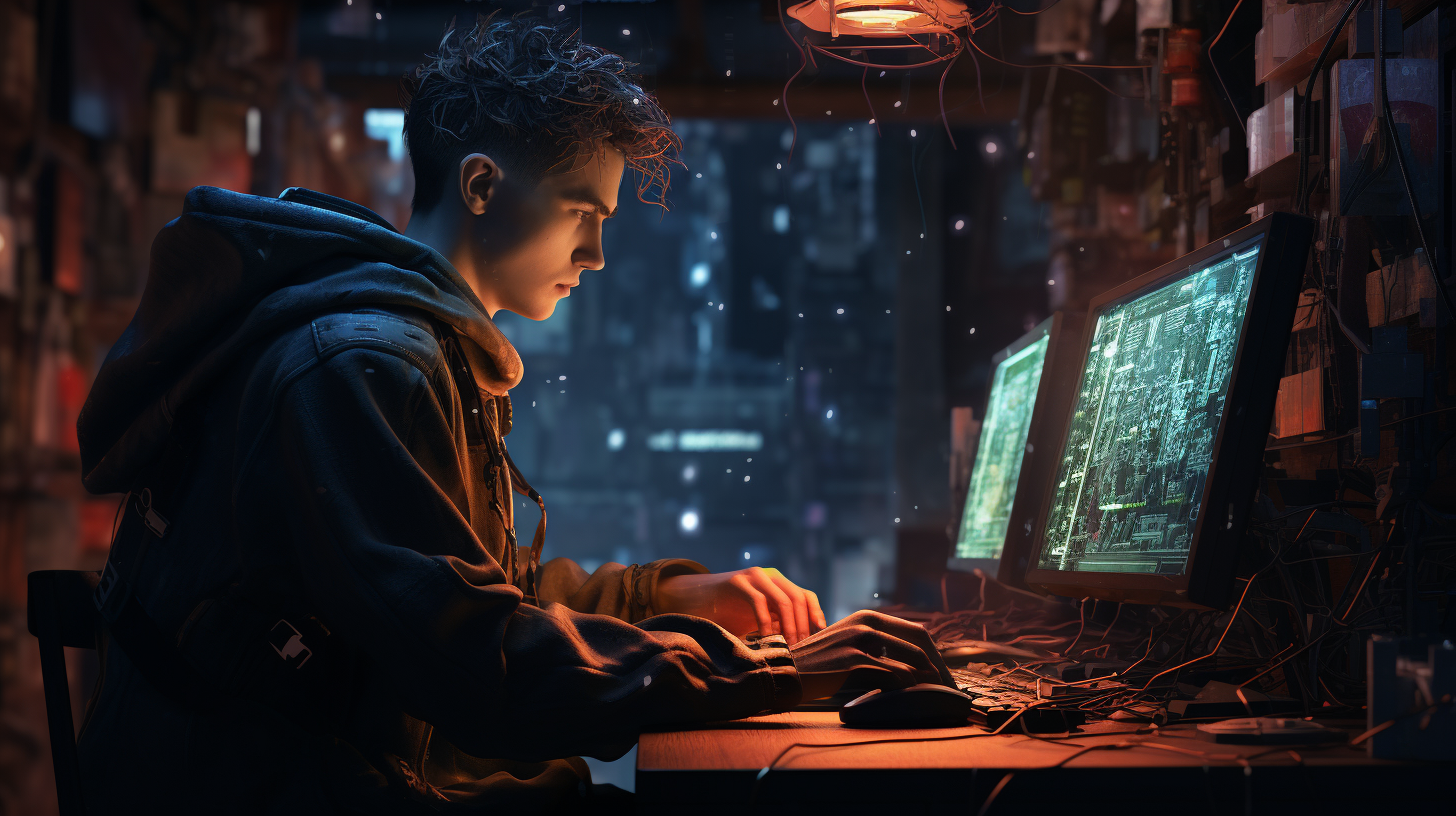
JavaScript and Cross-Browser Testing
Within the scope of web development, understanding cross-browser compatibility issues is paramount. JavaScript, being a dynamic language that manipulates the Document Object Model (DOM), often behaves differently across various browsers. This inconsistency can lead to frustrating user experiences and increased development time. Developers must grasp these discrepancies to ensure a seamless performance of their applications.
Different browsers have their own rendering engines, such as WebKit for Safari, Blink for Chrome, and Gecko for Firefox. Each engine interprets JavaScript and applies styles to HTML elements in slightly different ways, which can lead to issues such as:
- Certain JavaScript features may not be fully supported or implemented in all browsers. For instance, ES6 features like
Promise
orlet
may not work in older versions of Internet Explorer. - Variations in how browsers render CSS and JavaScript can lead to layout issues or unexpected behavior. For example, a flexbox layout may appear as intended in Chrome but break in Firefox.
- Event handling can differ significantly; some browsers may not trigger events in the same sequence, leading to bugs in interactive applications.
Ponder the following code snippet that demonstrates a simple JavaScript feature detection for fetch
, which may not be available in older browsers:
if (typeof fetch === 'function') { // Fetch is supported fetch('https://api.example.com/data') .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error fetching data:', error)); } else { console.error('Fetch API is not supported in this browser.'); }
By using feature detection rather than relying on browser-specific workarounds, developers can cater to a wider audience while maintaining functionality. Libraries such as Modernizr can also help identify features available to the user’s browser, thereby assisting in the development of fallbacks for unsupported features.
Another critical aspect is understanding the JavaScript execution context, which can differ among browsers. For instance, the way closures and scoping work in JavaScript can lead to confusion, especially when combined with asynchronous code. This discrepancy can be the source of bugs that are hard to track down when they only occur in specific browsers.
To tackle cross-browser compatibility issues effectively, it’s essential to recognize the underlying differences in how browsers interpret JavaScript and the DOM. Armed with this knowledge, developers can craft solutions that minimize discrepancies and enhance user experience across platforms.
Key Tools for Cross-Browser Testing
When it comes to cross-browser testing, having the right tools at your disposal can make a tremendous difference in efficiency and accuracy. There are several key tools that developers can utilize to ensure their JavaScript and web applications function seamlessly across different browsers.
One of the most widely used tools for cross-browser testing is BrowserStack. This platform provides access to a range of real devices and browsers, allowing developers to test their applications in real-time. With BrowserStack, you can run automated and live testing sessions, ensuring your JavaScript code behaves as expected on different platforms. It simplifies the process of identifying browser-specific issues by providing instant feedback on how your application renders and functions in various environments.
const browserStackUrl = "https://www.browserstack.com/"; console.log(`Access BrowserStack for cross-browser testing: ${browserStackUrl}`);
Another valuable resource is CrossBrowserTesting.com, which offers a similar suite of tools, including visual testing and automated screenshots. This service allows you to capture screenshots across multiple browsers and devices, making it easier to spot rendering issues that might not be immediately apparent in a live testing scenario. Its integration with popular frameworks like Selenium enables automated testing of JavaScript behaviors as well.
const cbtUrl = "https://crossbrowsertesting.com/"; console.log(`Explore CrossBrowserTesting for effective visual testing: ${cbtUrl}`);
For developers who prefer a more hands-on approach, LambdaTest offers cloud-based browser testing that helps in quickly validating your web applications against multiple browsers. It also supports real-time debugging and provides an extensive range of browser versions, ensuring compatibility for older users. This tool allows you to run your JavaScript code on platforms you may not have immediate access to, such as older versions of Safari or Internet Explorer.
const lambdaTestUrl = "https://www.lambdatest.com/"; console.log(`Check out LambdaTest for cloud-based testing solutions: ${lambdaTestUrl}`);
Moreover, using browser developer tools especially important for immediate feedback during development. Most major browsers, including Chrome, Firefox, and Edge, come equipped with built-in developer tools that enable you to inspect elements, debug JavaScript code, and analyze performance. Using these tools can help identify issues specific to a particular browser, such as console errors or specific rendering quirks that might affect your JavaScript code.
console.log('Utilize browser developer tools for debugging!'); debugger; // This will pause JavaScript execution in the developer console
Lastly, automated testing frameworks like Selenium and TestCafe play a significant role in cross-browser testing. They allow developers to write scripts that run tests across multiple browsers, identifying issues in JavaScript functionality automatically. Using these frameworks can save considerable time compared to manual testing, as they streamline the process of verifying that your code works as intended in every environment.
const { Builder, By } = require('selenium-webdriver'); (async function example() { let driver = await new Builder().forBrowser('firefox').build(); await driver.get('http://example.com'); await driver.quit(); })().catch(console.error);
Employing a mix of these tools can empower developers to produce robust, cross-browser compatible JavaScript applications. By investing time in understanding and using these resources, you can effectively mitigate compatibility issues and enhance the overall user experience across various browsers.
Best Practices for Writing Cross-Browser JavaScript
When it comes to writing cross-browser JavaScript, adhering to best practices is essential for ensuring that your code operates smoothly regardless of the environment. First and foremost, using feature detection over browser detection is vital. This approach focuses on checking whether a particular feature is available in the user’s browser, allowing for a more graceful degradation of functionality when necessary. Think the example of using the localStorage API, which may not be available in older browsers:
if (typeof Storage !== 'undefined') { // Local storage is supported localStorage.setItem('key', 'value'); } else { console.error('Local storage is not supported in this browser.'); }
Another best practice is to avoid using deprecated or non-standard features. JavaScript evolves rapidly, and features that were once popular can become obsolete. Relying on outdated techniques can lead to compatibility issues as new browser versions roll out. For instance, the use of document.all
for element selection is a relic of the past and should be avoided. Instead, use standard methods like document.getElementById
or document.querySelector
:
const element = document.querySelector('#myElement'); if (element) { element.textContent = 'Updated content'; } else { console.error('Element not found'); }
In addition to this, it’s crucial to utilize polyfills. A polyfill is a piece of code that implements a feature on browsers that do not support it natively. This allows you to write modern JavaScript while ensuring functionality in older browsers. A common polyfill is for the Array.prototype.includes
method:
if (!Array.prototype.includes) { Array.prototype.includes = function(value) { return this.indexOf(value) !== -1; }; }
Moreover, asynchronous programming can introduce complications if not handled correctly. It is prudent to use Promises or async/await patterns, but keep in mind that some older browsers may not support these features. In such cases, using transpilers like Babel can help you convert modern JavaScript into a version compatible with older browsers:
async function fetchData() { try { const response = await fetch('https://api.example.com/data'); const data = await response.json(); console.log(data); } catch (error) { console.error('Error fetching data:', error); } }
Finally, always ensure that your JavaScript code is modular. Using modules allows for better organization of code and facilitates debugging. Tools like Webpack or Rollup can help bundle your JavaScript files for deployment while ensuring compatibility across various environments.
Writing cross-browser JavaScript that stands the test of time requires a careful blend of best practices, feature detection, and modern development tools. By adhering to these guidelines, developers can minimize the risk of compatibility issues and deliver a consistent experience to users regardless of their chosen browser.
Automating Cross-Browser Testing with Frameworks
Automating cross-browser testing is a powerful technique that can significantly streamline the development process, allowing developers to quickly identify and rectify issues that may arise in different browser environments. By using automation frameworks, developers can write scripts that simulate user interactions, thereby testing the functionality and performance of their JavaScript code across multiple browsers efficiently. This method not only saves time but also enhances reliability, as automated tests can be run repeatedly with consistent results.
One of the most popular frameworks for automating cross-browser testing is Selenium. Selenium provides a robust platform for automating web applications for testing purposes and supports various programming languages, including JavaScript. By using Selenium WebDriver, developers can write scripts that perform actions like clicking buttons, filling out forms, and validating page content.
const { Builder, By, until } = require('selenium-webdriver'); (async function example() { let driver = await new Builder().forBrowser('chrome').build(); try { await driver.get('http://example.com'); await driver.wait(until.titleIs('Example Domain'), 1000); const element = await driver.findElement(By.css('h1')); const text = await element.getText(); console.log(`Header Text: ${text}`); } finally { await driver.quit(); } })().catch(console.error);
In the code snippet above, we create a simple Selenium test that opens a webpage, waits for the title to be correct, retrieves the text of a heading, and then prints it to the console. By changing the browser specified in `forBrowser`, developers can easily test the same script across various environments.
Another excellent framework is TestCafe, which simplifies the setup process and eliminates the need for browser plugins. TestCafe supports all modern browsers and allows you to run tests on remote devices. Its API is simpler and offers a rich set of features to manage test execution and reporting.
import { Selector } from 'testcafe'; fixture `Getting Started` .page `http://example.com`; test('My first test', async t => { const header = Selector('h1'); await t .expect(header.innerText).eql('Example Domain'); });
In this TestCafe example, we set up a fixture that points to the URL we want to test. The test checks that the text of the header matches the expected value. TestCafe’s simple syntax allows developers to focus on testing logic rather than implementation details.
For those looking to integrate their testing into a CI/CD pipeline, tools like Cypress offer an excellent solution. Cypress is designed specifically for modern web applications and provides fast, reliable testing. It operates directly in the browser and allows for easy setup of end-to-end tests that can run in the background as part of your development workflow.
describe('My First Test', () => { it('Visits the Example Domain', () => { cy.visit('http://example.com'); cy.get('h1').should('have.text', 'Example Domain'); }); });
In the Cypress example, we define a test suite using the `describe` function, then use `it` to specify a single test case. The `cy.visit` command navigates to the specified URL, while `cy.get` retrieves the header element and checks its text. This syntax is highly readable, which enhances code maintainability.
By adopting these automation frameworks, developers can ensure their JavaScript applications are tested across multiple browsers with accuracy and efficiency. Automated testing not only helps catch issues early in the development cycle, but it also allows for faster feedback loops, enabling teams to iterate quickly and deliver high-quality applications that perform consistently regardless of the user’s browser choice.
Debugging Techniques for Cross-Browser Issues
Debugging cross-browser issues is often likened to navigating a maze filled with hidden traps; each turn can introduce unexpected behavior that varies from one browser to another. To effectively tackle these issues, developers must be equipped with a systematic approach to debugging that leverages both browser-specific tools and general JavaScript debugging techniques.
One of the first steps in isolating a cross-browser issue is to utilize the built-in developer tools available in modern browsers. Each major browser has its own set of developer tools, which are invaluable for inspecting elements, monitoring console output, and debugging JavaScript code. For instance, in Chrome, pressing F12 opens the Developer Tools, so that you can navigate the Elements tab to inspect your HTML structure and the Console tab to view any logged errors. Similar functionality exists in Firefox and Edge, making these tools indispensable for any developer.
console.log('This message will be visible in the console'); debugger; // Use this to pause execution and inspect current variables in the console
Using the debugger statement in your JavaScript can also help pause execution at a specific point within your code, allowing you to inspect variable states and flow of execution. This can be particularly useful when trying to diagnose issues that are only manifesting in specific browsers. Ponder this simple function where we suspect an issue might arise:
function calculateSum(a, b) { debugger; // This will pause execution return a + b; } const result = calculateSum(5, '10'); // Implicit type coercion issue
In the example above, placing the debugger statement allows developers to step through the code and observe how JavaScript handles type coercion, which can lead to different results across browsers depending on their JavaScript engines.
Another critical debugging technique involves comparing the output of your JavaScript code across different consoles. Some errors may only appear in a specific browser’s console, and thus checking output across environments can provide insights into problems. For instance, a certain API may behave differently or return unexpected results based on the browser’s implementation of JavaScript features. Using console assertions can be particularly useful here:
console.assert(typeof Promise !== 'undefined', 'Promises are not supported in this browser');
In addition to console assertions, some browsers allow for capturing network activity, which can help identify issues related to API calls or resource loading. The Network tab in the Developer Tools will show you all HTTP requests, their statuses, and the time taken for each request. This information very important for diagnosing issues related to asynchronous operations in JavaScript.
Employing systematic logging throughout your code can also help identify where things are going awry. Instead of relying solely on console.log statements, think implementing a logging framework that can provide different logging levels (info, warning, error) and capture logs conditionally based on the environment:
const environment = 'development'; // Change this to 'production' for live deployments function log(level, message) { if (environment === 'development') { console[level](message); } } log('error', 'This is an error message');
By structuring your logging approach, you can control the output based on the environment, making it easier to debug issues without cluttering the console in production.
Lastly, when debugging cross-browser issues, it is essential to have a thorough understanding of the differences in JavaScript engines and their implementations. Tools like Babel can transpile modern JavaScript down to versions that are more widely supported, hence reducing the likelihood of encountering browser-specific issues. This can also serve as an intermediate debugging step, as you can isolate whether a feature is unsupported in a particular browser or simply behaving differently.
const userFeature = 'async function fetchData() {};'; // Example of modern JS console.log(userFeature); // Use transpilation to ensure compatibility
Through a combination of using browser developer tools, employing systematic logging, and understanding the underlying discrepancies between JavaScript engines, developers can effectively debug cross-browser issues. By cultivating these debugging techniques, you become better equipped to handle the idiosyncrasies of various browsers, ultimately leading to a smoother user experience in your applications.
Future Trends in Cross-Browser Development
As we look towards the future of cross-browser development, several trends are emerging that will shape how developers approach compatibility issues in JavaScript. The landscape of web development is continuously evolving, driven by technological advancements and user expectations. One prominent trend is the increasing adoption of modern JavaScript features and standards, primarily driven by the growing use of frameworks like React, Angular, and Vue.js. These frameworks encourage developers to write modular, component-based code, making it essential to ensure that this code remains compatible across all major browsers.
Another significant trend is the move towards progressive enhancement and graceful degradation. Developers are increasingly embracing the idea that a web application should provide a functional experience across all browsers, regardless of their capabilities. This means writing JavaScript that can adapt to different environments while maintaining baseline functionality. For instance, developers might choose to implement features using the Intersection Observer API for modern browsers while providing a fallback for older browsers that do not support this API.
if ('IntersectionObserver' in window) { const observer = new IntersectionObserver((entries) => { entries.forEach(entry => { if (entry.isIntersecting) { // Load or animate element } }); }); observer.observe(document.querySelector('.lazy-load')); } else { // Fallback for browsers that do not support Intersection Observer console.warn('Intersection Observer not supported, using fallback.'); }
The rise of containerized applications and microservices is also influencing how we approach cross-browser compatibility. With the backend increasingly decoupled from the frontend, developers are focusing on creating APIs that deliver data to various client-side applications. This trend highlights the importance of adhering to web standards when building JavaScript applications. By ensuring that the APIs are robust and standardized, developers can minimize discrepancies between how data is consumed across different browsers.
Moreover, the move towards server-side rendering (SSR) and static site generation (SSG) is gaining traction. Frameworks like Next.js and Nuxt.js allow developers to pre-render web pages, improving performance and SEO. This approach can mitigate some cross-browser issues by serving fully rendered HTML to the client, reducing reliance on JavaScript execution in the browser. However, developers must still ensure that any interactive elements are compatible across different environments.
Furthermore, the increasing focus on accessibility and inclusive design is reshaping how we develop cross-browser applications. Developers are now more aware of the need to create applications that are not only functional but also accessible to users with disabilities. This means adhering to web standards like the W3C’s Web Content Accessibility Guidelines (WCAG), which can sometimes reveal compatibility issues across different browsers. Using tools like axe-core can help identify accessibility issues during the development process, ensuring compliance with the latest standards.
import { axe } from 'axe-core'; axe.run(document, {}, (err, results) => { if (results.violations.length > 0) { console.warn('Accessibility violations found:', results.violations); } });
Finally, the evolution of testing frameworks is another trend to watch. Tools like Cypress and Playwright are becoming more prevalent, enabling developers to perform cross-browser testing more efficiently. As these tools continue to evolve, they will simplify the process of writing and maintaining tests, allowing developers to focus on building features rather than troubleshooting compatibility issues. The integration of testing frameworks into continuous integration/continuous deployment (CI/CD) pipelines is also set to become standard practice, ensuring that applications are tested across multiple environments before deployment.
The future of cross-browser development is poised for transformation. By staying informed about emerging trends and using modern tools and frameworks, developers can build resilient JavaScript applications that deliver consistent experiences across all browsers. The importance of adhering to web standards, focusing on accessibility, and adopting efficient testing practices will be crucial in navigating the evolving landscape of web development.