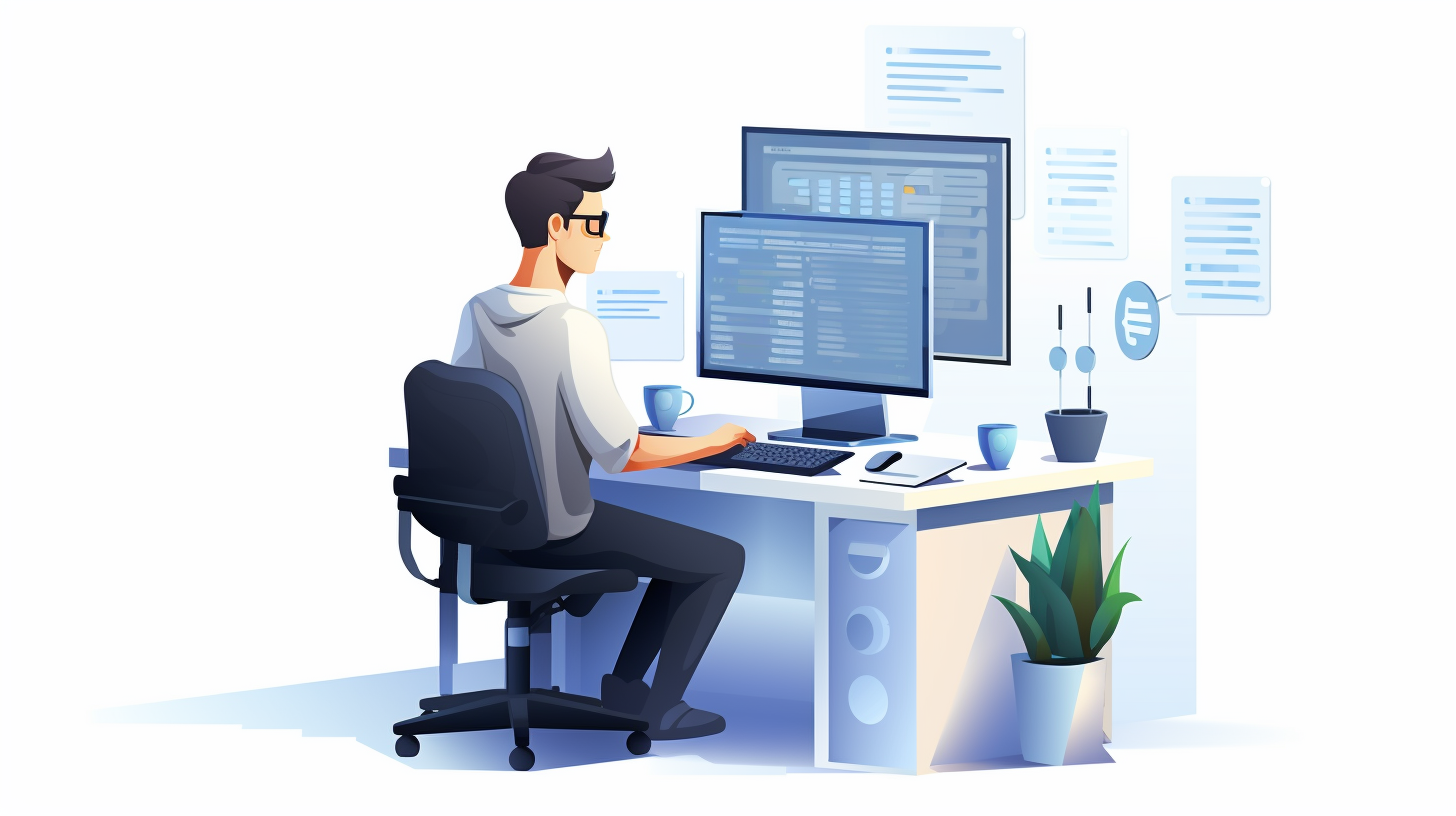
Java and Augmented Reality: Basics
Augmented Reality (AR) is a technology that overlays digital information—such as images, sounds, and other sensory enhancements—onto the real world, enhancing our perception of our surroundings. This fusion of the digital and the physical creates experiences that are not only immersive but also interactive. While traditional virtual reality immerses users in a completely synthetic environment, AR enriches the real world by adding layers of information and interaction that can be tailored to the user’s context.
At its core, AR operates by processing data from various sensors, including cameras, GPS, and accelerometers, to determine the user’s location and orientation in real time. This information is then used to project virtual elements onto the physical world. The result is a seamless blend of the actual and the virtual, allowing users to engage with digital content as if it were part of their real environment.
Understanding the technical aspects of AR involves delving into the concepts of computer vision, sensor fusion, and 3D modeling. Computer vision is the field that allows machines to interpret and understand visual information from the world, enabling the identification of surfaces and objects. Sensor fusion is the process of combining data from multiple sensors to produce more accurate and reliable information about the environment. 3D modeling is essential for creating the digital overlays that users will interact with, requiring a solid grasp of geometry and rendering techniques.
With Java, developers can leverage existing expertise in the language to build AR applications. Java’s cross-platform capabilities and its extensive ecosystem of libraries and tools make it an appealing choice for AR development. By using Java, developers can create applications that run seamlessly on a variety of devices, from smartphones to desktops, while taking full advantage of Java’s robust performance characteristics.
As AR technology continues to evolve, understanding its foundational principles becomes increasingly important. Mastery of these concepts will empower developers to create richer, more engaging experiences that push the boundaries of what is possible in augmented reality.
public class AugmentedRealityExample { public static void main(String[] args) { System.out.println("Welcome to Augmented Reality with Java!"); // Initialize AR components here initializeAR(); } private static void initializeAR() { // Code to setup AR environment System.out.println("AR environment initialized."); } }
Java’s Role in Augmented Reality Development
Java plays a pivotal role in the development of augmented reality applications, bridging the gap between robust software architecture and the immersive experiences that AR promises. The language’s inherent strengths—platform independence, a vast ecosystem, and a strong community—make it a compelling choice for developers looking to create engaging AR applications.
One of the key advantages of using Java in AR development is its cross-platform nature. With Java, developers can write code once and run it anywhere, whether on Android devices, desktops, or even embedded systems. This flexibility allows for a wider reach and simplifies the development process, as there’s no need to rewrite code for different platforms. Java’s Virtual Machine (JVM) ensures that applications maintain consistent behavior across various environments, which very important for AR scenarios where performance and user experience are paramount.
Furthermore, Java’s extensive libraries and frameworks offer a plethora of tools that streamline the development of AR applications. Libraries such as Java 3D and JOGL (Java OpenGL) provide robust support for rendering graphics and managing 3D objects, which are essential for AR experiences. Using these libraries, developers can focus more on creating interactive content rather than getting bogged down by the complexities of lower-level graphics programming.
Additionally, Java’s strong community support means that developers have access to a wealth of resources, tutorials, and third-party tools. This collaborative environment accelerates the learning curve for new developers entering the AR space. Whether it’s through forums, GitHub repositories, or comprehensive documentation, the support available helps developers troubleshoot issues, share best practices, and build upon each other’s work.
However, the role of Java in AR development is not without its challenges. While Java’s performance is generally robust, the demands of real-time processing required for AR applications can strain its capabilities, particularly when dealing with intensive graphical tasks or complex sensor data. Developers must be mindful of optimizing their code and using efficient algorithms to ensure smooth performance. For instance, when integrating sensor data to accurately place virtual objects in the real world, developers often need to implement fine-tuned algorithms that can handle the latency and variability inherent in sensor readings.
Incorporating Java into AR projects allows for the integration of sophisticated back-end services, enabling applications to pull data from cloud services or databases dynamically. This capability is invaluable for AR applications that require real-time data updates or interactions with online content. For example, consider a simple Java class that demonstrates how to connect to a web service to retrieve enriching content for an AR experience:
import java.io.BufferedReader; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; public class ARDataFetcher { private static final String API_URL = "https://api.example.com/arcontent"; public static void main(String[] args) { try { String jsonResponse = fetchARContent(); System.out.println("Received AR Content: " + jsonResponse); } catch (Exception e) { e.printStackTrace(); } } private static String fetchARContent() throws Exception { URL url = new URL(API_URL); HttpURLConnection conn = (HttpURLConnection) url.openConnection(); conn.setRequestMethod("GET"); BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream())); String inputLine; StringBuilder response = new StringBuilder(); while ((inputLine = in.readLine()) != null) { response.append(inputLine); } in.close(); return response.toString(); } }
In this example, the ARDataFetcher class connects to a hypothetical API to retrieve content that could be overlaid in an AR environment. Such integrations enhance the interactivity and richness of AR experiences, allowing users to engage with live data in meaningful ways.
Ultimately, Java’s role in augmented reality development is defined by its versatility and the powerful experiences it can help create. By using Java’s strengths, developers can build AR applications that not only function seamlessly across platforms but also captivate users with innovative and interactive content.
Key Libraries and Frameworks for AR in Java
When it comes to building robust augmented reality applications in Java, several libraries and frameworks stand out, each offering unique functionalities that cater to different aspects of AR development. Here’s a closer look at some of the most prominent tools that Java developers can leverage to bring their augmented reality visions to life.
ARToolKit is one of the most well-known libraries in the AR community. Originally developed for C, it has been ported to Java, allowing developers to create marker-based AR applications. The framework provides functionality for detecting and tracking markers, rendering 2D and 3D graphics, and managing user interaction. Using ARToolKit, developers can create applications that overlay digital content on real-world objects, making it a staple for many AR projects.
import org.artoolkit.ar.base.ARActivity; public class MyARActivity extends ARActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); // Initialize ARToolKit components initAR(); } private void initAR() { // Code to set up AR environment with ARToolKit System.out.println("ARToolKit initialized."); } }
Java 3D and JOGL (Java OpenGL) are essential for rendering graphics in AR applications. Java 3D provides a high-level API for 3D graphics, while JOGL gives developers the ability to utilize OpenGL directly within Java applications. Together, they provide the necessary tools to create complex 3D models and environments that can be seamlessly integrated into AR experiences. With these libraries, developers can render 3D objects that interact with the real world, allowing for immersive application experiences.
import com.jogamp.opengl.GL2; import com.jogamp.opengl.GLOffscreenAutoDrawable; public class GraphicsRenderer { public void display(GL2 gl) { // Set up rendering properties gl.glClear(GL2.GL_COLOR_BUFFER_BIT | GL2.GL_DEPTH_BUFFER_BIT); gl.glLoadIdentity(); // Render a 3D object here render3DModel(gl); } private void render3DModel(GL2 gl) { // Code to render a 3D model in AR System.out.println("Rendering 3D model in AR."); } }
Vuforia is another powerful framework for AR development, though primarily known for its use in mobile applications. It offers advanced image recognition capabilities, enabling applications to track complex images and objects in real-time. While Vuforia is often associated with native Android and iOS development, it can be integrated into Java applications through JNI (Java Native Interface) or using cross-platform tools like libGDX.
libGDX is a cross-platform game development framework that allows developers to create applications for multiple platforms using a single codebase. Although primarily aimed at gaming, libGDX provides extensive support for 2D and 3D graphics, input handling, and integration with other libraries, making it a viable option for developing AR applications. Its flexibility and the ability to deploy to desktop, Android, iOS, and web platforms make it an attractive choice for AR developers looking to reach a wider audience.
import com.badlogic.gdx.ApplicationAdapter; import com.badlogic.gdx.Gdx; public class ARGame extends ApplicationAdapter { @Override public void create() { // Initialization code for libGDX AR application System.out.println("AR Game created with libGDX."); } @Override public void render() { // Render loop for AR content System.out.println("Rendering AR content..."); } }
These libraries and frameworks form a solid foundation for developers eager to explore the exciting world of augmented reality in Java. Whether it’s creating compelling graphics, managing 3D models, or using advanced sensor data, each tool offers unique advantages that can help bring AR experiences to life. As developers experiment and innovate within this space, the possibilities for what can be achieved with Java and AR continue to expand.
Building Your First Augmented Reality Application with Java
Building your first augmented reality (AR) application with Java can be an exciting venture, blending your programming skills with the innovative realm of AR technology. This section will guide you through the fundamental steps of creating a basic AR application, using Java libraries and frameworks to bring your digital overlays to life.
Before diving into coding, it’s essential to have a clear understanding of the components necessary for an AR environment. At its core, an AR application requires a way to process real-world inputs, typically done through a camera feed, and a mechanism to overlay virtual content onto that feed. In Java, you can leverage libraries like ARToolKit or libGDX, which provide foundational support for these functionalities.
Let’s start by setting up a simple AR application using ARToolKit. First, ensure that you have ARToolKit set up in your Java development environment. With ARToolKit integrated, you can begin to initialize the AR components.
import org.artoolkit.ar.base.ARActivity; import org.artoolkit.ar.base.ARRenderer; public class MyFirstARApp extends ARActivity { private ARRenderer arRenderer; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); arRenderer = new ARRenderer(); initAR(); } private void initAR() { // Set up AR environment System.out.println("Initializing AR environment..."); // Additional setup for ARToolKit components arRenderer.init(); } @Override public void onDrawFrame(GL10 gl) { // Rendering logic here arRenderer.render(); } }
In this code snippet, we define a class MyFirstARApp
that extends ARActivity
, which is a core class in ARToolKit for handling AR functionalities. The onCreate
method initializes the AR environment and sets up the renderer. The onDrawFrame
method is where you would place the rendering logic, allowing the application to continuously draw the AR content on top of the camera feed.
Next, to create an engaging AR experience, we’ll need to overlay some virtual objects onto the real world. Typically, this involves detecting markers or surfaces. Here’s how you can implement a simple marker-based overlay:
import org.artoolkit.ar.base.ARToolKit; public class MyFirstARApp extends ARActivity { @Override public void onDrawFrame(GL10 gl) { // Check for detected markers int[] markerIds = ARToolKit.getMarkerIds(); for (int markerId : markerIds) { if (markerId == TARGET_MARKER_ID) { // Draw 3D object at marker position draw3DModel(gl); } } } private void draw3DModel(GL10 gl) { // Define your 3D model drawing logic here System.out.println("Drawing 3D model over the marker."); } }
In this example, the application continuously checks for detected markers using ARToolKit’s getMarkerIds
method. When a specific marker is detected, the draw3DModel
method is called to render the virtual object at the marker’s position. This creates the illusion that the virtual object is anchored in the real world.
It’s time to take a look at how you can enhance the interaction by integrating user input. You might want to allow users to manipulate the virtual object or trigger animations. Here’s a brief example of handling touch events:
@Override public boolean onTouchEvent(MotionEvent event) { if (event.getAction() == MotionEvent.ACTION_DOWN) { // Handle touch event to interact with the AR content System.out.println("Touch event detected on AR content."); // Logic to manipulate AR object return true; } return super.onTouchEvent(event); }
This touch event handler allows users to interact with the AR content displayed on their screens, opening up a realm of possibilities for engaging applications.
As you build out your AR application, remember to test it on real devices to ensure that the performance and user experience are optimal. With Java’s flexibility and the capabilities of ARToolKit or other frameworks, you can create compelling AR experiences that captivate users and enhance their interaction with the physical world.
Challenges and Considerations in Java AR Development
Developing augmented reality (AR) applications in Java presents several challenges that developers must navigate to create effective and engaging experiences. Understanding these challenges very important for success, as they often stem from the complex interplay between hardware capabilities, software architecture, and real-time processing requirements.
One of the most significant challenges in Java AR development is managing the performance and responsiveness of applications. AR applications frequently rely on real-time data from various sensors, such as cameras and accelerometers. This data must be processed quickly to maintain a seamless interactive experience. Java, while capable, can sometimes fall short in performance when dealing with intensive graphics or complex calculations due to its garbage collection mechanisms and the overhead of the Java Virtual Machine (JVM). Thus, developers need to be proactive in optimizing their code.
Optimizations can include reducing memory allocations in performance-critical sections of code, using efficient data structures, and minimizing the frequency of complex calculations. For instance, employing spatial partitioning techniques can help manage and query objects in a 3D space more efficiently, ensuring that only relevant objects are processed during rendering. Here’s a simple example of an efficient data structure implementation for managing AR objects:
import java.util.ArrayList; import java.util.List; public class ARObjectManager { private List arObjects; public ARObjectManager() { arObjects = new ArrayList(); } public void addObject(ARObject obj) { arObjects.add(obj); } public void updateObjects() { for (ARObject obj : arObjects) { obj.update(); // Efficiently update each object } } }
Another consideration is the integration of various hardware capabilities. Different devices have different specifications, which can lead to inconsistencies in how AR applications function across platforms. Developers must ensure that their applications can adapt to these variations. This often means implementing fallbacks or alternative methods for devices with less processing power or limited graphics capabilities. Java’s cross-platform nature helps here, but it also requires careful testing on multiple devices to ensure consistent performance.
Moreover, managing the accuracy of sensor data is critical. In AR applications, precise positioning is vital for the believable placement of virtual objects in the real world. Sensor data can be noisy and may require filtering or smoothing algorithms. The Kalman filter, for example, is widely used to enhance the accuracy of sensor readings by predicting the state of a system over time. Implementing such algorithms in Java can help mitigate issues arising from sensor inaccuracies.
public class KalmanFilter { private double q; // process noise covariance private double r; // measurement noise covariance private double x; // value private double p; // estimation error covariance private double k; // Kalman gain public KalmanFilter(double q, double r) { this.q = q; this.r = r; this.x = 0; // initial value this.p = 1; // initial estimation error covariance } public double update(double measurement) { // Prediction update p += q; // Measurement update k = p / (p + r); x += k * (measurement - x); p *= (1 - k); return x; // return filtered value } }
Finally, user interface design poses unique challenges in AR applications. Users interact with virtual content overlaid on their real-world environment, requiring intuitive controls and clear feedback. Balancing the digital and physical interactions is necessary to ensure users can easily manipulate AR content without feeling overburdened. Developers must invest time in designing user interactions that enhance the experience rather than detract from it.
The challenges of Java AR development are multifaceted, spanning performance optimization, hardware integration, sensor accuracy, and user interface design. By acknowledging these challenges and employing appropriate strategies, developers can create compelling AR experiences that leverage the strengths of Java while minimizing its limitations.
Future Trends in Java and Augmented Reality
As we venture into the future of augmented reality (AR) development using Java, it’s essential to recognize the trends shaping this dynamic field. With the rapid advancements in technology, developers are poised to explore new capabilities and opportunities that will redefine the AR experience. Java, with its extensive library support and cross-platform capabilities, is well-positioned to adapt and thrive in this evolving landscape.
One of the most significant trends on the horizon is the integration of artificial intelligence (AI) within AR applications. AI technologies, from machine learning to computer vision, can enhance the interactivity and personalization of AR experiences. For instance, using deep learning models, developers can create applications that not only recognize objects but also learn and adapt to user behaviors. This level of sophistication can lead to dynamic content delivery tailored to individual user preferences, enriching the overall AR experience.
Moreover, the advent of 5G technology is set to revolutionize AR applications. With its high bandwidth and low latency, 5G will facilitate more complex and immersive AR experiences. Developers will be able to stream high-quality content and enable multi-user interactions in real-time, making collaborative AR experiences more achievable. For instance, consider a scenario where multiple users can interact with the same virtual object in a shared space, leading to a more engaging and social experience.
Additionally, advancements in hardware, particularly in mobile devices, will enhance the capabilities of AR applications. As smartphones and tablets become more powerful, with better graphics processing units (GPUs) and enhanced sensors, developers can push the boundaries of what is possible within AR. This hardware evolution will allow for more complex rendering of 3D models and the integration of real-time environmental data, enabling applications that respond intelligently to the physical world.
In terms of frameworks and libraries, there is also a growing trend towards open-source solutions in the AR development space. The community-driven nature of open-source projects fosters innovation and collaboration, allowing developers to leverage and contribute to a shared pool of resources. Java developers can benefit from this trend by adopting and contributing to open-source AR libraries, thereby enhancing their projects while also giving back to the community.
Furthermore, the rise of web-based AR applications is becoming increasingly prominent. With the advent of WebXR, developers can create AR experiences that run directly in web browsers, eliminating the barriers associated with native app installations. This accessibility can significantly broaden the reach of AR applications, allowing users to engage with AR content simply by visiting a website. Java developers can explore integrating WebXR with existing Java frameworks to provide seamless AR experiences across different platforms.
Lastly, as privacy concerns continue to grow in the digital realm, developers will need to prioritize user data protection in AR applications. Ensuring compliance with regulations and implementing robust security measures will be crucial for fostering user trust. Java’s robust security features can be leveraged to create secure AR environments that safeguard user data while delivering immersive experiences.
The future of AR development in Java is rife with possibilities driven by AI integration, enhanced connectivity through 5G, advancements in hardware, the rise of open-source frameworks, and the emergence of web-based applications. By staying attuned to these trends, Java developers can create innovative AR solutions that not only meet user expectations but also pave the way for the next generation of augmented reality experiences.