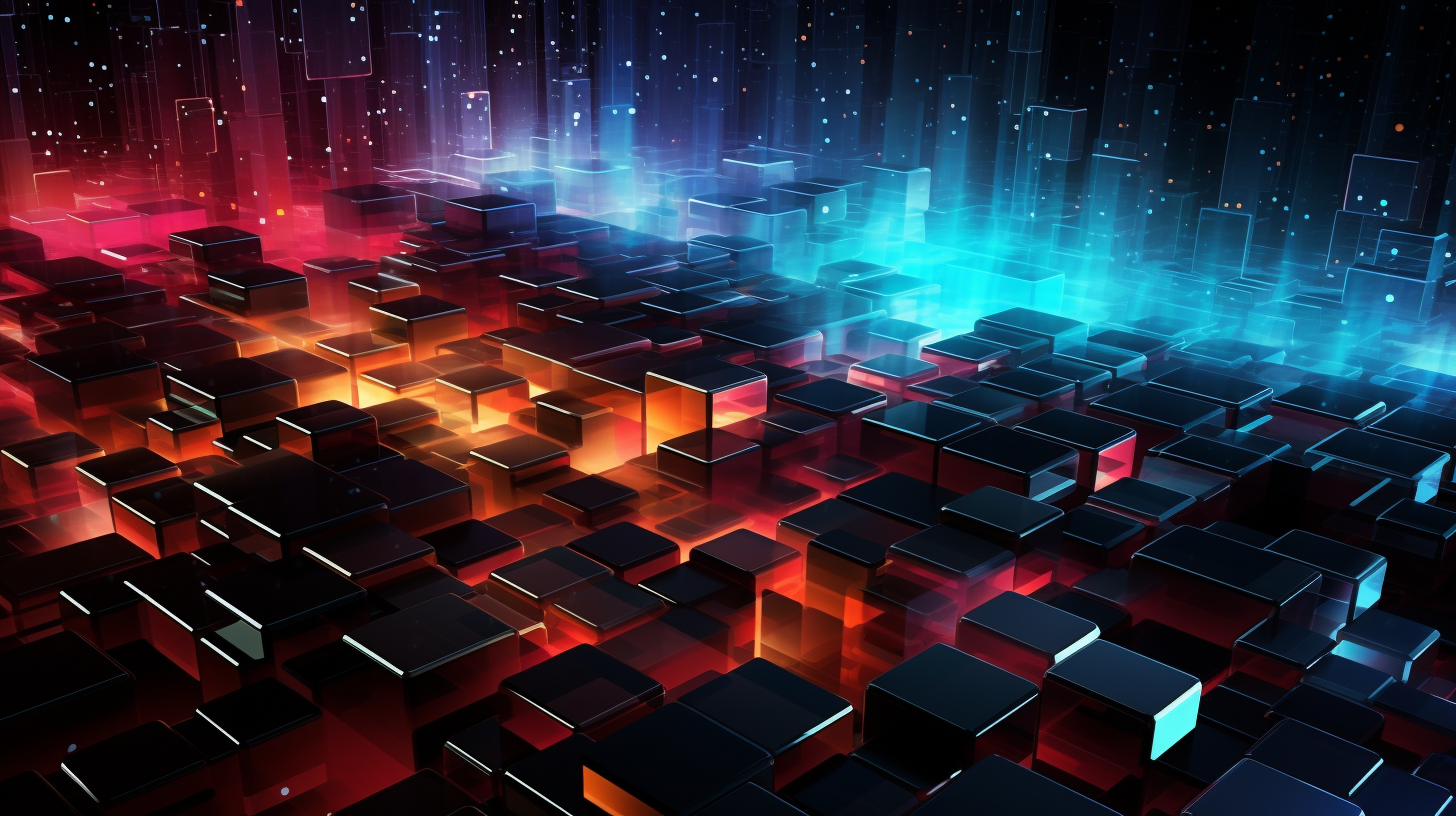
Java Design Patterns: Singleton, Factory, and More
Java design patterns are established solutions to common software design problems. They provide a blueprint for solving issues that arise frequently in software development, allowing developers to communicate using a standard vocabulary. Each design pattern encapsulates a specific design approach, which can lead to more efficient, maintainable, and scalable code.
Design patterns can be categorized into three primary types: creational, structural, and behavioral.
Creational patterns deal with object creation mechanisms. These patterns allow for greater flexibility in instantiating objects, which can lead to more reusable code. Some common creational patterns include:
- Ensures a class has only one instance and provides a global point of access to it.
- Defines an interface for creating an object, but lets subclasses alter the type of objects that will be created.
- Provides an interface for creating families of related or dependent objects without specifying their concrete classes.
Structural patterns focus on how classes and objects are composed to form larger structures. They help ensure that if one part of a system changes, the entire system doesn’t need to do the same. Examples include:
- Allows incompatible interfaces to work together by converting the interface of a class into another interface clients expect.
- Adds additional responsibilities to an object dynamically and provides a flexible alternative to subclassing for extending functionality.
- Provides a simplified interface to a complex system of classes, making it easier for clients to interact with the system.
Behavioral patterns are concerned with algorithms and the assignment of responsibilities between objects. They help define how objects interact and communicate with one another. Notable behavioral patterns include:
- Defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically.
- Enables selecting an algorithm’s behavior at runtime; it defines a family of algorithms, encapsulates each one, and makes them interchangeable.
- Encapsulates a request as an object, thereby allowing for parameterization of clients with queues, requests, and operations.
By using these design patterns, Java developers can create systems that are not only easier to understand and maintain but also promote code reuse and reduce the risk of introducing bugs during later enhancements.
To illustrate the Singleton pattern, here’s a simple Java implementation:
public class Singleton { private static Singleton instance; private Singleton() { } public static synchronized Singleton getInstance() { if (instance == null) { instance = new Singleton(); } return instance; } }
In this example, the Singleton
class restricts instantiation through a private constructor and provides a global access point via the getInstance
method. This ensures that only one instance of the class is created, adhering to the Singleton design pattern.
Understanding the Singleton Pattern
The Singleton pattern is particularly useful in scenarios where exactly one object is needed to coordinate actions across the system. This restriction can be beneficial in various situations, such as managing shared resources like databases or configurations. When implementing the Singleton pattern, one must also consider thread safety, especially in concurrent applications where multiple threads might attempt to create an instance simultaneously.
In the initial implementation, the synchronization on the getInstance method ensures that only one thread can access it at a time, preventing multiple instances from being created. However, this approach can lead to performance bottlenecks due to the overhead of synchronization during every call to getInstance.
To enhance performance while maintaining thread safety, one can use the Double-Checked Locking pattern. In this variant, synchronization is performed only when the instance is null, thereby reducing the overhead of acquiring a lock. Below is an implementation using this approach:
public class Singleton { private static volatile Singleton instance; private Singleton() { } public static Singleton getInstance() { if (instance == null) { synchronized (Singleton.class) { if (instance == null) { instance = new Singleton(); } } } return instance; } }
In this example, the getInstance method first checks if the instance is null before entering the synchronized block. This check allows multiple threads to access the method without waiting for the lock if the instance has already been created, thus improving efficiency.
Another approach to implementing the Singleton pattern is through the use of an eagerly initialized instance. This method creates the instance at the time of class loading, ensuring that it’s available for use without the need for synchronization:
public class Singleton { private static final Singleton instance = new Singleton(); private Singleton() { } public static Singleton getInstance() { return instance; } }
While this approach is simple and thread-safe, it comes with a caveat: the instance is created even if it may never be used, which can lead to wasted resources in some scenarios. Therefore, the choice between lazy initialization (with synchronization) and eager initialization depends on the specific requirements of your application.
Understanding and implementing the Singleton pattern effectively can greatly enhance the design of a Java application. By ensuring that a class has only one instance and provides a controlled access point, developers can manage resources efficiently and maintain a clean architecture.
Implementing the Factory Pattern
The Factory Pattern is a creational design pattern used to create objects without specifying the exact class of the object that will be created. It provides a way to encapsulate the instantiation logic and can help in making the code more flexible and easier to manage. In scenarios where a class cannot anticipate the type of objects it needs to create, the Factory Pattern becomes invaluable.
At its core, the Factory Pattern involves defining an interface or an abstract class for creating an object, and then allowing subclasses or specific implementations to decide which class to instantiate. This approach allows the code that uses these objects to remain decoupled from the concrete classes, promoting adherence to the Dependency Inversion Principle.
Let’s take a look at a simple example of the Factory Method Pattern. First, we’ll define a common interface for our products:
public interface Product { void use(); }
Next, we can create concrete implementations of this interface:
public class ConcreteProductA implements Product { @Override public void use() { System.out.println("Using Product A"); } } public class ConcreteProductB implements Product { @Override public void use() { System.out.println("Using Product B"); } }
Now, we can define the factory that will create these products:
public abstract class Creator { public abstract Product factoryMethod(); public void someOperation() { Product product = factoryMethod(); product.use(); } } public class ConcreteCreatorA extends Creator { @Override public Product factoryMethod() { return new ConcreteProductA(); } } public class ConcreteCreatorB extends Creator { @Override public Product factoryMethod() { return new ConcreteProductB(); } }
In this example, the Creator
class defines the factoryMethod
this is responsible for creating products. The concrete creators, ConcreteCreatorA
and ConcreteCreatorB
, implement this method to return specific product instances. This structure allows for easy extension; if a new product is needed, a new creator class can be added without modifying the existing code.
To use these classes, one can simply do the following:
public class Client { public static void main(String[] args) { Creator creatorA = new ConcreteCreatorA(); creatorA.someOperation(); // Outputs: Using Product A Creator creatorB = new ConcreteCreatorB(); creatorB.someOperation(); // Outputs: Using Product B } }
This example demonstrates how the Factory Pattern simplifies object creation and allows for flexibility in handling different types of products. By using factories, you can adhere to the principle of programming to an interface, not an implementation, leading to cleaner and more maintainable code.
Furthermore, the Factory Pattern can be extended to the Abstract Factory Pattern, which allows for the creation of families of related or dependent objects without specifying their concrete classes. That is particularly useful in scenarios where products must be created that are interdependent, as it enforces a consistent product creation process across multiple product types.
The Factory Pattern is an essential part of the Java developer’s toolkit, enabling more modular and flexible code through abstraction. By appropriately implementing this pattern, developers can ensure that their applications are more adaptable to change, easier to understand, and more efficient in resource management.
Exploring the Observer Pattern
The Observer Pattern is a behavioral design pattern that establishes a one-to-many dependency between objects. This means that when one object changes its state, all its dependents are notified and updated automatically. In scenarios where a subject’s state is of interest to multiple observers, the Observer Pattern provides an elegant solution. The classic example of this pattern can be found in user interfaces, where changes in data should be reflected immediately in the UI components that display that data.
At its core, the Observer Pattern involves two main components: the Subject and the Observer. The Subject maintains a list of its observers and provides methods to attach, detach, and notify them. Observers implement an interface that defines the update method, which is called by the Subject when its state changes.
Let’s implement this pattern with a simple example where a weather station (Subject) notifies various display elements (Observers) about temperature changes:
import java.util.ArrayList; import java.util.List; // Subject interface interface Subject { void attach(Observer observer); void detach(Observer observer); void notifyObservers(); } // Observer interface interface Observer { void update(float temperature); } // Concrete Subject class WeatherStation implements Subject { private List observers = new ArrayList(); private float temperature; public void setTemperature(float temperature) { this.temperature = temperature; notifyObservers(); } @Override public void attach(Observer observer) { observers.add(observer); } @Override public void detach(Observer observer) { observers.remove(observer); } @Override public void notifyObservers() { for (Observer observer : observers) { observer.update(temperature); } } } // Concrete Observer class TemperatureDisplay implements Observer { @Override public void update(float temperature) { System.out.println("Current temperature: " + temperature + "°C"); } } // Concrete Observer class WeatherAlert implements Observer { @Override public void update(float temperature) { if (temperature > 30.0) { System.out.println("Weather Alert: High temperature warning!"); } } } // Client Code public class Client { public static void main(String[] args) { WeatherStation weatherStation = new WeatherStation(); TemperatureDisplay display = new TemperatureDisplay(); WeatherAlert alert = new WeatherAlert(); weatherStation.attach(display); weatherStation.attach(alert); weatherStation.setTemperature(28.5f); // Outputs: Current temperature: 28.5°C weatherStation.setTemperature(31.0f); // Outputs: Current temperature: 31.0°C & Weather Alert: High temperature warning! } }
In this implementation, the WeatherStation
class acts as the Subject which maintains a list of registered observers. When the temperature is updated using the setTemperature
method, it notifies all attached observers by calling their update
method. The TemperatureDisplay
and WeatherAlert
classes serve as concrete observers, each defining its response to updates from the weather station.
The Observer Pattern is particularly beneficial in scenarios where the state of one object must be reflected in multiple other objects. By decoupling the Subject and Observer, it fosters a more flexible architecture, allowing observers to be added or removed dynamically without altering the Subject’s code. This leads to better maintainability and adherence to the Open/Closed Principle, as new observers can be introduced without modifying existing code.
However, care should be taken when implementing the Observer Pattern, as it can lead to memory leaks if observers are not properly removed. Additionally, if an Observer has a long-running process, it might delay the notification of other observers. To mitigate such issues, developers should ponder implementing asynchronous notifications or introducing a queue mechanism for observer updates.
The Observer Pattern provides a robust framework for managing state changes and propagating notifications efficiently. By using this pattern, developers can create responsive systems that react to changes in real-time, enhancing user experience and system interactivity.
The Strategy Pattern in Action
The Strategy Pattern is a behavioral design pattern that enables a client to choose an algorithm from a family of algorithms at runtime. It encapsulates these algorithms into separate classes, allowing them to be interchangeable without altering the client code. This separation promotes flexibility and adherence to the Open/Closed Principle, making it easier to extend the application with new algorithms without modifying existing code.
One of the primary use cases of the Strategy Pattern is when a class has multiple methods of performing a specific operation, and the exact method to be used can vary based on the situation. By defining a common interface for these algorithms, the Strategy Pattern allows a class to delegate the behavior to the strategy object, which can be changed dynamically.
Let’s take a closer look at an example of the Strategy Pattern. Suppose we have a context that can perform sorting. We will define a sorting strategy interface and implement various sorting algorithms:
// Strategy interface interface SortStrategy { void sort(int[] array); } // Concrete strategy for Bubble Sort class BubbleSort implements SortStrategy { @Override public void sort(int[] array) { int n = array.length; for (int i = 0; i < n - 1; i++) { for (int j = 0; j < n - i - 1; j++) { if (array[j] > array[j + 1]) { // Swap array[j] and array[j + 1] int temp = array[j]; array[j] = array[j + 1]; array[j + 1] = temp; } } } } } // Concrete strategy for Quick Sort class QuickSort implements SortStrategy { @Override public void sort(int[] array) { quickSort(array, 0, array.length - 1); } private void quickSort(int[] array, int low, int high) { if (low > high) { return; } int pivot = array[low]; int left = low + 1; int right = high; while (true) { while (left <= right && array[left] <= pivot) { left++; } while (left <= right && array[right] >= pivot) { right--; } if (left > right) { break; } else { // Swap array[left] and array[right] int temp = array[left]; array[left] = array[right]; array[right] = temp; } } // Swap array[low] and array[right] array[low] = array[right]; array[right] = pivot; quickSort(array, low, right - 1); quickSort(array, right + 1, high); } } // Context class class Sorter { private SortStrategy sortStrategy; public void setSortStrategy(SortStrategy sortStrategy) { this.sortStrategy = sortStrategy; } public void sort(int[] array) { sortStrategy.sort(array); } } // Client code public class Client { public static void main(String[] args) { Sorter sorter = new Sorter(); int[] data = {5, 3, 8, 4, 2}; // Using Bubble Sort sorter.setSortStrategy(new BubbleSort()); sorter.sort(data); System.out.println("Sorted with Bubble Sort: " + java.util.Arrays.toString(data)); // Using Quick Sort data = new int[]{5, 3, 8, 4, 2}; // Resetting data sorter.setSortStrategy(new QuickSort()); sorter.sort(data); System.out.println("Sorted with Quick Sort: " + java.util.Arrays.toString(data)); } }
In the example above, we defined the SortStrategy
interface with a method for sorting an array. Two concrete implementations, BubbleSort
and QuickSort
, encapsulate their respective sorting algorithms. The Sorter
class acts as the context that utilizes a SortStrategy
. It allows clients to set the desired sorting algorithm at runtime, enabling flexibility in how data is sorted.
This pattern showcases dynamic behavior in the application, as the sorting algorithm can be changed without modifying the context or the client code. The Strategy Pattern enhances the maintainability of the code, allowing for cleaner separation of concerns. New sorting algorithms can be added easily by implementing the SortStrategy
interface, ensuring that the application remains extensible and manageable.
In practical applications, the Strategy Pattern is not limited to sorting algorithms. It can be used to handle various behaviors, such as payment processing in an e-commerce system, different logging strategies, or even rendering graphics in a game engine. The key takeaway is that it provides a way to encapsulate behavior and make it interchangeable, promoting a clean and scalable codebase.
Best Practices for Using Design Patterns
When it comes to using design patterns in Java, adhering to best practices can significantly enhance the quality and maintainability of your code. Here are some crucial guidelines to think when working with design patterns:
1. Understand the Problem Domain
Before implementing any design pattern, it’s essential to thoroughly understand the problem you’re trying to solve. Design patterns are not one-size-fits-all solutions; each is tailored to a specific context. Take the time to analyze the requirements and constraints of your application, as this understanding will guide you in selecting the most appropriate design pattern.
2. Emphasize Readability
While design patterns can introduce efficiency and flexibility, they can also complicate code if not used judiciously. Strive for clarity over cleverness; the primary goal is to make your code understandable to others (and yourself in the future). Use meaningful names for classes and methods, and ensure that the flow of logic is easy to follow. Pattern implementations should enhance code readability rather than obscure it.
3. Avoid Overusing Patterns
It’s tempting to apply design patterns liberally, especially after learning about them. However, excessive use can lead to overly complex designs. Choose patterns that genuinely simplify your design or make it more adaptable. If a simple solution suffices, don’t overcomplicate matters by shoehorning in a pattern. Assess the trade-offs and remember that sometimes, simpler is better.
4. Favor Composition Over Inheritance
Many design patterns promote the use of composition instead of inheritance. By favoring composition, you can achieve greater flexibility and reusability. For instance, the Strategy Pattern exemplifies this principle by allowing behaviors to be defined as separate classes, which can be interchanged dynamically. This approach promotes loose coupling and allows for easier changes to behavior without modifying existing code.
5. Document Your Design Patterns
Proper documentation of design pattern implementations is critical for long-term maintenance. Make it a habit to comment on why a specific design pattern was chosen, how it integrates with the rest of the system, and any potential pitfalls. This documentation will be invaluable to future developers who encounter your code.
6. Test Your Implementations
Design patterns should not only be well-structured but also thoroughly tested. Because they encapsulate specific behaviors, testing ensures that the expected functionality is achieved and that limitations or bugs are identified early. Automated tests can help verify the integrity of the implemented patterns and provide a safety net for future changes.
7. Stay Agile
In software development, requirements can change rapidly. Keeping your codebase agile means you should be prepared to refactor or switch patterns as necessary. Design patterns should support your ability to adapt to changing requirements, not hinder it. Embrace refactoring as a natural part of the development process, ensuring that your design patterns remain relevant.
8. Learn from Real-World Examples
Studying real-world applications of design patterns can provide valuable insights into their effective usage. Analyze open-source projects or frameworks that utilize design patterns well. Understanding how experienced developers implement these patterns can improve your own coding practices and inspire more effective solutions.
By following these best practices, Java developers can harness the power of design patterns to create robust, maintainable, and resilient applications. The key is to remain thoughtful and deliberate in your approach, ensuring that the use of design patterns contributes positively to your development process.