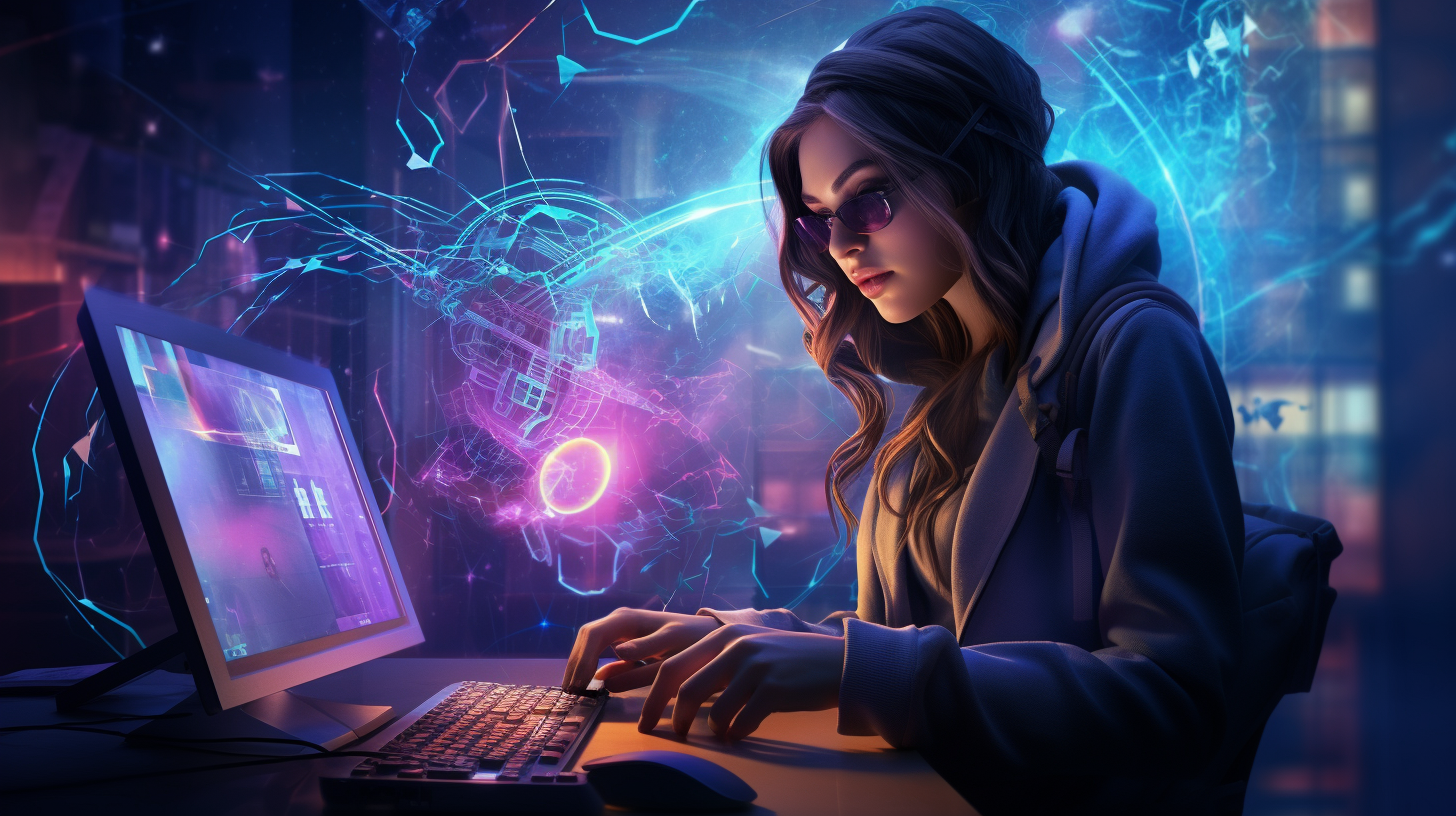
Java and Automated Testing: Frameworks and Tools
Java testing frameworks provide the essential foundation for developers to ensure that their code is functioning correctly and efficiently. They offer structured environments for writing, organizing, and executing tests, thus promoting better software quality and reliability. Among the most popular Java testing frameworks are JUnit, TestNG, and Mockito, each serving distinct purposes within the scope of automated testing.
JUnit is perhaps the most recognized Java testing framework. It’s primarily used for unit testing, allowing developers to write and run repeatable tests. JUnit’s simplicity and integration with various IDEs make it a go-to choice for many developers. With annotations like @Test
, @Before
, and @After
, it provides a systematic way to define test cases and manage the test lifecycle.
import org.junit.Test; import static org.junit.Assert.assertEquals; public class CalculatorTest { @Test public void testAddition() { Calculator calculator = new Calculator(); assertEquals(5, calculator.add(2, 3)); } }
TestNG is another popular framework that extends JUnit’s capabilities. It introduces advanced features such as data-driven testing, parallel test execution, and a flexible test configuration. TestNG’s annotations provide more granular control over test execution order and dependencies, which can be particularly useful in large projects with complex testing requirements.
import org.testng.annotations.Test; public class CalculatorTest { @Test public void testAddition() { Calculator calculator = new Calculator(); assertEquals(5, calculator.add(2, 3)); } }
Mockito is not a testing framework in the traditional sense but a mocking framework that works well in conjunction with JUnit or TestNG. It allows developers to create mock objects in automated tests, enabling them to simulate the behavior of complex dependencies. This is particularly valuable in unit testing when the focus is on testing a single component in isolation.
import static org.mockito.Mockito.*; import org.junit.Test; public class UserServiceTest { @Test public void testUserRetrieval() { UserRepository mockRepo = mock(UserRepository.class); when(mockRepo.findUser("john")).thenReturn(new User("john", "password")); UserService userService = new UserService(mockRepo); User user = userService.getUser("john"); assertEquals("john", user.getUsername()); } }
Using these frameworks in harmony allows developers to cover a wide range of testing scenarios, from unit tests ensuring individual components function correctly, to integration tests verifying that combined components work together as expected. Each framework brings its strengths to the table, and understanding how to leverage them effectively is important for modern Java development.
Popular Automated Testing Tools for Java
In addition to the well-known frameworks like JUnit, TestNG, and Mockito, the Java ecosystem boasts a variety of automated testing tools that further enhance the capabilities of developers. These tools cater to different aspects of testing, from unit and integration testing to performance and end-to-end testing, providing a comprehensive suite for quality assurance.
Selenium is one of the most prominent tools for automated functional testing of web applications. It allows developers to write test scripts in various programming languages, including Java, and can be easily integrated with testing frameworks like JUnit and TestNG. Selenium WebDriver interacts directly with the browser, enabling the automation of user interactions with web pages. Here’s a simple example of how Selenium can be used in combination with JUnit to test a web application’s login functionality:
import org.junit.After; import org.junit.Before; import org.junit.Test; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; public class LoginTest { private WebDriver driver; @Before public void setUp() { // Set the path to the chromedriver executable System.setProperty("webdriver.chrome.driver", "path/to/chromedriver"); driver = new ChromeDriver(); } @Test public void testLogin() { driver.get("http://example.com/login"); driver.findElement(By.name("username")).sendKeys("testuser"); driver.findElement(By.name("password")).sendKeys("testpass"); driver.findElement(By.id("loginButton")).click(); // Verify that login was successful String expectedUrl = "http://example.com/dashboard"; assertEquals(expectedUrl, driver.getCurrentUrl()); } @After public void tearDown() { driver.quit(); } }
Another noteworthy tool is Apache JMeter, primarily designed for performance testing. It is capable of simulating a heavy load on a server, group of servers, or network to test the performance and behavior under different conditions. JMeter supports various protocols, making it versatile for testing both web applications and APIs. Here’s how you might set up a basic performance test for a REST API:
/* JMeter configuration in XML format: - Define test plan - Add HTTP Request Sampler - Add Listener to view results */ /* Example of a simple JMeter test plan (in .jmx format): - Thread Group - HTTP Request This XML configuration can be loaded into JMeter to create a performance test scenario. */
For testing RESTful services, REST-assured is an elegant solution that simplifies the process of writing tests for REST APIs. It allows developers to write expressive tests using a domain-specific language that integrates seamlessly with JUnit or TestNG. Below is an example demonstrating how to use REST-assured to test an API endpoint:
import static io.restassured.RestAssured.*; import static org.hamcrest.Matchers.*; public class ApiTest { @Test public void testGetUser() { given() .pathParam("username", "john") .when() .get("http://example.com/api/users/{username}") .then() .statusCode(200) .body("username", equalTo("john")); } }
Furthermore, tools like Cucumber enable behavior-driven development (BDD) by allowing developers to write tests in plain language that can be easily understood by non-technical stakeholders. This fosters better collaboration between development and business teams while ensuring that the implemented features meet the required specifications.
These tools, among others, create a robust environment for automated testing in Java. Each tool has its unique strengths and use cases, making it crucial for developers to select the right combination that aligns with their project requirements and testing objectives. Embracing these popular testing tools allows development teams to enhance their testing efficiency and ultimately deliver higher quality software.
Integration of Testing Frameworks with CI/CD
Integrating testing frameworks with Continuous Integration and Continuous Deployment (CI/CD) pipelines is an important step toward streamlining the development process and ensuring high software quality. By automating the testing phase, developers can catch bugs early, reduce manual testing efforts, and facilitate a quicker release cycle. In Java development, the synergy between testing frameworks and CI/CD tools can be achieved using various approaches and strategies.
One of the fundamental aspects of CI/CD integration is the ability to automatically trigger tests when changes are made to the codebase. This can be accomplished using popular CI/CD tools such as Jenkins, GitLab CI, and CircleCI. These tools allow developers to define specific jobs in the pipeline that execute automated tests as part of the build process. For example, a Jenkins pipeline can be set up to run JUnit tests every time code is pushed to a repository.
pipeline { agent any stages { stage('Build') { steps { sh 'mvn clean install' } } stage('Test') { steps { sh 'mvn test' } } } }
In this simple Jenkins pipeline example, the ‘Build’ stage compiles the code using Maven, while the ‘Test’ stage executes the unit tests defined in the project. If any tests fail, the pipeline is marked as unsuccessful, alerting developers to issues that need to be addressed before proceeding.
For more complex scenarios, such as integration testing or end-to-end testing, additional stages can be incorporated into the pipeline. For instance, after unit tests are conducted, developers can run integration tests that verify how different components of the application interact with one another. Tools like TestNG or Mockito can be utilized in this phase, providing the necessary structure for executing these tests.
stage('Integration Test') { steps { sh 'mvn verify -P integration-tests' } }
In this stage, the Maven command is used to run integration tests as part of the verification process, ensuring that all components work together as expected. The parameterized profile ‘-P integration-tests’ can be defined in the Maven `pom.xml` to execute specific integration test cases.
Another key aspect to ponder is the reporting of test results. CI/CD tools can aggregate test results and provide visual feedback on the status of tests. This can be achieved by configuring plugins that generate test reports after each build. For JUnit, the Surefire plugin can be utilized to generate XML test reports that CI/CD tools can interpret and present in a effortless to handle format.
pom.xml configuration: <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-surefire-plugin</artifactId> <version>2.22.2</version> <configuration> <reportsDirectory>target/surefire-reports</reportsDirectory> </configuration> </plugin> </plugins> </build>
In this example, the Surefire plugin is set up to generate reports in the specified directory after running tests. These reports can then be integrated with CI/CD tools to provide developers with insights into test performance over time, trends in failures, and overall code health.
Furthermore, testing frameworks can also be integrated with other tools in the CI/CD pipeline. For instance, static code analysis tools like SonarQube can analyze the code quality during the build process, identifying potential vulnerabilities or code smells that could lead to issues down the line. By incorporating such tools, teams can enhance their code review processes, ensuring that automated tests are complemented by robust quality checks.
Finally, the integration of testing frameworks with CI/CD extends beyond mere execution. Developers should focus on maintaining and evolving their tests to adapt to changes in the code and project requirements. Agile methodologies encourage continuous feedback and collaboration, allowing teams to refine their testing strategies and frameworks based on project needs and developer input. This continuous evolution ensures that the automated tests remain relevant and effective throughout the software development lifecycle.
Best Practices for Writing Automated Tests in Java
Writing effective automated tests in Java requires a blend of strategic thinking and practical experience in coding. Here are some best practices that can help developers write efficient and maintainable automated tests, ensuring the code works as intended and remains adaptable to future changes.
1. Keep Tests Isolated
Tests should be independent of one another, meaning that the success or failure of one test should not affect others. This isolation allows for easier debugging and faster execution since tests can run in parallel without interference. Using frameworks like Mockito for mocking dependencies can help achieve this, ensuring that each test focuses solely on the unit being tested.
import static org.mockito.Mockito.*; import org.junit.Test; public class PaymentServiceTest { @Test public void testProcessPayment() { PaymentGateway mockGateway = mock(PaymentGateway.class); when(mockGateway.process(anyDouble())).thenReturn(true); PaymentService paymentService = new PaymentService(mockGateway); boolean result = paymentService.processPayment(100.0); assertTrue(result); verify(mockGateway).process(100.0); } }
2. Use Descriptive Test Names
Test names should clearly describe the expected behavior of the unit under test. This practice not only aids in understanding the purpose of each test but also makes it easier to identify failures. A naming convention that includes the method being tested and the scenario can be particularly effective.
@Test public void whenValidInput_thenReturnsExpectedResult() { // Test logic here }
3. Follow the Arrange-Act-Assert (AAA) Pattern
The AAA pattern provides a clear structure to tests, promoting readability and maintainability. The structure consists of three steps: Arrange the necessary preconditions and inputs, Act on the unit under test, and Assert that the expected outcomes occur. This pattern helps to keep the tests organized and understandable.
@Test public void testCalculateTotalPrice() { // Arrange ShoppingCart cart = new ShoppingCart(); cart.addItem(new Item("Apple", 0.99)); cart.addItem(new Item("Banana", 1.29)); // Act double total = cart.calculateTotal(); // Assert assertEquals(2.28, total, 0.01); }
4. Keep Tests Small and Focused
Each test should verify a single aspect of functionality. This approach makes it easier to identify the cause of a failure and simplifies the debugging process. Large tests that try to assert multiple outcomes can become unwieldy and obscure the underlying issues.
@Test public void testAddItemIncreasesItemCount() { ShoppingCart cart = new ShoppingCart(); cart.addItem(new Item("Apple", 0.99)); assertEquals(1, cart.getItemCount()); }
5. Use Assertions Wisely
Employ various assertions to validate different conditions and outcomes within your tests. Assert statements provide clarity on what the test is checking, and using a mix of equals, true, and exception assertions can cover a range of scenarios. The JUnit framework offers a rich set of assertion methods to help with this.
@Test(expected = IllegalArgumentException.class) public void testAddItemThrowsExceptionForNull() { ShoppingCart cart = new ShoppingCart(); cart.addItem(null); // This should throw an exception }
6. Maintain Your Tests
Automated tests are not set-and-forget; they require regular maintenance to remain relevant as the codebase evolves. Refactor tests alongside production code, remove obsolete tests, and update them as necessary to reflect changes in functionality. An effective test suite should be as agile as the application it tests.
7. Implement Continuous Testing
Incorporating automated tests into a continuous integration (CI) pipeline ensures that tests run regularly against the latest code changes. This practice helps catch regressions early, providing immediate feedback to developers and allowing for faster iterations. Automating the testing process ensures that quality is maintained throughout the development lifecycle.
By adhering to these best practices, Java developers can craft automated tests that are efficient, effective, and sustainable. This not only improves the quality of the code but also enhances the overall development process, allowing teams to deliver reliable software more rapidly.
Future Trends in Java Testing Automation
As we look toward the future of Java testing automation, several trends are emerging that promise to transform how developers approach testing in their software development practices. The landscape of automated testing is evolving rapidly due to advancements in tools, methodologies, and the increasing complexity of applications. Understanding these trends can help teams stay ahead of the curve and adopt practices that enhance their testing strategies.
1. Increased Adoption of AI and Machine Learning
Artificial Intelligence (AI) and Machine Learning (ML) are making significant inroads into automated testing. These technologies can help analyze test results, predict flaky tests, and even generate test cases based on application usage patterns. By using AI, developers can focus on high-risk areas and reduce the maintenance burden associated with large test suites. Tools integrating AI capabilities can automatically suggest optimizations, identify redundant tests, and improve overall test coverage.
public class AITest { public void optimizeTests(TestSuite suite) { AIAlgorithm algorithm = new AIAlgorithm(); List optimizedSuite = algorithm.optimize(suite); // Run the optimized test suite } }
2. Shift-Left Testing
The shift-left testing approach emphasizes the importance of testing early in the software development lifecycle. By integrating testing into the initial phases of development, teams can identify and address issues sooner, resulting in lower costs and reduced time to market. This trend is supported by the adoption of Behavior-Driven Development (BDD) practices, which encourage collaboration between technical and non-technical stakeholders to define test cases upfront.
public class BDDExample { @Given("a user is on the login page") public void userOnLoginPage() { // Code to navigate to the login page } @When("the user enters valid credentials") public void userEntersValidCredentials() { // Code to enter credentials } @Then("the user should be redirected to the dashboard") public void userRedirectedToDashboard() { // Code to verify redirection } }
3. Enhanced Test Automation Frameworks
The development of more sophisticated and effortless to handle test automation frameworks is another trend shaping the future of Java testing. Frameworks are evolving to support modularization, allowing teams to reuse components and create scalable test architectures. Tools like JUnit 5 and TestNG are continuously improving, offering better integration with state-of-the-art development environments and providing more powerful features for managing complex test scenarios.
import org.junit.jupiter.api.Test; public class ModularTest { @Test void testWithModularComponents() { Service service = new Service(new Repository()); // Assertions and test logic } }
4. Cloud-Based Testing Solutions
As applications increasingly move to the cloud, so too do testing solutions. Cloud-based testing platforms allow teams to run tests in a variety of environments without the overhead of maintaining physical infrastructure. This flexibility enables better resource allocation and supports parallel testing, leading to faster feedback cycles. The ability to simulate real-world user interactions in different browser and device configurations further enhances the robustness of automated tests.
public class CloudTest { public void runTestsInCloud() { CloudTestingService service = new CloudTestingService(); service.execute("http://example.com", "Chrome", "latest"); } }
5. Continuous Testing and DevOps Integration
Continuous Testing is becoming an integral part of DevOps practices, where testing is conducted at every stage of the software delivery pipeline. The integration of testing frameworks with CI/CD tools enables automated tests to run seamlessly, providing immediate feedback to developers. This trend emphasizes the importance of keeping tests up to date and actively managing test quality as part of the overall development process.
pipeline { agent any stages { stage('Continuous Testing') { steps { sh 'mvn clean test' } } } }
As we navigate the future of Java testing automation, embracing these trends will be essential for teams aiming to deliver high-quality software efficiently. By using advancements in technology and adopting modern practices, developers can enhance their automated testing efforts, ensuring that they meet the growing demands of software development in an increasingly complex landscape.