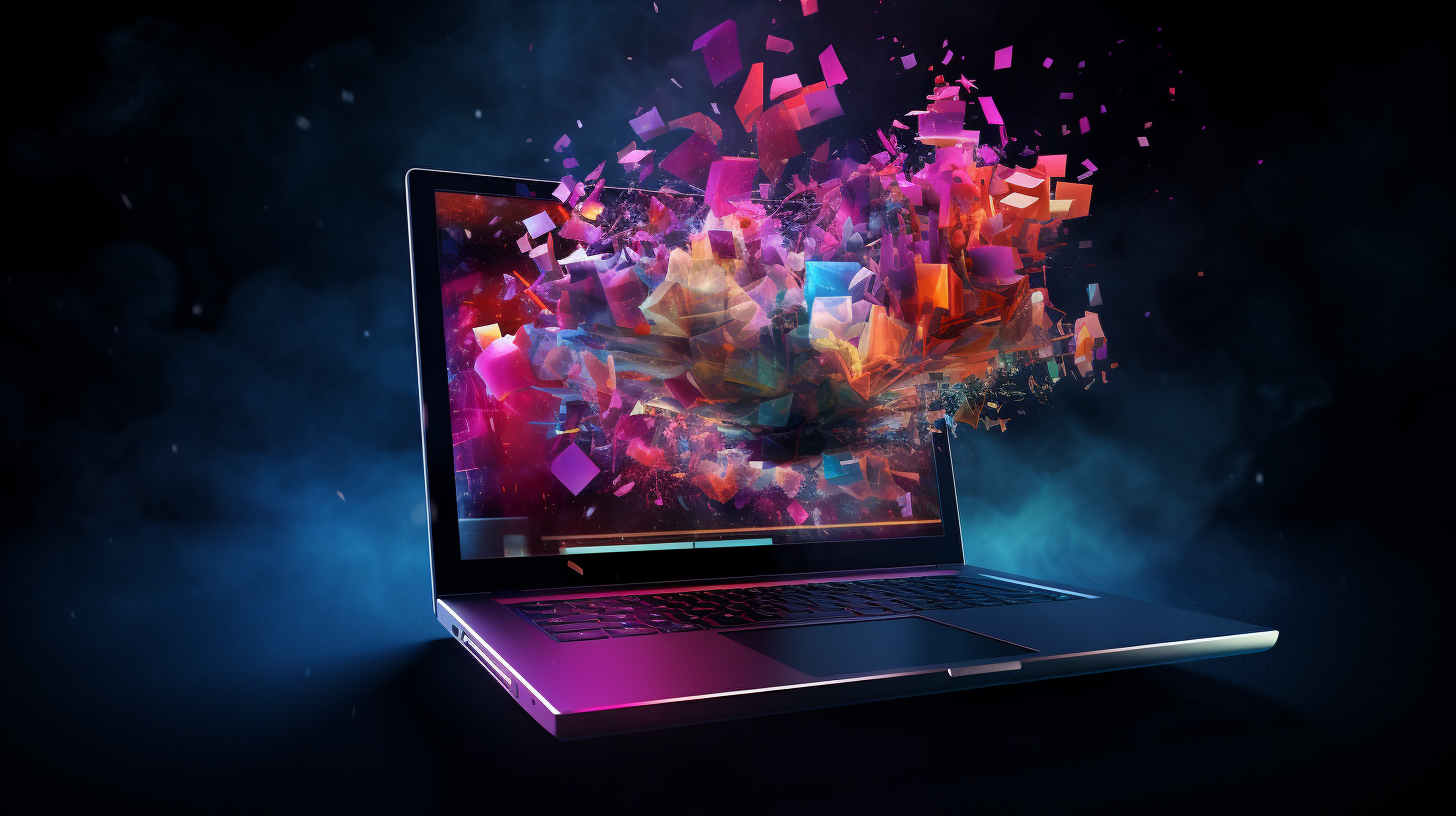
Java and Front-End Integration
Java has long been a staple within the scope of web development, primarily due to its robustness, portability, and extensive ecosystem. The language offers a solid foundation for building server-side applications, which can seamlessly interact with front-end technologies. Java’s architecture, particularly its use of the Java Virtual Machine (JVM), allows for cross-platform compatibility, making it an ideal choice for large-scale applications that demand reliability and scalability.
At the core of Java’s role in web development lies the Java Enterprise Edition (Java EE), which provides a set of specifications that extend the Java Standard Edition with features essential for building enterprise-level applications. Java EE introduces powerful APIs such as Servlets, JavaServer Pages (JSP), and JavaServer Faces (JSF), all designed to facilitate the creation of dynamic web content.
In a typical architecture, Java serves as the backbone of the server-side logic, handling data processing, business rules, and database interactions. The separation of concerns allows front-end developers to focus on user interface (UI) design while relying on Java to manage the underlying complexity. This division very important for maintaining clean, manageable codebases and enhancing team collaboration.
To illustrate Java’s capabilities in web development, think a simple servlet that processes HTTP requests and returns a response. This snippet showcases how Java can effectively handle web interactions:
import java.io.IOException; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; @WebServlet("/hello") public class HelloServlet extends HttpServlet { protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { response.setContentType("text/html"); response.getWriter().println("Hello, World!
"); } }
This simple servlet listens for GET requests at the “/hello” endpoint and responds with a basic HTML greeting. It demonstrates how Java can serve dynamic content, providing the foundation for more complex interactions.
Furthermore, Java’s integration with modern frameworks like Spring and Hibernate exemplifies its adaptability. Spring, in particular, simplifies web application development, offering dependency injection, aspect-oriented programming, and an extensive set of modules for building RESTful services. This level of abstraction allows developers to focus on business logic while efficiently managing interactions with the database and front-end.
In the context of RESTful architecture, a Java-based API can deliver JSON or XML data to front-end frameworks such as Angular, React, or Vue.js. The following example illustrates how to create a RESTful endpoint using Spring:
import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; @RestController public class ApiController { @GetMapping("/api/data") public String getData() { return "{"message":"Hello from Java!"}"; } }
In this example, the API responds with a JSON object, demonstrating how Java can serve as a powerful backend service that complements modern front-end frameworks. The ability to transmit structured data efficiently bridges the gap between server-side logic and client-side presentation.
Ultimately, Java’s role in web development is not just about server-side processing; it’s about creating a cohesive environment where front-end and back-end technologies work in harmony. As developers leverage Java’s strengths, they can build robust applications that stand the test of time, ensuring a seamless user experience across devices and platforms.
Connecting Java with Front-End Frameworks
To effectively connect Java with front-end frameworks, developers must establish clear communication channels between the server-side components and client-side interfaces. This integration often hinges on the use of RESTful APIs, which serve as a bridge for data exchange. The advantages of this approach are manifold; not only does it facilitate a clean separation of concerns, but it also allows front-end applications to be developed independently of the back-end, using the capabilities of modern Java frameworks.
Consider a scenario where a Java application powered by Spring Boot serves as the backend. The application can expose multiple endpoints, each designed to handle specific data requests. On the front-end side, frameworks like React or Angular can be employed to make asynchronous calls to these endpoints, allowing for dynamic updates without reloading the entire page. This not only enhances user experience but also aligns with the principles of Single Page Applications (SPAs), which are paramount in modern web development.
To exemplify this integration, below is a simple Java Spring Boot application that provides a RESTful service and a corresponding Angular front-end that consumes this service:
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; @SpringBootApplication public class Application { public static void main(String[] args) { SpringApplication.run(Application.class, args); } } @RestController class GreetingController { @GetMapping("/api/greet") public String greet() { return "Hello from the Java backend!"; } }
The above code sets up a Spring Boot application with a simple REST endpoint at “/api/greet”. When this endpoint is accessed, it returns a greeting string. It’s time to see how a front-end application can consume this API.
import React, { useEffect, useState } from 'react'; function App() { const [greeting, setGreeting] = useState(''); useEffect(() => { fetch('/api/greet') .then(response => response.text()) .then(data => setGreeting(data)); }, []); return (); } export default App;{greeting}
In the React component above, a fetch request is made to the Java backend to retrieve the greeting message. The useEffect hook ensures that this request is made upon the component’s mount, and the received data is stored in the component’s state. This pattern exemplifies the seamless interaction between Java and a front-end framework, illustrating how data can flow from server to client efficiently.
Integrating Java with front-end frameworks also necessitates attention to security, especially when dealing with sensitive data. Using authentication mechanisms such as OAuth2 or JWT (JSON Web Tokens) can help secure endpoints against unauthorized access. Moreover, adopting CORS (Cross-Origin Resource Sharing) policies allows for controlled access from front-end applications running on different domains.
As developers continue to exploit the capabilities of both Java and modern front-end frameworks, the synergy created between these technologies will pave the way for building highly responsive, maintainable, and scalable web applications. This not only enhances the development process but also significantly improves the overall user experience.
Best Practices for Java and Front-End Integration
When integrating Java with front-end frameworks, adhering to best practices very important for ensuring a smooth, efficient, and maintainable application architecture. Here are some essential best practices to think for successful integration:
1. Use RESTful APIs Effectively
RESTful APIs are the gold standard for communication between Java back-end services and front-end applications. When designing your API, ensure it follows REST principles, using appropriate HTTP methods (GET, POST, PUT, DELETE) for CRUD operations. This not only makes your API intuitive but also enhances its ease of use and scalability.
import org.springframework.web.bind.annotation.*; @RestController @RequestMapping("/api/users") public class UserController { @GetMapping("/{id}") public User getUserById(@PathVariable Long id) { return userService.findById(id); } @PostMapping public User createUser(@RequestBody User user) { return userService.save(user); } @PutMapping("/{id}") public User updateUser(@PathVariable Long id, @RequestBody User user) { return userService.update(id, user); } @DeleteMapping("/{id}") public void deleteUser(@PathVariable Long id) { userService.delete(id); } }
In this example, we define CRUD operations for a user resource. Each endpoint is clearly defined, making it simpler for front-end developers to understand how to interact with the back-end.
2. Employ Versioning for APIs
As your application evolves, so too will its API. To manage changes without breaking existing client applications, implement versioning in your API. This can be done via URL path (e.g., /api/v1/users
) or using request headers. Versioning helps maintain backward compatibility while allowing new features and improvements to be introduced in subsequent versions.
3. Focus on Data Serialization
When exchanging data between Java back-end and front-end applications, data serialization formats such as JSON or XML are commonly used. JSON is preferred in most cases due to its lightweight nature. Use libraries like Jackson or Gson to facilitate the conversion of Java objects into JSON and vice versa.
import com.fasterxml.jackson.databind.ObjectMapper; public class UserController { private final ObjectMapper objectMapper = new ObjectMapper(); @PostMapping public String createUser(@RequestBody User user) throws JsonProcessingException { // Convert user object to JSON string String json = objectMapper.writeValueAsString(user); // Process the user... return json; } }
This snippet demonstrates how to serialize a Java object into a JSON string using Jackson, enabling seamless data transmission to the front end.
4. Handle Errors Gracefully
Robust error handling is essential for user experience. Ensure your API provides meaningful error messages and appropriate HTTP status codes. This clarity allows front-end applications to respond correctly to various situations, such as validation errors or resource not found errors.
@ExceptionHandler(UserNotFoundException.class) @ResponseStatus(HttpStatus.NOT_FOUND) public String handleUserNotFound(UserNotFoundException ex) { return ex.getMessage(); }
The above code snippet shows how to handle a custom exception by returning a effortless to handle message along with the appropriate HTTP status code.
5. Security First
In today’s digital landscape, security cannot be an afterthought. Utilize HTTPS to encrypt data in transit and apply appropriate authentication and authorization mechanisms. Implementing OAuth2 or JWT for securing your APIs protects sensitive information and restricts access to authorized users only.
import org.springframework.security.config.annotation.web.builders.HttpSecurity; import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity; import org.springframework.security.web.SecurityFilterChain; @EnableWebSecurity public class SecurityConfig { public SecurityFilterChain securityFilterChain(HttpSecurity http) throws Exception { http .authorizeRequests() .antMatchers("/api/public/**").permitAll() .anyRequest().authenticated() .and() .oauth2Login(); return http.build(); } }
This configuration secures API endpoints while allowing public access to specific routes, exemplifying a layered approach to security.
6. Optimize Performance
Lastly, consider performance optimizations such as caching frequently accessed data, minimizing data payloads, and employing lazy loading techniques in your front-end application. Using tools like Spring Cache can significantly improve response times, thereby enhancing the overall user experience.
@Cacheable("users") @GetMapping("/{id}") public User getUserById(@PathVariable Long id) { return userService.findById(id); }
The above code enables caching for a specific user retrieval operation, reducing the need for repeated database access and improving application responsiveness.
By following these best practices, developers can create an integrated environment where Java back-end services and front-end frameworks work together seamlessly. This not only leads to cleaner code and better maintainability but also results in applications that deliver a superior user experience.
Case Studies: Successful Java and Front-End Projects
To comprehend the practical benefits of Java's integration with front-end frameworks, one can look at various case studies that showcase this synergy. One notable example is **Netflix**, which leverages Java extensively on the server side, particularly through its microservices architecture. The back-end, primarily built using Spring Boot, exposes RESTful APIs that interact with the front-end developed using React. This combination allows Netflix to deliver a highly responsive user experience, enabling seamless browsing and streaming of content. The scalability of the Java back-end ensures that millions of simultaneous users can be accommodated, demonstrating the robustness of Java in handling large-scale applications. Another remarkable case is **Airbnb**, which employs Java along with various front-end technologies. The back-end services utilize Java's Spring framework to manage complex data interactions and processing. On the front end, Airbnb utilizes React to build dynamic user interfaces that provide instant feedback and updates. The integration of Java with React's component model allows for efficient data handling, resulting in a smooth and engaging user experience. This approach not only simplifies development but also makes it easier to maintain the application code over time. A compelling case study involves **eBay**, which uses Java to power its back-end services while employing Angular for its front-end development. The Java services expose well-defined REST APIs to serve data to the Angular application. This architecture supports eBay's need for real-time updates and dynamic content delivery, crucial for a platform that operates in a highly competitive online marketplace. The combination of Java’s reliability and Angular’s capabilities results in a highly responsive interface for users. Think the following simplified example based on a fictional e-commerce application that illustrates how Java interacts with a React front end to manage product listings: ```java import org.springframework.web.bind.annotation.*; @RestController @RequestMapping("/api/products") public class ProductController { @GetMapping public List getAllProducts() { return productService.findAll(); } @PostMapping public Product createProduct(@RequestBody Product product) { return productService.save(product); } } ``` In this example, the Java back-end provides endpoints for fetching and creating product listings. The front end, built using React, can easily communicate with these endpoints, allowing users to view and add products seamlessly. On the React side, the integration may look as follows: ```javascript import React, { useEffect, useState } from 'react'; function ProductList() { const [products, setProducts] = useState([]); useEffect(() => { fetch('/api/products') .then(response => response.json()) .then(data => setProducts(data)); }, []); return (
-
{products.map(product => (
- {product.name} ))}