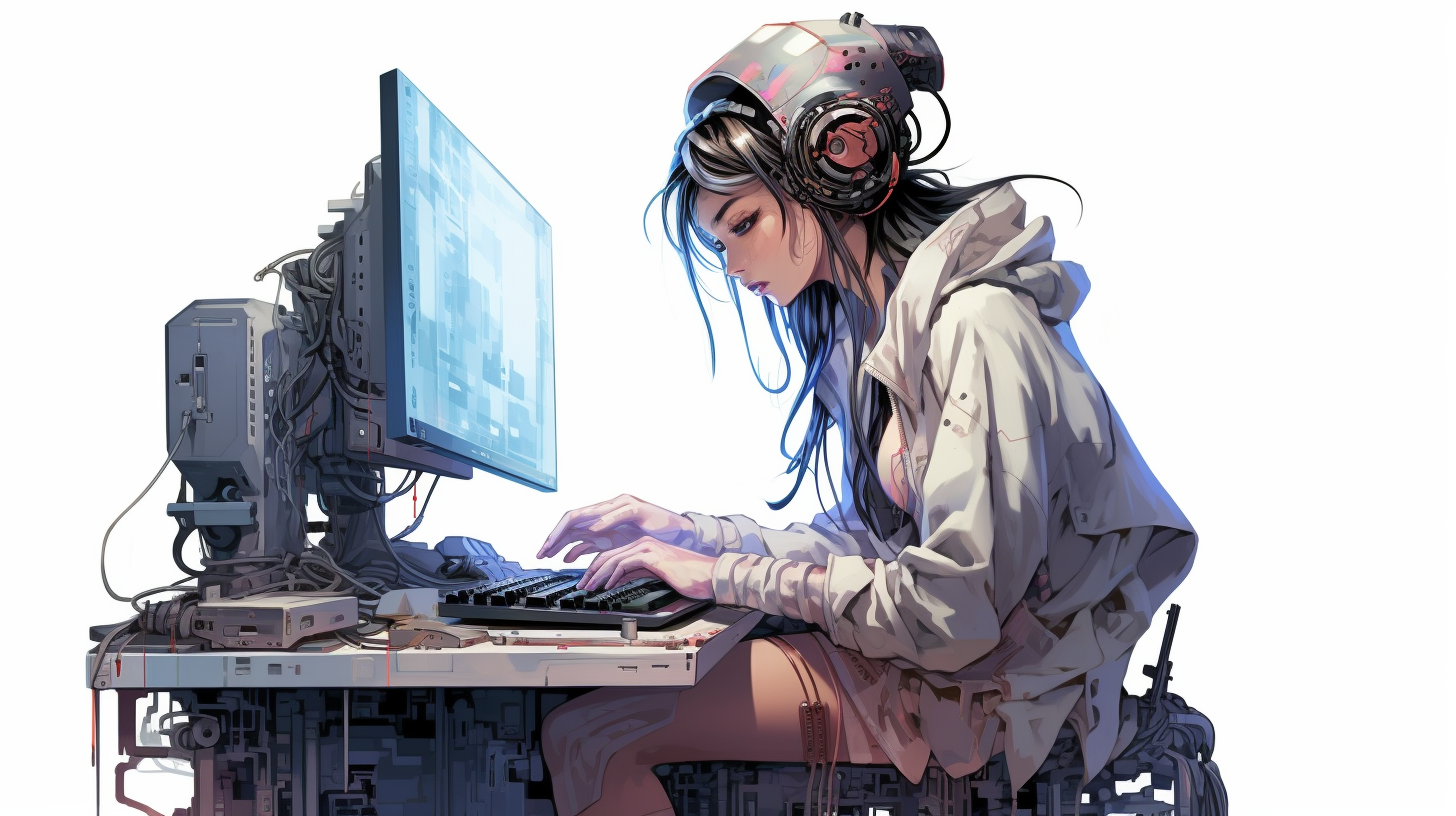
JavaScript and Custom Web Components
Web components are a set of standardized APIs that allow you to create reusable UI components that encapsulate both functionality and styling. At their core, web components consist of three main technologies: Custom Elements, Shadow DOM, and HTML Templates. Understanding these fundamentals especially important for using the full power of web components in your applications.
Custom Elements allow developers to define new HTML tags, complete with their own behavior and lifecycle. This is achieved by extending the native HTMLElement
class. With custom elements, you can create tags like or
that behave according to your specifications.
class MyButton extends HTMLElement { constructor() { super(); this.attachShadow({ mode: 'open' }); const button = document.createElement('button'); button.textContent = this.getAttribute('label'); this.shadowRoot.append(button); } } customElements.define('my-button', MyButton);
The above example showcases the creation of a simple custom button element. When you define your custom elements, you can take advantage of lifecycle callbacks such as connectedCallback
, disconnectedCallback
, and attributeChangedCallback
to manage your component’s behavior during various stages of its existence in the document.
Next up is the Shadow DOM, which provides a way to encapsulate styles and markup, preventing them from being affected by the global document styles. That’s akin to creating a mini-document within your main document, providing a clean separation of concerns. When you attach a shadow root to a custom element, you can define its own unique DOM tree.
class MyCard extends HTMLElement { constructor() { super(); const shadow = this.attachShadow({ mode: 'open' }); const wrapper = document.createElement('div'); wrapper.setAttribute('class', 'card'); const style = document.createElement('style'); style.textContent = ` .card { border: 1px solid #ccc; padding: 16px; border-radius: 5px; } `; shadow.appendChild(style); shadow.appendChild(wrapper); } } customElements.define('my-card', MyCard);
Here, the MyCard
element encapsulates its styling within its shadow DOM, allowing you to avoid conflicts with other page styles.
Finally, we have HTML Templates, which enable you to define markup that can be reused throughout your application. By using the tag, you can declare a block of HTML that isn’t rendered immediately. Instead, this template can be instantiated whenever needed, making your components more dynamic and efficient.
<template id="my-template"> <style> .content { color: blue; } </style> <div class="content">Hello, World!</div> </template>
With these fundamental building blocks, web components can dramatically improve the modularity and reusability of your code, making your web applications easier to maintain and scale. By mastering these concepts, you can create robust and flexible UI components that enhance user experience across your web projects.
Creating Custom Elements
Creating custom elements is a simpler yet powerful feature of the Web Components specification. When you define a custom element, you can introduce new, reusable HTML tags that encapsulate specific functionality and styling tailored to your application’s needs. This helps maintain a clean separation of UI concerns while promoting component reuse.
The process of creating a custom element begins with extending the base HTMLElement class. Here’s a simple example of creating a custom greeting element:
class MyGreeting extends HTMLElement { constructor() { super(); const shadow = this.attachShadow({ mode: 'open' }); const greeting = document.createElement('p'); greeting.textContent = `Hello, ${this.getAttribute('name') || 'World'}!`; shadow.appendChild(greeting); } } customElements.define('my-greeting', MyGreeting);
In this example, we see a custom element named . The constructor initializes the element, and we attach a shadow DOM to it. The
tag displays a greeting message, which can dynamically change based on the ‘name’ attribute passed to it. If no name is provided, it defaults to “World”.
Custom elements can also include lifecycle callbacks that allow you to hook into various stages of their existence. For instance, the connectedCallback method runs when the element is added to the document, which is an ideal place to initiate any necessary work, such as fetching data or adding event listeners. Here’s how you might enhance the previous example:
class MyGreeting extends HTMLElement { constructor() { super(); this.shadow = this.attachShadow({ mode: 'open' }); this.greeting = document.createElement('p'); this.shadow.appendChild(this.greeting); } connectedCallback() { this.updateGreeting(); } static get observedAttributes() { return ['name']; } attributeChangedCallback(name, oldValue, newValue) { if (name === 'name') { this.updateGreeting(); } } updateGreeting() { this.greeting.textContent = `Hello, ${this.getAttribute('name') || 'World'}!`; } } customElements.define('my-greeting', MyGreeting);
In this enhanced version, we add the connectedCallback method to initialize the greeting when the element is first attached to the DOM. Additionally, we define observedAttributes to react to changes in the ‘name’ attribute, allowing the greeting to update in real-time when the attribute changes.
Creating custom elements opens up numerous possibilities for building encapsulated, reusable components in your applications. By using their lifecycle methods and attributes, you can create sophisticated UI elements that maintain their own state and behavior, contributing to a more organized and maintainable codebase.
Using Shadow DOM for Encapsulation
The Shadow DOM is a powerful feature of web components that provides encapsulation for your custom elements. When you create a shadow root for a custom element, you essentially create a boundary around its internal DOM. This isolation means that styles defined in the shadow DOM won’t bleed out into the main document, nor will external styles affect the components inside the shadow root.
To illustrate this encapsulation, ponder a custom element that visually represents a notification badge. By using Shadow DOM, we can ensure that our badge styles remain unaffected by the global styles of the page.
class NotificationBadge extends HTMLElement { constructor() { super(); const shadow = this.attachShadow({ mode: 'open' }); // Create a wrapper div for the badge const wrapper = document.createElement('div'); wrapper.setAttribute('class', 'badge'); wrapper.textContent = this.getAttribute('count') || '0'; // Create style element for encapsulated styles const style = document.createElement('style'); style.textContent = ` .badge { display: inline-block; background-color: red; color: white; border-radius: 12px; padding: 5px 10px; font-weight: bold; } `; // Append styles and wrapper to the shadow root shadow.appendChild(style); shadow.appendChild(wrapper); } } customElements.define('notification-badge', NotificationBadge);
In this example, the NotificationBadge
custom element creates a badge that displays a count. The styles applied to the badge are confined within its shadow DOM, ensuring that they do not interfere with any other styles on the page. This is a significant advantage, especially in complex applications where style conflicts can lead to unexpected behavior and visual glitches.
Moreover, the encapsulation provided by the Shadow DOM allows you to create components that can be reused across different contexts without worrying about external influences. For instance, you can have multiple notification-badge
elements on a page, each with its own count, and the styling will remain consistent regardless of the surrounding content.
const badge1 = document.createElement('notification-badge'); badge1.setAttribute('count', '5'); document.body.appendChild(badge1); const badge2 = document.createElement('notification-badge'); badge2.setAttribute('count', '10'); document.body.appendChild(badge2);
This example demonstrates how you can instantiate multiple badges, each with different values, while maintaining their unique styles. The Shadow DOM not only provides a way to control styles but also acts as a barrier that prevents JavaScript events from affecting the outer document unless explicitly allowed.
Using the Shadow DOM for encapsulation very important for building robust web components. It allows you to create self-contained components that are less prone to conflicts, making your web applications more manageable and scalable. This encapsulation empowers developers to innovate without the fear of unintentional side effects across different parts of the user interface.
Defining Templates and Slots in Custom Components
When it comes to defining templates and slots in custom components, the HTML tag plays a pivotal role. It allows developers to declare a block of HTML that’s not rendered immediately. Instead, this template can be cloned and inserted into the DOM whenever it’s needed, enabling dynamic content creation and rendering efficiency. This is especially useful for custom components where you want to define a consistent structure that can be reused multiple times.
The element contains markup that can include styles, scripts, and other elements without being part of the main document flow. Templates provide a way to separate the design of a component from its logic, enhancing maintainability and clarity in your codebase.
Here’s an example of how to define and use a template within a custom element:
class MyList extends HTMLElement { constructor() { super(); const shadow = this.attachShadow({ mode: 'open' }); // Define a template const template = document.createElement('template'); template.innerHTML = ` .list { list-style-type: none; padding: 0; } .list-item { background: #f0f0f0; margin: 5px; padding: 10px; border-radius: 4px; }
In the example above, we create a custom element with an internal HTML template that defines the structure and styles for the list. When the component is instantiated, we append the cloned content of the template to the shadow DOM.
To add items to the list, the addItem
method creates new list items and appends them to the
- element in the shadow DOM. This method illustrates how dynamic content can be easily manipulated while maintaining the encapsulation provided by the shadow DOM.
Another powerful feature of templates comes into play with the introduction of slots. The element allows you to define placeholders in your custom components where users can insert their own markup. This provides a way to create flexible and reusable components that can adapt to different contexts without sacrificing structure or style.
Ponder the following example where we enhance our list component to include slots:
class MyListWithSlot extends HTMLElement { constructor() { super(); const shadow = this.attachShadow({ mode: 'open' }); const template = document.createElement('template'); template.innerHTML = ` .list { list-style-type: none; padding: 0; } .list-item { background: #f0f0f0; margin: 5px; padding: 10px; border-radius: 4px; }
In this modification, we define a slot inside the
- element. When users of the
component add their own content between the opening and closing tags, that content will be projected into the slot:
This approach fosters flexibility, allowing developers to create components that can be easily customized while still retaining their core functionality. Slots open the door to a new level of component composition, making it possible to design intricate UI elements that can adapt to their surroundings dynamically.
By effectively using templates and slots, you can build highly reusable and flexible web components that encapsulate both style and functionality, paving the way for robust web applications with well-structured and maintainable code.
Integrating Custom Components with Frameworks
Integrating custom components into modern frameworks is a simpler process, yet it offers the potential to create highly dynamic and reusable UI elements. Whether you’re using React, Vue, Angular, or any other framework, custom elements can be seamlessly plugged into your application, enhancing its modularity and component-based architecture. The beauty of web components lies in their standardization, which ensures that no matter the framework, their functionality remains consistent.
Let’s explore how to integrate a custom element into a framework by using React as an example. Consider we have previously defined a custom button element called my-button
. To use this custom element within a React component, we can simply include it like any other HTML element.
class App extends React.Component { render() { return (); } } ReactDOM.render(, document.getElementById('root'));My Web Components in React
In this example, the my-button
element is directly used within the React component’s JSX. React will treat this custom element just like any other HTML tag. This is a powerful feature because it allows you to leverage the reusability of web components while still using the component-based approach of React.
However, web components and frameworks can also have some interoperability issues, particularly with properties and events. When you work with custom elements in React, it is essential to remember that attributes on custom elements must be in kebab-case (hyphen-separated), while properties in React are camelCase. For instance, setting a property like label
should be done using setAttribute
if you need to update it dynamically.
class App extends React.Component { handleClick = () => { const button = document.querySelector('my-button'); button.setAttribute('label', 'Clicked!'); }; render() { return (); } } ReactDOM.render(, document.getElementById('root'));My Web Components in React
In this updated example, we add a click handler that updates the label of the my-button
element when it’s clicked. This demonstrates how to interact with custom elements and manage their state from within a React component.
When integrating with other frameworks like Vue or Angular, the approach is similar. In Vue, you can simply register the custom element and use it in your templates:
Vue.component('my-button', { template: '', }); new Vue({ el: '#app', });
Angular also allows the use of custom elements by registering them as Angular components. You can define a custom element in your Angular module and include it in your templates accordingly. This flexibility afforded by web components ensures that you can create a consistent design language across different parts of your application, regardless of the framework used.
Moreover, web components can emit events that can be listened to in your framework’s context. For instance, if my-button
emits a click event, you can listen for it within your framework’s event handling system:
class App extends React.Component { componentDidMount() { const button = document.querySelector('my-button'); button.addEventListener('click', () => { alert('Button clicked!'); }); } render() { return (); } } ReactDOM.render(, document.getElementById('root'));My Web Components in React
Integrating custom web components into modern frameworks is a powerful way to leverage the benefits of encapsulated and reusable UI elements. By understanding the nuances of both web components and your framework of choice, you can create a seamless user experience that maintains the encapsulation and usability of custom elements across your applications.
Best Practices for Performance and Accessibility
When developing custom web components, ensuring optimal performance and accessibility should be a priority. This not only enhances user experience but also aids in meeting compliance standards. Here, we explore some best practices to follow when integrating performance and accessibility features into your custom elements.
1. Minimize Reflows and Repaints: The way you manipulate the DOM can significantly impact your application’s performance. When creating or updating elements, aim to minimize reflows and repaints by making changes to the DOM in batches. For instance, instead of updating styles or attributes one at a time, try to group these changes. Using the shadow DOM helps here, as it allows for encapsulated styling without affecting the global document.
const myElement = document.querySelector('my-element'); const style = myElement.shadowRoot.querySelector('style'); style.textContent += '.new-class { color: blue; }';
2. Debounce Input Events: If your custom component responds to user input, such as text changes or scroll events, implement a debounce mechanism to limit the frequency at which these events are processed. This prevents excessive function calls that can degrade performance, especially in scenarios where the user interacts with your component rapidly.
let timeout; inputElement.addEventListener('input', (event) => { clearTimeout(timeout); timeout = setTimeout(() => { console.log(`Input Value: ${event.target.value}`); }, 300); });
3. Optimize Asset Loading: Ensure that any assets used in your custom components, such as images or stylesheets, are optimized for the web. Use modern formats like WebP for images and minify CSS and JavaScript files. Implement lazy loading for images that are not immediately visible to the user, which can help improve the initial load time of your application.
const lazyLoadImage = (img) => { const observer = new IntersectionObserver((entries) => { entries.forEach(entry => { if (entry.isIntersecting) { const image = entry.target; image.src = image.dataset.src; observer.unobserve(image); } }); }); observer.observe(img); };
4. Ensure Accessibility (a11y): Accessibility should be at the forefront of your design process. Use semantic HTML wherever possible, and ensure your custom elements are keyboard navigable. Provide appropriate ARIA (Accessible Rich Internet Applications) roles and attributes to inform assistive technologies about your components’ functionalities.
class AccessibleButton extends HTMLElement { constructor() { super(); this.attachShadow({ mode: 'open' }); const button = document.createElement('button'); button.setAttribute('role', 'button'); button.setAttribute('tabindex', '0'); button.textContent = this.getAttribute('label'); this.shadowRoot.appendChild(button); } } customElements.define('accessible-button', AccessibleButton);
5. Provide Feedback and Error Handling: Properly inform users of the outcome of their interactions with your custom components. This includes providing visual feedback for actions such as button clicks or form submissions. Additionally, ensure that any errors are clearly communicated to users, guiding them on how to resolve issues.
const formButton = document.querySelector('submit-button'); formButton.addEventListener('click', () => { if (!isValid()) { alert('Please fill out all required fields.'); } else { submitForm(); } });
By adhering to these best practices, your custom web components will not only perform well but also be accessible to a broader audience. This approach will foster an inclusive web experience while ensuring that your components are robust and maintainable over time. The focus on performance and accessibility will lead to a more seamless integration of custom components into any web application, ultimately enhancing the overall user experience.