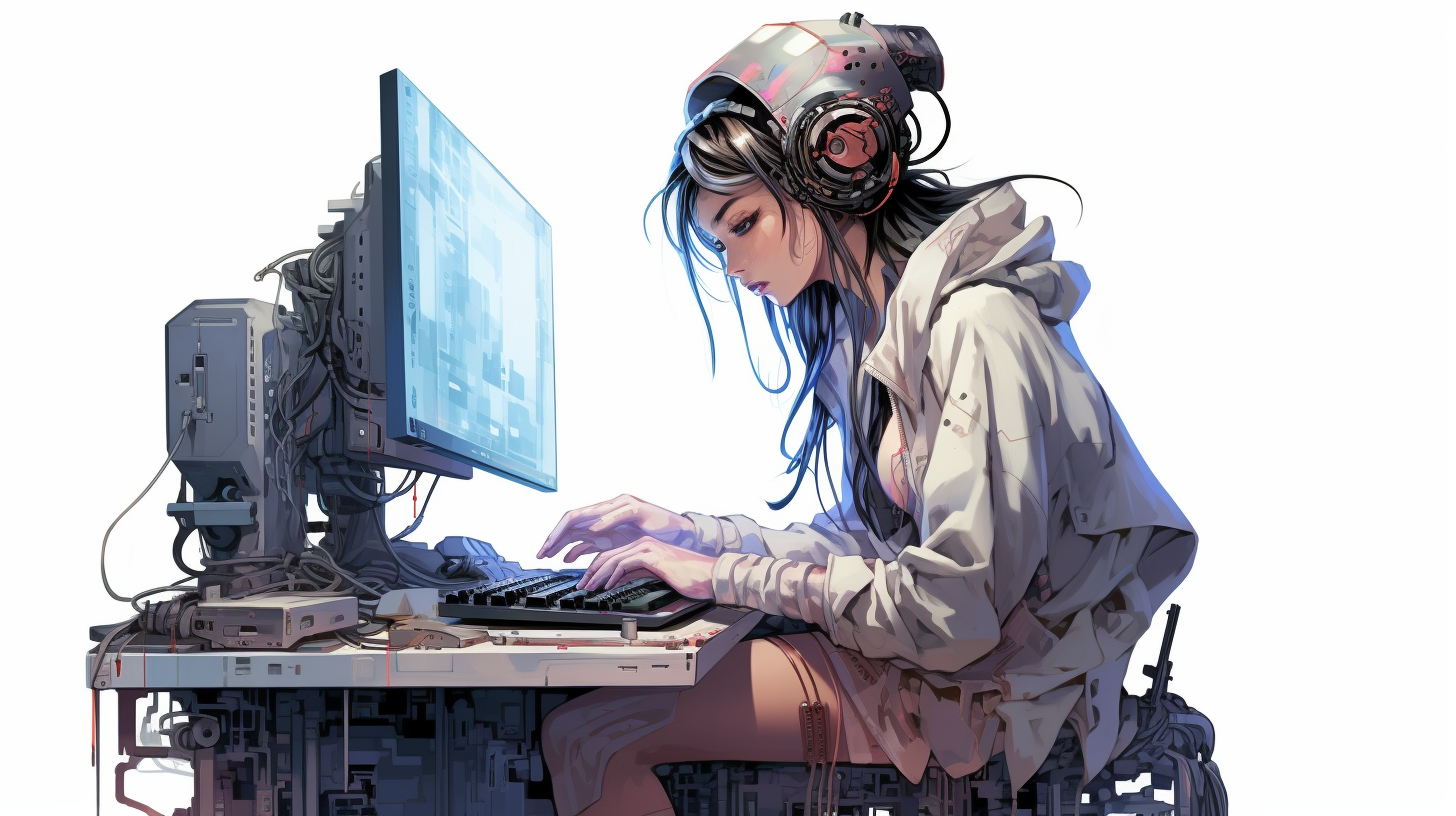
Java and Video Processing: Techniques and Tools
Video processing in Java can be efficiently accomplished through various frameworks that provide robust tools and APIs tailored for handling multimedia content. These frameworks streamline the complex tasks associated with video encoding, decoding, and manipulation, making it simpler for developers to focus on building applications rather than dealing with low-level video handling intricacies.
One of the most prominent frameworks available is JavaCV, which is a wrapper for the popular OpenCV library. It enables developers to harness powerful computer vision capabilities directly within their Java applications. By using native libraries, JavaCV provides an extensive set of functionalities, including face detection, object tracking, and image transformations.
import org.bytedeco.javacv.*; import org.bytedeco.javacv.FrameGrabber.Exception; public class VideoCaptureExample { public static void main(String[] args) { try (FrameGrabber grabber = OpenCVFrameGrabber.createDefault(0)) { grabber.start(); Frame frame; while ((frame = grabber.grab()) != null) { // Process the frame (e.g., display, analyze, etc.) // That is where you would add your video processing logic } grabber.stop(); } catch (Exception e) { e.printStackTrace(); } } }
Another noteworthy framework is JCodec, a pure Java implementation of video and audio codecs. Unlike JavaCV, which relies on native libraries, JCodec operates exclusively in Java, making it easier to deploy across diverse platforms without worrying about native dependencies. This framework excels in decoding video formats like H.264 and provides utilities for rendering video streams in a Java application.
import org.jcodec.api.awt.AWTSequenceEncoder8Bit; import java.awt.image.BufferedImage; import java.io.File; public class VideoEncodingExample { public static void main(String[] args) throws Exception { AWTSequenceEncoder8Bit encoder = AWTSequenceEncoder8Bit.createSequenceEncoder(new File("output.mp4")); for (int i = 0; i < 100; i++) { BufferedImage image = new BufferedImage(640, 480, BufferedImage.TYPE_INT_RGB); // Here you would draw or process the image encoder.encodeImage(image); } encoder.finish(); } }
Processing and playing video streams can also be accomplished using JavaFX. This framework provides a rich set of tools for building modern user interfaces and includes capabilities for media playback. With JavaFX, developers can smoothly integrate video functionalities within their applications, enhancing user experience.
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.layout.StackPane; import javafx.scene.media.Media; import javafx.scene.media.MediaPlayer; import javafx.scene.media.MediaView; import javafx.stage.Stage; public class VideoPlayerExample extends Application { @Override public void start(Stage primaryStage) { Media media = new Media("file:///path/to/video.mp4"); MediaPlayer mediaPlayer = new MediaPlayer(media); MediaView mediaView = new MediaView(mediaPlayer); StackPane root = new StackPane(); root.getChildren().add(mediaView); primaryStage.setScene(new Scene(root, 800, 600)); primaryStage.show(); mediaPlayer.play(); } public static void main(String[] args) { launch(args); } }
Each of these frameworks has its unique strengths and caters to different aspects of video processing. The right choice often depends on the specific requirements of the project, such as the need for native performance, ease of use, or advanced features. Understanding the capabilities of these frameworks will empower developers to build sophisticated video processing applications in Java.
Key Libraries for Video Manipulation
In addition to JavaCV, JCodec, and JavaFX, there are a number of other key libraries that can significantly enhance video manipulation in Java. FFmpeg, while not a Java library per se, offers a powerful set of tools for video processing and can be invoked from Java applications using JNI (Java Native Interface) or through wrappers. The FFmpeg library allows developers to handle nearly any video format, perform transcoding, and apply filters, making it an invaluable tool for comprehensive video processing tasks.
Another library worth mentioning is Xuggler, which provides a Java API for FFmpeg, allowing for easy access to its extensive capabilities. Xuggler can handle video encoding, decoding, and real-time streaming, making it particularly useful for applications that require high performance and flexibility. However, Xuggler development has slowed down, which could make it less appealing for new projects.
For those focused on 3D video processing and rendering, Java3D is an intriguing option. While primarily a 3D graphics API, it can be employed to process and display video textures in three-dimensional environments. This integration opens a realm of possibilities for creating immersive multimedia applications.
Think the following example that demonstrates how to use FFmpeg with Java to transcode a video file:
import java.io.IOException; public class FFmpegTranscoder { public static void main(String[] args) { String inputFile = "input.mp4"; String outputFile = "output.avi"; String command = String.format("ffmpeg -i %s -c:v libx264 -c:a aac %s", inputFile, outputFile); try { Process process = Runtime.getRuntime().exec(command); process.waitFor(); System.out.println("Transcoding completed."); } catch (IOException | InterruptedException e) { e.printStackTrace(); } } }
This snippet shows how to execute an FFmpeg command from a Java application. By encapsulating the command in a string and using the `Runtime.exec()` method, you gain access to the powerful transcoding capabilities of FFmpeg without directly handling its complexities.
When choosing libraries for video manipulation, it’s crucial to ponder not only the features they offer but also their community support and documentation. A well-supported library with an active user base can facilitate smoother development and troubleshooting, while extensive documentation can significantly reduce the learning curve associated with complex video processing tasks.
By using a combination of these libraries, Java developers can build applications that not only meet basic video processing needs but also push the boundaries of what is possible in multimedia handling, combining various libraries to leverage their unique strengths effectively.
Performance Optimization Techniques
Performance optimization is a critical aspect of video processing, especially in Java, where the inherent overhead of a virtual machine can impact the speed and efficiency of operations significantly. This section delves into various techniques that can enhance the performance of video processing applications built with Java.
1. Efficient Memory Management
Java’s garbage collection system can introduce latency, particularly in applications that process large amounts of video data. Therefore, managing memory allocation and deallocation efficiently is paramount. One effective strategy is to use memory pools for frequently used objects, such as frames or buffers. This approach reduces the pressure on the garbage collector and minimizes the overhead associated with object creation.
import java.awt.image.BufferedImage; import java.util.ArrayList; import java.util.List; public class FramePool { private final List pool = new ArrayList(); private final int poolSize; public FramePool(int poolSize) { this.poolSize = poolSize; for (int i = 0; i < poolSize; i++) { pool.add(new BufferedImage(640, 480, BufferedImage.TYPE_INT_RGB)); } } public BufferedImage getFrame() { if (pool.isEmpty()) { return new BufferedImage(640, 480, BufferedImage.TYPE_INT_RGB); } return pool.remove(pool.size() - 1); } public void releaseFrame(BufferedImage frame) { if (pool.size() < poolSize) { pool.add(frame); } } }
2. Multithreading
Using the power of multithreading can drastically improve performance. Video processing tasks like decoding, encoding, and applying effects can often be executed in parallel. Java’s ExecutorService
is ideal for managing threads efficiently. By dividing tasks into smaller chunks that can be processed simultaneously, you can make full use of the CPU’s capabilities and reduce the overall processing time.
import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; public class VideoProcessor { private final ExecutorService executor = Executors.newFixedThreadPool(Runtime.getRuntime().availableProcessors()); public void processFrames(List frames) { for (BufferedImage frame : frames) { executor.submit(() -> { // Process the frame (e.g., apply filters, encode, etc.) }); } executor.shutdown(); } }
3. Using Native Libraries
For tasks that require intensive computation, offloading some of the work to native libraries can yield significant performance improvements. Libraries like JavaCV and JCodec, which interface with optimized C/C++ implementations, can execute video processing tasks much faster than pure Java code. When using JNI (Java Native Interface), it is crucial to ensure that the native calls are minimized to avoid performance bottlenecks associated with context switching between Java and native code.
4. Efficient Encoding/Decoding
When working with video compression, selecting the right codec and configuring its parameters correctly can have a major effect on performance. For instance, using hardware acceleration available through libraries like JavaCV can expedite the encoding and decoding processes. In addition, adjusting the bitrate, resolution, and keyframe interval can help optimize the performance based on the specific requirements of the application.
import org.bytedeco.javacv.*; public class HardwareAcceleratedEncoding { public static void main(String[] args) { // Set up encoding parameters for accelerated encoding FFmpegFrameRecorder recorder = new FFmpegFrameRecorder("output.mp4", 640, 480); recorder.setVideoCodec(avcodec.AV_CODEC_ID_H264); recorder.setFormat("mp4"); recorder.setFrameRate(30); recorder.setVideoOption("preset", "ultrafast"); // Use hardware acceleration options if available // Start the recorder and encode frames... } }
5. Profiling and Benchmarking
Lastly, a fundamental aspect of optimization is to continuously profile and benchmark the application. Tools like VisualVM and YourKit can help identify bottlenecks in your code. Understanding where the performance lags occur allows for targeted optimizations rather than guesswork. It’s important to measure the performance impact of any changes made enabling you to assess their effectiveness quantitatively.
By employing these techniques, Java developers can significantly enhance the performance of their video processing applications, ensuring they can handle the demanding requirements of modern multimedia tasks while maximizing efficiency and resource use.
Real-World Applications and Use Cases
Real-world applications of Java in video processing span a diverse range of industries and use cases, showcasing the versatility and power of Java frameworks and libraries. From media streaming services to surveillance systems, Java’s robust ecosystem enables developers to build innovative solutions that leverage video content. Here are some notable applications:
1. Video Surveillance Systems
Java is a popular choice for developing video surveillance applications, thanks to its cross-platform capabilities and extensive libraries for video processing. Developers can implement features such as motion detection, object tracking, and video analytics using libraries like JavaCV. For example, a surveillance system can be designed to process live video feeds, analyzing them in real-time for suspicious activities. By using multithreading, these systems can efficiently handle multiple camera feeds concurrently.
import org.bytedeco.javacv.*; import org.bytedeco.javacv.FrameGrabber.Exception; public class SurveillanceSystem { public static void main(String[] args) { try (FrameGrabber grabber = OpenCVFrameGrabber.createDefault(0)) { grabber.start(); Frame frame; while ((frame = grabber.grab()) != null) { // Analyze the frame (e.g., detect motion, track objects) } grabber.stop(); } catch (Exception e) { e.printStackTrace(); } } }
2. Video Editing Software
Many video editing applications leverage Java for backend processing. With libraries like JCodec and JavaFX, developers can create tools that enable users to edit, trim, and apply effects to video clips. These applications often require a responsive user interface, which JavaFX provides with its rich set of UI components. The combination allows for seamless video playback and editing capabilities, empowering users to create professional-looking videos with ease.
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.layout.StackPane; import javafx.stage.Stage; public class VideoEditor extends Application { @Override public void start(Stage primaryStage) { Button editButton = new Button("Edit Video"); editButton.setOnAction(e -> { // Trigger video editing features }); StackPane root = new StackPane(); root.getChildren().add(editButton); primaryStage.setScene(new Scene(root, 400, 300)); primaryStage.show(); } public static void main(String[] args) { launch(args); } }
3. Live Streaming Services
Java is also extensively used in the development of live streaming platforms. By using frameworks like JavaCV, developers can implement real-time encoding and streaming of video content. These platforms often require low latency and high throughput, which can be achieved using Java’s concurrency features. Additionally, integrating with protocols such as RTMP (Real-Time Messaging Protocol) allows for efficient streaming of video content to a wide audience.
import org.bytedeco.javacv.*; public class LiveStreaming { public static void main(String[] args) { FFmpegFrameRecorder recorder = new FFmpegFrameRecorder("rtmp://live-streaming-server/app", 640, 480); recorder.setVideoCodec(avcodec.AV_CODEC_ID_H264); recorder.start(); // Capture and stream video frames FrameGrabber grabber = OpenCVFrameGrabber.createDefault(0); grabber.start(); Frame frame; while ((frame = grabber.grab()) != null) { recorder.record(frame); } recorder.stop(); grabber.stop(); } }
4. Educational and Training Tools
Educational institutions are increasingly adopting video-based learning tools, and Java can play a significant role in developing these applications. Features like interactive video lessons, quizzes, and assessment tools can be implemented using Java. By integrating video content with interactive elements, educators can enhance the learning experience. JavaFX provides the graphical capabilities needed to create engaging interfaces for these educational tools.
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.layout.StackPane; import javafx.stage.Stage; public class EducationalTool extends Application { @Override public void start(Stage primaryStage) { Button startLessonButton = new Button("Start Lesson"); startLessonButton.setOnAction(e -> { // Load and play educational video content }); StackPane root = new StackPane(); root.getChildren().add(startLessonButton); primaryStage.setScene(new Scene(root, 300, 200)); primaryStage.show(); } public static void main(String[] args) { launch(args); } }
These examples illustrate the diverse applications of Java in video processing. By using its powerful libraries and frameworks, developers can create innovative solutions that address real-world needs, making Java a valuable tool in the multimedia landscape.