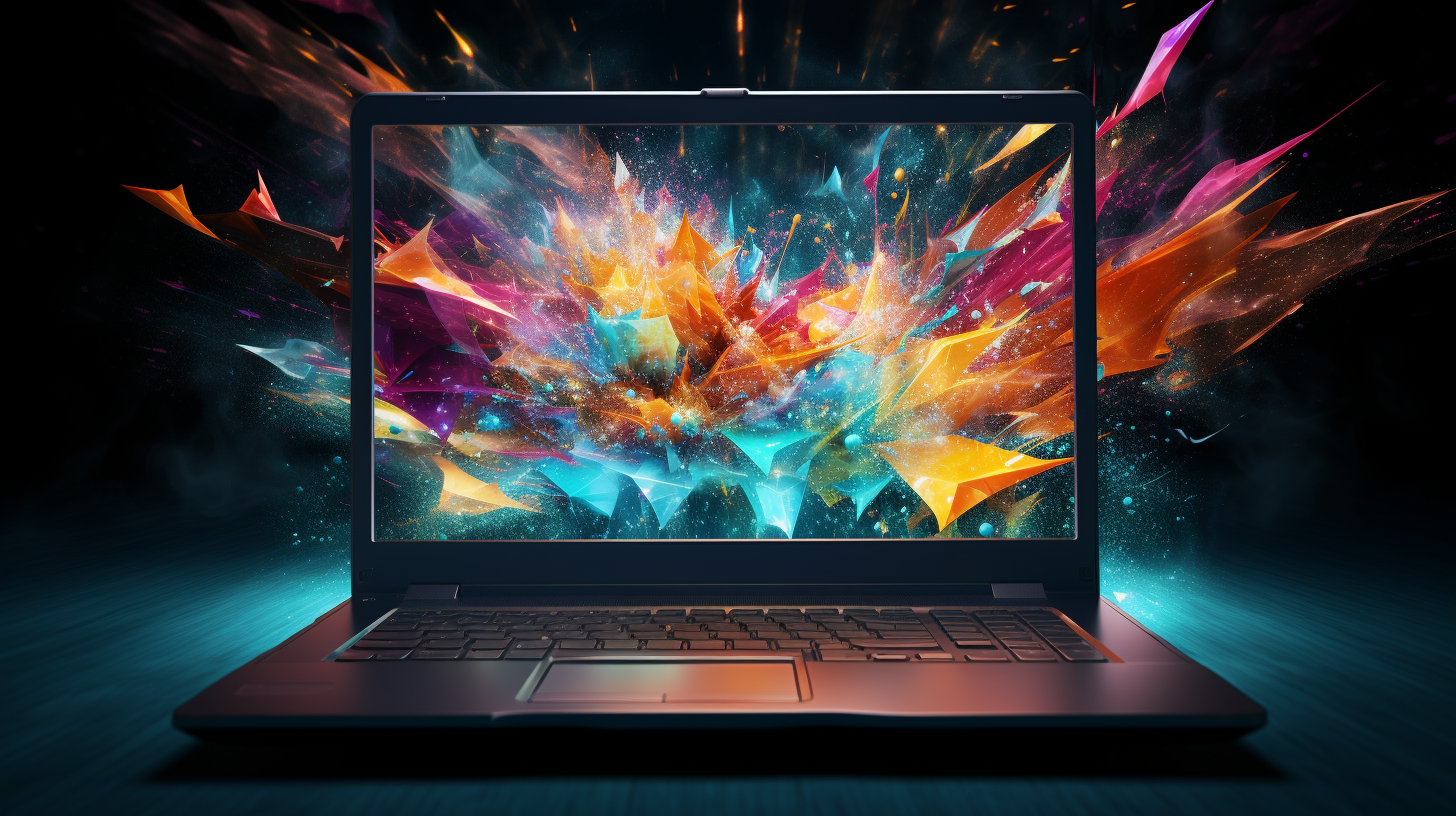
Java and E-commerce Platforms: Building Online Stores
Java has carved out a significant niche in the context of e-commerce development due to its robustness, scalability, and vast ecosystem of libraries and frameworks. The language’s platform independence, courtesy of the Java Virtual Machine (JVM), allows developers to build applications that can run on any operating system without modification. This characteristic is especially advantageous for online stores that must cater to diverse environments and user bases.
One of the primary features that make Java an excellent choice for e-commerce is its object-oriented programming paradigm. This allows developers to create modular and reusable code, which is essential in building complex e-commerce applications. For instance, an online store can have distinct classes for managing products, customer interactions, and order processing, leading to cleaner code and easier maintenance.
Moreover, Java’s strong typing system reduces the likelihood of runtime errors, which is important for e-commerce platforms where financial transactions are involved. The use of interfaces and abstract classes facilitates the development of flexible applications that can adapt to changes in business requirements without extensive rewrites.
Java also excels in performance and efficiency, especially when handling large volumes of transactions and user requests. Using multi-threading, developers can create applications that are capable of processing multiple transactions simultaneously, ensuring a smooth shopping experience for users. The following Java code snippet demonstrates how to implement a simple multi-threaded transaction processor:
public class TransactionProcessor extends Thread { private String transactionId; public TransactionProcessor(String transactionId) { this.transactionId = transactionId; } @Override public void run() { processTransaction(transactionId); } private void processTransaction(String transactionId) { // Simulate transaction processing logic System.out.println("Processing transaction: " + transactionId); // Add logic for payment processing, inventory update, etc. } } // Usage for (int i = 0; i < 10; i++) { new TransactionProcessor("TXN" + i).start(); }
Security is another pivotal aspect of e-commerce development, and Java provides a plethora of features to safeguard sensitive data. The Java Security API allows developers to implement encryption, secure sockets, and authentication mechanisms quite seamlessly. This ensures that customer data is protected throughout their interaction with the online store.
Furthermore, Java’s thriving community and extensive documentation can significantly reduce development times and enhance the quality of the final product. From building a simple online storefront to a complex, enterprise-level solution, Java’s capabilities make it an ideal choice for e-commerce development.
Key Java Frameworks for Building Online Stores
When it comes to building online stores, several key Java frameworks stand out, each providing unique functionalities that streamline the development process and enhance the overall performance of e-commerce applications. Understanding these frameworks very important for any developer looking to leverage Java’s full potential in the context of online retail.
Spring Framework is perhaps the most popular choice for developing Java-based e-commerce applications. Its comprehensive ecosystem includes modules like Spring MVC for building web applications, Spring Data for data access, and Spring Security for authentication and authorization. The framework’s dependency injection and aspect-oriented programming features promote loose coupling and better testability, which are vital for maintaining large e-commerce systems. Below is a basic example illustrating how to set up a simple Spring Boot application for managing products:
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class ECommerceApplication { public static void main(String[] args) { SpringApplication.run(ECommerceApplication.class, args); } }
Once the application is set up, developers can create a RESTful API for handling product data, using Spring’s powerful annotations to manage HTTP requests efficiently.
JavaServer Faces (JSF) is another framework that plays a significant role in e-commerce development. It provides a component-based approach to building user interfaces for web applications. With JSF, developers can create reusable UI components and bind them to backend data models seamlessly. That is particularly useful in e-commerce, where a dynamic, responsive user interface is critical for converting visitors into customers. Here’s a simple JSF backing bean example for managing product details:
import javax.faces.bean.ManagedBean; @ManagedBean public class ProductBean { private String name; private double price; public String getName() { return name; } public void setName(String name) { this.name = name; } public double getPrice() { return price; } public void setPrice(double price) { this.price = price; } public String save() { // Logic to save product details return "productList"; // Navigation to product list page } }
Hibernate is essential for data persistence in Java-based e-commerce applications. By implementing the Java Persistence API (JPA), Hibernate facilitates the mapping of Java objects to database tables, significantly simplifying database operations. That’s especially beneficial for e-commerce platforms that require complex queries and transaction management. Below is an example of a Hibernate entity representing a product:
import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.Id; @Entity public class Product { @Id @GeneratedValue private Long id; private String name; private double price; // Getters and Setters }
In addition to these frameworks, Apache Struts and Vaadin are also worth mentioning. Struts is a robust framework for building MVC-based applications, providing a solid foundation for developing scalable web applications. Vaadin, on the other hand, is a modern framework for building single-page applications, allowing developers to create rich user experiences with minimal JavaScript.
Each of these frameworks offers unique features that cater to different aspects of e-commerce development. By selecting the appropriate framework or combination of frameworks, developers can create efficient, maintainable, and high-performance online stores that stand out in a competitive market.
Integrating Payment Gateways with Java
Integrating payment gateways into an e-commerce platform is one of the most critical steps in ensuring a seamless shopping experience for customers. Java’s extensibility and its rich ecosystem of libraries make it an excellent choice for integrating various payment processing services. Whether you’re using a well-known payment provider or a custom-built solution, the process can be streamlined with the right tools and frameworks.
To begin with, it is essential to choose a payment gateway that fits your business model and meets your customers’ needs. Popular options like PayPal, Stripe, and Authorize.Net offer extensive APIs that are well-documented and widely supported. In this section, we will explore how to integrate a payment gateway using Java, focusing on the use of RESTful APIs, which are common among state-of-the-art payment gateways.
Let’s take a look at how to integrate Stripe as an example. First, you’ll need to include the Stripe Java library in your project. If you are using Maven, you can add the following dependency to your pom.xml
:
com.stripe stripe-java 22.0.0
Once you have the library added, you can start implementing the payment processing logic. Here is a simple example of how to create a charge using the Stripe API:
import com.stripe.Stripe; import com.stripe.model.Charge; import com.stripe.param.ChargeCreateParams; public class PaymentService { public PaymentService() { // Set your secret key. Remember to switch to your live secret key in production! Stripe.apiKey = "your-secret-key"; } public Charge createCharge(String token, double amount) throws Exception { ChargeCreateParams params = ChargeCreateParams.builder() .setAmount((long) (amount * 100)) // Amount in cents .setCurrency("usd") .setSource(token) // Obtained with Stripe.js .setDescription("Charge for product") .build(); return Charge.create(params); } }
In this example, the createCharge
method takes a payment token and the amount to be charged. The token is generated by Stripe.js, a library that securely collects payment information from your customers. This ensures that sensitive data never touches your server, maintaining PCI compliance.
Next, you’ll need to handle the payment token generation on the client side. Here’s a simple example of how to create a payment form using Stripe.js:
var stripe = Stripe('your-publishable-key'); var elements = stripe.elements(); var cardElement = elements.create('card'); cardElement.mount('#card-element'); document.getElementById('payment-form').addEventListener('submit', function(event) { event.preventDefault(); stripe.createToken(cardElement).then(function(result) { if (result.error) { // Inform the user if there was an error document.getElementById('card-errors').textContent = result.error.message; } else { // Send the token to your server sendTokenToServer(result.token); } }); }); function sendTokenToServer(token) { // Implement AJAX request to your server here }
In this code snippet, the payment form is created using Stripe.js, and when the user submits the form, a token is generated. This token can then be sent to your Java backend, where you can call the createCharge
method to process the payment.
Lastly, error handling is an important part of payment integration. You should always validate the response from the payment gateway and handle any potential exceptions properly. For example, if the charge creation fails, you should log the error and notify the user appropriately.
try { Charge charge = createCharge(token, amount); // Handle successful charge } catch (Exception e) { // Log the error and notify the user System.err.println("Payment processing failed: " + e.getMessage()); }
By following these steps, you can effectively integrate payment gateways into your Java-based e-commerce platform, offering a secure and efficient payment process for your users. The combination of Java’s robustness and the capabilities of contemporary payment APIs lays the groundwork for a successful online retail experience.
Implementing Security Best Practices in E-commerce
Driven by renewed attention to where data breaches and cyber threats are rampant, implementing security best practices in e-commerce is not just a checkbox on a developer’s to-do list; it is an imperative for protecting customer trust and business integrity. Java, with its built-in security features and extensive libraries, provides developers with the tools necessary to secure their applications effectively.
One of the fundamental aspects of securing an e-commerce platform is ensuring data transmission is encrypted. Using the Java Secure Socket Extension (JSSE), developers can implement SSL/TLS protocols to encrypt data in transit. This ensures that sensitive information such as credit card details and personal data cannot be intercepted by malicious actors. Below is an example of how to create an HTTPS connection using JSSE:
import javax.net.ssl.HttpsURLConnection; import java.net.URL; public class SecureConnection { public static void main(String[] args) { try { URL url = new URL("https://your-secure-api.com"); HttpsURLConnection connection = (HttpsURLConnection) url.openConnection(); connection.setRequestMethod("GET"); connection.connect(); // Handle response int responseCode = connection.getResponseCode(); System.out.println("Response Code: " + responseCode); } catch (Exception e) { e.printStackTrace(); } } }
Beyond data transmission, it is crucial to protect user credentials. Java provides a robust set of APIs for managing user authentication and authorization. The Java Authentication and Authorization Service (JAAS) is particularly useful for implementing security frameworks that involve user login systems. Here’s a basic example of how JAAS can be used to authenticate a user:
import javax.security.auth.login.LoginContext; import javax.security.auth.login.LoginException; public class UserAuthenticator { public void authenticate(String username, String password) { try { LoginContext lc = new LoginContext("YourLoginModule", new YourCallbackHandler(username, password)); lc.login(); System.out.println("Authentication successful!"); } catch (LoginException e) { System.err.println("Authentication failed: " + e.getMessage()); } } }
Storing passwords securely is another critical component of e-commerce security. Java developers should use modern hashing algorithms such as bcrypt or Argon2, which are specifically designed to be computationally intensive to thwart brute-force attacks. Here’s how you can hash a password using bcrypt:
import org.mindrot.jbcrypt.BCrypt; public class PasswordManager { public String hashPassword(String plainPassword) { return BCrypt.hashpw(plainPassword, BCrypt.gensalt()); } public boolean checkPassword(String plainPassword, String hashedPassword) { return BCrypt.checkpw(plainPassword, hashedPassword); } }
Furthermore, implementing proper access controls is vital. This involves defining user roles and ensuring that users can only access resources they are authorized to use. Java’s Spring Security framework provides a powerful mechanism for managing access controls in a granular way. For instance, you can secure REST endpoints by using annotations:
import org.springframework.security.access.prepost.PreAuthorize; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; @RestController public class ProductController { @PreAuthorize("hasRole('ADMIN')") @GetMapping("/admin/products") public String getAdminProducts() { return "Admin product list"; } @PreAuthorize("hasRole('USER')") @GetMapping("/user/products") public String getUserProducts() { return "User product list"; } }
In addition to these practices, it’s essential to regularly audit your application for vulnerabilities and apply security patches promptly. Using tools such as OWASP ZAP can help automate penetration testing, identifying weaknesses that could be exploited.
Finally, educating your development and operational teams on security best practices cannot be overstated. A well-informed team can significantly reduce the likelihood of human error, which is often the weakest link in security chains. Security is not a one-time implementation; it is an ongoing process that must evolve alongside emerging threats and vulnerabilities.
Scalability and Performance Optimization for Online Retail Platforms
Scalability and performance optimization are critical factors in the success of any e-commerce platform. As online stores grow, they need to handle increasing loads of transactions, users, and data without a hitch. Java’s architecture is well-suited for building scalable applications, allowing developers to leverage various techniques and best practices to ensure that their e-commerce systems can grow efficiently.
One of the primary strategies for achieving scalability in Java applications is to design them using a microservices architecture. By breaking down the application into smaller, independent services, developers can deploy and scale each service independently based on demand. This technique not only enhances maintainability but also improves performance. For instance, if an online store experiences a surge in traffic during a sale, only the services related to product browsing and checkout can be scaled up while others remain unchanged.
Here’s an example of a simple microservice implemented in Java using Spring Boot. This service handles product information:
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; @SpringBootApplication @RestController public class ProductService { public static void main(String[] args) { SpringApplication.run(ProductService.class, args); } @GetMapping("/products") public String getProducts() { // Logic to fetch products from database return "List of products"; } }
In addition to microservices, caching is another powerful technique to enhance performance. Caching helps reduce the load on databases by storing frequently accessed data in memory, thus speeding up response times. Java developers can use caching solutions like Ehcache or Redis to implement caching in their applications. Here’s an example of how to use Spring Cache with an in-memory cache:
import org.springframework.cache.annotation.Cacheable; import org.springframework.stereotype.Service; @Service public class ProductService { @Cacheable("products") public List getProducts() { // Simulate a database call return fetchProductsFromDatabase(); } private List fetchProductsFromDatabase() { // Logic to fetch products return new ArrayList(); } }
Another aspect of scalability is database optimization. In an e-commerce scenario, using a relational database with a well-defined schema can lead to performance bottlenecks as the amount of data grows. To address this, developers can leverage NoSQL databases like MongoDB or Cassandra, which offer better horizontal scalability. Here’s a simple example of how to connect to a MongoDB database in a Java application:
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.mongodb.core.MongoTemplate; import org.springframework.stereotype.Service; @Service public class ProductService { @Autowired private MongoTemplate mongoTemplate; public void saveProduct(Product product) { mongoTemplate.save(product); } }
Load balancing is also essential for managing high traffic loads. It distributes incoming requests across multiple instances of an application, ensuring that no single instance becomes overburdened. Tools like NGINX or HAProxy can be used to set up load balancing for Java applications. Additionally, deploying applications in containers using Docker and orchestrating them with Kubernetes can further enhance scalability. This setup allows for automatic scaling of application instances based on real-time demand.
Performance monitoring and profiling are critical for identifying bottlenecks in Java applications. Tools such as Java VisualVM or JProfiler can help developers analyze memory usage, CPU performance, and thread activity. By identifying performance bottlenecks, developers can make informed decisions about where to optimize code, whether it’s refining algorithms, minimizing object creation, or optimizing database queries.
Lastly, implementing asynchronous processing can significantly improve the responsiveness of a Java e-commerce application. By using message queues (e.g., RabbitMQ or Apache Kafka), developers can offload time-consuming tasks, like sending emails or processing payments, to background workers. Here’s an example of how to send a message to a RabbitMQ queue:
import org.springframework.amqp.rabbit.core.RabbitTemplate; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; @Service public class NotificationService { @Autowired private RabbitTemplate rabbitTemplate; public void sendEmailNotification(String email) { String message = "Your order has been placed successfully!"; rabbitTemplate.convertAndSend("emailQueue", message); } }
By using these strategies and techniques, Java developers can ensure that their e-commerce platforms remain responsive, efficient, and capable of handling increased demands as their businesses grow. The combination of Java’s robust capabilities, coupled with contemporary architectural practices, provides a solid foundation for building scalable and high-performance e-commerce applications.