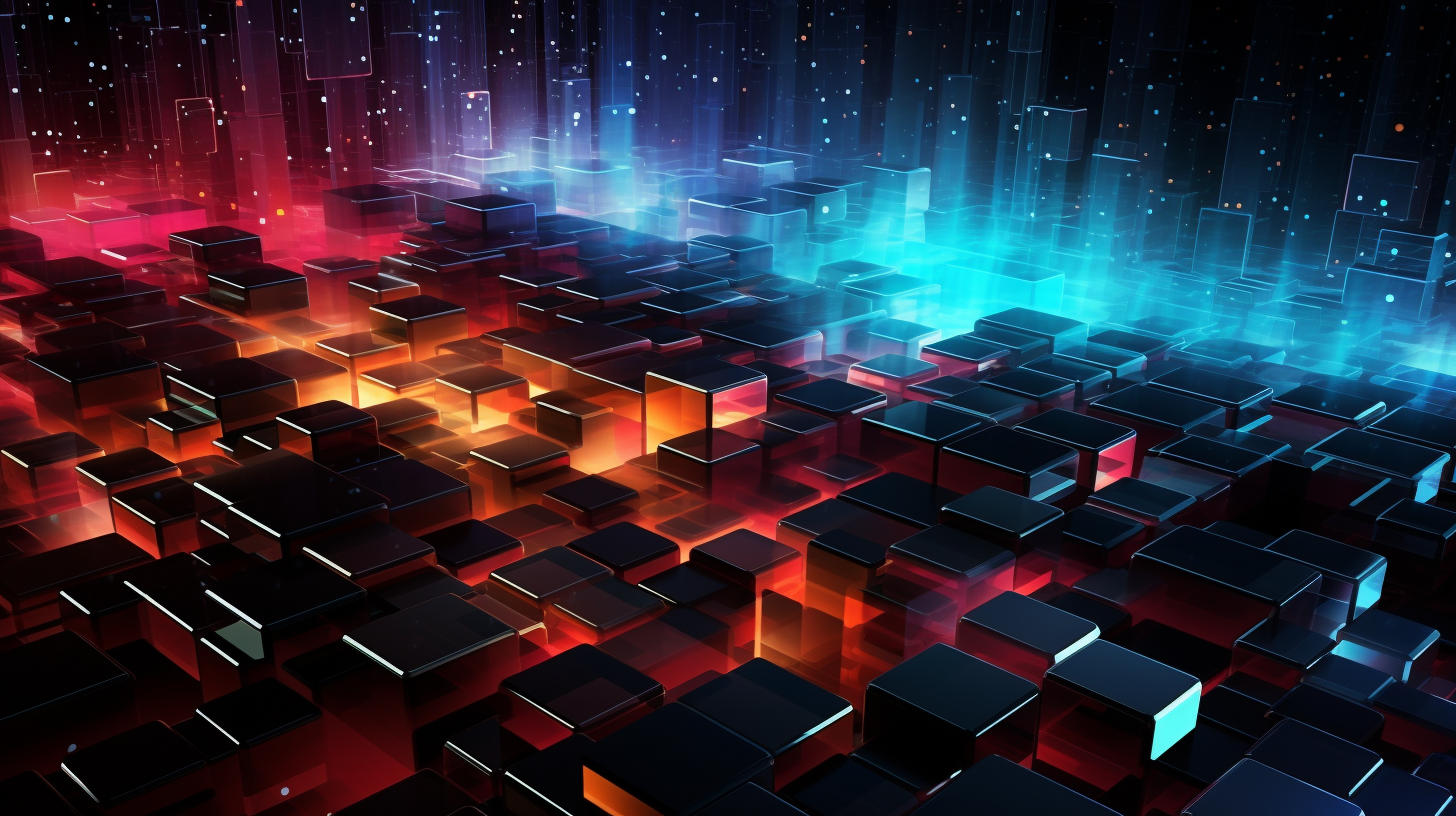
Java and WebSockets: Real-Time Communication
WebSockets represent a modern protocol that provides full-duplex communication channels over a single TCP connection. Unlike the traditional request-response model of HTTP, WebSockets allow for a persistent connection, enabling real-time data transfer between clients and servers. This capability especially important for applications that require quick updates and interactions, such as chat applications, online gaming, and live data feeds.
In Java, WebSocket support is provided through the Java API for WebSocket, which is part of the Java EE specification. This API simplifies the creation and management of WebSocket connections, allowing developers to focus on building their applications rather than dealing with the underlying protocol complexities.
To understand how WebSockets fit into the Java ecosystem, it’s important to ponder a few key concepts:
- An endpoint is a WebSocket connection that can communicate with a client or another server. In Java, you define an endpoint by creating a class that extends either
javax.websocket.Endpoint
or uses the@ClientEndpoint
and@ServerEndpoint
annotations. - A session represents a connection between the client and server. It allows you to send and receive messages. The session is initialized when the connection is established and can be used throughout the lifecycle of that connection.
- Messages can be sent in different formats, typically text or binary. The WebSocket API provides methods to handle incoming messages and send responses back to the client.
Here’s a simple example of a WebSocket server endpoint in Java:
import javax.websocket.OnMessage; import javax.websocket.Session; import javax.websocket.server.ServerEndpoint; @ServerEndpoint("/echo") public class EchoEndpoint { @OnMessage public String onMessage(String message, Session session) { return "Echo: " + message; } }
In the above code, we define a WebSocket server endpoint at the path /echo
. The onMessage
method is annotated with @OnMessage
, indicating that it will handle incoming messages. When a message is received, it simply echoes back the message prefixed with Echo:
.
On the client side, a WebSocket connection can be established using the WebSocket API available in Java. The client can initiate a connection to the server and listen for messages using similar endpoint conventions. This two-way communication is what makes WebSockets a powerful tool for developing interactive web applications.
Understanding the WebSocket lifecycle is also crucial. The connection progresses through several states:
- The initial state when a client is trying to establish a connection.
- The two-way communication channel is established, allowing messages to flow freely.
- One of the parties has initiated a closing handshake.
- The connection has been closed and is no longer available for communication.
By using WebSockets in Java, developers can create dynamic and responsive applications that can handle real-time interactions effectively. The combination of the Java API for WebSocket and the rich ecosystem of Java libraries and frameworks provides a solid foundation for building powerful web applications.
Setting Up a Java WebSocket Server
Setting up a Java WebSocket server involves a few essential steps. First, you need to ensure that your environment is prepared to use the Java WebSocket API. This typically requires a Java EE-compatible server, such as Apache Tomcat or WildFly, which can handle WebSocket connections.
Once your server is set up, you can start by creating a WebSocket server endpoint. That is done by defining a class that will handle WebSocket events. In the Java EE environment, a server endpoint can be defined using the @ServerEndpoint annotation. This annotation specifies the URI at which clients will connect to your WebSocket server.
Here’s a basic example of how to set up a WebSocket server endpoint in Java:
import javax.websocket.OnOpen; import javax.websocket.OnMessage; import javax.websocket.OnClose; import javax.websocket.Session; import javax.websocket.server.ServerEndpoint; @ServerEndpoint("/chat") public class ChatEndpoint { @OnOpen public void onOpen(Session session) { System.out.println("Connected: " + session.getId()); } @OnMessage public String onMessage(String message, Session session) { System.out.println("Message from client: " + message); return "Server received: " + message; } @OnClose public void onClose(Session session) { System.out.println("Disconnected: " + session.getId()); } }
In this example, the ChatEndpoint class defines a WebSocket server at the “/chat” path. The onOpen method is called when a new client connection is established, which will allow you to perform any initial setup or logging. The onMessage method handles incoming messages, which can be processed and responded to as needed. Finally, the onClose method is executed when the client disconnects, providing an opportunity for cleanup tasks.
To run this WebSocket server, you need to deploy it on a Java EE-compliant server. You’ll typically package your application as a .war file and deploy it to your server. Once deployed, clients can connect to your WebSocket server using the specified URI, initiating real-time communication.
It’s also important to configure your server to support WebSocket connections. Ensure that your web.xml file (if you’re using one) does not block WebSocket traffic and that your server runtime supports the necessary features. For instance, in Tomcat, you may need to ensure that the WebSocket API is included in your server installation.
With everything in place, you can start your server and test the functionality by connecting a WebSocket client. This setup serves as the foundation for building more complex applications, where you can implement features such as broadcasting messages to multiple clients, maintaining user sessions, or handling different message types.
Creating a WebSocket Client in Java
Creating a WebSocket client in Java is a simpler process, enabling you to establish a connection to a WebSocket server and engage in real-time communication. To implement a WebSocket client, you’ll typically use the Java API for WebSocket, which provides a convenient interface for managing connections and handling messages.
The client can be created using the javax.websocket.ContainerProvider
class, which provides an entry point for obtaining a WebSocket connection. You can use the connectToServer
method to connect to the server endpoint. To handle incoming messages and connection events, you will implement a client endpoint class, which can be annotated with @ClientEndpoint
.
Here’s a basic example of how to create a WebSocket client in Java:
import javax.websocket.ClientEndpoint; import javax.websocket.OnOpen; import javax.websocket.OnMessage; import javax.websocket.OnClose; import javax.websocket.Session; import javax.websocket.ContainerProvider; import javax.websocket.WebSocketContainer; import java.net.URI; @ClientEndpoint public class MyWebSocketClient { @OnOpen public void onOpen(Session session) { System.out.println("Connected to server: " + session.getId()); } @OnMessage public void onMessage(String message) { System.out.println("Received message: " + message); } @OnClose public void onClose(Session session) { System.out.println("Disconnected from server: " + session.getId()); } public static void main(String[] args) { try { WebSocketContainer container = ContainerProvider.getWebSocketContainer(); URI uri = URI.create("ws://localhost:8080/chat"); container.connectToServer(MyWebSocketClient.class, uri); } catch (Exception e) { e.printStackTrace(); } } }
In this example, the MyWebSocketClient
class serves as the client endpoint. The onOpen
method is invoked when the connection to the WebSocket server is established, allowing you to perform any setup needed at that point. The onMessage
method processes incoming messages from the server, and the onClose
method is executed when the connection is closed, providing a chance to clean up resources or log the disconnection.
The main
method demonstrates how to create a WebSocket client. The WebSocketContainer
is obtained using the ContainerProvider
. The connectToServer
method is then called with the endpoint class and the URI of the WebSocket server. This establishes the connection and allows the client to start communicating with the server.
It’s essential to handle potential exceptions that may arise during the connection process, such as DeploymentException
or IOException
. Implementing robust error handling will ensure that your application can gracefully recover from issues that may occur during communication.
By creating a WebSocket client in Java, you can seamlessly connect to a WebSocket server and participate in real-time data exchanges, making your applications more interactive and responsive to user actions. This capability is particularly useful for applications that require live updates, such as online collaboration tools, live chats, or gaming applications.
Handling WebSocket Events and Messages
Handling WebSocket events and messages in Java is where the real power of the protocol shines. This is the phase where you define how your application reacts to incoming messages, what happens when a connection is opened, and the procedures for cleaning up when a connection is closed. The Java API for WebSocket makes this process intuitive, allowing developers to focus on the application logic rather than the underlying complexities of the protocol.
When a WebSocket connection is established, the server and client enter a state where they can send and receive messages. That is facilitated through various annotated methods in your endpoint classes, which respond to different events in the WebSocket lifecycle. Key events include:
- Triggered when a new connection is established.
- Invoked when a message is received from the client or server.
- Called when the connection is closed.
- Occurs when there is an error in the WebSocket connection.
Let’s delve deeper into these events by enhancing our earlier ChatEndpoint class to include error handling and additional logic for processing messages.
import javax.websocket.OnOpen; import javax.websocket.OnMessage; import javax.websocket.OnClose; import javax.websocket.OnError; import javax.websocket.Session; import javax.websocket.server.ServerEndpoint; @ServerEndpoint("/chat") public class ChatEndpoint { @OnOpen public void onOpen(Session session) { System.out.println("Connected: " + session.getId()); } @OnMessage public String onMessage(String message, Session session) { System.out.println("Message from client: " + message); // Validate and process the incoming message if (message == null || message.trim().isEmpty()) { return "Error: Message cannot be empty."; } // Echo back the processed message return "Server received: " + message; } @OnClose public void onClose(Session session) { System.out.println("Disconnected: " + session.getId()); } @OnError public void onError(Session session, Throwable throwable) { System.err.println("Error on session " + session.getId() + ": " + throwable.getMessage()); } }
In this revised ChatEndpoint class, we added an onError method to handle any exceptions that may occur during communication. This is critical for debugging and maintaining robust applications. The onMessage method now includes simple validation to ensure that the incoming message is not empty, returning an error response if it is. This validation step exemplifies how you can enforce application rules and improve user experience by providing feedback directly through the WebSocket connection.
When dealing with real-time applications, particularly in scenarios like chat applications or collaborative tools, you might want to broadcast messages to all connected users. To achieve this, you would typically maintain a list of active sessions and loop through them to send messages as needed. Here’s how you can adapt the ChatEndpoint class to support broadcasting:
import javax.websocket.OnOpen; import javax.websocket.OnMessage; import javax.websocket.OnClose; import javax.websocket.Session; import javax.websocket.server.ServerEndpoint; import java.util.Set; import java.util.concurrent.CopyOnWriteArraySet; @ServerEndpoint("/chat") public class ChatEndpoint { private static Set chatSessions = new CopyOnWriteArraySet(); @OnOpen public void onOpen(Session session) { chatSessions.add(session); System.out.println("Connected: " + session.getId()); } @OnMessage public void onMessage(String message, Session senderSession) { System.out.println("Message from client: " + message); // Broadcast message to all clients for (Session session : chatSessions) { if (session.isOpen() && !session.equals(senderSession)) { session.getAsyncRemote().sendText(message); } } } @OnClose public void onClose(Session session) { chatSessions.remove(session); System.out.println("Disconnected: " + session.getId()); } }
In this example, we maintain a Set of active sessions. When a message is received, we loop through this set and send the message to all other connected clients. This broadcasting approach is fundamental in chat applications where multiple clients need to receive messages in real time.
Handling WebSocket events and messages in Java is not only about reacting to inputs; it’s about crafting a seamless interaction model that enhances user experience. By strategically managing these events, developers can build applications that are not only responsive but also resilient to errors and capable of handling multiple connections gracefully.
Implementing Real-Time Features in Your Application
Implementing real-time features in your application using WebSockets is a game-changer, which will allow you to leverage the full-duplex communication channel to create responsive and dynamic user experiences. The essence of real-time functionality is in how efficiently your application can push data to clients and respond to user actions without the delays typically associated with traditional HTTP requests.
Real-time features often manifest in a variety of use cases, such as notifications, live updates, collaborative environments, and even multimedia streaming. The key is to exploit the persistent connection provided by WebSockets, which allows for immediate data exchange as events occur.
To illustrate how to implement real-time features, let’s enhance our previous ChatEndpoint example to support a simple notification system. This will allow clients to receive real-time updates whenever a new user connects or disconnects, fostering a more interactive environment.
import javax.websocket.OnOpen; import javax.websocket.OnMessage; import javax.websocket.OnClose; import javax.websocket.Session; import javax.websocket.server.ServerEndpoint; import java.util.Set; import java.util.concurrent.CopyOnWriteArraySet; @ServerEndpoint("/chat") public class ChatEndpoint { private static Set<Session> chatSessions = new CopyOnWriteArraySet<>(); @OnOpen public void onOpen(Session session) { chatSessions.add(session); broadcast("User connected: " + session.getId()); } @OnMessage public void onMessage(String message, Session senderSession) { System.out.println("Message from client: " + message); broadcast(message, senderSession); } @OnClose public void onClose(Session session) { chatSessions.remove(session); broadcast("User disconnected: " + session.getId()); } private void broadcast(String message, Session senderSession) { for (Session session : chatSessions) { if (session.isOpen() && !session.equals(senderSession)) { session.getAsyncRemote().sendText(message); } } } private void broadcast(String message) { for (Session session : chatSessions) { if (session.isOpen()) { session.getAsyncRemote().sendText(message); } } } }
In this updated ChatEndpoint class, we’ve implemented a broadcast
method that enables sending messages to all connected clients. When a user connects, they receive a notification about the new user, and when they disconnect, all clients are informed. This not only enhances user engagement but also provides a sense of community within the application.
Another crucial aspect of implementing real-time features is the ability to handle messages efficiently. For applications that require complex interactions, such as gaming or collaborative editing tools, the messages exchanged might need to include structured data. In these scenarios, ponder using JSON or other serialization formats to encapsulate the data effectively. Here’s a brief example of how to send structured messages:
import com.fasterxml.jackson.databind.ObjectMapper; public void sendStructuredMessage(String eventType, Object payload, Session senderSession) { ObjectMapper mapper = new ObjectMapper(); try { String message = mapper.writeValueAsString(new Message(eventType, payload)); broadcast(message, senderSession); } catch (Exception e) { e.printStackTrace(); } } private static class Message { private String eventType; private Object payload; public Message(String eventType, Object payload) { this.eventType = eventType; this.payload = payload; } // Getters and setters omitted for brevity }
Using a library like Jackson for JSON serialization allows you to cleanly encapsulate events and data into structured messages. This approach is particularly effective in applications where different types of messages may need to be handled differently.
Lastly, ponder the scalability of your real-time features. If your application grows to support a large number of concurrent users, you may need to implement additional strategies such as message queuing, clustering, or even using dedicated WebSocket servers to manage connections efficiently. Technologies like Redis or Apache Kafka can help in managing state and distributing messages across multiple instances of your WebSocket server.
By thoughtfully implementing real-time features using WebSockets in Java, you can create applications that not only respond to user actions instantaneously but also foster dynamic and engaging user experiences. This capability marks a significant advancement over traditional request-response models, placing your application at the forefront of modern web technologies.
Best Practices for WebSocket Development in Java
When developing applications that utilize WebSockets, adhering to best practices is important for ensuring scalability, maintainability, and performance. Here are several strategies to ponder when implementing WebSocket communication in your Java applications:
1. Optimize Connection Management
WebSocket connections are persistent, which means they can consume server resources over time. It’s essential to close connections that are no longer needed. Implement a timeout mechanism to automatically close inactive sessions, which will help free up resources and prevent memory leaks. You can use the setMaxIdleTimeout
method on the Session
object to specify a timeout period. For example:
session.setMaxIdleTimeout(30000); // 30 seconds timeout
2. Use Asynchronous Communication
Leverage the asynchronous capabilities provided by the WebSocket API to handle message sending and receiving. This helps in maintaining responsiveness, especially in high-load scenarios. Use the getAsyncRemote()
method of the Session
object for non-blocking message delivery:
session.getAsyncRemote().sendText("Hello, World!");
3. Implement Robust Error Handling
Errors can occur during WebSocket communication due to various reasons such as network issues or server overload. Implement comprehensive error handling in your onError
method to catch exceptions and potentially recover from failures:
@OnError public void onError(Session session, Throwable throwable) { System.err.println("Error on session " + session.getId() + ": " + throwable.getMessage()); // Additional logic to handle the error, such as notifying clients or logging }
4. Secure Your WebSocket Connections
Security is paramount when dealing with real-time communication. Use the secure WebSocket protocol (WSS) to encrypt data in transit. Ensure that you validate incoming messages and implement authentication mechanisms to verify users before allowing them to establish a connection. Ponder using JSON Web Tokens (JWT) for this purpose.
5. Scale Your WebSocket Server
If your application is expected to handle a large number of concurrent connections, consider horizontal scaling. This can be achieved by deploying multiple instances of your WebSocket server behind a load balancer. Use sticky sessions to ensure that all messages from a particular client are directed to the same server instance, which simplifies session management.
6. Monitor and Log WebSocket Activity
Implement logging to capture important events such as connections, disconnections, and message transmissions. Monitoring tools can help you track performance metrics, including connection counts and latency. This information is invaluable for diagnosing issues and optimizing your infrastructure.
7. Regularly Update Dependencies
Keep your WebSocket library and server environment up to date with the latest security patches and performance improvements. Regular updates can help avoid vulnerabilities and ensure you have access to the latest features.
Implementing these best practices in your WebSocket development can lead to a more stable, scalable, and effortless to handle application. By focusing on efficient resource management, robust error handling, and security, you can harness the full potential of real-time communication in your Java applications.