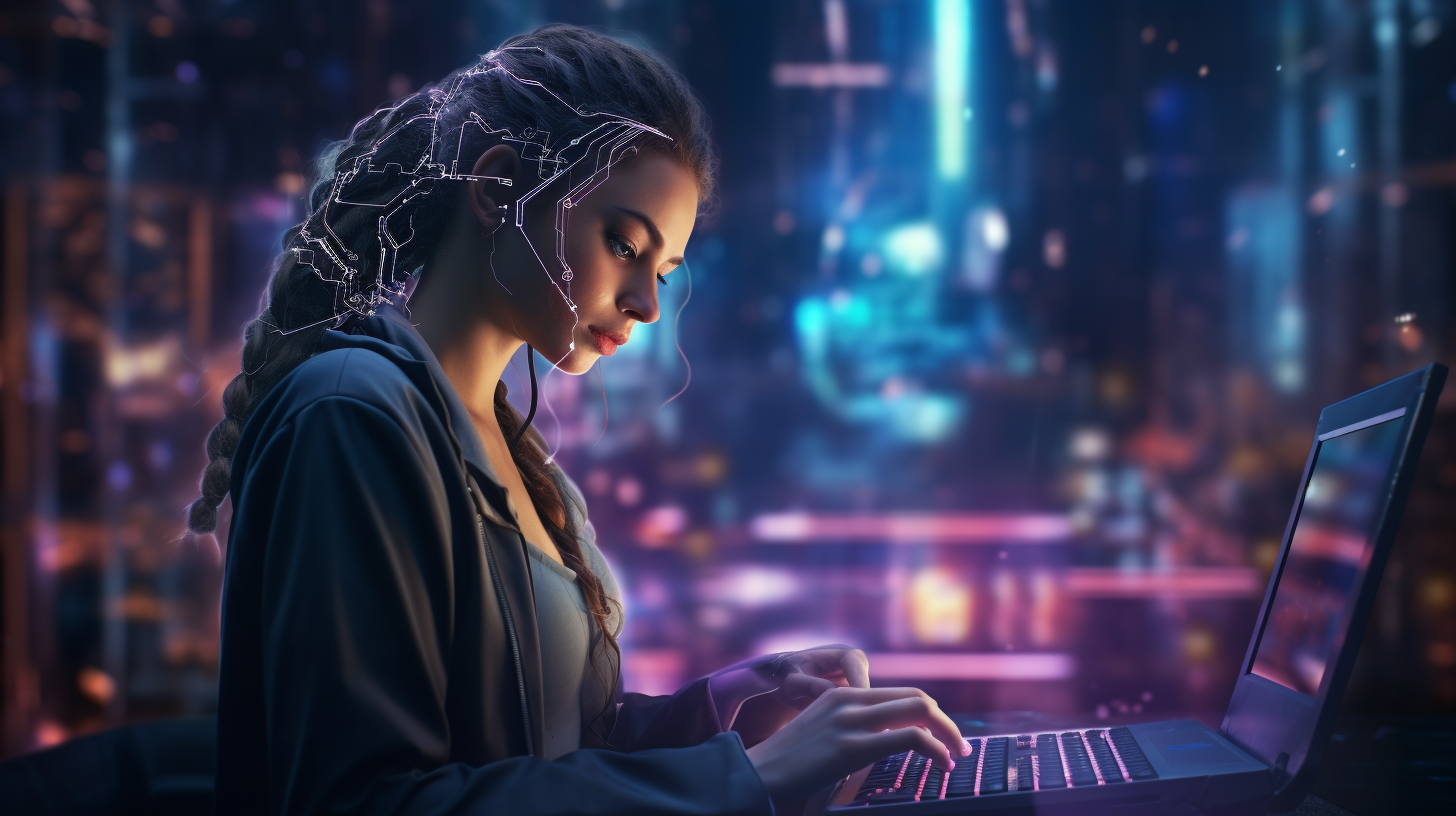
Java and Reactive Programming: An Introduction
Reactive programming is a paradigm that emphasizes the flow of data and the propagation of changes. It allows developers to construct applications that are more resilient to changes and failures, primarily by embracing asynchronous data streams. At its core, reactive programming allows you to manage the complexity of asynchronous programming in a more intuitive manner.
One of the fundamental principles of reactive programming is the asynchronous data stream. In contrast to traditional programming models where data flows in a synchronous manner (blocking the execution until a response is received), reactive programming allows operations to occur independently of each other. This means that a program can continue executing while waiting for data to arrive, significantly improving performance and responsiveness.
Another key concept is non-blocking I/O. Non-blocking I/O operations let the application handle multiple operations concurrently without getting bogged down by waiting for individual operations to complete. In Java, this can be achieved using constructs like CompletableFuture
or libraries that support reactive streams.
Additionally, reactive programming focuses on the Observer pattern, where objects (observers) can subscribe to changes in other objects (the subject). When the state of the subject changes, all subscribed observers are notified automatically, thus providing a mechanism to react to changes dynamically.
Let’s explore a simple example that illustrates how to create a reactive stream using the Flux
class from the Project Reactor library:
import reactor.core.publisher.Flux; public class ReactiveExample { public static void main(String[] args) { Flux reactiveStream = Flux.just("Java", "Reactive", "Programming") .map(String::toUpperCase); reactiveStream.subscribe(System.out::println); } }
In this example, we create a Flux
from a simple list of strings. By using the map
method, we transform each element into uppercase. The subscribe
method then allows us to define how to react to the emitted items. When executed, this code prints:
JAVA REACTIVE PROGRAMMING
Through reactive programming, developers can achieve a higher level of abstraction in handling asynchronous tasks, leading to cleaner and more maintainable code. As applications become more data-driven and event-oriented, understanding these principles becomes essential for building robust and efficient systems.
Core Concepts of Reactive Streams
To fully grasp the core concepts of reactive streams, it’s essential to understand the key components that drive their operation. At the heart of the reactive streams API are four main interfaces: Publisher, Subscriber, Subscription, and Processor. These interfaces work together to facilitate the flow of data between producers and consumers in a reactive manner.
1. Publisher: The Publisher interface defines a source of data. It can emit zero or more items to its subscribers. Publishers are responsible for producing data and notifying subscribers about the availability of new data. The following code snippet demonstrates a simple custom Publisher:
import org.reactivestreams.Publisher; import org.reactivestreams.Subscriber; import org.reactivestreams.Subscription; public class SimplePublisher implements Publisher { private final String[] messages; public SimplePublisher(String[] messages) { this.messages = messages; } @Override public void subscribe(Subscriber subscriber) { subscriber.onSubscribe(new SimpleSubscription(subscriber, messages)); } private static class SimpleSubscription implements Subscription { private final Subscriber subscriber; private final String[] messages; private int index = 0; public SimpleSubscription(Subscriber subscriber, String[] messages) { this.subscriber = subscriber; this.messages = messages; } @Override public void request(long n) { for (int i = 0; i < n && index = messages.length) { subscriber.onComplete(); } } @Override public void cancel() { // Logic to cancel the subscription can be added here } } }
2. Subscriber: The Subscriber interface represents the entities that receive data from a Publisher. Subscribers can react to the emitted data through three core methods: onNext, onError, and onComplete. The following code snippet shows an implementation of a Subscriber that processes data:
import org.reactivestreams.Subscriber; import org.reactivestreams.Subscription; public class SimpleSubscriber implements Subscriber { @Override public void onSubscribe(Subscription subscription) { subscription.request(Long.MAX_VALUE); // Requesting unlimited items } @Override public void onNext(String item) { System.out.println("Received: " + item); } @Override public void onError(Throwable throwable) { System.err.println("Error occurred: " + throwable.getMessage()); } @Override public void onComplete() { System.out.println("All items received."); } }
3. Subscription: This interface represents the link between a Publisher and a Subscriber. It allows the Subscriber to request more data and manage the flow of data emitted by the Publisher. The request method signals how many items the Subscriber is ready to handle. This backpressure mechanism especially important for maintaining control over data flow and preventing overwhelming the Subscriber.
4. Processor: The Processor interface acts as both a Subscriber and a Publisher. It enables transformation, filtering, or other processing of the data before it reaches the final Subscriber. By implementing the Processor interface, developers can create complex pipelines that manipulate the data as it flows through the system. An example implementation of a Processor is shown below:
import org.reactivestreams.Publisher; import org.reactivestreams.Subscriber; import org.reactivestreams.Processor; import org.reactivestreams.Subscription; public class SimpleProcessor implements Processor { private Subscriber subscriber; @Override public void subscribe(Subscriber subscriber) { this.subscriber = subscriber; } @Override public void onSubscribe(Subscription subscription) { subscriber.onSubscribe(subscription); } @Override public void onNext(String item) { // Transform the data before passing it along subscriber.onNext(item.toLowerCase()); } @Override public void onError(Throwable throwable) { subscriber.onError(throwable); } @Override public void onComplete() { subscriber.onComplete(); } }
These core concepts and interfaces form the backbone of reactive streams in Java. They enable the development of responsive, non-blocking applications by providing a structured way to handle asynchronous data flows. Understanding and effectively using these components will empower you to craft reactive applications that make optimal use of resources and deliver an enhanced user experience.
Integrating Reactive Programming with Java
To effectively integrate reactive programming with Java, you can leverage libraries that provide reactive capabilities, such as Project Reactor and RxJava. These libraries enhance the standard Java concurrency model by offering abstractions for asynchronous and event-driven programming.
Project Reactor, which is built on the Reactive Streams specification, provides two primary types: Flux and Mono. A Flux represents a stream of 0 to N elements, while a Mono represents a stream of 0 to 1 element. This distinction allows developers to handle a range of scenarios, from processing multiple items to returning single results.
For instance, creating a simple reactive pipeline using Mono can look like this:
import reactor.core.publisher.Mono; public class MonoExample { public static void main(String[] args) { Mono helloMono = Mono.just("Hello, Reactive World!"); helloMono.subscribe(System.out::println); } }
In this example, we create a Mono instance that emits a single value. Upon subscription, it prints the message to the console. This demonstrates how easy it is to work with reactive types in Java, encapsulating asynchronous operations within a fluent API.
Next, let’s delve into error handling, which very important for building resilient applications. Reactive programming allows for elegant error management through operators like onErrorReturn or onErrorResume. Here’s an example that showcases error handling in a Flux:
import reactor.core.publisher.Flux; public class ErrorHandlingExample { public static void main(String[] args) { Flux flux = Flux.just("One", "Two", "Three") .concatWith(Flux.error(new RuntimeException("Error occurred!"))) .onErrorReturn("Fallback Value"); flux.subscribe(System.out::println, error -> System.out.println("Error: " + error.getMessage()), () -> System.out.println("Completed!")); } }
In this example, the onErrorReturn operator provides a fallback value if an error occurs within the stream. This keeps the application running smoothly, allowing for a seamless user experience even when issues arise.
Moreover, integrating reactive programming with existing Java applications can be done alongside traditional synchronous processing, allowing for a gradual evolution towards a more reactive architecture. You can wrap blocking calls in CompletableFuture, enabling a non-blocking style while interacting with existing code.
Here’s a small example to illustrate this:
import java.util.concurrent.CompletableFuture; public class CompletableFutureExample { public static void main(String[] args) { CompletableFuture.supplyAsync(() -> { // Simulating a blocking operation try { Thread.sleep(1000); } catch (InterruptedException e) { Thread.currentThread().interrupt(); } return "Result from blocking call"; }).thenAccept(System.out::println); } }
This code snippet demonstrates how a blocking operation can be executed asynchronously using CompletableFuture. The supplyAsync method executes the computation in a separate thread, allowing the main thread to remain responsive.
By embedding reactive programming into your Java applications, you enhance their ability to handle asynchronous events and improve overall responsiveness. As you become more familiar with these libraries and patterns, you will find that they offer a powerful toolkit for developing modern, scalable applications that deal efficiently with the complexity of concurrency and asynchronous data flow.
Building Reactive Applications Using Spring WebFlux
Building reactive applications using Spring WebFlux offers a powerful way to handle web interactions in a non-blocking manner. WebFlux is part of the Spring Framework and is designed to work seamlessly with reactive programming paradigms. By using WebFlux, developers can create high-performance applications capable of handling a large number of concurrent users with minimal resource consumption.
To get started with Spring WebFlux, ensure that you have the appropriate dependencies in your Maven or Gradle project. For a Maven project, you would typically include the following dependency:
org.springframework.boot spring-boot-starter-webflux
Once you have the dependency set up, you can create a simple reactive REST controller. Here’s how you can define a controller that returns a reactive stream of data:
import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; import reactor.core.publisher.Flux; @RestController public class ReactiveController { @GetMapping("/messages") public Flux getMessages() { return Flux.just("Hello", "World", "from", "Spring", "WebFlux"); } }
In this snippet, we define a REST controller with a single endpoint, `/messages`, which returns a Flux of strings. When a client sends a GET request to this endpoint, Spring WebFlux handles the request asynchronously and returns the data as a streaming response.
To run this application, you would typically set up your Spring Boot application class like this:
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class ReactiveApplication { public static void main(String[] args) { SpringApplication.run(ReactiveApplication.class, args); } }
Now, when you access the `/messages` endpoint, you should see the individual messages being sent one at a time in a reactive manner. This allows your application to remain responsive during data processing and transmission.
Spring WebFlux also provides excellent support for reactive data access through Spring Data, which allows you to interact with databases in a non-blocking way. For example, if you’re using MongoDB, you can use the reactive repositories provided by Spring Data MongoDB:
import org.springframework.data.mongodb.repository.ReactiveMongoRepository; public interface UserRepository extends ReactiveMongoRepository { }
This interface definition allows you to perform CRUD operations on the `User` entity without blocking. The repository will return Flux or Mono types, making it simple to integrate with the rest of your reactive application.
Moreover, when dealing with error handling in WebFlux, you can utilize reactive operators and filters to manage exceptions gracefully. For instance, you could implement an error handling mechanism for your controller like this:
@GetMapping("/safe-messages") public Flux getSafeMessages() { return Flux.just("Hello", "World") .concatWith(Flux.error(new RuntimeException("An error occurred!"))) .onErrorReturn("Fallback Message"); }
This method illustrates how you can manage errors within a reactive stream, providing a fallback message in case of an error during processing. This approach enhances the resilience of your application and ensures a better user experience.
By taking advantage of Spring WebFlux, developers can build reactive applications that are capable of handling the increasing demands of modern software. The framework’s ability to process requests asynchronously and efficiently opens the door to developing applications that can scale seamlessly while remaining responsive to user interactions.
Testing and Debugging Reactive Java Applications
Testing and debugging reactive Java applications presents unique challenges compared to traditional synchronous applications. The nature of asynchronous data streams means that operations may not complete in the sequence expected, introducing potential race conditions, timing issues, and difficulties in tracing execution flow. However, with the right tools and techniques, developers can effectively test and debug their reactive applications.
One of the first steps in testing reactive applications is to ensure that your reactive streams produce the expected results. Libraries such as Project Reactor provide testing utilities that allow you to write assertions against the emitted items in a Flux or Mono. For example, you can use the StepVerifier class to validate the output of your reactive streams. Here’s a simple example:
import org.junit.jupiter.api.Test; import reactor.core.publisher.Flux; import reactor.test.StepVerifier; public class ReactiveStreamTest { @Test public void testFluxEmissions() { Flux flux = Flux.just("Java", "Reactive", "Programming"); StepVerifier.create(flux) .expectNext("Java") .expectNext("Reactive") .expectNext("Programming") .verifyComplete(); } }
In the above example, the StepVerifier is used to create a test for a Flux that emits three strings. The expectNext methods assert that the emitted values are as expected, and verifyComplete checks that the stream has completed without errors. This provides a clear and concise way to validate the behavior of reactive streams.
Error handling is another critical aspect of testing reactive applications. You should ensure that your applications can gracefully handle errors and that appropriate fallback values or mechanisms are in place. The StepVerifier also allows you to test error scenarios effectively:
import reactor.core.publisher.Flux; import reactor.test.StepVerifier; public class ErrorHandlingTest { @Test public void testErrorHandling() { Flux flux = Flux.just("One", "Two") .concatWith(Flux.error(new RuntimeException("Error occurred!"))) .onErrorReturn("Fallback Value"); StepVerifier.create(flux) .expectNext("One") .expectNext("Two") .expectNext("Fallback Value") .verifyComplete(); } }
In this example, we simulate an error within the Flux stream and utilize onErrorReturn to provide a fallback value. The StepVerifier checks that the fallback value is emitted as expected after the error, ensuring that the application behaves correctly in the presence of issues.
Debugging reactive applications can also be enhanced by using logging and debugging tools. By using operators such as doOnNext, doOnError, and doOnComplete, you can insert logging statements into your reactive pipeline, which will help you trace the execution flow and understand how data is transformed as it moves through the stream:
import reactor.core.publisher.Flux; public class DebuggingExample { public static void main(String[] args) { Flux.just("One", "Two", "Three") .doOnNext(item -> System.out.println("Processing: " + item)) .concatWith(Flux.error(new RuntimeException("Oops!"))) .doOnError(error -> System.out.println("Error: " + error.getMessage())) .subscribe(); } }
This code snippet illustrates how you can track the items being processed and log any errors encountered in the stream. By strategically placing these logging operators, you can gain insights into how your streams are executing, aiding in diagnosing issues.
Furthermore, tools such as the Reactor Debug Agent can be invaluable for debugging reactive applications. By enabling debug mode, you can obtain more detailed stack traces and insights into the execution context of your reactive chains, simplifying the identification of issues that arise from complex data flows.
As your applications grow in complexity, consider using integration tests to validate the behavior of multiple components working together in a reactive manner. Integration tests can help ensure that your entire application functions correctly under real-world conditions, taking into account the asynchronous nature of your streams.
By combining the strategies discussed above, you can establish a robust testing and debugging framework for your reactive Java applications. This ensures that your applications remain reliable, maintainable, and responsive, even as they evolve to meet changing requirements.