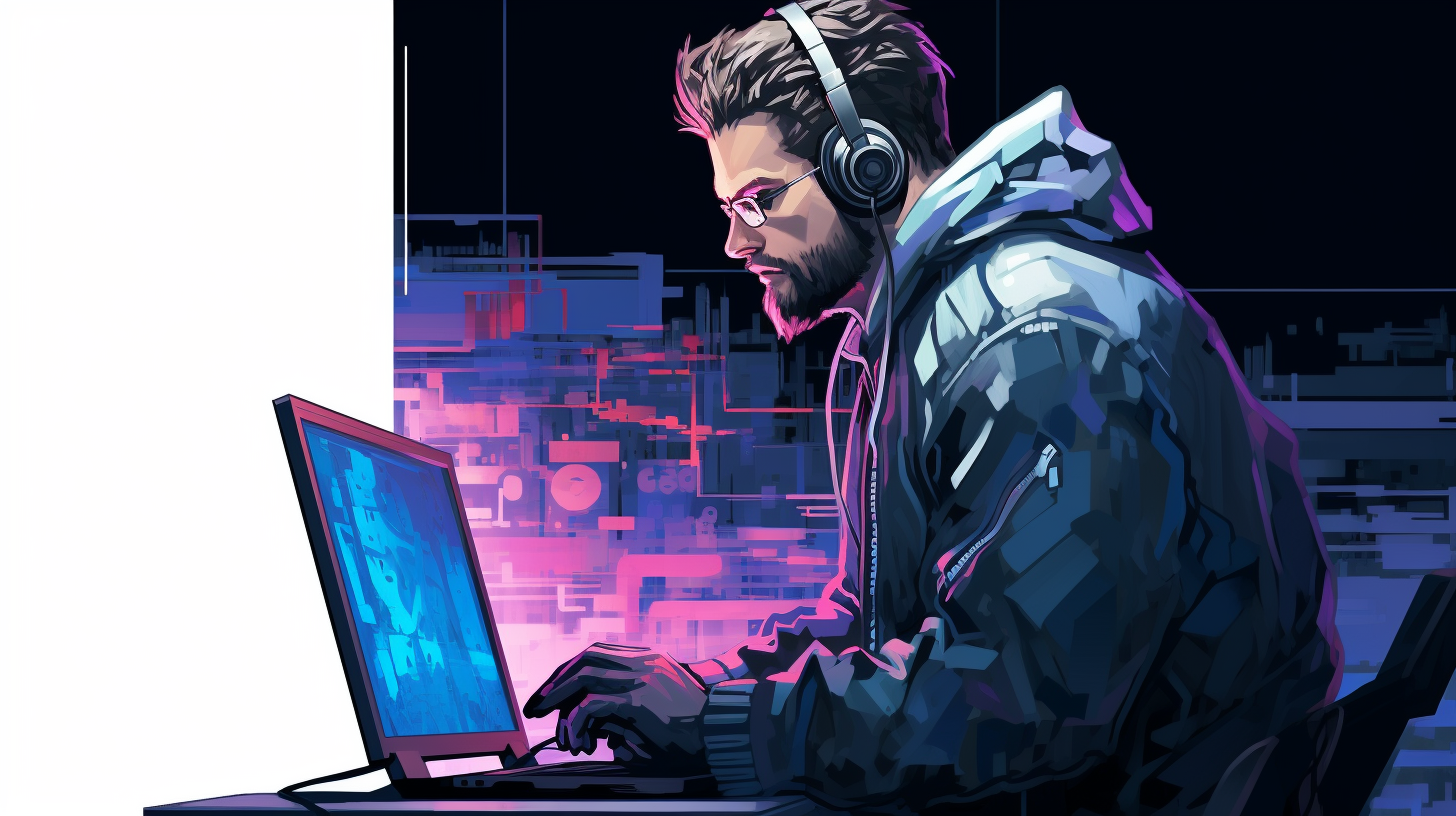
Java and Dependency Injection: Advanced Techniques
Dependency Injection (DI) is a design pattern used in Java that allows a programmer to remove hard-coded dependencies between classes, promoting greater flexibility and easier testing. By employing DI, you are essentially letting an external entity, often a framework or a container, manage the instantiation and binding of dependent components. This leads to decoupled code, enabling you to change implementations without significant refactoring.
At its core, DI revolves around the inversion of control (IoC) principle, where the control of creating and managing dependencies shifts from the class itself to an external entity. This makes your code more modular and reusable. The main idea is to inject the required dependencies during the creation of the object, rather than having the object construct them itself.
Think a simple example involving a service class that depends on a repository class. Without DI, the service class would instantiate the repository directly:
public class UserService { private UserRepository userRepository; public UserService() { this.userRepository = new UserRepository(); // Hard-coded dependency } public void createUser(String name) { userRepository.save(name); } }
This tight coupling makes it difficult to test UserService
in isolation, as it always creates a new instance of UserRepository
. With DI, you can inject the dependency through the constructor:
public class UserService { private UserRepository userRepository; public UserService(UserRepository userRepository) { // Dependency Injection via constructor this.userRepository = userRepository; } public void createUser(String name) { userRepository.save(name); } }
Now, the UserService
class is decoupled from UserRepository
. You can pass any implementation of UserRepository
when creating a UserService
instance, which is particularly useful for testing. For instance, you can create a mock repository when testing:
public class UserServiceTest { @Test public void testCreateUser() { UserRepository mockRepository = Mockito.mock(UserRepository.class); UserService userService = new UserService(mockRepository); userService.createUser("Alice"); Mockito.verify(mockRepository).save("Alice"); } }
In this example, the test becomes more simpler because you can control the behavior of the UserRepository
without relying on its actual implementation. By using DI, you enhance the testability of your code significantly.
Dependency Injection in Java champions the principles of loose coupling and high cohesion. It facilitates cleaner code architecture and better testing strategies, making it an integral part of modern Java development practices.
Types of Dependency Injection
When delving deeper into Dependency Injection (DI), it’s essential to understand the various types of DI commonly used in Java applications. The three primary types of Dependency Injection are Constructor Injection, Setter Injection, and Interface Injection. Each method serves the purpose of supplying dependencies to an object, but they differ in their approach and suitability for different scenarios.
Constructor Injection is perhaps the most common form of DI. In this approach, dependencies are provided to a class via its constructor. This method ensures that the class cannot be instantiated without its required dependencies, promoting immutability and ensuring that the object is in a fully initialized state immediately upon creation. Here’s an example:
public class PaymentService { private PaymentProcessor paymentProcessor; public PaymentService(PaymentProcessor paymentProcessor) { // Constructor Injection this.paymentProcessor = paymentProcessor; } public void processPayment(Double amount) { paymentProcessor.process(amount); } }
In this case, the PaymentService
class is dependent on a PaymentProcessor
, which is supplied through its constructor. This design makes it clear what dependencies are necessary for the class to function.
Setter Injection is another popular method. In this type, dependencies are provided through setter methods after the object is constructed. This allows for more flexibility, allowing you to change the dependencies at any point after the object is created. However, it can also lead to partially constructed objects if not managed carefully. Here’s how you might implement Setter Injection:
public class NotificationService { private MessageSender messageSender; public void setMessageSender(MessageSender messageSender) { // Setter Injection this.messageSender = messageSender; } public void notifyUser(String message) { messageSender.send(message); } }
In the above example, the NotificationService
class can have its MessageSender
set after it has been instantiated, allowing for more dynamic behavior, but it also requires that the developer remembers to set the dependency before usage.
Interface Injection is a less common form of DI that uses interfaces to inject dependencies. With this approach, the dependency provides an implementation of an interface that the client class requires. However, this method is less favored in practice compared to the first two due to its added complexity and potential for misuse. Here’s a simplistic illustration:
public interface Initializable { void init(Dependency dependency); } public class MyClass implements Initializable { private Dependency dependency; @Override public void init(Dependency dependency) { // Interface Injection this.dependency = dependency; } }
In this example, MyClass
implements the Initializable
interface, which requires an init
method to be defined for injecting a dependency. While this does provide a way to inject dependencies, it can lead to less clear design and is often avoided in favor of the more simpler Constructor and Setter Injection methods.
Understanding these types of Dependency Injection allows developers to select the most appropriate method based on their application architecture, leading to cleaner, more maintainable, and testable code. Each method has its strengths and weaknesses, and the choice often depends on the specific requirements and context of the project.
Advanced DI Frameworks: Spring and Guice
When we turn our attention to advanced Dependency Injection (DI) frameworks in the Java ecosystem, two names often come to the forefront: Spring and Guice. Both frameworks elegantly manage dependencies and provide a rich set of features that enable developers to build scalable, maintainable applications. However, they approach dependency management in slightly different ways, each offering unique advantages depending on the use case.
Spring, arguably the most widely used DI framework, is part of the larger Spring Framework. It provides a comprehensive environment for developing Java applications, encompassing not just DI but also aspects of web development, data access, and transaction management. At its core, Spring employs the IoC (Inversion of Control) principle via a powerful application context that manages the lifecycle of beans.
In Spring, you define your beans and their dependencies in a configuration file or through annotations. Here’s a simple example of using annotations for dependency injection:
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Component; @Component public class UserService { private final UserRepository userRepository; @Autowired public UserService(UserRepository userRepository) { // Constructor Injection this.userRepository = userRepository; } public void createUser(String name) { userRepository.save(name); } }
In this example, the Spring container automatically injects the UserRepository bean when instantiating UserService. The @Autowired annotation is key here; it tells Spring to resolve and inject the required dependency. This makes testing simpler, as you can simply mock the UserRepository for unit tests.
On the other hand, Guice, developed by Google, is a lightweight DI framework that focuses on simplicity and performance. Guice’s approach relies more heavily on Java’s type system and offers a more programmatic way to configure dependencies. Guice uses modules to define how dependencies are bound. Here’s an example of a Guice module:
import com.google.inject.AbstractModule; public class UserModule extends AbstractModule { @Override protected void configure() { bind(UserRepository.class).to(JsonUserRepository.class); // Binding } }
With this module, you specify that whenever a UserRepository is requested, Guice should provide an instance of JsonUserRepository. Guice uses annotations like @Inject to manage dependency injection, similar to Spring:
import com.google.inject.Inject; public class UserService { private final UserRepository userRepository; @Inject public UserService(UserRepository userRepository) { // Constructor Injection this.userRepository = userRepository; } public void createUser(String name) { userRepository.save(name); } }
Both frameworks provide robust support for dependency injection, but they cater to different preferences and use cases. Spring is more suitable for larger enterprise applications that require extensive features beyond DI, while Guice is an excellent choice for smaller applications that value simplicity and performance.
When integrating DI frameworks into your Java applications, it’s vital to think the configuration and management of your dependencies carefully. Both Spring and Guice allow for advanced features like scopes, lifecycle management, and AOP (Aspect-Oriented Programming), which can further enhance your application’s modularity and testability.
Ultimately, the choice between Spring and Guice—or any other DI framework—depends on the specific needs of your project, your team’s familiarity with the framework, and the architecture you aim to implement. As you explore these advanced DI frameworks, you’ll find that they provide powerful tools to streamline dependency management, reduce boilerplate code, and promote cleaner, more maintainable designs.
Best Practices for Managing Dependencies
When managing dependencies in Java, especially in larger applications that utilize Dependency Injection (DI), following best practices very important for maintaining readable, maintainable, and testable code. Here are several best practices to consider:
1. Favor Constructor Injection Over Setter Injection
Constructor Injection is the preferred method for dependency injection. It clearly defines the required dependencies at the time of object creation, ensuring that the object is always in a valid state. This avoids the risk of creating partially initialized objects, which can happen with Setter Injection. Additionally, using constructor injection fosters immutability, a desirable property in many applications.
public class OrderService { private final PaymentProcessor paymentProcessor; public OrderService(PaymentProcessor paymentProcessor) { // Constructor Injection this.paymentProcessor = paymentProcessor; } public void processOrder(Order order) { paymentProcessor.process(order.getTotal()); } }
2. Use Interfaces for Dependencies
When defining dependencies, prefer to program against interfaces rather than concrete implementations. This design principle promotes loose coupling and allows for easier swapping of implementations, which is particularly useful for testing. By depending on interfaces, your code remains flexible and can adapt to changes more easily.
public interface PaymentProcessor { void process(double amount); } public class StripePaymentProcessor implements PaymentProcessor { public void process(double amount) { // Implementation for Stripe processing } } public class PaymentService { private final PaymentProcessor paymentProcessor; public PaymentService(PaymentProcessor paymentProcessor) { // Dependency on interface this.paymentProcessor = paymentProcessor; } }
3. Keep Dependencies Minimal
Strive to minimize the number of dependencies a class has. A class with too many dependencies becomes challenging to manage, leading to complex interrelations that can hinder testing and maintenance. Implementing the Single Responsibility Principle can aid in achieving this goal; each class should have only one reason to change, which translates to fewer dependencies.
public class UserService { private final UserRepository userRepository; public UserService(UserRepository userRepository) { // Only one dependency this.userRepository = userRepository; } public void createUser(String name) { userRepository.save(name); } }
4. Leverage DI Framework Features
Frameworks such as Spring and Guice provide various features that enhance dependency management. Utilize these features, including scopes, lifecycle management, and aspect-oriented programming (AOP), to reduce boilerplate code and improve maintainability. For instance, use @Singleton to manage instance lifetimes effectively in a Spring context.
@Component @Scope("singleton") public class UserService { private final UserRepository userRepository; @Autowired public UserService(UserRepository userRepository) { this.userRepository = userRepository; } }
5. Document Your Dependencies
Ensure that your dependencies are well-documented, including the purpose of each dependency and any specific configurations required for their use. This not only aids current developers working on the code but also helps future developers understand the architecture and rationale behind the dependency choices made.
/** * UserService manages user-related operations. * It requires a UserRepository instance for data persistence. */ public class UserService { private final UserRepository userRepository; public UserService(UserRepository userRepository) { this.userRepository = userRepository; } }
6. Regularly Review Dependencies
As your application evolves, so will its dependencies. Regularly review and refactor your code to eliminate unused or unnecessary dependencies. This helps to keep the codebase clean and maintainable.
By adhering to these best practices, you can effectively manage dependencies in your Java applications, leading to cleaner, more maintainable code this is easier to test and adapt over time. Each practice contributes to a more robust architecture that can handle the complexities of modern software development.
Testing Strategies with Dependency Injection
When it comes to testing strategies in Java applications that utilize Dependency Injection (DI), the separation of concerns facilitated by DI becomes a powerful ally. By managing dependencies externally, you can write tests that focus on the behavior of a class without being encumbered by the complexities of its dependencies. This leads to more effective unit tests and overall better software quality.
One of the primary advantages of using DI is that it allows for the easy substitution of real implementations with mocks or stubs during testing. This very important for isolating the unit of work you want to test. For instance, ponder a service that sends notifications. By injecting an interface for the notification sender, you can replace it with a mock implementation during testing, so that you can verify that the service behaves as expected without actually sending notifications.
public class NotificationService { private final MessageSender messageSender; public NotificationService(MessageSender messageSender) { // Constructor Injection this.messageSender = messageSender; } public void notifyUser(String message) { messageSender.send(message); } } public class NotificationServiceTest { @Test public void testNotifyUser() { MessageSender mockSender = Mockito.mock(MessageSender.class); NotificationService notificationService = new NotificationService(mockSender); notificationService.notifyUser("Hello, User!"); Mockito.verify(mockSender).send("Hello, User!"); // Verify interaction } }
In this example, the NotificationService
class is tested in isolation. The real MessageSender
implementation is replaced with a mock, so that you can assert that the send
method was called with the expected argument. This approach not only validates the behavior of the service but also keeps the test fast and independent of external systems.
Another strategy is to leverage testing frameworks like JUnit and Mockito. These frameworks provide a rich set of annotations and utilities that simplify the process of creating mocks, verifying interactions, and asserting conditions. Using annotations such as @Mock
and @InjectMocks
can streamline your tests further:
public class UserServiceTest { @Mock private UserRepository mockRepository; @InjectMocks private UserService userService; // UserService is injected with the mocked UserRepository @Before public void setUp() { MockitoAnnotations.openMocks(this); } @Test public void testCreateUser() { userService.createUser("Alice"); Mockito.verify(mockRepository).save("Alice"); } }
This example uses Mockito’s annotations to manage the creation and injection of mocks automatically, promoting cleaner and more readable test code. The setUp
method initializes the mocks before each test, ensuring a fresh context for testing.
Moreover, when using DI frameworks such as Spring, you can also take advantage of integration tests that involve actual instances of your beans. Spring’s testing support provides context loading capabilities, so that you can run tests with the application context and verify not just the behavior of individual classes but also how they interact with each other:
@RunWith(SpringJUnit4ClassRunner.class) @ContextConfiguration(classes = {AppConfig.class}) // Load the application context public class UserServiceIntegrationTest { @Autowired private UserService userService; // Autowired bean from the context @Test public void testCreateUserIntegration() { userService.createUser("Alice"); // Additional assertions can be made against the database or other components } }
Using an integration test allows you to verify that all components work together seamlessly. That is beneficial in scenarios where the interactions between components are complex, and you want to ensure that the entire system behaves as expected.
As your test suite grows, it is also important to organize your tests effectively. Grouping tests by functionality or behavior, and naming them consistently, enhances readability and maintainability. Additionally, always aim for high test coverage while being pragmatic—focus on testing the most critical paths and edge cases to strike the right balance between quality and effort.
By adopting these strategies in your testing approach, you can ensure that your Java applications are robust, maintainable, and adaptable to changes, all while using the full power of Dependency Injection. Ultimately, effective testing is a pillar of high-quality software development, and using DI correctly plays a significant role in achieving that goal.