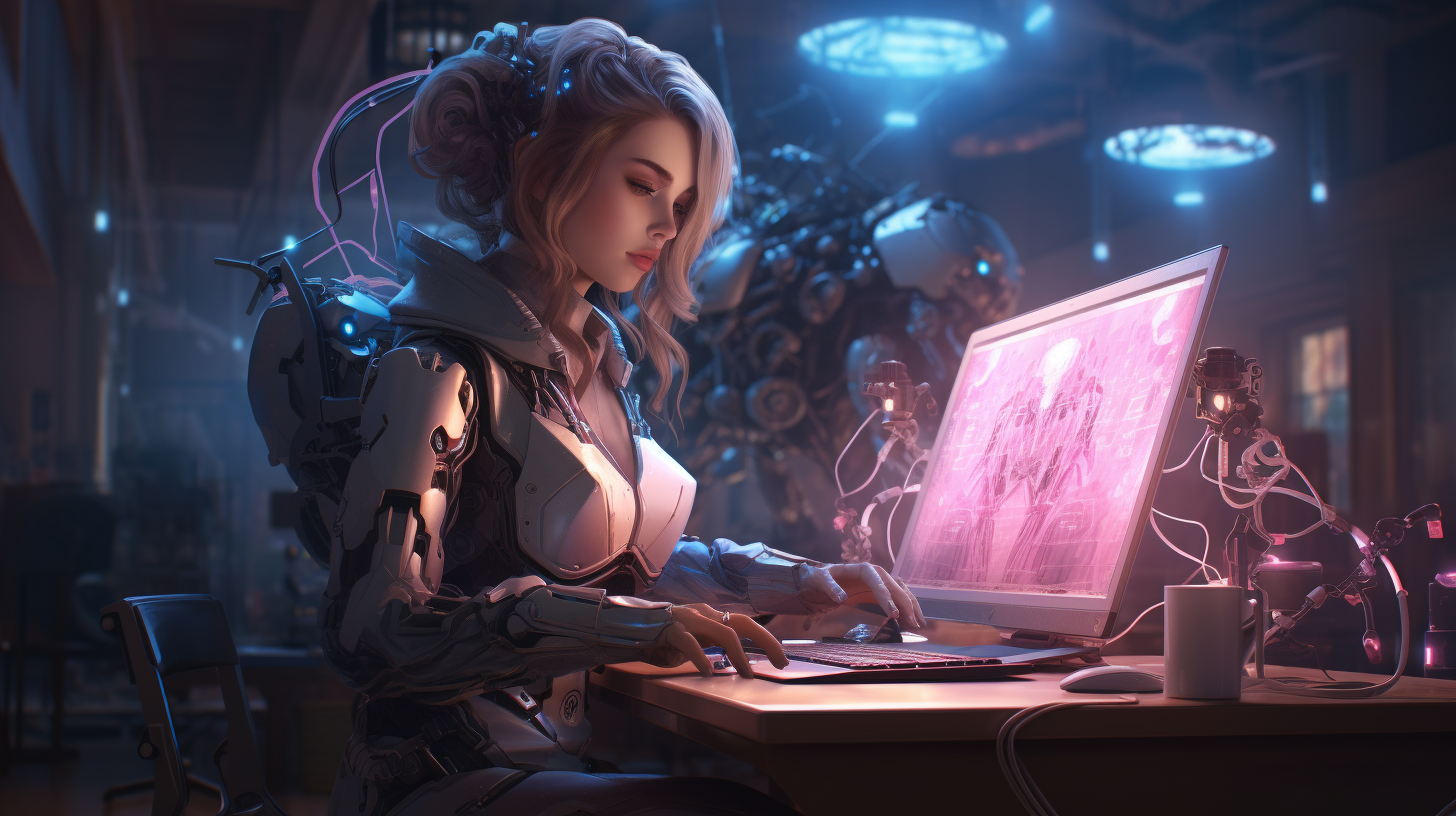
Java Annotations: Overview and Types
Java annotations are a powerful feature of the Java programming language, enabling developers to add metadata to their code without altering its actual functionality. This metadata can be processed by the compiler or at runtime, allowing for more flexible and dynamic programming paradigms. At their core, annotations serve as a mechanism to provide information about the program to the compiler, development tools, frameworks, and even other developers.
Annotations in Java are defined using the @interface
keyword, which allows for the creation of custom annotations. These annotations can then be applied to various program elements such as classes, methods, variables, and parameters. By using annotations, developers can specify behaviors or configurations that can be leveraged by frameworks such as Spring, Hibernate, or JUnit.
One of the main advantages of using annotations is the ability to separate configuration from code. This separation allows developers to write cleaner and more maintainable code by keeping the configuration in one place, thereby avoiding cluttering the actual business logic with configuration details. Annotations also promote readability, as they provide a clear indication of the purpose and behavior of the annotated elements.
Think the following example of a simple custom annotation:
import java.lang.annotation.ElementType; import java.lang.annotation.Retention; import java.lang.annotation.RetentionPolicy; import java.lang.annotation.Target; @Retention(RetentionPolicy.RUNTIME) @Target(ElementType.METHOD) public @interface MyCustomAnnotation { String value(); }
In this snippet, we define an annotation called MyCustomAnnotation
. The Retention
annotation specifies that this annotation should be available at runtime, while the Target
annotation indicates that it can only be applied to methods.
Once defined, this annotation can be applied to any method in your code, so that you can add descriptive metadata:
public class Example { @MyCustomAnnotation(value = "This method does something important") public void importantMethod() { // Method implementation } }
Annotations can also have attributes, as seen in the MyCustomAnnotation
example. This allows the annotation to carry additional information that can be accessed when the annotation is processed. The flexibility of annotations enables developers to create more expressive and informative APIs.
Java annotations are an essential part of the language that provide a clean and effective way to add metadata to code elements. They enhance the capabilities of the Java programming language by allowing developers to define behavior in a declarative manner, promoting clean code practices and greater maintainability.
Standard Annotations in Java
In Java, several standard annotations are provided out-of-the-box, each serving specific purposes that enhance the functionality and usability of the language. These annotations are part of the Java Standard Library and are widely used in various frameworks and libraries to provide functionality such as dependency injection, transaction management, and unit testing. Here, we will explore some of the most commonly used standard annotations and their significance.
1. @Override
The @Override annotation indicates that a method is intended to override a method in a superclass. This annotation not only serves as documentation but also enables the compiler to generate an error if the method does not actually override a method from the parent class. This helps catch potential bugs early in the development process.
class Parent { public void display() { System.out.println("Parent display"); } } class Child extends Parent { @Override public void display() { System.out.println("Child display"); } }
In this example, if the @Override annotation were omitted and the method signature in Child did not match that in Parent, the compiler would not produce an error, potentially leading to unintended behavior.
2. @Deprecated
The @Deprecated annotation marks a method or class as obsolete, suggesting that it should no longer be used. This can help developers transition away from outdated code by signaling that an alternative exists or that the current implementation may be removed in future versions.
class LegacyClass { @Deprecated public void oldMethod() { // Old implementation } }
In this case, any call to oldMethod() will generate a warning, prompting developers to find a newer, preferred method.
3. @SuppressWarnings
This annotation tells the compiler to suppress specific warnings that may be generated during compilation. For instance, if you’re aware of a potential issue but have deemed it safe to ignore, you can use @SuppressWarnings to prevent compiler warnings from cluttering your output.
class WarningExample { @SuppressWarnings("unchecked") public void uncheckedConversion() { List rawList = new ArrayList(); List stringList = rawList; // Warning suppressed } }
In this snippet, the unchecked warning is suppressed, allowing the developer to maintain code that may otherwise raise concerns.
4. @FunctionalInterface
The @FunctionalInterface annotation is used to indicate that an interface is intended to be a functional interface, meaning it contains exactly one abstract method. This annotation serves as a compile-time check and enhances code clarity when using lambdas or method references.
@FunctionalInterface interface MyFunctionalInterface { void performAction(); }
By marking the interface with @FunctionalInterface, the compiler ensures that it adheres to the functional interface contract.
5. @Entity and JPA Annotations
In the context of Java Persistence API (JPA), annotations like @Entity, @Table, and @Column define how objects are mapped to database tables. Annotations like @Entity indicate that a class is an entity bean, while @Table allows the specification of the database table associated with the entity.
import javax.persistence.Entity; import javax.persistence.Table; @Entity @Table(name = "user") public class User { private String username; private String password; }
In this example, the User class is mapped to a database table named ‘user’, facilitating seamless database interactions.
Standard annotations in Java play an important role in enhancing code clarity, maintainability, and functionality. By using these annotations effectively, developers can streamline their code, reduce errors, and leverage the extensive capabilities of the Java ecosystem.
Custom Annotations: Creating Your Own
Creating custom annotations in Java is an essential skill for developers looking to extend the functionality of their applications and frameworks. The process involves defining an annotation with specific attributes and applying it to various code elements. This permits the addition of metadata that can be processed at compile time or runtime, enabling developers to achieve a more dynamic and flexible codebase.
When designing a custom annotation, it’s crucial to think its intended use and the elements to which it can be applied. Annotations can be utilized on classes, methods, fields, parameters, and other program elements. To define an annotation, you use the @interface
keyword. Below is an example of a custom annotation that can be applied to classes and methods:
import java.lang.annotation.ElementType; import java.lang.annotation.Retention; import java.lang.annotation.RetentionPolicy; import java.lang.annotation.Target; @Retention(RetentionPolicy.RUNTIME) @Target({ElementType.TYPE, ElementType.METHOD}) public @interface MyCustomAnnotation { String description(); int version() default 1; }
In this example, we define MyCustomAnnotation
, which includes two attributes: description
and version
. The description
is a required attribute, while version
has a default value of 1. The Retention
annotation specifies that it’s available at runtime, and the Target
annotation allows it to be applied to both classes and methods.
Applying this annotation to a class and a method would look like this:
@MyCustomAnnotation(description = "This is a sample class.") public class SampleClass { @MyCustomAnnotation(description = "This method performs an action.", version = 2) public void performAction() { // Method implementation } }
After defining and applying custom annotations, the next step is to process them. This typically involves using Reflection to access the annotations at runtime. By using Reflection, you can retrieve metadata from the annotations and implement logic based on that data. Here’s how you can retrieve and use the annotation data:
import java.lang.reflect.Method; public class AnnotationProcessor { public static void main(String[] args) throws Exception { Class<?> clazz = SampleClass.class; if (clazz.isAnnotationPresent(MyCustomAnnotation.class)) { MyCustomAnnotation annotation = clazz.getAnnotation(MyCustomAnnotation.class); System.out.println("Class Description: " + annotation.description()); } for (Method method : clazz.getDeclaredMethods()) { if (method.isAnnotationPresent(MyCustomAnnotation.class)) { MyCustomAnnotation annotation = method.getAnnotation(MyCustomAnnotation.class); System.out.println("Method: " + method.getName() + ", Description: " + annotation.description() + ", Version: " + annotation.version()); } } } }
In this AnnotationProcessor
example, we check if SampleClass
is annotated with MyCustomAnnotation
. If it’s, we retrieve the annotation and print its description. The same process is repeated for each method in the class, allowing us to access the custom metadata defined in the annotations. This approach illustrates the power of custom annotations, as they can significantly improve the way you manage configurations and behaviors in your codebase.
Custom annotations can streamline your code, enhance readability, and enable advanced functionalities within various frameworks. By understanding how to create and process these annotations, you can harness their full potential in your Java applications.
Retention Policies and Target Elements
Retention policies and target elements are fundamental aspects of Java annotations that dictate how and where annotations can be applied, as well as their lifecycle within the application. Understanding these concepts is important for effectively using annotations in your Java projects.
Java provides three main retention policies that determine how long annotations are retained and accessible:
import java.lang.annotation.Retention; import java.lang.annotation.RetentionPolicy; @Retention(RetentionPolicy.SOURCE) public @interface SourceRetentionAnnotation { } @Retention(RetentionPolicy.CLASS) public @interface ClassRetentionAnnotation { } @Retention(RetentionPolicy.RUNTIME) public @interface RuntimeRetentionAnnotation { }
1. RetentionPolicy.SOURCE: Annotations with this retention policy are discarded by the compiler and are not included in the bytecode. They are typically used for annotations that are meant only for development-time tools and do not need to be present at runtime.
2. RetentionPolicy.CLASS: Annotations marked with this policy are recorded in the class file but are not retained at runtime. This means they are available for use by tools and frameworks at compile time and can be accessed during the compilation process, but will not be available for reflection when the program is running.
3. RetentionPolicy.RUNTIME: Annotations annotated with this retention policy are retained in the runtime environment. They can be accessed through reflection, allowing developers to read annotation attributes during execution. That’s the most useful retention policy for annotations that serve a runtime purpose.
Next, the Target annotation specifies the kinds of Java elements to which an annotation type is applicable. Here are some common targets:
import java.lang.annotation.ElementType; import java.lang.annotation.Target; @Target(ElementType.TYPE) public @interface TypeTargetAnnotation { } @Target(ElementType.METHOD) public @interface MethodTargetAnnotation { } @Target(ElementType.FIELD) public @interface FieldTargetAnnotation { } @Target(ElementType.PARAMETER) public @interface ParameterTargetAnnotation { } @Target({ElementType.TYPE, ElementType.METHOD}) public @interface MultiTargetAnnotation { }
1. ElementType.TYPE: This target indicates that the annotation can be applied to classes, interfaces, or enums.
2. ElementType.METHOD: This target allows the annotation to be applied to methods. It is useful for annotating methods with behavior-related metadata.
3. ElementType.FIELD: This target is used for annotations applied to member variables (fields) within a class.
4. ElementType.PARAMETER: Annotations with this target can be applied to parameters in method declarations.
5. Multi-target: By providing an array of targets, annotations can be applied to multiple elements. For example, a single annotation could be used on both classes and methods.
Understanding how to set retention policies and target elements effectively allows you to leverage annotations to their fullest potential. When designing annotations, think their purpose and the appropriate retention policy and targets. This will ensure that your annotations are not only correctly applied but also function as intended throughout the lifecycle of your application.
Practical Use Cases for Java Annotations
Java annotations serve an essential role in modern software development, with practical applications that extend far beyond mere metadata tagging. They allow developers to implement cross-cutting concerns, reduce boilerplate code, and enhance program behavior dynamically. Let’s explore some of the practical use cases where Java annotations shine.
One notable area where annotations are heavily utilized is in the context of frameworks. For instance, in the Spring Framework, annotations like @Autowired
, @Controller
, and @Service
simplify dependency injection and the configuration of beans. With these annotations, developers can configure their applications without requiring extensive XML configuration files, making the codebase more readable and maintainable.
import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.GetMapping; @Controller public class HomeController { @GetMapping("/") public String home() { return "home"; } }
In this example, the @Controller
annotation marks the class as a Spring MVC controller, while the @GetMapping
annotation maps HTTP GET requests to the home()
method. This declarative approach allows for cleaner routing and clearer intent compared to traditional routing mechanisms.
Annotations also play a significant role in validation frameworks. For instance, the Java Bean Validation API utilizes annotations like @NotNull
, @Size
, and @Email
to enforce validation rules directly on the model class fields. This allows developers to keep validation logic close to the data it validates, thus improving code cohesion and reducing redundancy.
import javax.validation.constraints.Email; import javax.validation.constraints.NotNull; import javax.validation.constraints.Size; public class User { @NotNull @Size(min = 2, max = 30) private String username; @NotNull @Email private String email; // Getters and setters }
In this snippet, the @NotNull
annotation ensures that the username
cannot be null, while @Size
restricts its length. The @Email
annotation guarantees that the email
field contains a valid email format. These constraints can be automatically validated using a validation framework, significantly reducing the need for manual checks and enhancing overall application reliability.
Another compelling use case for annotations is in the context of testing. Frameworks such as JUnit leverage annotations like @Test
, @Before
, and @After
to define test cases and setup/teardown procedures. This approach allows for clear and simple test definitions, streamlining the testing process while improving readability.
import org.junit.Before; import org.junit.Test; import static org.junit.Assert.*; public class CalculatorTest { private Calculator calculator; @Before public void setUp() { calculator = new Calculator(); } @Test public void testAddition() { assertEquals(5, calculator.add(2, 3)); } }
In the example above, the @Before
annotation indicates that the setUp()
method should run before each test, ensuring a fresh instance of Calculator
for every test case. The @Test
annotation marks a method as a test, allowing JUnit to execute it and report the results.
Annotations can also be instrumental in aspect-oriented programming (AOP), where they are used to define cross-cutting concerns such as logging, security, and transaction management. By annotating methods or classes with custom annotations like @Transactional
, developers can declaratively control transactions without scattering transactional logic throughout the codebase.
import org.springframework.transaction.annotation.Transactional; public class UserService { @Transactional public void createUser(User user) { // Logic to create a user } }
Here, the @Transactional
annotation indicates that the createUser()
method should be executed within a transactional context, encompassing all database operations within a single transaction. This encapsulation greatly simplifies error handling and rollback procedures.
Overall, Java annotations provide a robust mechanism for enhancing code functionality, improving maintainability, and fostering clearer intent within your applications. By using their capabilities in frameworks, validation, testing, and aspect-oriented programming, developers can create more efficient, readable, and manageable codebases. The possibilities are vast, limited only by your creativity and the requirements of your project.