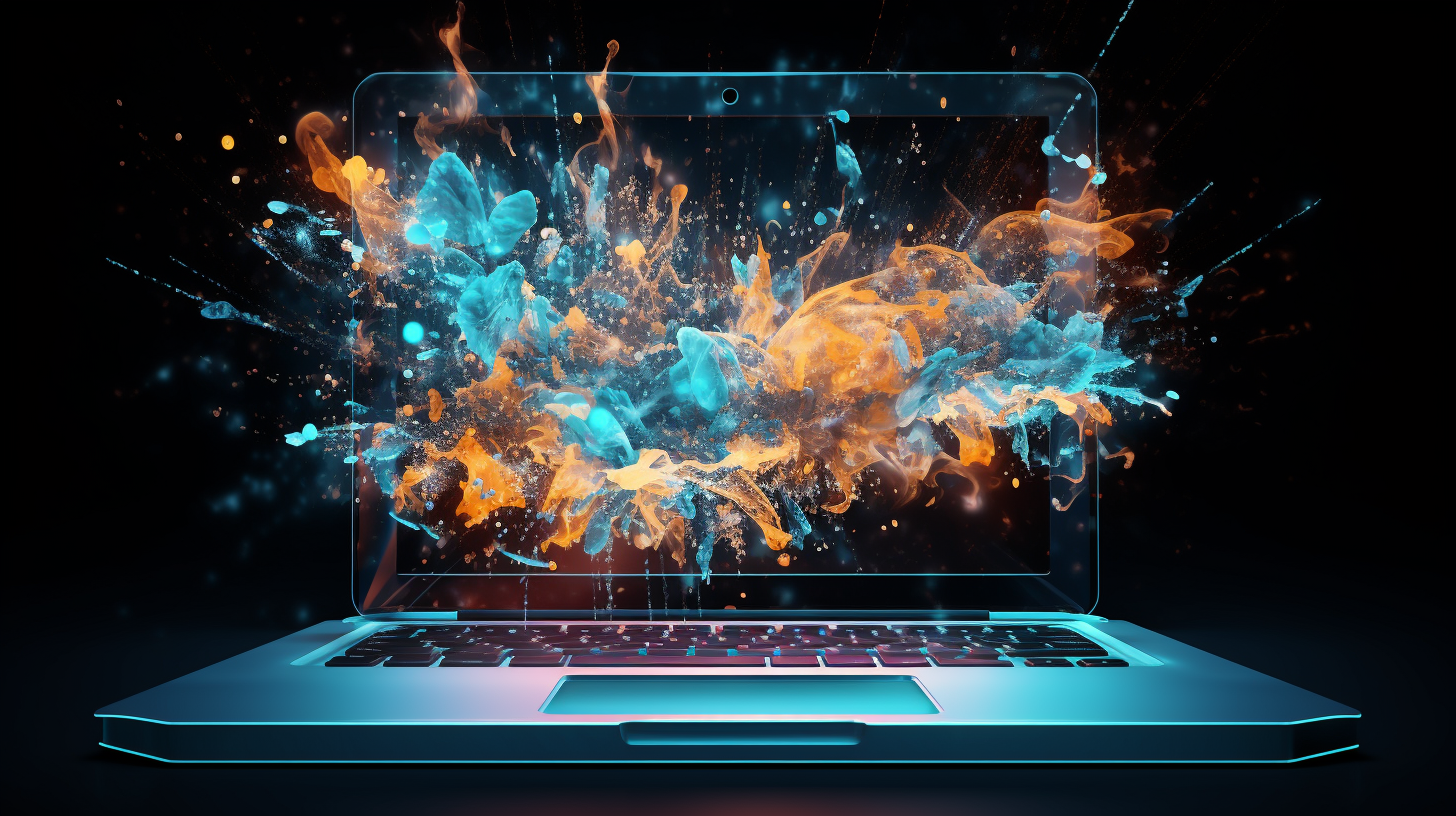
Java and Kubernetes: Orchestrating Containers
In the sphere of modern software development, the intersection of Java applications and containerization has become a pivotal area of focus. Java, known for its portability and robustness, integrates seamlessly with container technologies, enabling developers to encapsulate applications along with their dependencies into lightweight, portable containers. This encapsulation allows for greater consistency across different environments, whether for development, testing, or production.
A containerized environment allows Java applications to run in isolation, which eliminates the traditional “it works on my machine” dilemma. Each container can contain a specific version of the Java Runtime Environment (JRE) and libraries, ensuring that the application behaves consistently regardless of where it’s deployed.
To understand how Java fits into this paradigm, let’s think the lifecycle of a typical Java application within a container. First, developers package their Java application – typically a JAR or WAR file – along with a Dockerfile that specifies how to build the container image.
# Example Dockerfile for a Java application FROM openjdk:11-jre-slim VOLUME /tmp COPY target/my-java-app.jar app.jar ENTRYPOINT ["java","-jar","/app.jar"]
This Dockerfile specifies an OpenJDK base image, copies the built JAR file into the image, and sets the entry point to run the Java application when the container starts. The use of a slim JRE image helps to keep the container lightweight, which is important for performance and efficiency.
Once the Docker image is built, it can be deployed on various platforms that support container orchestration, with Kubernetes being one of the most popular choices. Kubernetes abstracts away the complexities of managing containers at scale, allowing developers to focus more on application development rather than infrastructure concerns.
Within a containerized architecture, several Java frameworks and libraries enhance the performance and efficiency of applications. For instance, Spring Boot simplifies the setup of Java applications by providing out-of-the-box configuration options that are particularly useful in cloud-native environments. It can be easily integrated with Kubernetes to facilitate deployment and management.
In a containerized setup, best practices such as keeping containers stateless, using external databases or storage, and employing microservices architecture are recommended. This approach not only enhances scalability but also allows individual components of the application to be updated or scaled independently.
Moreover, the ability to manage Java applications via Kubernetes manifests, which are written in YAML, provides a declarative way to define the desired state of applications. Each manifest describes how Kubernetes should run a container, including aspects such as resource requirements, networking, and storage.
# Example Kubernetes Deployment for a Java application apiVersion: apps/v1 kind: Deployment metadata: name: my-java-app spec: replicas: 3 selector: matchLabels: app: my-java-app template: metadata: labels: app: my-java-app spec: containers: - name: my-java-app image: my-java-app:latest ports: - containerPort: 8080
This YAML snippet defines a deployment for the Java application, specifying that three replicas should run for fault tolerance and load balancing. Kubernetes will automatically manage these replicas, ensuring that the desired state is maintained.
Kubernetes Architecture and Components
To effectively harness the power of Kubernetes for managing Java applications, it’s essential to comprehend the architecture and components that form the backbone of this orchestration platform. Kubernetes is designed to provide a robust framework for deploying, scaling, and operating application containers across clusters of hosts, offering a significant edge in managing containerized applications in dynamic environments.
At its core, Kubernetes operates on a cluster model comprising master and worker nodes. The master node is responsible for managing the Kubernetes cluster, coordinating activities, and making decisions regarding the deployment and scaling of applications. In contrast, worker nodes host the containers and run the application workloads. This separation of responsibilities enables Kubernetes to maintain high availability and scalability.
Key components of the Kubernetes architecture include:
- The API server is the central management entity that exposes the Kubernetes API. It serves as the primary interface for users and components to interact with the cluster. All operations, including deployments and scaling, are executed through the API server.
- That’s a distributed key-value store that Kubernetes uses to maintain the state of the cluster. All configuration data and state information are stored in etcd, ensuring that the desired state of the system is preserved and recoverable.
- Controllers are background processes that regulate the state of the cluster. The controller manager runs various controllers that handle routine tasks such as ensuring the desired number of replicas and managing node statuses.
- The scheduler is responsible for placing newly created pods onto available nodes based on resource availability and constraints defined in the deployment specifications. Its role especially important for optimizing resource use across the cluster.
- These are the machines (physical or virtual) that run the containerized applications. Each node contains the necessary services to run pods, including a container runtime (like Docker), kubelet, and kube-proxy.
- The smallest deployable units in Kubernetes, pods can host one or more containers that share the same network namespace. In the context of Java applications, a pod typically contains one Java application container and can be scaled as needed.
- Services provide stable networking for pods, enabling communication between them. They abstract the underlying pods, allowing users to interact with a consistent endpoint rather than dealing with dynamic IP addresses.
In a Kubernetes environment, managing Java applications involves deploying these applications as pods, defining their desired state through deployment manifests, and ensuring network and storage configurations are in place. Kubernetes allows for declarative configurations, which means developers can specify what they want rather than how to achieve it, leading to a more simpler management experience.
With a solid understanding of Kubernetes architecture and components, Java developers can harness its capabilities to orchestrate their applications efficiently. The seamless interaction between Java and Kubernetes fosters a resilient and scalable environment, ideal for microservices-oriented architectures.
Deploying Java Applications on Kubernetes
Deploying Java applications on Kubernetes is a critical step in realizing the benefits of container orchestration. The deployment process involves a series of well-defined steps that ensure your Java application runs smoothly in the Kubernetes environment. By using Kubernetes’ capabilities, developers can create resilient, scalable, and easily manageable applications.
The deployment begins with packaging your Java application into a container image, which has already been covered with the Dockerfile example. Once you have your Docker image ready, the next step is to push this image to a container registry, whether it be Docker Hub, Google Container Registry, or another choice. This registry acts as a central repository from where Kubernetes can pull your application image during deployment.
To deploy the Java application, you will create a Kubernetes Deployment manifest. This manifest defines the desired state of your application, including the container image to use, the number of replicas, and the ports to expose. Here’s a more detailed look at the Kubernetes Deployment manifest for a Java application:
apiVersion: apps/v1 kind: Deployment metadata: name: my-java-app labels: app: my-java-app spec: replicas: 3 selector: matchLabels: app: my-java-app template: metadata: labels: app: my-java-app spec: containers: - name: my-java-app image: my-java-app:latest ports: - containerPort: 8080 env: - name: SPRING_PROFILES_ACTIVE value: "prod"
This manifest outlines several key components:
- This field indicates that three instances of the Java application should be running at all times, providing redundancy and load balancing.
- This section identifies which pods the deployment will manage based on the labels defined.
- The template describes the pod configuration, including the image to be used, the ports to expose, and environment variables needed for the application.
After preparing your Deployment manifest, the next step is to apply it using the Kubernetes command-line tool, kubectl:
kubectl apply -f my-java-app-deployment.yaml
Upon execution, Kubernetes will create the specified number of pods according to the manifest. You can monitor the status of the deployment with the following command:
kubectl get deployments
Once the pods are up and running, you’ll need to expose them to the outside world. That is typically done using a Kubernetes Service, which provides stable networking to your application. Here’s an example of a Service manifest to expose your Java application:
apiVersion: v1 kind: Service metadata: name: my-java-app-service spec: type: LoadBalancer ports: - port: 80 targetPort: 8080 selector: app: my-java-app
This Service manifests allows external traffic to reach your application by mapping port 80 on the load balancer to port 8080 on your Java application pods.
To create the service, you would again use the kubectl command:
kubectl apply -f my-java-app-service.yaml
After your service is deployed, it will provide an external IP address through which you can access your Java application. You can retrieve the service information by running:
kubectl get services
With the service in place, your Java application is now fully deployed on Kubernetes, seamlessly handling incoming requests while benefiting from the orchestration capabilities offered by the platform.
Managing Configurations and Secrets in Kubernetes
In a Kubernetes environment, managing configurations and secrets effectively is paramount, especially for Java applications that often rely on various external configurations such as database URLs, API keys, and other sensitive information. Kubernetes provides powerful mechanisms to handle these scenarios through ConfigMaps and Secrets, allowing developers to decouple configuration data from container images, thereby promoting better application management and security.
A ConfigMap in Kubernetes is an object that allows you to store non-sensitive configuration data in key-value pairs. That is particularly useful for Java applications, which can be configured to read these values at runtime. For instance, if your Java application relies on different environment configurations, a ConfigMap can be used to manage these settings without altering the application code or rebuilding the Docker images.
Here’s an example of how you can create a ConfigMap for a Java application:
apiVersion: v1 kind: ConfigMap metadata: name: my-java-app-config data: DATABASE_URL: jdbc:mysql://db-host:3306/mydb DATABASE_USER: myuser DATABASE_PASSWORD: mypassword
In this example, we create a ConfigMap named my-java-app-config
that holds database connection details. The Java application can access these values via environment variables, ensuring that sensitive information remains outside of the application code.
To use the ConfigMap in a deployment, you can reference the keys in your manifest like so:
apiVersion: apps/v1 kind: Deployment metadata: name: my-java-app spec: replicas: 3 selector: matchLabels: app: my-java-app template: metadata: labels: app: my-java-app spec: containers: - name: my-java-app image: my-java-app:latest ports: - containerPort: 8080 env: - name: DATABASE_URL valueFrom: configMapKeyRef: name: my-java-app-config key: DATABASE_URL - name: DATABASE_USER valueFrom: configMapKeyRef: name: my-java-app-config key: DATABASE_USER - name: DATABASE_PASSWORD valueFrom: configMapKeyRef: name: my-java-app-config key: DATABASE_PASSWORD
By using the valueFrom
field, we ensure that our Java application can access the configuration seamlessly, without hardcoding sensitive values.
For sensitive information, Kubernetes provides the Secret resource, which is specifically designed to hold sensitive data such as passwords, OAuth tokens, and SSH keys. Secrets are encoded in base64 and can be mounted as environment variables or files in a pod. This mechanism ensures that sensitive data is kept secure while still accessible by the applications that require them.
Here’s an example of creating a Secret for sensitive configuration:
apiVersion: v1 kind: Secret metadata: name: my-java-app-secret type: Opaque data: DATABASE_PASSWORD: bXlwYXNzd29yZA== # base64 encoded 'mypassword'
Similar to the ConfigMap, you can reference the Secret in your deployment manifest:
apiVersion: apps/v1 kind: Deployment metadata: name: my-java-app spec: replicas: 3 selector: matchLabels: app: my-java-app template: metadata: labels: app: my-java-app spec: containers: - name: my-java-app image: my-java-app:latest ports: - containerPort: 8080 env: - name: DATABASE_PASSWORD valueFrom: secretKeyRef: name: my-java-app-secret key: DATABASE_PASSWORD
Scaling Java Microservices with Kubernetes
Scaling Java microservices with Kubernetes is a necessity in today’s dynamic application landscape, where demand can fluctuate dramatically. Kubernetes provides powerful capabilities to automatically manage application scaling, ensuring that your Java microservices can handle varying workloads efficiently without manual intervention.
At the heart of Kubernetes’ scaling capabilities is the concept of the Horizontal Pod Autoscaler (HPA). The HPA automatically adjusts the number of pods in a deployment based on observed metrics, typically CPU use or custom metrics. This allows your Java microservices to scale out when traffic increases and scale in when demand decreases, optimizing resource usage and cost.
To implement HPA for your Java application, you first need to ensure that your application is capable of providing metrics for scaling. One common approach is to integrate with the Kubernetes Metrics Server, which collects resource usage data. You can easily set this up in your cluster by deploying the Metrics Server with the following command:
kubectl apply -f https://github.com/kubernetes-sigs/metrics-server/releases/latest/download/components.yaml
Next, you can create an HPA resource that specifies the desired scaling behavior for your Java microservice. Here’s an example manifest for an HPA that scales the my-java-app
deployment based on CPU usage:
apiVersion: autoscaling/v2beta2 kind: HorizontalPodAutoscaler metadata: name: my-java-app-hpa spec: scaleTargetRef: apiVersion: apps/v1 kind: Deployment name: my-java-app minReplicas: 2 maxReplicas: 10 metrics: - type: Resource resource: name: cpu target: type: Use averageUtilization: 50
This manifest defines an HPA that will maintain between 2 and 10 replicas of the my-java-app
deployment, adjusting the number of pods based on CPU use. If the average CPU usage across the pods exceeds 50%, Kubernetes will automatically scale up the number of replicas to meet the demand, and scale down when the use drops.
To apply the HPA configuration, run the following command:
kubectl apply -f my-java-app-hpa.yaml
Once the HPA is in place, you can monitor its status and the scaling activity with:
kubectl get hpa
In addition to the HPA, Kubernetes supports other scaling features such as manually scaling deployments and using custom metrics for more granular control. For instance, you can manually scale the number of replicas of your Java application using:
kubectl scale deployment my-java-app --replicas=5
This command sets the number of running pods to 5, allowing you to respond to immediate needs without relying solely on automation.
For more complex scenarios, you might want to leverage custom metrics. By using tools such as Prometheus to collect application-specific metrics and configuring the HPA to use these metrics, you can create a highly responsive scaling solution tailored to the unique performance characteristics of your Java microservices.
Monitoring and Troubleshooting Java Applications in Kubernetes
Monitoring and troubleshooting Java applications in Kubernetes is an important aspect that cannot be overlooked. As applications become distributed and stateless, understanding their performance, behavior, and potential issues in real-time becomes essential. Kubernetes provides a robust framework for monitoring, while also allowing Java developers to utilize various tools and libraries that can help track application health and performance metrics.
Java applications often integrate with monitoring solutions such as Prometheus, Grafana, and ELK Stack (Elasticsearch, Logstash, Kibana) to provide insights into application performance and to facilitate troubleshooting. By collecting metrics, logs, and traces, these tools help developers identify performance bottlenecks, error rates, and resource use, leading to faster resolutions of production issues.
To enable monitoring for your Java application running in Kubernetes, you typically start by instrumenting your application code using libraries like Micrometer or Spring Boot Actuator. These libraries allow your application to expose metrics in a format that Prometheus can scrape. Here’s how you can integrate Micrometer with a Spring Boot application:
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; @SpringBootApplication public class MyJavaApp { public static void main(String[] args) { SpringApplication.run(MyJavaApp.class, args); } @RestController public class HelloController { @GetMapping("/hello") public String hello() { return "Hello, Kubernetes!"; } } }
With Micrometer integrated, you can expose various application metrics by simply including the following dependency in your `pom.xml` if you’re using Maven:
io.micrometer micrometer-registry-prometheus
Once your application is instrumented, you must also deploy Prometheus to scrape the metrics. Here’s a sample configuration for a Prometheus deployment in Kubernetes that targets your Java application’s metrics endpoint:
apiVersion: apps/v1 kind: Deployment metadata: name: prometheus spec: replicas: 1 selector: matchLabels: app: prometheus template: metadata: labels: app: prometheus spec: containers: - name: prometheus image: prom/prometheus ports: - containerPort: 9090 volumeMounts: - name: config-volume mountPath: /etc/prometheus/ volumes: - name: config-volume configMap: name: prometheus-config --- apiVersion: v1 kind: ConfigMap metadata: name: prometheus-config data: prometheus.yml: | global: scrape_interval: 15s scrape_configs: - job_name: 'java-app' metrics_path: '/actuator/prometheus' static_configs: - targets: ['my-java-app-service:8080']
In this configuration, Prometheus is set to scrape metrics from the Java application exposed at the `/actuator/prometheus` endpoint every 15 seconds. Make sure you have the appropriate network policies and service configurations to allow Prometheus to access your application.
Once your application metrics are being collected, you can visualize them using Grafana, which allows you to create dashboards that provide a clear overview of your Java application’s performance. Integrating Grafana with Prometheus is straightforward; you just need to configure Grafana to use Prometheus as a data source, and you can create visualizations based on the metrics collected.
In addition to metrics monitoring, logging is a critical component for troubleshooting. By using a centralized logging solution such as the ELK Stack, you can aggregate logs from all your Java application instances running in Kubernetes. Each log entry can include critical context like timestamps, error levels, and stack traces, enabling quick diagnosis of issues.
You can configure your Java application to send logs to Elasticsearch using a logging framework like Logback or Log4j, typically through an HTTP appender. Here’s a basic example using Logback: