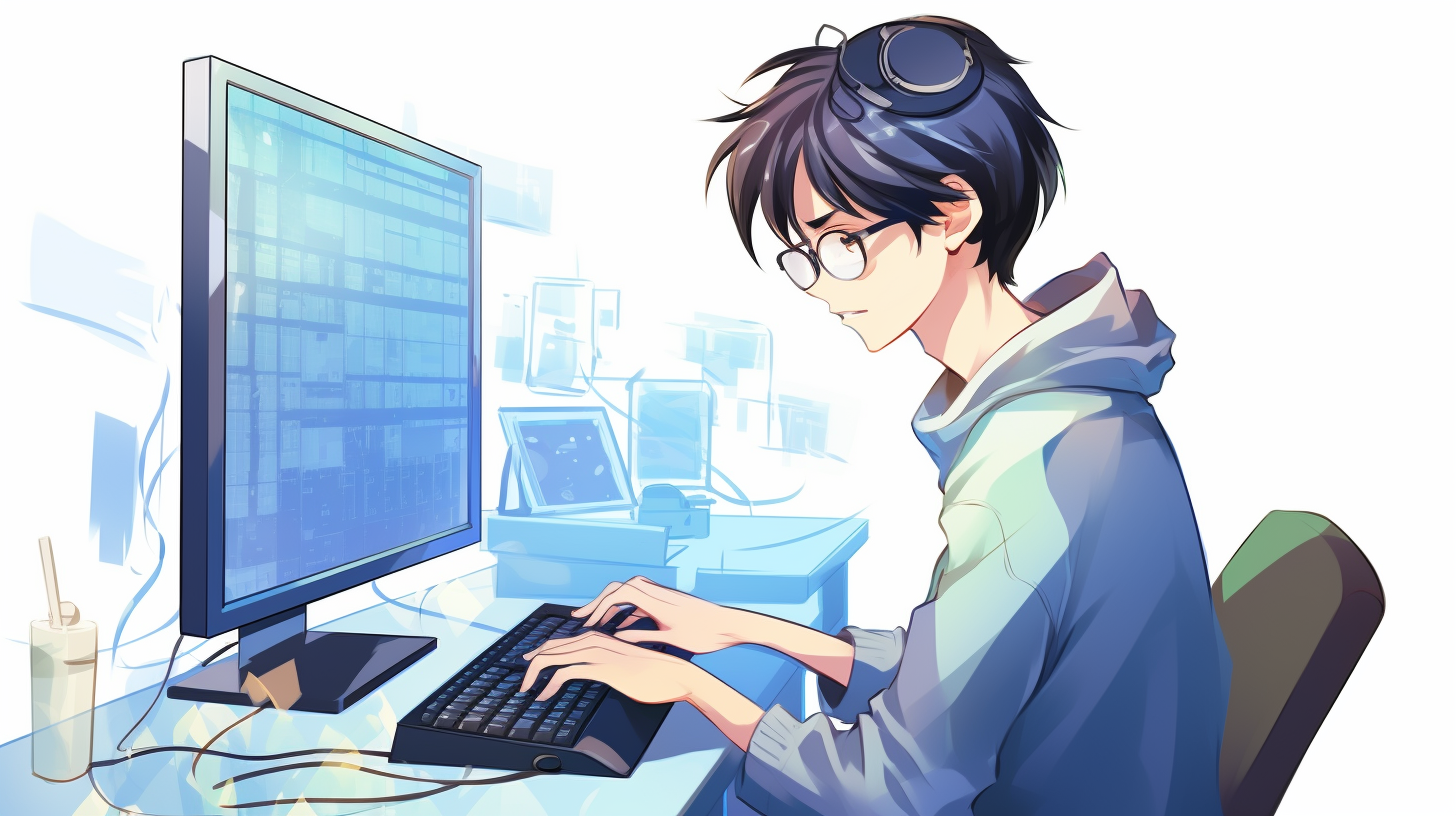
Java and Image Processing: Techniques and Libraries
Java has established itself as a versatile programming language, and its capabilities extend well into the realm of image processing. The ability to manipulate images programmatically allows developers to create dynamic applications that can analyze, edit, and enhance visual data. At its core, image processing in Java revolves around the manipulation of pixel data, which can be accomplished through various built-in libraries and techniques.
Java’s strong object-oriented principles provide a clear framework for handling images as objects, making it easier to implement complex algorithms. The main class for handling image data in Java is the BufferedImage class, part of the java.awt.image package. This class represents an image with an accessible buffer of image data, allowing for pixel-level manipulation.
Moreover, Java’s ImageIO class simplifies the process of reading and writing image files in various formats including JPEG, PNG, and GIF. Combining these classes, developers can perform a myriad of image processing tasks such as filtering, transforming, and analyzing images in real-time.
Key operations often performed in image processing include:
- Reading image files into memory.
- Rendering images onto a GUI component.
- Changing individual pixel values to alter image characteristics.
- Applying algorithms to enhance or de-emphasize certain features of an image.
- Rotating, scaling, or cropping images.
Here’s a quick example demonstrating how to load and display an image using Java’s ImageIO:
import javax.imageio.ImageIO; import javax.swing.*; import java.awt.*; import java.awt.image.BufferedImage; import java.io.File; import java.io.IOException; public class ImageLoader { public static void main(String[] args) { try { // Load the image BufferedImage image = ImageIO.read(new File("path/to/image.jpg")); // Create a JFrame to display the image JFrame frame = new JFrame(); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setSize(new Dimension(image.getWidth(), image.getHeight())); JLabel label = new JLabel(new ImageIcon(image)); frame.add(label); frame.setVisible(true); } catch (IOException e) { e.printStackTrace(); } } }
This snippet demonstrates the elementary step of loading an image file and rendering it in a window, encapsulating the power of Java’s image processing capabilities. As one delves deeper into image processing in Java, the potential expands exponentially, especially when employing popular libraries and frameworks that enhance functionality and performance.
Common Image Processing Techniques in Java
In the sphere of image processing, a variety of techniques can be employed to modify and enhance images. Each technique serves specific purposes, and understanding them especially important for any developer aiming to work with images effectively in Java. Here, we explore some of the most common image processing techniques you can implement using Java.
Image Loading: The first step in any image processing task is loading an image into memory. Java’s ImageIO class makes this simpler, enabling developers to read various image formats. Once loaded, the image can be manipulated pixel by pixel or processed through algorithms.
BufferedImage image = ImageIO.read(new File("path/to/image.png"));
Image Display: After loading an image, the next logical step is to display it. This can be accomplished easily with Java Swing components, particularly using the JLabel class to render an image icon. That is essential for applications where user feedback is necessary.
JLabel label = new JLabel(new ImageIcon(image));
Pixel Manipulation: This technique allows developers to access and modify individual pixels in an image, enabling granular control over its appearance. By manipulating pixel values—represented as integers that combine color information—you can change brightness, contrast, and even apply effects such as grayscale or sepia tones.
for (int x = 0; x < image.getWidth(); x++) { for (int y = 0; y < image.getHeight(); y++) { // Get pixel color int pixel = image.getRGB(x, y); // Extract color components int alpha = (pixel >> 24) & 0xff; int red = (pixel >> 16) & 0xff; int green = (pixel >> 8) & 0xff; int blue = pixel & 0xff; // Example: Convert to grayscale int gray = (red + green + blue) / 3; pixel = (alpha << 24) | (gray << 16) | (gray << 8) | gray; // Set new pixel value image.setRGB(x, y, pixel); } }
Filtering: Image filtering is a technique used to enhance or suppress certain features within an image. Common filters include blur, sharpen, and edge detection. These filters can be implemented using convolution matrices that traverse the image, computing new pixel values based on their neighbors.
float[] kernel = { 0, -1, 0, -1, 5, -1, 0, -1, 0 }; BufferedImage filteredImage = new BufferedImage(image.getWidth(), image.getHeight(), image.getType()); for (int x = 1; x < image.getWidth() - 1; x++) { for (int y = 1; y < image.getHeight() - 1; y++) { float redSum = 0, greenSum = 0, blueSum = 0; for (int kx = -1; kx <= 1; kx++) { for (int ky = -1; ky <= 1; ky++) { int pixel = image.getRGB(x + kx, y + ky); redSum += ((pixel >> 16) & 0xff) * kernel[(kx + 1) * 3 + (ky + 1)]; greenSum += ((pixel >> 8) & 0xff) * kernel[(kx + 1) * 3 + (ky + 1)]; blueSum += (pixel & 0xff) * kernel[(kx + 1) * 3 + (ky + 1)]; } } int newRed = Math.min(255, Math.max(0, (int) redSum)); int newGreen = Math.min(255, Math.max(0, (int) greenSum)); int newBlue = Math.min(255, Math.max(0, (int) blueSum)); filteredImage.setRGB(x, y, (image.getRGB(x, y) & 0xff000000) | (newRed << 16) | (newGreen << 8) | newBlue); } }
Image Transformation: This technique encompasses operations such as rotation, scaling, and cropping. These transformations are fundamental in applications that need to adjust image orientation or size dynamically. The Graphics2D class in Java facilitates these operations effectively.
Graphics2D g2d = (Graphics2D) image.getGraphics(); g2d.rotate(Math.toRadians(90), image.getWidth() / 2, image.getHeight() / 2); g2d.drawImage(image, 0, 0, null);
By implementing these techniques, developers can leverage Java’s powerful libraries to undertake a variety of image processing tasks, from simple to complex. Mastery of these operations not only enhances your skill set but also opens doors to sophisticated image manipulation and analysis applications.
Popular Java Libraries for Image Manipulation
When it comes to image manipulation in Java, several libraries stand out for their capabilities and ease of use. Each of these libraries brings unique features to the table, allowing developers to perform a wide range of image processing tasks with efficiency and precision. Below are some of the most popular Java libraries for image manipulation:
1. Java Advanced Imaging (JAI)
Java Advanced Imaging (JAI) is a robust library designed specifically for advanced image processing. It provides a rich set of tools for manipulating images at a high level, including support for multi-dimensional imaging and advanced filtering operations. JAI also offers a range of built-in operations, from simple image enhancements to complex image analysis.
One of the key advantages of JAI is its ability to handle large images efficiently, making it an excellent choice for applications that require processing of high-resolution images. However, JAI may be less intuitive for beginners, but its capabilities make it a powerful ally for seasoned developers.
2. Apache Commons Imaging
Apache Commons Imaging, formerly known as Sanselan, is another excellent library focused on image file format handling. This library supports a variety of formats such as JPEG, PNG, BMP, and GIF, and allows for reading and writing image metadata. It is particularly useful for applications that need to manipulate image properties without getting bogged down in specific image processing algorithms.
The simplicity of the API makes it easy to integrate into applications, allowing developers to focus on higher-level functionality rather than low-level image manipulations.
3. ImageJ
ImageJ is a powerful open-source image processing program this is often used in scientific and medical applications. It allows for extensive image analysis, offering a suite of plugins that enhance its functionality. ImageJ is well-suited for applications that require specialized image processing capabilities, such as analyzing biological data or performing measurements on images.
With its user-friendly interface and robust scripting capabilities, ImageJ is both accessible to beginners and powerful enough for advanced users. Developers can extend its functionality through Java plugins, making it a flexible tool for complex image processing tasks.
4. Processing
The Processing library is not just an image manipulation tool; it’s a flexible software sketchbook that serves as a creative coding platform. It’s designed for artists and designers, enabling them to easily create visual art through programming. While Processing is ideal for 2D and 3D graphics, it also includes a wide range of functionalities for image manipulation.
Developers can load, display, and manipulate images with minimal code, allowing for rapid prototyping and experimentation. Its simplicity and focus on visual output make it a popular choice for educational purposes and creative projects.
5. OpenCV for Java
OpenCV (Open Source Computer Vision Library) is a popular library for real-time computer vision. Although primarily known for its capabilities in areas such as video analysis and object recognition, OpenCV also provides extensive support for image processing. The Java bindings for OpenCV allow developers to leverage its powerful features directly in Java applications.
OpenCV excels in performance and is optimized for real-time operations, making it suitable for applications that require fast processing, such as live video feeds or mobile applications. It also includes advanced functionalities like machine learning and deep learning integration, providing a comprehensive suite for developers working on complex image-processing tasks.
Here is a simple example of how to use OpenCV in Java to read and display an image:
import org.opencv.core.Core; import org.opencv.core.Mat; import org.opencv.highgui.HighGui; import org.opencv.highgui.HighGui; public class OpenCVExample { static { System.loadLibrary(Core.NATIVE_LIBRARY_NAME); } public static void main(String[] args) { // Load an image from file Mat image = HighGui.imread("path/to/image.jpg"); // Display the image HighGui.imshow("Loaded Image", image); HighGui.waitKey(); } }
Choosing the right library for image manipulation in Java depends on the specific requirements of your project. Whether you need high-level image processing capabilities, simple file format support, or advanced computer vision functionalities, the above libraries provide a solid foundation for any image-related task. Each library has its strengths and weaknesses, and understanding these will enable you to select the best tools for your image processing needs.
Working with BufferedImage Class
The BufferedImage class is a cornerstone for image manipulation in Java, providing a powerful structure for handling images at the pixel level. This class extends the Image class and implements the WritableRenderedImage interface, allowing not only for pixel access but also for direct manipulation of the image data. A BufferedImage can represent images with various color models, making it adaptable to numerous image processing tasks.
One of the primary advantages of using BufferedImage is its ability to store pixel data in an accessible format. Each pixel in a BufferedImage can be manipulated using integer values that represent color information, including alpha (transparency), red, green, and blue components. This pixel-level access enables fine control over image editing, allowing developers to implement sophisticated image processing algorithms.
To create a BufferedImage, developers can utilize one of several constructors available in the class, specifying the width, height, and image type. The image type can be one of several predefined constants, such as TYPE_INT_ARGB, which supports transparency. Here’s a simple example illustrating how to create an empty BufferedImage and fill it with a color:
import java.awt.Color; import java.awt.image.BufferedImage; public class BufferedImageExample { public static void main(String[] args) { // Create a BufferedImage with width 200, height 100, and TYPE_INT_RGB BufferedImage bufferedImage = new BufferedImage(200, 100, BufferedImage.TYPE_INT_RGB); // Fill the image with a color (e.g., blue) for (int x = 0; x < bufferedImage.getWidth(); x++) { for (int y = 0; y < bufferedImage.getHeight(); y++) { bufferedImage.setRGB(x, y, Color.BLUE.getRGB()); } } } }
Beyond basic creation and manipulation, BufferedImage is equipped with methods to perform a vast range of operations, including drawing shapes, text, and other images, using the Graphics2D class. This capability is particularly useful for creating image overlays or for dynamically generating graphical content. For example, drawing text onto an image can be accomplished as follows:
import java.awt.Graphics2D; import java.awt.Font; public class DrawTextOnImage { public static void main(String[] args) { BufferedImage image = new BufferedImage(400, 200, BufferedImage.TYPE_INT_RGB); Graphics2D g2d = image.createGraphics(); // Set background color and clear the image g2d.setColor(Color.WHITE); g2d.fillRect(0, 0, image.getWidth(), image.getHeight()); // Set text color and font g2d.setColor(Color.RED); g2d.setFont(new Font("Arial", Font.BOLD, 24)); // Draw the text onto the image g2d.drawString("Hello, BufferedImage!", 50, 100); g2d.dispose(); // Dispose the graphics context // Save or display the image as needed } }
Moreover, the BufferedImage class can be used effectively in conjunction with image filtering techniques. For instance, developers can apply convolution filters to images by directly manipulating pixel data stored within the BufferedImage. This allows for advanced processing capabilities such as blurring or sharpening images, achieving results that meet specific project requirements.
In addition to pixel manipulation and drawing capabilities, BufferedImage supports image transformations. Developers can leverage the AffineTransform class to perform operations such as rotation, scaling, and shearing. That is particularly helpful when manipulating images for graphical user interfaces or when preparing images for different display resolutions.
import java.awt.Graphics2D; import java.awt.geom.AffineTransform; public class RotateBufferedImage { public static void main(String[] args) { BufferedImage originalImage = // Load or create your BufferedImage double rotationRequired = Math.toRadians(45); double locationX = originalImage.getWidth() / 2; double locationY = originalImage.getHeight() / 2; // Create a transformation for rotation AffineTransform tx = AffineTransform.getRotateInstance(rotationRequired, locationX, locationY); BufferedImage rotatedImage = new BufferedImage(originalImage.getWidth(), originalImage.getHeight(), originalImage.getType()); Graphics2D g2d = rotatedImage.createGraphics(); g2d.setTransform(tx); g2d.drawImage(originalImage, 0, 0, null); g2d.dispose(); } }
The BufferedImage class in Java is an essential tool for any developer engaged in image processing tasks. Its versatility and comprehensive feature set enable developers to perform a wide range of manipulations, from simple pixel edits to complex graphic transformations, all within the robust framework of the Java programming language.
Integrating Java with OpenCV for Advanced Processing
Integrating Java with OpenCV opens a world of possibilities for advanced image processing tasks that leverage the power of computer vision. OpenCV, renowned for its comprehensive capabilities in image and video analysis, allows Java developers to utilize its extensive feature set directly within Java applications. This integration is particularly beneficial when high performance and sophisticated algorithms are necessary, such as in robotics, augmented reality, and facial recognition applications.
To get started with OpenCV in Java, the first step is to ensure that you have the OpenCV library installed and correctly configured in your Java environment. This typically involves downloading the OpenCV package, locating the Java bindings within the package, and adding them to your project’s classpath. Once set up, the rich functionality of OpenCV can be accessed through its Java API.
Here’s a simple example demonstrating how to load an image, convert it to grayscale, and display the result using OpenCV:
import org.opencv.core.Core; import org.opencv.core.Mat; import org.opencv.core.CvType; import org.opencv.imgcodecs.Imgcodecs; import org.opencv.imgproc.Imgproc; import org.opencv.highgui.HighGui; public class OpenCVBasicExample { static { System.loadLibrary(Core.NATIVE_LIBRARY_NAME); } public static void main(String[] args) { // Load an image from file Mat image = Imgcodecs.imread("path/to/image.jpg"); // Check if image is loaded successfully if (image.empty()) { System.out.println("Error: Image not found!"); return; } // Create an empty Mat for grayscale image Mat grayImage = new Mat(image.cols(), image.rows(), CvType.CV_8UC1); // Convert the image to grayscale Imgproc.cvtColor(image, grayImage, Imgproc.COLOR_BGR2GRAY); // Display the original and grayscale images HighGui.imshow("Original Image", image); HighGui.imshow("Grayscale Image", grayImage); HighGui.waitKey(0); } }
This code snippet effectively demonstrates the entire workflow of loading an image, performing a color conversion, and displaying the results. The integration with OpenCV allows for efficient image processing operations that can be scaled to meet more complex requirements.
In addition to basic operations, OpenCV provides numerous advanced functionalities such as feature detection, image segmentation, and optical flow estimation. For instance, applying edge detection using the Canny algorithm can be done with just a few lines of code:
import org.opencv.core.Scalar; public class CannyEdgeDetection { static { System.loadLibrary(Core.NATIVE_LIBRARY_NAME); } public static void main(String[] args) { // Load an image Mat image = Imgcodecs.imread("path/to/image.jpg"); Mat edges = new Mat(); // Convert to grayscale Mat gray = new Mat(); Imgproc.cvtColor(image, gray, Imgproc.COLOR_BGR2GRAY); // Perform Canny edge detection Imgproc.Canny(gray, edges, 100, 200); // Display edges HighGui.imshow("Canny Edges", edges); HighGui.waitKey(0); } }
Furthermore, OpenCV’s support for machine learning algorithms allows developers to implement advanced techniques such as object detection and recognition. For example, using pre-trained models for face detection can be achieved seamlessly:
import org.opencv.objdetect.CascadeClassifier; public class FaceDetection { static { System.loadLibrary(Core.NATIVE_LIBRARY_NAME); } public static void main(String[] args) { // Load the cascade classifier for face detection CascadeClassifier faceDetector = new CascadeClassifier("path/to/haarcascade_frontalface_default.xml"); // Load an image Mat image = Imgcodecs.imread("path/to/image.jpg"); MatOfRect faceDetections = new MatOfRect(); // Detect faces faceDetector.detectMultiScale(image, faceDetections); for (Rect rect : faceDetections.toArray()) { // Draw rectangle around detected faces Imgproc.rectangle(image, new Point(rect.x, rect.y), new Point(rect.x + rect.width, rect.y + rect.height), new Scalar(255, 0, 0)); } // Display the result HighGui.imshow("Detected Faces", image); HighGui.waitKey(0); } }
This snippet illustrates the power of integrating OpenCV with Java to harness sophisticated image processing capabilities, enabling tasks that are often complex or computationally intensive, such as real-time facial recognition.
The integration of Java with OpenCV provides developers with a robust toolkit for advanced image processing. By using OpenCV’s extensive algorithms and capabilities, Java developers can build high-performance applications that elevate the potential of their software solutions in areas such as real-time image analysis and complex visual tasks.
Performance Optimization Tips for Image Processing in Java
Optimizing performance in image processing tasks using Java very important for achieving efficient and responsive applications. With images often comprising millions of pixels, even minor inefficiencies can lead to significant slowdowns. Below are several strategies and best practices that can help developers enhance performance when working with image processing in Java.
1. Efficient Image Representation
Choosing the right image representation is vital. The BufferedImage
class, while powerful, can consume a lot of memory, especially with large images. Using image types that match your needs—such as TYPE_BYTE_GRAY
for grayscale images—can help reduce memory usage and enhance processing speed. Here’s how to create a grayscale image:
BufferedImage grayImage = new BufferedImage(width, height, BufferedImage.TYPE_BYTE_GRAY);
2. Parallel Processing
Image processing tasks are inherently parallelizable because pixel operations can often be performed independently. Using Java’s ForkJoinPool
or ExecutorService
can significantly speed up operations by distributing tasks across multiple threads. For example, you could parallelize pixel manipulation as follows:
import java.util.concurrent.RecursiveAction; public class PixelManipulationTask extends RecursiveAction { private final BufferedImage image; private final int start; private final int end; public PixelManipulationTask(BufferedImage image, int start, int end) { this.image = image; this.start = start; this.end = end; } @Override protected void compute() { if (end - start < THRESHOLD) { for (int i = start; i < end; i++) { // Perform pixel manipulation int pixel = image.getRGB(i % image.getWidth(), i / image.getWidth()); // Process pixel... } } else { int mid = (start + end) / 2; invokeAll(new PixelManipulationTask(image, start, mid), new PixelManipulationTask(image, mid, end)); } } }
3. Avoiding Redundant Operations
Minimize repeated calculations by caching results wherever possible. For instance, if you’re applying a filter, pre-compute the kernel values outside of the pixel loop. This avoids recalculating values that do not change per pixel:
float[] kernel = { 0, -1, 0, -1, 5, -1, 0, -1, 0 }; // Cache kernel usage instead of re-computing it inside the loop for (int x = 1; x < image.getWidth() - 1; x++) { // Process pixels using kernel... }
4. Use Native Libraries
For performance-critical applications, ponder using native libraries through Java Native Interface (JNI) or Java Native Access (JNA). Native libraries such as those in OpenCV or TensorFlow can execute image processing algorithms in lower-level languages, potentially providing significant speed boosts. Here’s how you might call a native method:
public class NativeImageProcessor { public native void processImage(String path); static { System.loadLibrary("ImageProcessorLib"); } }
5. Profiling and Monitoring
Regularly profile your application using tools like VisualVM or Java Mission Control. Identifying bottlenecks in your image processing code allows for targeted optimizations. For instance, you may discover that a particular method is consuming excessive CPU time, prompting a closer look for optimization opportunities.
6. Use Buffered Streams for I/O Operations
When reading or writing images, use buffered streams to reduce the number of I/O operations. This can dramatically speed up file access. For example:
try (BufferedInputStream bis = new BufferedInputStream(new FileInputStream("path/to/image.jpg"))) { BufferedImage image = ImageIO.read(bis); }
By employing these optimization techniques, developers can significantly improve the performance of their image processing applications in Java. Each approach contributes to a more efficient use of resources, ultimately leading to faster processing times and smoother user experiences.