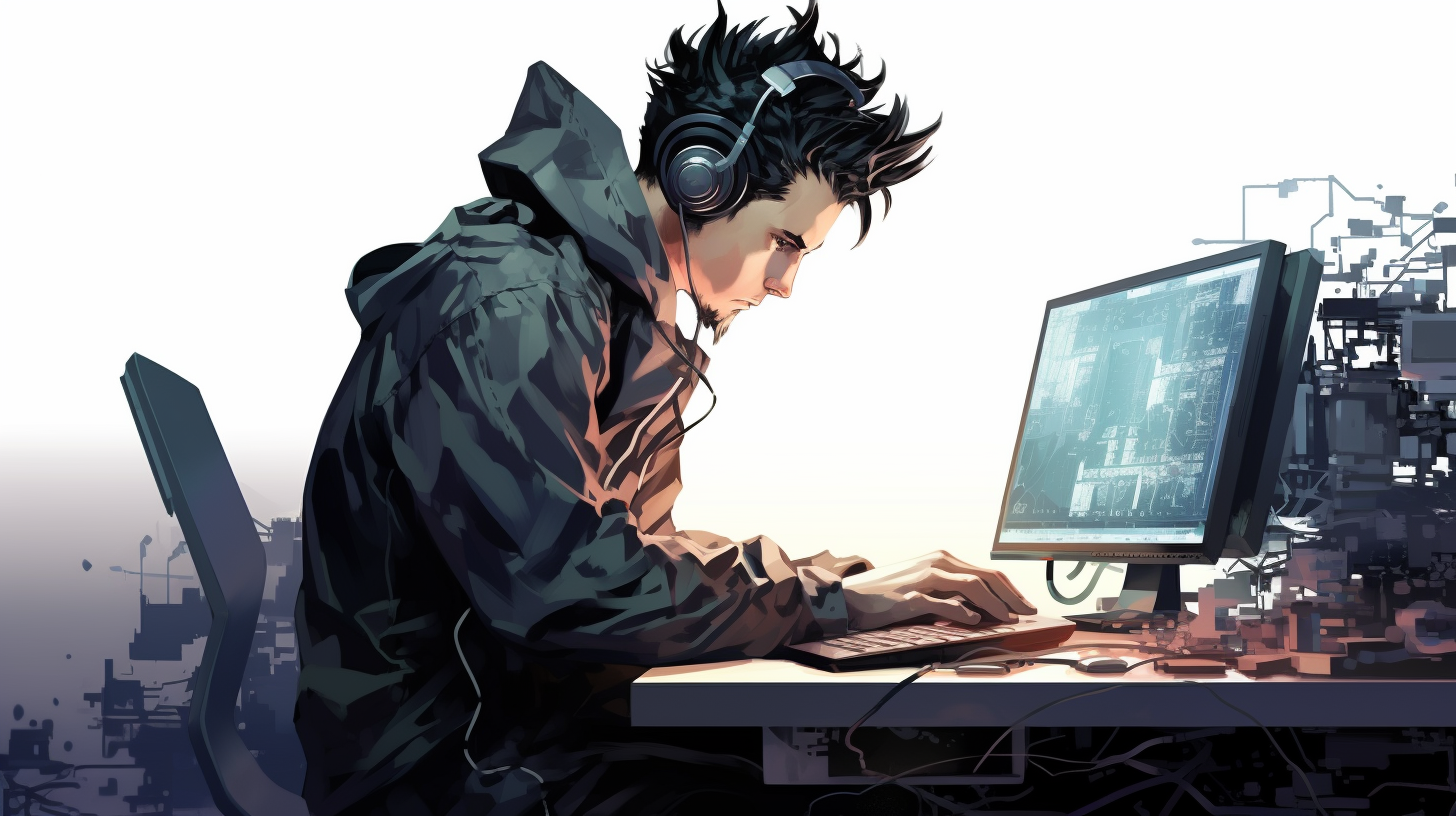
Java and Workflow Automation: Tools and Techniques
Workflow automation in Java brings a blend of efficiency and control to the development process. By using Java’s robust ecosystem and its ability to integrate with various systems, developers can create automated workflows that streamline repetitive tasks, enhance productivity, and reduce human error. Workflows can range from simple data processing tasks to complex integrations involving multiple services and applications.
At its core, workflow automation in Java allows you to define a series of tasks that should be executed in a specific order based on certain conditions. The power of Java lies in its ability to handle these tasks simultaneously, enabling you to manage workflows that can run in parallel while ensuring data consistency and integrity.
Java provides several frameworks and libraries that facilitate workflow automation. These tools allow developers to design, execute, and monitor workflows with minimal overhead. Understanding these tools especially important for building robust automation solutions.
For instance, think a simple automated workflow that processes incoming data, performs some validation, and then stores it in a database. In Java, this can be managed through a combination of threads, tasks, and interactions with database APIs.
Here’s an example of a basic Java workflow that demonstrates how to manage tasks sequentially:
import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; public class SimpleWorkflow { public static void main(String[] args) { ExecutorService executor = Executors.newFixedThreadPool(3); executor.execute(() -> { System.out.println("Task 1: Data Retrieval"); // Simulate data retrieval }); executor.execute(() -> { System.out.println("Task 2: Data Processing"); // Simulate data processing }); executor.execute(() -> { System.out.println("Task 3: Data Storage"); // Simulate data storage }); executor.shutdown(); } }
This snippet illustrates the use of an ExecutorService
to manage three tasks that could represent different stages in a workflow. By using a thread pool, we can efficiently handle multiple tasks in parallel, improving overall performance.
As workflows grow in complexity, integrating additional libraries or tools becomes essential. Java’s versatility allows for easy integration with RESTful APIs, messaging queues, and other automation tools, making it a strong candidate for enterprise-level workflow automation. With the right approach and tools, Java developers can build comprehensive solutions that address specific business needs through effective workflow automation.
Key Java Libraries for Workflow Automation
When delving into the key Java libraries available for workflow automation, we find a rich tapestry of tools that enhance the capabilities of Java developers. Each library offers unique features, catering to various requirements in the workflow automation spectrum. Understanding these libraries is paramount for using their strengths in building efficient automation solutions.
Apache Camel stands out as a powerful integration framework that simplifies the task of connecting various systems through routing and mediation. It supports numerous protocols and data formats, allowing developers to create routes that dictate how data flows between endpoints. With a simple, domain-specific language (DSL), you can define workflows in a concise manner. Here’s a quick example of how you might set up a route in Camel:
import org.apache.camel.CamelContext; import org.apache.camel.impl.DefaultCamelContext; import org.apache.camel.builder.RouteBuilder; public class WorkflowExample { public static void main(String[] args) throws Exception { CamelContext context = new DefaultCamelContext(); context.addRoutes(new RouteBuilder() { @Override public void configure() { from("file:input?noop=true") .to("processor:processData") .to("file:output"); } }); context.start(); Thread.sleep(5000); context.stop(); } }
This code snippet showcases a simple file processing workflow. It reads files from an input directory, processes them, and outputs them to another directory. Apache Camel’s extensive component library allows you to customize and extend this workflow further, incorporating various sources and destinations seamlessly.
Spring Batch is another indispensable library, particularly suited for processing large volumes of data. It provides comprehensive support for batch processing, including transaction management, job processing statistics, and job partitioning. That is invaluable in workflows that require data transformations and aggregations. Here is a simplified example of a Spring Batch job:
import org.springframework.batch.core.Job; import org.springframework.batch.core.Step; import org.springframework.batch.core.configuration.annotation.EnableBatchProcessing; import org.springframework.batch.core.configuration.annotation.JobBuilderFactory; import org.springframework.batch.core.configuration.annotation.StepBuilderFactory; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; @Configuration @EnableBatchProcessing public class BatchConfiguration { @Bean public Job myJob(JobBuilderFactory jobBuilderFactory, StepBuilderFactory stepBuilderFactory) { Step step = stepBuilderFactory.get("step1") .tasklet((contribution, chunkContext) -> { System.out.println("Executing step 1"); return null; }).build(); return jobBuilderFactory.get("myJob") .start(step) .build(); } }
This example defines a simple batch job with a single step that prints a message. Spring Batch allows for more complex workflows with multiple steps, job parameters, and the ability to handle failures and retries, making it a robust choice for batch-oriented workflows.
JBPM (Java Business Process Model) is another significant player in the sphere of workflow automation. As a business process management suite, JBPM allows you to model, execute, and monitor business processes. It provides a graphical interface for creating processes and supports BPMN 2.0, which is important for defining complex workflows. Below is a basic example of how to integrate JBPM into a Java application:
import org.kie.api.KieServices; import org.kie.api.runtime.KieContainer; import org.kie.api.runtime.KieSession; public class JBPMExample { public static void main(String[] args) { KieServices kieServices = KieServices.Factory.get(); KieContainer kieContainer = kieServices.getKieClasspathContainer(); KieSession kieSession = kieContainer.newKieSession("mySession"); kieSession.startProcess("com.example.myProcess"); kieSession.dispose(); } }
This snippet initializes a JBPM session and starts a defined process. The ability to model processes visually, combined with Java’s extensibility, makes JBPM a powerful tool for managing complex workflows that require monitoring and human interactions.
In addition to these libraries, there are many other tools like Activiti, Camunda, and Quartz that further enhance Java’s workflow automation capabilities. Each library comes with its strengths and integrations, allowing developers to choose the one that best fits their project needs. As workflows evolve, employing the right combinations of these libraries can significantly elevate the efficiency and reliability of automation solutions.
Designing Workflow Automation Solutions in Java
Designing workflow automation solutions in Java involves a methodical approach that encapsulates both the high-level structure of the workflows and the intricate details of task management. The key to effective design lies in understanding the requirements of the automation task at hand and how best to represent that in code. A well-designed workflow should be modular, scalable, and maintainable, ensuring that as business needs evolve, the automation solution can adapt with minimal friction.
At the outset, it’s essential to identify the various components of the workflow. Each component generally corresponds to a specific task or operation that contributes to the overall process. A good practice is to define these tasks as discrete classes or methods, allowing for clear separation of concerns. This modularity facilitates testing and debugging, as each part of the workflow can be developed and validated independently.
One common design pattern in Java workflows is the Chain of Responsibility. This pattern allows tasks to be executed sequentially, with each task capable of passing the request to the next task in the chain if it cannot process it. This is particularly useful in scenarios where tasks can have conditional flows based on the output of previous tasks.
interface Task { void execute(); } class TaskA implements Task { public void execute() { System.out.println("Executing Task A"); } } class TaskB implements Task { public void execute() { System.out.println("Executing Task B"); } } class TaskChain { private List tasks = new ArrayList(); public void addTask(Task task) { tasks.add(task); } public void execute() { for (Task task : tasks) { task.execute(); } } } public class WorkflowDemo { public static void main(String[] args) { TaskChain taskChain = new TaskChain(); taskChain.addTask(new TaskA()); taskChain.addTask(new TaskB()); taskChain.execute(); } }
In this example, the TaskChain class manages a sequence of tasks. Each individual task implements the Task interface, promoting loose coupling and enhancing the flexibility of the workflow. When you need to add or modify tasks, you simply adjust the composition of the TaskChain without altering the logic of the tasks themselves.
As workflows become more complex, incorporating asynchronous processing can greatly enhance performance. Java’s CompletableFuture can be employed to manage tasks that can run independently of one another. By using this feature, you can design workflows that execute tasks in parallel, reducing overall execution time while maintaining order where needed.
import java.util.concurrent.CompletableFuture; public class AsyncWorkflow { public static void main(String[] args) { CompletableFuture task1 = CompletableFuture.runAsync(() -> { System.out.println("Executing Task 1"); }); CompletableFuture task2 = CompletableFuture.runAsync(() -> { System.out.println("Executing Task 2"); }); CompletableFuture combinedFuture = CompletableFuture.allOf(task1, task2); combinedFuture.join(); // Wait for all tasks to complete } }
This snippet demonstrates how two tasks can be executed asynchronously using CompletableFuture. By using futures, you gain the ability to manage complex workflows that can scale with performance demands, as multiple operations occur concurrently.
Another essential consideration in designing Java-based workflows is error handling and recovery. An effective workflow should gracefully handle failures, allowing the system to either retry the operation, roll back changes, or escalate the issue for manual intervention. Implementing comprehensive logging within each task can aid in diagnosing issues quickly.
Incorporating a state management system can also improve the robustness of your workflow design. This allows you to track the progress of tasks, manage dependencies, and ensure that the workflow can resume from where it left off in the event of a failure. Java’s native capabilities, combined with tools like Spring State Machine, can be leveraged to implement these advanced features.
Ultimately, the design phase of workflow automation in Java should be driven by a clear understanding of the business requirements, careful selection of design patterns, and an eye toward future scalability and maintainability. Through thoughtful architecture and using Java’s extensive libraries, developers can create powerful automation solutions that meet the demands of state-of-the-art business environments.
Integrating Java with Third-Party Automation Tools
Integrating Java with third-party automation tools enhances workflow automation capabilities significantly. This integration enables Java applications to harness the features and efficiencies offered by external systems, thereby simplifying complex processes and fostering interoperability. A well-planned integration strategy can turn Java-based applications into robust automation engines capable of interacting seamlessly with other tools and services.
One of the most common approaches to integrate Java with third-party automation tools is through RESTful APIs. Most modern automation tools offer REST APIs that allow developers to interact with the services programmatically. Using libraries such as Apache HttpClient or Spring’s RestTemplate makes it easy to make HTTP requests from Java. Here’s an example of how to use Spring’s RestTemplate to interact with an external automation service:
import org.springframework.web.client.RestTemplate; public class ApiIntegration { public static void main(String[] args) { RestTemplate restTemplate = new RestTemplate(); String url = "https://api.example.com/trigger-automation"; String response = restTemplate.postForObject(url, null, String.class); System.out.println("Response from automation service: " + response); } }
This snippet demonstrates a simple POST request to an external automation service. The response can be logged or processed further based on the needs of the workflow. By implementing such integrations, Java developers can expand the functionality of their applications and enable workflows that span multiple platforms.
Messaging systems also play an important role in integrating Java applications with automation tools. Tools like RabbitMQ or Apache Kafka facilitate asynchronous communication between different systems. By producing and consuming messages, Java applications can trigger workflows based on events, enhancing responsiveness and scalability. Below is an example of using RabbitMQ with Java:
import com.rabbitmq.client.ConnectionFactory; import com.rabbitmq.client.Connection; import com.rabbitmq.client.Channel; public class RabbitMQExample { private final static String QUEUE_NAME = "workflow_queue"; public static void main(String[] argv) throws Exception { ConnectionFactory factory = new ConnectionFactory(); factory.setHost("localhost"); try (Connection connection = factory.newConnection(); Channel channel = connection.createChannel()) { channel.queueDeclare(QUEUE_NAME, false, false, false, null); String message = "Triggering workflow"; channel.basicPublish("", QUEUE_NAME, null, message.getBytes()); System.out.println("Sent: '" + message + "'"); } } }
In this example, a message is sent to a RabbitMQ queue. Other components of the workflow can listen to this queue and trigger automation tasks based on incoming messages. This decoupled architecture promotes a more responsive, event-driven approach to workflow automation.
For scenario-based integrations, Java applications can also connect to orchestration platforms like Apache Airflow or Camunda. These tools provide a higher-level abstraction for managing complex workflows, so that you can define dependencies and execution flow declaratively. For instance, invoking a Camunda process from Java can be accomplished with the following code:
import org.camunda.bpm.engine.ProcessEngines; import org.camunda.bpm.engine.RuntimeService; public class CamundaIntegration { public static void main(String[] args) { RuntimeService runtimeService = ProcessEngines.getDefaultProcessEngine().getRuntimeService(); runtimeService.startProcessInstanceByKey("myProcess"); System.out.println("Started Camunda process instance"); } }
This code showcases how to start a process instance in Camunda using its Java API. Such integrations can significantly enhance the operational efficiency of Java applications, enabling them to leverage advanced workflow management capabilities.
Moreover, Java applications can also leverage cloud-based automation services like Zapier or Integromat by using their APIs. These platforms allow for the creation of automated workflows that can connect multiple services without deep technical integration. By simply making HTTP requests to these services, Java developers can create powerful automations that bridge different ecosystems.
The integration of Java with third-party automation tools not only extends the capabilities of Java applications but also facilitates the creation of more sophisticated and responsive workflows. By strategically using RESTful APIs, messaging systems, and orchestration platforms, developers can build comprehensive solutions that adapt to evolving business needs while maintaining a high level of reliability and performance.
Best Practices for Java Workflow Automation
When embarking on the journey of Java workflow automation, adhering to best practices is paramount to ensure that your solutions are not only effective but also maintainable and scalable. These practices encompass design principles, coding standards, and operational strategies that can significantly enhance the quality and efficiency of your automation efforts.
Modularity and Reusability are essential characteristics of a well-designed workflow. By structuring your automation tasks into independent modules or services, you improve the maintainability of your code. Each module should encapsulate a specific functionality, which can be reused across different workflows. This separation allows for easier testing and debugging as any changes in one module do not directly affect others. For instance, if you have a module for data validation, you can utilize it across various workflows without duplicating code.
public class DataValidator { public boolean validate(String data) { // Implement validation logic return data != null && !data.isEmpty(); } } public class Workflow { private DataValidator validator = new DataValidator(); public void execute(String data) { if (validator.validate(data)) { System.out.println("Data is valid, proceeding with workflow."); // Continue with the workflow } else { System.out.println("Invalid data, aborting workflow."); } } }
Another critical best practice is asynchronous processing. Java provides powerful concurrency utilities that can be leveraged to run tasks at once, using resources more effectively and reducing overall execution time. Employing constructs like CompletableFuture
allows you to initiate multiple tasks that can be executed in parallel, improving responsiveness and scalability of your workflows.
import java.util.concurrent.CompletableFuture; public class ParallelWorkflow { public static void main(String[] args) { CompletableFuture task1 = CompletableFuture.runAsync(() -> { System.out.println("Task 1 executing..."); }); CompletableFuture task2 = CompletableFuture.runAsync(() -> { System.out.println("Task 2 executing..."); }); CompletableFuture allTasks = CompletableFuture.allOf(task1, task2); allTasks.join(); // Wait for all tasks to complete } }
Error Handling is another vital aspect of workflow automation. Robust error handling ensures that your workflows can gracefully recover from unexpected failures. Implementing retry mechanisms, logging errors, and providing meaningful feedback are integral to maintaining reliability. Using a centralized logging framework like SLF4J or Log4j can help track issues effectively.
import org.slf4j.Logger; import org.slf4j.LoggerFactory; public class ErrorHandlingExample { private static final Logger logger = LoggerFactory.getLogger(ErrorHandlingExample.class); public void executeTask() { try { // Simulate task execution throw new RuntimeException("Simulated task failure."); } catch (Exception e) { logger.error("An error occurred during task execution: {}", e.getMessage()); // Implement retry logic or fallback } } }
Another best practice is version control for your workflows. As your automation solutions evolve, maintaining version history can help track changes, roll back to previous versions if needed, and ensure that updates do not disrupt existing operations. Tools like Git can be used to manage and version your workflow codebases effectively.
Lastly, testing and validation of workflows should not be overlooked. Automated testing frameworks such as JUnit can be employed to ensure that each component of your workflow functions correctly under various conditions. Comprehensive unit tests, integration tests, and end-to-end tests will help catch issues early, improving the overall robustness of your automation solutions.
import org.junit.Test; import static org.junit.Assert.assertTrue; public class DataValidatorTest { private DataValidator validator = new DataValidator(); @Test public void testValidData() { assertTrue(validator.validate("Valid Data")); } @Test public void testInvalidData() { assertFalse(validator.validate("")); } }
By adhering to these best practices—modularity, asynchronous processing, robust error handling, version control, and comprehensive testing—Java developers can craft workflows that are not only efficient but also resilient and adaptable to changing business needs. This disciplined approach lays the foundation for creating powerful workflow automation solutions that leverage the full potential of the Java ecosystem.
Real-World Use Cases of Java in Workflow Automation
Real-world use cases of Java in workflow automation reveal its versatility and power across various industries. From data processing and reporting to integration with complex business processes, Java has proven itself as a reliable backbone for automation solutions. One compelling example is in the finance sector, where Java-driven automated systems are employed to handle large volumes of transactions, compliance checks, and reporting.
In a banking environment, consider a scenario where incoming transactions must be validated and processed efficiently. Java can be utilized to create a workflow that retrieves transaction data, applies business rules, and updates customer accounts. Here’s an illustration of how such a workflow might look:
import java.util.concurrent.CompletableFuture; public class BankingWorkflow { public static void main(String[] args) { CompletableFuture transactionValidation = CompletableFuture.runAsync(() -> { System.out.println("Validating transaction..."); // Simulate validation logic }); CompletableFuture accountUpdate = transactionValidation.thenRun(() -> { System.out.println("Updating account balance..."); // Simulate account update logic }); accountUpdate.join(); } }
This example leverages Java’s CompletableFuture to execute transaction validation and account updates asynchronously. This ensures that the workflow remains responsive, allowing for multiple transactions to be processed concurrently without blocking. Such efficiency is critical in environments where timing and accuracy are paramount.
Another prominent use case is in supply chain management. Companies often deal with the complexities of managing inventory, orders, and shipments. Java can automate these processes by integrating with various systems through APIs, messaging queues, and databases. For instance, a company could automate order fulfillment by using a combination of Java and Apache Camel to route information between different systems involved in the supply chain:
import org.apache.camel.CamelContext; import org.apache.camel.impl.DefaultCamelContext; import org.apache.camel.builder.RouteBuilder; public class SupplyChainWorkflow { public static void main(String[] args) throws Exception { CamelContext context = new DefaultCamelContext(); context.addRoutes(new RouteBuilder() { @Override public void configure() { from("direct:order") .to("service:inventoryService") .to("service:shippingService"); } }); context.start(); Thread.sleep(5000); context.stop(); } }
In this workflow, orders are received through a direct endpoint and processed sequentially through inventory and shipping services. This establishes a clear flow of information, ensuring that each step of the order fulfillment process is automated and monitored effectively.
Healthcare is another domain where Java’s workflow automation shines. Patient care processes often require multi-step workflows to manage appointments, treatments, and billing. Java applications can facilitate these workflows by integrating with Electronic Health Record (EHR) systems, enabling seamless data transfer and ensuring that patient information is up-to-date. Here’s a simplistic example:
import java.util.concurrent.CompletableFuture; public class HealthcareWorkflow { public static void main(String[] args) { CompletableFuture appointmentScheduling = CompletableFuture.runAsync(() -> { System.out.println("Scheduling appointment..."); // Simulate appointment scheduling }); CompletableFuture treatmentUpdate = appointmentScheduling.thenRun(() -> { System.out.println("Updating treatment plans..."); // Simulate treatment update logic }); treatmentUpdate.join(); } }
The above code demonstrates an asynchronous workflow that schedules patient appointments and updates treatment plans. This use of Java ensures that healthcare providers can manage multiple tasks efficiently, improving patient experience and operational efficiency.
Finally, in the context of manufacturing, Java is employed for automating production lines, monitoring equipment, and collecting data for analysis. The integration of IoT devices with Java applications allows manufacturers to implement workflows that optimize production processes. For example:
import java.util.concurrent.CompletableFuture; public class ManufacturingWorkflow { public static void main(String[] args) { CompletableFuture machineMonitor = CompletableFuture.runAsync(() -> { System.out.println("Monitoring machine performance..."); // Simulate monitoring logic }); CompletableFuture qualityCheck = machineMonitor.thenRun(() -> { System.out.println("Conducting quality checks..."); // Simulate quality check logic }); qualityCheck.join(); } }
In this example, machine performance is monitored, followed by quality checks, ensuring that manufacturing processes remain efficient and that any issues are promptly addressed.
These examples illustrate the adaptability of Java in designing and executing workflows across a diverse array of industries. By using Java’s concurrency features, integration capabilities, and robust libraries, organizations can automate complex processes, resulting in enhanced efficiency, reduced errors, and improved overall productivity.