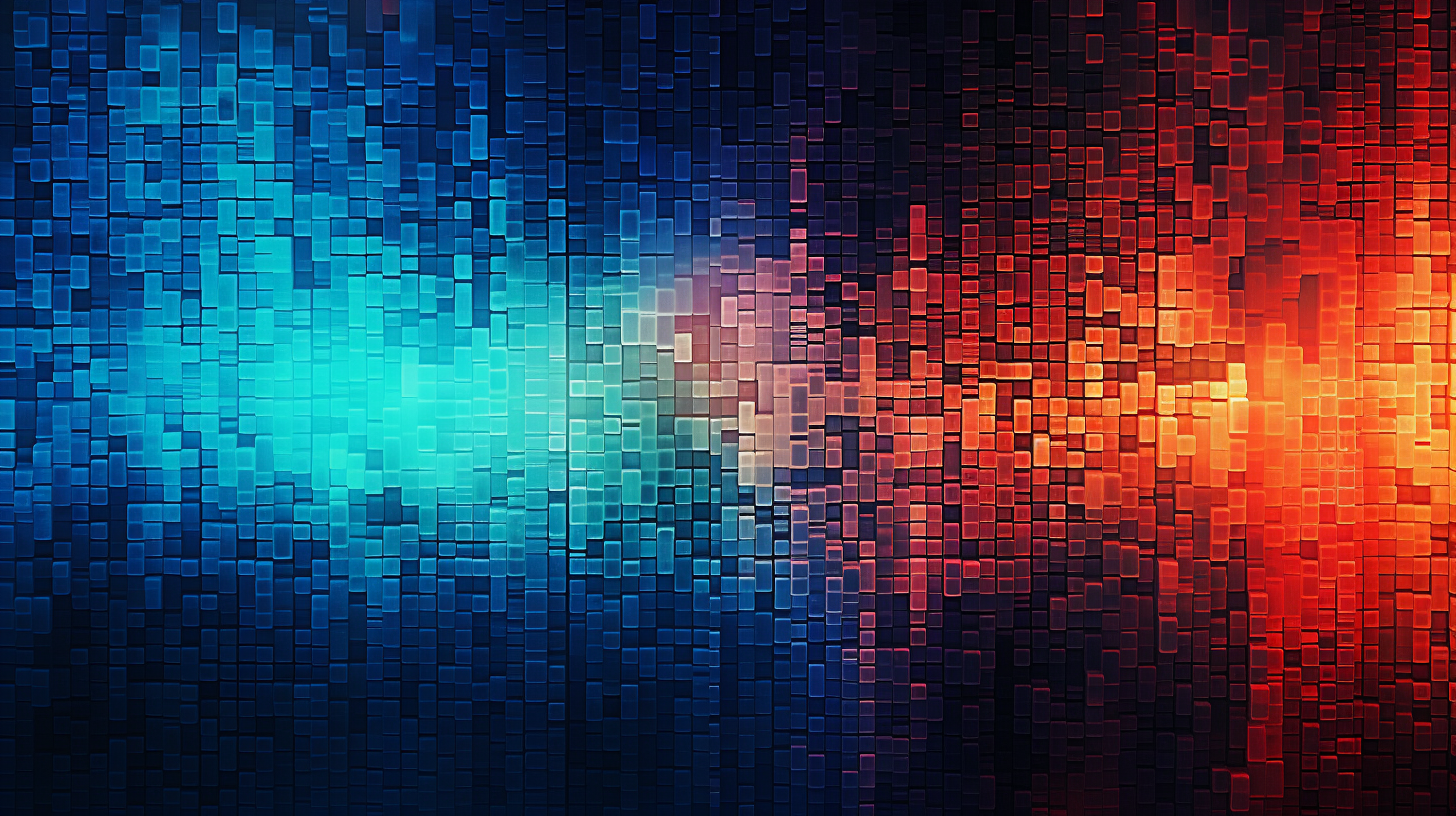
Java String Manipulation Techniques
Strings in Java are a fundamental data type, representing a sequence of characters. They’re encapsulated in the String class, which resides in the java.lang package, making it readily accessible without requiring any import statements. Understanding the basics of strings is essential for effective Java programming, as they’re widely used for handling text.
One of the first things to appreciate is that strings in Java are objects. This means that every string is instantiated from the String class, providing a rich set of methods to manipulate the character sequences. For example, to create a simple string, you can use the following syntax:
String greeting = "Hello, World!";
Strings can also be created using the new keyword, which can be useful in certain scenarios:
String farewell = new String("Goodbye, World!");
It’s important to note that strings in Java are immutable. This means once a string is created, its value cannot be changed. Any operation that seems to modify a string actually creates a new string object. This property can significantly impact performance and memory usage, especially in scenarios where strings are frequently modified.
For example, if you try to change the greeting string as follows:
greeting = greeting + " How are you?";
What actually happens is that a new string object is created, leaving the original greeting string intact. This can lead to unnecessary memory usage if not managed correctly, especially in loops or repeated operations.
To effectively work with mutable sequences of characters, Java provides the StringBuilder and StringBuffer classes. Both classes allow for modifications without the creation of new objects, which can enhance performance in scenarios requiring extensive string manipulation. Here’s a simple example of using StringBuilder:
StringBuilder sb = new StringBuilder("Hello"); sb.append(", World!"); String result = sb.toString(); // result is "Hello, World!"
Common String Methods and Their Applications
Java provides a rich set of methods within the String class that allows developers to manipulate and interact with strings in various ways. Familiarity with these methods can significantly enhance your coding efficiency and capability. Here, we’ll explore some of the most commonly used string methods and their practical applications.
One of the most frequently used methods is length(). This method returns the number of characters in a string. It’s a simpler yet essential tool for string processing:
String text = "Hello, World!"; int length = text.length(); // length is 13
Another critical method is charAt(int index), which allows you to access a specific character in a string based on its index. Remember, string indexing in Java is zero-based:
char firstChar = text.charAt(0); // firstChar is 'H'
To extract a substring, the substring(int beginIndex) and substring(int beginIndex, int endIndex) methods can be employed. These methods return a new string that’s a portion of the original string:
String sub = text.substring(7); // sub is "World!" String subRange = text.substring(0, 5); // subRange is "Hello"
Another useful method is indexOf(String str), which returns the index of the first occurrence of a specified substring. If the substring is not found, it returns -1:
int index = text.indexOf("World"); // index is 7
For string comparison, you can use equals(Object obj) to check if two strings are equal, or compareTo(String anotherString) to compare two strings lexicographically:
String str1 = "apple"; String str2 = "banana"; boolean isEqual = str1.equals("apple"); // isEqual is true int comparison = str1.compareTo(str2); // comparison is negative
String manipulation also includes operations like toUpperCase() and toLowerCase(), which convert the entire string to uppercase or lowercase, respectively:
String upper = text.toUpperCase(); // upper is "HELLO, WORLD!" String lower = text.toLowerCase(); // lower is "hello, world!"
Whitespace trimming is facilitated through the trim() method, which removes leading and trailing whitespaces:
String padded = " Hello, World! "; String trimmed = padded.trim(); // trimmed is "Hello, World!"
Finally, the replace(char oldChar, char newChar) method allows you to replace all occurrences of a specified character in the string:
String replaced = text.replace('o', '0'); // replaced is "Hell0, W0rld!"
String Immutability and Performance Considerations
Java’s approach to strings, particularly their immutability, plays a pivotal role in how developers manage performance and memory in their applications. String immutability means that once a string object is created, it cannot be altered. This design choice leads to several advantages, such as thread safety and predictable behavior, but it also introduces specific performance considerations that developers must navigate.
Every time a modification appears to be made to a string, Java actually creates a new string object. For instance, consider the following code snippet:
String message = "Hello"; message += ", World!";
In this example, the original string “Hello” remains unchanged, while a new string “Hello, World!” is created. This leads to increased memory consumption, especially if such operations are performed in loops or frequent method calls. The garbage collector must later reclaim those unused string objects, which can lead to additional performance overhead.
To mitigate these issues in scenarios involving frequent string modifications, the introduction of StringBuilder and StringBuffer is essential. Both classes provide mutable sequences of characters, allowing for efficient modifications without creating new objects. While both classes offer similar functionalities, it is worth noting that StringBuilder is unsynchronized and hence faster in a single-threaded environment, whereas StringBuffer is synchronized, making it safer in a multi-threaded context.
Here’s a quick illustration of how to use StringBuilder effectively:
StringBuilder sb = new StringBuilder("Hello"); sb.append(", World!"); String finalMessage = sb.toString(); // finalMessage is "Hello, World!"
By using StringBuilder, the performance cost associated with frequent string concatenations can be significantly reduced. This becomes especially beneficial in loops:
StringBuilder sb = new StringBuilder(); for (int i = 0; i < 1000; i++) { sb.append("Number: ").append(i).append("n"); } String result = sb.toString();
In the above loop, StringBuilder allows for numerous concatenations without creating a new string object each time, making it much more memory-efficient compared to using immutable strings.
It’s critical to choose the appropriate string handling mechanism based on your application’s requirements. In cases where immutability is beneficial—such as when strings are shared across multiple threads—using String is appropriate. However, when string manipulation is frequent and performance is a priority, opting for StringBuilder or StringBuffer will yield better results.
Advanced String Manipulation Techniques
When dealing with advanced string manipulation techniques in Java, developers can leverage a combination of built-in methods and specialized classes to manipulate strings efficiently. This goes beyond basic operations and dives into more nuanced string processing that can significantly streamline your code and improve performance in complex applications.
One of the common advanced techniques involves the use of the StringBuilder and StringBuffer classes for concatenation and modification. While these classes were briefly introduced earlier, it’s worth exploring some of their more advanced capabilities. For instance, the insert method allows you to add characters or strings at a specific index:
StringBuilder sb = new StringBuilder("Hello, World!"); sb.insert(7, "Java "); String result = sb.toString(); // result is "Hello, Java World!"
This feature can be particularly useful when dynamically constructing strings based on user input or other variables. Additionally, StringBuilder provides methods like delete and replace, which allow for efficient removal or replacement of content within the string:
sb.delete(5, 7); // Removes ", J" String replaced = sb.replace(7, 12, "Universe"); // result is "Hello, Universe!"
Another advanced technique involves using regular expressions for pattern matching and string manipulation. This can be particularly powerful when you need to validate input or extract specific data from strings. The Java Pattern and Matcher classes allow developers to compile regular expressions and perform operations like matching and replacing:
import java.util.regex.Pattern; import java.util.regex.Matcher; String input = "The rain in Spain stays mainly in the plain."; Pattern pattern = Pattern.compile("ain"); Matcher matcher = pattern.matcher(input); String modified = matcher.replaceAll("***"); // result is "The r*** in Sp*** stays m***ly in the pl***."
Regular expressions also enable sophisticated string validation techniques. For example, if you want to check if a string contains only digits, you can use the following snippet:
String numericInput = "123456"; boolean isNumeric = numericInput.matches("\d+"); // isNumeric is true
Using these advanced string manipulation techniques can lead to cleaner code, especially in applications requiring extensive text processing and validation. For example, ponder a scenario where you need to format a list of names, ensuring each is capitalized and separated by commas:
String[] names = {"john doe", "jane smith", "alice jones"}; StringBuilder formattedNames = new StringBuilder(); for (String name : names) { String capitalized = Character.toUpperCase(name.charAt(0)) + name.substring(1); formattedNames.append(capitalized).append(", "); } // Remove the trailing comma and space if (formattedNames.length() > 0) { formattedNames.setLength(formattedNames.length() - 2); } String result = formattedNames.toString(); // result is "John doe, Jane smith, Alice jones"
Regular Expressions for String Processing
Regular expressions are a powerful feature in Java that provides a way to work with text by defining search patterns. These patterns can be used for string matching, searching, replacing, and splitting operations. Mastering regular expressions can significantly enhance your ability to manipulate strings and validate input effectively.
In Java, the java.util.regex package contains the classes necessary to use regular expressions, primarily Pattern and Matcher. A Pattern is a compiled representation of a regular expression, and a Matcher is an engine that interprets the pattern and performs matching operations on a character sequence.
To create a pattern, you can use the Pattern.compile() method, which takes a string representation of a regular expression and returns a Pattern object. Here’s a simple example of using regular expressions to find all occurrences of a specific substring:
import java.util.regex.Pattern; import java.util.regex.Matcher; String input = "The quick brown fox jumps over the lazy dog. The dog was not quick."; Pattern pattern = Pattern.compile("quick"); Matcher matcher = pattern.matcher(input); while (matcher.find()) { System.out.println("Found at index: " + matcher.start()); }
In this example, we search for the word “quick” in the input string, and the program prints the starting index of each match found, demonstrating how you can locate specific text within a larger string.
Regular expressions also allow for potentially complex string matching tasks. For instance, if you want to validate whether a string is a valid email address, you can employ a regular expression that captures the required format:
String email = "[email protected]"; String emailRegex = "^[\w-\.]+@[\w-]+\.[a-z]{2,}$"; // Basic email pattern boolean isValidEmail = email.matches(emailRegex); // Returns true if valid System.out.println("Is valid email: " + isValidEmail);
This code snippet uses a simple regex pattern to check if the email string conforms to the expected format. The matches() method of the String class evaluates whether the entire string matches the pattern.
Moreover, you can utilize the replaceAll() method provided by the Matcher class to perform replacements based on regex patterns. This is extremely useful for data cleansing tasks. For instance, you might want to remove all non-digit characters from a string:
String dirtyInput = "Phone: (123) 456-7890"; Pattern digitsPattern = Pattern.compile("\D"); // Matches all non-digit characters Matcher digitsMatcher = digitsPattern.matcher(dirtyInput); String cleanInput = digitsMatcher.replaceAll(""); // Removes non-digit characters System.out.println("Clean input: " + cleanInput); // Outputs: "1234567890"
Regular expressions also allow you to split strings based on a delimiter. The split() method of the String class can take a regex pattern as a parameter. For example, splitting a CSV string into individual elements could look like this:
String csv = "apple,banana,cherry"; String[] fruits = csv.split(","); // Split by comma for (String fruit : fruits) { System.out.println(fruit.trim()); // Outputs each fruit, trimmed of whitespace }
In this scenario, the input string representing a list of fruits is split into an array, with each fruit processed individually. Using regex for splitting is advantageous when dealing with complex delimiters or patterns.
Best Practices for Efficient String Handling
When it comes to efficient string handling in Java, adhering to best practices can make a notable difference in both performance and code clarity. Strings are immensely versatile, but their immutable nature—and the operations performed on them—can introduce inefficiencies if not handled properly. Below are some strategies and insights that can help developers manage strings effectively.
First and foremost, ponder the context in which strings are used. If your application involves heavy string manipulation—such as concatenation within loops—using StringBuilder
or StringBuffer
is often a better choice than using the traditional String
class. These mutable classes allow appending and modifying characters without creating new string instances, thus improving performance. Here’s a quick example:
StringBuilder sb = new StringBuilder(); for (int i = 0; i < 1000; i++) { sb.append("Line ").append(i).append("n"); } String result = sb.toString();
Furthermore, when working with known sets of strings, ponder using constants. In Java, you can define strings as static final
to ensure they are reused rather than recreated. That’s especially useful in scenarios involving repetitive string literals:
public class Constants { public static final String ERROR_MESSAGE = "An error occurred."; }
This approach not only saves memory but also enhances maintainability, as you can easily update the value in one place without altering it throughout your codebase.
Another critical aspect of string handling is the use of proper string formatting instead of simple concatenation. The String.format()
method or the MessageFormat
class can be particularly useful for creating complex strings. This helps separate logic from presentation, making your code cleaner and easier to read:
String name = "Alice"; int age = 30; String formattedString = String.format("Name: %s, Age: %d", name, age);
In addition, when parsing strings, especially in cases involving user input or data from external sources, always validate and sanitize the input. This not only protects against potential errors but also ensures that your application behaves predictably. Consider using try-catch
blocks to handle exceptions that may arise from invalid input:
String input = "123abc"; try { int number = Integer.parseInt(input); } catch (NumberFormatException e) { System.out.println("Invalid number format."); }
Lastly, don’t overlook the advantages of using regular expressions in scenarios that require pattern matching or text validation. Regular expressions can simplify many string-processing tasks and improve the performance of complex string manipulations:
String email = "[email protected]"; if (email.matches("^[\w-\.]+@[\w-]+\.[a-z]{2,}$")) { System.out.println("Valid email format."); } else { System.out.println("Invalid email format."); }