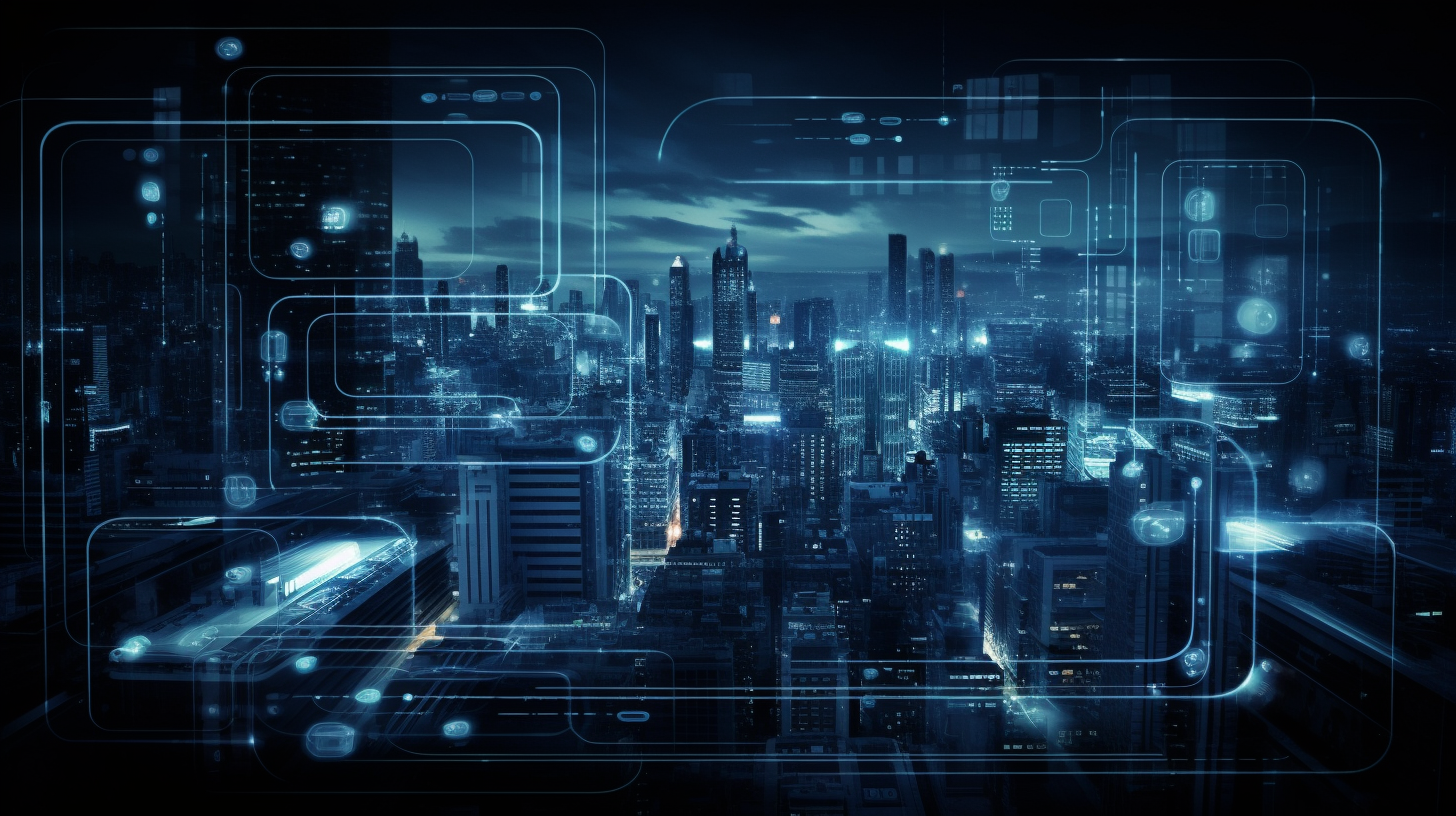
Java Persistence API (JPA): Basics
The Java Persistence API (JPA) stands as a pivotal component in the Java ecosystem, specifically designed for the management of relational data in Java applications. It provides a standardized way to interact with databases using Java objects, thereby promoting a more object-oriented approach to data management. By abstracting the underlying database interactions, JPA allows developers to focus more on business logic rather than the intricacies of SQL.
At its core, JPA facilitates the mapping of Java objects to database tables through a declarative mechanism, simplifying CRUD (Create, Read, Update, Delete) operations. JPA leverages the power of annotations or XML descriptors to define how Java classes and their attributes correlate to database entities and columns, allowing for a seamless integration between the application and the database.
JPA operates on the principle of an EntityManager, which acts as a bridge between the application and the underlying database. This manager provides various methods to manage the lifecycle of entity instances, including persisting new entities, merging detached entities, and removing entities from the database. The following code snippet illustrates how to create and persist a new entity:
EntityManager em = entityManagerFactory.createEntityManager(); em.getTransaction().begin(); MyEntity myEntity = new MyEntity(); myEntity.setName("Sample Entity"); myEntity.setValue(42); em.persist(myEntity); em.getTransaction().commit(); em.close();
Another essential feature of JPA is its support for the Java Persistence Query Language (JPQL), which allows developers to execute queries on their entity objects rather than directly on the database tables. This abstraction not only enhances readability but also makes the code less dependent on the specific database implementation.
JPA also emphasizes the importance of managing transactions effectively. It ensures data integrity and consistency by allowing developers to define transaction boundaries around their operations. The following example demonstrates how to use a transaction with JPA:
EntityManager em = entityManagerFactory.createEntityManager(); EntityTransaction transaction = em.getTransaction(); try { transaction.begin(); // Business logic (e.g., multiple entity operations) transaction.commit(); } catch (RuntimeException e) { if (transaction.isActive()) { transaction.rollback(); } throw e; // Optionally rethrow the exception } finally { em.close(); }
Core Concepts of JPA
The core concepts of JPA revolve around the concept of entities, which represent the data model of an application. Each entity corresponds to a database table, and its attributes correspond to the table’s columns. This mapping very important for enabling the persistence of data as Java objects. The JPA specification defines several key concepts that underpin its functionality, such as entities, relationships, inheritance, and the lifecycle of entities.
Entities and Their Lifecycle
At the heart of JPA are entities, which are typically plain old Java objects (POJOs) annotated with JPA annotations. These entities must have a no-argument constructor, and they should be serializable to facilitate their storage and retrieval. Each entity is uniquely identified by its primary key, which is usually annotated with @Id
. Below is an example of a simple entity class:
import javax.persistence.Entity; import javax.persistence.Id; @Entity public class MyEntity { @Id private Long id; private String name; private int value; // No-argument constructor public MyEntity() {} // Getters and setters public Long getId() { return id; } public void setId(Long id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getValue() { return value; } public void setValue(int value) { this.value = value; } }
Entities can exist in various states during their lifecycle: persistent, transient, and detached. A transient entity is one that has just been instantiated and is not associated with any database record. A persistent entity is managed by the EntityManager
and is synchronized with the database. Conversely, a detached entity is one that was once persistent but is no longer managed by the EntityManager
. Understanding these states is important for correctly managing data operations.
Relationships Between Entities
JPA also supports relationships between entities, allowing for more complex data models. The main types of relationships are one-to-one, one-to-many, many-to-one, and many-to-many. These relationships are defined using annotations such as @OneToMany
, @ManyToOne
, and @ManyToMany
. For example, a one-to-many relationship can be established as follows:
import javax.persistence.*; @Entity public class Author { @Id private Long id; private String name; @OneToMany(mappedBy = "author") private List books; // Getters and setters... } @Entity public class Book { @Id private Long id; private String title; @ManyToOne @JoinColumn(name = "author_id") private Author author; // Getters and setters... }
In this example, an Author
can have multiple Book
entities associated with it. The mappedBy
attribute indicates that the relationship is managed on the Book
side. This clarity in relationships enhances the ability to navigate complex object graphs seamlessly.
Inheritance in JPA
JPA also provides support for inheritance, allowing entities to inherit attributes and behaviors from other entities. There are three inheritance strategies available: SINGLE_TABLE
, TABLE_PER_CLASS
, and JOINED
. Each strategy comes with its own implications regarding database schema and performance.
For instance, with the SINGLE_TABLE
strategy, all entities are stored in a single table, and a discriminator column is used to differentiate between them:
@Entity @Inheritance(strategy = InheritanceType.SINGLE_TABLE) @DiscriminatorColumn(name = "entity_type") public abstract class BaseEntity { @Id private Long id; } @Entity @DiscriminatorValue("type_a") public class TypeA extends BaseEntity { private String attributeA; } @Entity @DiscriminatorValue("type_b") public class TypeB extends BaseEntity { private String attributeB; }
By encapsulating shared behavior and properties in a common base class, JPA facilitates a cleaner design and reduces redundancy.
Entity Management in JPA
In JPA, entity management plays an important role in ensuring that Java objects are correctly persisted in the underlying database. The EntityManager is the primary interface for interacting with the persistence context, which tracks the state of entity instances. It provides methods for CRUD operations, as well as for querying and managing the lifecycle of entities.
When an entity is persisted, it transitions from a transient state to a persistent state. This means that any changes made to the entity are tracked and synchronized with the database automatically. The EntityManager handles this synchronization, making the developer’s job considerably easier. Below is an example of how to persist an entity:
EntityManager em = entityManagerFactory.createEntityManager(); em.getTransaction().begin(); MyEntity myEntity = new MyEntity(); myEntity.setId(1L); myEntity.setName("Sample Entity"); myEntity.setValue(42); // Persisting the entity em.persist(myEntity); em.getTransaction().commit(); em.close();
Once the entity is persisted, any subsequent changes to this entity can be made directly, and they will be reflected in the database upon calling commit. This automatic synchronization is one of the key advantages of using JPA, as it abstracts away much of the boilerplate code required for standard database operations.
Another important aspect of entity management is merging detached entities. When an entity is detached, it means it’s no longer managed by the EntityManager and any changes made to it will not be automatically synchronized with the database. To reattach or merge a detached entity, you can use the merge
method of the EntityManager:
EntityManager em = entityManagerFactory.createEntityManager(); em.getTransaction().begin(); // Assume myEntity is a detached instance MyEntity myEntity = new MyEntity(); myEntity.setId(1L); myEntity.setName("Updated Entity"); myEntity.setValue(100); // Merging the detached entity MyEntity mergedEntity = em.merge(myEntity); em.getTransaction().commit(); em.close();
In this example, the detached myEntity
instance is merged back into the persistence context, and any changes are reflected in the database. The merge operation returns a managed instance, which can be used for further operations if necessary.
Entity removal is another critical function provided by the EntityManager. To delete an entity, you can call the remove
method after ensuring that the entity is in a persistent state. This operation will remove the entity from the database:
EntityManager em = entityManagerFactory.createEntityManager(); em.getTransaction().begin(); // Finding the entity to remove MyEntity myEntity = em.find(MyEntity.class, 1L); // Removing the entity if (myEntity != null) { em.remove(myEntity); } em.getTransaction().commit(); em.close();
In this snippet, the entity is located using the find
method, and if it exists, it’s removed from the database. The transaction management ensures that the operation is atomic, preserving data integrity.
Mapping Entities to Database Tables
Mapping entities to database tables is a foundational aspect of the Java Persistence API (JPA), providing developers with a simpler mechanism to define how Java classes correspond to relational database structures. This mapping can be achieved using annotations directly in the Java code or through XML configuration files. However, the annotation-based approach is far more prevalent due to its simplicity and ease of use.
At its core, each entity class is annotated with @Entity to indicate that it represents a table in the database. Additionally, the primary key of the entity is marked with the @Id annotation. This primary key uniquely identifies each record in the associated database table. Here’s a basic example:
import javax.persistence.Entity; import javax.persistence.Id; @Entity public class Product { @Id private Long id; private String name; private Double price; // No-argument constructor public Product() {} // Getters and setters public Long getId() { return id; } public void setId(Long id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public Double getPrice() { return price; } public void setPrice(Double price) { this.price = price; } }
In this example, the Product entity has three attributes: id, name, and price. The JPA provider will handle the mapping to a corresponding PRODUCT table in the database, where each attribute becomes a column.
Beyond basic mapping, JPA offers various annotations to customize the mapping behavior further. For instance, if the primary key is generated automatically by the database (such as in auto-increment scenarios), you can use the @GeneratedValue annotation:
import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; @Entity public class Order { @Id @GeneratedValue(strategy = GenerationType.AUTO) private Long id; private String customerName; private Double totalAmount; // No-argument constructor public Order() {} // Getters and setters... }
In this Order entity, the @GeneratedValue annotation specifies that the id will be generated automatically. The strategy can be adjusted based on the specific requirements, such as using IDENTITY, SEQUENCE, or AUTO.
Relationships between entities are another critical component of the mapping process. JPA allows you to define relationships using several annotations, allowing for a more nuanced data model. For example, in a one-to-many relationship, you can annotate the parent entity with @OneToMany and the child entity with @ManyToOne:
import javax.persistence.*; import java.util.List; @Entity public class Customer { @Id private Long id; private String name; @OneToMany(mappedBy = "customer") private List orders; // Getters and setters... } @Entity public class Order { @Id private Long id; private Double amount; @ManyToOne @JoinColumn(name = "customer_id") private Customer customer; // Getters and setters... }
In this scenario, each Customer can have multiple Order entities associated with it, while each Order is linked back to a single Customer. The mappedBy attribute in the Customer entity informs JPA that the relationship is managed from the Order side. The JoinColumn annotation is utilized to specify the foreign key in the Order table that references the primary key of the Customer table.
Inheritance is another powerful feature supported by JPA, which will allow you to establish hierarchies of entities. You can use the @Inheritance annotation to define how subclasses will be mapped to the database. The following example demonstrates how to implement inheritance using the SINGLE_TABLE strategy:
import javax.persistence.*; @Entity @Inheritance(strategy = InheritanceType.SINGLE_TABLE) @DiscriminatorColumn(name = "vehicle_type") public abstract class Vehicle { @Id private Long id; private String brand; // Getters and setters... } @Entity @DiscriminatorValue("car") public class Car extends Vehicle { private int numberOfDoors; // Getters and setters... } @Entity @DiscriminatorValue("bike") public class Bike extends Vehicle { private boolean hasCarrier; // Getters and setters... }
Here, the Vehicle class serves as a base class for Car and Bike entities. All instances are stored in a single table, with a vehicle_type column used to differentiate the types. This approach can reduce the number of tables in the database but requires careful consideration of performance implications based on the application’s needs.
JPA Query Language (JPQL)
The Java Persistence Query Language (JPQL) is an integral part of the Java Persistence API (JPA), allowing developers to perform operations on entity objects rather than directly on database tables. This abstraction layer enhances readability and maintainability of the code, making it easier to work with complex data models while remaining database-agnostic. JPQL is similar to SQL in syntax but operates on the entity model rather than the underlying database schema.
JPQL queries can be expressed in two main forms: named queries and dynamic queries. Named queries are defined statically in the entity class using the @Query annotation or in a separate XML configuration file, which can then be referenced by name, promoting reuse and clarity. Dynamic queries, on the other hand, are constructed programmatically at runtime, offering greater flexibility in query formulation. Below is an example of a named query:
import javax.persistence.*; @Entity @NamedQuery(name = "MyEntity.findByName", query = "SELECT e FROM MyEntity e WHERE e.name = :name") public class MyEntity { @Id private Long id; private String name; private int value; // No-argument constructor, getters, and setters... }
To execute this named query, you would utilize the EntityManager as follows:
EntityManager em = entityManagerFactory.createEntityManager(); TypedQuery query = em.createNamedQuery("MyEntity.findByName", MyEntity.class); query.setParameter("name", "Sample Entity"); List results = query.getResultList(); em.close();
Dynamic queries provide the flexibility to formulate queries based on variable criteria. JPQL supports a rich set of operations, including filtering, ordering, and grouping. For instance, a simple dynamic query can be created like this:
EntityManager em = entityManagerFactory.createEntityManager(); String jpql = "SELECT e FROM MyEntity e WHERE e.value > :value ORDER BY e.name"; TypedQuery query = em.createQuery(jpql, MyEntity.class); query.setParameter("value", 10); List results = query.getResultList(); em.close();
JPQL also supports joins, so that you can retrieve data from related entities. For instance, if you have a one-to-many relationship and want to fetch all books for a specific author, you can write a query like this:
String jpql = "SELECT b FROM Book b JOIN b.author a WHERE a.name = :authorName"; TypedQuery query = em.createQuery(jpql, Book.class); query.setParameter("authorName", "Author Name"); List results = query.getResultList();
In addition to standard queries, JPQL offers support for aggregate functions like COUNT, MAX, and AVG, making it suitable for more complex reporting needs. For example, to count the number of entities in a specific state, you could use:
String countQuery = "SELECT COUNT(e) FROM MyEntity e WHERE e.value > :value"; Long count = em.createQuery(countQuery, Long.class) .setParameter("value", 10) .getSingleResult();
JPQL also supports a powerful feature called subqueries, which allows embedding one query within another. This capability can be leveraged for more advanced filtering and data retrieval. For example:
String subQuery = "SELECT e FROM MyEntity e WHERE e.value IN (SELECT e2.value FROM MyEntity e2 WHERE e2.name = :name)"; List results = em.createQuery(subQuery, MyEntity.class) .setParameter("name", "Sample Entity") .getResultList();
Transactions and Concurrency in JPA
The management of transactions in JPA especially important for ensuring data consistency and integrity throughout the lifecycle of an application. Transactions serve as a means to group multiple operations into a single, atomic unit of work, allowing changes to be committed to the database only when all operations succeed. If any operation fails, the entire transaction can be rolled back, leaving the database in a consistent state.To begin a transaction in JPA, the EntityManager must first be created. Once the EntityManager is instantiated, a transaction can be initiated, followed by the business logic operations. After the operations are performed, the transaction can either be committed, if all operations are successful, or rolled back if an exception occurs. Below is an example demonstrating the use of transactions in JPA:
```java
EntityManager em = entityManagerFactory.createEntityManager();
EntityTransaction transaction = em.getTransaction();try {
transaction.begin();// Perform multiple operations
MyEntity myEntity1 = new MyEntity();
myEntity1.setName("Entity One");
myEntity1.setValue(10);
em.persist(myEntity1);MyEntity myEntity2 = new MyEntity();
myEntity2.setName("Entity Two");
myEntity2.setValue(20);
em.persist(myEntity2);// If everything is successful, commit the transaction
transaction.commit();
} catch (RuntimeException e) {
if (transaction.isActive()) {
transaction.rollback(); // Rollback if any exception occurs
}
throw e; // Rethrow the exception for further handling
} finally {
em.close(); // Ensure the EntityManager is closed
}
```In this example, two entities are created and persisted within a single transaction. If any error occurs during the persistence of either entity, the transaction will be rolled back, preventing any partial updates that might leave the database in an inconsistent state.
Concurrency in JPA is another important aspect that developers must consider, particularly in multi-threaded environments where multiple transactions may attempt to access or modify the same data at the same time. JPA provides several strategies to handle concurrency, the most common of which are optimistic and pessimistic locking.
Optimistic locking assumes that multiple transactions can complete without affecting each other. It uses a version field, typically annotated with @Version, which JPA checks before committing a transaction. If the version has changed since the entity was loaded, an OptimisticLockException is thrown, indicating that another transaction has modified the entity. Here’s an example:
```java
import javax.persistence.*;@Entity
public class MyEntity {
@Id
private Long id;@Version
private int version; // Version field for optimistic lockingprivate String name;
private int value;// Getters and setters...
}
```In this example, the `version` field is automatically incremented by JPA with each update, allowing the framework to handle version checks during transaction commits.
On the other hand, pessimistic locking prevents other transactions from accessing an entity until the current transaction is completed. This can be implemented using the `LockModeType.PESSIMISTIC_WRITE` option when querying an entity:
```java
EntityManager em = entityManagerFactory.createEntityManager();
MyEntity myEntity = em.find(MyEntity.class, 1L, LockModeType.PESSIMISTIC_WRITE);try {
em.getTransaction().begin();
myEntity.setValue(100); // Modify the entity
em.getTransaction().commit();
} catch (Exception e) {
if (em.getTransaction().isActive()) {
em.getTransaction().rollback();
}
throw e; // Handle exception
} finally {
em.close();
}
```In the case of pessimistic locking, the chosen entity is locked for writing, preventing other transactions from reading or modifying it until the current transaction is finished. This approach can be useful in scenarios where conflicts are likely and ensures that data integrity is maintained.