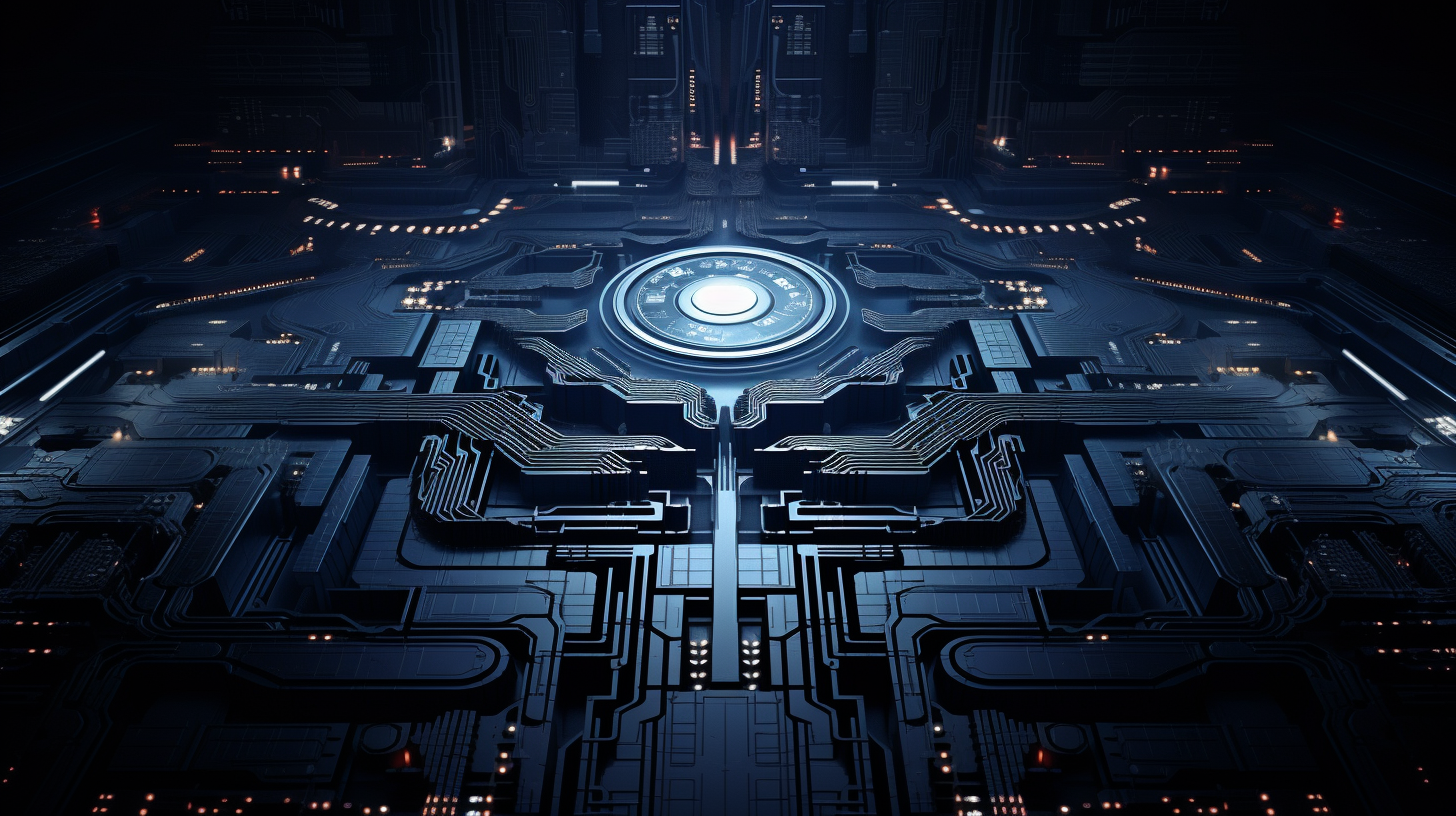
Java and JMX: Monitoring and Management
Java Management Extensions (JMX) is a powerful framework that provides a standardized way to manage and monitor Java applications. At its core, JMX allows developers to expose application resources and management operations in a way that can be easily accessed and manipulated. This capability is particularly useful for monitoring application performance, managing resources, and providing insight into the internal workings of the application.
JMX operates through a set of components that include:
- These are the central components of JMX. MBeans are Java objects that represent resources to be managed, such as applications, system resources, or any other Java object. They expose their attributes and operations, allowing external systems to interact with them.
- That’s the component responsible for managing the MBeans. It acts as a mediator between the MBeans and the external management applications. The agent provides the necessary infrastructure for the MBeans to be registered and accessed.
- JMX supports remote management capabilities, allowing applications to be monitored and managed from a remote location. This is particularly useful in large distributed systems where direct access to each application instance is not feasible.
The design of JMX is both extensible and flexible, enabling developers to define custom MBeans for their specific needs. For example, if you have an application that requires monitoring the number of active sessions, you could create a custom MBean that exposes this information.
public class SessionManager implements SessionManagerMBean { private int activeSessions; public int getActiveSessions() { return activeSessions; } public void incrementSessions() { activeSessions++; } public void decrementSessions() { if (activeSessions > 0) { activeSessions--; } } }
In this example, SessionManager
implements an MBean interface SessionManagerMBean
, exposing the number of active sessions and methods to modify that count. Once registered with the JMX agent, this MBean can be accessed remotely using JMX clients.
The use of JMX not only simplifies the management of Java applications but also enhances their maintainability. By adhering to the JMX standard, developers can leverage a variety of tools and libraries that integrate seamlessly with JMX, enabling more sophisticated monitoring and management strategies.
Key Features of JMX
One of the standout features of JMX is its ability to provide dynamic management and monitoring for Java applications without requiring significant changes to the application code. By using MBeans, developers can easily expose attributes and methods for management, making it possible to inspect and modify application state at runtime. This dynamic nature allows for real-time adjustments and monitoring, leading to more responsive and adaptive applications.
Another key feature of JMX is its support for notifications. MBeans can emit notifications when certain conditions are met, allowing management applications to respond proactively. For instance, if a system resource becomes critically low, an MBean can send a notification that can trigger automated scaling actions or alert administrators. Here’s a simple example of how an MBean might define a notification:
public class ResourceMonitor implements ResourceMonitorMBean { private int currentUsage; private NotificationBroadcasterSupport notifier = new NotificationBroadcasterSupport(); private int notificationSequenceNumber = 0; public int getCurrentUsage() { return currentUsage; } public void setCurrentUsage(int usage) { this.currentUsage = usage; if (usage > THRESHOLD) { Notification notification = new Notification("resource.usage.high", this, notificationSequenceNumber++, System.currentTimeMillis(), "Resource usage exceeded threshold"); notifier.sendNotification(notification); } } public void addNotificationListener(NotificationListener listener) { notifier.addNotificationListener(listener, null, null); } public void removeNotificationListener(NotificationListener listener) { notifier.removeNotificationListener(listener); } }
This example showcases a ResourceMonitor MBean that monitors resource usage and sends a notification if the usage exceeds a predefined threshold. The use of notifications adds a layer of responsiveness to JMX, enabling administrators to maintain control over system health dynamically.
JMX also supports a variety of protocols for remote management. The default RMI (Remote Method Invocation) protocol allows JMX clients to connect to MBeans running in application servers, making it simple to manage and monitor applications from different locations. In larger environments, this capability simplifies operational overhead, as system administrators can manage multiple instances of applications without needing to log into each one individually.
Moreover, JMX is also designed to be extensible, allowing developers to create custom protocols and serialization formats if required. This flexibility is particularly beneficial in complex architectures where standard solutions might not fit all use cases.
In addition to its core capabilities, JMX seamlessly integrates with existing Java technologies, such as Java EE and Spring, which further enhances its applicability across different projects. The ability to leverage frameworks that already support JMX allows developers to focus on building functionality rather than reinventing the wheel when it comes to management features.
Setting Up JMX in Java Applications
Setting up JMX in Java applications involves several steps to ensure that MBeans are properly registered, and the JMX agent is configured to facilitate management and monitoring. The following outlines the essential steps to integrate JMX into your Java application.
Step 1: Define MBeans
The first step is to define MBeans that represent the resources you want to manage. Each MBean should implement an interface that defines the management operations and attributes. For instance, you can create a configuration class for your MBeans:
public interface MyMBean { String getName(); void setName(String name); int getValue(); void resetValue(); }
Then, implement this interface in a class:
public class My implements MyMBean { private String name; private int value; public String getName() { return name; } public void setName(String name) { this.name = name; } public int getValue() { return value; } public void resetValue() { this.value = 0; } }
Step 2: Register MBeans with the MBean Server
Once you have defined your MBeans, you need to register them with the MBean server. The MBean server is a core component of JMX that manages the lifecycle of MBeans:
import javax.management.MBeanServer; import javax.management.ObjectName; import javax.management.MBeanServerFactory; public class JMXSetup { private static MBeanServer mBeanServer; public static void main(String[] args) throws Exception { mBeanServer = MBeanServerFactory.createMBeanServer(); My myMBean = new My(); ObjectName objectName = new ObjectName("com.example:type=MyMBean"); mBeanServer.registerMBean(myMBean, objectName); } }
Step 3: Expose the JMX Connector
To enable remote access to your MBeans, you need to expose a JMX connector. The simplest way to do this is by using the RMI connector:
import javax.management.remote.JMXConnectorServer; import javax.management.remote.JMXConnectorServerFactory; import javax.management.remote.server.RMIExporter; import javax.management.remote.rmi.RMIConnectorServer; import javax.management.remote.rmi.RMIConnectorServerFactory; import java.rmi.registry.LocateRegistry; public class JMXConnectorSetup { public static void main(String[] args) throws Exception { LocateRegistry.createRegistry(9999); JMXConnectorServer connectorServer = JMXConnectorServerFactory.newJMXConnectorServer(objectName, null, mBeanServer); connectorServer.start(); } }
In this code, we create an RMI registry on port 9999 and start a JMX connector server that allows remote management clients to access the registered MBeans.
Step 4: Configure Security
When exposing a JMX interface, especially in a production environment, security is a paramount concern. You can protect your JMX MBeans by implementing authentication and authorization mechanisms. This typically involves configuring the JMX agent to use SSL and enabling user credentials:
System.setProperty("javax.management.remote.authenticator", "com.example.MyAuthenticator"); System.setProperty("com.sun.management.jmxremote.password.file", "jmxremote.password"); System.setProperty("com.sun.management.jmxremote.access.file", "jmxremote.access"); System.setProperty("com.sun.management.jmxremote.ssl", "true");
In this configuration, the `MyAuthenticator` class would handle user authentication, and the password and access files specify permissions for different users.
Step 5: Test the JMX Setup
After setting everything up, it is essential to test the JMX configuration. You can use tools such as JConsole or VisualVM to connect to your application and inspect the registered MBeans. These tools allow you to interact with the MBeans, invoke operations, and modify attributes in real-time.
Monitoring Java Applications with JMX
Monitoring Java applications with JMX provides a robust framework for gaining insights into application performance and health. Through the use of MBeans, developers can expose pertinent metrics and operational controls, making it feasible to observe and manage applications dynamically. This capability is pivotal in modern application development, where maintaining optimal performance is essential.
When monitoring Java applications, the first step is to define the MBeans that you want to use. Each MBean can represent a specific resource or a set of related operations. For instance, consider an MBean designed to monitor the performance of a service:
public interface PerformanceMonitorMBean { long getRequestCount(); double getAverageResponseTime(); void resetMetrics(); } public class PerformanceMonitor implements PerformanceMonitorMBean { private long requestCount; private double totalResponseTime; public long getRequestCount() { return requestCount; } public double getAverageResponseTime() { return requestCount == 0 ? 0 : totalResponseTime / requestCount; } public void recordResponseTime(double responseTime) { totalResponseTime += responseTime; requestCount++; } public void resetMetrics() { requestCount = 0; totalResponseTime = 0; } }
This example creates a `PerformanceMonitor` MBean that tracks the total number of requests and calculates the average response time. Developers can invoke `recordResponseTime` whenever a request is processed, thereby keeping metrics up to date.
Once the MBeans are defined, the next step is to register them with the MBean server:
import javax.management.MBeanServer; import javax.management.ObjectName; import javax.management.MBeanServerFactory; public class MonitoringSetup { private static MBeanServer mBeanServer; public static void main(String[] args) throws Exception { mBeanServer = MBeanServerFactory.createMBeanServer(); PerformanceMonitor monitor = new PerformanceMonitor(); ObjectName objectName = new ObjectName("com.example:type=PerformanceMonitor"); mBeanServer.registerMBean(monitor, objectName); } }
With the `PerformanceMonitor` registered, it can now be accessed for monitoring through JMX clients. This registration very important for exposing the metrics that will be monitored.
To actually monitor the application, tools like JConsole or VisualVM can be utilized. These tools provide graphical interfaces to connect to the JMX-enabled application and allow administrators to view attributes and invoke operations on the MBeans. For example, in JConsole, you can observe the `PerformanceMonitor` MBean and see real-time updates of the `requestCount` and `averageResponseTime` metrics.
JMX also supports the capability to set up notifications, which enables your application to proactively inform you of critical conditions. For instance, you could enhance the `PerformanceMonitor` to notify when the average response time exceeds a certain threshold:
import javax.management.Notification; import javax.management.NotificationBroadcasterSupport; public class EnhancedPerformanceMonitor extends PerformanceMonitor { private NotificationBroadcasterSupport notifier = new NotificationBroadcasterSupport(); private int notificationSequenceNumber = 0; @Override public void recordResponseTime(double responseTime) { super.recordResponseTime(responseTime); if (getAverageResponseTime() > THRESHOLD) { Notification notification = new Notification("performance.threshold.exceeded", this, notificationSequenceNumber++, System.currentTimeMillis(), "Average response time exceeded threshold"); notifier.sendNotification(notification); } } public void addNotificationListener(NotificationListener listener) { notifier.addNotificationListener(listener, null, null); } public void removeNotificationListener(NotificationListener listener) { notifier.removeNotificationListener(listener); } }
In this enhanced example, the `EnhancedPerformanceMonitor` class extends the `PerformanceMonitor` and adds notification functionality. If the average response time exceeds a predefined threshold, a notification is sent out. This feature allows for reactive monitoring, enabling administrators to take action as needed.
Managing Resources with JMX
Managing resources in Java applications using JMX involves using the capabilities of MBeans to provide a structured approach to resource management. Through JMX, developers can not only monitor the state of application resources but also manage them dynamically based on real-time conditions.
One of the core advantages of using JMX for resource management is the ability to encapsulate resource-related functionality within MBeans. This encapsulation allows specific resources, such as database connections, thread pools, or cache instances, to be controlled and monitored efficiently. Here’s how you can set up an MBean for managing a simple thread pool:
public interface ThreadPoolMBean { int getActiveCount(); void setMaxPoolSize(int maxSize); void execute(Runnable task); } public class ThreadPool implements ThreadPoolMBean { private final ExecutorService executorService; private int maxPoolSize; public ThreadPool(int maxPoolSize) { this.maxPoolSize = maxPoolSize; this.executorService = Executors.newFixedThreadPool(maxPoolSize); } public int getActiveCount() { return ((ThreadPoolExecutor) executorService).getActiveCount(); } public void setMaxPoolSize(int maxSize) { this.maxPoolSize = maxSize; ((ThreadPoolExecutor) executorService).setCorePoolSize(maxSize); } public void execute(Runnable task) { executorService.execute(task); } }
In this example, the `ThreadPool` class implements the `ThreadPoolMBean` interface. It exposes methods to get the number of active threads, set the maximum pool size, and execute tasks. This allows administrators to modify the behavior of the thread pool at runtime by changing the maximum pool size based on load.
To manage the lifecycle of this MBean, you need to register it with the MBean server. Here’s how you can do that:
import javax.management.MBeanServer; import javax.management.ObjectName; import javax.management.MBeanServerFactory; public class ResourceManagementSetup { private static MBeanServer mBeanServer; public static void main(String[] args) throws Exception { mBeanServer = MBeanServerFactory.createMBeanServer(); ThreadPool threadPool = new ThreadPool(10); // Initial pool size of 10 ObjectName objectName = new ObjectName("com.example:type=ThreadPool"); mBeanServer.registerMBean(threadPool, objectName); } }
Once the `ThreadPool` MBean is registered, you can access it via JMX clients like JConsole or VisualVM. These tools enable you to invoke the `setMaxPoolSize` method dynamically to adjust resource allocation according to current application demands.
Another critical aspect of resource management with JMX is the ability to handle resource limits and constraints effectively. For instance, you can implement custom logic in your MBean to prevent over-utilization of resources:
public class SafeThreadPool extends ThreadPool { private final int maxUsage; public SafeThreadPool(int maxPoolSize, int maxUsage) { super(maxPoolSize); this.maxUsage = maxUsage; } @Override public void execute(Runnable task) { if (getActiveCount() < maxUsage) { super.execute(task); } else { throw new RuntimeException("Maximum usage exceeded!"); } } }
In the `SafeThreadPool`, an additional constraint is introduced to limit the maximum number of active tasks. Attempting to execute a new task when the limit is reached results in an exception. This ensures applications remain stable even under heavy load.
Furthermore, JMX supports notifications, making it possible for MBeans to alert administrators about critical resource conditions. For example, we could modify our thread pool to notify when the maximum usage threshold is approached:
import javax.management.Notification; import javax.management.NotificationBroadcasterSupport; public class NotifyingThreadPool extends SafeThreadPool { private NotificationBroadcasterSupport notifier = new NotificationBroadcasterSupport(); private int notificationSequenceNumber = 0; public NotifyingThreadPool(int maxPoolSize, int maxUsage) { super(maxPoolSize, maxUsage); } @Override public void execute(Runnable task) { if (getActiveCount() >= maxUsage) { Notification notification = new Notification("threadpool.usage.high", this, notificationSequenceNumber++, System.currentTimeMillis(), "Thread pool usage is high"); notifier.sendNotification(notification); } super.execute(task); } public void addNotificationListener(NotificationListener listener) { notifier.addNotificationListener(listener, null, null); } public void removeNotificationListener(NotificationListener listener) { notifier.removeNotificationListener(listener); } }
The `NotifyingThreadPool` extends `SafeThreadPool` and sends a notification if the maximum usage limit is reached. This feature allows proactive resource management, enabling system administrators to respond swiftly to potential issues.
Best Practices for JMX Implementation
When implementing JMX in your Java applications, adhering to best practices especially important for ensuring performance, scalability, and maintainability. Here are several key guidelines that can help you effectively leverage JMX’s capabilities.
1. Define Clear MBean Interfaces
Start by designing MBean interfaces that are logical and cohesive. Each MBean should represent a single concept or resource. This not only simplifies the implementation but also makes it easier for consumers of your MBeans to understand and use them. For instance, having a distinct MBean for thread pools, database connections, or application metrics can help in organizing management tasks efficiently.
public interface ThreadPoolMBean { int getActiveCount(); void setMaxPoolSize(int maxSize); void execute(Runnable task); }
2. Limit the Number of MBeans
While it may be tempting to create an MBean for every small feature, it’s essential to maintain a balance. Excessive MBeans can clutter the MBean server and lead to performance issues. Group related functionalities into fewer MBeans where practical. For example, instead of separate MBeans for each cache instance, ponder a single MBean that manages multiple caches as a collection.
3. Use Notifications Wisely
Notifications can enhance your application’s responsiveness by alerting you to state changes. However, they can also lead to performance degradation if overused. Limit notifications to critical events that require immediate attention. This will reduce the noise in your monitoring systems and help focus on significant issues. For example, notify only when resource usage crosses a critical threshold.
public class ResourceMonitor implements ResourceMonitorMBean {
private NotificationBroadcasterSupport notifier = new NotificationBroadcasterSupport();