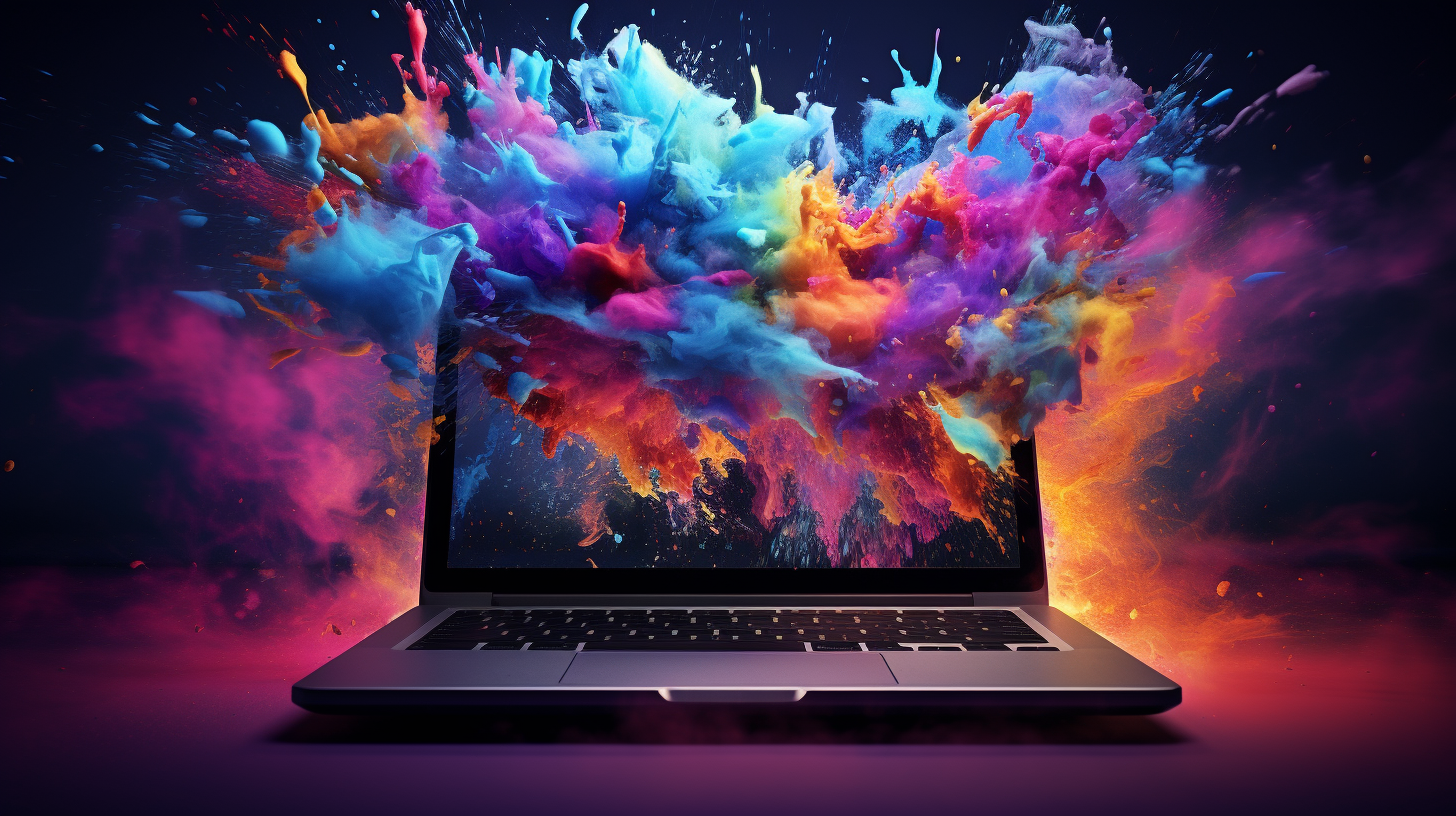
JavaScript and Data Backup Solutions
In the sphere of JavaScript applications, the significance of data backup cannot be overstated. As developers, we often sit at the intersection of user expectations and technical limitations, where data integrity and availability can make or break the user experience. A lost connection, a failed API response, or a simple browser crash can lead to data loss that’s not just inconvenient, but potentially disastrous. Thus, understanding the nuances of data backup is important for any serious JavaScript developer.
JavaScript applications are increasingly complex, often handling vast amounts of data generated through user interactions, real-time updates, and server communications. In Single Page Applications (SPAs) and Progressive Web Apps (PWAs), user data is frequently stored in local storage, session storage, or even indexed databases. These storage solutions, while efficient, come with their own set of vulnerabilities. A power failure or a browser update can wipe out the stored data, highlighting the need for a robust backup strategy.
Moreover, as applications scale, the need for data consistency across multiple sessions and devices becomes essential. Users expect their preferences, progress, and critical information to remain intact regardless of device changes or application updates. Here, backup solutions play a pivotal role, ensuring that data remains consistent, reliable, and accessible.
Additionally, legal and ethical obligations regarding user data cannot be ignored. With regulations like GDPR and CCPA, developers must ensure that personal data is protected, which includes having a solid backup plan. Failure to comply can result in severe penalties, making it not just a technical requirement but a legal necessity.
From a technical perspective, the processes behind backing up data can be automated and integrated seamlessly within the application lifecycle. This allows developers to focus on creating feature-rich, simple to operate experiences without constantly worrying about unexpected data loss. Implementing data backup as a core component of the application architecture is not just a best practice; it is a fundamental requirement in today’s digital landscape.
Here’s a simple example of creating a backup of local storage data using JavaScript:
const backupLocalStorage = () => { const backup = {}; for (let i = 0; i < localStorage.length; i++) { const key = localStorage.key(i); backup[key] = localStorage.getItem(key); } return JSON.stringify(backup); }; // Usage const localStorageBackup = backupLocalStorage(); console.log(localStorageBackup);
This code snippet demonstrates how to traverse the local storage and create a JSON string representing the data that can be stored or sent to a server for safekeeping. This approach lays the groundwork for a more comprehensive backup strategy that can be built upon as application needs evolve.
Popular Data Backup Techniques for JavaScript Developers
As JavaScript developers explore the landscape of data backup, several techniques emerge that cater to different needs and scenarios. Understanding these techniques very important, as they provide varying levels of complexity, scalability, and reliability, allowing developers to choose the right fit for their specific application requirements.
One prevalent technique is using browser-based solutions, such as localStorage and sessionStorage. While these storage options offer convenience for smaller datasets, they are inherently limited in terms of capacity and durability. LocalStorage can persist data even after the browser is closed, while sessionStorage is limited to the duration of the page session. Here’s an example demonstrating how to save and retrieve data using these storage options:
// Saving data to localStorage localStorage.setItem('key', 'value'); // Retrieving data from localStorage const value = localStorage.getItem('key'); console.log(value); // Output: value
For applications dealing with larger datasets or needing more structured storage, IndexedDB serves as a more robust alternative. IndexedDB provides asynchronous access to a more complex data structure, enabling developers to store significant amounts of data in a transactional database. Below is a fundamental example of how to use IndexedDB for storing and retrieving objects:
// Open (or create) a database const request = indexedDB.open('MyDatabase', 1); request.onupgradeneeded = (event) => { const db = event.target.result; db.createObjectStore('myStore', { keyPath: 'id' }); }; request.onsuccess = (event) => { const db = event.target.result; // Add data to the store const transaction = db.transaction('myStore', 'readwrite'); const store = transaction.objectStore('myStore'); store.add({ id: 1, name: 'Item 1' }); }; // Retrieve data from the store const getRequest = db.transaction('myStore').objectStore('myStore').get(1); getRequest.onsuccess = (event) => { console.log(event.target.result); // Output: { id: 1, name: 'Item 1' } };
In more advanced scenarios, developers may opt for cloud-based backup solutions, where data is transmitted and stored on remote servers. This technique is particularly beneficial for applications that require data consistency across multiple devices or user accounts. Using APIs to send data to cloud services can automate backup processes seamlessly. For instance, consider the following example of sending local storage data to a hypothetical cloud API:
const backupToCloud = async (data) => { try { const response = await fetch('https://api.example.com/backup', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify(data) }); const result = await response.json(); console.log('Backup successful:', result); } catch (error) { console.error('Backup failed:', error); } }; // Usage const localStorageData = backupLocalStorage(); backupToCloud(localStorageData);
In conjunction with these techniques, developers may also integrate versioning systems that enable snapshots of the data at various points. This not only assists in backup but also facilitates easy rollbacks in case a previous version of the data is required. Such systems can be built using a combination of local storage and server-side versions, providing a comprehensive safety net for critical data.
Ultimately, the choice of backup technique will depend on the application’s specific requirements, the expected volume of data, the need for accessibility, and the complexity developers are willing to manage. By understanding and implementing these diverse backup strategies, JavaScript developers can establish a resilient data management framework, significantly reducing the risk of data loss in their applications.
Implementing Automated Backup Solutions in JavaScript
Implementing automated backup solutions in JavaScript requires a strategic approach that integrates these techniques into the application seamlessly. The goal is to create a system that not only backs up data periodically but also responds to specific triggers—like user actions or application states—to ensure data integrity without overwhelming the user with manual backup processes. Below are some methods and examples to help you automate data backups in JavaScript applications.
One effective way to implement automated backups is by using setInterval to create a timed backup mechanism. This method allows developers to schedule backups at regular intervals, ensuring that data is consistently saved without needing user intervention. Here’s a simple implementation:
const autoBackup = () => { const backupData = backupLocalStorage(); // using the previous function backupToCloud(backupData); // using cloud backup function }; // Schedule backups every 15 minutes setInterval(autoBackup, 15 * 60 * 1000);
This code snippet sets up a backup every 15 minutes, automatically sending local storage data to the cloud. It’s crucial to ponder how this might impact performance and user experience, especially with larger datasets. Thus, developers should gauge the appropriate frequency based on their application’s specific needs.
In addition to timed backups, responding to user events can significantly enhance the backup process. For instance, you might want to trigger a backup when a user saves a significant change in the application or closes a tab. This can be achieved using the beforeunload event.
window.addEventListener('beforeunload', (event) => { const backupData = backupLocalStorage(); backupToCloud(backupData); });
This example ensures that any time a user attempts to leave the page, their data will be backed up. While that is a powerful approach, care must be taken to avoid performance degradation during the backup process, particularly if the data size is substantial.
For applications that require enhanced data consistency across different user sessions or devices, implementing a version control mechanism can be beneficial. This involves not only backing up the current state but also keeping track of different states of the data over time. You can manage this by maintaining a history of backups and allowing users to revert to previous states when necessary.
const versionedBackup = () => { const history = JSON.parse(localStorage.getItem('backupHistory')) || []; const currentBackup = backupLocalStorage(); // Limit history to the last 5 backups if (history.length >= 5) { history.shift(); // Remove the oldest backup } history.push(currentBackup); localStorage.setItem('backupHistory', JSON.stringify(history)); }; // Call versioned backup at specified intervals or triggers setInterval(versionedBackup, 30 * 60 * 1000); // Backup every 30 minutes
This code allows you to keep a history of backups in local storage, ensuring that users can access prior versions of their data. This can be particularly useful in applications where data changes frequently, and users might need to revert to an earlier state.
Lastly, it is vital to consider user notifications during the backup process. A background backup might go unnoticed, but communicating progress or success can enhance user trust. You can use simple alert messages or more sophisticated UI notifications to inform users about the backup status.
const notifyUser = (message) => { alert(message); // Replace with a more sophisticated notification system if desired }; // Example usage backupToCloud(backupData) .then(() => notifyUser('Backup successful!')) .catch(() => notifyUser('Backup failed, please try again.'));
By seamlessly integrating these automated backup solutions into the JavaScript application lifecycle, developers can create a more resilient data management framework. Users will not only feel secure about their data but also appreciate the smooth functionality of the application, allowing developers to focus on building features rather than worrying about potential data loss.
Best Practices for Data Recovery and Restoration in JavaScript
Data recovery and restoration are critical aspects of the data management lifecycle, particularly within JavaScript applications where user data is often transient and highly dependent on client-side storage mechanisms. Understanding best practices for recovery and restoration can empower developers to mitigate data loss and enhance user trust in their applications.
Firstly, it’s essential to establish a clear recovery strategy that outlines how data will be restored in various scenarios, such as application crashes, browser updates, or user errors. A well-documented recovery plan should include procedures for restoring data from backups and guidelines for data integrity checks. This plan should also account for different storage types, such as localStorage, SessionStorage, or IndexedDB, and how to recover data from each.
One of the foundational practices for effective data recovery involves regular integrity checks. By routinely verifying the state of stored data, developers can identify potential corruption or loss before it impacts the user experience. Here’s a simple implementation demonstrating how to check for the presence of expected data in localStorage:
const checkDataIntegrity = (key) => { if (localStorage.getItem(key) === null) { console.warn(`Missing data for key: ${key}`); // Trigger recovery process or notify user } }; // Example usage checkDataIntegrity('importantData');
In addition to integrity checks, implementing a rollback mechanism can be invaluable. This allows users to restore previous versions of their data in case of unexpected changes or deletions. Using the earlier versioning backup technique, developers can provide users with a simple interface to select and revert to previous data states. Below is an example of how one might implement a rollback function:
const rollbackData = (versionIndex) => { const history = JSON.parse(localStorage.getItem('backupHistory')) || []; if (versionIndex = history.length) { console.error('Invalid version index'); return; } const dataToRestore = history[versionIndex]; for (const [key, value] of Object.entries(dataToRestore)) { localStorage.setItem(key, value); } console.log('Data restored to version:', versionIndex); }; // Example usage rollbackData(2); // Restores data to the state from the third backup
Another critical best practice in data recovery is to facilitate user-triggered backups. This strategy allows users to take control of their data, ensuring they feel secure about the state of their information. Implementing a manual backup button alongside automatic backups can significantly enhance user confidence. Here’s how one could provide a simpler backup option:
const manualBackupButton = document.getElementById('manualBackup'); manualBackupButton.addEventListener('click', () => { const backupData = backupLocalStorage(); // Reuses the backup function backupToCloud(backupData); alert('Manual backup initiated!'); });
Moreover, developers should ensure that the recovery process is transparent and effortless to handle. Displaying notifications about the status of recovery actions—whether successful or failed—can significantly improve user experience. Here’s an example of how to implement user notifications during the restoration process:
const notifyUserRecovery = (status) => { const message = status ? 'Data recovery successful!' : 'Data recovery failed. Please check your backups.'; alert(message); }; // Usage within a recovery function const recoverData = async () => { try { // Simulate recovery process await restoreFromBackup(); notifyUserRecovery(true); } catch (error) { console.error('Recovery error:', error); notifyUserRecovery(false); } };
Lastly, comprehensive logging of recovery actions plays a vital role in diagnosing issues and improving the recovery strategy over time. Developers should keep track of recovery attempts, the state of the data before and after restoration, and any errors encountered during the process. This information can help refine both backup and recovery processes for more effective data management.
By adopting these best practices in data recovery and restoration, JavaScript developers can significantly enhance the reliability of their applications. Implementing robust recovery strategies not only safeguards user data but also fosters a sense of trust and security, which is paramount in today’s data-driven world.