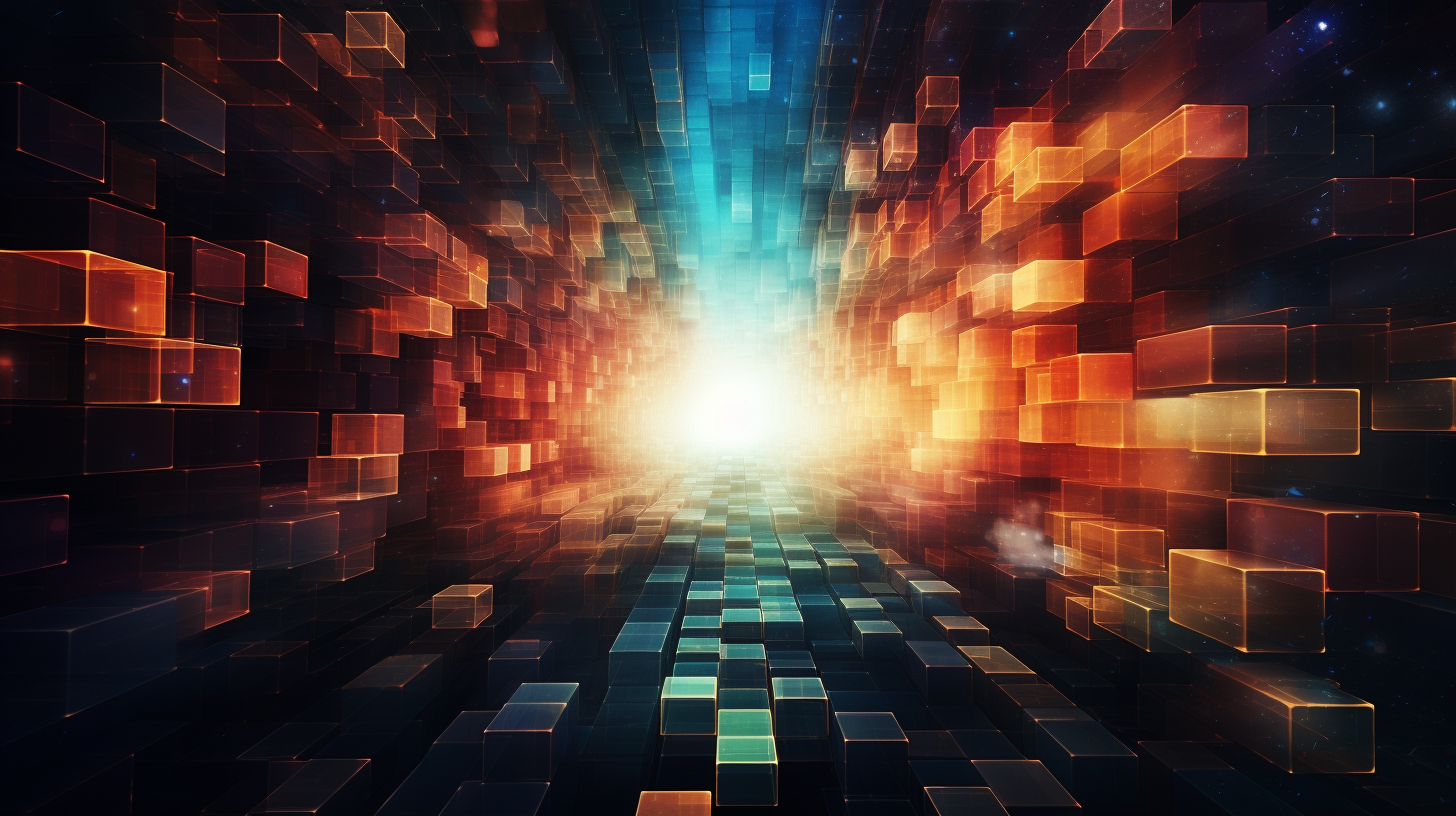
Java and Aspect-Oriented Programming (AOP)
Aspect-Oriented Programming (AOP) is a programming paradigm that enhances modularity by allowing the separation of cross-cutting concerns. In Java, AOP provides a way to define and manage aspects, which encapsulate behaviors that affect multiple classes. Such behaviors include logging, security, transaction management, and performance monitoring, which are often scattered throughout the codebase, making it harder to maintain and evolve over time.
In traditional programming, a developer typically deals with concerns that are specific to a single module or class. However, AOP allows developers to define these cross-cutting concerns separately from the main business logic, creating cleaner, more modular code. That’s achieved through the use of aspects, which are made up of join points, pointcuts, and advice.
A join point is a specific point in the execution of the program, such as method calls or object instantiations. A pointcut defines a set of join points where an aspect’s advice should be applied. Finally, advice is the action taken by an aspect at a particular join point, which can be executed before, after, or around the join point itself.
For example, think the following simple AOP setup in Java. This demonstrates a logging aspect that captures method execution:
import org.aspectj.lang.annotation.After; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.Pointcut; @Aspect public class LoggingAspect { @Pointcut("execution(* com.example.service.*.*(..))") public void serviceMethod() {} @After("serviceMethod()") public void logAfterMethod() { System.out.println("Method executed."); } }
In this example, the LoggingAspect class uses annotations provided by AspectJ, a popular AOP framework for Java. The @Pointcut annotation defines where the advice should be applied, targeting all methods within the com.example.service
package. The @After annotation indicates that the logAfterMethod
should execute after the join points matched by the pointcut.
This separation of concerns allows for cleaner code management and promotes reusability. By defining aspects in a modular way, developers can easily apply the same behavior across different parts of an application without the need to duplicate code, significantly reducing the chances of errors and inconsistencies.
Key Concepts of Aspect-Oriented Programming
At the heart of Aspect-Oriented Programming are several key concepts that fundamentally reshape how developers approach the design of their Java applications. These concepts are intertwined and collectively enable the powerful capabilities of AOP.
1. Aspects: An aspect is a module that encapsulates a cross-cutting concern. It can be thought of as a bundle of related functionalities that can be applied across multiple classes. In Java, aspects are typically implemented using annotations or XML configuration, depending on the AOP framework in use. The use of aspects allows developers to focus on the core business logic without being distracted by concerns that affect multiple parts of the application.
2. Join Points: Join points refer to specific points in the execution of a program where an aspect can be applied. Common join points include method calls, object instantiations, and exception handling. In AOP, the granularity of join points allows for precise control over where and when aspects are woven into the application.
3. Pointcuts: A pointcut is an expression that specifies a set of join points. It defines the criteria for selecting join points where advice should be applied. This allows developers to fine-tune their aspects, ensuring that the right advice is executed at the right time. For instance, a pointcut could define that a logging aspect should only apply to specific methods within a service class.
4. Advice: Advice is the action taken by an aspect at a particular join point. There are several types of advice, including:
- This advice runs before the join point.
- This advice runs after the join point has executed.
- This advice wraps the join point, allowing for actions to be taken both before and after the join point executes, and even the ability to skip the join point altogether.
The following example demonstrates different types of advice in a logging aspect:
import org.aspectj.lang.annotation.Around; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.proceedingJoinPoint; @Aspect public class EnhancedLoggingAspect { @Pointcut("execution(* com.example.service.*.*(..))") public void serviceMethod() {} @Before("serviceMethod()") public void logBeforeMethod() { System.out.println("Method execution started."); } @After("serviceMethod()") public void logAfterMethod() { System.out.println("Method execution ended."); } @Around("serviceMethod()") public Object logAroundMethod(ProceedingJoinPoint joinPoint) throws Throwable { System.out.println("Before method: " + joinPoint.getSignature()); Object result = joinPoint.proceed(); System.out.println("After method: " + joinPoint.getSignature()); return result; } }
In this example, the EnhancedLoggingAspect uses three different types of advice. The @Before
advice logs a message before the method execution begins, while the @After
advice logs a message after the method execution ends. The @Around
advice provides additional functionality by allowing the method execution to be controlled, logging information both before and after the method is called.
5. Weaving: Weaving is the process of integrating aspects into the main codebase. This can occur at different times: compile-time, load-time, or runtime. Each weaving strategy has its own advantages and is chosen based on the specific requirements of the application. In the case of Spring AOP, for instance, weaving typically occurs at runtime using proxies.
By understanding these key concepts, developers can harness the full potential of AOP in Java, leading to a more modular, maintainable, and scalable codebase. This paradigm shift not only simplifies the programming model but also empowers developers to address complex cross-cutting concerns without polluting the core logic of their applications.
Implementing AOP in Java with Spring
Implementing Aspect-Oriented Programming (AOP) in Java is elegantly facilitated by the Spring Framework, which provides a robust AOP module. Spring AOP allows developers to seamlessly integrate cross-cutting concerns into their applications using a series of annotations and configurations. The primary benefit is that it allows one to keep the business logic clean while managing concerns such as transaction management, logging, and security in a centralized manner. This results in reduced code duplication and improved maintainability.
To implement AOP with Spring, you first need to ensure that your project is set up with the necessary Spring dependencies, particularly the Spring AOP library. If you’re using Maven, you can include the following dependency in your pom.xml
:
org.springframework spring-aop 5.3.10
Next, you will create your aspect class. As in the previous examples, you can define a logging aspect. This time, let’s also add transaction management as a cross-cutting concern:
import org.aspectj.lang.annotation.After; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.Before; import org.springframework.transaction.annotation.Transactional; @Aspect public class TransactionAspect { @Before("execution(* com.example.service.*.*(..))") @Transactional public void beginTransaction() { System.out.println("Transaction started."); } @After("execution(* com.example.service.*.*(..))") public void commitTransaction() { System.out.println("Transaction committed."); } }
In this example, the TransactionAspect
class defines two advice methods: beginTransaction
, which is annotated with @Transactional
, indicating that the method should execute within a transaction context, and commitTransaction
, which signifies the completion of the transaction.
Once your aspects are defined, you need to configure your Spring application context to enable AOP. This can be done through XML configuration or Java configuration. Here’s how you can do it using Java configuration:
import org.springframework.context.annotation.AdviceMode; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.context.annotation.EnableAspectJAutoProxy; @Configuration @EnableAspectJAutoProxy public class AppConfig { @Bean public TransactionAspect transactionAspect() { return new TransactionAspect(); } }
The @EnableAspectJAutoProxy
annotation allows Spring to recognize and process the aspect annotations in your application. By defining your aspect as a Spring bean, you ensure that it is managed by the Spring container, enabling AOP features.
Now, let’s see how this works in practice. Suppose you have a simple service class where these aspects will be applied:
import org.springframework.stereotype.Service; @Service public class UserService { public void createUser(String username) { System.out.println("Creating user: " + username); } public void deleteUser(String username) { System.out.println("Deleting user: " + username); } }
When you invoke the methods of UserService
, the defined aspects will automatically trigger:
import org.springframework.context.ApplicationContext; import org.springframework.context.annotation.AnnotationConfigApplicationContext; public class Main { public static void main(String[] args) { ApplicationContext context = new AnnotationConfigApplicationContext(AppConfig.class); UserService userService = context.getBean(UserService.class); userService.createUser("john_doe"); userService.deleteUser("john_doe"); } }
Running this code will produce output indicating that transactions have begun and ended, demonstrating how AOP seamlessly integrates with the application’s core logic without cluttering the business methods.
Implementing AOP in Java with Spring greatly simplifies the management of cross-cutting concerns, enhancing the modularity and maintainability of your applications. By using Spring’s powerful AOP capabilities, developers can focus on building robust business logic while efficiently handling transactions, logging, and other concerns in a centralized manner.
Common AOP Use Cases in Java Applications
Aspect-Oriented Programming (AOP) is particularly useful for addressing common scenarios encountered in Java applications. By allowing cross-cutting concerns to be handled separately from the core business logic, AOP not only improves code organization but also enhances maintainability and scalability. Below are several common use cases where AOP shines in Java applications:
Logging
One of the most prevalent use cases for AOP is logging. Instead of scattering logging statements throughout your codebase, you can encapsulate logging logic within an aspect. This enables centralized logging management and reduces the risk of inconsistencies. For example, you might want to log method execution times across various service classes:
import org.aspectj.lang.ProceedingJoinPoint; import org.aspectj.lang.annotation.Around; import org.aspectj.lang.annotation.Aspect; @Aspect public class PerformanceLoggingAspect { @Around("execution(* com.example.service.*.*(..))") public Object logPerformance(ProceedingJoinPoint joinPoint) throws Throwable { long startTime = System.currentTimeMillis(); Object result = joinPoint.proceed(); long endTime = System.currentTimeMillis(); System.out.println("Execution time of " + joinPoint.getSignature() + ": " + (endTime - startTime) + " ms"); return result; } }
In this example, the logPerformance
method wraps the execution of service methods, measuring and logging their execution time without cluttering the service logic.
Transaction Management
In enterprise applications, the management of transactions especially important for maintaining data integrity. AOP allows for elegant handling of transaction boundaries. By defining transaction aspects, you can ensure that transactions are started and committed properly without mixing transaction management code with business logic:
import org.aspectj.lang.annotation.After; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.Before; import org.springframework.transaction.annotation.Transactional; @Aspect public class TransactionManagementAspect { @Before("execution(* com.example.repository.*.*(..))") @Transactional public void beginTransaction() { System.out.println("Transaction started."); } @After("execution(* com.example.repository.*.*(..))") public void commitTransaction() { System.out.println("Transaction committed."); } }
This aspect ensures that every method in the repository layer is executed within a transaction, enhancing clarity and maintainability.
Security
Security concerns, such as authentication and authorization checks, are another area where AOP is beneficial. By creating security aspects, you can enforce security policies consistently across various application components. For example:
import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.Before; @Aspect public class SecurityAspect { @Before("execution(* com.example.service.AdminService.*(..))") public void checkAdminPermission() { // Logic to check if the current user has admin permissions System.out.println("Admin permission checked."); } }
This aspect checks for admin permissions before executing any method in the AdminService
, ensuring that security checks are centralized and easily managed.
Performance Monitoring
Monitoring performance in production environments is essential. AOP can facilitate the collection of performance metrics without invasive changes to application code. You can use aspects to gather metrics and send them to monitoring systems:
@Aspect public class MonitoringAspect { @Around("execution(* com.example.service.*.*(..))") public Object monitor(MethodInvocation invocation) throws Throwable { long start = System.currentTimeMillis(); Object result = invocation.proceed(); long duration = System.currentTimeMillis() - start; System.out.println("Method " + invocation.getMethod().getName() + " took " + duration + " ms"); return result; } }
This allows developers to gather insights on method performance across the application without embedding timing logic into individual methods.
Exception Handling
Another useful application of AOP is centralized exception handling. By defining exception handling aspects, you can manage exceptions thrown from various parts of your application in a consistent manner:
@Aspect public class ExceptionHandlingAspect { @AfterThrowing(pointcut = "execution(* com.example.service.*.*(..))", throwing = "ex") public void handleServiceException(Exception ex) { System.out.println("Exception: " + ex.getMessage()); // Additional handling, like logging or notifying an error tracking system } }
This aspect captures exceptions thrown by service methods, allowing for comprehensive error management and reducing repetitive error handling code.
These use cases illustrate the versatility of AOP in Java applications. By abstracting cross-cutting concerns, developers can maintain cleaner code and focus on delivering robust business functionality. The power of AOP lies in its ability to promote reusability and separation of concerns, ultimately leading to more maintainable and scalable software solutions.
Advantages and Challenges of AOP
Aspect-Oriented Programming (AOP) offers a myriad of advantages and challenges that developers should consider when adopting this paradigm in their Java applications. On the one hand, AOP enhances modularity and promotes cleaner code management, allowing developers to isolate cross-cutting concerns from the core business logic. This isolation leads to improved maintainability, as changes to these concerns can be made in one place without affecting the main application flow.
One significant advantage of AOP is the ability to promote code reusability. By defining aspects separately, developers can easily apply the same behavior, such as logging or security checks, across multiple classes or methods without duplicating code. This not only reduces the risk of inconsistencies but also minimizes the potential for errors that arise from having scattered, repetitive code.
Performance can also be improved through AOP. By centralizing cross-cutting concerns, such as logging or monitoring, developers can implement performance-related logic that runs transparently and efficiently, ensuring that the core logic remains unencumbered. For instance, a monitoring aspect can gather and report metrics without altering the service methods, allowing for unobtrusive performance tracking.
However, while AOP provides these advantages, it also introduces its own set of challenges. One of the primary challenges is the complexity that AOP can add to the codebase. Since aspects weave their behavior into the application at runtime or compile time, it can become difficult for developers to trace the flow of execution. Understanding how different aspects interact and affect the application can pose a steep learning curve, especially for those new to AOP.
Another challenge lies in debugging. When issues arise, the separation of concerns can make it harder to pinpoint the source of a problem, as the behavior of an application may be influenced by various aspects that are not immediately visible in the core logic. This can lead to a situation where developers spend considerable time tracing through multiple layers of aspects to fully understand the application behavior.
Moreover, the potential for performance overhead should not be overlooked. Although AOP can improve performance by eliminating code duplication, the additional indirection introduced by aspects can lead to runtime overhead. That’s particularly relevant in performance-sensitive applications where method invocation times are critical. Careful consideration must be given to how and when aspects are applied to avoid degrading application performance.
Additionally, the misuse of AOP can lead to over-engineering. With the power to apply cross-cutting concerns liberally, developers might be tempted to create aspects for trivial concerns, leading to an overly complex design that can be difficult to maintain. Striking the right balance between using AOP to improve modularity and avoiding excessive complexity is key to using its benefits effectively.
While AOP offers significant advantages in terms of modularity, reusability, and performance monitoring, it is essential to be aware of the challenges it brings, including debugging complexity, potential performance overhead, and the risk of over-engineering. By carefully weighing these factors, developers can make informed decisions about incorporating AOP into their Java applications, ultimately leading to a more organized and maintainable codebase.
Best Practices for Using AOP in Java Development
When working with Aspect-Oriented Programming (AOP) in Java, employing best practices can significantly enhance the effectiveness of your implementations, ensuring that your code remains clean, maintainable, and efficient. Understanding the nuances of AOP, alongside its capabilities, informs how you structure aspects and when to apply them. Below are some key best practices to follow:
1. Keep Aspects Focused: Each aspect should encapsulate a single cross-cutting concern. This separation of concerns simplifies debugging and promotes reusability. For instance, if you have logging and security checks, create separate aspects for each rather than merging their functionalities. This not only avoids complexity but also ensures that any changes required in one aspect do not inadvertently affect the other.
import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.Before; @Aspect public class LoggingAspect { @Before("execution(* com.example.service.*.*(..))") public void logMethodExecution() { System.out.println("Method is about to execute."); } } @Aspect public class SecurityAspect { @Before("execution(* com.example.service.AdminService.*(..))") public void checkAdminAccess() { System.out.println("Checking admin permissions."); } }
2. Use Descriptive Pointcuts: Ensure that your pointcut expressions are descriptive and precise. This clarity aids other developers in understanding when and where aspects are applied. Avoid overly broad pointcuts, as they can lead to unintended side effects and make the code less predictable. Document your pointcuts to clarify their purposes.
@Pointcut("execution(* com.example.service.UserService.*(..))") public void userServiceMethods() {}
3. Limit the Use of Around Advice: While around advice provides powerful capabilities, it should be used judiciously. Overusing around advice can lead to complex interactions that are difficult to manage and understand. Keep around advice simple and focused on aspects like performance monitoring or transaction management, where you truly need control over method execution.
@Around("execution(* com.example.service.*.*(..))") public Object monitorExecutionTime(ProceedingJoinPoint joinPoint) throws Throwable { long start = System.currentTimeMillis(); Object result = joinPoint.proceed(); long duration = System.currentTimeMillis() - start; System.out.println("Execution time: " + duration + "ms"); return result; }
4. Handle Exceptions Gracefully: When using AOP for exception handling, ensure that your aspects can gracefully manage exceptions thrown from various methods. Provide meaningful error messages and consider logging exceptions to an error tracking system for further analysis. This practice helps maintain application stability and enhances debuggability.
@AfterThrowing(pointcut = "execution(* com.example.service.*.*(..))", throwing = "ex") public void logException(Exception ex) { System.err.println("Exception occurred: " + ex.getMessage()); }
5. Test Aspects Independently: Just like any other component of your application, aspects should be independently tested to validate their behavior. Create unit tests to verify that your aspects correctly apply behavior as expected. This practice ensures reliability and helps catch issues before they propagate into production environments.
import static org.mockito.Mockito.*; import org.junit.Test; public class LoggingAspectTest { @Test public void testLogMethodExecution() { // Setup and test the logging aspect } }
6. Monitor Performance Impact: AOP can introduce performance overhead, especially if aspects are invoked frequently. Monitor the performance of your application to identify any potential bottlenecks caused by aspect weaving. Utilize profiling tools to analyze method call times and optimize aspects as necessary.
7. Document Aspect Behavior: Maintain thorough documentation for your aspects, including what they do, when they are applied, and any dependencies or interactions with other parts of the application. This documentation serves as a valuable resource for future developers and aids in maintaining code quality.
8. Avoid Overuse: While AOP is a powerful tool, over-reliance on it can lead to convoluted code. Use AOP for true cross-cutting concerns and evaluate whether a simpler design pattern might suffice for certain scenarios. A balanced approach ensures that your application remains easy to understand and maintain.
By following these best practices, Java developers can maximize the potential of Aspect-Oriented Programming while minimizing the risks associated with complexity and performance. Embracing these guiding principles fosters a cleaner codebase and promotes a more organized approach to handling cross-cutting concerns.