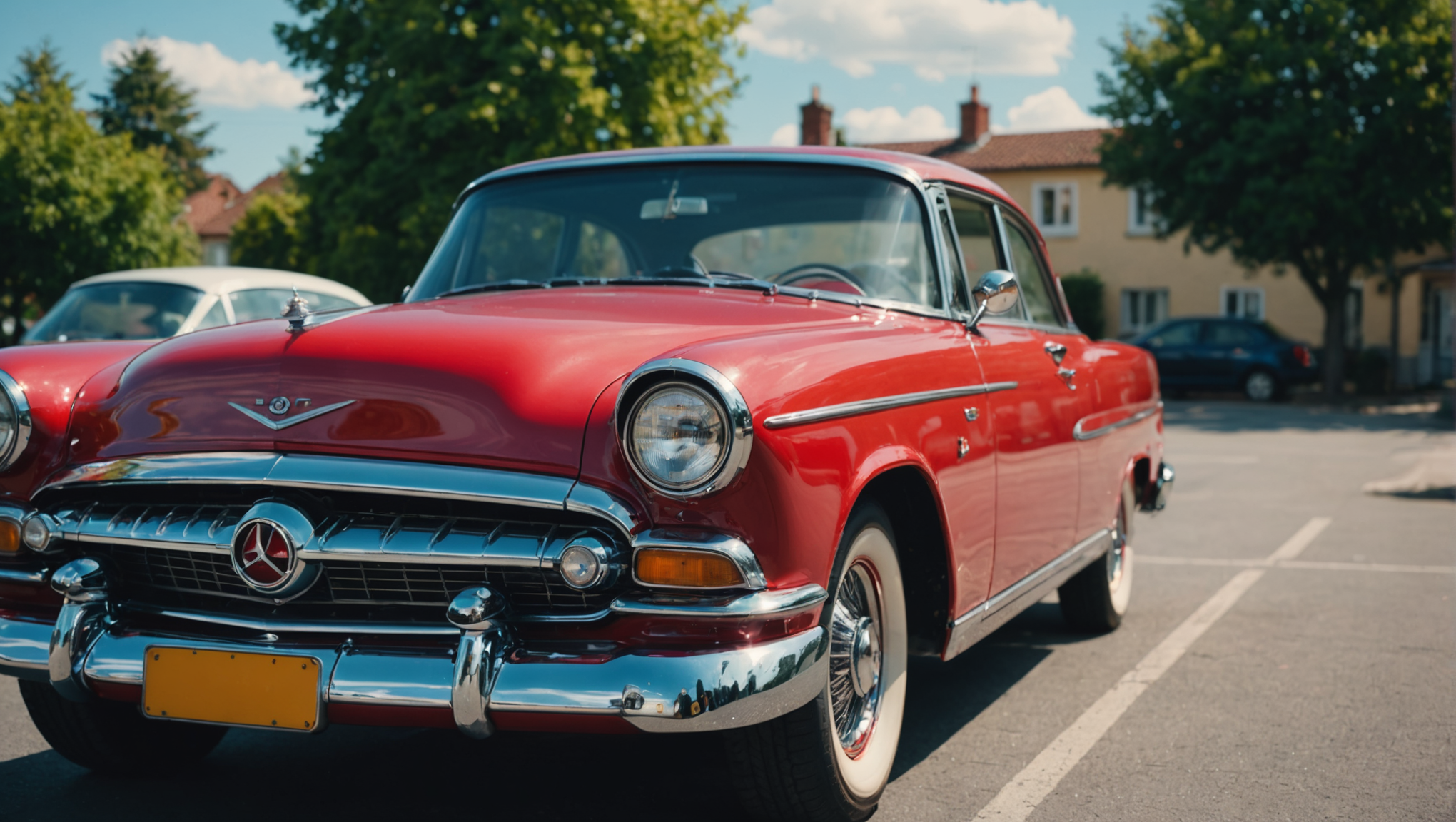
Java Data Types Explained
In Java, primitive data types are the building blocks of data manipulation. They’re predefined by the language and serve as the most basic form of data representation. Java defines eight primitive data types, each with its own size and range, which plays an important role in how data is stored and how operations are performed on it.
1. byte: A byte is an 8-bit signed integer. It has a minimum value of -128 and a maximum value of 127. It’s particularly useful for saving memory in large arrays, where the memory savings are critical.
byte b = 100;
2. short: A short is a 16-bit signed integer. Its range is from -32,768 to 32,767. Like byte, short is utilized for memory-efficient arrays.
short s = 15000;
3. int: An int is a 32-bit signed integer, which is the default data type for integral values. Its value ranges from -2,147,483,648 to 2,147,483,647. This data type is widely used in scenarios where whole numbers are required.
int i = 123456;
4. long: A long is a 64-bit signed integer, allowing for a much larger range of values than an int, from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807. It’s used when you need a wider range than int can provide.
long l = 123456789012345L;
5. float: A float is a single-precision 32-bit IEEE 754 floating point. It’s used for saving memory in large arrays of floating point numbers. However, it’s important to remember that it may not provide the precision needed for certain calculations.
float f = 19.99f;
6. double: A double is a double-precision 64-bit IEEE 754 floating point. It is the default choice for decimal values in Java due to its precision and range. The range is approximately ±4.9 × 10-324 to ±1.8 × 10308.
double d = 19.99;
7. char: A char is a single 16-bit Unicode character. It can represent any character from the Unicode character set, making it versatile for internationalization.
char c = 'A';
8. boolean: A boolean data type has only two possible values: true and false. It is used for simple flags that track true/false conditions, especially in control flow statements.
boolean isJavaFun = true;
Understanding these primitive data types is vital, as they form the foundation of data representation in Java applications. Each type has its use case and storage requirements, and knowing when to use which type can significantly affect both performance and memory consumption in your Java programs.
Reference Data Types and Objects
While primitive data types are fundamental to Java, reference data types and objects elevate the language to a higher level of abstraction, allowing for more complex data structures. Reference data types, unlike primitives, do not hold the actual data value but rather a reference or pointer to an object that contains the data. This distinction especially important in understanding how Java manages memory and processes data.
In Java, reference data types encompass all objects, including arrays, strings, and user-defined classes. When you declare a reference variable, it essentially creates a reference to an object stored in the heap memory. This means that the variable itself does not contain the object; instead, it holds the address of the memory location where the object is stored. Here’s a simple example:
String greeting = "Hello, World!";
In the example above, the variable greeting
is a reference type that points to a String object containing “Hello, World!”. It’s important to note that when you assign one reference variable to another, you’re not creating a new object, but merely copying the reference. Therefore, changes made through one reference will reflect in the other:
String original = "Hello"; String reference = original; reference = "World"; System.out.println(original); // Output: Hello
Here, although reference
initially pointed to the same String object as original
, when we reassign reference
to “World”, it points to a new String object, leaving original
unchanged.
Java also employs classes and objects to create more complex data types. A class is essentially a blueprint for creating objects, encapsulating both data and methods that operate on that data. For example:
class Car { String color; String model; void displayDetails() { System.out.println("Car model: " + model); System.out.println("Car color: " + color); } } Car myCar = new Car(); myCar.color = "Red"; myCar.model = "Toyota"; myCar.displayDetails();
In this code snippet, we define a class Car
with properties color
and model
, along with a method displayDetails
to showcase the car’s details. When we instantiate myCar
, it becomes an object of type Car
, showcasing how reference types allow for modeling real-world entities and behavior in code.
Arrays in Java are another vital aspect of reference data types. They can store multiple values of the same type in a single variable, significantly simplifying data management. An array is also an object in Java, which means it is stored in the heap memory:
int[] numbers = {1, 2, 3, 4, 5}; System.out.println(numbers[0]); // Output: 1
In this example, numbers
is a reference to an array object that holds integers. Accessing its elements is done with the index, starting from 0. This ability to manage collections of data through reference types leads to easier data manipulation and organization.
Understanding reference data types and objects is essential for Java developers looking to build sophisticated applications. They enable the representation of complex data structures, promote code modularity through classes, and facilitate efficient data handling with arrays. Mastery of these concepts will empower you to write more effective and scalable Java code.
Type Casting and Conversion
In Java, type casting is the process of converting a variable from one data type to another. It becomes necessary when you want to perform operations on mixed data types or when you want to store a value of one type in a variable of another type. Type casting is divided into two main categories: implicit casting (or widening) and explicit casting (or narrowing).
Implicit Casting (Widening): This type of casting happens automatically when you assign a smaller type to a larger type without any data loss. For instance, if you convert an int
to a double
, Java does this automatically, as a double
can hold all possible values of an int
.
int intValue = 100; double doubleValue = intValue; // Implicit casting System.out.println(doubleValue); // Output: 100.0
In the code above, the integer value of 100
is implicitly converted into a double without any need for special syntax.
Explicit Casting (Narrowing): This type of casting requires a manual conversion as it may lead to data loss. When you convert a larger primitive type to a smaller one, you must explicitly specify the target type in parentheses.
double doubleValue = 100.99; int intValue = (int) doubleValue; // Explicit casting System.out.println(intValue); // Output: 100
In the example above, the double
value 100.99
is explicitly cast to an int
. This results in the loss of the decimal part, demonstrating why explicit casting should be approached with caution.
Type casting also applies to reference types. In Java, you can cast an object reference to a different type within the same inheritance hierarchy. That is referred to as downcasting and can be done safely with an instance check using the instanceof
operator. Consider the following example:
class Animal { void sound() { System.out.println("Animal makes sound"); } } class Dog extends Animal { void sound() { System.out.println("Dog barks"); } } Animal myAnimal = new Dog(); // Upcasting myAnimal.sound(); // Output: Dog barks if (myAnimal instanceof Dog) { Dog myDog = (Dog) myAnimal; // Downcasting myDog.sound(); // Output: Dog barks }
In this example, Animal
is the superclass of Dog
. The myAnimal
reference is initially an instance of Dog
cast to Animal
(upcasting). When we perform a downcast using (Dog) myAnimal
, we can access Dog
‘s specific methods safely after checking the type with instanceof
.
Type conversion also plays a significant role when dealing with strings. Java provides several methods to convert strings to various primitive data types, such as Integer.parseInt()
for converting a string to an int or Double.parseDouble()
for a double. Here’s an example:
String numberString = "12345"; int number = Integer.parseInt(numberString); // String to int System.out.println(number); // Output: 12345 String doubleString = "123.45"; double dNumber = Double.parseDouble(doubleString); // String to double System.out.println(dNumber); // Output: 123.45
However, be cautious when converting strings that may not represent a valid number, as it will throw a NumberFormatException
. Proper exception handling should be employed to avoid runtime errors during such conversions.
Understanding type casting and conversion is critical for Java developers, as it enables effective data manipulation across different data types, optimizes memory usage, and allows for seamless interactions between objects in an inheritance hierarchy. Mastering these concepts empowers you to write robust and flexible Java applications.
Wrapper Classes and Autoboxing
Wrapper classes in Java provide a way to use primitive data types as objects. Each of the eight primitive data types has a corresponding wrapper class: Byte, Short, Integer, Long, Float, Double, Character, and Boolean. The purpose of these wrapper classes is to encapsulate the primitive values in an object, allowing for greater manipulation and using the features of Java’s object-oriented capabilities.
One of the key benefits of using wrapper classes is that they provide methods to convert between different data types, perform operations, and even manipulate the values contained within them. For instance, the Integer class offers static methods for converting strings to integers and vice versa:
String numberString = "123"; int number = Integer.parseInt(numberString); // String to int System.out.println(number); // Output: 123 String backToString = Integer.toString(number); // int to String System.out.println(backToString); // Output: "123"
These conversions are fundamental in contexts where you may receive data in string format, such as user input or data from files, and need to work with these values as integers.
Another aspect of wrapper classes is that they provide a way to utilize primitive types in Java Collections. For example, you can’t directly store primitive types in data structures like ArrayList. Instead, you need to use their corresponding wrapper classes:
import java.util.ArrayList; ArrayList<Integer> integerList = new ArrayList<>(); integerList.add(10); // Autoboxing converts int to Integer integerList.add(20); integerList.add(30); for (Integer value : integerList) { System.out.println(value); // Output: 10, 20, 30 }
In the example above, when adding an integer to the ArrayList, Java automatically converts the primitive int to an Integer object. This feature is known as autoboxing, which Java performs automatically to bridge the gap between primitive types and their corresponding wrapper classes.
Conversely, when retrieving an object from a collection and assigning it to a primitive type variable, Java performs unboxing. That’s also done automatically:
int firstValue = integerList.get(0); // Unboxing converts Integer back to int System.out.println(firstValue); // Output: 10
However, keep in mind that reliance on wrapper classes can introduce performance overhead due to the creation of objects, especially in scenarios where primitive types are preferred for performance reasons, such as high-performance computing applications.
Wrapper classes also introduce the idea of nullability. While primitive types cannot represent the absence of a value, wrapper classes can be set to null, allowing for more flexible data structures. This can be particularly useful in databases or when indicating optional values:
Integer nullableInteger = null; if (nullableInteger == null) { System.out.println("No value assigned!"); // Output: No value assigned! }
Wrapper classes in Java provide essential functionality by enabling the use of primitive data types as objects, facilitating operations like type conversion and manipulation within collections. The seamless integration of autoboxing and unboxing enhances the flexibility of Java programming, allowing for more complex data structures while still retaining the simplicity of primitive types.