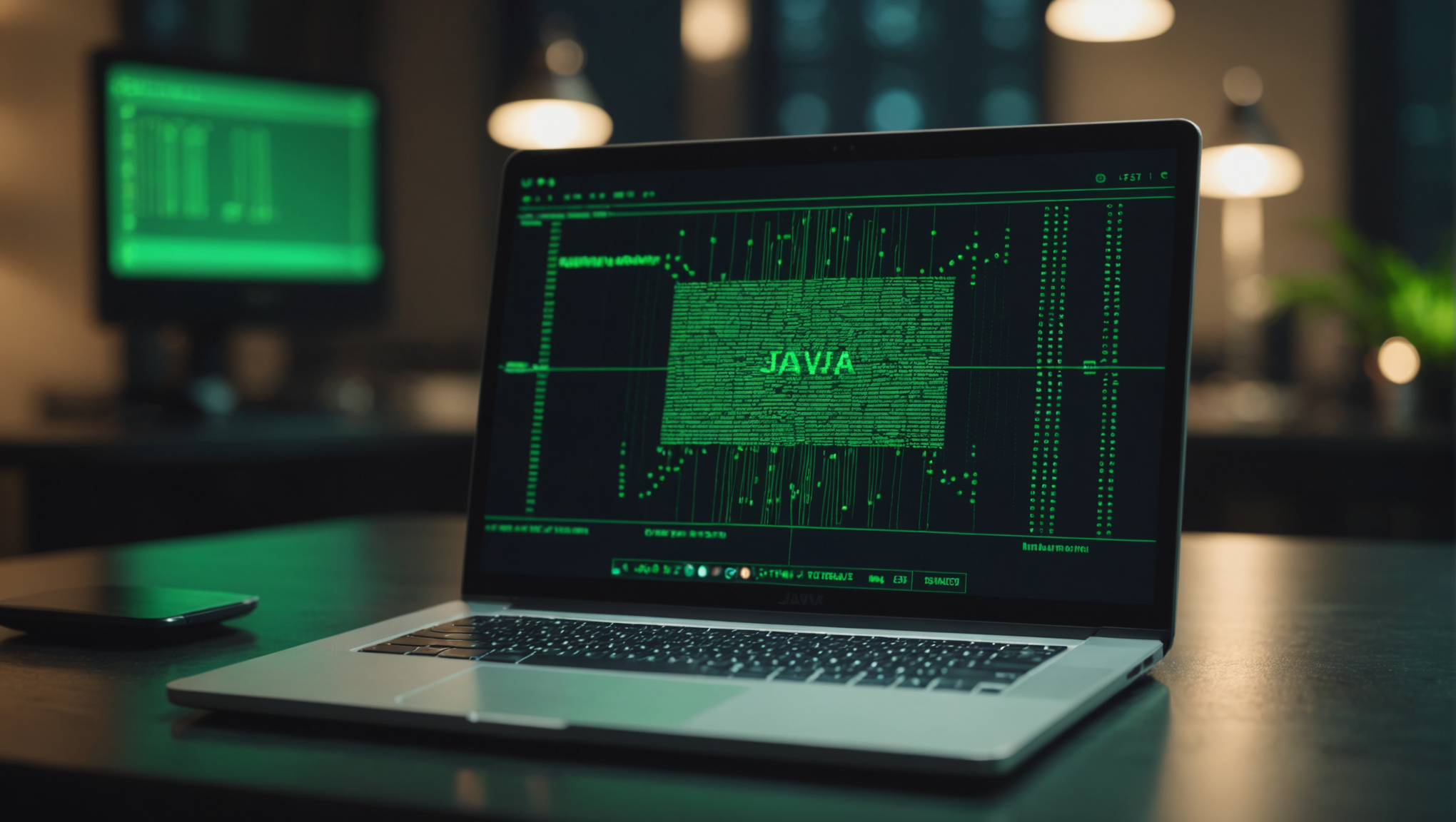
Java and Artificial Intelligence: Libraries and Frameworks
Java has established itself as a prominent language in the realm of Artificial Intelligence (AI) due to its platform independence, object-oriented structure, and robust performance. With the rapid advancement of AI technologies, Java continues to provide a solid foundation for developing AI applications that are scalable and maintainable.
One of the core strengths of Java is its extensive ecosystem of libraries and tools that facilitate the integration of AI functionalities. Java’s ability to manage memory efficiently and its built-in garbage collection contribute to the stability necessary for handling complex AI algorithms. Moreover, Java’s multi-threading capabilities allow developers to perform concurrent operations, which is essential in tasks like data processing and model training.
Java’s versatility is further enhanced by its interplay with distributed computing frameworks such as Apache Hadoop and Apache Spark. This ability to process large datasets efficiently is vital for training machine learning models and executing AI tasks that require significant computational resources.
Furthermore, Java’s community support is immense, providing access to a wealth of resources, tutorials, and forums. This collaborative environment fosters innovation and helps developers tackle challenges associated with AI development.
When it comes to implementing machine learning algorithms, Java offers libraries like Weka, Deeplearning4j, and MOA, which simplify the process of building and training models. These libraries are crafted to serve a wide array of AI applications, from predictive analytics to complex neural networks.
Here is an example of how to use Weka for a simple machine learning task, such as classification:
import weka.classifiers.Classifier; import weka.classifiers.trees.J48; import weka.core.Instances; import weka.core.converters.ConverterUtils; public class WekaExample { public static void main(String[] args) throws Exception { // Load dataset ConverterUtils.DataSource source = new ConverterUtils.DataSource("data/your_dataset.arff"); Instances data = source.getDataSet(); // Setting class attribute if (data.classIndex() == -1) data.setClassIndex(data.numAttributes() - 1); // Build classifier Classifier classifier = new J48(); // Using decision tree classifier.buildClassifier(data); // Output the classifier System.out.println(classifier); } }
This example illustrates the simpler process of loading a dataset, setting the class label, training a classifier, and then printing the classifier’s structure. Such simplicity allows developers to focus on solving AI problems rather than getting bogged down by complicated code.
Moreover, Java’s security features, like the extensive API for cryptography, are invaluable in developing AI applications that deal with sensitive data. That is particularly important in areas such as healthcare and finance, where data integrity and privacy are paramount.
Ultimately, Java’s role in the AI landscape is characterized by its adaptability and the breadth of tools it offers. As AI technologies evolve, Java continues to be a reliable choice for developers looking to harness the power of artificial intelligence in their applications.
Frameworks for Natural Language Processing in Java
In the realm of Natural Language Processing (NLP), Java offers a variety of frameworks that empower developers to build sophisticated applications capable of understanding and interpreting human language. These frameworks provide essential tools for tasks such as text analysis, sentiment detection, and language translation. Below are some of the prominent frameworks that have made significant contributions to NLP in Java.
Apache OpenNLP is one of the most widely used Java libraries for NLP tasks. It supports various functions such as tokenization, part-of-speech tagging, named entity recognition, and parsing. OpenNLP is designed to be uncomplicated to manage and integrates seamlessly into Java applications. Its model training capabilities allow developers to create custom models tailored to specific tasks.
import opennlp.tools.cmdline.perc.PerceptronModelLoader; import opennlp.tools.sentdetect.SentenceModel; import opennlp.tools.sentdetect.SentenceDetectorME; public class OpenNLPExample { public static void main(String[] args) throws Exception { // Load the sentence model SentenceModel model = new SentenceModel(new FileInputStream("en-sent.bin")); SentenceDetectorME sentenceDetector = new SentenceDetectorME(model); String paragraph = "Hello there! How are you doing today? This is an example of sentence detection."; String[] sentences = sentenceDetector.sentDetect(paragraph); // Output the detected sentences for (String sentence : sentences) { System.out.println(sentence); } } }
This example demonstrates how to use OpenNLP for sentence detection. After loading the appropriate model, the `SentenceDetectorME` class is used to detect and output individual sentences from a paragraph, showcasing how simpler it’s to implement NLP functionalities using this framework.
Stanford CoreNLP is another powerful toolkit that provides a range of NLP tools. It includes features for tokenization, named entity recognition, and sentiment analysis. CoreNLP is built with a focus on deep learning and offers capabilities that facilitate the integration of neural network models into NLP tasks. The framework also supports various languages, which broadens its applicability across different linguistic contexts.
import edu.stanford.nlp.pipeline.*; public class StanfordCoreNLPExample { public static void main(String[] args) { // Set up pipeline properties Properties props = new Properties(); props.setProperty("annotators", "tokenize,ssplit,pos,lemma,ner"); props.setProperty("outputFormat", "text"); // Build pipeline StanfordCoreNLP pipeline = new StanfordCoreNLP(props); // Create an empty Annotation just with the given text String text = "Barack Obama was born in Hawaii. He was elected president in 2008."; Annotation document = new Annotation(text); // Annotate the document pipeline.annotate(document); // Output the results System.out.println(document.toString()); } }
This snippet illustrates how to set up a Stanford CoreNLP pipeline and annotate a text for various NLP tasks. By specifying the desired annotators in the properties, developers can efficiently process text and extract valuable information.
Apache Lucene is not strictly an NLP framework, but it plays an important role in text indexing and search functionality, which are essential components of many NLP applications. Lucene allows for efficient full-text searches, and it provides features like tokenization and stemming, which are integral to processing and analyzing textual data.
import org.apache.lucene.analysis.standard.StandardAnalyzer; import org.apache.lucene.index.IndexWriter; import org.apache.lucene.index.IndexWriterConfig; import org.apache.lucene.store.Directory; import org.apache.lucene.store.RAMDirectory; public class LuceneExample { public static void main(String[] args) throws Exception { // Create a RAMDirectory to hold the index Directory directory = new RAMDirectory(); StandardAnalyzer analyzer = new StandardAnalyzer(); // Setup the IndexWriter IndexWriterConfig config = new IndexWriterConfig(analyzer); IndexWriter writer = new IndexWriter(directory, config); // Add documents to the index (not shown here) // Close the writer writer.close(); } }
This example demonstrates how to set up an index using Apache Lucene. Although it does not include document addition, it shows the fundamental steps to create an index, which is essential for building search functionalities in NLP applications.
The integration of these frameworks into Java applications highlights the language’s capability to handle complex NLP tasks efficiently. By using these tools, developers can create applications that not only process text but also glean insights from it, paving the way for more intuitive user interactions with technology.
Java Tools for Neural Networks and Deep Learning
Java provides a robust infrastructure for developing neural networks and deep learning applications, with several libraries and tools designed specifically for these tasks. Among the most notable are Deeplearning4j, DL4J, and Neuroph, each offering unique features that cater to different aspects of neural network implementation. Deeplearning4j (DL4J) is an open-source, distributed deep learning library designed to work on the Java Virtual Machine (JVM). It supports both CPU and GPU computations, making it suitable for large-scale neural network training. One of the key features of DL4J is its integration with Hadoop and Spark, enabling efficient processing of big data. DL4J also provides a rich set of pre-built neural network architectures, making it easier to implement complex models. Here’s a simple example of using Deeplearning4j to create and train a multi-layer perceptron (MLP) for a classification task: import org.deeplearning4j.nn.conf.MultiLayerConfiguration; import org.deeplearning4j.nn.conf.NeuralNetConfiguration; import org.deeplearning4j.nn.multilayer.MultiLayerNetwork; import org.deeplearning4j.optimize.listeners.ScoreIterationListener; import org.nd4j.linalg.activations.Activation; import org.nd4j.linalg.dataset.api.iterator.DataSetIterator; import org.nd4j.linalg.dataset.api.iterator.IrisDataSetIterator; import org.nd4j.linalg.lossfunctions.LossFunctions; public class DL4JExample { public static void main(String[] args) { // Load the Iris dataset DataSetIterator irisIterator = new IrisDataSetIterator(150, 150); // Configure the neural network MultiLayerConfiguration configuration = new NeuralNetConfiguration.Builder() .seed(12345) .updater(new Adam(0.01)) .list() .layer(0, new DenseLayer.Builder().nIn(4).nOut(10).activation(Activation.RELU).build()) .layer(1, new OutputLayer.Builder(LossFunctions.LossFunction.NEGATIVELOGLIKELIHOOD) .activation(Activation.SOFTMAX) .nIn(10).nOut(3).build()) .build(); // Create and initialize the network MultiLayerNetwork model = new MultiLayerNetwork(configuration); model.init(); model.setListeners(new ScoreIterationListener(100)); // Train the model model.fit(irisIterator); } } In the above example, we create a simple feedforward neural network with one hidden layer to classify iris species based on their features. The model is configured with an Adam optimizer and uses a softmax activation function for the output layer. This simplicity allows developers to focus on the architecture and training data rather than the underlying intricacies of neural network development. Neuroph is another popular Java framework, which is lightweight and simple to operate, making it ideal for beginners. It provides a simple API for creating and training neural networks and supports different types of networks including MLPs and convolutional networks. Neuroph also has a built-in GUI for designing neural network architectures visually. Here's a quick example of creating and training a simple neural network using Neuroph: import org.neuroph.core.NeuralNetwork; import org.neuroph.core.data.DataSet; import org.neuroph.core.data.DataSetRow; import org.neuroph.nnet.MultiLayerPerceptron; import org.neuroph.nnet.learning.LearningRule; import org.neuroph.nnet.learning.TrainingEvent; import org.neuroph.nnet.learning.TrainingEventListener; import org.neuroph.nnet.learning.TrainingEventType; public class NeurophExample { public static void main(String[] args) { // Create a dataset for XOR problem DataSet dataSet = new DataSet(2, 1); dataSet.add(new DataSetRow(new double[]{0, 0}, new double[]{0})); dataSet.add(new DataSetRow(new double[]{0, 1}, new double[]{1})); dataSet.add(new DataSetRow(new double[]{1, 0}, new double[]{1})); dataSet.add(new DataSetRow(new double[]{1, 1}, new double[]{0})); // Create the neural network MultiLayerPerceptron neuralNet = new MultiLayerPerceptron(2, 4, 1); // Train the neural network neuralNet.learn(dataSet); // Test the trained network neuralNet.setInput(0, 1); neuralNet.calculate(); System.out.println("Output for (0, 1): " + neuralNet.getOutput()[0]); } } In this example, we tackle the XOR problem using a multi-layer perceptron. The dataset is created manually, and the network is trained accordingly. After training, the network can be tested with the input (0, 1), providing an intuitive understanding of its output. Java’s strong type system and multi-threading capabilities make it an excellent choice for building complex deep learning models. The interoperability with other technologies and frameworks, such as TensorFlow and Keras through the Java API, further expands the possibilities for Java developers venturing into deep learning. To wrap it up, the availability of powerful tools and libraries for neural networks and deep learning in Java enhances its credibility as a viable language for AI development. By providing developers with the necessary infrastructure to implement and experiment with sophisticated neural architectures, Java paves the way for innovative AI solutions.
Integrating Java with Other AI Technologies
As the field of Artificial Intelligence continues to evolve, the ability to integrate Java with other AI technologies has become a critical aspect of crafting sophisticated AI applications. Java’s robust architecture allows it to seamlessly interact with various other programming languages and frameworks, enabling developers to leverage the strengths of multiple technologies in their projects.
One of the most notable integrations is with Python, a language that has gained immense popularity in the AI and machine learning community. Many machine learning frameworks and libraries, such as TensorFlow and PyTorch, are primarily developed in Python. However, Java developers can utilize the Java Native Interface (JNI) to call Python code directly from Java. This interaction allows Java applications to harness the extensive capabilities of Python libraries without compromising on the performance and stability that Java offers.
import org.python.util.PythonInterpreter; public class PythonIntegrationExample { public static void main(String[] args) { try (PythonInterpreter python = new PythonInterpreter()) { // Execute a Python script python.exec("import numpy as np"); python.exec("a = np.array([1, 2, 3])"); python.exec("print(a)"); // Call a Python function python.exec("def sum_array(arr): return np.sum(arr)"); python.set("arr", new PyArray(new double[]{1, 2, 3})); python.exec("result = sum_array(arr)"); PyInteger result = python.get("result"); System.out.println("Sum: " + result); } } }
This example demonstrates how to use the Python Interpreter from within Java to execute Python code. By doing so, Java applications can utilize Python’s powerful libraries for data manipulation and machine learning, while maintaining Java’s robust architecture.
Another common integration involves using web services, where Java applications can communicate with AI services hosted on cloud platforms such as AWS, Google Cloud, or Azure. These platforms provide APIs for various AI functionalities, including image recognition, natural language processing, and machine learning model hosting. Java’s comprehensive support for RESTful web services makes it easy to send and receive data from these services.
import java.net.HttpURLConnection; import java.net.URL; import java.io.OutputStream; public class WebServiceIntegrationExample { public static void main(String[] args) throws Exception { String url = "https://api.example.com/predict"; URL obj = new URL(url); HttpURLConnection connection = (HttpURLConnection) obj.openConnection(); connection.setRequestMethod("POST"); connection.setRequestProperty("Content-Type", "application/json"); connection.setDoOutput(true); String jsonInputString = "{"data": [1, 2, 3]}"; try (OutputStream os = connection.getOutputStream()) { byte[] input = jsonInputString.getBytes("utf-8"); os.write(input, 0, input.length); } // Handle the response (not shown here) } }
In this code snippet, we illustrate how to send a JSON payload to a web service endpoint using Java’s HttpURLConnection. That’s particularly useful for integrating AI functionalities from cloud-based services into Java applications, enabling rapid development and deployment of AI solutions.
Moreover, Java’s compatibility with message brokers like Apache Kafka and RabbitMQ opens up additional avenues for integration. By using these systems, Java applications can process and analyze data streams in real-time, which is especially valuable in applications requiring immediate insights, such as fraud detection and recommendation systems.
import org.apache.kafka.clients.producer.KafkaProducer; import org.apache.kafka.clients.producer.ProducerRecord; import java.util.Properties; public class KafkaIntegrationExample { public static void main(String[] args) { Properties props = new Properties(); props.put("bootstrap.servers", "localhost:9092"); props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer"); props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer"); try (KafkaProducer producer = new KafkaProducer(props)) { String topic = "AI-Data"; String message = "{"data": [1, 2, 3]}"; producer.send(new ProducerRecord(topic, message)); System.out.println("Message sent successfully"); } } }
This example showcases how to publish a message to a Kafka topic, which can be consumed by other applications or services for further processing and analysis. With such integrations, Java applications can become part of larger, distributed systems designed for advanced AI functionalities.
Integrating Java with other AI technologies enhances its flexibility and applicability in the AI domain. By strategically using the strengths of different programming languages, frameworks, and platforms, developers can create powerful, efficient, and innovative AI solutions that address complex challenges across various industries.