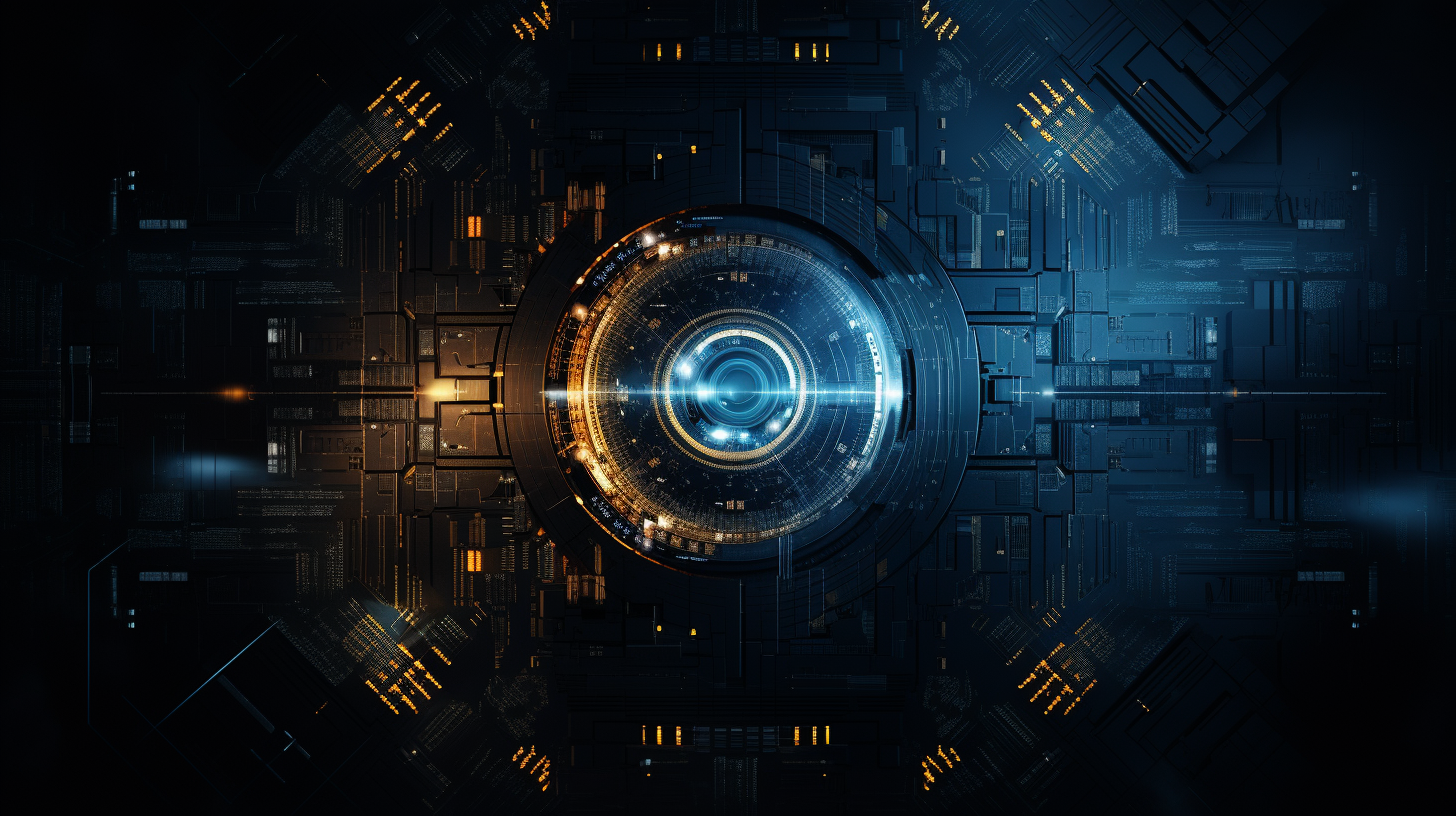
Java Reflection API: Basics and Applications
The Java Reflection API is a powerful mechanism that allows programs to inspect and manipulate classes, methods, and fields at runtime, without knowing their names at compile time. This capability is what makes Java dynamic and flexible, enabling a range of advanced programming techniques that can greatly enhance the functionality of applications.
At its core, reflection provides a way to access the metadata of classes and objects in a way this is not possible through regular Java features. Through reflection, you can
- Examine the properties of classes, such as their fields, methods, constructors, and annotations.
- Create new instances of classes dynamically.
- Invoke methods and access fields, including private ones, regardless of their visibility.
This ability to manipulate classes at runtime opens the door to a variety of programming paradigms, such as:
- Frameworks like Spring utilize reflection to manage object lifecycles and dependencies.
- Reflection can be used to serialize objects without needing explicit serialization logic for each class.
- Java’s built-in proxy mechanism leverages reflection to create proxy instances.
To understand how reflection works, consider the Class
class, which serves as the entry point for reflection. You can obtain the Class
object corresponding to a class by using the getClass()
method or by calling Class.forName()
.
public class ReflectionExample { public static void main(String[] args) { try { // Get the Class object associated with the String class Class<?> stringClass = Class.forName("java.lang.String"); // Print the name of the class System.out.println("Class Name: " + stringClass.getName()); // Getting all methods of the class Method[] methods = stringClass.getDeclaredMethods(); System.out.println("Methods:"); for (Method method : methods) { System.out.println(method.getName()); } } catch (ClassNotFoundException e) { e.printStackTrace(); } } }
In this example, we retrieve the Class
object for the String
class, print its name, and list its methods. This illustrates how reflection allows us to explore the structure of a class dynamically.
Moreover, reflection isn’t just limited to examining classes; you can also manipulate them. For instance, you can create new instances of a class using its constructor:
import java.lang.reflect.Constructor; public class ReflectionConstructorExample { public static void main(String[] args) { try { // Get the Class object for the StringBuilder class Class<?> stringBuilderClass = Class.forName("java.lang.StringBuilder"); // Get the default constructor Constructor<?> constructor = stringBuilderClass.getConstructor(); // Create a new instance of StringBuilder Object stringBuilderInstance = constructor.newInstance(); System.out.println("Created Instance: " + stringBuilderInstance); } catch (Exception e) { e.printStackTrace(); } } }
This code snippet demonstrates how to obtain a constructor and create a new instance of the StringBuilder
class. Reflection thus provides a means to instantiate classes dynamically based on runtime information.
While the Java Reflection API is incredibly useful, it comes with performance overhead. Since reflection involves type resolution and dynamic method invocation, it’s typically slower than direct method calls. Therefore, it’s essential to use reflection judiciously and only when necessary.
Key Features and Components
The Java Reflection API comprises several key features and components that enhance its utility and versatility in application development. Understanding these components is essential for developers who aim to leverage reflection effectively.
At the heart of the Reflection API is the Class class, which represents the metadata of a Java class or interface. Every class in Java automatically has a Class object associated with it, which can be accessed using the getClass()
method or by using Class.forName()
to retrieve the Class object from a string representation of the class name. This Class object serves as the entry point for all reflection operations.
public class ClassExample { public static void main(String[] args) { Class<?> clazz = String.class; // Accessing Class object using the class literal System.out.println("Class Name: " + clazz.getName()); } }
Once you have the Class object, you can utilize various methods provided by the Class class to inspect the class’s structure. For instance, you can retrieve information about its constructors, methods, fields, and annotations. Here are some of the essential methods:
- Returns an array of
Method
objects reflecting all the methods declared by the class. - Returns an array of
Field
objects representing all fields declared by the class. - Returns an array of
Constructor
objects representing all constructors declared by the class.
These methods allow developers to gather comprehensive information about the class they’re working with, enabling dynamic behavior that would be impossible with static type information alone.
Reflection also allows for dynamic method invocation. This means you can call methods on an object without knowing the method’s name at compile time. Using the Method class, you can invoke methods by obtaining the appropriate Method object first:
import java.lang.reflect.Method; public class ReflectionMethodExample { public void displayMessage() { System.out.println("Hello, Reflection!"); } public static void main(String[] args) { try { Class<?> clazz = ReflectionMethodExample.class; Object instance = clazz.getDeclaredConstructor().newInstance(); Method method = clazz.getDeclaredMethod("displayMessage"); method.invoke(instance); // Invoking the method dynamically } catch (Exception e) { e.printStackTrace(); } } }
In this example, we create an instance of ReflectionMethodExample
, retrieve the displayMessage
method, and invoke it without directly calling it in the code. This illustrates the dynamic capabilities provided by the Reflection API.
Another critical aspect of the Reflection API is its ability to access and modify fields, including those marked as private. The Field class is used here, which will allow you to get and set field values even if they are not accessible by standard means:
import java.lang.reflect.Field; public class ReflectionFieldExample { private String message = "Initial Message"; public static void main(String[] args) { try { ReflectionFieldExample example = new ReflectionFieldExample(); Class<?> clazz = example.getClass(); Field field = clazz.getDeclaredField("message"); field.setAccessible(true); // Bypass the private access modifier System.out.println("Original: " + field.get(example)); field.set(example, "Modified Message"); System.out.println("Modified: " + field.get(example)); } catch (Exception e) { e.printStackTrace(); } } }
In this snippet, we illustrate how to access a private field, modify its value, and retrieve it again, showcasing the power of reflection to manipulate object state dynamically.
Additionally, the Reflection API supports the use of Annotations. You can retrieve annotations applied to classes, methods, and fields, allowing for runtime analysis of metadata. That is particularly useful in frameworks that rely on annotations to define behaviors and configurations, such as dependency injection frameworks.
Common Use Cases of Reflection
Common use cases for Java Reflection span a wide range of programming paradigms, enabling developers to write dynamic and highly configurable applications. One of the most prevalent applications is in frameworks that rely on Dependency Injection (DI). Frameworks like Spring leverage reflection to instantiate beans and manage their lifecycles and dependencies automatically. By using reflection, these frameworks can inject dependencies into classes without needing to hard-code these relationships, promoting loose coupling and greater flexibility.
Another significant use case is Serialization. Java’s built-in serialization mechanism makes use of reflection to inspect the fields of an object and serialize them into a byte stream. This allows for the seamless saving and loading of object states without requiring dedicated serialization logic for each individual class. The Java Reflection API facilitates this by allowing the serialization framework to dynamically access private fields, ensuring that necessary data is captured during the serialization process.
Dynamic Proxies are another exciting application of reflection. Java provides a Proxy class that allows for the creation of proxy instances that implement specified interfaces. That’s particularly useful in implementing design patterns such as Aspect-Oriented Programming (AOP), where behavior can be added to existing code without modifying its structure. By using reflection to create and manage these proxies, developers can implement cross-cutting concerns like logging, security, and transactions in a modular fashion.
Here is a simple example of creating a dynamic proxy using reflection:
import java.lang.reflect.InvocationHandler; import java.lang.reflect.Method; import java.lang.reflect.Proxy; interface Greeting { void sayHello(String name); } class GreetingImpl implements Greeting { public void sayHello(String name) { System.out.println("Hello, " + name); } } class GreetingInvocationHandler implements InvocationHandler { private final Greeting original; public GreetingInvocationHandler(Greeting original) { this.original = original; } @Override public Object invoke(Object proxy, Method method, Object[] args) throws Throwable { System.out.println("Before method: " + method.getName()); Object result = method.invoke(original, args); System.out.println("After method: " + method.getName()); return result; } } public class DynamicProxyExample { public static void main(String[] args) { Greeting greeting = new GreetingImpl(); Greeting proxy = (Greeting) Proxy.newProxyInstance( Greeting.class.getClassLoader(), new Class[]{Greeting.class}, new GreetingInvocationHandler(greeting)); proxy.sayHello("Alice"); } }
In this example, we create a dynamic proxy for the Greeting interface. The proxy intercepts method calls to add additional behavior before and after the invocation, which illustrates how reflection can be used to augment functionality without changing the original implementation.
Moreover, reflection is instrumental in frameworks that use Annotations. In such cases, reflection allows for the retrieval of annotations and their values at runtime. This capability enables developers to create highly configurable and reusable components that can adapt their behavior based on metadata, often defined through annotations. A common example is the use of custom annotations to specify transaction boundaries in a persistence framework. By letting reflection manage the underlying logic, developers can focus on defining the business rules without being bogged down by boilerplate code.
Finally, testing frameworks like JUnit and Mockito heavily utilize reflection to instantiate test classes, manage test lifecycle, and invoke test methods. This reliance on reflection allows for a clear separation of test code from business logic, enabling developers to write more maintainable and extensible tests.
Best Practices and Performance Considerations
When working with the Java Reflection API, it’s critical to adhere to best practices and be mindful of performance considerations to reap its benefits without incurring unnecessary overhead. Reflection can significantly alter the behavior of applications, but it can also introduce complexities and inefficiencies if misused.
Use Reflection Sparingly
First and foremost, developers should use reflection sparingly and only when absolutely necessary. While reflection provides powerful capabilities, it’s inherently slower than direct method calls. This slowdown arises from the additional operations needed to resolve types and methods at runtime. Therefore, where possible, prefer standard object-oriented programming techniques over reflection. Only resort to reflection for scenarios where flexibility and runtime adaptability are paramount, such as in frameworks that require dynamic behavior.
Caching Reflection Results
Another important practice is to cache reflection results. Since obtaining metadata through reflection involves overhead, caching frequently accessed reflection objects—such as Class
, Method
, and Field
instances—can yield significant performance improvements. For instance, if you are repeatedly accessing the same method, store the Method
object after the first retrieval to avoid repeated lookups:
import java.lang.reflect.Method; import java.util.HashMap; import java.util.Map; public class ReflectionCacheExample { private static final Map methodCache = new HashMap(); public static Method getCachedMethod(Class<?> clazz, String methodName) throws NoSuchMethodException { String key = clazz.getName() + methodName; if (!methodCache.containsKey(key)) { Method method = clazz.getDeclaredMethod(methodName); methodCache.put(key, method); } return methodCache.get(key); } }
This caching mechanism optimizes performance by reducing the number of reflection calls, making your application more efficient when using reflection.
Think Access Modifiers
When accessing fields and methods, be aware of access modifiers. Reflection allows you to bypass encapsulation by accessing private fields and methods, which might lead to fragile code. Use setAccessible(true)
judiciously, and consider whether it’s necessary or if you can modify the class design to expose the required functionality through public interfaces. Overusing this feature can lead to maintenance challenges and unexpected behavior, particularly when changes are made to the class structure in the future.
import java.lang.reflect.Field;public class ReflectionFieldAccess {
private String secret = "Hidden Message";public static void main(String[] args) {
try {
ReflectionFieldAccess example = new ReflectionFieldAccess();
Field field = ReflectionFieldAccess.class.getDeclaredField("secret");
field.setAccessible(true); // Caution: Accessing private field