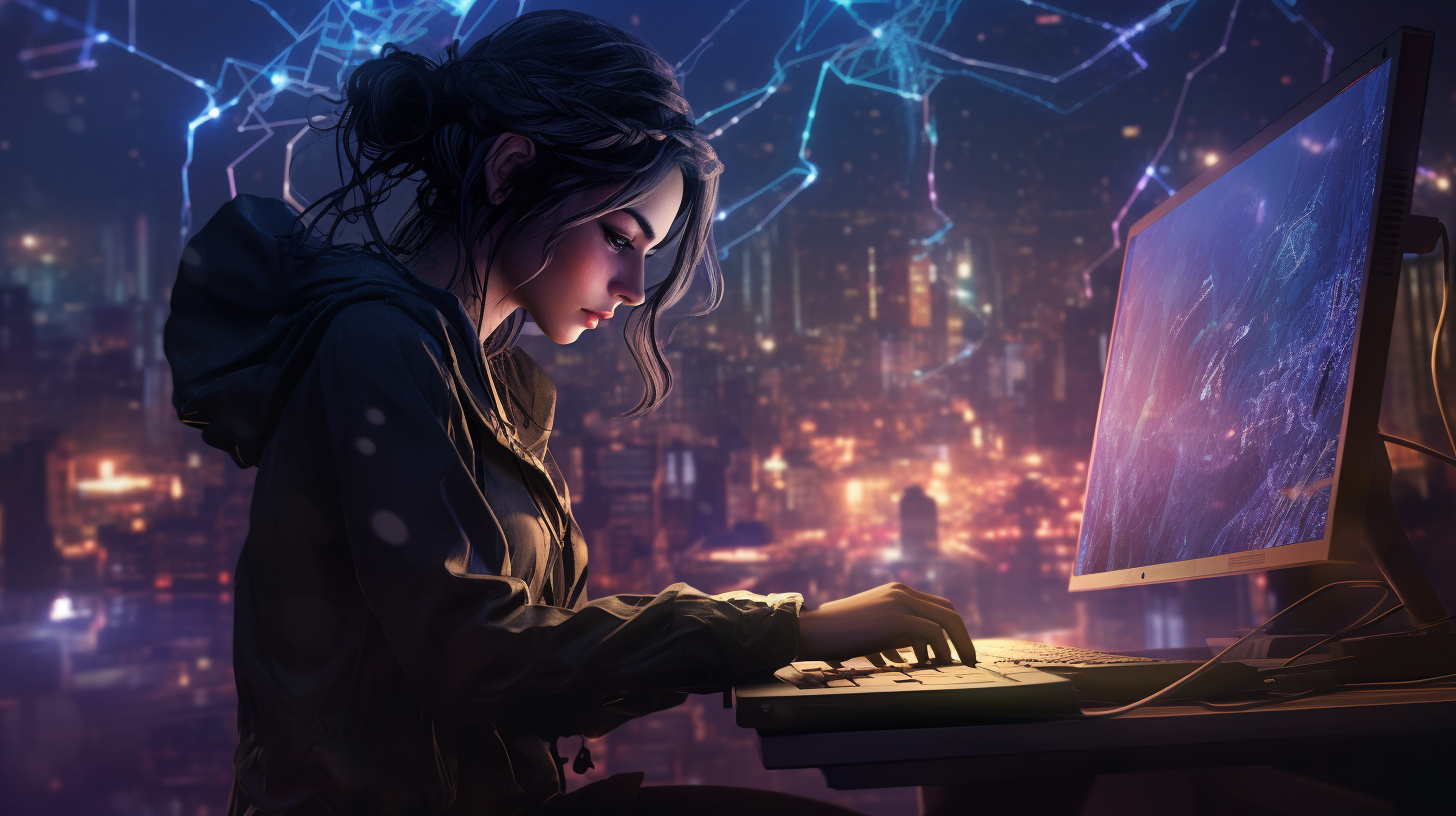
Java and Docker: Containerization
Containerization is a powerful paradigm in software development, particularly for Java applications. By using containers, developers can encapsulate their applications along with all their dependencies, ensuring that they run consistently across various environments. The key to understanding containerization lies in how it abstracts the underlying infrastructure, allowing developers to focus on writing code rather than dealing with operational specifics.
In the context of Java, containerization offers a distinct advantage. Traditionally, Java applications have been deployed on dedicated servers or virtual machines, which can lead to complications such as dependency conflicts, inconsistent environments, and resource wastage. Containers, on the other hand, provide a lightweight alternative by packaging the application and its environment into a self-sufficient unit. This guarantees that the application runs the same way, regardless of where it’s deployed—be it on a developer’s laptop, a staging server, or in production.
At its core, containerization uses the operating system’s kernel to allow multiple isolated user-space instances. Java applications can be packaged within these isolated environments, enabling them to share the kernel while maintaining separation. This leads to enhanced efficiency, as containers utilize fewer resources than traditional virtual machines.
Moreover, Docker simplifies the process of managing these containers. With Docker, developers can create, manage, and orchestrate containers using simpler commands and configuration files. This not only streamlines deployment but also facilitates continuous integration and continuous delivery (CI/CD) workflows.
To illustrate this concept, ponder a simple Java application that prints “Hello, World!” to the console. Below is a sample Java code snippet:
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } }
This basic application can be containerized using Docker. By defining a Dockerfile
, you can specify the environment needed to run your Java application, including the base image, libraries, and any necessary configurations. Here’s an example of a simple Dockerfile
for the above Java application:
# Use the official Java image from the Docker Hub FROM openjdk:11-jre-slim # Set the working directory WORKDIR /app # Copy the compiled Java application into the container COPY HelloWorld.class . # Specify the command to run the application CMD ["java", "HelloWorld"]
With this Dockerfile
, you can build an image that contains your Java application. When you run a container from this image, it will execute the HelloWorld
class and output “Hello, World!”—all while ensuring that your development and production environments remain consistent.
Benefits of Using Docker with Java Applications
Integrating Docker with Java applications yields a multitude of benefits that enhance both development and operational efficiencies. One of the most significant advantages is the ability to create isolated environments for each application instance. This isolation ensures that different applications can coexist on the same host without interfering with each other, thus minimizing the risk of dependency conflicts. For example, if two Java applications require different versions of a library, containers allow you to run both applications concurrently without any issues.
Furthermore, containerization leads to improved resource use. Since containers share the host OS kernel, they are much lighter than virtual machines, which require a full OS for each instance. This lightness translates into faster startup times and the ability to run more applications on the same hardware. In a scenario where multiple microservices are developed using Java, containerization can significantly reduce the overhead and increase the density of deployment, making it possible to scale applications efficiently.
Another notable benefit is the consistency of development environments. Docker enables developers to create a “production-like” environment locally, reducing the infamous “it works on my machine” problem. Once a Docker image is built, it remains consistent across all stages of development, testing, and production. This consistency simplifies the onboarding process for new developers and facilitates smoother collaboration among teams.
Moreover, Docker integrates seamlessly with existing CI/CD pipelines. By automating the build and deployment processes with Docker, teams can achieve faster release cycles and maintain high quality through continuous testing. Changes made to a Java application can be rapidly packaged into a Docker image, tested, and deployed, ensuring that any issues are caught early in the development lifecycle.
Here’s a snippet to showcase how Docker can integrate with a simple Java application configured for continuous delivery:
public class ContinuousIntegrationDemo { public static void main(String[] args) { System.out.println("Continuous integration with Docker is a game changer!"); } }
In this example, the application can be packaged using a Dockerfile that ensures it adheres to the required structure for deployment. This not only makes the deployment process predictable but also allows for easy rollbacks in case of failures.
# Dockerfile for Continuous Integration Demo FROM openjdk:11-jre-slim WORKDIR /app COPY ContinuousIntegrationDemo.class . CMD ["java", "ContinuousIntegrationDemo"]
Setting Up a Java Application in a Docker Container
Setting up a Java application in a Docker container requires a few essential steps that involve preparing your Java application, creating a suitable Dockerfile, and building and running the Docker image. Let’s delve deeper into each of these stages to see how we can effectively encapsulate our Java applications within Docker containers.
First, ensure that your Java application is compiled and ready for deployment. If we use the javac
command to compile our Java code, we can create the necessary class files. For instance, if we have the following Java code:
public class MyApp { public static void main(String[] args) { System.out.println("Running MyApp in Docker!"); } }
We would compile it as follows:
javac MyApp.java
This command generates a MyApp.class
file, which is the output we need to package inside our Docker container.
Next, we craft our Dockerfile. This file defines the environment for our application, including the base image and necessary commands to run the application. A simple Dockerfile for the above Java application would look like this:
# Use the official Java image from the Docker Hub FROM openjdk:11-jre-slim # Set the working directory WORKDIR /app # Copy the compiled Java application into the container COPY MyApp.class . # Specify the command to run the application CMD ["java", "MyApp"]
In this Dockerfile, we start by specifying the base image, which is the official OpenJDK image. The WORKDIR
instruction creates a working directory called /app
. We then use the COPY
command to transfer the compiled MyApp.class
file into the working directory of the container. Finally, the CMD
instruction defines the command that runs the application when the container starts.
With our Dockerfile ready, it’s time to build the Docker image. That is accomplished using the docker build
command. Make sure you run this command in the directory where your Dockerfile is located:
docker build -t my-java-app .
The -t
flag tags the image with the name my-java-app
. The dot at the end signifies that the build context is the current directory.
Once the image is built, we can run it using the docker run
command:
docker run my-java-app
Executing this command will start a container from the my-java-app
image, and you should see the output:
Running MyApp in Docker!
This output confirms that our Java application is successfully running inside a Docker container. The encapsulation of our Java application within the Docker environment ensures that it operates independently of the host environment, thus eliminating compatibility issues and enabling seamless deployment across various platforms.
Best Practices for Java and Docker Integration
When integrating Java applications with Docker, adhering to best practices very important for maximizing the advantages of containerization while minimizing potential pitfalls. These best practices not only improve application performance but also enhance maintainability and scalability over time.
1. Use Multi-Stage Builds: Multi-stage builds allow you to separate the build environment from the runtime environment in your Dockerfile. This approach dramatically reduces the size of the final image by excluding unnecessary dependencies and files used during the build process. Here’s an example:
# Use a builder image to compile the application FROM openjdk:11-jdk-slim AS builder # Set the working directory WORKDIR /app # Copy the source code to the container COPY . . # Compile the Java application RUN javac MyApp.java # Use a lightweight JRE image for the final image FROM openjdk:11-jre-slim # Set the working directory WORKDIR /app # Copy the compiled class from the builder image COPY --from=builder /app/MyApp.class . # Specify the command to run the application CMD ["java", "MyApp"]
This structure not only keeps the runtime image lean but also significantly reduces the attack surface by limiting the number of packages included in the final image.
2. Leverage Docker Volumes: To persist data generated by your Java application or to share files between the host and the container, utilize Docker volumes. This practice is particularly useful for databases or applications that require file storage. An example command to run a container with a volume would look like this:
docker run -v mydata:/app/data my-java-app
In this command, ‘mydata’ is a named volume that will persist even if the container is stopped or removed, making it simple to manage data across container instances.
3. Keep Your Images Small: Smaller images are not only faster to pull from a registry but also reduce deployment times. To achieve smaller images, think using a minimal base image like Alpine
or Distroless
images. These images contain only the essential components needed to run your Java application, stripping out unnecessary packages.
4. Optimize Your Dockerfile: Follow best practices for writing Dockerfiles, such as minimizing the number of layers by combining commands where possible, and ordering your commands strategically to leverage Docker’s caching mechanism. Here’s a refined example of a Dockerfile:
FROM openjdk:11-jre-slim WORKDIR /app COPY MyApp.class . # Combine multiple commands into one RUN command RUN echo "Optimizing Dockerfile" && chmod +x MyApp.class CMD ["java", "MyApp"]
By optimizing your Dockerfile, you can speed up the build process and reduce the final image size.
5. Implement Health Checks: To ensure your Java application is running correctly, use the Docker HEALTHCHECK instruction. This allows Docker to monitor the state of the application and take actions if it becomes unresponsive. An example health check for a Java application could be:
HEALTHCHECK --interval=30s --timeout=10s --retries=3 CMD curl -f http://localhost:8080/health || exit 1
In this scenario, Docker will periodically send requests to the health endpoint of your application, checking the status and reporting any issues.
6. Document Your Images: Use comments within your Dockerfile to explain the purpose of each instruction. Good documentation helps team members understand the rationale behind certain choices, making it easier to maintain or update the Dockerfile in the future.
7. Version Control Your Docker Images: When deploying Java applications, implement versioning on your Docker images. Tagging your images appropriately allows you to track changes and facilitates rollback if a new version introduces issues. For example:
docker build -t my-java-app:1.0 .
Troubleshooting Common Issues in Java Docker Containers
Troubleshooting Java applications running in Docker containers can sometimes feel like navigating a maze, especially when unexpected issues arise. Fortunately, understanding common pitfalls and their solutions can make this journey much smoother. Below are some frequent problems developers encounter, along with practical approaches to resolving them.
1. Java Application Fails to Start
One of the most common issues is that the Java application fails to start, often due to missing dependencies or incorrect configurations. To diagnose this, check the logs of your Docker container using the following command:
docker logs
Inspect the output for any exceptions or errors that indicate what went wrong. Ensure that all necessary libraries are included in your Docker image. If you’re using a multi-stage build, verify that you are copying all required files from the build stage to the runtime stage.
2. Port Binding Issues
Another frequent issue is related to port binding. If your application listens on a specific port but isn’t accessible, ensure that you have correctly published the port when running the container:
docker run -p 8080:8080 my-java-app
This command maps port 8080 of the container to port 8080 on the host. If you forget the -p flag, the application won’t be reachable from outside the container.
3. Memory Limit Exceeded
Java applications can consume considerable memory, especially with large data sets or complex operations. If your application runs out of memory, you might see an error like “Java heap space.” You can allocate more memory to your container using the -m flag when starting it:
docker run -m 512m my-java-app
This command sets a memory limit of 512 MB. Ensure that your Docker host has enough available memory to accommodate the settings you apply.
4. Networking Issues
Sometimes, networking problems occur, especially when your Java application depends on external services or databases. To resolve this, ensure that the container network is correctly set up. You can connect your container to an existing network using:
docker run --net my-network my-java-app
Also, check if the application’s configuration files contain the correct endpoints for the services it interacts with.
5. File Not Found Errors
File paths in the container may differ from those on your local machine. If your application tries to access a file and fails, verify that the file exists in the container’s filesystem. Use the following command to check:
docker exec -it sh
This command opens a shell in the container, so that you can explore its filesystem. Ensure that all necessary files are copied into the container during the image build process.
6. Environment Variable Misconfigurations
Environment variables are often crucial for configuring Java applications. If your application behaves unexpectedly, double-check that you’ve set the environment variables correctly using the -e flag:
docker run -e "ENV_VAR_NAME=value" my-java-app
It’s also wise to log the environment variables at the start of your application to confirm their values.
7. Debugging Inside the Container
When all else fails, you may need to debug directly within the container. You can run your Java application with a debugging flag:
docker run -p 5005:5005 -e "JAVA_TOOL_OPTIONS=-agentlib:jdwp=transport=dt_socket,server=y,suspend=n,address=*:5005" my-java-app
This command enables remote debugging on port 5005, which will allow you to connect your IDE to the running container for deeper inspection of your application’s behavior.