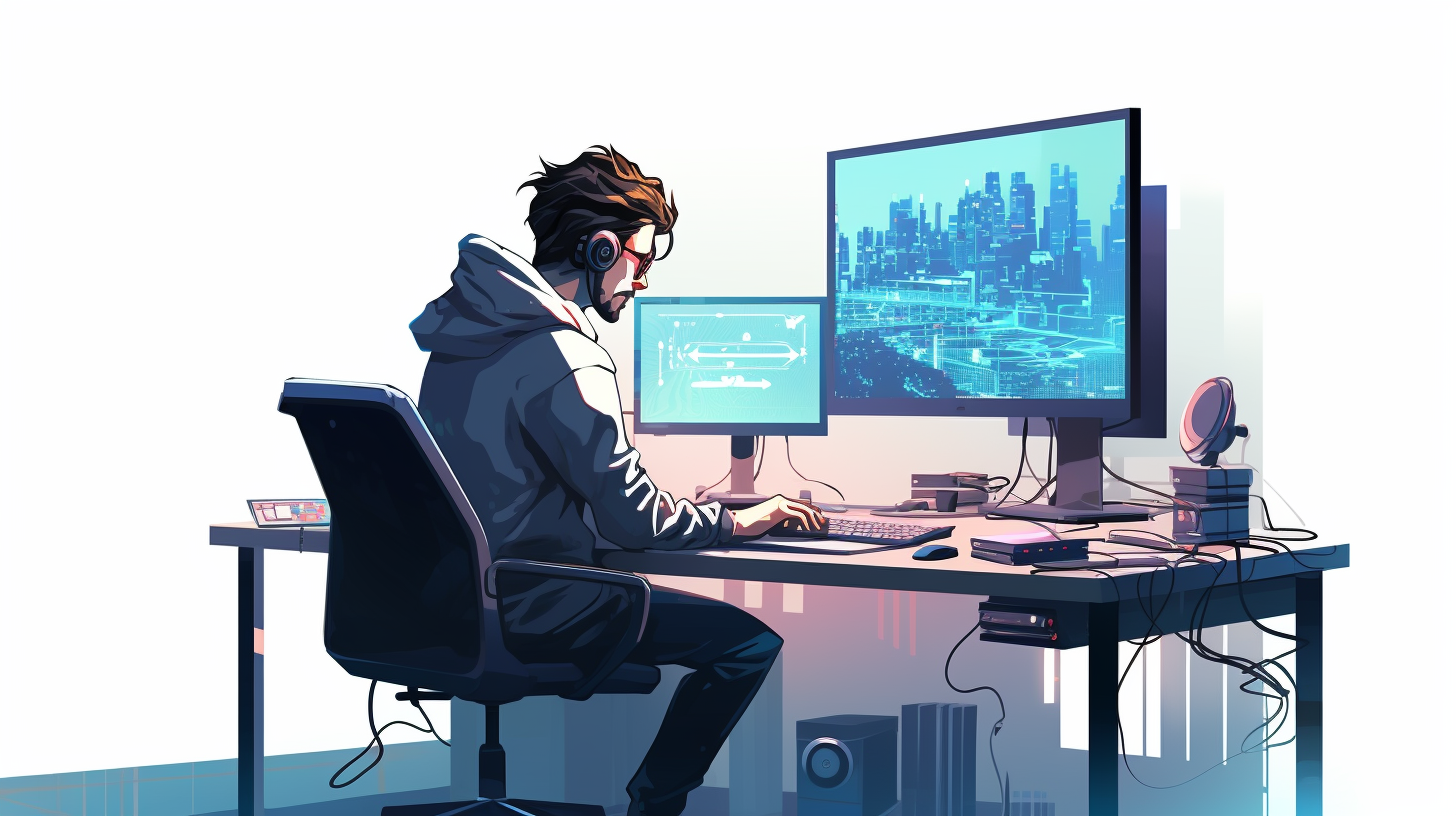
Java Multithreading: Creating Threads
In Java, a thread is a lightweight process, which is the smallest unit of processing that can be scheduled by the operating system. Each thread shares the same memory space, which makes communication between threads easier, but also introduces challenges such as data inconsistency and thread interference. Understanding threads very important for developing responsive and high-performance applications that can leverage the power of modern multi-core processors.
When a Java program starts, it has at least one thread, known as the main thread. That is the thread that begins execution of the application. Additional threads can be created to perform various tasks concurrently, which enables your program to handle multiple operations concurrently. By using threads, applications can significantly improve their performance, especially in scenarios involving I/O operations, animations, or handling network requests.
Java provides a robust threading model this is built on top of the operating system’s native threading capabilities. That is made possible through the Java Virtual Machine (JVM), which allows Java applications to create and manage threads. The threading model adheres to the concept of preemptive multitasking, meaning that the thread scheduler can interrupt a currently running thread to give time to another thread, resulting in an efficient and responsive application.
Threads in Java can be represented by either the Thread class or the Runnable interface. The Thread class is a built-in class that provides the functionality to create and manage threads directly, while the Runnable interface allows a class to be executed by a thread without being tightly coupled to the thread itself.
To create a thread, you typically extend the Thread class or implement the Runnable interface. Regardless of the approach, understanding how these threads operate within the Java threading model is essential for effective multithreading. Below is a simple example of creating a thread by extending the Thread class:
class MyThread extends Thread { public void run() { for (int i = 0; i < 5; i++) { System.out.println("Thread: " + Thread.currentThread().getName() + " - Count: " + i); } } } public class ThreadExample { public static void main(String[] args) { MyThread thread1 = new MyThread(); MyThread thread2 = new MyThread(); thread1.start(); // Start the first thread thread2.start(); // Start the second thread } }
In this example, the MyThread class overrides the run() method, which serves as the entry point for the new thread. When the start() method is called, the JVM creates a new thread and invokes the run() method in that thread context, allowing concurrent execution.
Understanding threads also involves recognizing the complexities that come with concurrent execution. Issues like race conditions, deadlocks, and thread starvation can arise if proper care is not taken. Java provides several synchronization mechanisms, such as synchronized blocks and locks, to manage access to shared resources effectively, ensuring that your application remains stable and reliable in a multithreaded environment.
Creating Threads using the Thread Class
Creating threads in Java can be accomplished by extending the Thread class. This approach allows you to define a new thread that can run independently of other threads in your program. When you create a subclass of Thread, you need to override its run() method. This method contains the code that will be executed when the thread is started.
Here’s how you can create and start multiple threads by extending the Thread class:
class MyThread extends Thread { public void run() { for (int i = 0; i < 5; i++) { System.out.println("Thread: " + Thread.currentThread().getName() + " - Count: " + i); try { // Simulating work by sleeping for a short duration Thread.sleep(100); } catch (InterruptedException e) { e.printStackTrace(); } } } } public class ThreadExample { public static void main(String[] args) { MyThread thread1 = new MyThread(); MyThread thread2 = new MyThread(); thread1.start(); // Start the first thread thread2.start(); // Start the second thread try { thread1.join(); // Wait for thread1 to finish thread2.join(); // Wait for thread2 to finish } catch (InterruptedException e) { e.printStackTrace(); } System.out.println("Both threads have finished execution."); } }
In this example, the run() method is responsible for running the loop five times, printing the name of the current thread and the loop count. The sleep() method simulates some processing time by causing the thread to pause for 100 milliseconds. This demonstrates how thread execution can be managed in a controlled manner.
The start() method very important for beginning the thread’s execution. It doesn’t run the run() method directly; instead, it creates a new thread and then calls run() in that new thread’s context. This allows both thread1
and thread2
to run simultaneously.
After starting the threads, the main thread waits for both thread1
and thread2
to complete using the join() method. This ensures that the main program does not terminate before the threads finish executing, providing a clean and orderly completion of tasks.
By employing the Thread class, developers gain a powerful tool for concurrent programming. However, care must be taken to handle potential concurrency issues that arise from shared data access. That’s where Java’s synchronization features come into play, so that you can manage data consistency and prevent conflicts in a multithreaded environment.
Implementing Runnable Interface
Implementing the Runnable interface is another effective way to create threads in Java. This approach offers greater flexibility than extending the Thread class, especially when you want to create multiple threads that share the same instance of a class. By implementing Runnable, you decouple the task from the thread, allowing the same task to be executed by multiple threads independently.
The Runnable interface defines a single method, run(), which contains the code that will be executed by a thread. The main advantage of using Runnable is that it enables your class to extend another class, since Java does not support multiple inheritance.
Here’s a simple example demonstrating how to implement the Runnable interface:
class MyRunnable implements Runnable { public void run() { for (int i = 0; i < 5; i++) { System.out.println("Thread: " + Thread.currentThread().getName() + " - Count: " + i); try { // Simulating work by sleeping for a short duration Thread.sleep(100); } catch (InterruptedException e) { e.printStackTrace(); } } } } public class RunnableExample { public static void main(String[] args) { MyRunnable myRunnable = new MyRunnable(); Thread thread1 = new Thread(myRunnable); Thread thread2 = new Thread(myRunnable); thread1.start(); // Start the first thread thread2.start(); // Start the second thread try { thread1.join(); // Wait for thread1 to finish thread2.join(); // Wait for thread2 to finish } catch (InterruptedException e) { e.printStackTrace(); } System.out.println("Both threads have finished execution."); } }
In this example, the MyRunnable class implements the Runnable interface, overriding the run() method. Inside the run() method, we have a loop that prints the name of the thread and its count. The Thread class is then used to create two new threads, each associated with the same instance of MyRunnable.
When you call the start() method on both threads, each thread executes the run() method at once, independently printing their counts. This scenario demonstrates how multiple threads can share the same Runnable instance, allowing for a more efficient use of resources, particularly when the task is inherently thread-safe or does not rely on instance-specific data.
As with any multithreaded application, care must be taken to manage shared resources and ensure thread safety. Java provides various synchronization tools, such as synchronized blocks and locks, which can help control access to shared data and prevent issues such as race conditions. By implementing Runnable, developers have a flexible and powerful means of creating and managing threads in their applications, aligning beautifully with the principles of object-oriented design.
Thread Lifecycle and Management
The lifecycle of a thread in Java is a fundamental concept that every developer working with multithreading should understand. The thread lifecycle defines the states that a thread can be in during its existence, as well as the transitions between those states. Understanding these states is important for effective thread management and for avoiding common pitfalls in concurrent programming.
There are five primary states in the thread lifecycle:
- A thread that has been created but not yet started is in the new state. At this point, the thread object is created, but the thread has not begun executing.
- Once the thread’s start() method is invoked, the thread enters the runnable state. In this state, the thread is ready to run but may not be executing immediately, as the thread scheduler determines when it runs. A thread can remain in this state until it’s chosen by the scheduler to execute.
- A thread can enter the blocked state when it tries to access a synchronized block that’s currently held by another thread. While in this state, the thread is waiting for the lock to be released so that it can continue execution.
- A thread enters the waiting state when it calls methods such as wait(), join(), or LockSupport.park(). The thread remains in this state until another thread signals it to wake up.
- When a thread has completed its execution, it enters the terminated state. This can happen either by completing the run() method or by throwing an unhandled exception. Once in this state, the thread cannot be started again.
Understanding these states is integral not just for recognizing what a thread is currently doing, but also for implementing efficient thread management. For example, below is a code snippet demonstrating the states of a thread:
class ThreadLifeCycleExample extends Thread { public void run() { System.out.println(Thread.currentThread().getName() + " is in RUNNABLE state."); try { Thread.sleep(1000); // Simulates work } catch (InterruptedException e) { e.printStackTrace(); } System.out.println(Thread.currentThread().getName() + " is in TERMINATED state."); } } public class Main { public static void main(String[] args) { ThreadLifeCycleExample thread1 = new ThreadLifeCycleExample(); System.out.println(thread1.getName() + " is in NEW state."); thread1.start(); // Moves to RUNNABLE state try { thread1.join(); // Main thread waits for thread1 to finish } catch (InterruptedException e) { e.printStackTrace(); } System.out.println("Main thread finished execution."); } }
In this example, when the `ThreadLifeCycleExample` thread is created, it’s in the new state. Once the `start()` method is invoked, it transitions to the runnable state where it can be executed by the thread scheduler. If `sleep()` is called, the thread will be in a blocked state temporarily until the sleep duration is over. After its run() method completes, it enters the terminated state.
Another important aspect of thread management is the use of the `join()` method, which allows one thread to wait for another to complete before proceeding. This very important for coordinating the execution order of threads and ensures that resources are synchronized properly. Without careful management, multithreaded applications can suffer from various issues such as race conditions, deadlocks, and excessive context switching.
Java provides extensive support for concurrency control through its `java.util.concurrent` package. This package contains various synchronization primitives, thread pools, and higher-level abstractions that can simplify thread management and improve performance. By mastering the thread lifecycle and management techniques, developers can build robust and efficient multithreaded applications that take full advantage of modern CPU architectures.