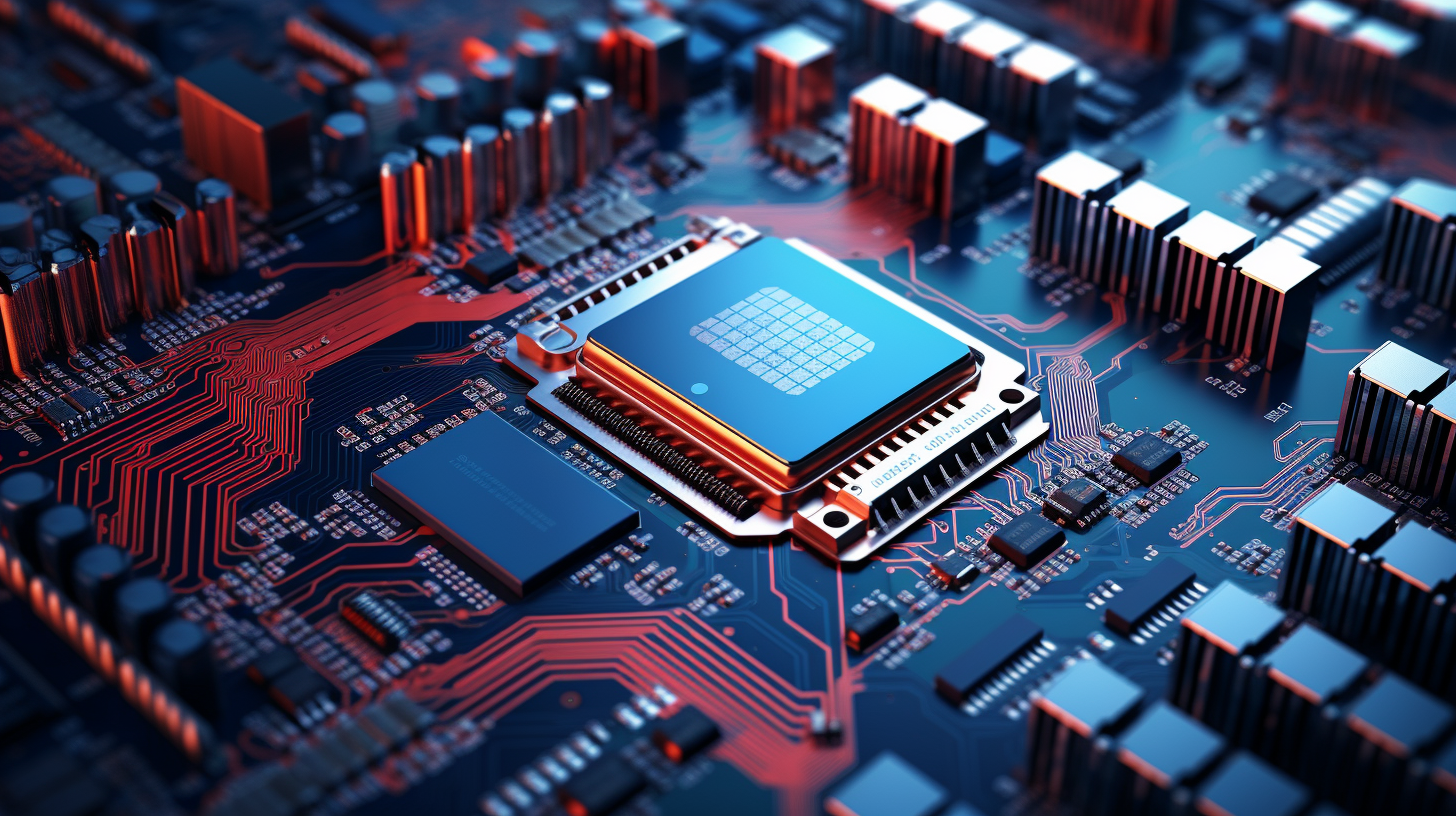
JavaScript and Custom Chatbots
JavaScript serves as the backbone for many modern web applications, and its role in chatbot development is no exception. At its core, JavaScript enables dynamic, asynchronous interactions that are essential for creating responsive and engaging chatbots. The language allows developers to implement real-time communication between users and chatbots, ensuring that messages are sent and received instantly without the need for page reloads.
In a typical chatbot architecture, JavaScript can be utilized both on the client side and the server side. On the client side, JavaScript is responsible for handling user inputs, rendering the chat interface, and managing the flow of conversation. That’s achieved using event listeners that respond to user actions, such as clicking on buttons or submitting text input.
document.getElementById('send-button').addEventListener('click', function() { const userInput = document.getElementById('user-input').value; displayUserMessage(userInput); sendMessageToBot(userInput); });
In the example above, when the user clicks the ‘send’ button, the input is captured and displayed in the chat interface through the displayUserMessage
function. The message is then sent to the chatbot backend using the sendMessageToBot
function.
On the server side, Node.js—a JavaScript runtime—plays an important role in managing the chatbot’s logic and interactions. By using frameworks such as Express, developers can set up an API endpoint that listens for incoming messages from users, processes them, and returns appropriate responses. This allows for easy integration of natural language processing (NLP) services and other functionalities.
const express = require('express'); const bodyParser = require('body-parser'); const app = express(); app.use(bodyParser.json()); app.post('/chatbot', (req, res) => { const userMessage = req.body.message; const botResponse = processUserMessage(userMessage); // Custom function to handle logic res.json({ response: botResponse }); }); app.listen(3000, () => { console.log('Chatbot server listening on port 3000'); });
In this server-side example, we set up an Express application that listens for POST requests to the ‘/chatbot’ route. Upon receiving a message, it processes the input through a custom logic function and sends back a generated response. This structure allows chatbots to easily handle multiple conversations at once, using JavaScript’s event-driven, non-blocking nature.
Furthermore, the use of WebSockets can enhance real-time communication between the client and server, creating a seamless user experience. This enables chatbots to push messages to users instantly, improving engagement and satisfaction. By using the full potential of JavaScript, developers can build sophisticated and interactive chatbots that meet the diverse needs of users.
Key Libraries and Frameworks for Building Chatbots
When it comes to building effective chatbots, using the right libraries and frameworks can significantly streamline development and enhance functionality. A plethora of JavaScript libraries are available, each designed to facilitate various aspects of chatbot creation, from user interaction to backend processing.
Bot frameworks are a great starting point for developers looking to kick off their chatbot projects. Microsoft Bot Framework, for instance, provides a comprehensive platform for building conversational AI. It allows developers to create sophisticated bots that can interact across multiple channels, such as web, SMS, and messaging apps. The framework supports a range of programming languages, including JavaScript, enabling seamless integration with existing web applications.
Another noteworthy mention is Botpress, an open-source conversational platform built with Node.js. Botpress allows developers to create, manage, and deploy chatbots smoothly. It offers a rich user interface for designing conversation flows and managing intents, making it easier to handle user inputs without delving deep into the code. The built-in natural language understanding (NLU) capabilities provide robust tools for interpreting user queries accurately.
To get started with Botpress, you can install it using npm:
npm install -g botpress
After installation, you can initiate a new bot project with:
botpress init my-chatbot
Once your bot is set up, you can customize it via the Botpress Studio, where you can define the dialogue flows and integrations.
Socket.io is another essential library that enhances real-time communication within chatbots. By enabling real-time, bidirectional communication between the client and server, Socket.io helps maintain an interactive chat experience. That is particularly useful in scenarios where immediate user feedback very important. Incorporating Socket.io into a chatbot application can be done with the following code:
const socket = require('socket.io')(server); socket.on('connection', (client) => { client.on('sendMessage', (message) => { // Process the message and respond const response = processMessage(message); client.emit('receiveMessage', response); }); });
In this example, when a user sends a message, it’s processed, and a response is emitted back to the user in real-time. This creates a more dynamic interaction model, allowing for a fluid conversation with the chatbot.
Additionally, Rasa is an open-source framework that combines both machine learning and dialogue management for building chatbots. Although primarily Python-based, Rasa allows for integration with JavaScript applications, enabling developers to create advanced chatbots with custom machine learning models. Rasa’s ability to understand context and multi-turn conversations makes it a powerful tool for developers aiming for sophisticated dialogue capabilities.
For those focused on the frontend, libraries like React and Vue.js can be instrumental in crafting engaging user interfaces for chatbots. By using these frameworks, developers can create interactive UI components that enhance user experience, such as message bubbles, typing indicators, and quick reply options. The integration of these libraries with a chatbot backend can be achieved smoothly through API calls, allowing for a responsive and uncomplicated to manage interaction.
The selection of libraries and frameworks can profoundly affect the efficiency and effectiveness of chatbot development. By using tools like Microsoft Bot Framework, Botpress, Socket.io, and Rasa, developers can harness the full potential of JavaScript to create chatbots that not only engage users but also adapt to their needs seamlessly.
Designing Conversational User Interfaces
Designing an effective conversational user interface (CUI) is pivotal to the success of any chatbot. A well-thought-out CUI creates an engaging experience that encourages users to interact while providing them with clear, actionable responses. JavaScript plays an important role here, enabling developers to create dynamic interfaces that respond to user actions in real-time.
When designing a CUI, it is essential to consider the flow of conversation, including how users initiate interactions and how the chatbot responds. A common approach is to utilize a message queue or a state machine to manage conversation context. This ensures that the chatbot can track where the user is in the conversation and respond appropriately.
const conversationState = { currentStep: 0, userMessages: [], botResponses: [] }; function handleUserMessage(message) { conversationState.userMessages.push(message); const botResponse = getBotResponse(conversationState.currentStep, message); conversationState.botResponses.push(botResponse); conversationState.currentStep++; displayConversation(); } function displayConversation() { const chatWindow = document.getElementById('chat-window'); chatWindow.innerHTML = ''; // Clear previous messages conversationState.userMessages.forEach((msg, index) => { chatWindow.innerHTML += `User: ${msg}`; chatWindow.innerHTML += `Bot: ${conversationState.botResponses[index]}`; }); } function getBotResponse(step, message) { // Basic response logic based on the current step const responses = [ "Hello! How can I assist you today?", "Can you please provide more details?", "Thank you for the information! How else can I help you?" ]; return responses[step] || "I'm here to help!"; }
In this code snippet, we track the conversation state using an object that holds the current step, user messages, and bot responses. The handleUserMessage
function updates the state and fetches the appropriate response based on the current conversation step. By dynamically rendering the conversation in the chat window, we create a fluid interaction that feels natural to the user.
Moreover, the use of visual elements such as buttons, quick replies, and typing indicators can enhance the user experience significantly. JavaScript allows developers to animate these elements, providing visual feedback that keeps users engaged. For instance, adding a typing indicator while the bot processes a response can mimic human interaction more closely:
function showTypingIndicator() { const chatWindow = document.getElementById('chat-window'); chatWindow.innerHTML += `Bot is typing...`; } function hideTypingIndicator() { const chatWindow = document.getElementById('chat-window'); const indicator = document.querySelector('.typing-indicator'); if (indicator) { chatWindow.removeChild(indicator); } } async function sendMessageToBot(message) { showTypingIndicator(); // Simulate a delay for the bot's response await new Promise(resolve => setTimeout(resolve, 1000)); hideTypingIndicator(); handleUserMessage(message); }
This example demonstrates how to manage a typing indicator with JavaScript, creating an engaging and responsive experience. By using setTimeout
, we can simulate a delay, allowing users to feel as though they’re in a real conversation.
Finally, accessibility should never be an afterthought in CUI design. Ensuring that your chatbot is usable for individuals with disabilities can involve implementing keyboard navigation and screen reader support. JavaScript can help enhance accessibility features by managing focus states and providing ARIA roles for UI elements.
document.getElementById('send-button').addEventListener('keypress', function(event) { if (event.key === 'Enter') { const userInput = document.getElementById('user-input').value; if (userInput) { sendMessageToBot(userInput); document.getElementById('user-input').value = ''; // Clear input field } } });
In this snippet, we listen for the ‘Enter’ key press on the send button, enabling users to submit their messages conveniently. Such enhancements ensure that the chatbot is accessible to a wider audience, promoting inclusivity.
The design of conversational user interfaces using JavaScript is a balancing act, combining usability, responsiveness, and accessibility. By focusing on these core principles, developers can create chatbots that not only engage users but also offer them an experience that feels both intuitive and enjoyable.
Integrating APIs for Enhanced Chatbot Functionality
Integrating APIs into chatbot applications is a game changer, allowing developers to enhance functionality and enrich user experiences. A chatbot can only be as intelligent as the data it can access, and APIs provide a bridge to a wealth of external information and services. With JavaScript, integrating APIs becomes a simpler task, enabling chatbots to understand context, retrieve relevant data, and provide useful responses.
APIs can serve various purposes in chatbot development, such as fetching weather updates, retrieving user data, or interacting with third-party services like payment gateways or social media platforms. By using JavaScript’s asynchronous capabilities, developers can make API calls without blocking the user interface, ensuring a smooth and responsive chat experience.
Let’s ponder a basic example of integrating a weather API into a chatbot. The following code snippet demonstrates how to fetch weather data using the Fetch API in JavaScript:
const fetchWeather = async (city) => { const apiKey = 'YOUR_API_KEY'; // Replace with your weather API key const response = await fetch(`https://api.openweathermap.org/data/2.5/weather?q=${city}&appid=${apiKey}&units=metric`); if (!response.ok) { throw new Error('Weather data not found'); } const data = await response.json(); return data; };
In this example, the fetchWeather
function takes a city name as input and constructs a URL for the OpenWeatherMap API. By using the Fetch API, we can get the weather data asynchronously. This function also handles potential errors, ensuring that the bot can gracefully inform users if the requested information is unavailable.
Once we have the weather data, we can integrate it into the chatbot’s response logic. Here’s how you might enhance the existing message-handling function to include weather information:
async function handleUserMessage(message) { conversationState.userMessages.push(message); if (message.toLowerCase().startsWith('weather in')) { const city = message.split('weather in')[1].trim(); try { const weatherData = await fetchWeather(city); const botResponse = `The current temperature in ${city} is ${weatherData.main.temp}°C with ${weatherData.weather[0].description}.`; conversationState.botResponses.push(botResponse); } catch (error) { conversationState.botResponses.push('Sorry, I could not fetch the weather data for that location.'); } } else { const botResponse = getBotResponse(conversationState.currentStep, message); conversationState.botResponses.push(botResponse); } conversationState.currentStep++; displayConversation(); }
In this modified handleUserMessage
function, we check if the user input requests weather information. If so, we extract the city name and call the fetchWeather
function. If the API returns valid data, the chatbot crafts a response that includes the current temperature and weather conditions. If there’s an error, it provides a user-friendly message instead.
Moreover, integrating APIs can also involve handling user authentication or personalization. For instance, if your chatbot needs to remember user preferences or store conversation history, you might integrate with a database API. This can be achieved using libraries like Axios for making HTTP requests:
const axios = require('axios'); const saveUserPreferences = async (userId, preferences) => { try { const response = await axios.post(`https://example.com/api/preferences`, { userId, preferences }); return response.data; } catch (error) { console.error('Error saving user preferences:', error); } };
In this example, we utilize Axios to post user preferences to a hypothetical API endpoint. By handling this in an asynchronous manner, we ensure that the chatbot remains responsive while the data is being saved.
JavaScript’s versatility allows for easy integration of a wide array of APIs, transforming a simple chatbot into a powerful tool capable of delivering personalized content and services. As developers explore these possibilities, the potential applications of chatbots continue to expand, providing users with increasingly meaningful interactions that leverage real-time data and personalized responses.