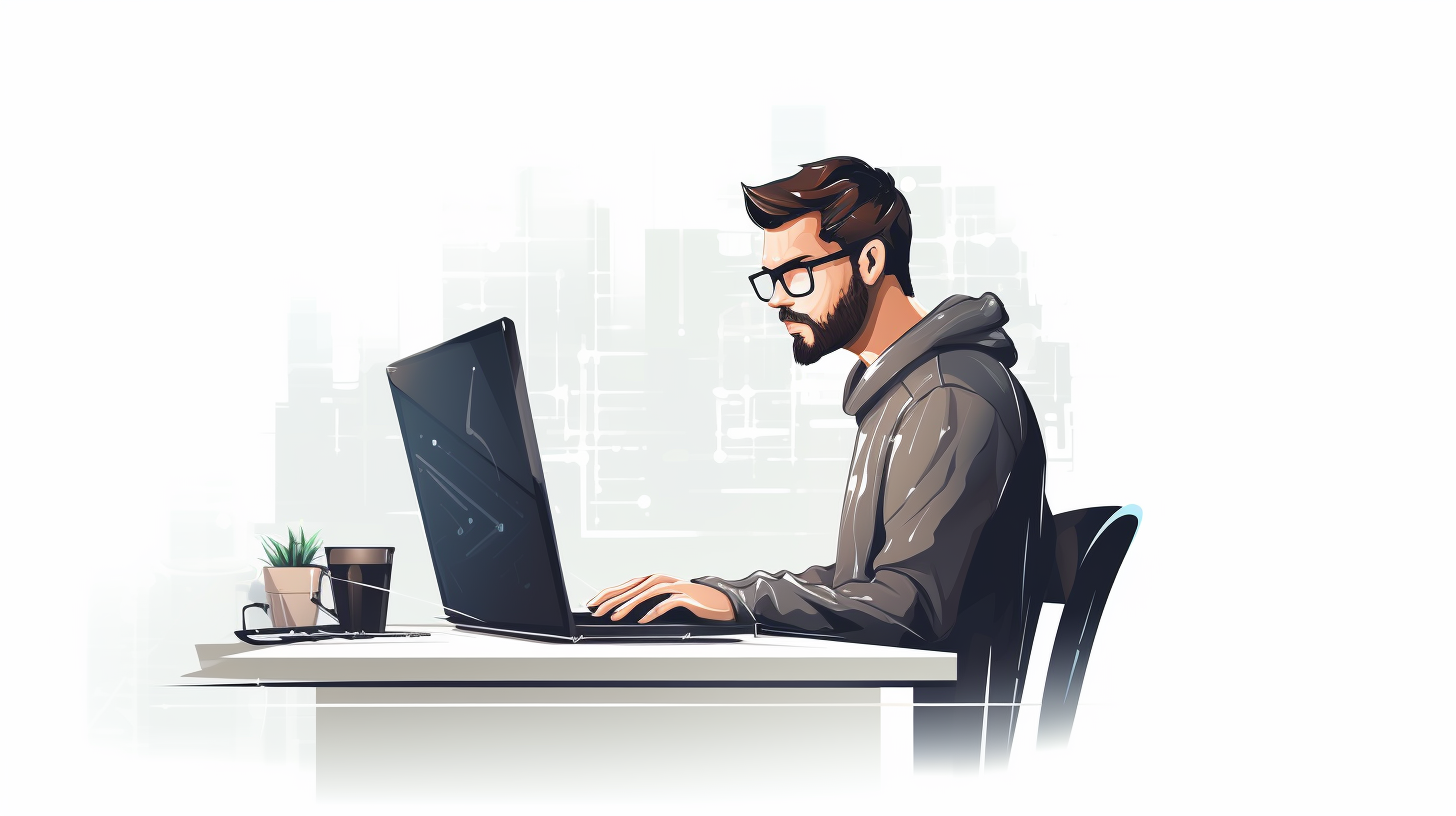
Java and Cloud Native Applications
Cloud Native Architecture is fundamentally about designing applications that leverage the vast capabilities of the cloud environment. In this paradigm, applications are built as a collection of loosely coupled services, enabling scalability, resilience, and rapid deployment. At its core, Cloud Native means making full use of cloud computing’s on-demand resources, elasticity, and flexibility.
To appreciate Cloud Native Architecture, one must first understand its key characteristics:
- Cloud Native applications are typically composed of microservices, which are small, independently deployable services that communicate over networks. Each microservice encapsulates a specific business function and can be developed, deployed, and scaled independently.
- Container technology, such as Docker, is often used to package microservices. This allows for consistent environments across development, testing, and production, as well as enabling efficient resource use.
- Cloud Native applications make use of orchestration tools like Kubernetes to manage the life cycle of containers, automate deployment and scaling, and facilitate self-healing capabilities.
- Cloud Native promotes a DevOps culture, where development and operations teams collaborate throughout the application lifecycle, from coding through production, enhancing efficiency and reducing time-to-market.
Java, with its robust ecosystem and extensive community support, plays a pivotal role in Cloud Native development. Frameworks like Spring Boot and Micronaut are designed specifically for building microservices, providing various features such as dependency injection, configuration management, and easy integration with cloud services. They allow developers to focus on writing business logic while abstracting the complexities associated with cloud environments.
Think the following Java code that utilizes Spring Boot to create a simple RESTful microservice:
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; @SpringBootApplication public class CloudNativeApp { public static void main(String[] args) { SpringApplication.run(CloudNativeApp.class, args); } } @RestController class GreetingController { @GetMapping("/greet") public String greet() { return "Hello, Cloud Native World!"; } }
In this example, we define a basic Spring Boot application that starts a web server and exposes a single endpoint. Navigating to “/greet” promises a greeting message, showcasing how simply a Java application can be tailored for the cloud.
Another crucial aspect of Cloud Native Architecture is the emphasis on resilience and fault tolerance. Instead of building monolithic applications that may have single points of failure, Cloud Native encourages designing systems that can gracefully handle failures. This can be achieved through techniques like circuit breakers, bulkheads, and service mesh architectures.
Additionally, monitoring and observability are vital for ensuring the health of Cloud Native applications. Tools like Prometheus, Grafana, and ELK Stack enable developers to track metrics, logs, and traces, providing insights into application performance and facilitating timely intervention when necessary.
Embracing Cloud Native Architecture enables developers to leverage the cloud’s elasticity, scalability, and resilience, while Java provides a rich framework to build robust, microservice-based applications. As the landscape of software development evolves, understanding and implementing these principles will be essential for creating modern applications that meet the demands of today’s digital world.
Key Java Frameworks for Cloud Native Development
When it comes to developing Cloud Native applications in Java, several frameworks stand out, each offering unique features tailored to facilitate the creation of scalable and resilient microservices. Key among these are Spring Boot, Micronaut, and Quarkus. Each of these frameworks not only enhances productivity but also aligns with cloud principles, making them quintessential tools for developers aiming to harness the power of the cloud.
Spring Boot has become synonymous with microservices development in the Java ecosystem. It builds on the Spring framework, simplifying the setup and configuration of new applications. With its convention-over-configuration philosophy, Spring Boot allows developers to get up and running quickly, with minimal boilerplate code. A notable feature is its auto-configuration capability, which intelligently sets up the application context based on the dependencies present in the classpath. This means developers can focus on writing business logic rather than wrestling with configurations.
Here’s an example of a Spring Boot application that connects to a database and exposes CRUD operations for a simple entity:
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.*; import java.util.List; @RestController @RequestMapping("/api/items") public class ItemController { @Autowired private ItemService itemService; @GetMapping public List getAllItems() { return itemService.findAll(); } @PostMapping public Item createItem(@RequestBody Item item) { return itemService.save(item); } }
In this code snippet, we create a REST controller for managing items. The dependency injection provided by Spring reduces coupling and enhances testability.
Micronaut is another modern framework tailored for building Cloud Native applications. It prides itself on being lightweight and offering fast startup times, making it ideal for microservices. Micronaut uses a compile-time dependency injection mechanism, which means there’s no reflection at runtime, leading to lower memory consumption and faster execution. That is particularly beneficial in a microservices architecture where resources are often constrained.
To demonstrate Micronaut’s capabilities, ponder the following example of a simple REST service:
import io.micronaut.http.annotation.Controller; import io.micronaut.http.annotation.Get; @Controller("/api/greet") public class GreetingController { @Get public String greet() { return "Hello from Micronaut!"; } }
In this example, a Micronaut controller is defined to handle HTTP GET requests. The simplicity and efficiency of Micronaut allow for rapid application development without sacrificing performance.
Quarkus is yet another framework that has garnered attention for its focus on Kubernetes-native development. It’s designed for Java virtual machines and provides a reactive programming model to support asynchronous communication between services. Quarkus optimizes Java specifically for containers, boasting features such as live reloading, which enhances developer productivity during local development. Quarkus applications can also be packaged as native executables using GraalVM, which dramatically reduces startup time and memory footprint.
Here’s a brief example of a Quarkus REST resource:
import javax.ws.rs.GET; import javax.ws.rs.Path; @Path("/api/hello") public class HelloResource { @GET public String hello() { return "Hello from Quarkus!"; } }
This Quarkus resource demonstrates the ease of creating cloud-ready microservices, emphasizing performance and efficiency, which are crucial in cloud environments.
The choice of framework can greatly influence the ease and efficiency of Cloud Native development in Java. By using the strengths of Spring Boot, Micronaut, or Quarkus, developers can build robust, maintainable, and scalable microservices that fully exploit the capabilities of the cloud. Each framework offers unique advantages, and the best choice often depends on specific project requirements and team familiarity. As the demand for Cloud Native applications continues to grow, mastering these frameworks will be essential for Java developers looking to stay relevant in the industry.
Best Practices for Building Scalable Java Applications
Building scalable Java applications in a Cloud Native environment requires a careful consideration of several best practices. These practices not only enhance performance but also ensure that the application can handle varying loads while maintaining availability and reliability. One of the most critical aspects is to adopt a microservices architecture, which divides the application into smaller, manageable services. Each service can be scaled independently based on demand, allowing for efficient use of resources.
Stateless Services: When designing microservices, aim to keep them stateless. A stateless service does not retain client session information between requests, which simplifies scaling. For example, if a service needs to handle a surge of traffic, you can conveniently add more instances of the service without worrying about session data. Consider the following code snippet that illustrates a stateless service using Spring Boot:
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; @SpringBootApplication public class StatelessService { public static void main(String[] args) { SpringApplication.run(StatelessService.class, args); } } @RestController class HelloController { @GetMapping("/hello") public String sayHello() { return "Hello, that is a stateless service!"; } }
In this example, the service responds to requests without storing any session state. This especially important for horizontal scaling, as multiple instances can handle requests independently.
Load Balancing: Implementing load balancing is essential to distribute incoming traffic evenly across instances of your services. This prevents any single instance from becoming a bottleneck. You can use cloud-native load balancers such as AWS Elastic Load Balancer or Kubernetes Ingress controllers to manage traffic effectively. The following snippet shows how to configure a simple load balancer in Kubernetes:
apiVersion: v1 kind: Service metadata: name: my-service spec: selector: app: my-app ports: - protocol: TCP port: 80 targetPort: 8080 type: LoadBalancer
This YAML configuration defines a service that will distribute traffic to multiple pod instances of your application, enhancing availability and responsiveness.
Caching: To enhance performance, consider implementing caching mechanisms. Caches can significantly reduce response times by storing frequently accessed data in memory. Java developers can utilize libraries like Caffeine or integrate in-memory data grids, such as Hazelcast or Redis. Here’s an example of how a simple in-memory cache can be implemented using Caffeine:
import com.github.benmanes.caffeine.cache.Cache; import com.github.benmanes.caffeine.cache.Caffeine; import java.util.concurrent.TimeUnit; public class CacheExample { private final Cache cache = Caffeine.newBuilder() .expireAfterWrite(10, TimeUnit.MINUTES) .maximumSize(100) .build(); public String getData(String key) { return cache.get(key, k -> fetchDataFromDatabase(k)); } private String fetchDataFromDatabase(String key) { // Simulate a database fetch return "Data for " + key; } }
This code demonstrates a simple caching strategy that fetches data from a hypothetical database, caching the result for faster subsequent access. Caching can drastically reduce load times and database calls, directly impacting application scalability.
Asynchronous Processing: To further enhance scalability, implement asynchronous processing wherever possible. Java provides robust support for asynchronous programming, particularly with libraries like CompletableFuture or reactive frameworks such as Project Reactor. By offloading long-running tasks, you ensure that your application can handle more concurrent requests. The following example showcases an asynchronous method using CompletableFuture:
import java.util.concurrent.CompletableFuture; public class AsyncService { public CompletableFuture performTask() { return CompletableFuture.supplyAsync(() -> { // Simulate a long-running task try { Thread.sleep(2000); } catch (InterruptedException e) { Thread.currentThread().interrupt(); } return "Task completed"; }); } }
This code illustrates how a task can be executed asynchronously, freeing up the main thread to respond to other requests. This approach significantly boosts the application’s ability to handle high loads.
Monitoring and Logging: Finally, adopt comprehensive monitoring and logging practices to maintain application health and performance. Utilize tools like Prometheus, Grafana, and ELK Stack to track metrics and logs. This allows you to proactively detect issues before they escalate. Here’s a basic example of how to log application events using SLF4J with Logback:
import org.slf4j.Logger; import org.slf4j.LoggerFactory; public class LoggingExample { private static final Logger logger = LoggerFactory.getLogger(LoggingExample.class); public void process() { logger.info("Processing started"); // business logic logger.info("Processing completed"); } }
By implementing these logging best practices, you ensure that you have the necessary insights to diagnose problems and optimize performance, which is critical for scalable applications.
By adhering to these best practices—creating stateless services, employing load balancing, implementing caching, using asynchronous processing, and monitoring your applications—you can build Java applications that simply scale in a Cloud Native environment. Each of these strategies plays a pivotal role in ensuring that your applications not only meet the demands of today’s users but are also resilient and maintainable in the long run.
Integrating Microservices with Java in the Cloud
Integrating microservices in Java within a cloud environment is a multifaceted endeavor that involves various design and implementation strategies to ensure that these services communicate efficiently, remain resilient, and can be deployed independently. One of the primary benefits of adopting a microservices architecture is the ability to develop and deploy services that are loosely coupled yet highly cohesive. This allows teams to work on individual services concurrently without causing disruptions to one another, ultimately accelerating the development lifecycle.
At the heart of microservice integration lies the concept of communication protocols. RESTful APIs are the most common choice due to their simplicity and widespread adoption. However, as systems grow in complexity, alternatives like gRPC or message brokers (e.g., Apache Kafka, RabbitMQ) may provide better performance and reliability. With REST, for instance, services expose endpoints that clients can call to perform operations. Here’s how you might implement a simple service that interacts with another microservice using REST:
import org.springframework.web.bind.annotation.*; import org.springframework.web.client.RestTemplate; @RestController @RequestMapping("/api/products") public class ProductController { private final RestTemplate restTemplate; public ProductController(RestTemplate restTemplate) { this.restTemplate = restTemplate; } @GetMapping("/{id}") public Product getProduct(@PathVariable String id) { return restTemplate.getForObject("http://product-service/api/products/" + id, Product.class); } }
In this example, the ProductController
class uses a RestTemplate
to fetch product details from a remote service. This showcases how microservices can communicate over HTTP, allowing for a clear separation of concerns.
Another fundamental aspect of integrating microservices is handling service discovery. In a microservices architecture, services need to dynamically locate each other, especially as instances scale up or down based on load. Tools like Netflix Eureka or Spring Cloud Consul facilitate this by enabling services to register themselves and discover others at runtime. Below is a simple configuration example using Spring Cloud:
import org.springframework.cloud.netflix.eureka.EnableEurekaClient; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication @EnableEurekaClient public class Application { public static void main(String[] args) { SpringApplication.run(Application.class, args); } }
In this code, the application is annotated with @EnableEurekaClient
, allowing it to register itself with an Eureka server. This integration especially important for building resilient architectures that can adapt to changes in the environment.
Resilience in microservices is equally paramount, particularly in the face of inevitable service failures. Implementing a circuit breaker pattern can prevent cascading failures across services. Libraries like Resilience4j offer simpler implementations of this pattern. A simple usage of a circuit breaker in a service might look like this:
import io.github.resilience4j.circuitbreaker.annotation.CircuitBreaker; import org.springframework.web.bind.annotation.*; @RestController @RequestMapping("/api/orders") public class OrderController { @CircuitBreaker @GetMapping("/{id}") public Order getOrder(@PathVariable String id) { return orderService.findOrderById(id); } }
With the above annotation, if the findOrderById
method fails repeatedly, the circuit breaker will open, preventing further calls to the failing service until it recovers. This ensures that individual service failures do not bring down the entire system.
Asynchronous messaging is also a powerful approach for integrating microservices, particularly in event-driven architectures. By using message brokers, services can publish and subscribe to events without the need for direct HTTP calls, thereby enhancing decoupling. A simple example using Spring’s messaging support might look like this:
import org.springframework.messaging.Message; import org.springframework.stereotype.Service; import org.springframework.kafka.annotation.KafkaListener; @Service public class OrderEventListener { @KafkaListener(topics = "order-events") public void listen(Message message) { OrderEvent event = message.getPayload(); // process the event } }
This listener listens for messages on the “order-events” topic, allowing the application to react to order events, such as creation or updates, in a non-blocking manner. This decoupling is essential for maintaining high availability and responsiveness in microservice architectures.
Lastly, adequate monitoring and logging strategies are vital for maintaining microservices. Centralized logging and distributed tracing can help identify performance bottlenecks and trace requests as they traverse through multiple services. Using tools like ELK Stack or Jaeger can provide insights into application health and behavior. Here’s a basic example of how to implement logging with SLF4J across microservices:
import org.slf4j.Logger; import org.slf4j.LoggerFactory; public class Microservice { private static final Logger logger = LoggerFactory.getLogger(Microservice.class); public void processOrder(Order order) { logger.info("Processing order: {}", order.getId()); // processing logic logger.info("Order processed successfully"); } }
This logging provides crucial context for operations, enabling developers to trace issues back to their source effectively.
Integrating microservices in Java within a cloud environment demands a well-considered approach that encompasses communication protocols, service discovery, resilience patterns, event-driven architectures, and robust monitoring practices. By using these strategies, developers can build efficient, maintainable, and scalable systems that take full advantage of the cloud’s capabilities.
Monitoring and Managing Cloud Native Java Applications
Monitoring and managing cloud-native Java applications is a critical aspect of ensuring their reliability, performance, and scalability. In cloud environments, where resources can be dynamic and distributed, effective monitoring not only allows developers to gain insights into application behavior but also enables proactive responses to potential issues before they impact users. To achieve this, several strategies and tools can be implemented, focusing on observability, centralized logging, and alerting mechanisms.
Observability in cloud-native applications typically involves collecting metrics, logs, and traces. Metrics provide quantitative insights into application performance, while logs offer detailed records of events and errors. Traces help track the flow of requests through microservices, making it easier to diagnose performance bottlenecks.
One widely adopted method for monitoring Java applications is using the Micrometer library, which provides a simple facade over instrumentation clients for various monitoring systems. Here’s how you can use Micrometer to expose application metrics:
import io.micrometer.core.instrument.MeterRegistry; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; @RestController public class MetricsController { private final MeterRegistry meterRegistry; @Autowired public MetricsController(MeterRegistry meterRegistry) { this.meterRegistry = meterRegistry; } @GetMapping("/api/metrics") public String metrics() { meterRegistry.counter("api.requests.total").increment(); return "Metrics recorded"; } }
In this example, a counter metric for total API requests is incremented every time the `/api/metrics` endpoint is hit. This data can be sent to monitoring systems like Prometheus, enabling visualization through Grafana.
Centralized logging is another key component in monitoring cloud-native applications. Using a logging framework like Logback in combination with the ELK Stack (Elasticsearch, Logstash, Kibana) allows developers to aggregate logs from multiple services into a single platform for analysis. Here’s a simple Logback configuration to log events in JSON format:
localhost:5044
This configuration sends log messages to a Logstash instance, which processes and forwards them to Elasticsearch. Kibana can then be used to visualize and analyze these logs, providing powerful insights into application performance and error rates.
Alerting mechanisms complement monitoring by notifying teams of potential issues before they escalate. Using tools like Prometheus Alertmanager, you can define rules that trigger alerts based on metrics thresholds. Here’s an example of a basic alerting rule:
groups: - name: example-alert rules: - alert: HighErrorRate expr: rate(http_server_requests_seconds_count[5m]) > 0.05 for: 5m labels: severity: critical annotations: summary: "High error rate detected" description: "More than 5% of requests failed in the last 5 minutes."
This YAML configuration sets up an alert that triggers if the error rate exceeds 5% over a 5-minute window. Alerts can be sent to various notification channels, including email, Slack, or PagerDuty, ensuring that the development and operations teams are promptly informed of critical issues.
Distributed tracing is vital for understanding how requests flow through microservices. It allows developers to see how long requests take across different services, helping identify inefficiencies. Libraries like Spring Sleuth and OpenTelemetry can be used to instrument applications for distributed tracing. Here’s a simple example of using Spring Sleuth:
import org.springframework.web.bind.annotation.*; import org.springframework.cloud.sleuth.annotation.NewSpan; @RestController @RequestMapping("/api/orders") public class OrderController { @NewSpan @GetMapping("/{id}") public Order getOrder(@PathVariable String id) { // business logic return orderService.findOrderById(id); } }
By annotating the method with `@NewSpan`, Spring Sleuth creates a new trace span for each order retrieval request, allowing developers to analyze the performance and latency of requests through a centralized tracing system.
Monitoring and managing cloud-native Java applications effectively involves a combination of collecting metrics, centralized logging, alerting, and distributed tracing. By implementing these practices, developers can ensure that their applications remain performant and resilient in the cloud environment, leading to improved user experiences and reduced downtime.