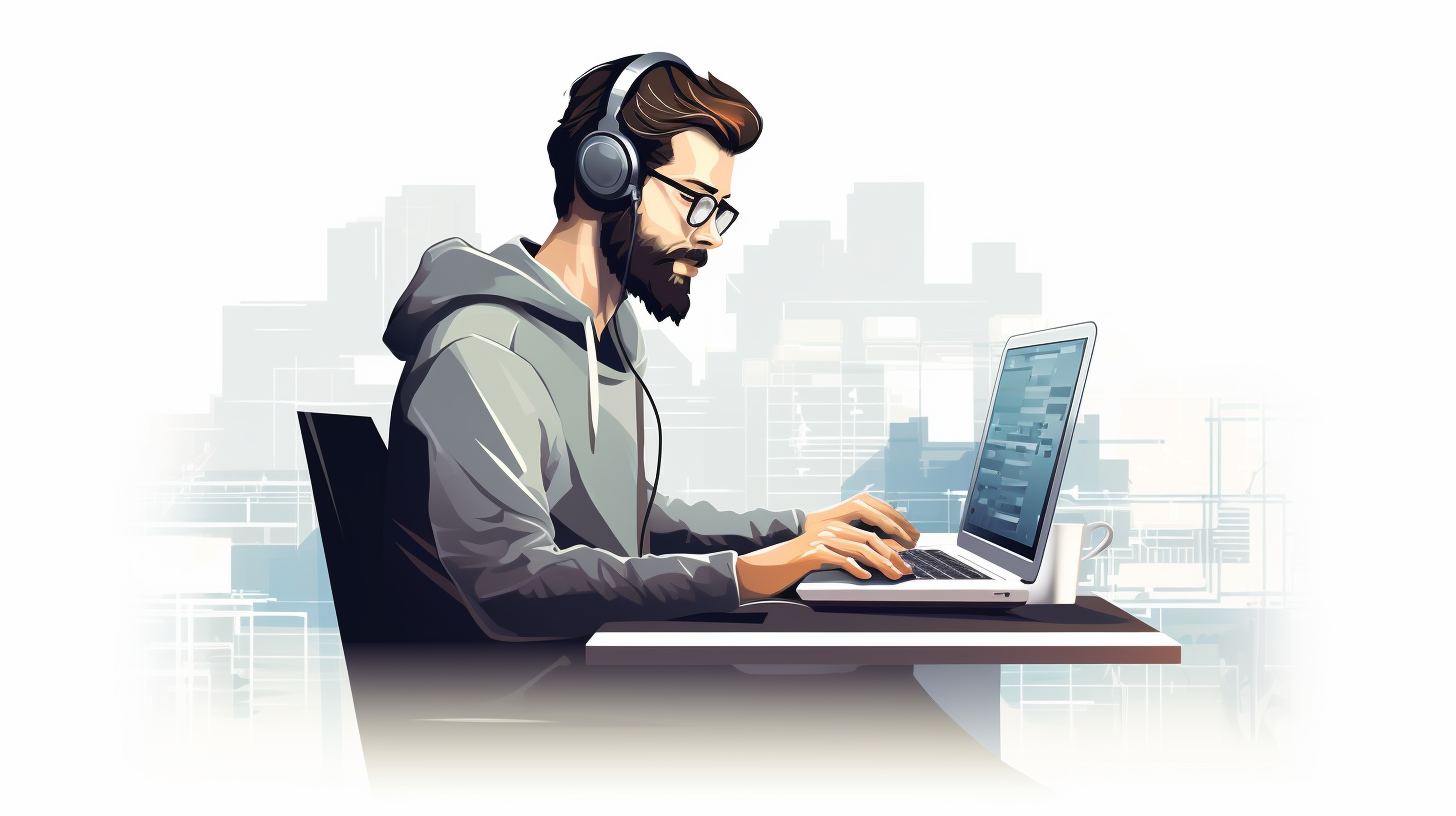
Java Spring Framework: An Introduction
The Java Spring Framework stands as a formidable pillar in the sphere of enterprise application development. It deftly tackles the intricate challenges posed by modern software development by providing a comprehensive programming and configuration model. Spring’s versatility allows developers to build applications that are not only robust but also scalable and maintainable.
At its core, Spring was designed to alleviate the complexity of Java EE development. By offering a simpler alternative to traditional Java EE technologies, Spring enables developers to focus on building features rather than managing boilerplate code. It does this through its modular architecture, which allows developers to include only the components they need.
One of the standout features of the Spring Framework is its dependency injection (DI) mechanism, which decouples the components of an application. This promotes a more organized and testable codebase. Let’s take a look at a basic example of how dependency injection works in Spring:
import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class MainApp { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext("beans.xml"); MyApp app = (MyApp) context.getBean("myApp"); app.run(); } } public class MyApp { private MyService myService; // Dependency injection via constructor public MyApp(MyService myService) { this.myService = myService; } public void run() { myService.execute(); } } public class MyService { public void execute() { System.out.println("Service Executed!"); } }
In the example above, the MyApp
class depends on the MyService
class. Instead of creating an instance of MyService
directly, Spring injects it, allowing MyApp
to remain agnostic about the concrete implementation of its dependencies.
Another pivotal aspect of Spring is its aspect-oriented programming (AOP) capabilities, which enable the separation of cross-cutting concerns, such as logging and transaction management, from the business logic. This further solidifies Spring’s power as it enhances modularization and code readability.
Spring also embraces a wide array of integration options, allowing seamless connections with various data sources, messaging services, and cloud platforms. By abstracting the complexities of these integrations, Spring enables developers to construct versatile applications that can adapt to various infrastructures and requirements.
The Java Spring Framework stands out as a comprehensive toolkit, simplifying the development of complex applications while promoting good design principles and best practices. Its robust features, such as dependency injection and aspect-oriented programming, form the foundation for crafting scalable and maintainable enterprise-level applications.
Core Concepts of Spring
To delve deeper into the core concepts of the Spring Framework, we must consider several key components that form the backbone of this powerful tool. At the heart of Spring’s architecture is the ApplicationContext, which serves as a central interface for accessing application components. It not only holds the bean definitions but also manages the complete lifecycle of beans. This becomes crucial for managing dependencies and ensuring that the application components are wired together correctly.
Spring beans are essentially the objects that form the backbone of a Spring application. These beans are instantiated, assembled, and managed by the Spring IoC (Inversion of Control) container. The definition of a bean can be configured through XML or Java annotations, providing flexibility in how developers choose to configure their applications.
Moreover, Spring’s configuration supports various scopes for beans, such as singleton, prototype, request, session, and global session. This flexibility allows developers to control the lifecycle and visibility of beans according to application-specific needs. For instance, a singleton scope ensures that a single instance of a bean is created and shared across the entire application, whereas a prototype scope creates a new instance each time the bean is requested.
Another important aspect of Spring is its support for Event Handling. The Spring Framework provides a robust event mechanism allowing beans to publish and listen for events. That is particularly useful for decoupled architectures where different components can react to events without direct dependencies on each other. Here’s a simple example of event publishing and listening:
import org.springframework.context.ApplicationEvent; import org.springframework.context.ApplicationListener; import org.springframework.context.annotation.AnnotationConfigApplicationContext; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; class CustomEvent extends ApplicationEvent { public CustomEvent(Object source) { super(source); } } class CustomEventListener implements ApplicationListener { @Override public void onApplicationEvent(CustomEvent event) { System.out.println("Custom event received: " + event.getSource()); } } @Configuration class AppConfig { @Bean public CustomEventListener customEventListener() { return new CustomEventListener(); } } public class EventDemo { public static void main(String[] args) { AnnotationConfigApplicationContext context = new AnnotationConfigApplicationContext(AppConfig.class); CustomEvent event = new CustomEvent("Hello Spring Events!"); context.publishEvent(event); context.close(); } }
In this example, we define a CustomEvent class, which extends ApplicationEvent. A listener, CustomEventListener, is created to handle such events. The AppConfig class configures the context, enabling event listening and publishing. When the event is published, the listener responds to it, demonstrating Spring’s event-driven capabilities.
Furthermore, Spring’s Profiles feature allows developers to define different beans or configurations for different environments (like development, testing, production). By activating specific profiles, Spring can load the appropriate configuration without code changes, thus adhering to the principle of separating configuration from code.
Lastly, the Spring Framework offers extensive support for integrating with numerous technologies, such as JPA for data persistence, Spring MVC for web applications, and WebFlux for reactive programming. This vast ecosystem empowers developers to create applications that not only meet current requirements but are also adaptable to future enhancements and modifications.
Dependency Injection and Inversion of Control
Dependency Injection (DI) and Inversion of Control (IoC) are foundational concepts that empower the Spring Framework, enabling developers to construct applications with enhanced flexibility and testability. At its essence, Inversion of Control refers to the design principle in which the control of object creation and management is inverted compared to traditional programming. Instead of the application code directly controlling the flow and instantiation of dependencies, the Spring container takes on this responsibility, allowing for a clean separation of concerns.
Within the context of Spring, Dependency Injection is the mechanism through which the IoC container provides the objects (or beans) that a class requires. This can be achieved through various methods such as constructor injection, setter injection, or method injection. Constructor injection is preferred as it ensures that the dependency is provided at the time of object creation, which aligns with the principles of immutability and avoids the pitfalls of null references.
To illustrate this, think the following example, which demonstrates both constructor injection and bean configuration through annotations:
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.beans.factory.annotation.Configurable; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.ComponentScan; import org.springframework.context.annotation.Configuration; @Configuration @ComponentScan(basePackages = "com.example") public class AppConfig { @Bean public MyService myService() { return new MyService(); } @Bean public MyApp myApp(MyService myService) { return new MyApp(myService); } } class MyApp { private final MyService myService; @Autowired public MyApp(MyService myService) { this.myService = myService; } public void run() { myService.execute(); } } class MyService { public void execute() { System.out.println("Service Executed!"); } }
In this configuration, the MyApp
class has a dependency on the MyService
class. The AppConfig
class declares beans for both MyService
and MyApp
, allowing Spring to inject the correct instance of MyService
into MyApp
at runtime. The use of the @Autowired
annotation indicates that Spring should automatically resolve the dependency.
With DI, testing becomes significantly easier because you can inject mock implementations of dependencies. This leads to more isolated and controlled unit tests. For instance, consider how one might write a test for the MyApp
class:
import static org.mockito.Mockito.*; public class MyAppTest { @Test public void testRun() { MyService mockService = mock(MyService.class); MyApp app = new MyApp(mockService); app.run(); verify(mockService).execute(); } }
In this test, a mocked MyService
is injected into MyApp
. When app.run()
is called, we can verify that the execute()
method was indeed invoked on the mock, asserting that our application flows correctly without needing the actual implementation of the service.
Inversion of Control and Dependency Injection work hand-in-hand to enhance modularity, making it possible to swap out components without altering the overall architecture of the application. This design pattern enables developers to focus on writing business logic instead of wrestling with dependency management, ultimately leading to cleaner and more maintainable code.
Furthermore, Spring’s ability to manage complex object graphs and their lifecycle means that developers can create intricate applications without the overhead of manual configuration. With a wealth of features like scope management, lifecycle callbacks, and environment-specific configurations, the Spring Framework provides a robust foundation for developing modern applications.
Spring Boot: Simplifying Spring Application Development
Spring Boot is a powerful extension of the Spring Framework that simplifies the process of building production-ready applications. By using convention over configuration, Spring Boot allows developers to get started quickly without the burdensome overhead of complex setup and configurations typically associated with Spring applications. That’s particularly advantageous in a fast-paced development environment where speed and agility are paramount.
At its core, Spring Boot eliminates the need for extensive XML configuration files and boilerplate code. It introduces the concept of “starter” dependencies, which are a set of convenient dependency descriptors you can include in your application. They aggregate common libraries for specific functionalities, allowing developers to add features to their applications efficiently. For example, if you want to create a web application, you simply include the spring-boot-starter-web
dependency. This starter handles all the necessary dependencies, including Spring MVC and embedded servers like Tomcat or Jetty.
// Example of a simple Spring Boot application import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; @SpringBootApplication @RestController public class MySpringBootApp { public static void main(String[] args) { SpringApplication.run(MySpringBootApp.class, args); } @GetMapping("/hello") public String hello() { return "Hello, Spring Boot!"; } }
In the example above, the @SpringBootApplication
annotation encapsulates three essential annotations: @Configuration
, @EnableAutoConfiguration
, and @ComponentScan
. This single annotation signifies that the class is a source of bean definitions and that Spring should automatically configure the application based on the dependencies present in the classpath.
Another significant feature of Spring Boot is the embedded server capability. With Spring Boot, you can package your application as a standalone JAR file, which includes an embedded server (like Tomcat). This means that you can run your application with a simple java -jar
command, eliminating the need to deploy to a separate server.
// Example of application.properties for configuration server.port=8080 spring.datasource.url=jdbc:mysql://localhost:3306/mydb spring.datasource.username=root spring.datasource.password=secret
Configuration in Spring Boot can be achieved through various means, including the application.properties
or application.yml
files, which allow for externalized configuration. This feature enables developers to manage application settings without altering the codebase, thus adhering to the twelve-factor app principles.
Moreover, Spring Boot’s Actuator module provides built-in monitoring and management features for your application. It exposes various endpoints that can deliver insights into application health, metrics, and environment properties, allowing developers to gain visibility into their application’s performance and behavior.
// Example of enabling Actuator in Spring Boot // Add the following dependency in your pom.xml // // org.springframework.boot // spring-boot-starter-actuator //
With the Actuator dependency included, you can access various endpoints, such as /actuator/health
and /actuator/metrics
, to monitor your application’s state. This feature is invaluable for DevOps practices, enabling teams to maintain application reliability and performance in production environments.
Spring Boot stands out by greatly simplifying the development process for Spring applications. Through the use of starters, embedded servers, advanced configuration options, and monitoring capabilities, it reduces the complexity and time required to get applications up and running. This allows developers to concentrate on building features and delivering value, rather than getting bogged down in configuration details and setup processes.
Building RESTful Web Services with Spring
Building RESTful web services with the Spring Framework is a simpler yet powerful endeavor. Spring MVC, a component of the Spring Framework, provides all the necessary tools and annotations to quickly create RESTful endpoints that can handle HTTP requests and return data in various formats, typically JSON or XML. This capability makes Spring a favored choice for implementing web services in modern enterprise applications.
To demonstrate how you can set up a simple RESTful service, let’s ponder an example where we create a service to manage a list of books. This service will allow users to perform operations such as retrieving, adding, updating, and deleting books.
import org.springframework.web.bind.annotation.*; import java.util.ArrayList; import java.util.List; @RestController @RequestMapping("/books") public class BookController { private List bookList = new ArrayList(); @GetMapping public List getAllBooks() { return bookList; } @PostMapping public void addBook(@RequestBody Book book) { bookList.add(book); } @PutMapping("/{id}") public void updateBook(@PathVariable int id, @RequestBody Book book) { bookList.set(id, book); } @DeleteMapping("/{id}") public void deleteBook(@PathVariable int id) { bookList.remove(id); } } class Book { private String title; private String author; // Getters and Setters public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } public String getAuthor() { return author; } public void setAuthor(String author) { this.author = author; } }
In the example above, the BookController
class defines several endpoints for managing books. The @RestController
annotation indicates that this class will handle HTTP requests and responses, while @RequestMapping
specifies the base URL path for the service.
Each method in the controller corresponds to an HTTP verb:
- This method retrieves the list of all books and returns it as a JSON response.
- This method takes a
Book
object from the request body and adds it to this book list. - This method updates an existing book by its index, allowing for modification of its details.
- This method removes a book from the list based on its index.
Notice how the @RequestBody
annotation is used to map the incoming JSON data to the Book
object. Similarly, the @PathVariable
annotation allows us to extract the book ID from the URL to perform operations on specific books.
To run this service, you can set up a Spring Boot application that includes the necessary dependencies for Spring Web. Below is a concise setup for a Spring Boot application:
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class BookServiceApplication { public static void main(String[] args) { SpringApplication.run(BookServiceApplication.class, args); } }
To test the various endpoints, you can use tools like Postman or cURL. Here’s how you might use cURL to add a new book:
curl -X POST -H "Content-Type: application/json" -d '{"title": "Effective Java", "author": "Joshua Bloch"}' http://localhost:8080/books
Building RESTful web services with Spring not only simplifies the creation of endpoints but also enhances flexibility and scalability. You can integrate security features, handle various content types, and manage exceptions gracefully, all while adhering to REST principles. With Spring’s powerful ecosystem and support for best practices, you can effectively expose your application’s functionality to clients and other services using a standardized approach.
Testing and Best Practices in Spring Applications
Within the scope of enterprise application development, testing is a vital aspect that ensures the reliability and correctness of applications. The Spring Framework provides a rich set of testing features, enabling developers to create tests that are both effective and easy to maintain. Testing in Spring applications can be broadly categorized into unit tests, integration tests, and end-to-end tests, each serving a distinct purpose and using different techniques.
Unit testing is focused on testing individual components or classes in isolation. Spring’s dependency injection allows for easy mocking of dependencies, which very important for unit tests. By using frameworks such as JUnit and Mockito, developers can create comprehensive test cases that ensure the correctness of their business logic without involving external systems.
import static org.mockito.Mockito.*; import static org.junit.Assert.*; import org.junit.Test; public class MyAppTest { @Test public void testRun() { // Create a mock instance of MyService MyService mockService = mock(MyService.class); // Create the application instance with the mocked service MyApp app = new MyApp(mockService); // Call the run method app.run(); // Verify that the execute method was called verify(mockService).execute(); } }
In this example, we utilize Mockito to create a mock of the MyService class. This allows us to verify that the execute method is invoked when the run method of MyApp is called. This approach keeps the tests focused and fast, as they do not rely on the actual implementation of MyService.
Integration testing, on the other hand, involves testing multiple components together to ensure that they interact correctly. The Spring TestContext Framework provides support for loading the application context and injecting beans into the test cases. This allows for a more realistic testing environment where you can test the behavior of your application across multiple layers.
import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.test.context.SpringBootTest; import org.springframework.test.context.junit4.SpringRunner; @RunWith(SpringRunner.class) @SpringBootTest public class BookServiceIntegrationTest { @Autowired private BookController bookController; @Test public void testGetAllBooks() { List books = bookController.getAllBooks(); assertNotNull(books); assertTrue(books.isEmpty()); // Assuming the list is initially empty } }
Here, we use the @SpringBootTest annotation to load the full application context, allowing us to test the BookController in a more integrated manner. By autowiring the controller, we can directly invoke its methods to verify that it behaves as expected.
End-to-end tests, or system tests, validate the entire application from the user’s perspective. These tests often involve simulating user interactions with the application, validating that the various components work together seamlessly. Tools like Spring’s MockMvc allow developers to test the web layer by simulating HTTP requests and verifying the responses.
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.*; import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.*; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.test.autoconfigure.web.servlet.WebMvcTest; import org.springframework.test.web.servlet.MockMvc; @WebMvcTest(BookController.class) public class BookControllerTest { @Autowired private MockMvc mockMvc; @Test public void testGetAllBooks() throws Exception { mockMvc.perform(get("/books")) .andExpect(status().isOk()) .andExpect(content().contentType("application/json")); } }
This test uses MockMvc to perform a GET request to the /books endpoint and expects a successful response with a JSON content type. This kind of testing allows you to ensure that the entire web layer of your application is functioning correctly, including routing and response generation.
To promote best practices in testing Spring applications, developers should follow certain guidelines:
- Each test case should verify a single behavior or outcome.
- Test method names should clearly indicate what they are testing.
- Avoid dependencies on external systems like databases or APIs in unit tests.
- Common initialization code for tests can be placed in a setup method annotated with @Before or @BeforeEach.
- Integrate tests into a continuous integration pipeline to catch issues early.
By adhering to these principles and using the powerful testing tools provided by the Spring Framework, developers can create robust tests that enhance the reliability of their applications. Furthermore, effective testing significantly contributes to the maintainability of codebases, enabling teams to confidently implement changes and introduce new features over time.