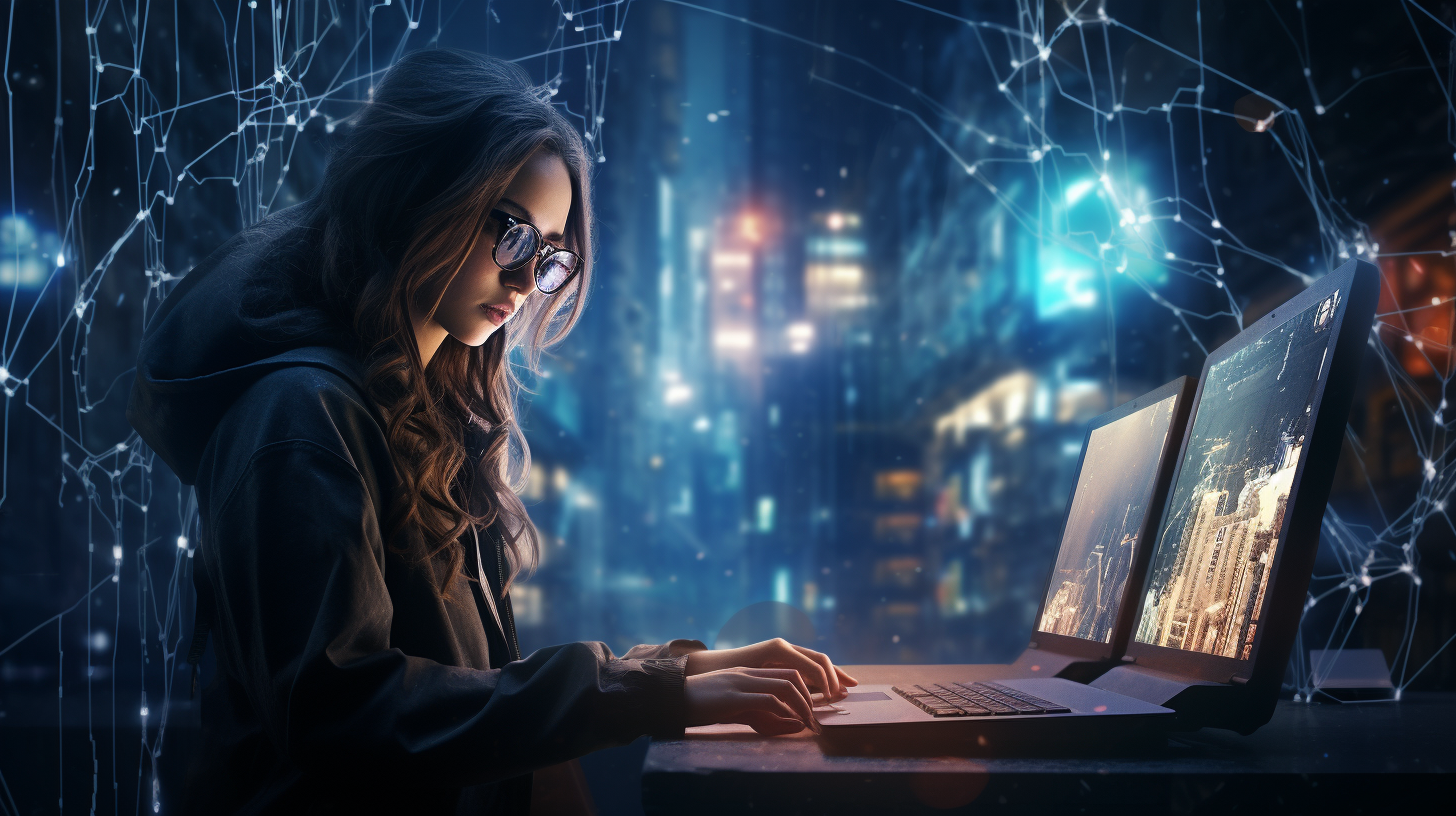
Java and Machine Learning: An Introduction
Java has long been a staple in the context of software development, but its role in machine learning is often underappreciated. Java’s platform independence, strong performance, and extensive ecosystem make it a formidable contender in the machine learning arena. What sets Java apart is its robust feature set that allows developers to build scalable, maintainable, and efficient applications. Unlike languages that cater specifically to data science, Java brings its rich set of libraries and frameworks to the table, making it an excellent choice for developing machine learning applications.
One of the key advantages of using Java for machine learning is its object-oriented nature. This allows for organized and reusable code, which is important in machine learning projects where complexity can grow rapidly. With Java, developers can encapsulate different components of their algorithms, making it easier to manage and update the codebase as the project evolves.
Java also excels in handling large-scale data processing tasks, which is essential in machine learning. Frameworks like Apache Hadoop and Apache Spark, both of which can be utilized in Java, allow for distributed data processing that can significantly speed up the training of machine learning models. The ability to process large datasets in parallel is a game-changer, especially when working with big data.
Additionally, Java’s strong typing system and exception handling capabilities contribute to more robust code. These features help catch errors at compile time, reducing runtime exceptions that could derail the training of a machine learning model. This is particularly beneficial in machine learning, where issues in data or logic can lead to flawed models.
A practical example of Java’s capabilities can be seen in the implementation of a simple linear regression model. Think the following code snippet:
import org.apache.commons.math3.stat.regression.SimpleRegression; public class LinearRegressionExample { public static void main(String[] args) { SimpleRegression regression = new SimpleRegression(); // Adding data points (x, y) regression.addData(1, 2); regression.addData(2, 3); regression.addData(3, 5); regression.addData(4, 7); // Getting the slope and intercept double slope = regression.getSlope(); double intercept = regression.getIntercept(); System.out.println("Slope: " + slope); System.out.println("Intercept: " + intercept); } }
In this example, the SimpleRegression
class from the Apache Commons Math library is used to perform linear regression. The code demonstrates how easily data points can be added and how the model parameters—slope and intercept—can be retrieved, showcasing Java’s capability to handle basic machine learning tasks effectively.
Moreover, Java’s integration capabilities with other technologies further enhance its role in machine learning. It can seamlessly interact with databases for data retrieval and storage, as well as with web services for data exchange. This interoperability means that Java can be a central player in a broader machine learning ecosystem, connecting various data sources and processing frameworks.
Java’s role in machine learning is characterized by its strong performance, rich feature set, and the ability to handle complex data processing tasks. Its robust libraries and frameworks, combined with the language’s inherent strengths, make it an ideal choice for developers looking to delve into the field of machine learning.
Key Libraries for Machine Learning in Java
When it comes to machine learning in Java, a few key libraries stand out that facilitate the development of robust machine learning applications. These libraries provide a range of tools that simplify modeling, data manipulation, and algorithm implementation, making Java a versatile choice for machine learning tasks.
Weka is one of the most popular machine learning libraries available in Java. It offers a comprehensive collection of machine learning algorithms for data mining tasks, including classification, regression, clustering, and association rules. Weka also comes with a graphical user interface, which allows users to visualize and analyze data without needing extensive programming knowledge. The following code snippet demonstrates how to use Weka to train a simple decision tree classifier:
import weka.classifiers.trees.J48; import weka.core.Instances; import weka.core.converters.ConverterUtils; public class WekaExample { public static void main(String[] args) throws Exception { // Load dataset ConverterUtils.DataSource source = new ConverterUtils.DataSource("path/to/your/dataset.arff"); Instances data = source.getDataSet(); // Set class index to the last attribute data.setClassIndex(data.numAttributes() - 1); // Build classifier J48 tree = new J48(); tree.buildClassifier(data); // Output model System.out.println(tree); } }
In this example, we use Weka’s J48 classifier, which implements the C4.5 algorithm for generating decision trees. The code shows how to load a dataset from an ARFF file, set the class index, and build the classifier, thereby providing a simpler pathway for users to implement machine learning models.
Deeplearning4j is another essential library, specifically designed for deep learning. It provides a rich set of tools for building, training, and deploying deep neural networks, and it integrates well with Hadoop and Spark for distributed computing. Here’s how you might define a simple neural network with Deeplearning4j:
import org.deeplearning4j.nn.conf.MultiLayerConfiguration; import org.deeplearning4j.nn.conf.NeuralNetConfiguration; import org.deeplearning4j.nn.multilayer.MultiLayerNetwork; import org.nd4j.linalg.learning.config.Adam; public class Deeplearning4jExample { public static void main(String[] args) { MultiLayerConfiguration config = new NeuralNetConfiguration.Builder() .updater(new Adam(0.001)) .list() .layer(0, new DenseLayer.Builder().nIn(784).nOut(100).activation(Activation.RELU).build()) .layer(1, new OutputLayer.Builder().nIn(100).nOut(10).activation(Activation.SOFTMAX).build()) .build(); MultiLayerNetwork model = new MultiLayerNetwork(config); model.init(); System.out.println("Model summary: " + model.summary()); } }
In this snippet, we configure a simple feedforward neural network with one hidden layer and an output layer using the Adam optimizer. The code demonstrates how to set up the layers and initialize the model, illustrating the ease with which Deeplearning4j allows developers to experiment with neural network architectures.
MOA (Massive Online Analysis) is another library worth mentioning, especially for those interested in stream data mining. MOA focuses on real-time machine learning, enabling the analysis of data streams and providing various algorithms optimized for such tasks. This capability is increasingly relevant in today’s data-driven applications, where data is generated continuously.
Additionally, Java has several other libraries like Apache Spark MLlib for scalable machine learning on large datasets and Java-ML, which offers a variety of algorithms and tools for machine learning in Java.
The richness of Java’s ecosystem, combined with these powerful libraries, positions Java as a strong candidate for machine learning tasks. Whether you are building simple models or complex neural networks, the libraries available in Java simplify the development process, allowing developers to focus on crafting effective algorithms rather than getting bogged down by implementation details.
Building Machine Learning Models with Java
Building machine learning models in Java involves using its rich library ecosystem and employing best practices to ensure efficient and maintainable code. The process typically begins with defining the problem you want to solve, selecting the appropriate algorithms, and preparing your data. With Java, you can construct various types of models, from simple linear regressions to complex neural networks.
As previously highlighted, clarity and organization are paramount when dealing with complex algorithms. Java’s object-oriented principles lend themselves well to this need. For example, you can encapsulate different components of your machine learning workflow—data loading, preprocessing, model training, and evaluation—into distinct classes. This modular approach not only enhances readability but also makes it easier to test and debug individual parts of your application.
When building a machine learning model, one common challenge is to ensure that the data is correctly preprocessed. This often involves normalization, handling missing values, and transforming categorical data into numerical formats. Java’s strong typing and built-in data structures help manage these tasks effectively. For instance, consider the following code snippet that demonstrates data normalization:
public class DataNormalization { public static double[] normalize(double[] data) { double min = Double.MAX_VALUE; double max = Double.MIN_VALUE; for (double value : data) { if (value max) max = value; } double[] normalizedData = new double[data.length]; for (int i = 0; i < data.length; i++) { normalizedData[i] = (data[i] - min) / (max - min); } return normalizedData; } public static void main(String[] args) { double[] rawData = {10, 20, 30, 40, 50}; double[] normalized = normalize(rawData); for (double value : normalized) { System.out.println(value); } } }
This example illustrates a simpler normalization method, scaling the input data to a range of [0, 1]. Normalization is important for many machine learning algorithms as it helps improve convergence during training.
Once the data is preprocessed, the next step is to select the model to use. Depending on the nature of the problem—classification, regression, clustering, etc.—you may choose different algorithms. Java libraries like Weka or Deeplearning4j provide a plethora of options to suit various needs. The following snippet demonstrates how to implement a support vector machine (SVM) using Weka:
import weka.classifiers.Classifier; import weka.classifiers.functions.SMO; import weka.core.Instances; import weka.core.converters.ConverterUtils; public class SVMExample { public static void main(String[] args) throws Exception { // Load dataset ConverterUtils.DataSource source = new ConverterUtils.DataSource("path/to/your/dataset.arff"); Instances data = source.getDataSet(); data.setClassIndex(data.numAttributes() - 1); // Build SVM classifier Classifier classifier = new SMO(); classifier.buildClassifier(data); // Output model details System.out.println(classifier); } }
In this SVM example, the code loads a dataset and builds an SVM classifier using the SMO (Sequential Minimal Optimization) algorithm. This highlights Java’s capability to handle complex models with minimal setup, further emphasizing its strength in machine learning development.
Furthermore, once the model is trained, evaluating its performance is critical. Java provides facilities to calculate various metrics such as accuracy, precision, recall, and F1 score, which are essential for gauging the effectiveness of the model. Here’s a simple approach to evaluate a classifier’s performance:
import weka.classifiers.Classifier; import weka.classifiers.Evaluation; import weka.core.Instances; import weka.core.converters.ConverterUtils; public class EvaluationExample { public static void main(String[] args) throws Exception { // Load dataset ConverterUtils.DataSource source = new ConverterUtils.DataSource("path/to/your/dataset.arff"); Instances data = source.getDataSet(); data.setClassIndex(data.numAttributes() - 1); // Build classifier Classifier classifier = new SMO(); classifier.buildClassifier(data); // Evaluate classifier Evaluation eval = new Evaluation(data); eval.crossValidateModel(classifier, data, 10, new java.util.Random(1)); // Output evaluation metrics System.out.println("Accuracy: " + eval.pctCorrect()); System.out.println("Precision: " + eval.precision(1)); System.out.println("Recall: " + eval.recall(1)); } }
This snippet demonstrates how to perform cross-validation and output various performance metrics, providing a comprehensive view of the model’s capabilities. Evaluating and refining models is an iterative process that often requires returning to earlier stages, tweaking parameters, or even selecting different algorithms altogether.
Building machine learning models in Java involves a systematic approach of data preparation, model selection, training, and evaluation. The language’s capabilities, coupled with its extensive libraries, provide developers the tools to create effective and robust machine learning applications. With consistent practice and an understanding of the underlying algorithms, Java can be a powerful ally in the quest to develop intelligent systems.
Data Processing and Preparation Techniques
Data processing and preparation are crucial steps in the machine learning pipeline, as the quality of data significantly influences the performance of models. In Java, there are various techniques and tools that can be utilized to ensure that the data is clean, well-structured, and ready for analysis. The importance of preprocessing cannot be overstated; raw data is often messy and unstructured, necessitating thorough cleaning and transformation.
One common preprocessing task is handling missing values. Incomplete datasets can lead to biased models or unexpected errors; thus, it’s essential to decide how to address this issue. Java developers can implement various strategies, including imputation (filling in missing values) or removal of data instances with missing values. Here’s a simple example demonstrating how to handle missing values in a dataset:
import java.util.ArrayList; import java.util.List; public class MissingValueHandler { public static List handleMissingValues(List data) { List cleanedData = new ArrayList(); for (Double value : data) { if (value != null) { cleanedData.add(value); } else { // Here we use the mean of the dataset as the imputed value double mean = cleanedData.stream().mapToDouble(Double::doubleValue).average().orElse(0.0); cleanedData.add(mean); } } return cleanedData; } public static void main(String[] args) { List dataWithMissingValues = new ArrayList(); dataWithMissingValues.add(1.0); dataWithMissingValues.add(null); dataWithMissingValues.add(3.0); dataWithMissingValues.add(4.0); List cleanedData = handleMissingValues(dataWithMissingValues); System.out.println(cleanedData); } }
This example shows a simple way of replacing missing values with the mean of the available data points. While this is just one approach, it’s essential to understand the implications of such decisions, as they can affect the model’s accuracy.
Another vital aspect of data preparation is normalization or scaling. Different features in datasets can have varying ranges, which may skew the results of machine learning algorithms. Normalization is the process of adjusting the scales of features, making them comparable. Here’s how you might implement min-max normalization in Java:
public class MinMaxNormalization { public static double[] minMaxNormalize(double[] data) { double min = Double.MAX_VALUE; double max = Double.MIN_VALUE; for (double value : data) { if (value max) max = value; } double[] normalizedData = new double[data.length]; for (int i = 0; i < data.length; i++) { normalizedData[i] = (data[i] - min) / (max - min); } return normalizedData; } public static void main(String[] args) { double[] rawData = {10, 20, 30, 40, 50}; double[] normalized = minMaxNormalize(rawData); for (double value : normalized) { System.out.println(value); } } }
In the above example, each data point is adjusted to a range between 0 and 1, which helps in reducing the influence of outliers and improving the convergence speed of many algorithms.
Feature extraction is another crucial technique in the data preparation phase. It involves selecting a subset of relevant features from the data to enhance model accuracy and reduce computational costs. This can be done using techniques such as Principal Component Analysis (PCA) or feature importance measures provided by various machine learning models. Here’s a conceptual representation of how features might be selected based on their importance:
import java.util.HashMap; import java.util.Map; public class FeatureSelection { public static Map selectFeatures(Map featureImportances) { Map selectedFeatures = new HashMap(); for (Map.Entry entry : featureImportances.entrySet()) { if (entry.getValue() > 0.1) { // selecting features with importance greater than 0.1 selectedFeatures.put(entry.getKey(), entry.getValue()); } } return selectedFeatures; } public static void main(String[] args) { Map featureImportances = new HashMap(); featureImportances.put("Feature1", 0.05); featureImportances.put("Feature2", 0.15); featureImportances.put("Feature3", 0.20); Map selected = selectFeatures(featureImportances); System.out.println("Selected Features: " + selected); } }
This code snippet illustrates a simple method for filtering features based on their importance scores, which are typically computed during the training of a machine learning model.
Lastly, data partitioning is essential for ensuring that models can generalize well to unseen data. This typically involves splitting the dataset into training, validation, and test sets. Here’s a basic approach to partitioning data:
import java.util.ArrayList; import java.util.List; public class DataPartitioning { public static void main(String[] args) { List dataset = new ArrayList(); for (int i = 1; i <= 100; i++) { dataset.add(i); } List trainingSet = dataset.subList(0, 70); // 70% for training List validationSet = dataset.subList(70, 85); // 15% for validation List testSet = dataset.subList(85, 100); // 15% for testing System.out.println("Training Set: " + trainingSet); System.out.println("Validation Set: " + validationSet); System.out.println("Test Set: " + testSet); } }
This code demonstrates how to create subsets from a dataset to ensure that models can be trained and evaluated effectively. Each partition serves a distinct purpose in the machine learning workflow, contributing to the development of robust and accurate models.
Java provides a wide range of tools and techniques for data processing and preparation. By implementing these methods, developers can significantly enhance the quality of their datasets, leading to better-performing machine learning models. The flexibility and robustness of Java, combined with its rich ecosystem of libraries, empower data scientists to tackle preprocessing challenges effectively.
Integrating Java with Other Machine Learning Frameworks
Java’s ability to integrate with other machine learning frameworks opens up a world of possibilities for developers looking to leverage existing tools and libraries. By connecting Java with frameworks like TensorFlow, Apache Spark, and Deeplearning4j, developers can utilize advanced machine learning capabilities and benefit from the scalability and performance optimizations these frameworks offer.
One prominent example of integrating Java with TensorFlow is through the TensorFlow Java API. This API allows developers to define, train, and execute machine learning models directly within Java applications. The TensorFlow library is highly regarded for its flexibility in building complex neural networks, and its Java counterpart extends this functionality to Java developers. Here’s a brief example that demonstrates how to load a pre-trained model and make predictions:
import org.tensorflow.Graph; import org.tensorflow.Session; import org.tensorflow.Tensor; public class TensorFlowExample { public static void main(String[] args) { try (Graph graph = new Graph()) { // Load the model byte[] graphDef = ...; // Load your model's graph definition here graph.importGraphDef(graphDef); try (Session session = new Session(graph)) { // Create a tensor for input data Tensor inputTensor = Tensor.create(new float[][] {{1.0f, 2.0f}}); // Run the model Tensor outputTensor = session.runner() .fetch("output_node") .feed("input_node", inputTensor) .run() .get(0); // Process output float[][] output = new float[1][1]; outputTensor.copyTo(output); System.out.println("Prediction: " + output[0][0]); } } } }
In this example, we load a TensorFlow model, create an input tensor, and execute the model using a TensorFlow session. The output from the model can then be processed as needed, illustrating how Java can effectively utilize the power of TensorFlow for machine learning tasks.
Furthermore, Apache Spark’s MLlib provides another layer of integration for Java developers, especially when dealing with large datasets. By using Spark’s distributed computing capabilities, developers can perform machine learning at scale. The following example demonstrates how to use Spark’s MLlib for building a logistic regression model:
import org.apache.spark.api.java.JavaSparkContext; import org.apache.spark.api.java.JavaRDD; import org.apache.spark.sql.SparkSession; import org.apache.spark.ml.classification.LogisticRegression; import org.apache.spark.ml.linalg.Vectors; import org.apache.spark.sql.Dataset; import org.apache.spark.sql.Row; public class SparkMLExample { public static void main(String[] args) { SparkSession spark = SparkSession.builder().appName("Spark ML Example").getOrCreate(); JavaSparkContext jsc = new JavaSparkContext(spark.sparkContext()); // Create training data JavaRDD data = jsc.parallelize(Arrays.asList( RowFactory.create(Vectors.dense(0.0, 1.1, 0.1), 1.0), RowFactory.create(Vectors.dense(2.0, 1.0, -1.0), 0.0) // Add additional data points )); Dataset trainingData = spark.createDataFrame(data, schema); // Create and fit the logistic regression model LogisticRegression lr = new LogisticRegression(); LogisticRegressionModel model = lr.fit(trainingData); // Print the coefficients and intercept System.out.println("Coefficients: " + model.coefficients() + " Intercept: " + model.intercept()); } }
In this snippet, we create a Spark session and define a simple training dataset for a logistic regression model. Spark’s MLlib simplifies the machine learning workflow and allows for processing large datasets across distributed systems—an essential capability when working with big data.
Moreover, Deeplearning4j, a deep learning library for Java, supports integration with Hadoop and Spark, enabling deep learning in a scalable fashion. This allows Java applications to utilize distributed computing resources for training complex neural networks, further enhancing performance and efficiency. Here’s a basic example of defining a recurrent neural network (RNN) with Deeplearning4j:
import org.deeplearning4j.nn.conf.MultiLayerConfiguration; import org.deeplearning4j.nn.conf.NeuralNetConfiguration; import org.deeplearning4j.nn.multilayer.MultiLayerNetwork; import org.deeplearning4j.nn.conf.layers.LSTM; import org.nd4j.linalg.activations.Activation; import org.nd4j.linalg.learning.config.Adam; public class RNNExample { public static void main(String[] args) { MultiLayerConfiguration config = new NeuralNetConfiguration.Builder() .updater(new Adam(0.01)) .list() .layer(0, new LSTM.Builder().nIn(10).nOut(20).activation(Activation.TANH).build()) .layer(1, new LSTM.Builder().nIn(20).nOut(10).activation(Activation.TANH).build()) .build(); MultiLayerNetwork model = new MultiLayerNetwork(config); model.init(); System.out.println("Model configuration: " + model.getLayerWiseConfigurations()); } }
This example showcases the creation of a simple LSTM network, suitable for sequence prediction tasks. The integration capabilities of Deeplearning4j with Java enable developers to efficiently build and train deep learning models while using Java’s strong performance characteristics.
Ultimately, the ability to integrate Java with other machine learning frameworks allows developers to harness the strengths of various tools and libraries. By combining Java’s robust performance, object-oriented design, and extensive ecosystem with the capabilities of frameworks like TensorFlow, Apache Spark, and Deeplearning4j, developers can create powerful machine learning applications that can scale effectively and deliver exceptional results in real-world scenarios.
Real-World Applications of Java in Machine Learning
import java.util.ArrayList;
import java.util.List;
public class DataPartitioning {
public static void main(String[] args) {
List dataset = new ArrayList();
for (int i = 1; i <= 100; i++) {
dataset.add((double) i);
}
// Shuffle dataset
java.util.Collections.shuffle(dataset);
// Split dataset into training (70%) and test (30%)
int trainSize = (int) (dataset.size() * 0.7);
List trainingSet = dataset.subList(0, trainSize);
List testSet = dataset.subList(trainSize, dataset.size());
System.out.println(“Training Set: ” + trainingSet);
System.out.println(“Test Set: ” + testSet);
}
}
This snippet illustrates how to shuffle a dataset randomly and partition it into training and test sets based on a ratio. Proper partitioning is fundamental in avoiding overfitting and ensuring that the model can generalize to new data.
In summary, the data processing and preparation techniques in Java are robust and versatile. By employing these techniques, developers can significantly enhance the quality of the data, which in turn leads to the development of more accurate and reliable machine learning models. The systematic approach that Java offers in handling these tasks aligns well with the complexities of machine learning, providing a solid foundation for creating intelligent systems.
“`