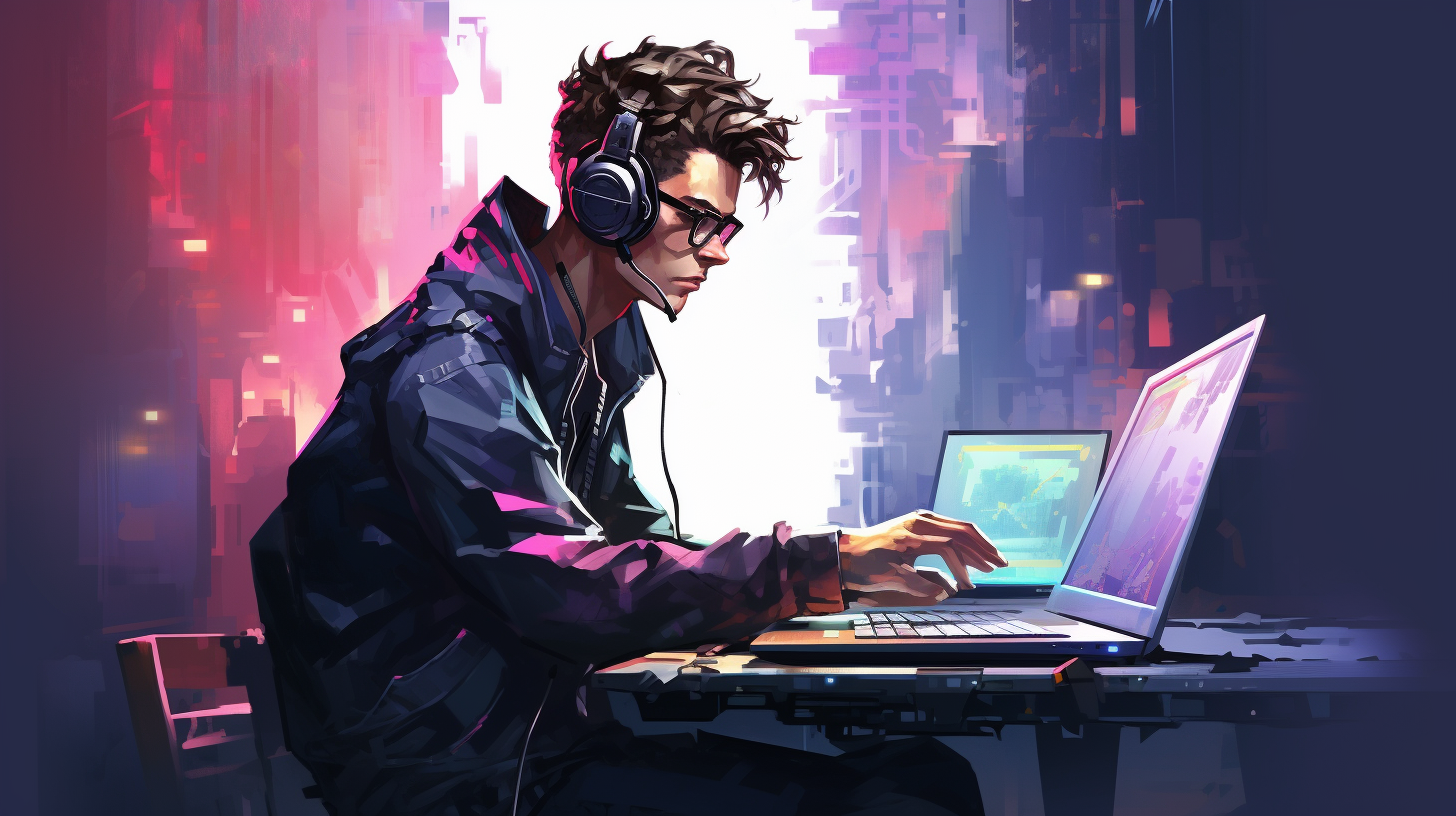
Java and Big Data: Hadoop and Spark
Big data technologies represent a paradigm shift in how large datasets are processed and analyzed. For Java developers, understanding these technologies is essential to leverage the power of data efficiently. The essence of big data lies in its volume, velocity, and variety, shaping the way applications are built and deployed. Java, as a versatile and robust programming language, has become a fundamental tool in the big data landscape.
At the heart of big data processing are distributed systems that allow for the storage and processing of data across many machines. Java plays a pivotal role in powering these systems due to its platform independence and strong concurrency features. Frameworks like Hadoop and Apache Spark are built with Java at their core, enabling developers to utilize their skills in building scalable data applications.
Hadoop, for instance, utilizes the Hadoop Distributed File System (HDFS) for storage and the MapReduce paradigm for processing. This architecture allows developers to write Java programs that can efficiently process large datasets in a distributed manner. The Java API provided by Hadoop simplifies the development of MapReduce jobs.
import org.apache.hadoop.fs.Path; import org.apache.hadoop.io.IntWritable; import org.apache.hadoop.io.Text; import org.apache.hadoop.mapreduce.Job; import org.apache.hadoop.mapreduce.Mapper; import org.apache.hadoop.mapreduce.Reducer; import java.io.IOException; public class WordCount { public static class TokenizerMapper extends Mapper
Apache Spark further enhances the Java experience in big data processing. It abstracts the complexity of distributed data processing while providing an easy-to-use API. With its in-memory data processing capabilities, Spark allows for faster data retrieval and computation, which is particularly beneficial for iterative algorithms and machine learning tasks.
import org.apache.spark.api.java.JavaRDD; import org.apache.spark.api.java.JavaSparkContext; import org.apache.spark.SparkConf; import java.util.Arrays; public class SparkExample { public static void main(String[] args) { SparkConf conf = new SparkConf().setAppName("SparkJavaExample").setMaster("local"); JavaSparkContext sc = new JavaSparkContext(conf); JavaRDD data = sc.parallelize(Arrays.asList("Hello", "World", "Hello", "Java")); long count = data.filter(s -> s.equals("Hello")).count(); System.out.println("Count of 'Hello': " + count); sc.close(); } }
Java serves as a cornerstone in the big data ecosystem, providing the necessary tools and frameworks to harness the power of distributed processing. By mastering the underlying technologies like Hadoop and Spark, Java developers can create applications that efficiently manage and analyze vast amounts of data, unlocking valuable insights that drive decision-making in today’s data-driven world.
Overview of Hadoop Ecosystem
The Hadoop ecosystem is an intricate assembly of tools and frameworks designed to facilitate the storage, processing, and analysis of big data. At its core lies Hadoop itself, which consists of several key components that work together to create a robust environment for handling massive datasets. Understanding these components is important for Java developers aiming to leverage Hadoop’s capabilities effectively.
Hadoop’s architecture is primarily composed of two significant components: the Hadoop Distributed File System (HDFS) and the MapReduce programming model. HDFS is responsible for the storage of data across a cluster of machines, ensuring high availability and fault tolerance. It operates on the principle of distributing data into blocks, which are then replicated across multiple nodes. This redundancy allows for seamless access and processing, even in the event of hardware failures.
HDFS Overview:
HDFS is designed to handle large files and is optimized for streaming data access. It employs a master/slave architecture, where the NameNode acts as the master server, managing metadata and namespace, while DataNodes serve as the workers, storing the actual data blocks. This separation of concerns allows for efficient data retrieval and management.
The MapReduce model, on the other hand, is a programming paradigm that processes large datasets in parallel across a Hadoop cluster. It breaks down tasks into two primary phases: the Map phase, where data is processed and transformed into key-value pairs, and the Reduce phase, where these pairs are aggregated to generate meaningful results. Java developers can easily write MapReduce applications using the provided Java API, as illustrated in the earlier example of the WordCount program.
Complementary Tools:
The Hadoop ecosystem encompasses several complementary projects that enhance its capabilities. Notable among these are:
- A data warehousing solution that provides a SQL-like interface for querying data stored in HDFS. It allows Java developers to run complex queries without needing to write low-level MapReduce code.
- A NoSQL database that operates on top of HDFS, providing real-time read/write access to large datasets. HBase is particularly useful for applications requiring random access to data.
- A high-level platform for creating programs that run on Hadoop. Pig Latin, its scripting language, simplifies the development of data processing tasks, enabling Java developers to focus on logic rather than the underlying complexity of MapReduce.
- A centralized service for maintaining configuration information, distributed synchronization, and providing group services. It plays an important role in managing the various services in a Hadoop cluster.
- A workflow scheduler for Hadoop jobs, enabling developers to manage complex job sequences and dependencies effectively.
These components together create a powerful ecosystem that allows Java developers to build scalable, fault-tolerant applications capable of processing terabytes or even petabytes of data. By mastering the Hadoop ecosystem, developers can harness the full potential of big data technologies, turning raw data into actionable insights with relative ease.
Introduction to Apache Spark
Apache Spark represents a significant evolution in big data processing. Unlike Hadoop’s MapReduce, which processes data in batches, Spark is designed for speed and efficiency through in-memory processing. This capability allows it to perform operations on large datasets much faster than its predecessors. Spark’s architecture is inherently flexible, enabling developers to write applications in various programming languages, including Java, Scala, and Python, while still providing robust support for Java-based systems.
At the core of Spark’s functionality are the concepts of Resilient Distributed Datasets (RDDs). RDDs are the fundamental data structures in Spark, allowing developers to perform transformations and actions on distributed data across a cluster seamlessly. This abstraction simplifies the complexities of managing distributed data while providing fault tolerance and scalability.
Java developers can leverage Spark’s powerful APIs to implement distributed data processing easily. Here’s a basic example demonstrating how to create an RDD, perform a transformation, and execute an action:
import org.apache.spark.api.java.JavaRDD; import org.apache.spark.api.java.JavaSparkContext; import org.apache.spark.SparkConf; import java.util.Arrays; public class SparkTransformationExample { public static void main(String[] args) { SparkConf conf = new SparkConf().setAppName("SparkJavaTransformation").setMaster("local"); JavaSparkContext sc = new JavaSparkContext(conf); // Create an RDD from a list of integers JavaRDD numbers = sc.parallelize(Arrays.asList(1, 2, 3, 4, 5)); // Perform a transformation: square each number JavaRDD squaredNumbers = numbers.map(num -> num * num); // Execute an action: collect and print the results squaredNumbers.collect().forEach(System.out::println); sc.close(); } }
In this example, we create a Spark application that initializes a Spark context and generates a simple RDD from a list of integers. The map
transformation is applied to square each number in the RDD, and finally, we collect the results back to the driver program to print them out.
One of the standout features of Spark is its ability to handle both batch and stream processing. This means that developers can work with real-time data ingestion alongside traditional batch workloads, making Spark an excellent choice for applications requiring immediate data processing and analytics.
Moreover, Spark provides extensive libraries and integrations for various big data tasks, such as:
- Enables querying structured data using SQL or DataFrame API, making it easier for analysts and data engineers to work with big data.
- A machine learning library that provides scalable algorithms and utilities for building machine learning pipelines.
- A library for graph processing, allowing for effective manipulation and analysis of graph data.
- Facilitates real-time stream processing, enabling applications to process live data as it arrives.
By using these libraries, Java developers can address a wide range of big data challenges without having to switch contexts or learn multiple frameworks, streamlining the development process and enhancing productivity.
Apache Spark offers a dynamic and powerful framework for big data processing, retaining the advantages of Java while introducing innovative features that cater to modern data processing needs. Its flexibility, speed, and broad ecosystem make it an indispensable tool in the toolkit of any Java developer working in the context of big data.
Comparing Hadoop and Spark
When comparing Hadoop and Apache Spark, it’s essential to understand their distinct architectures, processing models, and use cases. Both frameworks are integral to the big data landscape, but they cater to different needs and performance requirements, making them suitable for various scenarios.
Hadoop is primarily based on the MapReduce programming model, which processes data in batches. This traditional approach works well for large-scale data processing tasks, where the workload can be divided into discrete units that can be processed independently. However, the batch-oriented nature of MapReduce can lead to inefficiencies, particularly for applications requiring iterative computations or low-latency processing. In contrast, Spark utilizes an in-memory processing model that significantly accelerates data retrieval and computation. This capability allows Spark to outperform Hadoop in scenarios where quick data access very important, such as machine learning tasks or interactive data analysis.
One of the key differentiators between the two frameworks is fault tolerance. While both Hadoop and Spark handle failures gracefully, they do so in different ways. Hadoop’s resilience comes from its reliance on HDFS, which replicates data across multiple nodes. If a node fails, the system can retrieve the data from a replica, allowing the MapReduce job to continue running. Spark, on the other hand, employs a mechanism called lineage, which tracks the transformations applied to data through its RDDs. If a partition of an RDD is lost, Spark can recompute it using the original data and the transformations previously applied, making the recovery process efficient.
Another important aspect to think is the programming model. Java developers familiar with Hadoop will find the MapReduce paradigm simpler but can encounter a steep learning curve when transitioning to Spark’s RDD and DataFrame abstractions. Spark provides a more expressive API, enabling developers to write complex data processing tasks with less boilerplate code. This higher-level abstraction allows for a more intuitive coding style, particularly for operations that involve filtering, grouping, or joining datasets.
In terms of ecosystem support, Spark excels with its extensive set of libraries for machine learning (MLlib), stream processing (Spark Streaming), and graph processing (GraphX). These libraries enable Java developers to tackle diverse big data challenges without needing to integrate multiple separate tools. Conversely, while Hadoop has complementary projects like Apache Hive and Apache Pig that simplify data processing and querying, the integration tends to be less seamless compared to Spark’s unified approach.
import org.apache.spark.api.java.JavaSparkContext; import org.apache.spark.SparkConf; public class SparkVsHadoop { public static void main(String[] args) { SparkConf sparkConf = new SparkConf().setAppName("SparkVsHadoop").setMaster("local"); JavaSparkContext sparkContext = new JavaSparkContext(sparkConf); // Example: Using Spark to count occurrences of a word more efficiently long count = sparkContext.textFile("input.txt") .filter(line -> line.contains("Java")) .count(); System.out.println("Occurrences of 'Java': " + count); sparkContext.close(); } }
Ultimately, the choice between Hadoop and Spark hinges on the specific requirements of the project. For batch processing of large datasets where latency is not a critical concern, Hadoop remains a solid choice. However, for applications that demand speed, flexibility, and the ability to process both batch and stream data, Spark provides a compelling alternative. Understanding the strengths and limitations of each framework allows Java developers to make informed decisions that align with their project goals and performance needs.
Integrating Java with Hadoop and Spark
Integrating Java with big data technologies like Hadoop and Spark opens up a world of possibilities for developers aiming to leverage vast amounts of data efficiently. The integration process involves using the respective APIs provided by these frameworks to write applications that can perform complex data processing tasks seamlessly. Both Hadoop and Spark have their own Java APIs, making it simpler for Java developers to transition into the big data realm.
When working with Hadoop, the integration typically begins with setting up a Hadoop environment. This involves configuring HDFS and the MapReduce framework so that Java applications can communicate with the distributed file system and execute jobs across the cluster. The Hadoop API offers several classes and interfaces that Java developers can use to submit jobs, manage data, and handle the input and output formats required for processing. Below is an example of a simple Java program that integrates with Hadoop to read data from HDFS and perform a basic word count.
import org.apache.hadoop.conf.Configuration; import org.apache.hadoop.fs.FileSystem; import org.apache.hadoop.fs.Path; import org.apache.hadoop.io.IOUtils; import org.apache.hadoop.io.Text; import org.apache.hadoop.mapreduce.Job; import org.apache.hadoop.mapreduce.Mapper; import org.apache.hadoop.mapreduce.Reducer; import java.io.BufferedReader; import java.io.InputStream; import java.io.InputStreamReader; import java.io.IOException; public class HDFSWordCount { public static class TokenizerMapper extends Mapper
Spark, on the other hand, provides a more simple to operate approach to integration, focusing on in-memory data processing. To start using Spark with Java, developers need to set up a Spark context that serves as the entry point for all Spark functionality. This context can be configured to connect to local or cluster modes, depending on the scale of the application. The following example demonstrates how to load data from HDFS and perform transformations using the Spark Java API:
import org.apache.spark.api.java.JavaRDD; import org.apache.spark.api.java.JavaSparkContext; import org.apache.spark.SparkConf; import java.util.Arrays; public class SparkIntegrationExample { public static void main(String[] args) { SparkConf conf = new SparkConf().setAppName("SparkIntegrationExample").setMaster("local"); JavaSparkContext sc = new JavaSparkContext(conf); // Load data from HDFS JavaRDD input = sc.textFile("hdfs://path/to/input.txt"); // Perform transformations: filter and map JavaRDD wordCounts = input.flatMap(line -> Arrays.asList(line.split(" ")).iterator()) .map(word -> 1) .reduceByKey(Integer::sum); // Collect and print the results wordCounts.collect().forEach(count -> System.out.println("Count: " + count)); sc.close(); } }
In both cases, integrating Java with these frameworks allows developers to harness the power of distributed computing effectively. Hadoop excels in batch processing scenarios, making it suitable for long-running jobs that handle massive datasets. In contrast, Spark’s ability to process data in memory provides speed advantages, especially in iterative algorithms and real-time data processing tasks. Java developers can choose the framework that best suits their application’s requirements, ensuring that they can deliver robust, scalable solutions in a big data environment.
Best Practices for Java Development in Big Data Environments
When developing Java applications for big data environments, adhering to best practices very important for ensuring scalability, maintainability, and performance. As the landscape of big data technologies continues to evolve, Java developers must adapt their approaches to meet the unique challenges posed by large-scale data processing.
1. Optimize Data Serialization: Efficient data serialization is critical in big data applications, especially when transferring data across the network or between different components of the architecture. Java developers should consider using efficient serialization formats like Protocol Buffers, Avro, or Thrift. These formats not only reduce the amount of data transmitted but also improve the speed of data processing. Below is an example of how to use Avro for serialization:
import org.apache.avro.Schema; import org.apache.avro.generic.GenericData; import org.apache.avro.generic.GenericRecord; import org.apache.avro.io.DatumWriter; import org.apache.avro.io.Encoder; import org.apache.avro.io.EncoderFactory; import org.apache.avro.io.EncoderFactory; import org.apache.avro.specific.SpecificDatumWriter; import java.io.ByteArrayOutputStream; public class AvroSerializationExample { public static void main(String[] args) throws Exception { String schemaString = "{"type":"record","name":"User","fields":[{"name":"name","type":"string"},{"name":"age","type":"int"}]}"; Schema schema = new Schema.Parser().parse(schemaString); GenericRecord user = new GenericData.Record(schema); user.put("name", "Alice"); user.put("age", 30); // Serialize this record DatumWriter writer = new SpecificDatumWriter(schema); ByteArrayOutputStream outputStream = new ByteArrayOutputStream(); Encoder encoder = EncoderFactory.get().binaryEncoder(outputStream, null); writer.write(user, encoder); encoder.flush(); byte[] serializedData = outputStream.toByteArray(); System.out.println("Serialized user data: " + serializedData); } }
2. Leverage Configuration Management: Proper configuration management is essential for maintaining a big data application. Using libraries like Apache Commons Configuration or Spring Framework can help manage configurations effectively, enabling developers to handle different environments (development, testing, production) seamlessly. Centralizing configuration management reduces the chance of errors and simplifies updates. Here’s a snippet demonstrating the use of Apache Commons Configuration:
import org.apache.commons.configuration2.Configuration; import org.apache.commons.configuration2.builder.FileBasedBuilderParameters; import org.apache.commons.configuration2.builder.DefaultConfigurationBuilder; import org.apache.commons.configuration2.ex.ConfigurationException; import java.io.File; public class ConfigExample { public static void main(String[] args) throws ConfigurationException { DefaultConfigurationBuilder builder = new DefaultConfigurationBuilder(); builder.configure(new File("config.xml")); Configuration config = builder.getConfiguration(); String dbUrl = config.getString("database.url"); System.out.println("Database URL: " + dbUrl); } }
3. Manage Dependencies Wisely: With the complex nature of big data applications, managing dependencies effectively is vital. Using build tools like Maven or Gradle can simplify dependency management and ensure that the correct versions of libraries are included in the project. This practice helps avoid conflicts and enables developers to leverage the latest features and security updates in their libraries.
4. Implement Robust Error Handling: Big data applications can encounter various errors during processing, from data inconsistencies to network failures. Implementing a robust error-handling strategy is important to ensure that the application can recover gracefully from unexpected issues. Using try-catch blocks, logging frameworks like Log4j or SLF4J, and custom exception classes can help manage errors effectively. Here’s an example of a custom exception:
public class DataProcessingException extends Exception { public DataProcessingException(String message) { super(message); } public DataProcessingException(String message, Throwable cause) { super(message, cause); } }
5. Monitor Performance and Resource Usage: Performance monitoring is an ongoing process in big data development. Tools like Apache Spark’s UI, Hadoop’s ResourceManager, or third-party monitoring solutions can provide insights into resource usage, job performance, and bottlenecks. Integrating metrics and logging into the application can also help in diagnosing issues and improving performance over time.
6. Optimize for Parallelism: Big data frameworks are designed to leverage parallel processing. Java developers should write code with parallelism in mind, using features from the frameworks like Spark’s transformations and actions that apply operations in parallel across the dataset. Avoiding operations that require single-threaded execution or excessive shuffling of data can significantly improve performance.
By adhering to these best practices, Java developers can build robust, efficient big data applications that can handle massive datasets with ease, ensuring that their applications remain responsive and scalable in an ever-changing landscape.