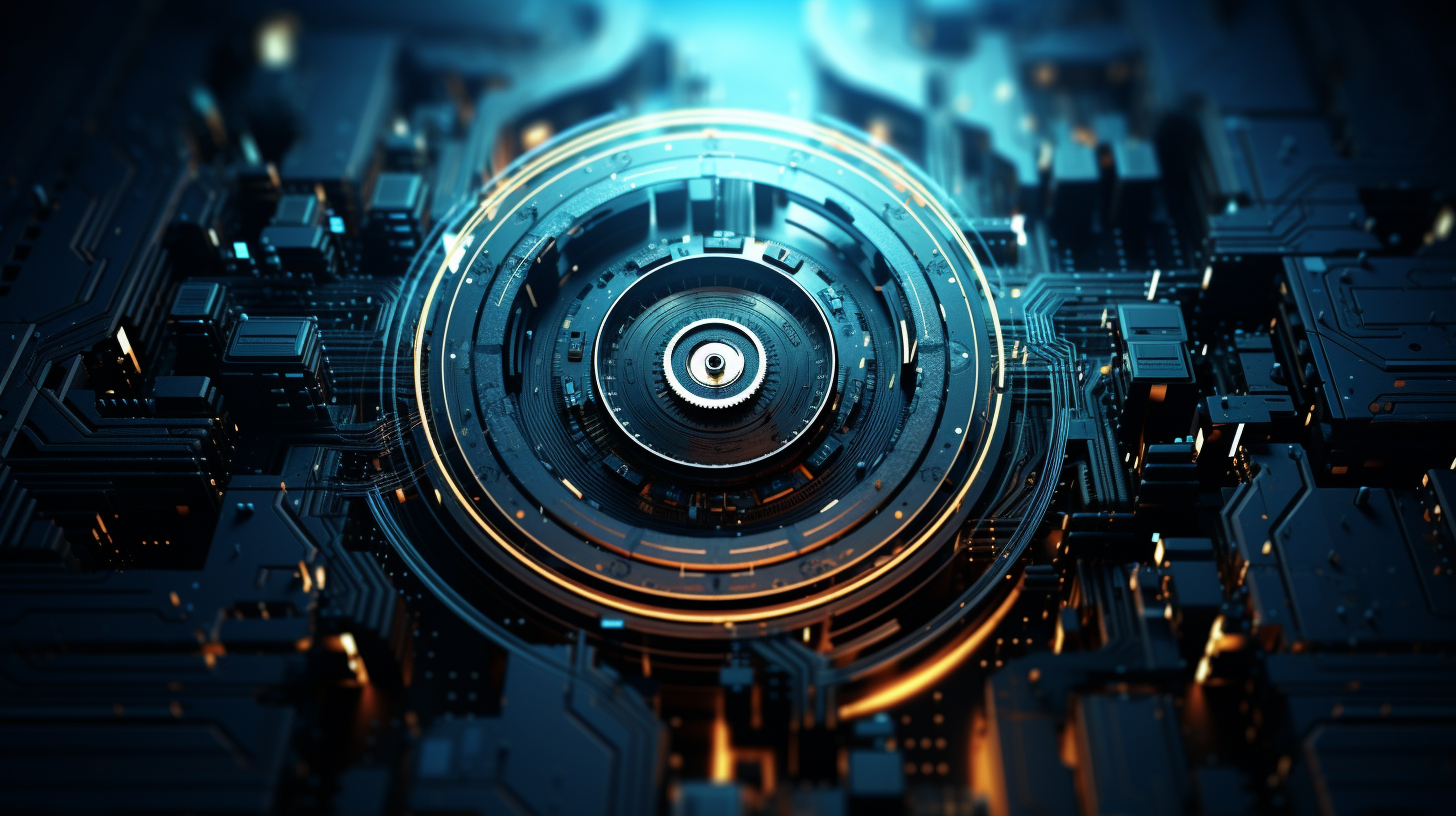
Java Swing for GUI Development
Java Swing is a powerful toolkit for building graphical user interfaces (GUIs) in Java applications. At the heart of Swing lies a well-defined architecture that allows developers to create flexible and dynamic user interfaces. Understanding this architecture is important for any Java developer aiming to harness the full potential of Swing.
Swing is built on the Model-View-Controller (MVC) design pattern, which separates the application’s data (the model), its user interface (the view), and the logic that ties the two together (the controller). This separation allows for a more organized and manageable codebase, enabling developers to make changes in one area without significantly impacting others.
The model in Swing represents the data and the business logic of the application. For instance, if you are building a simple text editor, the model would handle the text data, including its formatting and structure.
The view presents the model’s data to the user. In Swing, views are typically represented by various components like JButton
, JLabel
, and JTextField
. Each of these components is designed to display the model’s state and respond to user interactions.
The controller manages the communication between the model and the view. When a user interacts with a component, the controller responds to these events and updates the model or the view accordingly. In Swing, that’s often accomplished through event listeners that are attached to components.
Components in Swing are lightweight, meaning they’re implemented entirely in Java without relying heavily on the underlying operating system’s GUI components. This characteristic ensures a more consistent look and feel across different platforms. Swing components are also pluggable, allowing developers to customize or replace them to suit their application’s needs.
The entire architecture is layered, starting with the top-level containers such as JFrame
or JDialog
, which serve as the main windows for Swing applications. These containers hold various components and manage their layout and visibility. The layout managers play an important role in determining how components are arranged within these containers.
To illustrate the basic creation of a Swing application, ponder the following example:
import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JPanel; public class SimpleSwingApp { public static void main(String[] args) { // Create the top-level frame JFrame frame = new JFrame("Simple Swing Application"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // Create a panel to hold components JPanel panel = new JPanel(); // Create a button and add it to the panel JButton button = new JButton("Click Me!"); panel.add(button); // Add the panel to the frame frame.add(panel); // Set the frame size and make it visible frame.setSize(300, 200); frame.setVisible(true); } }
In this example, a simple JFrame is created, with a panel that contains a button. This basic structure exemplifies the MVC architecture, where the button serves as a view component, and the actions taken upon clicking it can be managed by an event listener, acting as the controller.
Understanding Java Swing architecture is the first step toward mastering GUI development in Java. By grasping the principles of MVC and the role of components, developers can create robust and efficient user interfaces that enhance the user’s experience.
Components and Layout Managers
Components are the building blocks of any Swing application. They represent the user interface elements that users interact with, such as buttons, text fields, labels, and tables. Each component in Swing is an instance of a class that extends the JComponent class, which provides a rich set of functionalities for rendering and handling user interactions.
In Swing, every component is a lightweight object, meaning that they’re drawn and managed by Java’s own graphics system rather than relying on the native GUI components of the host operating system. This leads to greater consistency in appearance and behavior across different platforms. However, this does not mean that Swing is devoid of performance. In fact, the lightweight nature of Swing components allows for high customization and ease of use, making them incredibly powerful for developers.
To organize these components within a container, Swing employs layout managers. Layout managers are responsible for determining the size and position of components within a container. They take care of the intricate details of how the components should be arranged, thereby allowing developers to focus more on the functionality of their applications rather than the specifics of positioning and sizing.
Swing provides several built-in layout managers, each serving different use cases. The most common ones are:
- Places components in a row, and when the row is filled, it moves to the next line.
- Divides the container into five regions: North, South, East, West, and Center. Components can be added to any of these regions.
- Arranges components in a grid of specified rows and columns.
- Aligns components either vertically or horizontally in a single column or row.
Here’s an example demonstrating how to use different layout managers in a Swing application:
import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JPanel; import java.awt.BorderLayout; import java.awt.FlowLayout; import java.awt.GridLayout; public class LayoutExample { public static void main(String[] args) { JFrame frame = new JFrame("Layout Manager Example"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setSize(400, 300); // Using FlowLayout JPanel flowPanel = new JPanel(new FlowLayout()); flowPanel.add(new JButton("Button 1")); flowPanel.add(new JButton("Button 2")); flowPanel.add(new JButton("Button 3")); // Using BorderLayout JPanel borderPanel = new JPanel(new BorderLayout()); borderPanel.add(new JButton("North"), BorderLayout.NORTH); borderPanel.add(new JButton("South"), BorderLayout.SOUTH); borderPanel.add(new JButton("East"), BorderLayout.EAST); borderPanel.add(new JButton("West"), BorderLayout.WEST); borderPanel.add(new JButton("Center"), BorderLayout.CENTER); // Using GridLayout JPanel gridPanel = new JPanel(new GridLayout(2, 2)); gridPanel.add(new JButton("1")); gridPanel.add(new JButton("2")); gridPanel.add(new JButton("3")); gridPanel.add(new JButton("4")); // Adding panels to frame frame.setLayout(new GridLayout(3, 1)); frame.add(flowPanel); frame.add(borderPanel); frame.add(gridPanel); frame.setVisible(true); } }
In this example, we create a JFrame and add three different panels, each demonstrating a different layout manager. The first panel uses FlowLayout, the second uses BorderLayout, and the third uses GridLayout. This illustrates how the layout manager controls the arrangement of the components, providing a clear visual structure to the user interface.
By understanding how to utilize components and layout managers effectively, developers can create aesthetically pleasing and user-friendly applications that adapt well to various screen sizes and resolutions. This flexibility is a key advantage of using Swing for Java GUI development.
Event Handling in Swing
In Swing, event handling is a critical aspect that enables applications to be interactive and responsive to user actions. The event handling mechanism in Swing revolves around a publish-subscribe model where components generate events, and listeners respond to these events. This allows for a clean separation of the application’s logic from the user interface elements. Understanding this mechanism is essential for creating dynamic and effortless to handle Java applications.
When a user interacts with a GUI component—by clicking a button, typing in a text field, or selecting an item from a list—an event is generated. Swing provides a rich set of event classes that correspond to different types of user interactions. For instance, ActionEvent
is used for button clicks, while MouseEvent
is employed for mouse movements and clicks.
To handle these events, developers need to implement event listeners. Each event listener is an interface that defines methods corresponding to the events it’s designed to handle. For example, the ActionListener
interface contains a single method, actionPerformed(ActionEvent e)
, which is invoked when an action event occurs.
Here’s a simple example demonstrating how to use an ActionListener
to respond to button clicks:
import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JPanel; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; public class EventHandlingExample { public static void main(String[] args) { JFrame frame = new JFrame("Event Handling Example"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setSize(300, 200); JPanel panel = new JPanel(); JButton button = new JButton("Click Me!"); // Adding ActionListener to the button button.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { System.out.println("Button was clicked!"); } }); panel.add(button); frame.add(panel); frame.setVisible(true); } }
In this example, an ActionListener
is added to the button. When the button is clicked, the actionPerformed
method is called, which in this case simply prints a message to the console. This illustrates the core concept of event handling: responding to user actions by executing relevant code.
In addition to ActionListener
, Swing provides several other listener interfaces for handling different types of events. These include:
- For handling mouse events like clicks, enters, and exits.
- For responding to keyboard events.
- For handling events related to the window, such as opening, closing, and minimizing.
Each of these listeners follows a similar pattern: they define specific methods that must be implemented to respond to the respective events. This modular approach allows developers to create clear and maintainable code.
For more complex applications, you might find it beneficial to use lambda expressions (available since Java 8) to reduce boilerplate code when creating listeners:
import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JPanel; public class LambdaEventHandlingExample { public static void main(String[] args) { JFrame frame = new JFrame("Lambda Event Handling Example"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setSize(300, 200); JPanel panel = new JPanel(); JButton button = new JButton("Click Me!"); // Using a lambda expression for ActionListener button.addActionListener(e -> System.out.println("Button was clicked!")); panel.add(button); frame.add(panel); frame.setVisible(true); } }
In this version, a lambda expression is used to handle the button click event, which results in cleaner and more concise code.
By mastering event handling in Swing, developers can create applications that are not only functional but also engaging and intuitive for users. The ability to respond to user interactions effectively is what transforms a static GUI into a dynamic and interactive experience.
Creating Custom Components
Creating custom components in Java Swing is a powerful way to extend the toolkit’s capabilities and tailor the user interface to your specific needs. While Swing provides a rich set of built-in components, there are times when those components may not fulfill all requirements. In such cases, building your own components allows you to encapsulate unique behavior and presentation in a reusable package.
Custom components in Swing are typically created by subclassing one of the existing Swing components, usually from JComponent
. This approach gives you access to all the capabilities of the Swing painting system, event handling, and layout management.
When you create a custom component, you need to override certain methods to control how the component is rendered and how it responds to user interactions. The two key methods that you will often override are paintComponent(Graphics g)
and getPreferredSize()
.
Below is an example of a simple custom component that draws a colored rectangle and displays a message:
import javax.swing.JComponent; import javax.swing.JFrame; import javax.swing.JPanel; import java.awt.Color; import java.awt.Dimension; import java.awt.Graphics; public class CustomComponentExample extends JComponent { private String message; private Color color; public CustomComponentExample(String message, Color color) { this.message = message; this.color = color; } @Override protected void paintComponent(Graphics g) { super.paintComponent(g); g.setColor(color); g.fillRect(10, 10, getWidth() - 20, getHeight() - 20); g.setColor(Color.BLACK); g.drawString(message, 20, 30); } @Override public Dimension getPreferredSize() { return new Dimension(200, 100); } public static void main(String[] args) { JFrame frame = new JFrame("Custom Component Example"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setSize(300, 200); CustomComponentExample customComponent = new CustomComponentExample("Hello, Custom Component!", Color.CYAN); JPanel panel = new JPanel(); panel.add(customComponent); frame.add(panel); frame.setVisible(true); } }
In this example, the CustomComponentExample
class extends JComponent
. In the paintComponent
method, we first call the superclass’s method to ensure proper rendering. We then set the color, fill a rectangle, and draw a string. The getPreferredSize
method provides the component’s preferred dimensions, ensuring that layout managers allocate adequate space for the component.
Custom components can also be interactive. You can add mouse listeners or key listeners to handle user input. Here’s how you might modify the previous example to change the color of the rectangle when the user clicks on the component:
import javax.swing.JComponent; import javax.swing.JFrame; import javax.swing.JPanel; import java.awt.Color; import java.awt.Dimension; import java.awt.Graphics; import java.awt.event.MouseAdapter; import java.awt.event.MouseEvent; public class InteractiveCustomComponent extends JComponent { private String message; private Color color; public InteractiveCustomComponent(String message) { this.message = message; this.color = Color.CYAN; // Initial color // Adding mouse listener to handle clicks addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { color = new Color((int)(Math.random() * 0x1000000)); // Change to a random color repaint(); // Request a repaint to update the display } }); } @Override protected void paintComponent(Graphics g) { super.paintComponent(g); g.setColor(color); g.fillRect(10, 10, getWidth() - 20, getHeight() - 20); g.setColor(Color.BLACK); g.drawString(message, 20, 30); } @Override public Dimension getPreferredSize() { return new Dimension(200, 100); } public static void main(String[] args) { JFrame frame = new JFrame("Interactive Custom Component Example"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setSize(300, 200); InteractiveCustomComponent customComponent = new InteractiveCustomComponent("Click me to change color!"); JPanel panel = new JPanel(); panel.add(customComponent); frame.add(panel); frame.setVisible(true); } }
In this version, we add a MouseListener
to respond to clicks. When the component is clicked, it generates a new random color and calls repaint()
to request a redraw of the component. This encapsulation of behavior makes the custom component not only visually appealing but also interactive, enhancing user engagement.
Creating custom components empowers developers to craft unique interfaces that can stand apart from standard components. By using Swing’s painting methods and event handling mechanisms, you can build a comprehensive range of user interface elements tailored to the specific demands of your application. This approach not only fosters creativity but also offers the flexibility to evolve the GUI as user requirements change.
Styling and Theming Swing Applications
Styling and theming in Java Swing applications is an essential aspect that allows developers to create visually appealing and cohesive user interfaces. Although the default look and feel of Swing components is functional, customizing their appearance can significantly enhance the user experience. Swing provides a flexible framework that enables developers to apply styles and themes consistently across the application while maintaining performance and usability.
One of the primary ways to customize the appearance of Swing components is by using the Look and Feel (L&F) architecture. Swing supports various built-in L&F options, such as Metal, Nimbus, and System look and feel, which allows applications to conform to the native appearance of the operating system. Additionally, developers can create their custom L&F or modify existing ones, providing complete control over the aesthetics of the application.
To apply a different look and feel, you can use the UIManager class. Here’s an example illustrating how to set the Nimbus look and feel:
import javax.swing.UIManager; import javax.swing.SwingUtilities; import javax.swing.JFrame; import javax.swing.JButton; public class LookAndFeelExample { public static void main(String[] args) { SwingUtilities.invokeLater(() -> { try { UIManager.setLookAndFeel("javax.swing.plaf.nimbus.NimbusLookAndFeel"); } catch (Exception e) { e.printStackTrace(); } JFrame frame = new JFrame("Look and Feel Example"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); JButton button = new JButton("Click Me!"); frame.add(button); frame.setSize(300, 200); frame.setVisible(true); }); } }
In this example, we set the Nimbus look and feel before creating and displaying the main frame. The Nimbus L&F offers a modern appearance with gradients and rounded corners, enhancing the overall look of the application.
Beyond changing the overall look and feel, developers can customize individual components using UIManager properties. Each component has a set of properties that can be modified through the UIManager. For instance, you can alter the background color, foreground color, font, and borders of components. Here’s how to customize a JButton:
import javax.swing.UIManager; import javax.swing.SwingUtilities; import javax.swing.JButton; import javax.swing.JFrame; import java.awt.Color; public class CustomButtonExample { public static void main(String[] args) { SwingUtilities.invokeLater(() -> { try { UIManager.put("Button.background", Color.CYAN); UIManager.put("Button.foreground", Color.BLACK); UIManager.put("Button.font", UIManager.getFont("Button.font").deriveFont(16f)); } catch (Exception e) { e.printStackTrace(); } JFrame frame = new JFrame("Custom Button Example"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); JButton button = new JButton("Custom Button"); frame.add(button); frame.setSize(300, 200); frame.setVisible(true); }); } }
In this code, we modify the background and foreground colors of the JButton, as well as change its font size. Any button created after these properties are set will reflect these changes, allowing for a consistent look throughout the application.
Moreover, developers can create custom painting to further enhance the visual appearance of components. By overriding the paintComponent method in a custom component, you can draw shapes, images, or even gradients. This allows you to create unique visual elements that align with the overall theme of your application. For instance:
import javax.swing.JComponent; import javax.swing.JFrame; import java.awt.Color; import java.awt.Graphics; public class CustomPanel extends JComponent { @Override protected void paintComponent(Graphics g) { super.paintComponent(g); g.setColor(Color.BLUE); g.fillRect(10, 10, 200, 100); g.setColor(Color.WHITE); g.drawString("Custom Painted Panel", 20, 50); } public static void main(String[] args) { JFrame frame = new JFrame("Custom Painting Example"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.add(new CustomPanel()); frame.setSize(250, 150); frame.setVisible(true); } }
In this example, we create a custom panel that paints a blue rectangle and writes a string on it. By encapsulating this behavior in a custom component, you have full control over the visual output, which is especially valuable when creating themed applications.
Finally, consider using external libraries like FlatLaf or Substance that offer additional styling capabilities and themes. These libraries provide pre-defined themes and an easy way to customize components without the complexity of writing extensive custom code.
Styling and theming are vital components of creating a visually attractive Swing application. By using the Look and Feel architecture, customizing UIManager properties, and using custom painting techniques, developers can craft a user interface that not only meets functional requirements but also captivates users with its aesthetic appeal.
Best Practices for Swing Development
When developing with Java Swing, adhering to best practices can significantly improve the quality, maintainability, and user experience of your applications. These practices not only enhance the performance of your GUI but also simplify the development process and make your code more manageable. Below are several key best practices to keep in mind:
1. Follow the MVC Pattern
As Swing is designed around the Model-View-Controller (MVC) architecture, ensure that you properly separate your application’s data, user interface, and control logic. This separation allows for easier management of code changes and enhances reusability. Make each component self-contained, handling only its specific responsibilities. For example, your model should handle data and business logic, while your view should focus on presenting that data.
2. Use Layout Managers Wisely
Leverage Swing’s layout managers to create flexible and responsive interfaces. Avoid using absolute positioning, as it can lead to poor user experience on different screen sizes and resolutions. Instead, choose the appropriate layout manager that best suits your needs, whether it be FlowLayout
, BorderLayout
, or GridLayout
. Proper use of layout managers ensures that your application looks good across various platforms.
import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JPanel; import java.awt.BorderLayout; public class LayoutBestPractice { public static void main(String[] args) { JFrame frame = new JFrame("Best Practice Layout"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setSize(300, 200); JPanel panel = new JPanel(new BorderLayout()); panel.add(new JButton("North"), BorderLayout.NORTH); panel.add(new JButton("Center"), BorderLayout.CENTER); panel.add(new JButton("South"), BorderLayout.SOUTH); frame.add(panel); frame.setVisible(true); } }
3. Optimize Event Handling
Minimize the complexity of event handling by keeping the listener code concise and efficient. Use lambda expressions for simplicity where applicable, especially for single-use listeners. This reduces boilerplate code and increases readability. Moreover, avoid long-running tasks in the event dispatch thread (EDT). Instead, think using SwingWorker
for background processing to keep the UI responsive.
import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JPanel; import javax.swing.SwingWorker; public class EventHandlingBestPractice { public static void main(String[] args) { JFrame frame = new JFrame("Efficient Event Handling"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); JPanel panel = new JPanel(); JButton button = new JButton("Start Task"); button.addActionListener(e -> { new SwingWorker() { @Override protected Void doInBackground() { // Simulate long task try { Thread.sleep(2000); } catch (InterruptedException ex) { ex.printStackTrace(); } return null; } @Override protected void done() { System.out.println("Task completed!"); } }.execute(); }); panel.add(button); frame.add(panel); frame.setSize(300, 200); frame.setVisible(true); } }
4. Keep GUI Updates on the EDT
Ensure that all updates to the GUI are executed on the Event Dispatch Thread (EDT). Swing is not thread-safe, and modifying GUI components from outside the EDT can lead to unpredictable behavior. Use SwingUtilities.invokeLater()
to schedule GUI updates safely.
import javax.swing.*; public class EDTExample { public static void main(String[] args) { SwingUtilities.invokeLater(() -> { JFrame frame = new JFrame("EDT Example"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.add(new JLabel("This is safe!")); frame.setSize(300, 200); frame.setVisible(true); }); } }
5. Use Resource Bundles for Localization
For applications that need to support multiple languages, use Java’s ResourceBundle
class to manage text and UI strings. This practice makes your application more adaptable and easier to maintain when adding new languages. By externalizing strings, you can easily swap out language files without changing your codebase.
import javax.swing.*; import java.util.ResourceBundle; public class LocalizationExample { public static void main(String[] args) { ResourceBundle messages = ResourceBundle.getBundle("MessagesBundle"); JFrame frame = new JFrame(messages.getString("frame.title")); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.add(new JLabel(messages.getString("label.message"))); frame.setSize(300, 200); frame.setVisible(true); } }
6. Maintain Consistent Styling and Theming
Ensure that your application presents a uniform look and feel. Use consistent fonts, colors, and component sizes throughout the application. Utilize the UIManager to set global properties, making it easier to manage changes and maintain a cohesive appearance. By doing so, you enhance the user experience, making the application intuitive and visually appealing.
7. Document Your Code
As with any coding project, clear documentation especially important. Comment your code adequately, particularly around complex event handling or custom components. Good documentation will aid not only your future self but also any other developers who might work on your project.
Incorporating these best practices into your Java Swing development process will lead to more robust, maintainable, and efficient applications. By focusing on good architecture, responsive design, and user-friendly interfaces, you can create powerful GUI applications that stand out in both functionality and presentation.