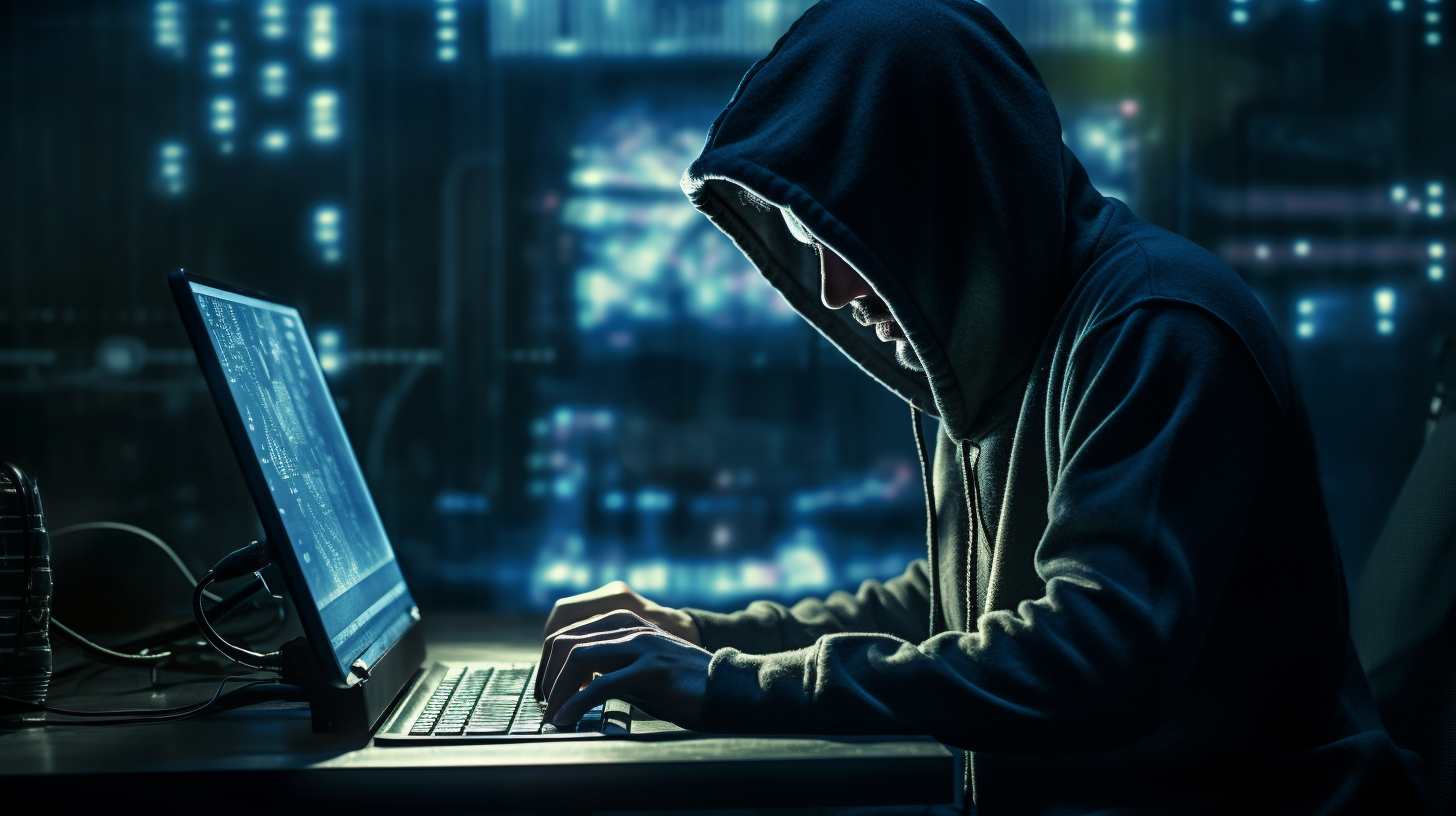
JavaScript and Dynamic Web Content
JavaScript plays a pivotal role in the context of dynamic web content, transforming static pages into interactive experiences that respond to user input and environmental factors. At its core, JavaScript allows developers to create a seamless flow of information on web pages, enhancing user engagement and satisfaction.
One of the primary functions of JavaScript in dynamic content creation is its ability to manipulate the Document Object Model (DOM). The DOM represents the structure of a web page, consisting of elements like headings, paragraphs, and images as nodes in a tree. When JavaScript is employed to modify the DOM, it can dynamically change the content, structure, and style of a page without requiring a full reload. This leads to a more fluid user experience and reduces server load.
Ponder a scenario where a user interacts with a button to fetch data from an external API. With JavaScript, you can capture that event, send an asynchronous request, and update the page with new content without disrupting the user’s flow. Here’s how that might look:
const button = document.getElementById('fetchDataButton'); button.addEventListener('click', () => { fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { const contentDiv = document.getElementById('content'); contentDiv.innerHTML = `${data.message}
`; }) .catch(error => console.error('Error fetching data:', error)); });
In this example, when a user clicks the button, JavaScript captures the action and triggers a fetch request to retrieve data from a remote server. The response is then used to update the content of a specific div
on the page, showcasing how JavaScript can adapt the user interface in real-time.
Moreover, JavaScript’s asynchronous capabilities, provided by promises and the async/await syntax, enable developers to fetch data or perform other time-consuming operations without freezing the UI. This non-blocking behavior very important for maintaining a responsive web application, ensuring that users can continue to interact with other elements while waiting for data to load.
Additionally, JavaScript frameworks and libraries like React, Angular, and Vue.js further streamline the process of creating dynamic web content. These tools offer powerful abstractions for managing state and rendering UI components efficiently, making it easier to build interactive applications while using the full potential of JavaScript’s capabilities in dynamic content creation.
The integration of JavaScript with other web technologies, such as HTML and CSS, enhances its effectiveness in creating rich user experiences. By combining these languages, developers can craft applications that not only react to user interactions but also exhibit a polished visual presentation. This harmonious relationship is the cornerstone of modern web development.
Key Features of JavaScript for Enhancing User Experience
JavaScript is endowed with a suite of features that significantly enhance user experience, bringing interactivity and responsiveness to the forefront of web applications. One of the most notable features is event handling, which allows developers to define specific actions in response to user behaviors, such as clicks, hovers, and key presses. By capturing these events, JavaScript enables a nuanced interaction model that can adapt dynamically to user inputs.
For instance, consider a scenario where you want to provide instant feedback as users fill out a form. By employing JavaScript to listen for input events, you can display validation messages in real-time. The following example demonstrates how to validate an email input field:
const emailInput = document.getElementById('email'); const feedback = document.getElementById('feedback'); emailInput.addEventListener('input', () => { const emailValue = emailInput.value; const isValidEmail = /^[^s@]+@[^s@]+.[^s@]+$/.test(emailValue); feedback.textContent = isValidEmail ? 'Valid email address!' : 'Invalid email address.'; });
This snippet provides immediate feedback, allowing users to correct mistakes before submitting the form, thereby improving their overall experience.
Another key feature of JavaScript is its ability to manipulate CSS styles directly through the DOM. This allows for dynamic styling based on user interactions or application state. For example, you might want to highlight menu items when the user hovers over them. The following code illustrates how to achieve this:
const menuItems = document.querySelectorAll('.menu-item'); menuItems.forEach(item => { item.addEventListener('mouseover', () => { item.style.backgroundColor = '#f0f0f0'; }); item.addEventListener('mouseout', () => { item.style.backgroundColor = 'transparent'; }); });
This not only enhances visual feedback but also contributes to a more engaging user experience by making the interface feel more responsive.
JavaScript also excels in providing interactive visual elements. Libraries such as D3.js and Chart.js allow developers to integrate complex data visualizations seamlessly into web pages. This capability is essential for applications that rely on data representation, empowering users to interpret data through interactive charts and graphics. Ponder the following example using Chart.js to create a simple pie chart:
const ctx = document.getElementById('myChart').getContext('2d'); const myChart = new Chart(ctx, { type: 'pie', data: { labels: ['Red', 'Blue', 'Yellow'], datasets: [{ label: '# of Votes', data: [12, 19, 3], backgroundColor: [ 'rgba(255, 99, 132, 0.2)', 'rgba(54, 162, 235, 0.2)', 'rgba(255, 206, 86, 0.2)' ], borderColor: [ 'rgba(255, 99, 132, 1)', 'rgba(54, 162, 235, 1)', 'rgba(255, 206, 86, 1)' ], borderWidth: 1 }] }, options: { responsive: true, plugins: { legend: { position: 'top', }, title: { display: true, text: 'Vote Distribution' } } } });
This code encapsulates the simplicity and power of integrating dynamic charts into web applications, allowing users to engage with data more intuitively.
All these features converge to create a robust platform for enhancing user experience. The ability to listen to user events, dynamically change styles, and visualize data transforms passive interactions into active engagements, ensuring that users remain connected and invested in the content. Consequently, JavaScript stands as a foundational technology in the development of modern, interactive web applications, where user experience is paramount.
Techniques for Manipulating the DOM with JavaScript
Manipulating the DOM with JavaScript is a fundamental technique that allows developers to create interactive, dynamic web pages. Understanding how to traverse and modify the DOM effectively can significantly enhance the user experience on your website. This involves not only changing the content of elements but also adding or removing elements, altering their attributes, and dynamically responding to user interactions.
One of the most powerful features of JavaScript is its ability to select and modify elements in the DOM. The document.querySelector()
and document.querySelectorAll()
methods provide a flexible way to access DOM elements using CSS selectors. For example, let’s say you want to change the text of a heading when a user clicks a button:
const changeTextButton = document.getElementById('changeTextButton'); const heading = document.querySelector('h1'); changeTextButton.addEventListener('click', () => { heading.textContent = 'Text has been changed!'; });
In this code, we first select the button and the heading. When the button is clicked, the text content of the heading is updated. This simple interaction demonstrates how JavaScript can make the web page responsive to user actions.
For more advanced scenarios, developers often need to create new elements and append them to the DOM. This can be done using the document.createElement()
method in conjunction with appendChild()
or insertBefore()
. Here’s how you can add a new list item to an existing unordered list:
const addItemButton = document.getElementById('addItemButton'); const list = document.getElementById('itemList'); addItemButton.addEventListener('click', () => { const newItem = document.createElement('li'); newItem.textContent = 'New List Item'; list.appendChild(newItem); });
This code listens for a click event on the button and creates a new list item, adding it to the end of the list. This showcases the dynamic nature of JavaScript, allowing for the real-time addition of content based on user input.
Removing elements from the DOM is equally important in managing the content displayed to users. The remove()
method makes it simpler to delete elements. For instance, if you want to remove an item from the list when it’s clicked, you can use the following code:
const listItems = document.querySelectorAll('#itemList li'); listItems.forEach(item => { item.addEventListener('click', () => { item.remove(); }); });
This snippet adds a click event listener to each list item. When an item is clicked, it is removed from the DOM, providing users with direct interaction capabilities.
For a richer interaction experience, developers can also manipulate CSS classes to control the visual appearance of elements. The classList
property simplifies adding, removing, or toggling classes. For example, to highlight an item on hover, ponder the following:
const items = document.querySelectorAll('.highlightable'); items.forEach(item => { item.addEventListener('mouseenter', () => { item.classList.add('highlight'); }); item.addEventListener('mouseleave', () => { item.classList.remove('highlight'); }); });
In this code, we add a ‘highlight’ class to an item when the mouse enters and remove it when the mouse leaves. This approach enriches user interaction, making the web page feel more alive.
Beyond these basic operations, JavaScript provides robust event delegation capabilities, which can optimize performance when dealing with many elements. Instead of attaching event listeners to each individual item, you can attach a single listener to a parent element. Here’s an illustration using a parent list:
const listContainer = document.getElementById('itemList'); listContainer.addEventListener('click', (event) => { if (event.target.tagName === 'LI') { event.target.remove(); } });
This method allows for efficient handling of events for multiple items, as the event listener is placed on the parent listContainer
. It checks if the target clicked is an LI
element and removes it if so. This reduces the number of event listeners in the DOM and enhances performance, particularly in applications with many interactive elements.
Mastering DOM manipulation with JavaScript opens the door to creating sophisticated, engaging web applications that respond intuitively to user inputs. By using the powerful methods and properties available, developers can ensure their web pages are not only functional but also provide a compelling user experience.
Best Practices for Optimizing Dynamic Web Content Performance
When it comes to optimizing dynamic web content performance, JavaScript developers must think several best practices that can significantly enhance both the speed and efficiency of web applications. By implementing these strategies, developers can ensure that their applications not only function smoothly but also provide a responsive experience for users.
One crucial aspect of performance optimization is minimizing the amount of JavaScript code that needs to be loaded and executed. This can be achieved through techniques such as code splitting, where only the necessary code for a specific page or component is loaded initially, while other parts can be loaded asynchronously as needed. Tools like Webpack or Rollup can help automate this process. Here’s an example of using dynamic imports for code splitting:
import('module.js').then(module => { module.default(); });
This code snippet demonstrates how to load a module only when it’s needed, rather than at the initial page load, thus reducing the initial load time and improving performance.
Another essential practice is minimizing DOM manipulations. Directly manipulating the DOM can be costly in terms of performance, especially if done repeatedly in a loop or during high-frequency events like scrolling or resizing. To mitigate this, developers should batch DOM changes together or use techniques like document fragments to make changes offscreen before applying them to the DOM. An example of this can be seen in the following code that uses a document fragment to append multiple items to a list:
const list = document.getElementById('itemList'); const fragment = document.createDocumentFragment(); for (let i = 0; i < 100; i++) { const newItem = document.createElement('li'); newItem.textContent = `Item ${i + 1}`; fragment.appendChild(newItem); } list.appendChild(fragment);
In this example, the use of a document fragment allows for all list items to be created and appended in one operation, significantly improving performance compared to appending each item directly to the DOM.
Furthermore, developers should be mindful of event handling. Instead of attaching multiple event listeners to individual elements, event delegation can be employed, wherein a single listener is set on a parent element. This not only reduces memory usage but also enhances performance by minimizing the number of event listeners in the DOM. Here’s a simple illustration:
const listContainer = document.getElementById('itemList'); listContainer.addEventListener('click', (event) => { if (event.target.tagName === 'LI') { event.target.classList.toggle('selected'); } });
In this example, clicking on any list item toggles a ‘selected’ class, demonstrating the effectiveness of event delegation.
Another vital area for optimization is ensuring that JavaScript executes efficiently by using the browser’s capabilities effectively. This includes using requestAnimationFrame for animations, which allows the browser to optimize rendering and improve performance:
function animate() { // animation logic here requestAnimationFrame(animate); } requestAnimationFrame(animate);
This approach synchronizes the animation with the browser’s repaint cycles, leading to smoother animations and reduced jank.
Lastly, it’s essential to keep an eye on network requests. Minimizing the number of HTTP requests and optimizing the size of JavaScript files can lead to significant performance improvements. Techniques such as minification and gzip compression can drastically reduce file sizes. Here’s a command for minifying JavaScript files with a tool like Terser:
terser input.js -o output.min.js
By incorporating these best practices into your development workflow, you can create dynamic web applications that not only captivate users with their interactivity but also perform efficiently, ensuring a pleasant browsing experience. As the web continues to evolve, keeping optimization in focus will remain a cornerstone of successful JavaScript development.