numbers is an array that stores a collection of integer values. You can access elements using their index, modify the array, and leverage various built-in methods to perform operations on collections.
Dictionaries allow you to store data in a key-value format, rendering it effortless to retrieve values based on unique keys. For example:
var person: [String: String] = ["name": "Alice", "age": "30"]
This dictionary stores a person’s name and age, which can be accessed using their respective keys. Swift’s syntax for declaring dictionaries is simpler, promoting clarity.
Swift’s handling of data types and variables is designed not only for safety but also for flexibility. With features like type inference, optionals, and the ability to create custom data types, Swift empowers developers to craft sophisticated applications without compromising on readability or maintainability.
Control Flow and Functions
Control flow is an essential aspect of programming, as it dictates how a program responds to different conditions and executes code accordingly. In Swift, control flow statements such as if, switch, for-in, while, and repeat-while allow developers to implement complex logic and handle different scenarios in a clean and organized manner.
One of the simplest control flow constructs in Swift is the if
statement. This statement allows you to execute a block of code based on a condition. For example:
let score = 85 if score >= 90 { print("You got an A!") } else if score >= 80 { print("You got a B!") } else { print("You need to study harder.") }
In this example, the program checks the value of score
and prints a message based on the grade threshold. This structure is not only simpler but also allows for easy expansion with additional conditions.
Another powerful control flow tool in Swift is the switch
statement. Unlike traditional switch statements that often rely on integer values, Swift’s switch
can evaluate a variety of types, including strings and tuples. Here’s an example:
let day = "Monday" switch day { case "Monday": print("Start of the work week.") case "Friday": print("Almost the weekend!") case "Saturday", "Sunday": print("It's the weekend!") default: print("Midweek days are busy.") }
This snippet showcases how the switch
statement can match multiple cases and execute corresponding code. The use of default
provides a catch-all for any values that don’t meet the specified cases, enhancing the robustness of the control flow.
Loops are another integral part of control flow, allowing code to be executed repeatedly based on a condition. Swift offers several types of loops, including for-in
, while
, and repeat-while
. The for-in
loop is particularly useful for iterating over collections, such as arrays or ranges:
let fruits = ["Apple", "Banana", "Cherry"] for fruit in fruits { print(fruit) }
In this example, the for-in
loop iterates through each element in the fruits
array and prints it. The syntax is clean, making it easy to understand and maintain.
The while
loop, in contrast, continues to execute as long as a specified condition is true:
var counter = 0 while counter < 5 { print("Counter is (counter)") counter += 1 }
In this case, the loop prints the value of counter
until it reaches 5. This construct is useful for scenarios where the number of iterations is not known in advance.
Swift also provides a repeat-while
loop, which guarantees that the code block is executed at least once before the condition is tested:
var number = 0 repeat { print("Number is (number)") number += 1 } while number < 3
This structure is beneficial in cases where the initial execution of the loop body is necessary, regardless of the condition.
Functions play a pivotal role in organizing code and enhancing reusability. In Swift, functions can be defined using the func
keyword, followed by a name, parameters, and a return type:
func addNumbers(a: Int, b: Int) -> Int { return a + b }
This function, addNumbers
, takes two integer parameters, adds them, and returns the result. Functions can also provide default parameter values, allowing for more flexible calling:
func greet(name: String, greeting: String = "Hello") { print("(greeting), (name)!") } greet(name: "Alice") // Uses default greeting greet(name: "Bob", greeting: "Welcome") // Custom greeting
Here, the function greet
uses a default value for greeting
. This feature simplifies function calls when the default behavior is desired while allowing customization when necessary.
Swift’s control flow and functions provide a powerful and expressive way to manage the logic of your applications. By using these constructs, developers can create clear, efficient, and maintainable code that effectively responds to various conditions and organizes behavior through reusable functions.
Object-Oriented Programming in Swift
Object-oriented programming (OOP) is a paradigm that enables developers to structure their code in a way that models real-world entities and relationships. Swift embraces OOP principles, allowing developers to create classes, manage inheritance, and implement encapsulation effectively. At the heart of OOP in Swift is the class, which serves as a blueprint for creating objects.
Defining a class in Swift is simpler. Here’s a simple example of a class that represents a `Car`:
class Car { var make: String var model: String var year: Int init(make: String, model: String, year: Int) { self.make = make self.model = model self.year = year } func displayInfo() { print("Car: (year) (make) (model)") } }
In this example, the `Car` class contains three properties: `make`, `model`, and `year`. The `init` method initializes these properties when a new `Car` object is created. The `displayInfo` method encapsulates the behavior of our `Car` class, enabling it to print its details.
Creating instances of a class in Swift is as simple as calling the initializer:
let myCar = Car(make: "Toyota", model: "Camry", year: 2020) myCar.displayInfo() // Output: Car: 2020 Toyota Camry
Inheritance allows one class to inherit properties and methods from another class, promoting code reuse. In Swift, you can create a subclass by using a colon to specify the superclass:
class ElectricCar: Car { var batteryLife: Int init(make: String, model: String, year: Int, batteryLife: Int) { self.batteryLife = batteryLife super.init(make: make, model: model, year: year) } override func displayInfo() { super.displayInfo() print("Battery life: (batteryLife)%") } }
In this case, `ElectricCar` inherits from `Car`. It adds a new property called `batteryLife` and overrides the `displayInfo` method to provide additional information. This demonstrates how Swift allows for extending the functionality of existing classes seamlessly.
Encapsulation, another key principle of OOP, involves restricting access to certain components of an object. Swift uses access control levels to manage visibility. You can specify whether a property or method is `public`, `internal`, `fileprivate`, or `private`. Here’s an example:
class BankAccount { private var balance: Double = 0.0 func deposit(amount: Double) { balance += amount } func withdraw(amount: Double) -> Bool { if amount Double { return balance } }
In the `BankAccount` class, the `balance` property is marked as `private`, meaning it cannot be accessed directly from outside the class. Instead, methods are provided to interact with the balance safely, illustrating how encapsulation helps protect state and maintain invariants.
Swift also supports protocols, which define a blueprint of methods, properties, and other requirements that suit a particular task or piece of functionality. Classes can adopt and conform to protocols, providing flexibility and enabling polymorphism. Here’s an example of a protocol and a class that conforms to it:
protocol Drivable { func drive() } class Motorcycle: Drivable { func drive() { print("The motorcycle is driving.") } }
In this code, `Drivable` is a protocol that requires any conforming class to implement the `drive` method. The `Motorcycle` class adopts this protocol and provides its implementation, allowing for a common interface that multiple vehicles can share.
Swift’s object-oriented programming features, including classes, inheritance, encapsulation, and protocols, empower developers to create organized, modular, and reusable code. By modeling real-world entities in a structured way, Swift enables powerful abstractions that lead to more maintainable applications.