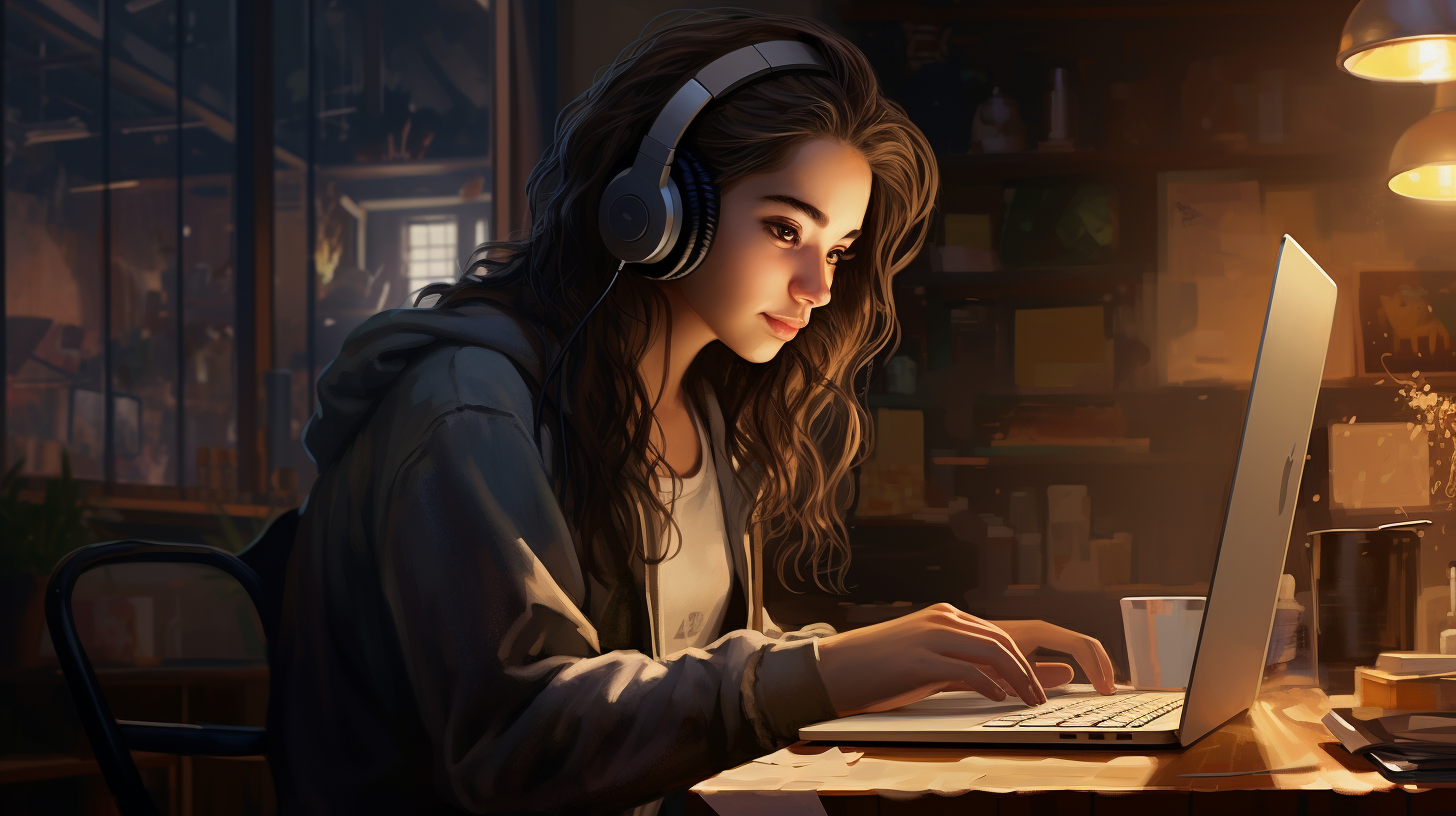
Java and Microbenchmarking: Performance Testing
Microbenchmarking in Java is a specialized technique aimed at measuring the performance of small units of code, often individual methods or functions, in isolation. Unlike traditional benchmarks that assess the performance of an entire application, microbenchmarks focus on granular operations, allowing developers to optimize code at a very detailed level. This level of scrutiny is critical when you are tuning performance-sensitive applications or libraries, where even minor improvements can lead to significant gains.
One of the essential aspects of microbenchmarking is the understanding of the Java Virtual Machine (JVM) and how it optimizes code at runtime. The JVM employs Just-In-Time (JIT) compilation, which translates Java bytecode into native machine code. This optimization can lead to misleading benchmark results if not handled correctly, as the JVM may optimize code paths differently over successive executions. To effectively measure performance, it is crucial to account for these optimizations.
When microbenchmarking, you typically want to avoid common pitfalls that can skew results. For example, the JVM may eliminate what it perceives as unused code or optimize away certain operations during repeated executions. To mitigate these issues, a typical practice is to include a warm-up phase in your benchmarks, allowing the JIT compiler to optimize the code before measuring performance.
Here is a basic example of how you might structure a microbenchmark in Java:
public class MicroBenchmark { private static final int ITERATIONS = 1000000; public static void main(String[] args) { // Warm up the JVM for (int i = 0; i < 10; i++) { runTest(); } // Now measure performance long startTime = System.nanoTime(); runTest(); long endTime = System.nanoTime(); System.out.println("Elapsed time: " + (endTime - startTime) + " ns"); } private static void runTest() { int result = 0; for (int i = 0; i < ITERATIONS; i++) { result += i; } // Prevent compiler optimization if (result == 0) { throw new RuntimeException("Should not be zero"); } } }
In this example, the runTest
method performs a simple computation that sums numbers from 0 to ITERATIONS
. The warm-up loop calls runTest
several times to allow the JVM to optimize the method before the actual timing begins. The result is printed in nanoseconds, providing a clear measurement of how long the test took to execute.
While this approach provides a simpler way to perform microbenchmarking, it is important to note that more sophisticated frameworks exist to handle many of the nuances and complexities associated with accurate performance measurement in Java. Using these frameworks can help ensure that your benchmarks are reliable and repeatable.
Key Tools and Frameworks for Java Microbenchmarking
When it comes to microbenchmarking in Java, using the right tools and frameworks can significantly improve the reliability of your performance measurements. While writing custom benchmarks, as demonstrated, is feasible, it often becomes cumbersome to handle all aspects of benchmarking accurately—especially with considerations such as JVM warm-up times, optimizations, and statistical reliability. Fortunately, there are several well-established libraries designed specifically for this purpose.
Java Microbenchmark Harness (JMH) is perhaps the most prominent framework for microbenchmarking in Java. Developed by the same team that works on the OpenJDK, JMH is designed to mitigate common pitfalls and offers a robust environment to create benchmarks. It takes care of the intricacies of JVM warm-up, ensures reliable measurement, and provides various modes of benchmarking (single-threaded, multi-threaded, etc.).
To get started with JMH, you first need to include it in your project. If you’re using Maven, add the following dependency:
org.openjdk.jmh jmh-core 1.34 org.openjdk.jmh jmh-generator-annprocess 1.34
Next, you can create a benchmark class. Below is an example showing how to measure the performance of a simple summation operation using JMH:
import org.openjdk.jmh.annotations.Benchmark; import org.openjdk.jmh.annotations.Warmup; import org.openjdk.jmh.annotations.Measurement; import org.openjdk.jmh.annotations.State; import org.openjdk.jmh.annotations.Scope; @State(Scope.Thread) public class BenchmarkExample { private static final int ITERATIONS = 1000000; @Benchmark @Warmup(iterations = 5) @Measurement(iterations = 10) public int sum() { int result = 0; for (int i = 0; i < ITERATIONS; i++) { result += i; } return result; } }
In this example, the @Benchmark
annotation marks the method to be measured. The @Warmup
annotation specifies how many warm-up iterations to run, while @Measurement
indicates how many measurement iterations to perform. JMH automatically handles the warm-up of the JVM, ensuring that the code is fully optimized before the actual measurements take place.
Another tool worth mentioning is Gatling, which is typically used for load testing but can also be adapted for microbenchmarking scenarios. It is designed for testing the performance of applications under load, offering capabilities to measure response times and throughput.
Additionally, Java’s Flight Recorder (JFR) can be a powerful tool for in-depth analysis of performance issues, including those revealed during microbenchmarking. JFR allows for profiling applications with minimal overhead, enabling developers to capture detailed metrics about performance bottlenecks during benchmark execution.
In conjunction with these frameworks, using Java’s built-in System.nanoTime()
method or the System.currentTimeMillis()
can still be beneficial for quick and dirty measurements where full-fledged frameworks may be overkill. However, for robust microbenchmarking, frameworks like JMH are highly recommended due to their comprehensive approach to handling the complexities of the Java runtime.
Best Practices for Accurate Performance Measurement
When it comes to ensuring accurate performance measurement in Java microbenchmarking, several best practices come into play. These practices help eliminate variability and enhance the reliability of your benchmark results. The microbenchmarking landscape is fraught with traps that can mislead developers, making adherence to these practices critical for meaningful performance analysis.
First and foremost, it’s essential to implement a warm-up phase effectively. The JVM, equipped with its JIT compiler, optimizes code at runtime. During initial executions, the code might not perform at its peak because the JIT has yet to apply its optimizations. Therefore, running a series of warm-up iterations allows the JVM to reach a stable state before you start measuring performance. A common approach is to execute the benchmark method multiple times before the actual measurement starts. This practice ensures that the JIT compiler has had sufficient opportunity to optimize the code paths.
Consider this enhanced version of the previous benchmark example, which includes a warm-up phase:
import org.openjdk.jmh.annotations.Benchmark; import org.openjdk.jmh.annotations.Warmup; import org.openjdk.jmh.annotations.Measurement; import org.openjdk.jmh.annotations.State; import org.openjdk.jmh.annotations.Scope; @State(Scope.Thread) public class BenchmarkExample { private static final int ITERATIONS = 1000000; @Benchmark @Warmup(iterations = 5) @Measurement(iterations = 10) public int sum() { int result = 0; for (int i = 0; i < ITERATIONS; i++) { result += i; } return result; } }
Another crucial factor is the isolation of the benchmarked code. External influences, such as garbage collection (GC) and other threads, can introduce noise into your measurements. To mitigate these effects, perform microbenchmarks in a controlled environment. You can run benchmarks in a dedicated thread or even using tools that provide thread isolation. This helps reduce the likelihood of interference from other processes, leading to more accurate results.
Additionally, it is essential to increase the number of repetitions in your measurement iterations. A single run of a benchmark might not capture the fluctuations inherent in the JVM’s execution. By running the benchmark multiple times and averaging the results, you can achieve a more reliable performance metric. You should also think using statistical analysis on the results to account for outliers, which can skew the average.
Moreover, always ensure that the data used in benchmarks is consistent. If the benchmark involves operations on external resources, such as I/O or databases, ensure that they are in a known and controlled state before measurement. Variability in input data can lead to inconsistent benchmark outcomes, undermining the validity of your results.
Lastly, avoid premature optimization. When assessing performance, focus on areas that are relevant to your application’s performance profile. Microbenchmarking can highlight inefficiencies, but it’s vital to ensure that the changes you make based on these benchmarks are justified by actual performance needs. Always measure before and after any optimizations to confirm that the changes have the desired effect.
By adhering to these best practices, you can significantly enhance the accuracy and reliability of your microbenchmarking efforts in Java. The goal is to create a stable environment where you can draw meaningful insights from performance measurements, ultimately guiding you to write more efficient and high-performing Java code.
Common Pitfalls in Java Microbenchmarking
In the sphere of Java microbenchmarking, several common pitfalls can easily undermine the validity of your performance measurements. Being aware of these issues especially important for anyone looking to achieve reliable results, as they can often lead to misleading conclusions about the performance of your code.
One of the most prevalent pitfalls is failing to account for the Just-In-Time (JIT) compilation process. As previously mentioned, the JVM optimizes code during runtime, and if your benchmark does not allow sufficient warm-up time, you may capture results that do not accurately reflect the optimized performance. This can lead to an underestimation of how well your code actually runs in a production environment. It’s essential to run a sufficient number of warm-up iterations before the actual measurement phase to let the JIT compiler do its job.
Another common error is neglecting to isolate the benchmarked code. When microbenchmarking, external factors like garbage collection (GC) activities or multithreading can introduce noise into the measurements. For instance, if a GC event occurs while your benchmark is running, it can cause significant delays that skew the results. To mitigate such issues, it’s advisable to isolate your benchmarking from other operations. Running benchmarks in a dedicated thread or using facilities provided by frameworks like JMH can help achieve a cleaner measurement environment.
Moreover, statistical validity is often overlooked. A single run of your benchmark may not capture the inherent variability in execution times due to various factors. It is a common best practice to run multiple iterations of your benchmark and compute averages or even use more sophisticated statistical techniques to analyze the results. This helps in understanding the distribution of the measurements and provides a clearer picture of performance characteristics.
In addition, one should be cautious of compiler optimizations that can elide code that appears redundant. The JVM may optimize away parts of your code if it determines those parts are not used. To prevent this, always ensure that your results are validated in a way that prevents the compiler from ignoring your computations. A common technique is to use an assertion or a dummy variable to require a non-zero result from your benchmarked method, as shown in the following example:
private static void runTest() { int result = 0; for (int i = 0; i < ITERATIONS; i++) { result += i; } // Prevent compiler optimization if (result == 0) { throw new RuntimeException("Should not be zero"); } }
Furthermore, a frequent misstep occurs when benchmarks inadvertently measure the wrong aspect of performance. For example, if you focus solely on execution speed without considering memory usage or other resources, you may miss out on critical insights into your application’s performance. It’s vital to determine what specific attributes of performance are most relevant to your application and to design benchmarks that reflect those needs.
Lastly, beware of the temptation to make premature optimizations based solely on microbenchmark results. Microbenchmarking is a powerful tool, but it should not be the sole determinant of performance improvements. Instead, use it as one of many indicators when assessing where optimizations are genuinely warranted. Ultimately, the goal is to refine your code in a manner that balances performance with maintainability and readability.
Identifying and understanding these common pitfalls will exponentially increase the accuracy and reliability of your Java microbenchmarking efforts. By approaching your performance testing with a careful and informed mindset, you’re much more likely to derive meaningful insights that lead to tangible improvements in your Java applications.
Interpreting and Analyzing Benchmark Results
Interpreting benchmark results in Java requires a careful analysis of the output, as it informs decisions that can significantly influence the performance of your application. The first step is to look at the raw numbers produced by your benchmark framework and understand what they signify. In typical benchmarking outputs, you will often encounter metrics such as mean execution time, standard deviation, and percentile values. These metrics provide different perspectives on the performance of your code.
For example, the mean execution time gives you a general idea of how long a benchmarked operation takes on average. However, relying solely on the mean can be misleading, especially if you have outliers that could skew the result. Hence, examining the standard deviation is vital, as it indicates the variability of your benchmark results. A small standard deviation suggests that your code’s performance is consistent, while a large standard deviation may signal that other factors are affecting execution time.
Percentiles also provide more granular insights into execution time distribution. For instance, a 90th percentile value indicates that 90% of your operations completed in that time or less. That is particularly useful for understanding the upper bounds of performance and can help you identify worst-case scenarios. A high 90th percentile time, compared to the mean, might suggest that while most executions are fast, occasional spikes in execution time occur, potentially due to GC pauses or other influences.
When using a framework like JMH, interpreting the results can be simpler as it provides a detailed report that breaks down these statistics. For instance, consider the output from a JMH benchmark:
Benchmark Mode Cnt Score Error Units BenchmarkExample.sum avgt 10 1234.567 ± 12.345 ns/op
In this result, “Score” represents the average time taken per operation (in nanoseconds), and “Error” specifies the margin of error for that measurement. The mode indicates the type of measurement being performed; in this case, “avgt” indicates that we are looking at the average time. With this information, you can assess whether the performance of your method meets the required criteria for your application.
Another critical aspect of interpreting benchmark results is to compare them against performance expectations or requirements. Establishing baseline performance metrics is key. Once you have a baseline, you can determine whether subsequent changes to the code have improved or degraded performance. This comparative analysis helps you make informed decisions about optimizations.
Furthermore, context is paramount when analyzing benchmark results. What might be a good performance metric for one application could be inadequate for another. For instance, a microbenchmark that takes 1 millisecond in isolation may be reasonable, but if it gets called in a tight loop millions of times, the cumulative effect can lead to performance bottlenecks. Always ponder the broader application context when analyzing the impact of any benchmarked code.
Lastly, document your benchmark results meticulously. Keeping a record of benchmarks, including the configuration, environment, and JVM flags used during testing, can prove invaluable when revisiting performance assessments in the future. This documentation allows you to track performance trends over time and helps in replicating results if needed.
Interpreting and analyzing Java microbenchmark results involves a multifaceted approach, incorporating statistical analysis, context, and historical data. By digging deeper into these metrics and understanding their implications, you can make better optimization decisions that significantly enhance the performance of your Java applications.