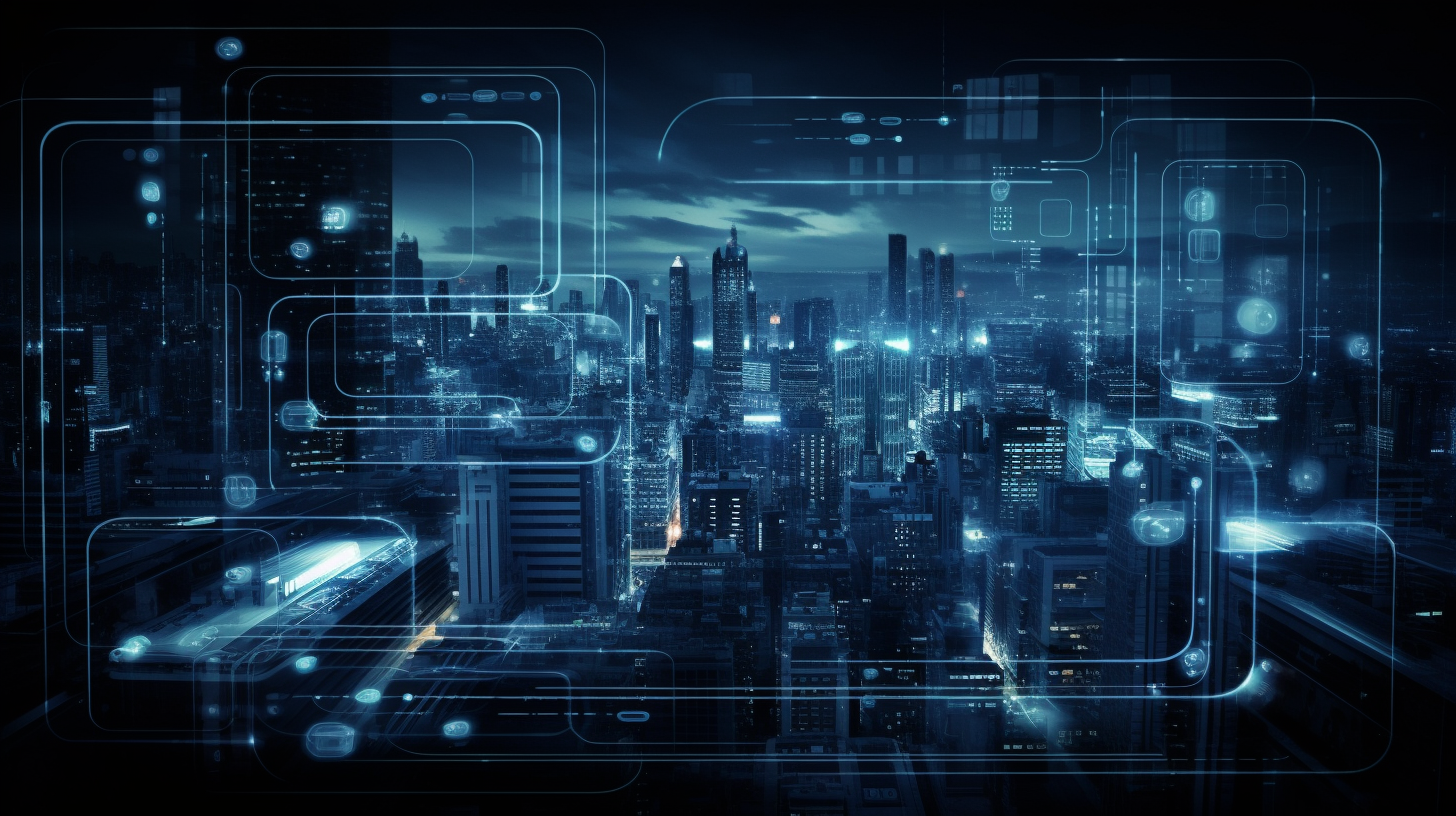
Java and Version Control: Git and GitHub
In the context of software development, version control systems (VCS) are indispensable tools that keep our projects organized and manageable. They allow developers to track changes in code, collaborate with others, and maintain a history of project evolution. By understanding the fundamental principles behind VCS, Java developers can harness these tools to streamline their workflows and enhance collaboration.
Version control systems can be categorized into centralized and distributed systems. Centralized version control systems (CVCS), like Subversion (SVN), store code in a single central repository. Developers check out files from the repository, make changes, and then check them back in. This model simplifies access management but introduces bottlenecks; if the central server fails or is offline, development grinds to a halt.
On the other hand, distributed version control systems (DVCS), such as Git, take a different approach. Each developer clones the entire repository, including its history, onto their local machine. This allows them to work offline and commit changes locally before synchronizing with a shared remote repository. This flexibility enhances productivity and minimizes the impact of network outages.
Git stands out within the scope of DVCS due to its speed, efficiency, and powerful branching and merging capabilities. These features enable developers to experiment with new ideas without jeopardizing the stability of the main codebase. Furthermore, Git’s ability to manage complex projects with multiple contributors makes it an essential tool for any Java developer working in collaborative environments.
Additionally, version control systems offer robust tracking capabilities. Every change made to the codebase is logged, allowing developers to easily identify who made changes, what those changes were, and when they occurred. This transparency is vital for maintaining code quality and accountability in collaborative projects.
To illustrate the power of version control, think a simple Java project where a developer implements a new feature. Without a VCS, tracking changes to the code can become cumbersome. However, with Git, the developer can create a branch for the new feature:
git checkout -b new-feature
Once the feature is complete, the developer can merge it back into the main branch:
git checkout main git merge new-feature
This branching and merging strategy ensures that the main codebase remains stable while allowing developers to work on features in isolation. It fosters a culture of experimentation and innovation, essential in today’s fast-paced development landscape.
Understanding version control systems very important for Java developers. It empowers them to manage their code effectively, collaborate seamlessly, and maintain a clear history of project changes. This foundation sets the stage for embracing more advanced tools like Git and GitHub, which enhance the development experience even further.
The Basics of Git
Git, the distributed version control system that has taken the software development world by storm, operates on a series of fundamental concepts that every developer should grasp. Central to Git’s functionality are the notions of commits, branches, and remotes. Understanding these components will enable Java developers to leverage Git’s strengths effectively.
A commit in Git represents a snapshot of your code at a particular point in time. Ponder of it as a save point in a game: if something goes wrong, you can return to that snapshot and continue from there. Each commit has a unique identifier, typically a long string of alphanumeric characters, which provides a way to track changes over time. A well-structured commit message is essential; it acts as documentation for others (and your future self) to understand why a change was made.
git commit -m "Implemented feature X"
Branches in Git allow developers to diverge from the main line of development without affecting the stable version of the project. When you create a branch, you essentially make a copy of the project at that point in time. This is particularly useful for Java developers who want to experiment with new features or tackle bugs without interrupting the main workflow. The primary branch in a Git repository is usually referred to as “main” or “master.” To create a new branch for a feature, you would use the following command:
git checkout -b feature-branch
Once your feature is complete and tested, you can merge the branch back into the main branch, ensuring that your main codebase remains stable while incorporating your changes:
git checkout main git merge feature-branch
Remotes are another crucial aspect of Git. They represent versions of your project hosted on external servers, such as GitHub or GitLab. By setting up remotes, developers can push their changes to a shared repository, making their work accessible to other collaborators. Adding a remote repository is straightforward:
git remote add origin https://github.com/username/repo.git
Once set up, pushing your commits to the remote repository is simple:
git push origin main
The basics of Git revolve around understanding how to make commits, create branches, and interact with remote repositories. Mastery of these concepts will empower Java developers to take full advantage of Git’s capabilities, fostering a more organized and efficient workflow. As you delve deeper into Git, you’ll discover that these foundational elements form the backbone of a powerful version control strategy, essential for modern software development.
Setting Up a Git Repository
Setting up a Git repository is an important first step in using the full potential of Git for your Java projects. Whether you’re starting a new project or integrating Git into an existing one, the setup process is simpler but essential for effective version control.
To begin, you need to have Git installed on your machine. You can verify the installation by running the following command in your terminal:
git --version
This should return the version of Git you have installed. If it doesn’t, you can download and install Git from git-scm.com.
Once Git is installed, you can set up your local repository. Navigate to your Java project directory using the terminal or command prompt:
cd path/to/your/java/project
With the terminal positioned in your project directory, you can initialize a new Git repository by executing:
git init
This command creates a new subdirectory called .git that contains all the necessary files for your Git repository, effectively transforming your project directory into a version-controlled environment.
In a typical Java project, you have source files, configurations, and possibly libraries or dependencies that you might not want to track. To manage this, you should create a .gitignore file. This file tells Git which files or directories to ignore. For a Java project, your .gitignore might look like this:
# Ignore Maven build files target/ # Ignore IntelliJ IDEA files .idea/ *.iml # Ignore Eclipse project files .classpath .project # Ignore other build files *.class *.jar *.war
After creating your .gitignore file, you can add your project files to the staging area using:
git add .
This command stages all your files, preparing them for the first commit. It’s a good practice to review what you’re staging by running:
git status
This will show you the current status of your files, including which are staged for commit and which are not.
Once you’ve staged your files, it’s time to make your first commit. This captures your current project state in the Git history:
git commit -m "Initial commit"
The commit message should be descriptive enough to convey the changes made. For the initial commit, a simple message like “Initial commit” suffices.
If you are collaborating with others or plan to back up your repository remotely, you’ll want to link your local repository to a remote one on GitHub. First, create a new repository on GitHub. Once that’s done, connect your local repository to the remote:
git remote add origin https://github.com/username/repo.git
Now, you can push your local commits to the GitHub repository:
git push -u origin main
This command pushes your changes and sets the upstream branch for your local main branch, allowing you to use simpler push commands in the future.
By setting up your Git repository effectively, you establish a solid foundation for version control in your Java projects. This setup not only helps manage changes but also enhances collaboration with other developers, making it an essential skill for modern software development.
Essential Git Commands for Java Developers
The essential Git commands that Java developers should master form the backbone of effective version control and collaboration in their projects. By using these commands, developers can keep their code organized, track changes, and facilitate teamwork seamlessly.
First and foremost, understanding how to check the status of your repository very important. The command git status
provides a snapshot of your current working directory and staging area. It informs you about which files are tracked, which are staged for commit, and which have uncommitted changes:
git status
This command is often the first step before making any changes, as it helps you understand the state of your project.
Next, adding files to the staging area is integral to preparing for a commit. As mentioned earlier, git add
can stage changes. You can add specific files or directories, or use git add .
to stage everything:
git add # or to add all changes git add .
Once the files are staged, creating a commit captures your changes in history. The command git commit
effectively saves your work, and a well-crafted message is key to providing context for the changes made:
git commit -m "Refactor user authentication logic"
To view the commit history, use git log
. This command lists all commits made to the repository, along with their unique identifiers and messages. It’s an excellent way to track the evolution of your project:
git log
As developers work collaboratively, merging changes becomes essential. If you want to combine the changes from one branch into another, you’ll need to switch to the target branch and use:
git checkout main git merge feature-branch
This command integrates the changes from feature-branch
into the main
branch, which will allow you to keep the main codebase up to date with the latest features or fixes.
In scenarios where you need to share your work with others, pushing your changes to a remote repository is vital. The command git push
uploads your local commits to a specified branch on the remote repository:
git push origin main
Conversely, to bring changes from the remote repository into your local repository, you would use git pull
. This command fetches and merges changes from the remote into your current branch:
git pull origin main
Another essential command is git branch
, which allows developers to create, list, and delete branches. That’s particularly useful for managing different features and fixes in a Java project. To create a new branch, you would run:
git branch new-feature
To switch to that branch, use git checkout
:
git checkout new-feature
Alternatively, you can combine both actions with:
git checkout -b new-feature
As you navigate through your Git workflow, these commands will become second nature. They form the core of interaction with Git, enabling Java developers to manage their code effectively while collaborating with others. Mastering these essential Git commands will not only streamline your coding process but will also enhance your ability to contribute to and maintain robust Java applications.
Collaborating with GitHub
Collaborating with GitHub takes the benefits of Git and amplifies them by providing an online platform for sharing and managing code. GitHub is not just a repository hosting service; it is a community where developers can share their projects, contribute to others’ work, and partake in discussions. For Java developers, mastering collaboration on GitHub is essential for successful teamwork and project management.
To begin collaborating on GitHub, you first need to create a repository on the platform. This can be done freely through the GitHub web interface. Once your repository is created, you can clone it to your local machine using the following command:
git clone https://github.com/username/repo.git
This command establishes a local copy of the repository, allowing you to work offline while still having access to the entire project history.
Once you’ve cloned the repository, you can start contributing. For effective collaboration, it’s essential to create branches for new features or bug fixes. This way, you can work independently without interfering with the main codebase. For example, to create a branch for a new feature, use:
git checkout -b feature-xyz
After making your changes and testing them thoroughly, you can stage and commit these changes:
git add . git commit -m "Add feature XYZ that improves user experience"
With your changes committed, it’s time to push your branch to the remote repository on GitHub:
git push origin feature-xyz
This command uploads your local branch to GitHub, making your changes accessible to other developers. Once pushed, you can open a Pull Request (PR) on GitHub. A PR is a request to merge your changes into another branch, commonly the main branch. It serves as a discussion thread where team members can review code, comment on changes, and suggest improvements.
When creating a Pull Request, it’s vital to provide a clear and simple description of the changes made. This documentation helps reviewers understand the context and purpose behind your modifications. Once your team members have reviewed the Pull Request and any necessary adjustments have been made, you can merge it into the main branch, which integrates your feature into the project:
git checkout main git merge feature-xyz
As you collaborate, you may encounter conflicts, especially if multiple developers modify the same lines of code. Git helps you handle these situations by highlighting the differences. You will need to manually resolve conflicts before you can complete the merge. After resolving, don’t forget to stage and commit the resolved files:
git add . git commit -m "Resolve merge conflicts"
Once the merge is complete, and your changes are in the main branch, it’s best practice to delete your feature branch, both locally and on GitHub, to keep the repository clean:
git branch -d feature-xyz git push origin --delete feature-xyz
Collaborating on GitHub also opens the door for community engagement through issues and discussions. You can report bugs, suggest features, or engage in conversations with other developers. This interactivity fosters a collaborative environment, facilitating knowledge sharing and peer support.
Moreover, GitHub provides tools for Continuous Integration/Continuous Deployment (CI/CD), allowing automated testing and deployment of Java applications. Setting up these workflows ensures that your code is always in a deployable state and helps catch issues early, improving code quality and reliability.
By using GitHub for collaboration, Java developers can work effectively in teams, contribute to open source projects, and benefit from the collective knowledge of the developer community. Mastering the collaborative features of GitHub will not only enhance your individual skills but also significantly improve the overall productivity of your development team.
Best Practices for Using Git in Java Projects
When it comes to using Git in Java projects, adhering to best practices can greatly enhance your workflow, improve collaboration, and maintain a clean codebase. These practices are not just about keeping your repository organized; they contribute to a more efficient and effective development process.
One of the fundamental best practices is to commit often with meaningful messages. Each commit should represent a logical unit of work. This principle encourages you to break down your changes into smaller, manageable chunks, making it easier to track progress and roll back changes if necessary. A well-structured commit message explains what was changed and why, which is invaluable for future reference. For example:
git commit -m "Fix NullPointerException in UserService when fetching user details"
In addition to meaningful commits, maintaining a clean branch structure is vital. Use descriptive names for your branches that convey the purpose of the changes being made. For instance, naming a branch feature/user-authentication
is far more informative than simply using branch1
. When working on multiple features or fixes, regularly merge these branches back into the main branch to avoid diverging too far from the master codebase. This practice keeps your repository organized and minimizes the risk of conflicts later on.
Another best practice is to use .gitignore
files effectively. This file specifies intentionally untracked files that Git should ignore. For Java projects, you might want to exclude compiled class files, logs, and IDE-specific files. Here’s an example of a .gitignore
for a Java project:
# Ignore compiled class files *.class # Ignore jar files *.jar # Ignore log files *.log # Ignore IDE-specific files .idea/ *.iml target/
Regularly syncing with the remote repository is another crucial practice. After pushing your changes, pull updates frequently to keep your local repository in sync with your collaborators. This helps prevent merge conflicts and ensures that you’re always working with the latest version of the code. Use:
git pull origin main
When working in teams, think implementing pull requests (PRs) for code reviews. Before merging a feature branch into the main branch, create a PR, allowing team members to review and discuss the changes. This process not only improves code quality but also fosters collaboration and knowledge sharing among team members.
Finally, leverage tagging in Git to mark specific points in your project’s history. Tags can be incredibly useful for marking release points, making it easier to navigate through the different versions of your application. Use the following command to create a tag:
git tag -a v1.0 -m "Release version 1.0"
Following these best practices will help you maintain a clean and effective workflow in your Java projects. By committing regularly, using meaningful messages, maintaining a clean branch structure, using pull requests, and effectively using the .gitignore
file, you can significantly enhance your development experience and collaboration with your team.