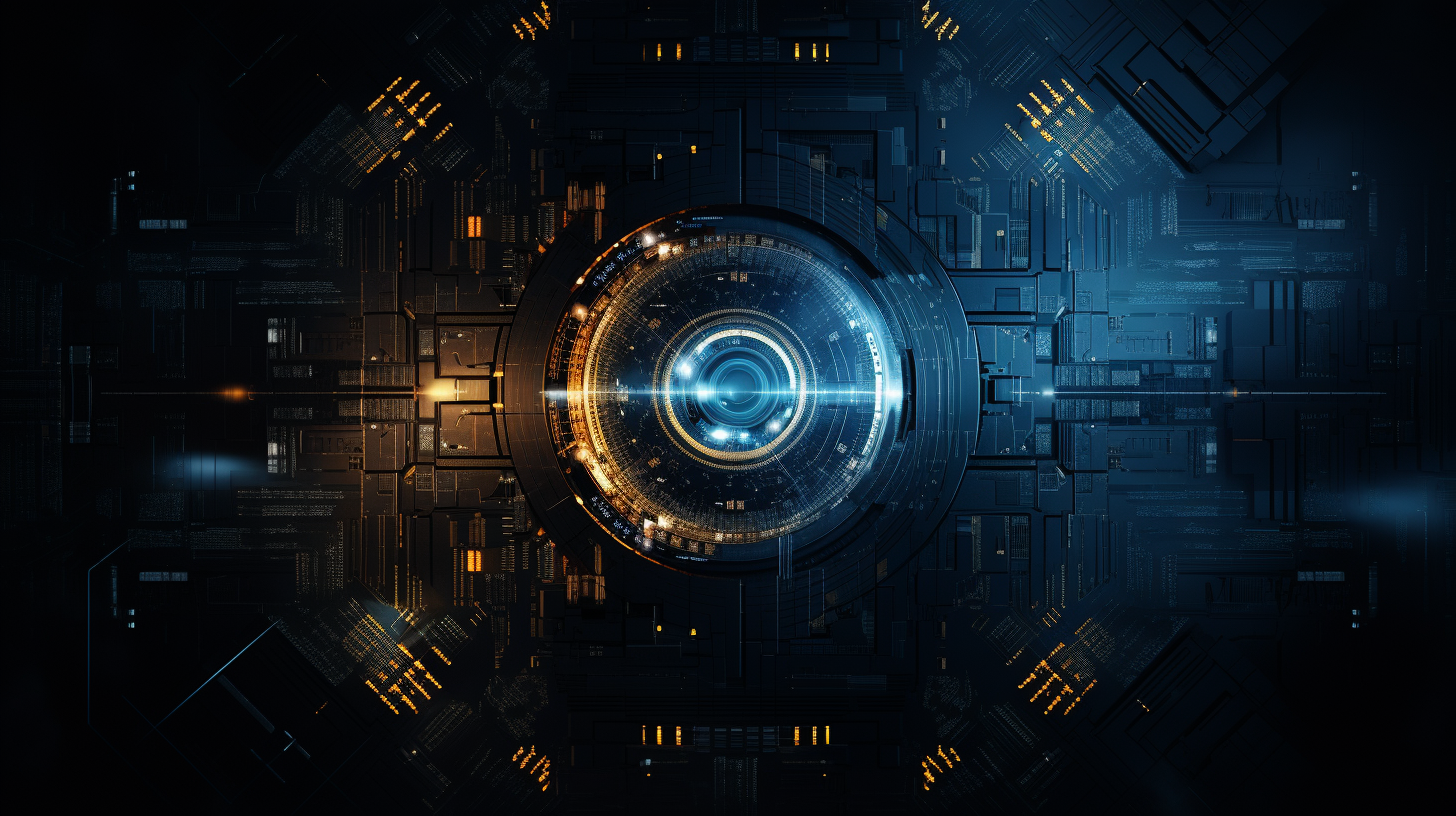
JavaScript and Cross-Platform Desktop Apps
Cross-platform desktop applications have gained significant traction in recent years, allowing developers to create software that runs on multiple operating systems without the need for extensive modifications. These applications leverage the power of web technologies—HTML, CSS, and JavaScript—to build user interfaces and functionality that can be packaged into native executables for Windows, macOS, and Linux.
One of the compelling advantages of cross-platform development is the reduction in overall development time and resource allocation. Instead of maintaining separate codebases for each operating system, developers can write a single codebase that serves all platforms. This not only streamlines the development process but also simplifies updates and bug fixes, as changes need to be made in just one place.
Cross-platform desktop applications typically utilize frameworks and tools that facilitate the seamless integration of web technologies with native capabilities. These tools enable developers to access system-level features such as the file system, notifications, and hardware resources through JavaScript APIs. This capability allows developers to create powerful applications that feel native to each operating system, enhancing user experience.
const { app, BrowserWindow } = require('electron'); function createWindow() { const win = new BrowserWindow({ width: 800, height: 600, webPreferences: { nodeIntegration: true } }); win.loadFile('index.html'); } app.whenReady().then(createWindow);
Frameworks like Electron, NW.js, and React Native for Windows and macOS have become popular choices among developers looking to build cross-platform applications. Electron, for instance, allows developers to use JavaScript, HTML, and CSS to create desktop applications that work across all major operating systems. This framework wraps web applications in a native shell, providing a rich API for accessing operating system features.
In essence, cross-platform desktop applications represent a convergence of web and desktop development, providing a flexible and efficient route to creating robust software solutions. As developers increasingly embrace the possibilities offered by these technologies, the landscape of desktop application development continues to evolve, breaking down traditional barriers and expanding the horizons of what can be achieved in the context of software development.
Popular Frameworks for Building Desktop Apps with JavaScript
Among the most prominent frameworks available for building cross-platform desktop applications with JavaScript, Electron stands out due to its impressive capabilities and widespread adoption. Its architecture is based on a combination of Chromium and Node.js, enabling developers to harness the best of web technologies while maintaining access to powerful native features.
Using Electron, developers can create applications by combining web development skills with native functionality. The framework allows you to package your HTML, CSS, and JavaScript files into a single executable application. This means that web developers can quickly transition to desktop app development without needing to learn new languages or paradigms.
const { app, BrowserWindow } = require('electron'); function createWindow() { const win = new BrowserWindow({ width: 800, height: 600, webPreferences: { nodeIntegration: true } }); win.loadFile('index.html'); // Load your HTML file } app.whenReady().then(createWindow); // Create the window when the app is ready app.on('window-all-closed', () => { if (process.platform !== 'darwin') { app.quit(); // Quit the app when all windows are closed, except on macOS } }); app.on('activate', () => { if (BrowserWindow.getAllWindows().length === 0) { createWindow(); // Re-create a window if none are open (macOS behavior) } });
Another notable framework is NW.js, which also combines Node.js with Chromium. NW.js provides an easy way to build native applications using web technologies. One of its key features is the ability to access Node.js modules directly within the browser context, making it easier to interact with the local file system or other native resources.
const nw = require('nw.gui'); function main() { const win = nw.Window.open('index.html', { width: 800, height: 600, }); } main(); // Start the application
For those who prefer to work within the React ecosystem, React Native for Windows and macOS offers a viable option. This framework allows developers to build applications that are not only cross-platform but also utilize the powerful UI components provided by React. It abstracts away many of the complexities of native development, allowing for a more streamlined approach when creating user interfaces.
Additionally, there’s a growing interest in frameworks like Tauri and Neutralinojs, which aim to provide lightweight alternatives to Electron and NW.js. Tauri, for example, focuses on using Rust for the backend, resulting in smaller application sizes and potentially improved performance. Neutralinojs offers a simplified approach by enabling developers to build applications using only web technologies without the overhead of a full-fledged framework.
Each of these frameworks has its strengths and weaknesses, and the choice often boils down to the specific requirements of the project and the preferences of the development team. As the landscape evolves, new tools and frameworks continue to emerge, making it an exciting time for JavaScript developers looking to create cross-platform desktop applications.
Advantages of Using JavaScript for Desktop Development
One of the most significant advantages of using JavaScript for desktop development is the ability to leverage existing web development skills. Many developers already possess a strong foundation in JavaScript, HTML, and CSS, making the transition to desktop application development relatively seamless. This skill overlap minimizes the learning curve associated with new languages or frameworks, allowing developers to focus on building features and enhancing their applications.
Furthermore, JavaScript’s non-blocking, asynchronous capabilities enable developers to create responsive applications that can handle multiple tasks concurrently. That’s particularly crucial for applications that require real-time interactions, such as chat applications or data visualization tools. The event-driven nature of JavaScript allows developers to manage user input and background processes effectively without freezing the user interface.
Another compelling benefit is the rich ecosystem of libraries and tools available to JavaScript developers. With a vast array of packages available via npm (Node Package Manager), developers can easily integrate additional functionality into their applications. This includes everything from UI component libraries to data handling utilities, streamlining the development process and enabling rapid prototyping.
const axios = require('axios'); async function fetchData(url) { try { const response = await axios.get(url); console.log(response.data); } catch (error) { console.error('Error fetching data:', error); } } fetchData('https://api.example.com/data');
JavaScript also benefits from a vibrant community and extensive documentation. This community support translates into a wealth of resources, tutorials, and forums where developers can seek help, share knowledge, and collaborate on projects. This collective wisdom fosters an environment where developers can quickly resolve issues and implement best practices, ultimately leading to higher-quality applications.
Moreover, frameworks like Electron and NW.js facilitate the integration of web technologies with native capabilities, providing robust APIs for system-level features. This integration allows developers to access functionalities such as file handling, notifications, and system tray interactions, enabling the creation of applications that feel native to the operating system.
const { dialog } = require('electron').remote; function openFile() { dialog.showOpenDialog({ properties: ['openFile'] }).then(result => { if (!result.canceled) { console.log('Selected file:', result.filePaths[0]); } }).catch(err => { console.error('Error opening file:', err); }); }
Not to be overlooked is JavaScript’s compatibility with various platforms. With the rise of web technologies, developers can build applications that not only run on desktops but can also be adapted for mobile devices or the web. This versatility ensures that applications can reach a broader audience without the need for extensive rewrites or re-engineering.
The advantages of using JavaScript for desktop development center around its accessibility, asynchronous capabilities, rich ecosystem, community support, and seamless integration with native features. These factors combine to create an environment well-suited for developing modern cross-platform desktop applications that can meet diverse user needs while maintaining a high degree of performance and usability.
Challenges and Considerations in Cross-Platform Development
Despite the myriad benefits of cross-platform desktop application development using JavaScript, there are notable challenges and considerations that developers must navigate. One of the primary challenges lies in the inherent differences between operating systems. Even though frameworks like Electron and NW.js strive for uniformity, variances in system behavior can lead to inconsistencies between platforms. For instance, file path handling differs across Windows, macOS, and Linux, necessitating conditional logic in the code to ensure a seamless experience.
const path = require('path'); function getFilePath(fileName) { const basePath = process.platform === 'win32' ? 'C:\Users\User\Documents' : '/Users/User/Documents'; return path.join(basePath, fileName); }
Another pressing consideration is performance. While JavaScript is adept at handling asynchronous tasks, its single-threaded nature can become a bottleneck in resource-intensive applications. For applications that require significant computational power, such as image processing or data analysis tools, developers may need to offload these tasks to worker threads or use native modules written in languages like C or Rust. This not only complicates the architecture but can also introduce additional overhead in terms of development time and maintenance.
const { Worker } = require('worker_threads'); function runWorker() { const worker = new Worker('./worker.js'); worker.on('message', message => { console.log('Received from worker:', message); }); worker.postMessage('Start processing data'); }
Security is another crucial factor to ponder when developing cross-platform desktop applications. With increasing concerns about data breaches and user privacy, developers must ensure that their applications adhere to security best practices. This includes implementing secure communication protocols, safeguarding sensitive user data, and validating inputs to prevent injection attacks. Frameworks often provide tools to support these practices, but it remains the developer’s responsibility to utilize them effectively.
Moreover, testing cross-platform applications can be cumbersome. Developers must rigorously test their applications on each target platform to identify and rectify any platform-specific issues. This adds to the overall workload and may slow down the release cycle. Continuous integration and testing tools can alleviate some of this burden, but setting up and maintaining these systems requires additional effort and expertise.
Finally, the evolving landscape of JavaScript and its frameworks presents both opportunities and challenges. As new versions of frameworks are released and new technologies emerge, developers must keep their skills and knowledge up to date. This can be daunting, especially for teams with limited resources. However, staying abreast of these changes is vital for using the best features and capabilities of the tools at hand.
While the promise of cross-platform development with JavaScript is indeed enticing, developers must carefully navigate the associated challenges. By addressing issues related to platform differences, performance, security, testing, and ongoing education, they can create robust, efficient, and secure applications that resonate with users across diverse environments.
Future Trends in JavaScript and Desktop Application Development
The future of JavaScript in desktop application development is shaped by several emerging trends that promise to improve the capabilities of cross-platform applications. As developers continue to push the boundaries of what is possible, several key areas stand out as focal points for innovation and growth.
One of the most significant trends is the increasing adoption of TypeScript in JavaScript applications. TypeScript, with its static typing and enhanced tooling support, provides a robust foundation for building large-scale applications. As developers recognize the advantages of using TypeScript, particularly in maintaining and scaling complex codebases, it is likely that its integration into cross-platform desktop development will become commonplace. This allows for improved code quality, better documentation, and easier collaboration among development teams.
const fs = require('fs'); function readFileSync(filePath: string): string { return fs.readFileSync(filePath, 'utf-8'); } const data: string = readFileSync('example.txt'); console.log(data);
Another notable trend is the growing emphasis on performance optimization. As applications become more feature-rich, the demand for efficient resource management and quick load times intensifies. Developers are increasingly exploring techniques such as code splitting, lazy loading, and optimizing rendering performance. Frameworks like React and Vue.js have introduced features that enable developers to load only the necessary components, enhancing user experience and reducing the initial load time significantly.
import React, { Suspense, lazy } from 'react'; const LazyComponent = lazy(() => import('./LazyComponent')); function App() { return (<Suspense fallback={); }Loading...}>
Furthermore, the integration of WebAssembly (Wasm) into JavaScript applications is set to revolutionize performance in desktop apps. By allowing developers to write critical parts of their applications in languages like C, C++, or Rust and compile them to WebAssembly, applications can achieve near-native performance levels. That’s particularly advantageous for resource-intensive tasks such as graphics processing, video editing, or scientific computing, making JavaScript applications more versatile and powerful.
const { add } = require('./math.wasm'); async function loadWasm() { const response = await fetch('math.wasm'); const bytes = await response.arrayBuffer(); const module = await WebAssembly.instantiate(bytes); return module.instance.exports; } loadWasm().then(math => { console.log('Result of add:', math.add(5, 10)); });
The shift towards progressive web applications (PWAs) is another trend that will influence desktop application development. PWAs offer the ability to create applications that function seamlessly across devices and platforms, combining the best features of web and mobile applications. As more users turn to PWAs for their offline capabilities, push notifications, and home screen access, developers will likely think how to leverage this technology in their desktop applications.
if ('serviceWorker' in navigator) { window.addEventListener('load', () => { navigator.serviceWorker.register('/service-worker.js') .then(registration => { console.log('Service Worker registered with scope:', registration.scope); }) .catch(error => { console.error('Service Worker registration failed:', error); }); }); }
Security also remains a top priority as applications evolve. With increasing scrutiny on data privacy and security protocols, developers must keep abreast of best practices and compliance regulations. Using modern encryption standards, implementing secure authentication methods, and adhering to frameworks that prioritize security will be integral to maintaining user trust and protecting sensitive data.
const crypto = require('crypto'); function encrypt(text: string): string { const cipher = crypto.createCipher('aes256', 'a_password'); let encrypted = cipher.update(text, 'utf8', 'hex'); encrypted += cipher.final('hex'); return encrypted; } console.log(encrypt('Sensitive Data'));
Lastly, the community surrounding JavaScript continues to thrive, leading to an explosion of resources and libraries that facilitate desktop application development. As new frameworks emerge and existing ones evolve, developers will have an ever-expanding toolkit at their disposal. This active engagement within the community not only fuels innovation but also fosters collaboration and knowledge sharing, further enhancing the overall development landscape.
As these trends unfold, they will undoubtedly shape the future trajectory of JavaScript and cross-platform desktop application development, paving the way for more powerful, efficient, and easy to use applications. The convergence of web and desktop technologies, combined with a commitment to optimizing performance and security, will empower developers to create next-generation software that meets the diverse needs of users across the globe.