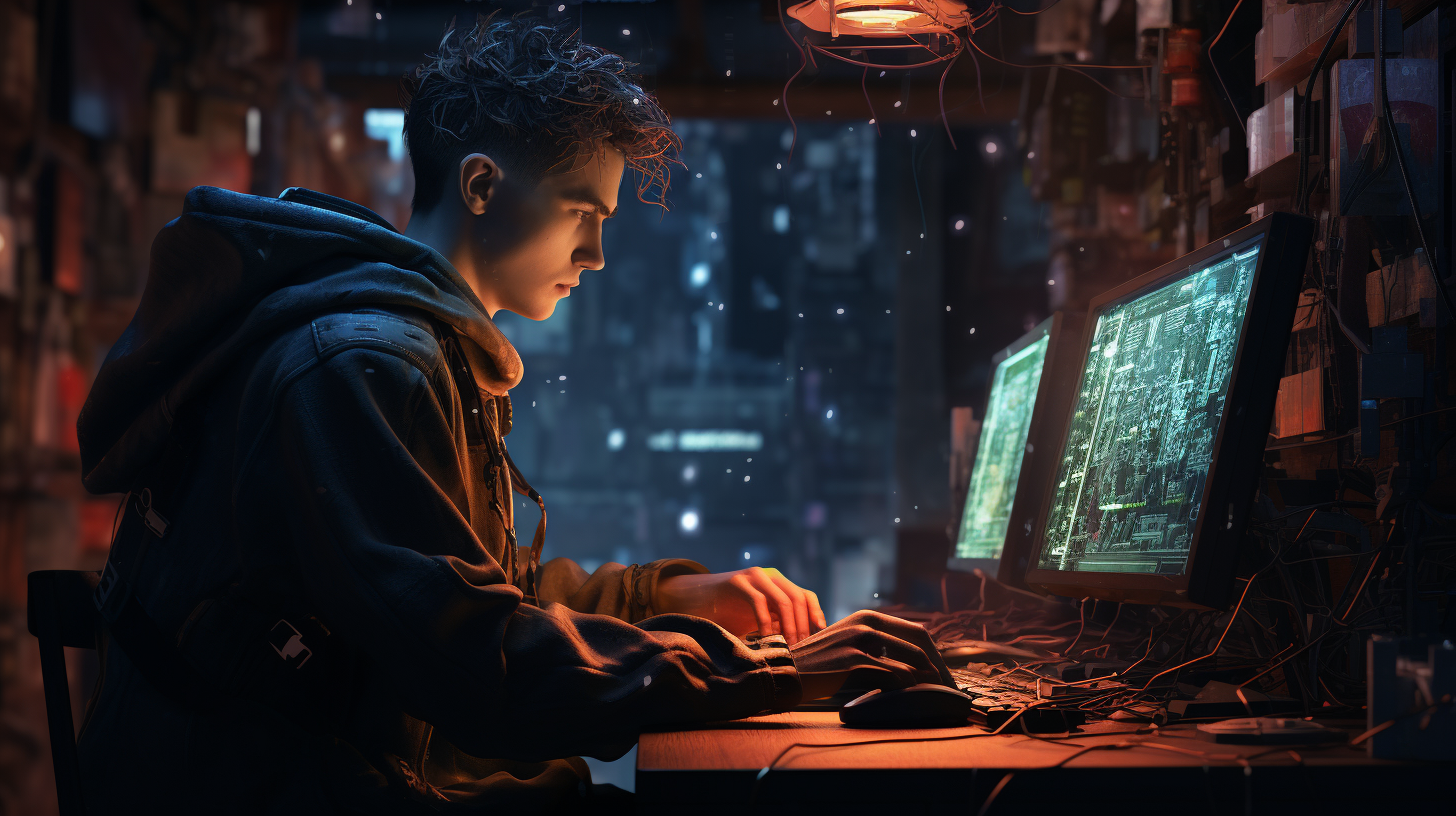
JavaScript and Audio Processing
The Web Audio API is a powerful tool that brings high-level audio processing capabilities to web applications. It is designed for the manipulation and synthesis of audio in real time, making it an excellent choice for developing interactive audio experiences, such as games and music applications. This API offers a wide range of functionalities, from simple sound playback to complex audio analyses and manipulation.
At its core, the Web Audio API operates on the notion of audio nodes. These nodes are the building blocks of the audio processing graph, where each node represents a distinct audio source, effect, or destination. The beauty of the API lies in its ability to connect these nodes together, allowing developers to create intricate audio processing chains.
When using the Web Audio API, you typically start by creating an AudioContext, which acts as the main interface for managing and controlling the audio operations. With the AudioContext in place, you’ll be able to create various types of audio nodes, such as OscillatorNode, GainNode, and AnalyserNode.
Here’s a simple example demonstrating how to create an audio context and an oscillator node:
const audioContext = new (window.AudioContext || window.webkitAudioContext)(); const oscillator = audioContext.createOscillator(); oscillator.type = 'sine'; // You can also use 'square', 'sawtooth', or 'triangle' oscillator.frequency.setValueAtTime(440, audioContext.currentTime); // A4 note oscillator.connect(audioContext.destination); oscillator.start(); // Starts the oscillator oscillator.stop(audioContext.currentTime + 2); // Stops the oscillator after 2 seconds
This example sets up a basic sine wave oscillator at a frequency of 440 Hz, which corresponds to the musical note A4. The oscillator is connected to the destination node, which is usually the speakers or headphones, and it plays sound for a duration of two seconds.
The flexibility of the Web Audio API enables developers to implement advanced audio features. For instance, you can create effects like reverb and delay, visualize audio data, and even analyze sound frequencies in real time. All of this is achievable by chaining nodes and controlling their parameters programmatically, offering unmatched control over audio playback and manipulation in web applications.
Moreover, the API is designed with performance in mind, allowing developers to process audio efficiently without compromising the user experience. By offloading tasks to the Web Audio API, you can ensure that your web applications remain responsive even when handling complex audio processing tasks.
As you delve deeper into the Web Audio API, you will discover a world of possibilities for crafting immersive audio experiences that engage users and enhance the overall interactivity of your web applications.
Creating and Manipulating Audio Nodes
To create a more complex audio processing graph, you can combine multiple audio nodes. The nodes can be connected in various ways to achieve different effects and sound manipulations. For example, if you want to include a GainNode to control the volume of the oscillator, you can easily do so. The GainNode allows you to increase or decrease the amplitude of the audio signal flowing through it.
Here’s how you can implement this:
const audioContext = new (window.AudioContext || window.webkitAudioContext)(); const oscillator = audioContext.createOscillator(); const gainNode = audioContext.createGain(); oscillator.type = 'sine'; oscillator.frequency.setValueAtTime(440, audioContext.currentTime); // A4 note gainNode.gain.setValueAtTime(0.5, audioContext.currentTime); // Set initial volume to 50% // Connect the nodes: oscillator -> gainNode -> destination oscillator.connect(gainNode); gainNode.connect(audioContext.destination); oscillator.start(); // Start the oscillator oscillator.stop(audioContext.currentTime + 2); // Stop after 2 seconds
In this code snippet, we first create an oscillator and a GainNode. The gainNode’s gain property is set to 0.5, which reduces the volume to 50%. By connecting the oscillator to the gainNode, and then connecting the gainNode to the destination, we effectively control the volume of the audio output.
Beyond simple oscillation and gain adjustments, you can employ an AnalyserNode to visualize audio data in real time. An AnalyserNode provides real-time frequency and time-domain analysis of the audio signal. It can output frequency data and waveform data, which can be used to create visual representations of the audio being played.
Here’s an example of how to integrate an AnalyserNode into your audio processing graph:
const audioContext = new (window.AudioContext || window.webkitAudioContext)(); const oscillator = audioContext.createOscillator(); const gainNode = audioContext.createGain(); const analyser = audioContext.createAnalyser(); oscillator.type = 'sine'; oscillator.frequency.setValueAtTime(440, audioContext.currentTime); // A4 note gainNode.gain.setValueAtTime(0.5, audioContext.currentTime); // Set volume to 50% // Connect the nodes: oscillator -> gainNode -> analyser -> destination oscillator.connect(gainNode); gainNode.connect(analyser); analyser.connect(audioContext.destination); oscillator.start(); // Start the oscillator oscillator.stop(audioContext.currentTime + 2); // Stop after 2 seconds
With this setup, the audio signal flows from the oscillator through the gainNode, then into the analyser, and finally to the output destination. This allows you to monitor and visualize the audio signal, providing insights into its frequency components. You can implement visualization techniques, such as drawing the waveform or frequency bars on a canvas element, to create dynamic audio visualizations.
These examples highlight the modularity and versatility of the Web Audio API. By creating and connecting various audio nodes, you can shape audio in ways that suit your application’s needs. As you build more intricate audio graphs, you unlock a treasure trove of creative possibilities, from simple sound effects to complex audio environments that respond dynamically to user interactions.
Real-Time Audio Processing Techniques
When diving into real-time audio processing techniques, the Web Audio API provides a plethora of options for manipulating audio signals on the fly. The key to using these capabilities lies in understanding how to effectively utilize audio nodes and control their parameters in real time.
One of the fundamental techniques in real-time audio processing is the use of modulation. Modulation allows you to alter an audio signal’s properties dynamically, creating rich and evolving soundscapes. For instance, you can modulate the frequency of an oscillator using another oscillator. That’s often referred to as Frequency Modulation (FM) synthesis, a classic technique that adds depth and complexity to sound.
const audioContext = new (window.AudioContext || window.webkitAudioContext)(); const carrierOscillator = audioContext.createOscillator(); const modulatorOscillator = audioContext.createOscillator(); const gainNode = audioContext.createGain(); // Set up the carrier oscillator carrierOscillator.type = 'sine'; carrierOscillator.frequency.setValueAtTime(440, audioContext.currentTime); // A4 note // Set up the modulator oscillator modulatorOscillator.type = 'sine'; modulatorOscillator.frequency.setValueAtTime(220, audioContext.currentTime); // 220 Hz frequency for modulation gainNode.gain.setValueAtTime(100, audioContext.currentTime); // Modulation depth // Connect modulator to gainNode, then to carrier modulatorOscillator.connect(gainNode); gainNode.connect(carrierOscillator.frequency); // Modulating the carrier frequency // Connect carrier to destination carrierOscillator.connect(audioContext.destination); // Start both oscillators modulatorOscillator.start(); carrierOscillator.start(); // Stop after 2 seconds modulatorOscillator.stop(audioContext.currentTime + 2); carrierOscillator.stop(audioContext.currentTime + 2);
In this code, we create two oscillators: a carrier and a modulator. The carrier oscillator produces the main audio signal, while the modulator oscillator alters the frequency of the carrier. By connecting the modulator’s output to the gain node, which controls the modulation depth, you can achieve rich, evolving tones typical of FM synthesis.
Another technique for real-time audio processing is applying effects using various audio nodes. For example, a ConvolverNode can be used to simulate the acoustic characteristics of a physical space by applying impulse responses to your audio. This can create immersive environments and enhance your audio experience significantly.
const audioContext = new (window.AudioContext || window.webkitAudioContext)(); const convolver = audioContext.createConvolver(); // Load an impulse response fetch('path/to/impulse-response.wav') .then(response => response.arrayBuffer()) .then(data => audioContext.decodeAudioData(data)) .then(buffer => { convolver.buffer = buffer; // Set the impulse response }); const source = audioContext.createBufferSource(); // Load a sound to be convolved fetch('path/to/sound.wav') .then(response => response.arrayBuffer()) .then(data => audioContext.decodeAudioData(data)) .then(buffer => { source.buffer = buffer; // Connect source to convolver, then to destination source.connect(convolver); convolver.connect(audioContext.destination); source.start(); // Start the sound });
In this example, the ConvolverNode processes an audio source by applying an impulse response, which simulates how sound behaves in a specific acoustic environment. This technique can dramatically shape your audio’s characteristics, making it sound as if it’s being played in a concert hall, a small room, or any other space.
Additionally, implementing real-time user interaction with audio processing can lead to truly engaging experiences. By using the Web Audio API in conjunction with user input, you can modify audio parameters based on user actions. For instance, you can change volume, pitch, or effects using sliders or buttons in your web interface.
const volumeSlider = document.getElementById('volumeSlider'); volumeSlider.addEventListener('input', (event) => { gainNode.gain.setValueAtTime(event.target.value, audioContext.currentTime); });
Here, we attach an event listener to a volume slider input, allowing users to control the gain node’s gain dynamically. This interactive approach not only enhances user engagement but also adds a layer of personalization to the audio experience.
As you explore these real-time audio processing techniques further, consider combining different methods—modulation, effects, and user interactions—to create unique and compelling audio experiences that captivate your audience.
Integrating Audio with Visuals
Integrating audio with visuals is a powerful way to enhance user engagement in web applications. By synchronizing sound with visual elements, you can create immersive experiences that resonate with users on multiple sensory levels. The Web Audio API, combined with the Canvas API or WebGL, offers a multitude of possibilities for such integration.
One common approach is to visualize audio data in real time. For instance, you can display a waveform or a frequency spectrum that dynamically reacts to the audio being played. This can be accomplished using an AnalyserNode to access frequency data and drawing it onto a canvas element. Below is a simple example of how to set up such a visualization:
const audioContext = new (window.AudioContext || window.webkitAudioContext)(); const analyser = audioContext.createAnalyser(); const canvas = document.getElementById('canvas'); const canvasContext = canvas.getContext('2d'); const oscillator = audioContext.createOscillator(); oscillator.type = 'sine'; oscillator.frequency.setValueAtTime(440, audioContext.currentTime); // A4 note oscillator.connect(analyser); analyser.connect(audioContext.destination); oscillator.start(); // Start the oscillator // Visualization function function draw() { requestAnimationFrame(draw); const dataArray = new Uint8Array(analyser.frequencyBinCount); analyser.getByteFrequencyData(dataArray); // Clear the canvas canvasContext.fillStyle = 'rgb(200, 200, 200)'; canvasContext.fillRect(0, 0, canvas.width, canvas.height); const barWidth = (canvas.width / dataArray.length) * 2.5; let barHeight; let x = 0; for (let i = 0; i < dataArray.length; i++) { barHeight = dataArray[i]; canvasContext.fillStyle = 'rgb(' + (barHeight + 100) + ',50,50)'; canvasContext.fillRect(x, canvas.height - barHeight / 2, barWidth, barHeight / 2); x += barWidth + 1; } } // Start the visualization draw(); oscillator.stop(audioContext.currentTime + 2); // Stop after 2 seconds
In this example, we create an oscillator and connect it to an AnalyserNode, which provides real-time frequency data. The draw function uses the canvas API to render a simple bar graph that visualizes the frequency spectrum of the audio signal. Each bar’s height corresponds to the amplitude of a specific frequency band, creating a dynamic response to the audio playing.
Furthermore, you can enhance your visualizations by integrating user interactions. For example, you might change the visual style based on user input or sync visual effects to the audio’s beat. You can listen for audio events and trigger animations or visual changes that correspond to the music’s rhythm or intensity. Here’s a simpler implementation that changes the canvas background color based on the audio’s amplitude:
const volumeThreshold = 100; // Threshold for visual change function draw() { requestAnimationFrame(draw); const dataArray = new Uint8Array(analyser.frequencyBinCount); analyser.getByteFrequencyData(dataArray); const average = dataArray.reduce((sum, value) => sum + value) / dataArray.length; // Change background color based on amplitude const bgColor = average > volumeThreshold ? 'rgb(255, 0, 0)' : 'rgb(50, 50, 50)'; canvasContext.fillStyle = bgColor; canvasContext.fillRect(0, 0, canvas.width, canvas.height); const barWidth = (canvas.width / dataArray.length) * 2.5; let barHeight; let x = 0; for (let i = 0; i < dataArray.length; i++) { barHeight = dataArray[i]; canvasContext.fillStyle = 'rgb(' + (barHeight + 100) + ',50,50)'; canvasContext.fillRect(x, canvas.height - barHeight / 2, barWidth, barHeight / 2); x += barWidth + 1; } } draw(); oscillator.stop(audioContext.currentTime + 2); // Stop after 2 seconds
Here, the average frequency amplitude is computed, and if it exceeds a certain threshold, the background color of the canvas changes, creating a striking visual cue that corresponds to the audio dynamics. This not only makes the audio experience more engaging but also adds an element of interactivity that can captivate users.
In addition to static visualizations, you can also create animations driven by audio input. For instance, you can animate elements on the screen to move, change size, or morph based on the audio’s properties. By using the Web Audio API’s capabilities in conjunction with CSS animations and transformations, you can create truly compelling audiovisual experiences.
By combining audio and visuals, you not only enhance the overall quality of your web applications but also create a richer, more engaging experience for users. The potential for creativity is immense, limited only by your imagination and the tools at your disposal within the realm of the Web Audio API and the browser’s graphics capabilities.
Best Practices for Performance Optimization
When optimizing for performance in audio applications using the Web Audio API, developers must be acutely aware of how audio processing can impact the responsiveness and overall user experience of their web applications. Here are some key strategies for ensuring optimal performance while working with audio.
1. Minimize Audio Context Creation: Creating an AudioContext is a resource-intensive operation. Therefore, it’s advisable to create a single AudioContext and reuse it throughout your application rather than generating new instances. This not only conserves resources but also helps to maintain seamless playback without interruptions.
const audioContext = new (window.AudioContext || window.webkitAudioContext)();
2. Use OfflineAudioContext for Pre-Processing: An OfflineAudioContext allows you to render audio in a non-real-time context. That is particularly beneficial for complex audio processing tasks that do not require immediate output to the user. You can prepare your audio data ahead of time and then play it back using a regular AudioContext. This method significantly reduces CPU load during playback.
const offlineContext = new OfflineAudioContext(2, audioContext.sampleRate * 2, audioContext.sampleRate); // Perform audio processing with offlineContext here
3. Control Node Connections Efficiently: Be mindful of how nodes are connected in your audio graph. Unnecessary connections can lead to increased CPU usage. Make sure to disconnect nodes that are no longer in use and avoid creating excessive nodes. For instance, if you have multiple audio sources, ensure they’re only connected when needed.
function cleanUpNode(node) { if (node) { node.disconnect(); } }
4. Use Audio Buffers Wisely: Loading audio files can incur latency, especially if you frequently load them during playback. Instead, use AudioBuffer objects to store audio data in memory once it has been loaded. This allows for instant playback without the overhead of repeatedly fetching audio data.
let audioBuffer; // Load audio data into audioBuffer here // Use audioBuffer for playback const source = audioContext.createBufferSource(); source.buffer = audioBuffer; source.connect(audioContext.destination); source.start();
5. Adjust Quality Settings: The Web Audio API provides options to adjust the quality of audio processing. For instance, if you are generating audio in real time, you can lower the sample rate or reduce the number of channels to decrease CPU usage at the expense of audio fidelity. This can be an important trade-off in performance-sensitive applications.
const lowQualityContext = new (window.AudioContext || window.webkitAudioContext)({ sampleRate: 22050 });
6. Use Web Workers for Heavy Processing: Offloading heavy computational tasks to Web Workers can help keep the main thread responsive. While the Web Audio API itself is designed to be efficient, complex audio calculations, like real-time synthesis or analysis, can benefit from asynchronous processing. This prevents audio processing from blocking the UI thread and improves the overall performance of your application.
const worker = new Worker('audioWorker.js'); // Send data to the worker for processing worker.postMessage({ audioData: audioBuffer });
7. Profile Performance Regularly: Utilize browser developer tools to monitor performance metrics, including CPU usage and memory allocation. Profiling your application during audio playback can help identify bottlenecks or areas in need of optimization. Adjusting your implementation based on these insights can lead to a smoother user experience.
By applying these best practices, developers can ensure that their audio applications are not only responsive and efficient but also maintain a high degree of audio quality. Optimizing performance when working with the Web Audio API is essential for delivering engaging and interactive audio experiences that delight users.