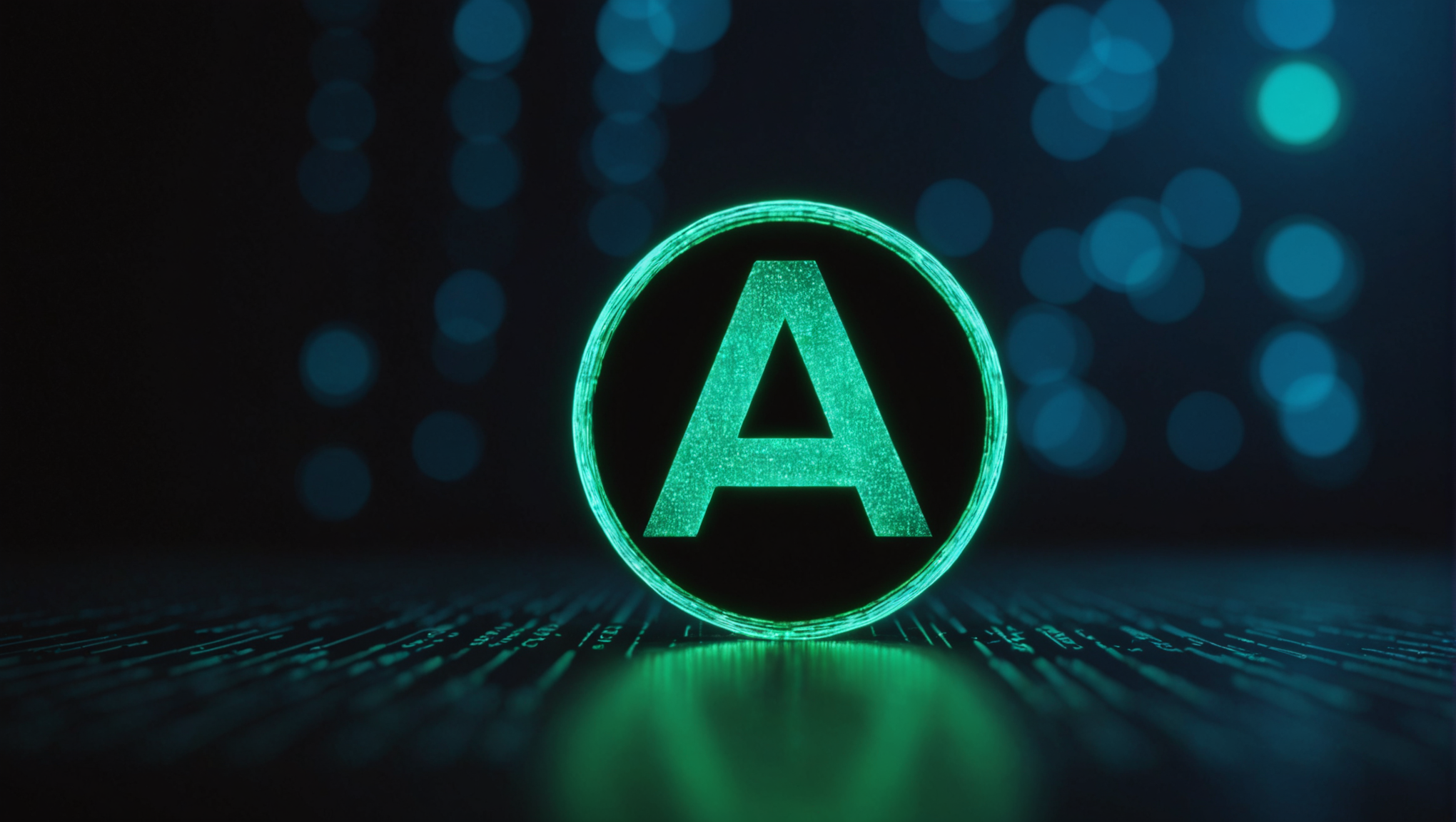
Java and Functional Programming: Concepts and Practices
In Java, lambda expressions serve as a powerful tool that enables developers to write concise and expressive code, primarily focusing on what to do rather than how to do it. They’re a form of syntactic sugar that simplifies the implementation of functional interfaces, allowing for cleaner code, especially when working with collections or streams.
A lambda expression can be understood as a shorthand notation for creating instances of functional interfaces. The beauty of lambda expressions lies in their ability to encapsulate functionality as an argument, facilitating higher-order programming. The general syntax of a lambda expression is:
parameters -> expression
For instance, think a simple example where we implement a lambda expression to perform addition:
@FunctionalInterface interface Adder { int add(int a, int b); } public class LambdaExample { public static void main(String[] args) { Adder adder = (a, b) -> a + b; System.out.println("Sum: " + adder.add(5, 3)); // Output: Sum: 8 } }
In the example above, we define a functional interface Adder with a single abstract method add. The lambda expression (a, b) -> a + b implements the interface, providing the logic for addition.
Lambda expressions are especially useful in scenarios where we need to pass behavior as a parameter. For example, when filtering a collection of data, we can use lambda expressions to define the criteria succinctly:
import java.util.Arrays; import java.util.List; public class FilterExample { public static void main(String[] args) { List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David"); names.stream() .filter(name -> name.startsWith("A")) .forEach(System.out::println); // Output: Alice } }
In this case, the lambda expression name -> name.startsWith(“A”) acts as a filter criterion, allowing us to extract only the names that start with the letter ‘A’.
Furthermore, lambda expressions enhance the readability of the code, making it easier to understand at a glance. They also lead to a reduction in the boilerplate code typically associated with anonymous classes. This reduction is particularly beneficial in scenarios involving event handling, callbacks, or multi-threading.
However, it’s essential to use lambda expressions judiciously. While they bring great advantages in terms of simplicity and expressiveness, overusing them can lead to less readable code, especially for developers unfamiliar with functional programming paradigms. Maintaining a balance between clarity and conciseness especially important for effective coding practices.
Ultimately, lambda expressions are not just a syntactical feature; they represent a paradigm shift, inviting developers to embrace a more functional style of programming within the object-oriented framework of Java. By using lambda expressions, we can write code that is not only more efficient but also more aligned with the principles of functional programming.
Stream API: Transforming Data with Functional Techniques
The Stream API in Java, introduced in Java 8, is a powerful abstraction that allows developers to process sequences of elements in a functional manner. This API provides a rich set of methods that can be used to perform operations such as filtering, mapping, and reducing on collections of objects. The beauty of the Stream API lies in its ability to transform data without altering the original data structure, promoting immutability and side-effect-free programming.
At its core, a stream is a sequence of elements that can be processed in parallel or sequentially. Streams can be created from various data sources, including collections, arrays, or I/O channels. The key operations on streams can be categorized into intermediate and terminal operations. Intermediate operations return a new stream, allowing for further processing, while terminal operations produce a result or a side effect, such as collecting elements or printing them.
Let’s ponder an example where we use the Stream API to process a list of integers. In this scenario, we will filter out even numbers, square the remaining integers, and collect the results into a new list:
import java.util.Arrays; import java.util.List; import java.util.stream.Collectors; public class StreamExample { public static void main(String[] args) { List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6); List<Integer> squares = numbers.stream() .filter(n → n % 2 != 0) // Filter out even numbers .map(n → n * n) // Square the remaining numbers .collect(Collectors.toList()); // Collect results into a list System.out.println("Squared odd numbers: " + squares); // Output: Squared odd numbers: [1, 9, 25] } }
In the example above, we create a list of integers and convert it into a stream. The intermediate method filter
is used to exclude even numbers, while map
applies a transformation to square each remaining integer. Finally, we collect the results into a new list using the collect
method.
Another powerful aspect of the Stream API is its ability to process data in parallel. This can be particularly beneficial for large datasets where performance improvements are crucial. To switch from sequential to parallel processing, one can simply call the parallelStream
method:
import java.util.Arrays; import java.util.List; public class ParallelStreamExample { public static void main(String[] args) { List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6); int sum = numbers.parallelStream() .filter(n → n % 2 != 0) // Filter odd numbers .mapToInt(n → n) // Mapping to int .sum(); // Terminal operation to sum the values System.out.println("Sum of odd numbers: " + sum); // Output: Sum of odd numbers: 9 } }
In this example, we leverage the parallelStream
method to calculate the sum of odd numbers from a list. The Stream API handles the parallelization internally, allowing the operation to take advantage of multiple CPU cores without the need for complex thread management.
Overall, the Stream API not only simplifies data manipulation in Java but also encourages a more functional approach to programming. By offering a blend of readability and efficiency, it empowers developers to express complex data processing tasks in a declarative style, ultimately leading to cleaner and more maintainable code.
Functional Interfaces: Building Blocks of Functional Programming
Functional interfaces are at the heart of functional programming in Java. They serve as the backbone for lambda expressions, enabling developers to pass behavior as parameters, thereby creating a more flexible and dynamic programming style. A functional interface is defined as an interface that contains exactly one abstract method. This one-method constraint allows lambda expressions to be used as implementations of the functional interface, representing a clear and simple way to define behavior.
Java provides several built-in functional interfaces in the java.util.function
package, which are commonly used in various contexts. Some of the most frequently used functional interfaces include:
- Represents a boolean-valued function of one argument. It’s often used for filtering collections.
- Represents a function that accepts one argument and produces a result. It is useful for transforming data.
- Represents an operation that accepts a single input argument and returns no result. It’s typically used for side effects.
- Represents a supplier of results, providing a method to get a value without input.
Let’s illustrate the idea of functional interfaces with a few practical examples. First, we’ll use the Predicate
functional interface to filter a list of strings based on their length:
import java.util.Arrays; import java.util.List; import java.util.function.Predicate; public class PredicateExample { public static void main(String[] args) { List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "Dave"); Predicate<String> isLongName = name -> name.length() > 3; names.stream() .filter(isLongName) .forEach(System.out::println); // Output: Alice, Charlie } }
In this example, we define a Predicate
called isLongName
that checks if a name’s length is greater than 3. We then use this predicate to filter the list of names, demonstrating how functional interfaces allow us to encapsulate behavior in a reusable manner.
Next, let’s explore the Function
interface to transform a list of integers by squaring each value:
import java.util.Arrays; import java.util.List; import java.util.function.Function; import java.util.stream.Collectors; public class FunctionExample { public static void main(String[] args) { List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5); Function<Integer, Integer> square = n -> n * n; List<Integer> squaredNumbers = numbers.stream() .map(square) .collect(Collectors.toList()); System.out.println("Squared Numbers: " + squaredNumbers); // Output: Squared Numbers: [1, 4, 9, 16, 25] } }
In this case, we define a Function
instance called square
that calculates the square of an integer. By applying this function with the map
method, we transform the original list of numbers into their squared values.
Functional interfaces not only enhance code readability but also improve reusability. They allow developers to define behavior separately from its execution context, promoting a more modular and maintainable code structure. Additionally, with the introduction of the @FunctionalInterface
annotation, developers can explicitly declare their intent to create a functional interface. This annotation will trigger a compile-time error if the interface does not meet the functional interface criteria, thus providing a safeguard against unintentional mistakes.
Embracing functional interfaces in Java programming can lead to cleaner, more expressive code that leverages the power of lambda expressions effectively. By adopting this functional approach, developers can harness the full potential of Java’s functional programming capabilities, leading to innovative and efficient solutions.
Best Practices for Implementing Functional Programming in Java
Transitioning to functional programming in Java is not just about adopting new syntax; it involves embracing best practices that enhance code quality, maintainability, and performance. Here are several key practices to ponder when implementing functional programming in Java.
1. Favor Immutability
One of the cornerstones of functional programming is immutability. Immutable objects are those that cannot be modified after they’re created. This characteristic simplifies reasoning about code, particularly in concurrent programming, since immutable data structures do not suffer from race conditions. In Java, you can leverage the final keyword for variables and use immutable collection classes such as List.of or Set.of. For example:
import java.util.List; public class ImmutableExample { public static void main(String[] args) { List<String> names = List.of("Alice", "Bob", "Charlie"); // Attempting to modify the list will throw an UnsupportedOperationException // names.add("David"); // Uncommenting this line will result in an error System.out.println(names); } }
2. Use Higher-Order Functions Wisely
Higher-order functions, which accept other functions as arguments or return them, are essential in functional programming. They enhance the flexibility and reusability of code. However, it is vital to use them judiciously. Overusing higher-order functions can lead to complex code that may be difficult to understand. Always ensure that the intent of your higher-order functions is clear and well-documented. Here’s an example using a higher-order function:
import java.util.function.Function; public class HigherOrderFunctionExample { public static void main(String[] args) { Function<Integer, Integer> square = x -> x * x; System.out.println("Square of 5: " + applyFunction(square, 5)); // Output: Square of 5: 25 } public static Integer applyFunction(Function<Integer, Integer> function, Integer value) { return function.apply(value); } }
3. Keep Code Readable and Maintainable
While functional programming promotes brevity, it’s essential to balance conciseness with readability. Use meaningful names for lambda expressions and functional interfaces, and avoid convoluted expressions that can confuse readers. For instance, instead of chaining many operations in a single line, break them into smaller, named functions when appropriate. This not only aids comprehension but also makes debugging easier.
import java.util.Arrays; import java.util.List; import java.util.stream.Collectors; public class ReadableStreamExample { public static void main(String[] args) { List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6); List<Integer> oddSquares = getOddSquares(numbers); System.out.println("Odd Squares: " + oddSquares); // Output: Odd Squares: [1, 9, 25] } private static List<Integer> getOddSquares(List<Integer> numbers) { return numbers.stream() .filter(n -> n % 2 != 0) // Filter odd numbers .map(n -> n * n) // Square the odd numbers .collect(Collectors.toList()); // Collect results } }
4. Leverage the Power of the Stream API
The Stream API is a powerful feature in Java for processing collections in a functional style. When working with the Stream API, prefer using method references instead of lambda expressions for improved readability. Additionally, be mindful of performance, especially with large datasets. Use parallel streams judiciously, as they can provide performance benefits when processing large collections but may introduce overhead for smaller datasets.
import java.util.Arrays; import java.util.List; public class ParallelStreamExample { public static void main(String[] args) { List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6); int sum = numbers.parallelStream() .filter(ParallelStreamExample::isOdd) // Using method reference .mapToInt(Integer::intValue) // Converting to int .sum(); System.out.println("Sum of odd numbers: " + sum); // Output: Sum of odd numbers: 9 } private static boolean isOdd(int n) { return n % 2 != 0; } }
5. Embrace Functional Interfaces
Using functional interfaces effectively can significantly enhance the modularity and reusability of your code. Define your own functional interfaces where necessary, but also make good use of Java’s built-in functional interfaces from the java.util.function package. By doing so, you make your intention clear and utilize a well-established framework that others in the Java community will recognize.
import java.util.function.Consumer; public class FunctionalInterfaceExample { public static void main(String[] args) { Consumer<String> printName = name -> System.out.println("Hello, " + name); printName.accept("Alice"); // Output: Hello, Alice } }
By following these best practices, Java developers can harness the full power of functional programming, leading to code this is not only efficient but also elegant and maintainable. The transition to a functional style may require a shift in thinking, but the benefits are well worth the effort.