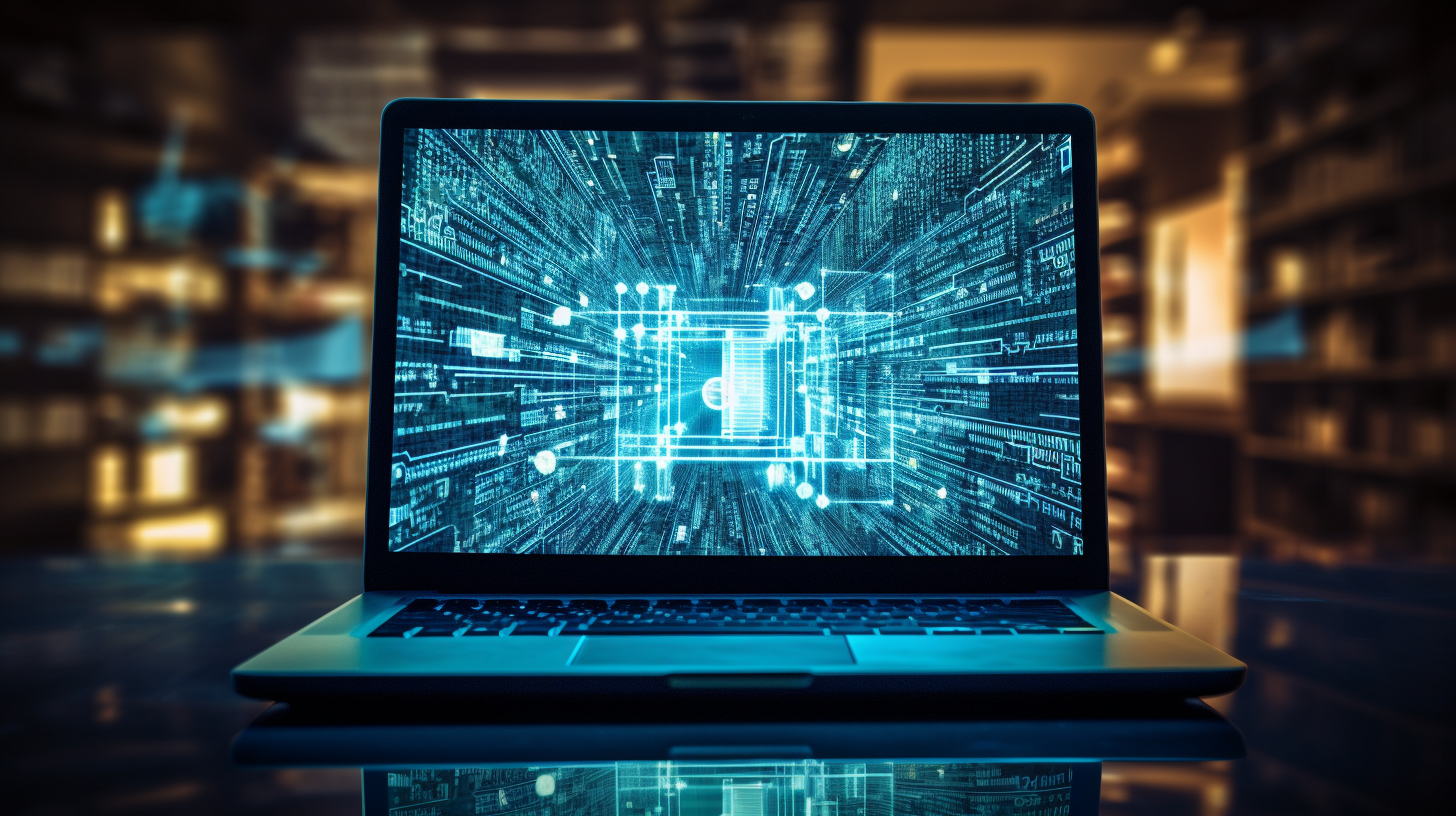
JavaScript and Email Integration
JavaScript, once primarily the language of the browser, has evolved significantly to play a vital role in email communication. At its core, JavaScript facilitates dynamic interactions in web applications, but it also enables developers to manage email functionalities effectively. This integration especially important for applications that require user notifications, confirmations, or any form of direct communication through email.
When it comes to email communication, JavaScript serves as a bridge between user interactions on the front-end and server-side processes that handle email sending. Using JavaScript, developers can capture user input, validate it, and then send it to a server-side script that interacts with an email service.
One common scenario involves sending emails after a user submits a form. Here’s a concise illustration of how you might capture this data using JavaScript:
const form = document.getElementById('contactForm'); form.addEventListener('submit', function(event) { event.preventDefault(); // Prevent the default form submission const formData = new FormData(form); // Capture form data sendEmail(formData); // Call the function to send email }); function sendEmail(data) { // Sending data to the server for email processing fetch('/send-email', { method: 'POST', body: data }) .then(response => response.json()) .then(data => { if (data.success) { alert('Email sent successfully!'); } else { alert('Email sending failed.'); } }) .catch(error => console.error('Error sending email:', error)); }
In this example, when the user submits the form, JavaScript captures the input and uses the Fetch API to send it to a server-side endpoint. This asynchronous operation allows for a smooth user experience, as users receive feedback without needing to reload the page.
Moreover, JavaScript can enhance the email process by allowing for real-time updates and notifications. For instance, after sending an email, you can update the user interface to reflect the current status of their request. This responsiveness especially important in contemporary web applications, where user experience is paramount.
However, while JavaScript can initiate email sending, it often requires external services or APIs to actually send the email. This is where services like SMTP servers or third-party email APIs come into play. The integration of these services with JavaScript is seamless and allows for rich features, such as email templates and tracking.
By understanding the role of JavaScript in email communication, developers can create more interactive and responsive applications that engage users effectively. The integration of client-side scripting with server-side email handling opens up a wealth of possibilities for building robust and feature-rich email functionalities.
Integrating Email APIs with JavaScript
To leverage the capabilities of JavaScript in sending emails, developers often turn to various email APIs. These APIs abstract away the complexities involved in SMTP configurations and provide a simplified interface for sending emails. Integrating these APIs into your JavaScript application allows you to easily send emails programmatically, manage templates, and handle responses without delving too deep into the underlying protocols.
One of the most popular services for email integration is SendGrid, which offers a powerful API that you can harness within your JavaScript applications. Setting up SendGrid involves obtaining an API key and integrating it into your JavaScript code. Here’s a step-by-step example of how to send an email using SendGrid’s API:
const sgMail = require('@sendgrid/mail'); // Import the SendGrid library sgMail.setApiKey('YOUR_SENDGRID_API_KEY'); // Set your API key function sendEmail(to, subject, text) { const msg = { to: to, // Recipient email from: '[email protected]', // Verified sender email subject: subject, // Subject of the email text: text, // Email body content }; sgMail.send(msg) .then(() => { console.log('Email sent successfully'); }) .catch((error) => { console.error('Error sending email:', error); }); } // Example usage sendEmail('[email protected]', 'Hello from SendGrid', 'This is a test email using SendGrid API.');
In this example, we first import the SendGrid library and set our API key. The sendEmail
function constructs the email message, specifying the recipient, sender, subject, and content. When the function is called, it sends the email and handles any errors that may occur. This approach abstracts the complexity of email handling, allowing developers to focus on building features instead of worrying about configuration.
Another widely used service is Mailgun, which offers similar capabilities. The process is much like SendGrid, but with its own unique interface and features. Integrating Mailgun into your JavaScript application can be done as follows:
const mailgun = require('mailgun-js'); // Import Mailgun library const mg = mailgun({ apiKey: 'YOUR_MAILGUN_API_KEY', domain: 'YOUR_DOMAIN_NAME' }); function sendMail(to, subject, text) { const data = { from: '[email protected]', // Verified sender email to: to, // Recipient email subject: subject, // Subject of the email text: text, // Email body content }; mg.messages().send(data, (error, body) => { if (error) { console.error('Error sending email:', error); } else { console.log('Email sent successfully:', body); } }); } // Example usage sendMail('[email protected]', 'Greetings from Mailgun', 'This is a test email using Mailgun API.');
Both SendGrid and Mailgun provide robust solutions for email delivery, but the choice between them may depend on specific project requirements, such as pricing, ease of integration, and available features.
Additionally, it is worth noting that many email services support advanced functionalities, including email tracking, analytics, and A/B testing of email campaigns. By using JavaScript to interact with these services, developers can create dynamic user experiences that respond to user actions and preferences.
The integration of email APIs with JavaScript not only simplifies the process of sending emails but also enhances the capabilities of web applications. By using these tools, developers can build powerful applications that ensure timely and effective communication with users.
Best Practices for Email Validation in JavaScript
When dealing with email validation in JavaScript, it’s essential to ensure that the data entered by users meets the specific format and criteria of valid email addresses. That is important to avoid unnecessary errors during the email sending process and to maintain data integrity. Using JavaScript for validation provides immediate feedback to users, enhancing the overall user experience.
A typical approach for validating email addresses involves using regular expressions (regex). Regular expressions are powerful tools for pattern matching and can be effectively utilized to verify the format of email addresses. Below is an example of a simple email validation function using regex:
const validateEmail = (email) => { const regex = /^[^s@]+@[^s@]+.[^s@]+$/; // Regex pattern for validating email return regex.test(email); // Returns true if email matches the pattern }; // Example usage const email = "[email protected]"; if (validateEmail(email)) { console.log('Valid email address'); } else { console.log('Invalid email address'); }
This function checks if the input string matches the regex pattern defined for email addresses. The regex looks for the presence of characters before and after the ‘@’ symbol, as well as a domain suffix, ensuring the email format is valid.
While regex provides a solid foundation for email validation, relying solely on it might not cover all edge cases. For instance, certain valid email formats may be missed. It’s advisable to combine regex validation with additional checks, such as verifying the domain’s existence or checking if the email is in a known disposable email provider list.
Moreover, it’s essential to handle user feedback effectively. Providing immediate feedback can help users correct their input before submission. A simple way to implement this would be to display error messages next to the input field when the validation fails. Here’s an example:
const emailInput = document.getElementById('email'); const errorMessage = document.getElementById('error-message'); emailInput.addEventListener('input', () => { if (!validateEmail(emailInput.value)) { errorMessage.textContent = 'Please enter a valid email address.'; errorMessage.style.color = 'red'; } else { errorMessage.textContent = ''; } });
This snippet listens for input events on an email field and uses the validation function to provide real-time feedback. If the email is invalid, an error message is displayed, guiding the user to correct their input before form submission.
Effective email validation in JavaScript utilizes a combination of regular expressions and user interface feedback mechanisms. By implementing these best practices, developers can enhance user experience and ensure that only valid email addresses are processed, ultimately improving the reliability of email communications in their applications.
Handling Async Operations in Email Sending Scripts
Handling asynchronous operations in email sending scripts is an important aspect of modern web development. JavaScript is inherently asynchronous, and using this feature is essential for creating responsive applications. When sending emails, especially in a user-facing context, it’s important to ensure that your application remains interactive while waiting for a response from the email service.
In typical scenarios where an email is sent after form submission, the asynchronous nature of JavaScript allows the application to process the request without blocking the user interface. That’s commonly implemented using Promises or async/await syntax, which simplifies the handling of asynchronous calls and improves code readability.
Here’s a refined example of how to send an email using async/await with the Fetch API:
const form = document.getElementById('contactForm'); form.addEventListener('submit', async function(event) { event.preventDefault(); // Prevent the default form submission const formData = new FormData(form); // Capture form data const result = await sendEmail(formData); // Await the email sending function handleResult(result); // Handle the success or failure response }); async function sendEmail(data) { try { const response = await fetch('/send-email', { method: 'POST', body: data }); if (!response.ok) { throw new Error('Network response was not ok'); } return await response.json(); // Return the JSON response } catch (error) { console.error('Error sending email:', error); return { success: false }; // Return a failure response } } function handleResult(data) { if (data.success) { alert('Email sent successfully!'); } else { alert('Email sending failed.'); } }
In this code, the `sendEmail` function is marked as `async`, allowing us to use `await` for the fetch call. This ensures that the function execution pauses until the email sending process completes, while still allowing the rest of the application to remain responsive. The error handling mechanism is simplified, catching any issues that arise during the fetch operation.
Another important aspect of handling asynchronous operations is providing feedback to users during the email sending process. This can be achieved by displaying loading indicators or disabling form inputs while waiting for the email response. This not only improves user experience but also prevents multiple submissions or interactions that could complicate the email sending process.
const submitButton = document.getElementById('submitButton'); form.addEventListener('submit', async function(event) { event.preventDefault(); submitButton.disabled = true; // Disable button to prevent multiple submissions const formData = new FormData(form); const result = await sendEmail(formData); handleResult(result); submitButton.disabled = false; // Re-enable button after processing });
This approach ensures that the user can only submit the form once until the email operation is complete, reducing the chance of errors and providing a smoother experience.
Effectively managing asynchronous operations in email sending scripts is essential for creating a seamless user experience. By using async/await syntax, implementing proper error handling, and providing user feedback, developers can build robust applications that handle email communication efficiently and elegantly.