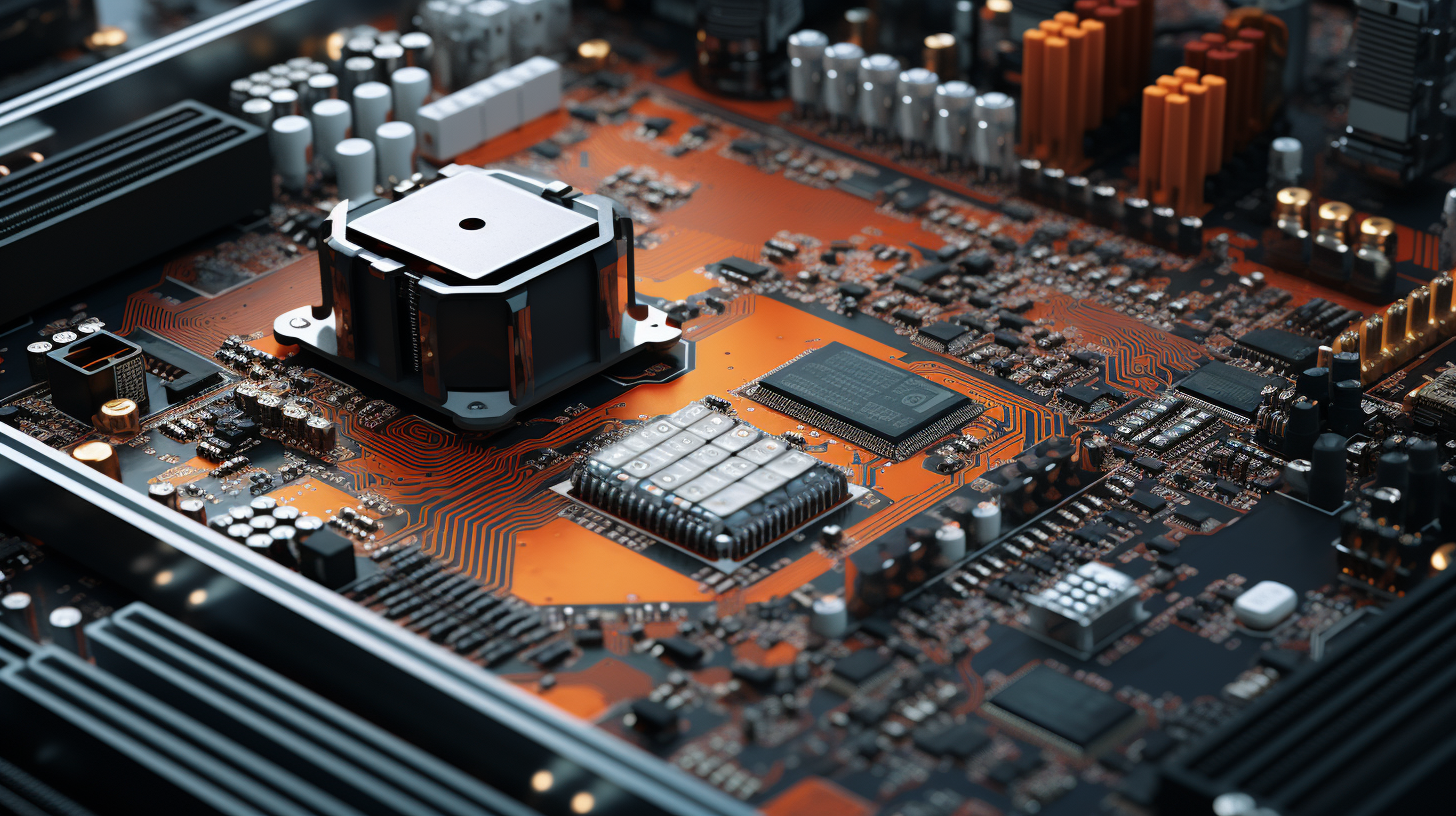
JavaScript and File Upload Handling
Within the scope of web development, file uploads are a fundamental feature that allows users to send files from their local machine to a server. JavaScript plays an important role in enhancing the user experience during this process. There are several mechanisms through which file uploads can be handled in JavaScript, each with its own set of advantages and trade-offs.
Traditionally, file uploads were managed through HTML forms that utilize the “ element. This method, while simpler, often led to a full page reload when submitting the form. With the advent of AJAX and modern APIs, developers can now perform file uploads asynchronously, providing a smoother user experience.
One of the primary methods for handling file uploads in JavaScript is through the XMLHttpRequest object. This allows developers to send files to the server without interrupting the flow of the web application. Here’s how you can use XMLHttpRequest
for a simple file upload:
const fileInput = document.getElementById('fileInput'); const uploadButton = document.getElementById('uploadButton'); uploadButton.addEventListener('click', () => { const file = fileInput.files[0]; const xhr = new XMLHttpRequest(); xhr.open('POST', '/upload', true); xhr.setRequestHeader('X-Requested-With', 'XMLHttpRequest'); xhr.onload = () => { if (xhr.status === 200) { console.log('File uploaded successfully!'); } else { console.error('File upload failed.'); } }; const formData = new FormData(); formData.append('file', file); xhr.send(formData); });
Another modern approach to handling file uploads is the Fetch API, which provides a more powerful and flexible feature set than XMLHttpRequest
. The Fetch API allows you to work with promises, making your code cleaner and easier to manage. Here’s an example of how to implement a file upload using the Fetch API:
const fileInput = document.getElementById('fileInput'); const uploadButton = document.getElementById('uploadButton'); uploadButton.addEventListener('click', () => { const file = fileInput.files[0]; const formData = new FormData(); formData.append('file', file); fetch('/upload', { method: 'POST', body: formData, }) .then(response => { if (response.ok) { return response.json(); } throw new Error('File upload failed.'); }) .then(data => { console.log('File uploaded successfully!', data); }) .catch(error => { console.error('Error:', error); }); });
Both the XMLHttpRequest and Fetch API methods are widely supported in modern browsers, making them reliable choices for file uploads. Additionally, they can be combined with other features such as progress tracking and error handling, enhancing the overall functionality and user experience.
As we delve deeper into file upload handling, understanding the FormData API will illuminate how to package and send files effectively.
Understanding FormData API
The FormData API is a powerful tool that allows you to easily construct a set of key/value pairs to send data, including files, using the XMLHttpRequest or Fetch API. It acts as an interface for easily building the data structure that will be sent to the server, allowing for a more seamless interaction when uploading files.
To utilize the FormData API effectively, you can start by creating an instance of FormData. This instance can be populated with various types of data, including files selected via an HTML element. Here’s how you can create a FormData object:
const formData = new FormData();
Once you have a FormData object, you can append data to it using the append
method. This method takes two parameters: the name of the field and its value. For file uploads, you’ll typically use the file input element to get the selected file and append it to the FormData object:
const fileInput = document.getElementById('fileInput'); const file = fileInput.files[0]; formData.append('file', file);
FormData can also handle multiple files if the file input allows for multiple selections. By specifying the same field name, you can append several files to the FormData object:
const files = fileInput.files; for (let i = 0; i < files.length; i++) { formData.append('files[]', files[i]); }
Besides files, you can add other data types to the FormData instance, such as strings or numbers, which can be useful for sending additional metadata along with the file upload:
formData.append('description', 'User uploaded file'); formData.append('userId', 12345);
When sending this FormData object using XMLHttpRequest or Fetch API, it automatically sets the Content-Type
to multipart/form-data
, which especially important for file uploads. This makes it easy to handle the transmission of files and associated data without needing to manually define this header.
The FormData API abstracts away many complexities associated with file uploads, allowing developers to focus on building features without getting bogged down in the minutiae of data formatting. By using this API in your file upload mechanism, you can create a more robust, uncomplicated to manage experience.
Implementing File Validation
Implementing file validation is a critical step in the file upload process, ensuring that only the appropriate files are accepted and processed by your application. This not only enhances security by preventing malicious files from being uploaded but also improves user experience by providing immediate feedback on file selection.
File validation can be performed on the client side using JavaScript before the file is sent to the server. This process typically involves checking the file type, size, and other relevant attributes. Using the File API, which provides access to file properties, allows for a simpler and efficient validation approach.
Here’s how you can implement basic file type validation:
const fileInput = document.getElementById('fileInput'); const uploadButton = document.getElementById('uploadButton'); uploadButton.addEventListener('click', () => { const file = fileInput.files[0]; // Check if a file is selected if (!file) { console.error('No file selected.'); return; } // Validate file type const validTypes = ['image/jpeg', 'image/png', 'application/pdf']; if (!validTypes.includes(file.type)) { console.error('Invalid file type. Please upload a JPEG, PNG, or PDF file.'); return; } // Proceed with file upload const formData = new FormData(); formData.append('file', file); // Use XMLHttpRequest or Fetch API for upload });
In this example, the file upload is triggered by the click event on the upload button. Before the upload process begins, the selected file is validated against an array of acceptable MIME types. If the type doesn’t match the criteria, an error message is logged, and the function exits early, preventing the upload.
In addition to file type validation, it’s also prudent to check the file size. Limiting the size of uploaded files helps manage server storage and can improve performance. Here’s how you can implement file size validation:
const MAX_FILE_SIZE = 5 * 1024 * 1024; // 5 MB uploadButton.addEventListener('click', () => { const file = fileInput.files[0]; // Check file size if (file.size > MAX_FILE_SIZE) { console.error('File size exceeds the maximum limit of 5 MB.'); return; } // Proceed with file upload });
With this additional validation step, the script checks if the selected file exceeds the defined maximum size of 5 MB. If it does, an error message is displayed, and the upload process is halted.
By combining these client-side validations, you not only create a more robust file handling mechanism but also enhance the user experience by providing instant feedback. However, it’s crucial to remember that client-side validation should never replace server-side validation. Always implement server-side checks to ensure that uploaded files are safe and conform to your application’s requirements.
As you continue to build out your file upload functionality, think integrating additional validation features such as checking for specific file names or ensuring that file extensions match the MIME types, further refining your approach to file validation.
Handling File Upload Progress
When dealing with file uploads, providing users with feedback about the upload progress is indispensable. This capability not only enhances user experience but also gives them a sense of control and reassurance while the file upload is in progress. JavaScript makes it simpler to implement file upload progress tracking using the XMLHttpRequest object or the Fetch API.
To track the progress of a file upload using XMLHttpRequest, you can make use of the onprogress event handler. This event is triggered periodically during the upload, so that you can access information about how much data has been sent and its total size. Below is an example demonstrating how to track and display the upload progress:
const fileInput = document.getElementById('fileInput'); const uploadButton = document.getElementById('uploadButton'); const progressBar = document.getElementById('progressBar'); uploadButton.addEventListener('click', () => { const file = fileInput.files[0]; const xhr = new XMLHttpRequest(); xhr.open('POST', '/upload', true); xhr.setRequestHeader('X-Requested-With', 'XMLHttpRequest'); // Update progress bar xhr.upload.onprogress = (event) => { if (event.lengthComputable) { const percentComplete = (event.loaded / event.total) * 100; progressBar.style.width = percentComplete + '%'; progressBar.innerText = Math.round(percentComplete) + '%'; } }; xhr.onload = () => { if (xhr.status === 200) { console.log('File uploaded successfully!'); } else { console.error('File upload failed.'); } }; const formData = new FormData(); formData.append('file', file); xhr.send(formData); });
In this code snippet, the xhr.upload.onprogress event listener is set up to calculate the upload progress. The event.lengthComputable property checks if the total size of the upload is known. If it is, you can compute the percentage of the file that has been uploaded and update the user interface accordingly. The progressBar element can be styled via CSS to visually indicate the upload progress.
For those who prefer using the Fetch API, tracking upload progress is a bit more involved since the Fetch API does not natively support progress events. However, you can wrap the Fetch call in a custom function that utilizes the XMLHttpRequest under the hood. Here’s how you can achieve that:
function uploadFile(file) { return new Promise((resolve, reject) => { const xhr = new XMLHttpRequest(); const formData = new FormData(); formData.append('file', file); xhr.open('POST', '/upload', true); xhr.upload.onprogress = (event) => { if (event.lengthComputable) { const percentComplete = (event.loaded / event.total) * 100; progressBar.style.width = percentComplete + '%'; progressBar.innerText = Math.round(percentComplete) + '%'; } }; xhr.onload = () => { if (xhr.status === 200) { resolve(xhr.response); } else { reject(new Error('File upload failed.')); } }; xhr.send(formData); }); } uploadButton.addEventListener('click', () => { const file = fileInput.files[0]; uploadFile(file) .then(response => { console.log('File uploaded successfully!', response); }) .catch(error => { console.error('Error:', error); }); });
This function encapsulates the XMLHttpRequest upload logic within a Promise, making it easy to handle the upload asynchronously. By using the onprogress event within the Promise, you can effectively provide feedback to the user during the upload process.
Incorporating upload progress feedback not only improves the user’s understanding of the upload status but also enhances the overall experience. Whether using XMLHttpRequest or wrapping it around Fetch API functionality, these techniques allow you to deliver a more responsive and engaging file upload interface.
Error Handling in File Uploads
Error handling in file uploads is a critical aspect of web development that ensures a robust user experience. Users may encounter various issues during the file upload process, such as network failures, server errors, or even client-side file issues. Handling these errors gracefully not only improves application resilience but also fosters user trust and satisfaction.
When an upload fails, it’s essential to provide clear and actionable feedback to the user. Using the onload and onerror event handlers in the XMLHttpRequest object, you can effectively capture and convey errors that occur during the upload process. Here’s an example of how to implement error handling with XMLHttpRequest:
const fileInput = document.getElementById('fileInput'); const uploadButton = document.getElementById('uploadButton'); uploadButton.addEventListener('click', () => { const file = fileInput.files[0]; const xhr = new XMLHttpRequest(); xhr.open('POST', '/upload', true); xhr.setRequestHeader('X-Requested-With', 'XMLHttpRequest'); xhr.onload = () => { if (xhr.status === 200) { console.log('File uploaded successfully!'); } else { console.error('Server responded with status: ' + xhr.status); alert('File upload failed: ' + xhr.statusText); } }; xhr.onerror = () => { console.error('An error occurred while uploading the file.'); alert('Network error: Please check your internet connection and try again.'); }; const formData = new FormData(); formData.append('file', file); xhr.send(formData); });
In this example, when the upload completes, the status is checked. If the status indicates a failure (anything other than 200), an error message is displayed, allowing the user to understand what went wrong. Additionally, by listening to the onerror event, you can capture network errors and alert the user about potential connectivity issues.
For those who prefer the Fetch API, error handling can be accomplished through promise chaining. While Fetch automatically rejects promises on network errors, you still need to handle HTTP errors manually. Here’s how to implement error handling using the Fetch API:
const fileInput = document.getElementById('fileInput'); const uploadButton = document.getElementById('uploadButton'); uploadButton.addEventListener('click', () => { const file = fileInput.files[0]; const formData = new FormData(); formData.append('file', file); fetch('/upload', { method: 'POST', body: formData, }) .then(response => { if (!response.ok) { throw new Error('Server responded with status: ' + response.status); } return response.json(); }) .then(data => { console.log('File uploaded successfully!', data); }) .catch(error => { console.error('Error:', error); alert('File upload failed: ' + error.message); }); });
In this Fetch API example, the response is checked for a successful status. If the response is not okay, an error is thrown, which is then caught in the catch block. This allows you to present meaningful error messages to the user, whether the issue stems from the server or a network problem.
Moreover, it’s beneficial to implement client-side validation errors, as mentioned previously, before attempting to upload the file. These checks can prevent unnecessary requests and provide immediate feedback to the user, reducing frustration associated with failed uploads due to invalid files.
Integrating comprehensive error handling into your file upload process is paramount. It not only safeguards against unexpected failures but also enhances the overall user experience by delivering clarity and support during what could be a frustrating part of their interaction with your application.
Best Practices for File Upload Management
When it comes to file upload management, adhering to best practices is essential for maintaining a seamless user experience and ensuring the security of your application. Here are several important guidelines to ponder when implementing file uploads in JavaScript.
1. Implement Client-Side Validation: Always validate file types and sizes on the client side before sending any requests to the server. This immediate feedback can prevent unnecessary network traffic and reduce server load. Ensure that the checks are robust; for example, validate the MIME types as well as the file extensions.
const validTypes = ['image/jpeg', 'image/png', 'application/pdf']; const MAX_FILE_SIZE = 5 * 1024 * 1024; // 5 MB function validateFile(file) { if (!validTypes.includes(file.type)) { throw new Error('Invalid file type. Please upload a JPEG, PNG, or PDF file.'); } if (file.size > MAX_FILE_SIZE) { throw new Error('File size exceeds the maximum limit of 5 MB.'); } }
2. Use Asynchronous Uploads: Take advantage of asynchronous file uploads using XMLHttpRequest or Fetch API. This enables your application to remain responsive, allowing users to interact with other parts of the application while uploads are in progress.
const uploadFile = (file) => { return fetch('/upload', { method: 'POST', body: new FormData().append('file', file), }); };
3. Provide User Feedback: Implement visual indicators such as progress bars or spinners to inform users about the upload status. This not only enhances user engagement but also alleviates anxiety associated with waiting for uploads to complete.
const progressBar = document.getElementById('progressBar'); xhr.upload.onprogress = (event) => { if (event.lengthComputable) { const percentComplete = (event.loaded / event.total) * 100; progressBar.style.width = percentComplete + '%'; progressBar.innerText = Math.round(percentComplete) + '%'; } };
4. Handle Errors Gracefully: Always plan for error handling in your file upload logic. Implement clear, actionable error messages to guide users on any issues they might encounter. Distinguish between client-side validation errors and server-side errors to provide more relevant feedback.
xhr.onerror = () => { alert('Network error: Please check your internet connection and try again.'); }; fetch('/upload', { method: 'POST', body: formData }) .then(response => { if (!response.ok) throw new Error('Upload failed with status: ' + response.status); }) .catch(error => { alert('File upload failed: ' + error.message); });
5. Secure Your Uploads: Security should be a top priority when dealing with file uploads. Always validate file types and sizes on the server side as well, as client-side checks can be bypassed. Use secure protocols (e.g., HTTPS) to protect data in transit. Additionally, think scanning uploaded files for malware before processing.
6. Limit Upload Size: Enforce limits on the size of files that can be uploaded. This ensures that your server does not become overloaded with large files, which can impact performance and resource allocation.
app.post('/upload', (req, res) => { if (req.file.size > MAX_FILE_SIZE) { return res.status(400).send('File size exceeds the maximum limit.'); } });
7. Optimize for Mobile: Given the prevalence of mobile device usage, ensure that your file upload interface is optimized for smaller screens. This includes using touch-friendly controls and providing clear instructions for users to easily select and upload files.
By adhering to these best practices, developers can create a file upload system this is efficient, uncomplicated to manage, and secure. A thorough approach to file uploads can significantly enhance user satisfaction while safeguarding application integrity.