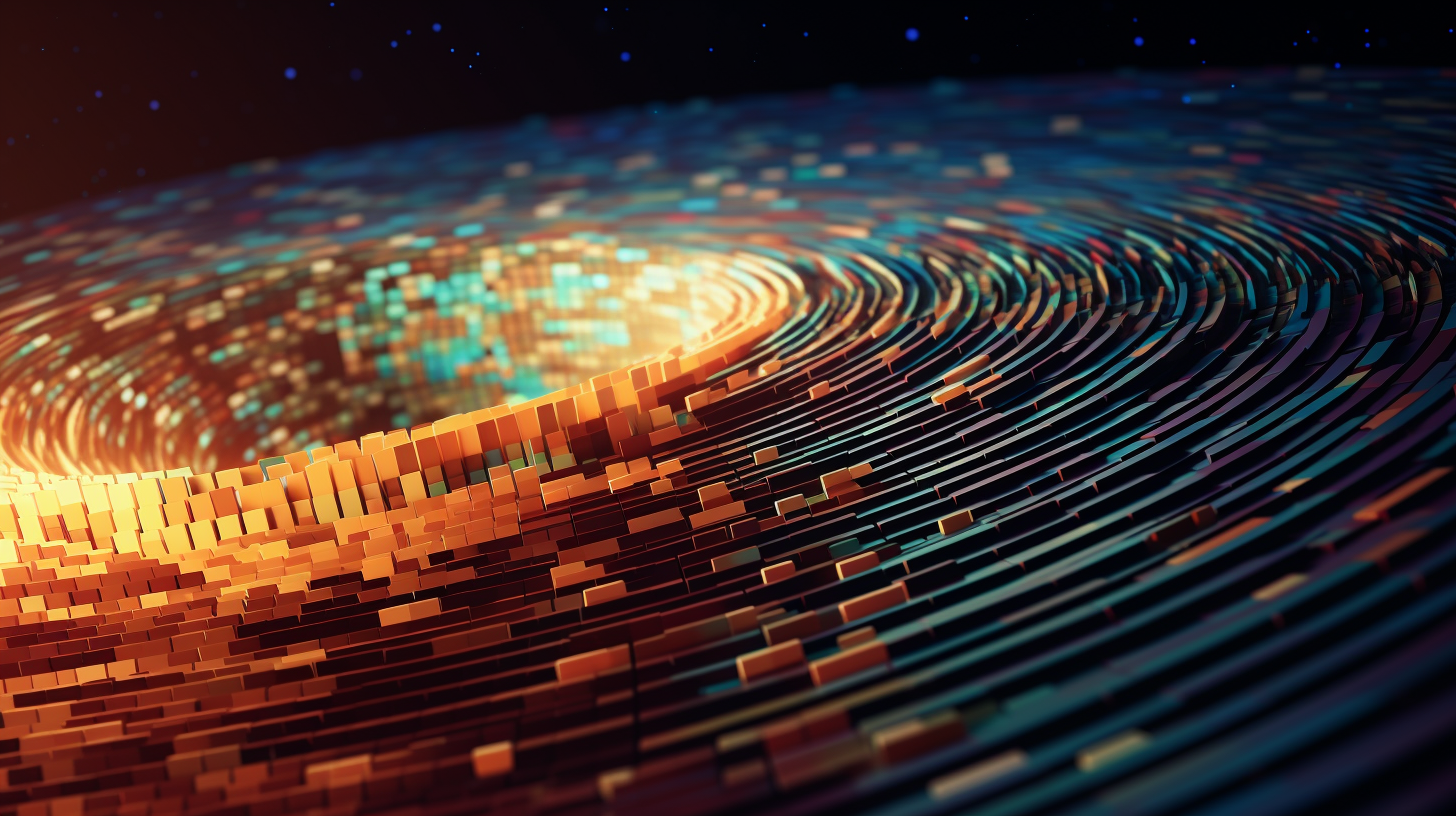
Java and Cross-Platform Development
The Java programming language has long been celebrated for its write-once-run-anywhere capability, positioning itself as a strong contender within the scope of cross-platform development. One of the primary benefits of cross-platform development in Java is the significant reduction in time and resources required for application development. Rather than developing separate codebases for different operating systems, developers can create a single codebase that functions seamlessly across various platforms.
Another significant advantage is the extensive ecosystem of libraries and frameworks that Java offers. This rich environment allows developers to leverage pre-built solutions, drastically accelerating the development process. For instance, using frameworks like Spring or JavaFX can simplify complex tasks, allowing developers to focus on building features rather than reinventing the wheel.
The Java Virtual Machine (JVM) is a cornerstone of its cross-platform capabilities. By compiling Java code into bytecode that runs on the JVM, Java ensures consistent performance regardless of the underlying architecture. This translates to fewer platform-specific bugs and a more stable user experience. It also allows teams to work collaboratively across different platforms without the fear of compatibility issues.
Furthermore, cross-platform development in Java enhances market reach. By targeting multiple operating systems—such as Windows, macOS, and Linux—developers can cater to a broader audience, which is important in an increasingly competitive software market. This broader accessibility often leads to higher user adoption rates, ultimately benefiting the application’s success.
Lastly, the community support and extensive documentation available for Java contribute to its viability as a cross-platform development tool. Developers can easily find solutions to common problems and share knowledge across forums and repositories, fostering an environment of collaboration and innovation.
Key Frameworks and Tools for Java Cross-Platform Applications
When discussing key frameworks and tools for Java cross-platform applications, it’s essential to recognize the variety of options available that facilitate the development process. Each framework or tool brings its own strengths, allowing developers to choose the solution that best fits their project requirements.
JavaFX stands out as a powerful framework for building rich client applications. It provides a modern UI toolkit that simplifies the creation of visually appealing applications. JavaFX supports the development of cross-platform applications by allowing developers to utilize FXML for designing user interfaces, which can be combined with Java code for application logic. Here’s a simple example demonstrating a JavaFX application:
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.layout.StackPane; import javafx.stage.Stage; public class HelloJavaFX extends Application { @Override public void start(Stage primaryStage) { Button btn = new Button("Say 'Hello, World'"); btn.setOnAction(event -> System.out.println("Hello, World!")); StackPane root = new StackPane(); root.getChildren().add(btn); Scene scene = new Scene(root, 300, 250); primaryStage.setTitle("JavaFX Example"); primaryStage.setScene(scene); primaryStage.show(); } public static void main(String[] args) { launch(args); } }
Another robust option is the Spring framework, particularly Spring Boot, which provides a rapid development environment for web applications. It abstracts much of the configuration and setup required in traditional Java web applications, allowing developers to focus on writing functional code. With Spring Boot, you can create stand-alone, production-grade Spring-based applications with minimal fuss. Here’s a simple RESTful service example:
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; @SpringBootApplication @RestController public class HelloSpringBoot { @GetMapping("/hello") public String sayHello() { return "Hello, World!"; } public static void main(String[] args) { SpringApplication.run(HelloSpringBoot.class, args); } }
Apache Cordova is another notable tool that enables the development of mobile applications using Java. By allowing developers to write applications in HTML, CSS, and JavaScript while using Java for back-end logic, it provides a bridge between web technologies and native device capabilities. This framework is particularly useful when targeting mobile platforms while maintaining a Java-centric codebase.
For those looking to build applications with a more game-oriented approach, LibGDX is an open-source framework that offers a unified environment for game development across multiple platforms, including desktop and mobile. It leverages Java’s capabilities while providing features tailored for graphics, physics, and input handling, making it a preferred choice for indie game developers.
Regarding integrated development environments (IDEs), IntelliJ IDEA and Eclipse are the heavyweights, providing extensive support for Java development. Both IDEs come equipped with tools that streamline the coding process, debugging, and testing in cross-platform environments. They also support a plethora of plugins that enhance functionality, which can be especially useful when working with different frameworks.
Best Practices for Developing Cross-Platform Java Applications
When developing cross-platform Java applications, adhering to best practices can dramatically enhance the maintainability, performance, and overall user experience of your software. These practices not only streamline the development process but also ensure that the final product is robust and adaptable across multiple platforms.
1. Use Platform-Independent Libraries
One of the first steps in cross-platform development is to utilize libraries that are designed to be platform-independent. By doing this, you minimize the risk of encountering platform-specific issues. Libraries like Apache Commons and JUnit provide functionalities that work seamlessly across different environments. For example, using Apache Commons for file manipulation can save you from dealing with file path differences across operating systems.
import org.apache.commons.io.FileUtils; import java.io.File; public class FileUtilExample { public static void main(String[] args) { File file = new File("test.txt"); try { FileUtils.writeStringToFile(file, "Hello, Cross-Platform!", "UTF-8"); } catch (IOException e) { e.printStackTrace(); } } }
2. Embrace the MVC Architecture
Embracing the Model-View-Controller (MVC) architecture can greatly enhance the organization of your code. MVC separates the application logic into three interconnected components, which fosters modularity and makes your application easier to maintain and test. This structure is particularly beneficial in UI frameworks like JavaFX, where the separation of UI and logic can lead to cleaner code and a better user experience.
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.layout.StackPane; import javafx.stage.Stage; public class MVCPatternExample extends Application { private Model model; private View view; @Override public void start(Stage primaryStage) { model = new Model(); view = new View(model); view.setController(new Controller(model, view)); Scene scene = new Scene(view.getLayout(), 300, 250); primaryStage.setTitle("MVC Example"); primaryStage.setScene(scene); primaryStage.show(); } public static void main(String[] args) { launch(args); } }
3. Optimize for Performance
Performance optimization especially important in cross-platform applications. Java’s garbage collection can sometimes lead to performance issues if not properly managed. Developers should be mindful of memory usage, especially when dealing with large data sets. Using efficient data structures and minimizing object creation can help mitigate performance bottlenecks. Additionally, profiling tools such as VisualVM can be invaluable for identifying performance issues during development.
import java.util.HashMap; import java.util.Map; public class PerformanceExample { public static void main(String[] args) { Map map = new HashMap(); for (int i = 0; i < 100000; i++) { map.put("Key" + i, "Value" + i); } System.out.println("Map size: " + map.size()); } }
4. Thorough Testing Across Platforms
Comprehensive testing across different platforms is essential to ensure that your application behaves as expected. Automated testing frameworks like JUnit or TestNG should be employed to run tests on various operating systems. Additionally, using Continuous Integration (CI) tools like Jenkins can automate the testing process, allowing for more frequent and efficient testing cycles.
import org.junit.Test; import static org.junit.Assert.assertEquals; public class CrossPlatformTest { @Test public void testGreeting() { String expected = "Hello, Cross-Platform!"; assertEquals(expected, "Hello, Cross-Platform!"); } }
5. Maintain Consistency in UI/UX
Lastly, maintaining a consistent user interface and user experience across platforms is vital. This can be achieved by adhering to design guidelines specific to each platform while ensuring that the core functionality remains the same. Frameworks like JavaFX provide tools to create responsive UIs, which can dynamically adjust according to the platform. Testing on various devices and resolutions is also crucial to ensure a seamless experience.
Challenges and Solutions in Java Cross-Platform Development
Despite the a high number of benefits that Java brings to cross-platform development, several challenges can complicate the process. Developers often encounter issues related to compatibility, performance, user interface consistency, and the complexities of managing diverse environments. However, understanding these challenges and implementing appropriate solutions can significantly enhance the development experience.
One of the predominant challenges is the compatibility of various Java versions across different platforms. While Java is designed to be platform-independent, differences in the implementation of the Java Virtual Machine (JVM) can lead to inconsistencies. For instance, certain features may be available in newer versions of Java but not supported in older versions that some users might still be using. To mitigate this, it is essential to carefully manage the Java version your application targets and provide clear documentation for users regarding system requirements.
To handle version differences, consider using build tools like Maven or Gradle, which allow you to specify dependencies and compile your application for a specific version of Java. For example, setting the Java version in your `pom.xml` file for Maven can ensure that your application behaves consistently:
<properties> <maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target> </properties>
Another challenge arises with performance. Cross-platform applications may suffer from performance issues due to the overhead of the JVM and differing system resources. For instance, applications may run smoothly on one platform but encounter latency or memory issues on another. Profiling tools such as VisualVM or JProfiler can help identify bottlenecks in your code. To optimize performance, prioritize efficient data structures and algorithms and avoid unnecessary object creation, as shown in the following example:
import java.util.ArrayList; import java.util.List; public class PerformanceOptimization { public static void main(String[] args) { List<String> list = new ArrayList<>(); for (int i = 0; i < 1000000; i++) { list.add("Item " + i); } System.out.println("List created with size: " + list.size()); } }
Maintaining user interface consistency across different platforms also poses a challenge. UI components that look and behave differently on various operating systems can lead to a disjointed user experience. To tackle this, utilize JavaFX’s CSS capabilities to style your applications consistently. Here’s a snippet showing how you can apply CSS to ensure a uniform appearance:
.button { -fx-background-color: #007BFF; -fx-text-fill: white; -fx-font-size: 14px; }
Furthermore, employing a responsive design approach can adapt the UI based on the platform and screen size, which improves usability. JavaFX allows you to create layouts that can change dynamically, catering to different resolutions and aspect ratios.
Lastly, managing dependencies and external libraries can become complex in cross-platform development. Each platform may have its own set of libraries that can present compatibility issues. To address this, ponder using a dependency management system like Maven or Gradle to manage versions and ensure that libraries are compatible with your application’s Java version.